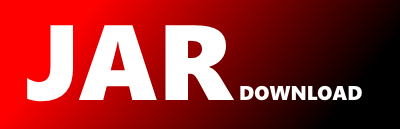
software.amazon.awssdk.services.cognitosync.model.Dataset Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cognitosync Show documentation
Show all versions of cognitosync Show documentation
The AWS Java SDK for Amazon Cognito Sync module holds the client classes that are used for
communicating with Amazon Cognito Sync Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cognitosync.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* A collection of data for an identity pool. An identity pool can have multiple datasets. A dataset is per identity and
* can be general or associated with a particular entity in an application (like a saved game). Datasets are
* automatically created if they don't exist. Data is synced by dataset, and a dataset can hold up to 1MB of key-value
* pairs.
*/
@Generated("software.amazon.awssdk:codegen")
public final class Dataset implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField IDENTITY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IdentityId").getter(getter(Dataset::identityId)).setter(setter(Builder::identityId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IdentityId").build()).build();
private static final SdkField DATASET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DatasetName").getter(getter(Dataset::datasetName)).setter(setter(Builder::datasetName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DatasetName").build()).build();
private static final SdkField CREATION_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreationDate").getter(getter(Dataset::creationDate)).setter(setter(Builder::creationDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationDate").build()).build();
private static final SdkField LAST_MODIFIED_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastModifiedDate").getter(getter(Dataset::lastModifiedDate)).setter(setter(Builder::lastModifiedDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastModifiedDate").build()).build();
private static final SdkField LAST_MODIFIED_BY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LastModifiedBy").getter(getter(Dataset::lastModifiedBy)).setter(setter(Builder::lastModifiedBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastModifiedBy").build()).build();
private static final SdkField DATA_STORAGE_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("DataStorage").getter(getter(Dataset::dataStorage)).setter(setter(Builder::dataStorage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataStorage").build()).build();
private static final SdkField NUM_RECORDS_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("NumRecords").getter(getter(Dataset::numRecords)).setter(setter(Builder::numRecords))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumRecords").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(IDENTITY_ID_FIELD,
DATASET_NAME_FIELD, CREATION_DATE_FIELD, LAST_MODIFIED_DATE_FIELD, LAST_MODIFIED_BY_FIELD, DATA_STORAGE_FIELD,
NUM_RECORDS_FIELD));
private static final long serialVersionUID = 1L;
private final String identityId;
private final String datasetName;
private final Instant creationDate;
private final Instant lastModifiedDate;
private final String lastModifiedBy;
private final Long dataStorage;
private final Long numRecords;
private Dataset(BuilderImpl builder) {
this.identityId = builder.identityId;
this.datasetName = builder.datasetName;
this.creationDate = builder.creationDate;
this.lastModifiedDate = builder.lastModifiedDate;
this.lastModifiedBy = builder.lastModifiedBy;
this.dataStorage = builder.dataStorage;
this.numRecords = builder.numRecords;
}
/**
* A name-spaced GUID (for example, us-east-1:23EC4050-6AEA-7089-A2DD-08002EXAMPLE) created by Amazon Cognito. GUID
* generation is unique within a region.
*
* @return A name-spaced GUID (for example, us-east-1:23EC4050-6AEA-7089-A2DD-08002EXAMPLE) created by Amazon
* Cognito. GUID generation is unique within a region.
*/
public final String identityId() {
return identityId;
}
/**
* A string of up to 128 characters. Allowed characters are a-z, A-Z, 0-9, '_' (underscore), '-' (dash), and '.'
* (dot).
*
* @return A string of up to 128 characters. Allowed characters are a-z, A-Z, 0-9, '_' (underscore), '-' (dash), and
* '.' (dot).
*/
public final String datasetName() {
return datasetName;
}
/**
* Date on which the dataset was created.
*
* @return Date on which the dataset was created.
*/
public final Instant creationDate() {
return creationDate;
}
/**
* Date when the dataset was last modified.
*
* @return Date when the dataset was last modified.
*/
public final Instant lastModifiedDate() {
return lastModifiedDate;
}
/**
* The device that made the last change to this dataset.
*
* @return The device that made the last change to this dataset.
*/
public final String lastModifiedBy() {
return lastModifiedBy;
}
/**
* Total size in bytes of the records in this dataset.
*
* @return Total size in bytes of the records in this dataset.
*/
public final Long dataStorage() {
return dataStorage;
}
/**
* Number of records in this dataset.
*
* @return Number of records in this dataset.
*/
public final Long numRecords() {
return numRecords;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(identityId());
hashCode = 31 * hashCode + Objects.hashCode(datasetName());
hashCode = 31 * hashCode + Objects.hashCode(creationDate());
hashCode = 31 * hashCode + Objects.hashCode(lastModifiedDate());
hashCode = 31 * hashCode + Objects.hashCode(lastModifiedBy());
hashCode = 31 * hashCode + Objects.hashCode(dataStorage());
hashCode = 31 * hashCode + Objects.hashCode(numRecords());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Dataset)) {
return false;
}
Dataset other = (Dataset) obj;
return Objects.equals(identityId(), other.identityId()) && Objects.equals(datasetName(), other.datasetName())
&& Objects.equals(creationDate(), other.creationDate())
&& Objects.equals(lastModifiedDate(), other.lastModifiedDate())
&& Objects.equals(lastModifiedBy(), other.lastModifiedBy()) && Objects.equals(dataStorage(), other.dataStorage())
&& Objects.equals(numRecords(), other.numRecords());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Dataset").add("IdentityId", identityId()).add("DatasetName", datasetName())
.add("CreationDate", creationDate()).add("LastModifiedDate", lastModifiedDate())
.add("LastModifiedBy", lastModifiedBy()).add("DataStorage", dataStorage()).add("NumRecords", numRecords())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "IdentityId":
return Optional.ofNullable(clazz.cast(identityId()));
case "DatasetName":
return Optional.ofNullable(clazz.cast(datasetName()));
case "CreationDate":
return Optional.ofNullable(clazz.cast(creationDate()));
case "LastModifiedDate":
return Optional.ofNullable(clazz.cast(lastModifiedDate()));
case "LastModifiedBy":
return Optional.ofNullable(clazz.cast(lastModifiedBy()));
case "DataStorage":
return Optional.ofNullable(clazz.cast(dataStorage()));
case "NumRecords":
return Optional.ofNullable(clazz.cast(numRecords()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy