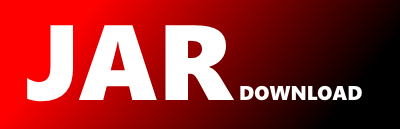
software.amazon.awssdk.services.cognitoidentity.DefaultCognitoIdentityClient Maven / Gradle / Ivy
Show all versions of cognitoidentity Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cognitoidentity;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.cognitoidentity.model.CognitoIdentityException;
import software.amazon.awssdk.services.cognitoidentity.model.CognitoIdentityRequest;
import software.amazon.awssdk.services.cognitoidentity.model.ConcurrentModificationException;
import software.amazon.awssdk.services.cognitoidentity.model.CreateIdentityPoolRequest;
import software.amazon.awssdk.services.cognitoidentity.model.CreateIdentityPoolResponse;
import software.amazon.awssdk.services.cognitoidentity.model.DeleteIdentitiesRequest;
import software.amazon.awssdk.services.cognitoidentity.model.DeleteIdentitiesResponse;
import software.amazon.awssdk.services.cognitoidentity.model.DeleteIdentityPoolRequest;
import software.amazon.awssdk.services.cognitoidentity.model.DeleteIdentityPoolResponse;
import software.amazon.awssdk.services.cognitoidentity.model.DescribeIdentityPoolRequest;
import software.amazon.awssdk.services.cognitoidentity.model.DescribeIdentityPoolResponse;
import software.amazon.awssdk.services.cognitoidentity.model.DescribeIdentityRequest;
import software.amazon.awssdk.services.cognitoidentity.model.DescribeIdentityResponse;
import software.amazon.awssdk.services.cognitoidentity.model.DeveloperUserAlreadyRegisteredException;
import software.amazon.awssdk.services.cognitoidentity.model.ExternalServiceException;
import software.amazon.awssdk.services.cognitoidentity.model.GetCredentialsForIdentityRequest;
import software.amazon.awssdk.services.cognitoidentity.model.GetCredentialsForIdentityResponse;
import software.amazon.awssdk.services.cognitoidentity.model.GetIdRequest;
import software.amazon.awssdk.services.cognitoidentity.model.GetIdResponse;
import software.amazon.awssdk.services.cognitoidentity.model.GetIdentityPoolRolesRequest;
import software.amazon.awssdk.services.cognitoidentity.model.GetIdentityPoolRolesResponse;
import software.amazon.awssdk.services.cognitoidentity.model.GetOpenIdTokenForDeveloperIdentityRequest;
import software.amazon.awssdk.services.cognitoidentity.model.GetOpenIdTokenForDeveloperIdentityResponse;
import software.amazon.awssdk.services.cognitoidentity.model.GetOpenIdTokenRequest;
import software.amazon.awssdk.services.cognitoidentity.model.GetOpenIdTokenResponse;
import software.amazon.awssdk.services.cognitoidentity.model.GetPrincipalTagAttributeMapRequest;
import software.amazon.awssdk.services.cognitoidentity.model.GetPrincipalTagAttributeMapResponse;
import software.amazon.awssdk.services.cognitoidentity.model.InternalErrorException;
import software.amazon.awssdk.services.cognitoidentity.model.InvalidIdentityPoolConfigurationException;
import software.amazon.awssdk.services.cognitoidentity.model.InvalidParameterException;
import software.amazon.awssdk.services.cognitoidentity.model.LimitExceededException;
import software.amazon.awssdk.services.cognitoidentity.model.ListIdentitiesRequest;
import software.amazon.awssdk.services.cognitoidentity.model.ListIdentitiesResponse;
import software.amazon.awssdk.services.cognitoidentity.model.ListIdentityPoolsRequest;
import software.amazon.awssdk.services.cognitoidentity.model.ListIdentityPoolsResponse;
import software.amazon.awssdk.services.cognitoidentity.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.cognitoidentity.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.cognitoidentity.model.LookupDeveloperIdentityRequest;
import software.amazon.awssdk.services.cognitoidentity.model.LookupDeveloperIdentityResponse;
import software.amazon.awssdk.services.cognitoidentity.model.MergeDeveloperIdentitiesRequest;
import software.amazon.awssdk.services.cognitoidentity.model.MergeDeveloperIdentitiesResponse;
import software.amazon.awssdk.services.cognitoidentity.model.NotAuthorizedException;
import software.amazon.awssdk.services.cognitoidentity.model.ResourceConflictException;
import software.amazon.awssdk.services.cognitoidentity.model.ResourceNotFoundException;
import software.amazon.awssdk.services.cognitoidentity.model.SetIdentityPoolRolesRequest;
import software.amazon.awssdk.services.cognitoidentity.model.SetIdentityPoolRolesResponse;
import software.amazon.awssdk.services.cognitoidentity.model.SetPrincipalTagAttributeMapRequest;
import software.amazon.awssdk.services.cognitoidentity.model.SetPrincipalTagAttributeMapResponse;
import software.amazon.awssdk.services.cognitoidentity.model.TagResourceRequest;
import software.amazon.awssdk.services.cognitoidentity.model.TagResourceResponse;
import software.amazon.awssdk.services.cognitoidentity.model.TooManyRequestsException;
import software.amazon.awssdk.services.cognitoidentity.model.UnlinkDeveloperIdentityRequest;
import software.amazon.awssdk.services.cognitoidentity.model.UnlinkDeveloperIdentityResponse;
import software.amazon.awssdk.services.cognitoidentity.model.UnlinkIdentityRequest;
import software.amazon.awssdk.services.cognitoidentity.model.UnlinkIdentityResponse;
import software.amazon.awssdk.services.cognitoidentity.model.UntagResourceRequest;
import software.amazon.awssdk.services.cognitoidentity.model.UntagResourceResponse;
import software.amazon.awssdk.services.cognitoidentity.model.UpdateIdentityPoolRequest;
import software.amazon.awssdk.services.cognitoidentity.model.UpdateIdentityPoolResponse;
import software.amazon.awssdk.services.cognitoidentity.paginators.ListIdentityPoolsIterable;
import software.amazon.awssdk.services.cognitoidentity.transform.CreateIdentityPoolRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.DeleteIdentitiesRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.DeleteIdentityPoolRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.DescribeIdentityPoolRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.DescribeIdentityRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.GetCredentialsForIdentityRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.GetIdRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.GetIdentityPoolRolesRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.GetOpenIdTokenForDeveloperIdentityRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.GetOpenIdTokenRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.GetPrincipalTagAttributeMapRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.ListIdentitiesRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.ListIdentityPoolsRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.LookupDeveloperIdentityRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.MergeDeveloperIdentitiesRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.SetIdentityPoolRolesRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.SetPrincipalTagAttributeMapRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.UnlinkDeveloperIdentityRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.UnlinkIdentityRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.cognitoidentity.transform.UpdateIdentityPoolRequestMarshaller;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link CognitoIdentityClient}.
*
* @see CognitoIdentityClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultCognitoIdentityClient implements CognitoIdentityClient {
private static final Logger log = Logger.loggerFor(DefaultCognitoIdentityClient.class);
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultCognitoIdentityClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Creates a new identity pool. The identity pool is a store of user identity information that is specific to your
* AWS account. The keys for SupportedLoginProviders
are as follows:
*
*
* -
*
* Facebook: graph.facebook.com
*
*
* -
*
* Google: accounts.google.com
*
*
* -
*
* Amazon: www.amazon.com
*
*
* -
*
* Twitter: api.twitter.com
*
*
* -
*
* Digits: www.digits.com
*
*
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param createIdentityPoolRequest
* Input to the CreateIdentityPool action.
* @return Result of the CreateIdentityPool operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws LimitExceededException
* Thrown when the total number of user pools has exceeded a preset limit.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.CreateIdentityPool
* @see AWS API Documentation
*/
@Override
public CreateIdentityPoolResponse createIdentityPool(CreateIdentityPoolRequest createIdentityPoolRequest)
throws InvalidParameterException, NotAuthorizedException, ResourceConflictException, TooManyRequestsException,
InternalErrorException, LimitExceededException, AwsServiceException, SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateIdentityPoolResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createIdentityPoolRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateIdentityPool");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateIdentityPool").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createIdentityPoolRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateIdentityPoolRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes identities from an identity pool. You can specify a list of 1-60 identities that you want to delete.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param deleteIdentitiesRequest
* Input to the DeleteIdentities
action.
* @return Result of the DeleteIdentities operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.DeleteIdentities
* @see AWS API Documentation
*/
@Override
public DeleteIdentitiesResponse deleteIdentities(DeleteIdentitiesRequest deleteIdentitiesRequest)
throws InvalidParameterException, TooManyRequestsException, InternalErrorException, AwsServiceException,
SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteIdentitiesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteIdentitiesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteIdentities");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteIdentities").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteIdentitiesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteIdentitiesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes an identity pool. Once a pool is deleted, users will not be able to authenticate with the pool.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param deleteIdentityPoolRequest
* Input to the DeleteIdentityPool action.
* @return Result of the DeleteIdentityPool operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.DeleteIdentityPool
* @see AWS API Documentation
*/
@Override
public DeleteIdentityPoolResponse deleteIdentityPool(DeleteIdentityPoolRequest deleteIdentityPoolRequest)
throws InvalidParameterException, ResourceNotFoundException, NotAuthorizedException, TooManyRequestsException,
InternalErrorException, AwsServiceException, SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteIdentityPoolResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteIdentityPoolRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteIdentityPool");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteIdentityPool").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteIdentityPoolRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteIdentityPoolRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns metadata related to the given identity, including when the identity was created and any associated linked
* logins.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param describeIdentityRequest
* Input to the DescribeIdentity
action.
* @return Result of the DescribeIdentity operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.DescribeIdentity
* @see AWS API Documentation
*/
@Override
public DescribeIdentityResponse describeIdentity(DescribeIdentityRequest describeIdentityRequest)
throws InvalidParameterException, ResourceNotFoundException, NotAuthorizedException, TooManyRequestsException,
InternalErrorException, AwsServiceException, SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeIdentityResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeIdentityRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeIdentity");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeIdentity").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeIdentityRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeIdentityRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets details about a particular identity pool, including the pool name, ID description, creation date, and
* current number of users.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param describeIdentityPoolRequest
* Input to the DescribeIdentityPool action.
* @return Result of the DescribeIdentityPool operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.DescribeIdentityPool
* @see AWS API Documentation
*/
@Override
public DescribeIdentityPoolResponse describeIdentityPool(DescribeIdentityPoolRequest describeIdentityPoolRequest)
throws InvalidParameterException, ResourceNotFoundException, NotAuthorizedException, TooManyRequestsException,
InternalErrorException, AwsServiceException, SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeIdentityPoolResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeIdentityPoolRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeIdentityPool");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeIdentityPool").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeIdentityPoolRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeIdentityPoolRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns credentials for the provided identity ID. Any provided logins will be validated against supported login
* providers. If the token is for cognito-identity.amazonaws.com, it will be passed through to AWS Security Token
* Service with the appropriate role for the token.
*
*
* This is a public API. You do not need any credentials to call this API.
*
*
* @param getCredentialsForIdentityRequest
* Input to the GetCredentialsForIdentity
action.
* @return Result of the GetCredentialsForIdentity operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InvalidIdentityPoolConfigurationException
* Thrown if the identity pool has no role associated for the given auth type (auth/unauth) or if the
* AssumeRole fails.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws ExternalServiceException
* An exception thrown when a dependent service such as Facebook or Twitter is not responding
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.GetCredentialsForIdentity
* @see AWS API Documentation
*/
@Override
public GetCredentialsForIdentityResponse getCredentialsForIdentity(
GetCredentialsForIdentityRequest getCredentialsForIdentityRequest) throws InvalidParameterException,
ResourceNotFoundException, NotAuthorizedException, ResourceConflictException, TooManyRequestsException,
InvalidIdentityPoolConfigurationException, InternalErrorException, ExternalServiceException, AwsServiceException,
SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetCredentialsForIdentityResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getCredentialsForIdentityRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetCredentialsForIdentity");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetCredentialsForIdentity").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getCredentialsForIdentityRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetCredentialsForIdentityRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Generates (or retrieves) a Cognito ID. Supplying multiple logins will create an implicit linked account.
