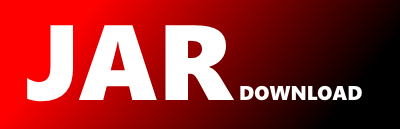
software.amazon.awssdk.services.cognitosync.model.GetIdentityPoolConfigurationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cognitosync Show documentation
Show all versions of cognitosync Show documentation
The AWS Java SDK for Amazon Cognito Sync module holds the client classes that are used for
communicating with Amazon Cognito Sync Service
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cognitosync.model;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The output for the GetIdentityPoolConfiguration operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetIdentityPoolConfigurationResponse extends CognitoSyncResponse implements
ToCopyableBuilder {
private final String identityPoolId;
private final PushSync pushSync;
private final CognitoStreams cognitoStreams;
private GetIdentityPoolConfigurationResponse(BuilderImpl builder) {
super(builder);
this.identityPoolId = builder.identityPoolId;
this.pushSync = builder.pushSync;
this.cognitoStreams = builder.cognitoStreams;
}
/**
*
* A name-spaced GUID (for example, us-east-1:23EC4050-6AEA-7089-A2DD-08002EXAMPLE) created by Amazon Cognito.
*
*
* @return A name-spaced GUID (for example, us-east-1:23EC4050-6AEA-7089-A2DD-08002EXAMPLE) created by Amazon
* Cognito.
*/
public String identityPoolId() {
return identityPoolId;
}
/**
*
* Options to apply to this identity pool for push synchronization.
*
*
* @return Options to apply to this identity pool for push synchronization.
*/
public PushSync pushSync() {
return pushSync;
}
/**
* Options to apply to this identity pool for Amazon Cognito streams.
*
* @return Options to apply to this identity pool for Amazon Cognito streams.
*/
public CognitoStreams cognitoStreams() {
return cognitoStreams;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(identityPoolId());
hashCode = 31 * hashCode + Objects.hashCode(pushSync());
hashCode = 31 * hashCode + Objects.hashCode(cognitoStreams());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetIdentityPoolConfigurationResponse)) {
return false;
}
GetIdentityPoolConfigurationResponse other = (GetIdentityPoolConfigurationResponse) obj;
return Objects.equals(identityPoolId(), other.identityPoolId()) && Objects.equals(pushSync(), other.pushSync())
&& Objects.equals(cognitoStreams(), other.cognitoStreams());
}
@Override
public String toString() {
return ToString.builder("GetIdentityPoolConfigurationResponse").add("IdentityPoolId", identityPoolId())
.add("PushSync", pushSync()).add("CognitoStreams", cognitoStreams()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "IdentityPoolId":
return Optional.ofNullable(clazz.cast(identityPoolId()));
case "PushSync":
return Optional.ofNullable(clazz.cast(pushSync()));
case "CognitoStreams":
return Optional.ofNullable(clazz.cast(cognitoStreams()));
default:
return Optional.empty();
}
}
public interface Builder extends CognitoSyncResponse.Builder, CopyableBuilder {
/**
*
* A name-spaced GUID (for example, us-east-1:23EC4050-6AEA-7089-A2DD-08002EXAMPLE) created by Amazon Cognito.
*
*
* @param identityPoolId
* A name-spaced GUID (for example, us-east-1:23EC4050-6AEA-7089-A2DD-08002EXAMPLE) created by Amazon
* Cognito.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder identityPoolId(String identityPoolId);
/**
*
* Options to apply to this identity pool for push synchronization.
*
*
* @param pushSync
* Options to apply to this identity pool for push synchronization.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder pushSync(PushSync pushSync);
/**
*
* Options to apply to this identity pool for push synchronization.
*
* This is a convenience that creates an instance of the {@link PushSync.Builder} avoiding the need to create
* one manually via {@link PushSync#builder()}.
*
* When the {@link Consumer} completes, {@link PushSync.Builder#build()} is called immediately and its result is
* passed to {@link #pushSync(PushSync)}.
*
* @param pushSync
* a consumer that will call methods on {@link PushSync.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #pushSync(PushSync)
*/
default Builder pushSync(Consumer pushSync) {
return pushSync(PushSync.builder().applyMutation(pushSync).build());
}
/**
* Options to apply to this identity pool for Amazon Cognito streams.
*
* @param cognitoStreams
* Options to apply to this identity pool for Amazon Cognito streams.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cognitoStreams(CognitoStreams cognitoStreams);
/**
* Options to apply to this identity pool for Amazon Cognito streams. This is a convenience that creates an
* instance of the {@link CognitoStreams.Builder} avoiding the need to create one manually via
* {@link CognitoStreams#builder()}.
*
* When the {@link Consumer} completes, {@link CognitoStreams.Builder#build()} is called immediately and its
* result is passed to {@link #cognitoStreams(CognitoStreams)}.
*
* @param cognitoStreams
* a consumer that will call methods on {@link CognitoStreams.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #cognitoStreams(CognitoStreams)
*/
default Builder cognitoStreams(Consumer cognitoStreams) {
return cognitoStreams(CognitoStreams.builder().applyMutation(cognitoStreams).build());
}
}
static final class BuilderImpl extends CognitoSyncResponse.BuilderImpl implements Builder {
private String identityPoolId;
private PushSync pushSync;
private CognitoStreams cognitoStreams;
private BuilderImpl() {
}
private BuilderImpl(GetIdentityPoolConfigurationResponse model) {
super(model);
identityPoolId(model.identityPoolId);
pushSync(model.pushSync);
cognitoStreams(model.cognitoStreams);
}
public final String getIdentityPoolId() {
return identityPoolId;
}
@Override
public final Builder identityPoolId(String identityPoolId) {
this.identityPoolId = identityPoolId;
return this;
}
public final void setIdentityPoolId(String identityPoolId) {
this.identityPoolId = identityPoolId;
}
public final PushSync.Builder getPushSync() {
return pushSync != null ? pushSync.toBuilder() : null;
}
@Override
public final Builder pushSync(PushSync pushSync) {
this.pushSync = pushSync;
return this;
}
public final void setPushSync(PushSync.BuilderImpl pushSync) {
this.pushSync = pushSync != null ? pushSync.build() : null;
}
public final CognitoStreams.Builder getCognitoStreams() {
return cognitoStreams != null ? cognitoStreams.toBuilder() : null;
}
@Override
public final Builder cognitoStreams(CognitoStreams cognitoStreams) {
this.cognitoStreams = cognitoStreams;
return this;
}
public final void setCognitoStreams(CognitoStreams.BuilderImpl cognitoStreams) {
this.cognitoStreams = cognitoStreams != null ? cognitoStreams.build() : null;
}
@Override
public GetIdentityPoolConfigurationResponse build() {
return new GetIdentityPoolConfigurationResponse(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy