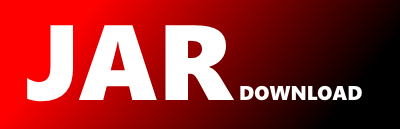
software.amazon.awssdk.services.computeoptimizer.ComputeOptimizerClient Maven / Gradle / Ivy
Show all versions of computeoptimizer Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.computeoptimizer;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.computeoptimizer.model.AccessDeniedException;
import software.amazon.awssdk.services.computeoptimizer.model.ComputeOptimizerException;
import software.amazon.awssdk.services.computeoptimizer.model.DescribeRecommendationExportJobsRequest;
import software.amazon.awssdk.services.computeoptimizer.model.DescribeRecommendationExportJobsResponse;
import software.amazon.awssdk.services.computeoptimizer.model.ExportAutoScalingGroupRecommendationsRequest;
import software.amazon.awssdk.services.computeoptimizer.model.ExportAutoScalingGroupRecommendationsResponse;
import software.amazon.awssdk.services.computeoptimizer.model.ExportEc2InstanceRecommendationsRequest;
import software.amazon.awssdk.services.computeoptimizer.model.ExportEc2InstanceRecommendationsResponse;
import software.amazon.awssdk.services.computeoptimizer.model.GetAutoScalingGroupRecommendationsRequest;
import software.amazon.awssdk.services.computeoptimizer.model.GetAutoScalingGroupRecommendationsResponse;
import software.amazon.awssdk.services.computeoptimizer.model.GetEbsVolumeRecommendationsRequest;
import software.amazon.awssdk.services.computeoptimizer.model.GetEbsVolumeRecommendationsResponse;
import software.amazon.awssdk.services.computeoptimizer.model.GetEc2InstanceRecommendationsRequest;
import software.amazon.awssdk.services.computeoptimizer.model.GetEc2InstanceRecommendationsResponse;
import software.amazon.awssdk.services.computeoptimizer.model.GetEc2RecommendationProjectedMetricsRequest;
import software.amazon.awssdk.services.computeoptimizer.model.GetEc2RecommendationProjectedMetricsResponse;
import software.amazon.awssdk.services.computeoptimizer.model.GetEnrollmentStatusRequest;
import software.amazon.awssdk.services.computeoptimizer.model.GetEnrollmentStatusResponse;
import software.amazon.awssdk.services.computeoptimizer.model.GetLambdaFunctionRecommendationsRequest;
import software.amazon.awssdk.services.computeoptimizer.model.GetLambdaFunctionRecommendationsResponse;
import software.amazon.awssdk.services.computeoptimizer.model.GetRecommendationSummariesRequest;
import software.amazon.awssdk.services.computeoptimizer.model.GetRecommendationSummariesResponse;
import software.amazon.awssdk.services.computeoptimizer.model.InternalServerException;
import software.amazon.awssdk.services.computeoptimizer.model.InvalidParameterValueException;
import software.amazon.awssdk.services.computeoptimizer.model.LimitExceededException;
import software.amazon.awssdk.services.computeoptimizer.model.MissingAuthenticationTokenException;
import software.amazon.awssdk.services.computeoptimizer.model.OptInRequiredException;
import software.amazon.awssdk.services.computeoptimizer.model.ResourceNotFoundException;
import software.amazon.awssdk.services.computeoptimizer.model.ServiceUnavailableException;
import software.amazon.awssdk.services.computeoptimizer.model.ThrottlingException;
import software.amazon.awssdk.services.computeoptimizer.model.UpdateEnrollmentStatusRequest;
import software.amazon.awssdk.services.computeoptimizer.model.UpdateEnrollmentStatusResponse;
/**
* Service client for accessing AWS Compute Optimizer. This can be created using the static {@link #builder()} method.
*
*
* AWS Compute Optimizer is a service that analyzes the configuration and utilization metrics of your AWS compute
* resources, such as EC2 instances, Auto Scaling groups, AWS Lambda functions, and Amazon EBS volumes. It reports
* whether your resources are optimal, and generates optimization recommendations to reduce the cost and improve the
* performance of your workloads. Compute Optimizer also provides recent utilization metric data, as well as projected
* utilization metric data for the recommendations, which you can use to evaluate which recommendation provides the best
* price-performance trade-off. The analysis of your usage patterns can help you decide when to move or resize your
* running resources, and still meet your performance and capacity requirements. For more information about Compute
* Optimizer, including the required permissions to use the service, see the AWS Compute Optimizer User Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface ComputeOptimizerClient extends SdkClient {
String SERVICE_NAME = "compute-optimizer";
/**
* Create a {@link ComputeOptimizerClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static ComputeOptimizerClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link ComputeOptimizerClient}.
*/
static ComputeOptimizerClientBuilder builder() {
return new DefaultComputeOptimizerClientBuilder();
}
/**
*
* Describes recommendation export jobs created in the last seven days.
*
*
* Use the ExportAutoScalingGroupRecommendations
or ExportEC2InstanceRecommendations
* actions to request an export of your recommendations. Then use the DescribeRecommendationExportJobs
* action to view your export jobs.
*
*
* @param describeRecommendationExportJobsRequest
* @return Result of the DescribeRecommendationExportJobs operation returned by the service.
* @throws OptInRequiredException
* The account is not opted in to AWS Compute Optimizer.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.DescribeRecommendationExportJobs
* @see AWS API Documentation
*/
default DescribeRecommendationExportJobsResponse describeRecommendationExportJobs(
DescribeRecommendationExportJobsRequest describeRecommendationExportJobsRequest) throws OptInRequiredException,
InternalServerException, ServiceUnavailableException, AccessDeniedException, InvalidParameterValueException,
ResourceNotFoundException, MissingAuthenticationTokenException, ThrottlingException, AwsServiceException,
SdkClientException, ComputeOptimizerException {
throw new UnsupportedOperationException();
}
/**
*
* Describes recommendation export jobs created in the last seven days.
*
*
* Use the ExportAutoScalingGroupRecommendations
or ExportEC2InstanceRecommendations
* actions to request an export of your recommendations. Then use the DescribeRecommendationExportJobs
* action to view your export jobs.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeRecommendationExportJobsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeRecommendationExportJobsRequest#builder()}
*
*
* @param describeRecommendationExportJobsRequest
* A {@link Consumer} that will call methods on {@link DescribeRecommendationExportJobsRequest.Builder} to
* create a request.
* @return Result of the DescribeRecommendationExportJobs operation returned by the service.
* @throws OptInRequiredException
* The account is not opted in to AWS Compute Optimizer.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.DescribeRecommendationExportJobs
* @see AWS API Documentation
*/
default DescribeRecommendationExportJobsResponse describeRecommendationExportJobs(
Consumer describeRecommendationExportJobsRequest)
throws OptInRequiredException, InternalServerException, ServiceUnavailableException, AccessDeniedException,
InvalidParameterValueException, ResourceNotFoundException, MissingAuthenticationTokenException, ThrottlingException,
AwsServiceException, SdkClientException, ComputeOptimizerException {
return describeRecommendationExportJobs(DescribeRecommendationExportJobsRequest.builder()
.applyMutation(describeRecommendationExportJobsRequest).build());
}
/**
*
* Exports optimization recommendations for Auto Scaling groups.
*
*
* Recommendations are exported in a comma-separated values (.csv) file, and its metadata in a JavaScript Object
* Notation (.json) file, to an existing Amazon Simple Storage Service (Amazon S3) bucket that you specify. For more
* information, see Exporting
* Recommendations in the Compute Optimizer User Guide.
*
*
* You can have only one Auto Scaling group export job in progress per AWS Region.
*
*
* @param exportAutoScalingGroupRecommendationsRequest
* @return Result of the ExportAutoScalingGroupRecommendations operation returned by the service.
* @throws OptInRequiredException
* The account is not opted in to AWS Compute Optimizer.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws LimitExceededException
* The request exceeds a limit of the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.ExportAutoScalingGroupRecommendations
* @see AWS API Documentation
*/
default ExportAutoScalingGroupRecommendationsResponse exportAutoScalingGroupRecommendations(
ExportAutoScalingGroupRecommendationsRequest exportAutoScalingGroupRecommendationsRequest)
throws OptInRequiredException, InternalServerException, ServiceUnavailableException, AccessDeniedException,
InvalidParameterValueException, MissingAuthenticationTokenException, ThrottlingException, LimitExceededException,
AwsServiceException, SdkClientException, ComputeOptimizerException {
throw new UnsupportedOperationException();
}
/**
*
* Exports optimization recommendations for Auto Scaling groups.
*
*
* Recommendations are exported in a comma-separated values (.csv) file, and its metadata in a JavaScript Object
* Notation (.json) file, to an existing Amazon Simple Storage Service (Amazon S3) bucket that you specify. For more
* information, see Exporting
* Recommendations in the Compute Optimizer User Guide.
*
*
* You can have only one Auto Scaling group export job in progress per AWS Region.
*
*
*
* This is a convenience which creates an instance of the
* {@link ExportAutoScalingGroupRecommendationsRequest.Builder} avoiding the need to create one manually via
* {@link ExportAutoScalingGroupRecommendationsRequest#builder()}
*
*
* @param exportAutoScalingGroupRecommendationsRequest
* A {@link Consumer} that will call methods on {@link ExportAutoScalingGroupRecommendationsRequest.Builder}
* to create a request.
* @return Result of the ExportAutoScalingGroupRecommendations operation returned by the service.
* @throws OptInRequiredException
* The account is not opted in to AWS Compute Optimizer.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws LimitExceededException
* The request exceeds a limit of the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.ExportAutoScalingGroupRecommendations
* @see AWS API Documentation
*/
default ExportAutoScalingGroupRecommendationsResponse exportAutoScalingGroupRecommendations(
Consumer exportAutoScalingGroupRecommendationsRequest)
throws OptInRequiredException, InternalServerException, ServiceUnavailableException, AccessDeniedException,
InvalidParameterValueException, MissingAuthenticationTokenException, ThrottlingException, LimitExceededException,
AwsServiceException, SdkClientException, ComputeOptimizerException {
return exportAutoScalingGroupRecommendations(ExportAutoScalingGroupRecommendationsRequest.builder()
.applyMutation(exportAutoScalingGroupRecommendationsRequest).build());
}
/**
*
* Exports optimization recommendations for Amazon EC2 instances.