*
*
* This is a public API. You do not need any credentials to call this API.
*
*
* @param getIdRequest
* Input to the GetId action.
* @return Result of the GetId operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws LimitExceededException
* Thrown when the total number of user pools has exceeded a preset limit.
* @throws ExternalServiceException
* An exception thrown when a dependent service such as Facebook or Twitter is not responding
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.GetId
* @see AWS API
* Documentation
*/
@Override
public GetIdResponse getId(GetIdRequest getIdRequest) throws InvalidParameterException, ResourceNotFoundException,
NotAuthorizedException, ResourceConflictException, TooManyRequestsException, InternalErrorException,
LimitExceededException, ExternalServiceException, AwsServiceException, SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetIdResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getIdRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetId");
return clientHandler.execute(new ClientExecutionParams().withOperationName("GetId")
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler).withInput(getIdRequest)
.withMetricCollector(apiCallMetricCollector).withMarshaller(new GetIdRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets the roles for an identity pool.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param getIdentityPoolRolesRequest
* Input to the GetIdentityPoolRoles
action.
* @return Result of the GetIdentityPoolRoles operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.GetIdentityPoolRoles
* @see AWS API Documentation
*/
@Override
public GetIdentityPoolRolesResponse getIdentityPoolRoles(GetIdentityPoolRolesRequest getIdentityPoolRolesRequest)
throws InvalidParameterException, ResourceNotFoundException, NotAuthorizedException, ResourceConflictException,
TooManyRequestsException, InternalErrorException, AwsServiceException, SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetIdentityPoolRolesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getIdentityPoolRolesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetIdentityPoolRoles");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetIdentityPoolRoles").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getIdentityPoolRolesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetIdentityPoolRolesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets an OpenID token, using a known Cognito ID. This known Cognito ID is returned by GetId. You can
* optionally add additional logins for the identity. Supplying multiple logins creates an implicit link.
*
*
* The OpenID token is valid for 10 minutes.
*
*
* This is a public API. You do not need any credentials to call this API.
*
*
* @param getOpenIdTokenRequest
* Input to the GetOpenIdToken action.
* @return Result of the GetOpenIdToken operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws ExternalServiceException
* An exception thrown when a dependent service such as Facebook or Twitter is not responding
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.GetOpenIdToken
* @see AWS API Documentation
*/
@Override
public GetOpenIdTokenResponse getOpenIdToken(GetOpenIdTokenRequest getOpenIdTokenRequest) throws InvalidParameterException,
ResourceNotFoundException, NotAuthorizedException, ResourceConflictException, TooManyRequestsException,
InternalErrorException, ExternalServiceException, AwsServiceException, SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetOpenIdTokenResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getOpenIdTokenRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetOpenIdToken");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetOpenIdToken").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getOpenIdTokenRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetOpenIdTokenRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Registers (or retrieves) a Cognito IdentityId
and an OpenID Connect token for a user authenticated
* by your backend authentication process. Supplying multiple logins will create an implicit linked account. You can
* only specify one developer provider as part of the Logins
map, which is linked to the identity pool.
* The developer provider is the "domain" by which Cognito will refer to your users.
*
*
* You can use GetOpenIdTokenForDeveloperIdentity
to create a new identity and to link new logins (that
* is, user credentials issued by a public provider or developer provider) to an existing identity. When you want to
* create a new identity, the IdentityId
should be null. When you want to associate a new login with an
* existing authenticated/unauthenticated identity, you can do so by providing the existing IdentityId
.
* This API will create the identity in the specified IdentityPoolId
.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param getOpenIdTokenForDeveloperIdentityRequest
* Input to the GetOpenIdTokenForDeveloperIdentity
action.
