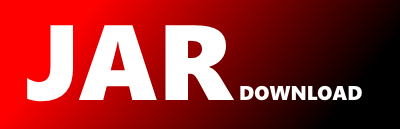
software.amazon.awssdk.services.cognitosync.model.GetBulkPublishDetailsResponse Maven / Gradle / Ivy
Show all versions of cognitosync Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.cognitosync.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* The output for the GetBulkPublishDetails operation.
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetBulkPublishDetailsResponse extends CognitoSyncResponse implements
ToCopyableBuilder {
private static final SdkField IDENTITY_POOL_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IdentityPoolId").getter(getter(GetBulkPublishDetailsResponse::identityPoolId))
.setter(setter(Builder::identityPoolId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IdentityPoolId").build()).build();
private static final SdkField BULK_PUBLISH_START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("BulkPublishStartTime").getter(getter(GetBulkPublishDetailsResponse::bulkPublishStartTime))
.setter(setter(Builder::bulkPublishStartTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BulkPublishStartTime").build())
.build();
private static final SdkField BULK_PUBLISH_COMPLETE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("BulkPublishCompleteTime").getter(getter(GetBulkPublishDetailsResponse::bulkPublishCompleteTime))
.setter(setter(Builder::bulkPublishCompleteTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BulkPublishCompleteTime").build())
.build();
private static final SdkField BULK_PUBLISH_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BulkPublishStatus").getter(getter(GetBulkPublishDetailsResponse::bulkPublishStatusAsString))
.setter(setter(Builder::bulkPublishStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BulkPublishStatus").build()).build();
private static final SdkField FAILURE_MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FailureMessage").getter(getter(GetBulkPublishDetailsResponse::failureMessage))
.setter(setter(Builder::failureMessage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureMessage").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(IDENTITY_POOL_ID_FIELD,
BULK_PUBLISH_START_TIME_FIELD, BULK_PUBLISH_COMPLETE_TIME_FIELD, BULK_PUBLISH_STATUS_FIELD, FAILURE_MESSAGE_FIELD));
private final String identityPoolId;
private final Instant bulkPublishStartTime;
private final Instant bulkPublishCompleteTime;
private final String bulkPublishStatus;
private final String failureMessage;
private GetBulkPublishDetailsResponse(BuilderImpl builder) {
super(builder);
this.identityPoolId = builder.identityPoolId;
this.bulkPublishStartTime = builder.bulkPublishStartTime;
this.bulkPublishCompleteTime = builder.bulkPublishCompleteTime;
this.bulkPublishStatus = builder.bulkPublishStatus;
this.failureMessage = builder.failureMessage;
}
/**
* A name-spaced GUID (for example, us-east-1:23EC4050-6AEA-7089-A2DD-08002EXAMPLE) created by Amazon Cognito. GUID
* generation is unique within a region.
*
* @return A name-spaced GUID (for example, us-east-1:23EC4050-6AEA-7089-A2DD-08002EXAMPLE) created by Amazon
* Cognito. GUID generation is unique within a region.
*/
public final String identityPoolId() {
return identityPoolId;
}
/**
* The date/time at which the last bulk publish was initiated.
*
* @return The date/time at which the last bulk publish was initiated.
*/
public final Instant bulkPublishStartTime() {
return bulkPublishStartTime;
}
/**
* If BulkPublishStatus is SUCCEEDED, the time the last bulk publish operation completed.
*
* @return If BulkPublishStatus is SUCCEEDED, the time the last bulk publish operation completed.
*/
public final Instant bulkPublishCompleteTime() {
return bulkPublishCompleteTime;
}
/**
* Status of the last bulk publish operation, valid values are:
*
* NOT_STARTED - No bulk publish has been requested for this identity pool
*
*
* IN_PROGRESS - Data is being published to the configured stream
*
*
* SUCCEEDED - All data for the identity pool has been published to the configured stream
*
*
* FAILED - Some portion of the data has failed to publish, check FailureMessage for the cause.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #bulkPublishStatus}
* will return {@link BulkPublishStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #bulkPublishStatusAsString}.
*
*
* @return Status of the last bulk publish operation, valid values are:
*
* NOT_STARTED - No bulk publish has been requested for this identity pool
*
*
* IN_PROGRESS - Data is being published to the configured stream
*
*
* SUCCEEDED - All data for the identity pool has been published to the configured stream
*
*
* FAILED - Some portion of the data has failed to publish, check FailureMessage for the cause.
*
* @see BulkPublishStatus
*/
public final BulkPublishStatus bulkPublishStatus() {
return BulkPublishStatus.fromValue(bulkPublishStatus);
}
/**
* Status of the last bulk publish operation, valid values are:
*
* NOT_STARTED - No bulk publish has been requested for this identity pool
*
*
* IN_PROGRESS - Data is being published to the configured stream
*
*
* SUCCEEDED - All data for the identity pool has been published to the configured stream
*
*
* FAILED - Some portion of the data has failed to publish, check FailureMessage for the cause.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #bulkPublishStatus}
* will return {@link BulkPublishStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #bulkPublishStatusAsString}.
*
*
* @return Status of the last bulk publish operation, valid values are:
*
* NOT_STARTED - No bulk publish has been requested for this identity pool
*
*
* IN_PROGRESS - Data is being published to the configured stream
*
*
* SUCCEEDED - All data for the identity pool has been published to the configured stream
*
*
* FAILED - Some portion of the data has failed to publish, check FailureMessage for the cause.
*
* @see BulkPublishStatus
*/
public final String bulkPublishStatusAsString() {
return bulkPublishStatus;
}
/**
* If BulkPublishStatus is FAILED this field will contain the error message that caused the bulk publish to fail.
*
* @return If BulkPublishStatus is FAILED this field will contain the error message that caused the bulk publish to
* fail.
*/
public final String failureMessage() {
return failureMessage;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(identityPoolId());
hashCode = 31 * hashCode + Objects.hashCode(bulkPublishStartTime());
hashCode = 31 * hashCode + Objects.hashCode(bulkPublishCompleteTime());
hashCode = 31 * hashCode + Objects.hashCode(bulkPublishStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(failureMessage());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetBulkPublishDetailsResponse)) {
return false;
}
GetBulkPublishDetailsResponse other = (GetBulkPublishDetailsResponse) obj;
return Objects.equals(identityPoolId(), other.identityPoolId())
&& Objects.equals(bulkPublishStartTime(), other.bulkPublishStartTime())
&& Objects.equals(bulkPublishCompleteTime(), other.bulkPublishCompleteTime())
&& Objects.equals(bulkPublishStatusAsString(), other.bulkPublishStatusAsString())
&& Objects.equals(failureMessage(), other.failureMessage());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetBulkPublishDetailsResponse").add("IdentityPoolId", identityPoolId())
.add("BulkPublishStartTime", bulkPublishStartTime()).add("BulkPublishCompleteTime", bulkPublishCompleteTime())
.add("BulkPublishStatus", bulkPublishStatusAsString()).add("FailureMessage", failureMessage()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "IdentityPoolId":
return Optional.ofNullable(clazz.cast(identityPoolId()));
case "BulkPublishStartTime":
return Optional.ofNullable(clazz.cast(bulkPublishStartTime()));
case "BulkPublishCompleteTime":
return Optional.ofNullable(clazz.cast(bulkPublishCompleteTime()));
case "BulkPublishStatus":
return Optional.ofNullable(clazz.cast(bulkPublishStatusAsString()));
case "FailureMessage":
return Optional.ofNullable(clazz.cast(failureMessage()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function