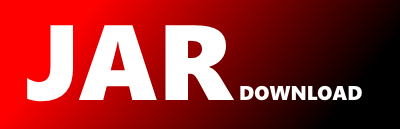
software.amazon.awssdk.services.cognitoidentityprovider.model.SetUserPoolMfaConfigResponse Maven / Gradle / Ivy
Show all versions of cognitoidentityprovider Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cognitoidentityprovider.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class SetUserPoolMfaConfigResponse extends CognitoIdentityProviderResponse implements
ToCopyableBuilder {
private static final SdkField SMS_MFA_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("SmsMfaConfiguration")
.getter(getter(SetUserPoolMfaConfigResponse::smsMfaConfiguration)).setter(setter(Builder::smsMfaConfiguration))
.constructor(SmsMfaConfigType::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SmsMfaConfiguration").build())
.build();
private static final SdkField SOFTWARE_TOKEN_MFA_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("SoftwareTokenMfaConfiguration")
.getter(getter(SetUserPoolMfaConfigResponse::softwareTokenMfaConfiguration))
.setter(setter(Builder::softwareTokenMfaConfiguration))
.constructor(SoftwareTokenMfaConfigType::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SoftwareTokenMfaConfiguration")
.build()).build();
private static final SdkField MFA_CONFIGURATION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MfaConfiguration").getter(getter(SetUserPoolMfaConfigResponse::mfaConfigurationAsString))
.setter(setter(Builder::mfaConfiguration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MfaConfiguration").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SMS_MFA_CONFIGURATION_FIELD,
SOFTWARE_TOKEN_MFA_CONFIGURATION_FIELD, MFA_CONFIGURATION_FIELD));
private final SmsMfaConfigType smsMfaConfiguration;
private final SoftwareTokenMfaConfigType softwareTokenMfaConfiguration;
private final String mfaConfiguration;
private SetUserPoolMfaConfigResponse(BuilderImpl builder) {
super(builder);
this.smsMfaConfiguration = builder.smsMfaConfiguration;
this.softwareTokenMfaConfiguration = builder.softwareTokenMfaConfiguration;
this.mfaConfiguration = builder.mfaConfiguration;
}
/**
*
* The SMS text message MFA configuration.
*
*
* @return The SMS text message MFA configuration.
*/
public final SmsMfaConfigType smsMfaConfiguration() {
return smsMfaConfiguration;
}
/**
*
* The software token MFA configuration.
*
*
* @return The software token MFA configuration.
*/
public final SoftwareTokenMfaConfigType softwareTokenMfaConfiguration() {
return softwareTokenMfaConfiguration;
}
/**
*
* The MFA configuration. Valid values include:
*
*
* -
*
* OFF
MFA won't be used for any users.
*
*
* -
*
* ON
MFA is required for all users to sign in.
*
*
* -
*
* OPTIONAL
MFA will be required only for individual users who have an MFA factor enabled.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mfaConfiguration}
* will return {@link UserPoolMfaType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #mfaConfigurationAsString}.
*
*
* @return The MFA configuration. Valid values include:
*
* -
*
* OFF
MFA won't be used for any users.
*
*
* -
*
* ON
MFA is required for all users to sign in.
*
*
* -
*
* OPTIONAL
MFA will be required only for individual users who have an MFA factor enabled.
*
*
* @see UserPoolMfaType
*/
public final UserPoolMfaType mfaConfiguration() {
return UserPoolMfaType.fromValue(mfaConfiguration);
}
/**
*
* The MFA configuration. Valid values include:
*
*
* -
*
* OFF
MFA won't be used for any users.
*
*
* -
*
* ON
MFA is required for all users to sign in.
*
*
* -
*
* OPTIONAL
MFA will be required only for individual users who have an MFA factor enabled.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mfaConfiguration}
* will return {@link UserPoolMfaType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #mfaConfigurationAsString}.
*
*
* @return The MFA configuration. Valid values include:
*
* -
*
* OFF
MFA won't be used for any users.
*
*
* -
*
* ON
MFA is required for all users to sign in.
*
*
* -
*
* OPTIONAL
MFA will be required only for individual users who have an MFA factor enabled.
*
*
* @see UserPoolMfaType
*/
public final String mfaConfigurationAsString() {
return mfaConfiguration;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(smsMfaConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(softwareTokenMfaConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(mfaConfigurationAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SetUserPoolMfaConfigResponse)) {
return false;
}
SetUserPoolMfaConfigResponse other = (SetUserPoolMfaConfigResponse) obj;
return Objects.equals(smsMfaConfiguration(), other.smsMfaConfiguration())
&& Objects.equals(softwareTokenMfaConfiguration(), other.softwareTokenMfaConfiguration())
&& Objects.equals(mfaConfigurationAsString(), other.mfaConfigurationAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SetUserPoolMfaConfigResponse").add("SmsMfaConfiguration", smsMfaConfiguration())
.add("SoftwareTokenMfaConfiguration", softwareTokenMfaConfiguration())
.add("MfaConfiguration", mfaConfigurationAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "SmsMfaConfiguration":
return Optional.ofNullable(clazz.cast(smsMfaConfiguration()));
case "SoftwareTokenMfaConfiguration":
return Optional.ofNullable(clazz.cast(softwareTokenMfaConfiguration()));
case "MfaConfiguration":
return Optional.ofNullable(clazz.cast(mfaConfigurationAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function