* @return Result of the GetOpenIdTokenForDeveloperIdentity operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws DeveloperUserAlreadyRegisteredException
* The provided developer user identifier is already registered with Cognito under a different identity ID.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.GetOpenIdTokenForDeveloperIdentity
* @see AWS API Documentation
*/
@Override
public GetOpenIdTokenForDeveloperIdentityResponse getOpenIdTokenForDeveloperIdentity(
GetOpenIdTokenForDeveloperIdentityRequest getOpenIdTokenForDeveloperIdentityRequest)
throws InvalidParameterException, ResourceNotFoundException, NotAuthorizedException, ResourceConflictException,
TooManyRequestsException, InternalErrorException, DeveloperUserAlreadyRegisteredException, AwsServiceException,
SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetOpenIdTokenForDeveloperIdentityResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getOpenIdTokenForDeveloperIdentityRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetOpenIdTokenForDeveloperIdentity");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetOpenIdTokenForDeveloperIdentity").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getOpenIdTokenForDeveloperIdentityRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetOpenIdTokenForDeveloperIdentityRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Use GetPrincipalTagAttributeMap
to list all mappings between PrincipalTags
and user
* attributes.
*
*
* @param getPrincipalTagAttributeMapRequest
* @return Result of the GetPrincipalTagAttributeMap operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.GetPrincipalTagAttributeMap
* @see AWS API Documentation
*/
@Override
public GetPrincipalTagAttributeMapResponse getPrincipalTagAttributeMap(
GetPrincipalTagAttributeMapRequest getPrincipalTagAttributeMapRequest) throws InvalidParameterException,
ResourceNotFoundException, NotAuthorizedException, TooManyRequestsException, InternalErrorException,
AwsServiceException, SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetPrincipalTagAttributeMapResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getPrincipalTagAttributeMapRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetPrincipalTagAttributeMap");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetPrincipalTagAttributeMap").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getPrincipalTagAttributeMapRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetPrincipalTagAttributeMapRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists the identities in an identity pool.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param listIdentitiesRequest
* Input to the ListIdentities action.
* @return Result of the ListIdentities operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.ListIdentities
* @see AWS API Documentation
*/
@Override
public ListIdentitiesResponse listIdentities(ListIdentitiesRequest listIdentitiesRequest) throws InvalidParameterException,
ResourceNotFoundException, NotAuthorizedException, TooManyRequestsException, InternalErrorException,
AwsServiceException, SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListIdentitiesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listIdentitiesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListIdentities");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListIdentities").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listIdentitiesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListIdentitiesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all of the Cognito identity pools registered for your account.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param listIdentityPoolsRequest
* Input to the ListIdentityPools action.
* @return Result of the ListIdentityPools operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.ListIdentityPools
* @see AWS API Documentation
*/
@Override
public ListIdentityPoolsResponse listIdentityPools(ListIdentityPoolsRequest listIdentityPoolsRequest)
throws InvalidParameterException, NotAuthorizedException, TooManyRequestsException, ResourceNotFoundException,
InternalErrorException, AwsServiceException, SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListIdentityPoolsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listIdentityPoolsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListIdentityPools");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListIdentityPools").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listIdentityPoolsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListIdentityPoolsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all of the Cognito identity pools registered for your account.
*
*
* You must use AWS Developer credentials to call this API.
*
*
*
* This is a variant of
* {@link #listIdentityPools(software.amazon.awssdk.services.cognitoidentity.model.ListIdentityPoolsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.cognitoidentity.paginators.ListIdentityPoolsIterable responses = client.listIdentityPoolsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.cognitoidentity.paginators.ListIdentityPoolsIterable responses = client
* .listIdentityPoolsPaginator(request);
* for (software.amazon.awssdk.services.cognitoidentity.model.ListIdentityPoolsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.cognitoidentity.paginators.ListIdentityPoolsIterable responses = client.listIdentityPoolsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listIdentityPools(software.amazon.awssdk.services.cognitoidentity.model.ListIdentityPoolsRequest)}
* operation.
*
*
* @param listIdentityPoolsRequest
* Input to the ListIdentityPools action.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.ListIdentityPools
* @see AWS API Documentation
*/
@Override
public ListIdentityPoolsIterable listIdentityPoolsPaginator(ListIdentityPoolsRequest listIdentityPoolsRequest)
throws InvalidParameterException, NotAuthorizedException, TooManyRequestsException, ResourceNotFoundException,
InternalErrorException, AwsServiceException, SdkClientException, CognitoIdentityException {
return new ListIdentityPoolsIterable(this, applyPaginatorUserAgent(listIdentityPoolsRequest));
}
/**
*
* Lists the tags that are assigned to an Amazon Cognito identity pool.
*
*
* A tag is a label that you can apply to identity pools to categorize and manage them in different ways, such as by
* purpose, owner, environment, or other criteria.
*
*
* You can use this action up to 10 times per second, per account.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws InvalidParameterException, ResourceNotFoundException, NotAuthorizedException, TooManyRequestsException,
InternalErrorException, AwsServiceException, SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listTagsForResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the IdentityID
associated with a DeveloperUserIdentifier
or the list of
* DeveloperUserIdentifier
values associated with an IdentityId
for an existing identity.
* Either IdentityID
or DeveloperUserIdentifier
must not be null. If you supply only one
* of these values, the other value will be searched in the database and returned as a part of the response. If you
* supply both, DeveloperUserIdentifier
will be matched against IdentityID
. If the values
* are verified against the database, the response returns both values and is the same as the request. Otherwise a
* ResourceConflictException
is thrown.
*
*
* LookupDeveloperIdentity
is intended for low-throughput control plane operations: for example, to
* enable customer service to locate an identity ID by username. If you are using it for higher-volume operations
* such as user authentication, your requests are likely to be throttled. GetOpenIdTokenForDeveloperIdentity
* is a better option for higher-volume operations for user authentication.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param lookupDeveloperIdentityRequest
* Input to the LookupDeveloperIdentityInput
action.
* @return Result of the LookupDeveloperIdentity operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.LookupDeveloperIdentity
* @see AWS API Documentation
*/
@Override
public LookupDeveloperIdentityResponse lookupDeveloperIdentity(LookupDeveloperIdentityRequest lookupDeveloperIdentityRequest)
throws InvalidParameterException, ResourceNotFoundException, NotAuthorizedException, ResourceConflictException,
TooManyRequestsException, InternalErrorException, AwsServiceException, SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, LookupDeveloperIdentityResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, lookupDeveloperIdentityRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "LookupDeveloperIdentity");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("LookupDeveloperIdentity").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(lookupDeveloperIdentityRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new LookupDeveloperIdentityRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Merges two users having different IdentityId
s, existing in the same identity pool, and identified by
* the same developer provider. You can use this action to request that discrete users be merged and identified as a
* single user in the Cognito environment. Cognito associates the given source user (
* SourceUserIdentifier
) with the IdentityId
of the DestinationUserIdentifier
* . Only developer-authenticated users can be merged. If the users to be merged are associated with the same public
* provider, but as two different users, an exception will be thrown.
*
*
* The number of linked logins is limited to 20. So, the number of linked logins for the source user,
* SourceUserIdentifier
, and the destination user, DestinationUserIdentifier
, together
* should not be larger than 20. Otherwise, an exception will be thrown.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param mergeDeveloperIdentitiesRequest
* Input to the MergeDeveloperIdentities
action.
* @return Result of the MergeDeveloperIdentities operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.MergeDeveloperIdentities
* @see AWS API Documentation
*/
@Override
public MergeDeveloperIdentitiesResponse mergeDeveloperIdentities(
MergeDeveloperIdentitiesRequest mergeDeveloperIdentitiesRequest) throws InvalidParameterException,
ResourceNotFoundException, NotAuthorizedException, ResourceConflictException, TooManyRequestsException,
InternalErrorException, AwsServiceException, SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, MergeDeveloperIdentitiesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, mergeDeveloperIdentitiesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "MergeDeveloperIdentities");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("MergeDeveloperIdentities").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(mergeDeveloperIdentitiesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new MergeDeveloperIdentitiesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Sets the roles for an identity pool. These roles are used when making calls to GetCredentialsForIdentity
* action.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param setIdentityPoolRolesRequest
* Input to the SetIdentityPoolRoles
action.
