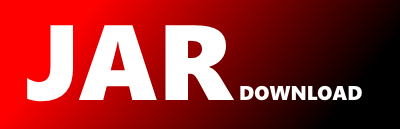
software.amazon.awssdk.services.codestarconnections.model.SyncConfiguration Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.codestarconnections.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information, such as repository, branch, provider, and resource names for a specific sync configuration.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SyncConfiguration implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField BRANCH_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Branch")
.getter(getter(SyncConfiguration::branch)).setter(setter(Builder::branch))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Branch").build()).build();
private static final SdkField CONFIG_FILE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ConfigFile").getter(getter(SyncConfiguration::configFile)).setter(setter(Builder::configFile))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConfigFile").build()).build();
private static final SdkField OWNER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OwnerId").getter(getter(SyncConfiguration::ownerId)).setter(setter(Builder::ownerId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OwnerId").build()).build();
private static final SdkField PROVIDER_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ProviderType").getter(getter(SyncConfiguration::providerTypeAsString))
.setter(setter(Builder::providerType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProviderType").build()).build();
private static final SdkField REPOSITORY_LINK_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RepositoryLinkId").getter(getter(SyncConfiguration::repositoryLinkId))
.setter(setter(Builder::repositoryLinkId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RepositoryLinkId").build()).build();
private static final SdkField REPOSITORY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RepositoryName").getter(getter(SyncConfiguration::repositoryName))
.setter(setter(Builder::repositoryName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RepositoryName").build()).build();
private static final SdkField RESOURCE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResourceName").getter(getter(SyncConfiguration::resourceName)).setter(setter(Builder::resourceName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceName").build()).build();
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RoleArn").getter(getter(SyncConfiguration::roleArn)).setter(setter(Builder::roleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleArn").build()).build();
private static final SdkField SYNC_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SyncType").getter(getter(SyncConfiguration::syncTypeAsString)).setter(setter(Builder::syncType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SyncType").build()).build();
private static final SdkField PUBLISH_DEPLOYMENT_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PublishDeploymentStatus").getter(getter(SyncConfiguration::publishDeploymentStatusAsString))
.setter(setter(Builder::publishDeploymentStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PublishDeploymentStatus").build())
.build();
private static final SdkField TRIGGER_RESOURCE_UPDATE_ON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TriggerResourceUpdateOn").getter(getter(SyncConfiguration::triggerResourceUpdateOnAsString))
.setter(setter(Builder::triggerResourceUpdateOn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TriggerResourceUpdateOn").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(BRANCH_FIELD,
CONFIG_FILE_FIELD, OWNER_ID_FIELD, PROVIDER_TYPE_FIELD, REPOSITORY_LINK_ID_FIELD, REPOSITORY_NAME_FIELD,
RESOURCE_NAME_FIELD, ROLE_ARN_FIELD, SYNC_TYPE_FIELD, PUBLISH_DEPLOYMENT_STATUS_FIELD,
TRIGGER_RESOURCE_UPDATE_ON_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String branch;
private final String configFile;
private final String ownerId;
private final String providerType;
private final String repositoryLinkId;
private final String repositoryName;
private final String resourceName;
private final String roleArn;
private final String syncType;
private final String publishDeploymentStatus;
private final String triggerResourceUpdateOn;
private SyncConfiguration(BuilderImpl builder) {
this.branch = builder.branch;
this.configFile = builder.configFile;
this.ownerId = builder.ownerId;
this.providerType = builder.providerType;
this.repositoryLinkId = builder.repositoryLinkId;
this.repositoryName = builder.repositoryName;
this.resourceName = builder.resourceName;
this.roleArn = builder.roleArn;
this.syncType = builder.syncType;
this.publishDeploymentStatus = builder.publishDeploymentStatus;
this.triggerResourceUpdateOn = builder.triggerResourceUpdateOn;
}
/**
*
* The branch associated with a specific sync configuration.
*
*
* @return The branch associated with a specific sync configuration.
*/
public final String branch() {
return branch;
}
/**
*
* The file path to the configuration file associated with a specific sync configuration. The path should point to
* an actual file in the sync configurations linked repository.
*
*
* @return The file path to the configuration file associated with a specific sync configuration. The path should
* point to an actual file in the sync configurations linked repository.
*/
public final String configFile() {
return configFile;
}
/**
*
* The owner ID for the repository associated with a specific sync configuration, such as the owner ID in GitHub.
*
*
* @return The owner ID for the repository associated with a specific sync configuration, such as the owner ID in
* GitHub.
*/
public final String ownerId() {
return ownerId;
}
/**
*
* The connection provider type associated with a specific sync configuration, such as GitHub.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #providerType} will
* return {@link ProviderType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #providerTypeAsString}.
*
*
* @return The connection provider type associated with a specific sync configuration, such as GitHub.
* @see ProviderType
*/
public final ProviderType providerType() {
return ProviderType.fromValue(providerType);
}
/**
*
* The connection provider type associated with a specific sync configuration, such as GitHub.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #providerType} will
* return {@link ProviderType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #providerTypeAsString}.
*
*
* @return The connection provider type associated with a specific sync configuration, such as GitHub.
* @see ProviderType
*/
public final String providerTypeAsString() {
return providerType;
}
/**
*
* The ID of the repository link associated with a specific sync configuration.
*
*
* @return The ID of the repository link associated with a specific sync configuration.
*/
public final String repositoryLinkId() {
return repositoryLinkId;
}
/**
*
* The name of the repository associated with a specific sync configuration.
*
*
* @return The name of the repository associated with a specific sync configuration.
*/
public final String repositoryName() {
return repositoryName;
}
/**
*
* The name of the connection resource associated with a specific sync configuration.
*
*
* @return The name of the connection resource associated with a specific sync configuration.
*/
public final String resourceName() {
return resourceName;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role associated with a specific sync configuration.
*
*
* @return The Amazon Resource Name (ARN) of the IAM role associated with a specific sync configuration.
*/
public final String roleArn() {
return roleArn;
}
/**
*
* The type of sync for a specific sync configuration.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #syncType} will
* return {@link SyncConfigurationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #syncTypeAsString}.
*
*
* @return The type of sync for a specific sync configuration.
* @see SyncConfigurationType
*/
public final SyncConfigurationType syncType() {
return SyncConfigurationType.fromValue(syncType);
}
/**
*
* The type of sync for a specific sync configuration.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #syncType} will
* return {@link SyncConfigurationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #syncTypeAsString}.
*
*
* @return The type of sync for a specific sync configuration.
* @see SyncConfigurationType
*/
public final String syncTypeAsString() {
return syncType;
}
/**
*
* Whether to enable or disable publishing of deployment status to source providers.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #publishDeploymentStatus} will return {@link PublishDeploymentStatus#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #publishDeploymentStatusAsString}.
*
*
* @return Whether to enable or disable publishing of deployment status to source providers.
* @see PublishDeploymentStatus
*/
public final PublishDeploymentStatus publishDeploymentStatus() {
return PublishDeploymentStatus.fromValue(publishDeploymentStatus);
}
/**
*
* Whether to enable or disable publishing of deployment status to source providers.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #publishDeploymentStatus} will return {@link PublishDeploymentStatus#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #publishDeploymentStatusAsString}.
*
*
* @return Whether to enable or disable publishing of deployment status to source providers.
* @see PublishDeploymentStatus
*/
public final String publishDeploymentStatusAsString() {
return publishDeploymentStatus;
}
/**
*
* When to trigger Git sync to begin the stack update.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #triggerResourceUpdateOn} will return {@link TriggerResourceUpdateOn#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #triggerResourceUpdateOnAsString}.
*
*
* @return When to trigger Git sync to begin the stack update.
* @see TriggerResourceUpdateOn
*/
public final TriggerResourceUpdateOn triggerResourceUpdateOn() {
return TriggerResourceUpdateOn.fromValue(triggerResourceUpdateOn);
}
/**
*
* When to trigger Git sync to begin the stack update.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #triggerResourceUpdateOn} will return {@link TriggerResourceUpdateOn#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #triggerResourceUpdateOnAsString}.
