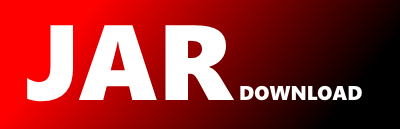
software.amazon.awssdk.services.cognitosync.model.SubscribeToDatasetRequest Maven / Gradle / Ivy
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cognitosync.model;
import java.util.Optional;
import javax.annotation.Generated;
import software.amazon.awssdk.core.AmazonWebServiceRequest;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A request to SubscribeToDatasetRequest.
*
*/
@Generated("software.amazon.awssdk:codegen")
public class SubscribeToDatasetRequest extends AmazonWebServiceRequest implements
ToCopyableBuilder {
private final String identityPoolId;
private final String identityId;
private final String datasetName;
private final String deviceId;
private SubscribeToDatasetRequest(BuilderImpl builder) {
this.identityPoolId = builder.identityPoolId;
this.identityId = builder.identityId;
this.datasetName = builder.datasetName;
this.deviceId = builder.deviceId;
}
/**
*
* A name-spaced GUID (for example, us-east-1:23EC4050-6AEA-7089-A2DD-08002EXAMPLE) created by Amazon Cognito. The
* ID of the pool to which the identity belongs.
*
*
* @return A name-spaced GUID (for example, us-east-1:23EC4050-6AEA-7089-A2DD-08002EXAMPLE) created by Amazon
* Cognito. The ID of the pool to which the identity belongs.
*/
public String identityPoolId() {
return identityPoolId;
}
/**
*
* Unique ID for this identity.
*
*
* @return Unique ID for this identity.
*/
public String identityId() {
return identityId;
}
/**
*
* The name of the dataset to subcribe to.
*
*
* @return The name of the dataset to subcribe to.
*/
public String datasetName() {
return datasetName;
}
/**
*
* The unique ID generated for this device by Cognito.
*
*
* @return The unique ID generated for this device by Cognito.
*/
public String deviceId() {
return deviceId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + ((identityPoolId() == null) ? 0 : identityPoolId().hashCode());
hashCode = 31 * hashCode + ((identityId() == null) ? 0 : identityId().hashCode());
hashCode = 31 * hashCode + ((datasetName() == null) ? 0 : datasetName().hashCode());
hashCode = 31 * hashCode + ((deviceId() == null) ? 0 : deviceId().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SubscribeToDatasetRequest)) {
return false;
}
SubscribeToDatasetRequest other = (SubscribeToDatasetRequest) obj;
if (other.identityPoolId() == null ^ this.identityPoolId() == null) {
return false;
}
if (other.identityPoolId() != null && !other.identityPoolId().equals(this.identityPoolId())) {
return false;
}
if (other.identityId() == null ^ this.identityId() == null) {
return false;
}
if (other.identityId() != null && !other.identityId().equals(this.identityId())) {
return false;
}
if (other.datasetName() == null ^ this.datasetName() == null) {
return false;
}
if (other.datasetName() != null && !other.datasetName().equals(this.datasetName())) {
return false;
}
if (other.deviceId() == null ^ this.deviceId() == null) {
return false;
}
if (other.deviceId() != null && !other.deviceId().equals(this.deviceId())) {
return false;
}
return true;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder("{");
if (identityPoolId() != null) {
sb.append("IdentityPoolId: ").append(identityPoolId()).append(",");
}
if (identityId() != null) {
sb.append("IdentityId: ").append(identityId()).append(",");
}
if (datasetName() != null) {
sb.append("DatasetName: ").append(datasetName()).append(",");
}
if (deviceId() != null) {
sb.append("DeviceId: ").append(deviceId()).append(",");
}
if (sb.length() > 1) {
sb.setLength(sb.length() - 1);
}
sb.append("}");
return sb.toString();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "IdentityPoolId":
return Optional.of(clazz.cast(identityPoolId()));
case "IdentityId":
return Optional.of(clazz.cast(identityId()));
case "DatasetName":
return Optional.of(clazz.cast(datasetName()));
case "DeviceId":
return Optional.of(clazz.cast(deviceId()));
default:
return Optional.empty();
}
}
public interface Builder extends CopyableBuilder {
/**
*
* A name-spaced GUID (for example, us-east-1:23EC4050-6AEA-7089-A2DD-08002EXAMPLE) created by Amazon Cognito.
* The ID of the pool to which the identity belongs.
*
*
* @param identityPoolId
* A name-spaced GUID (for example, us-east-1:23EC4050-6AEA-7089-A2DD-08002EXAMPLE) created by Amazon
* Cognito. The ID of the pool to which the identity belongs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder identityPoolId(String identityPoolId);
/**
*
* Unique ID for this identity.
*
*
* @param identityId
* Unique ID for this identity.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder identityId(String identityId);
/**
*
* The name of the dataset to subcribe to.
*
*
* @param datasetName
* The name of the dataset to subcribe to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder datasetName(String datasetName);
/**
*
* The unique ID generated for this device by Cognito.
*
*
* @param deviceId
* The unique ID generated for this device by Cognito.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder deviceId(String deviceId);
}
static final class BuilderImpl implements Builder {
private String identityPoolId;
private String identityId;
private String datasetName;
private String deviceId;
private BuilderImpl() {
}
private BuilderImpl(SubscribeToDatasetRequest model) {
identityPoolId(model.identityPoolId);
identityId(model.identityId);
datasetName(model.datasetName);
deviceId(model.deviceId);
}
public final String getIdentityPoolId() {
return identityPoolId;
}
@Override
public final Builder identityPoolId(String identityPoolId) {
this.identityPoolId = identityPoolId;
return this;
}
public final void setIdentityPoolId(String identityPoolId) {
this.identityPoolId = identityPoolId;
}
public final String getIdentityId() {
return identityId;
}
@Override
public final Builder identityId(String identityId) {
this.identityId = identityId;
return this;
}
public final void setIdentityId(String identityId) {
this.identityId = identityId;
}
public final String getDatasetName() {
return datasetName;
}
@Override
public final Builder datasetName(String datasetName) {
this.datasetName = datasetName;
return this;
}
public final void setDatasetName(String datasetName) {
this.datasetName = datasetName;
}
public final String getDeviceId() {
return deviceId;
}
@Override
public final Builder deviceId(String deviceId) {
this.deviceId = deviceId;
return this;
}
public final void setDeviceId(String deviceId) {
this.deviceId = deviceId;
}
@Override
public SubscribeToDatasetRequest build() {
return new SubscribeToDatasetRequest(this);
}
}
}