* @return Result of the SetIdentityPoolRoles operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws ConcurrentModificationException
* Thrown if there are parallel requests to modify a resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.SetIdentityPoolRoles
* @see AWS API Documentation
*/
@Override
public SetIdentityPoolRolesResponse setIdentityPoolRoles(SetIdentityPoolRolesRequest setIdentityPoolRolesRequest)
throws InvalidParameterException, ResourceNotFoundException, NotAuthorizedException, ResourceConflictException,
TooManyRequestsException, InternalErrorException, ConcurrentModificationException, AwsServiceException,
SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, SetIdentityPoolRolesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, setIdentityPoolRolesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SetIdentityPoolRoles");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("SetIdentityPoolRoles").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(setIdentityPoolRolesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new SetIdentityPoolRolesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* You can use this operation to use default (username and clientID) attribute or custom attribute mappings.
*
*
* @param setPrincipalTagAttributeMapRequest
* @return Result of the SetPrincipalTagAttributeMap operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.SetPrincipalTagAttributeMap
* @see AWS API Documentation
*/
@Override
public SetPrincipalTagAttributeMapResponse setPrincipalTagAttributeMap(
SetPrincipalTagAttributeMapRequest setPrincipalTagAttributeMapRequest) throws InvalidParameterException,
ResourceNotFoundException, NotAuthorizedException, TooManyRequestsException, InternalErrorException,
AwsServiceException, SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, SetPrincipalTagAttributeMapResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, setPrincipalTagAttributeMapRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SetPrincipalTagAttributeMap");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SetPrincipalTagAttributeMap").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(setPrincipalTagAttributeMapRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new SetPrincipalTagAttributeMapRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Assigns a set of tags to the specified Amazon Cognito identity pool. A tag is a label that you can use to
* categorize and manage identity pools in different ways, such as by purpose, owner, environment, or other
* criteria.
*
*
* Each tag consists of a key and value, both of which you define. A key is a general category for more specific
* values. For example, if you have two versions of an identity pool, one for testing and another for production,
* you might assign an Environment
tag key to both identity pools. The value of this key might be
* Test
for one identity pool and Production
for the other.
*
*
* Tags are useful for cost tracking and access control. You can activate your tags so that they appear on the
* Billing and Cost Management console, where you can track the costs associated with your identity pools. In an IAM
* policy, you can constrain permissions for identity pools based on specific tags or tag values.
*
*
* You can use this action up to 5 times per second, per account. An identity pool can have as many as 50 tags.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.TagResource
* @see AWS
* API Documentation
*/
@Override
public TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws InvalidParameterException,
ResourceNotFoundException, NotAuthorizedException, TooManyRequestsException, InternalErrorException,
AwsServiceException, SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("TagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(tagResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new TagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Unlinks a DeveloperUserIdentifier
from an existing identity. Unlinked developer users will be
* considered new identities next time they are seen. If, for a given Cognito identity, you remove all federated
* identities as well as the developer user identifier, the Cognito identity becomes inaccessible.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param unlinkDeveloperIdentityRequest
* Input to the UnlinkDeveloperIdentity
action.
* @return Result of the UnlinkDeveloperIdentity operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.UnlinkDeveloperIdentity
* @see AWS API Documentation
*/
@Override
public UnlinkDeveloperIdentityResponse unlinkDeveloperIdentity(UnlinkDeveloperIdentityRequest unlinkDeveloperIdentityRequest)
throws InvalidParameterException, ResourceNotFoundException, NotAuthorizedException, ResourceConflictException,
TooManyRequestsException, InternalErrorException, AwsServiceException, SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UnlinkDeveloperIdentityResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, unlinkDeveloperIdentityRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UnlinkDeveloperIdentity");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UnlinkDeveloperIdentity").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(unlinkDeveloperIdentityRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UnlinkDeveloperIdentityRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Unlinks a federated identity from an existing account. Unlinked logins will be considered new identities next
* time they are seen. Removing the last linked login will make this identity inaccessible.
*
*
* This is a public API. You do not need any credentials to call this API.
*
*
* @param unlinkIdentityRequest
* Input to the UnlinkIdentity action.