*
*
* @return When to trigger Git sync to begin the stack update.
* @see TriggerResourceUpdateOn
*/
public final String triggerResourceUpdateOnAsString() {
return triggerResourceUpdateOn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(branch());
hashCode = 31 * hashCode + Objects.hashCode(configFile());
hashCode = 31 * hashCode + Objects.hashCode(ownerId());
hashCode = 31 * hashCode + Objects.hashCode(providerTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(repositoryLinkId());
hashCode = 31 * hashCode + Objects.hashCode(repositoryName());
hashCode = 31 * hashCode + Objects.hashCode(resourceName());
hashCode = 31 * hashCode + Objects.hashCode(roleArn());
hashCode = 31 * hashCode + Objects.hashCode(syncTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(publishDeploymentStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(triggerResourceUpdateOnAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SyncConfiguration)) {
return false;
}
SyncConfiguration other = (SyncConfiguration) obj;
return Objects.equals(branch(), other.branch()) && Objects.equals(configFile(), other.configFile())
&& Objects.equals(ownerId(), other.ownerId())
&& Objects.equals(providerTypeAsString(), other.providerTypeAsString())
&& Objects.equals(repositoryLinkId(), other.repositoryLinkId())
&& Objects.equals(repositoryName(), other.repositoryName())
&& Objects.equals(resourceName(), other.resourceName()) && Objects.equals(roleArn(), other.roleArn())
&& Objects.equals(syncTypeAsString(), other.syncTypeAsString())
&& Objects.equals(publishDeploymentStatusAsString(), other.publishDeploymentStatusAsString())
&& Objects.equals(triggerResourceUpdateOnAsString(), other.triggerResourceUpdateOnAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SyncConfiguration").add("Branch", branch()).add("ConfigFile", configFile())
.add("OwnerId", ownerId()).add("ProviderType", providerTypeAsString())
.add("RepositoryLinkId", repositoryLinkId()).add("RepositoryName", repositoryName())
.add("ResourceName", resourceName()).add("RoleArn", roleArn()).add("SyncType", syncTypeAsString())
.add("PublishDeploymentStatus", publishDeploymentStatusAsString())
.add("TriggerResourceUpdateOn", triggerResourceUpdateOnAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Branch":
return Optional.ofNullable(clazz.cast(branch()));
case "ConfigFile":
return Optional.ofNullable(clazz.cast(configFile()));
case "OwnerId":
return Optional.ofNullable(clazz.cast(ownerId()));
case "ProviderType":
return Optional.ofNullable(clazz.cast(providerTypeAsString()));
case "RepositoryLinkId":
return Optional.ofNullable(clazz.cast(repositoryLinkId()));
case "RepositoryName":
return Optional.ofNullable(clazz.cast(repositoryName()));
case "ResourceName":
return Optional.ofNullable(clazz.cast(resourceName()));
case "RoleArn":
return Optional.ofNullable(clazz.cast(roleArn()));
case "SyncType":
return Optional.ofNullable(clazz.cast(syncTypeAsString()));
case "PublishDeploymentStatus":
return Optional.ofNullable(clazz.cast(publishDeploymentStatusAsString()));
case "TriggerResourceUpdateOn":
return Optional.ofNullable(clazz.cast(triggerResourceUpdateOnAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("Branch", BRANCH_FIELD);
map.put("ConfigFile", CONFIG_FILE_FIELD);
map.put("OwnerId", OWNER_ID_FIELD);
map.put("ProviderType", PROVIDER_TYPE_FIELD);
map.put("RepositoryLinkId", REPOSITORY_LINK_ID_FIELD);
map.put("RepositoryName", REPOSITORY_NAME_FIELD);
map.put("ResourceName", RESOURCE_NAME_FIELD);
map.put("RoleArn", ROLE_ARN_FIELD);
map.put("SyncType", SYNC_TYPE_FIELD);
map.put("PublishDeploymentStatus", PUBLISH_DEPLOYMENT_STATUS_FIELD);
map.put("TriggerResourceUpdateOn", TRIGGER_RESOURCE_UPDATE_ON_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function