* @return Result of the UnlinkIdentity operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws ExternalServiceException
* An exception thrown when a dependent service such as Facebook or Twitter is not responding
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.UnlinkIdentity
* @see AWS API Documentation
*/
@Override
public UnlinkIdentityResponse unlinkIdentity(UnlinkIdentityRequest unlinkIdentityRequest) throws InvalidParameterException,
ResourceNotFoundException, NotAuthorizedException, ResourceConflictException, TooManyRequestsException,
InternalErrorException, ExternalServiceException, AwsServiceException, SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UnlinkIdentityResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, unlinkIdentityRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UnlinkIdentity");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UnlinkIdentity").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(unlinkIdentityRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UnlinkIdentityRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes the specified tags from the specified Amazon Cognito identity pool. You can use this action up to 5 times
* per second, per account
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.UntagResource
* @see AWS API Documentation
*/
@Override
public UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws InvalidParameterException,
ResourceNotFoundException, NotAuthorizedException, TooManyRequestsException, InternalErrorException,
AwsServiceException, SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UntagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(untagResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates an identity pool.
*
*
* You must use AWS Developer credentials to call this API.
*
*
* @param updateIdentityPoolRequest
* An object representing an Amazon Cognito identity pool.
* @return Result of the UpdateIdentityPool operation returned by the service.
* @throws InvalidParameterException
* Thrown for missing or bad input parameter(s).
* @throws ResourceNotFoundException
* Thrown when the requested resource (for example, a dataset or record) does not exist.
* @throws NotAuthorizedException
* Thrown when a user is not authorized to access the requested resource.
* @throws ResourceConflictException
* Thrown when a user tries to use a login which is already linked to another account.
* @throws TooManyRequestsException
* Thrown when a request is throttled.
* @throws InternalErrorException
* Thrown when the service encounters an error during processing the request.
* @throws ConcurrentModificationException
* Thrown if there are parallel requests to modify a resource.
* @throws LimitExceededException
* Thrown when the total number of user pools has exceeded a preset limit.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CognitoIdentityException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CognitoIdentityClient.UpdateIdentityPool
* @see AWS API Documentation
*/
@Override
public UpdateIdentityPoolResponse updateIdentityPool(UpdateIdentityPoolRequest updateIdentityPoolRequest)
throws InvalidParameterException, ResourceNotFoundException, NotAuthorizedException, ResourceConflictException,
TooManyRequestsException, InternalErrorException, ConcurrentModificationException, LimitExceededException,
AwsServiceException, SdkClientException, CognitoIdentityException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateIdentityPoolResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateIdentityPoolRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cognito Identity");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateIdentityPool");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateIdentityPool").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateIdentityPoolRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateIdentityPoolRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(CognitoIdentityException::builder)
.protocol(AwsJsonProtocol.AWS_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("ConcurrentModificationException")
.exceptionBuilderSupplier(ConcurrentModificationException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidParameterException")
.exceptionBuilderSupplier(InvalidParameterException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DeveloperUserAlreadyRegisteredException")
.exceptionBuilderSupplier(DeveloperUserAlreadyRegisteredException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceConflictException")
.exceptionBuilderSupplier(ResourceConflictException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ExternalServiceException")
.exceptionBuilderSupplier(ExternalServiceException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("NotAuthorizedException")
.exceptionBuilderSupplier(NotAuthorizedException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidIdentityPoolConfigurationException")
.exceptionBuilderSupplier(InvalidIdentityPoolConfigurationException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalErrorException")
.exceptionBuilderSupplier(InternalErrorException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyRequestsException")
.exceptionBuilderSupplier(TooManyRequestsException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LimitExceededException")
.exceptionBuilderSupplier(LimitExceededException::builder).build());
}
@Override
public void close() {
clientHandler.close();
}
private T applyPaginatorUserAgent(T request) {
Consumer userAgentApplier = b -> b.addApiName(ApiName.builder()
.version(VersionInfo.SDK_VERSION).name("PAGINATED").build());
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(userAgentApplier).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(userAgentApplier).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
}