*
*
* Recommendations are exported in a comma-separated values (.csv) file, and its metadata in a JavaScript Object
* Notation (.json) file, to an existing Amazon Simple Storage Service (Amazon S3) bucket that you specify. For more
* information, see Exporting
* Recommendations in the Compute Optimizer User Guide.
*
*
* You can have only one Amazon EC2 instance export job in progress per AWS Region.
*
*
* @param exportEc2InstanceRecommendationsRequest
* @return Result of the ExportEC2InstanceRecommendations operation returned by the service.
* @throws OptInRequiredException
* The account is not opted in to AWS Compute Optimizer.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws LimitExceededException
* The request exceeds a limit of the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.ExportEC2InstanceRecommendations
* @see AWS API Documentation
*/
default ExportEc2InstanceRecommendationsResponse exportEC2InstanceRecommendations(
ExportEc2InstanceRecommendationsRequest exportEc2InstanceRecommendationsRequest) throws OptInRequiredException,
InternalServerException, ServiceUnavailableException, AccessDeniedException, InvalidParameterValueException,
MissingAuthenticationTokenException, ThrottlingException, LimitExceededException, AwsServiceException,
SdkClientException, ComputeOptimizerException {
throw new UnsupportedOperationException();
}
/**
*
* Exports optimization recommendations for Amazon EC2 instances.
*
*
* Recommendations are exported in a comma-separated values (.csv) file, and its metadata in a JavaScript Object
* Notation (.json) file, to an existing Amazon Simple Storage Service (Amazon S3) bucket that you specify. For more
* information, see Exporting
* Recommendations in the Compute Optimizer User Guide.
*
*
* You can have only one Amazon EC2 instance export job in progress per AWS Region.
*
*
*
* This is a convenience which creates an instance of the {@link ExportEc2InstanceRecommendationsRequest.Builder}
* avoiding the need to create one manually via {@link ExportEc2InstanceRecommendationsRequest#builder()}
*
*
* @param exportEc2InstanceRecommendationsRequest
* A {@link Consumer} that will call methods on {@link ExportEC2InstanceRecommendationsRequest.Builder} to
* create a request.
* @return Result of the ExportEC2InstanceRecommendations operation returned by the service.
* @throws OptInRequiredException
* The account is not opted in to AWS Compute Optimizer.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws LimitExceededException
* The request exceeds a limit of the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.ExportEC2InstanceRecommendations
* @see AWS API Documentation
*/
default ExportEc2InstanceRecommendationsResponse exportEC2InstanceRecommendations(
Consumer exportEc2InstanceRecommendationsRequest)
throws OptInRequiredException, InternalServerException, ServiceUnavailableException, AccessDeniedException,
InvalidParameterValueException, MissingAuthenticationTokenException, ThrottlingException, LimitExceededException,
AwsServiceException, SdkClientException, ComputeOptimizerException {
return exportEC2InstanceRecommendations(ExportEc2InstanceRecommendationsRequest.builder()
.applyMutation(exportEc2InstanceRecommendationsRequest).build());
}
/**
*
* Returns Auto Scaling group recommendations.
*
*
* AWS Compute Optimizer generates recommendations for Amazon EC2 Auto Scaling groups that meet a specific set of
* requirements. For more information, see the Supported resources and
* requirements in the AWS Compute Optimizer User Guide.
*
*
* @param getAutoScalingGroupRecommendationsRequest
* @return Result of the GetAutoScalingGroupRecommendations operation returned by the service.
* @throws OptInRequiredException
* The account is not opted in to AWS Compute Optimizer.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.GetAutoScalingGroupRecommendations
* @see AWS API Documentation
*/
default GetAutoScalingGroupRecommendationsResponse getAutoScalingGroupRecommendations(
GetAutoScalingGroupRecommendationsRequest getAutoScalingGroupRecommendationsRequest) throws OptInRequiredException,
InternalServerException, ServiceUnavailableException, AccessDeniedException, InvalidParameterValueException,
ResourceNotFoundException, MissingAuthenticationTokenException, ThrottlingException, AwsServiceException,
SdkClientException, ComputeOptimizerException {
throw new UnsupportedOperationException();
}
/**
*
* Returns Auto Scaling group recommendations.
*
*
* AWS Compute Optimizer generates recommendations for Amazon EC2 Auto Scaling groups that meet a specific set of
* requirements. For more information, see the Supported resources and
* requirements in the AWS Compute Optimizer User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetAutoScalingGroupRecommendationsRequest.Builder}
* avoiding the need to create one manually via {@link GetAutoScalingGroupRecommendationsRequest#builder()}
*
*
* @param getAutoScalingGroupRecommendationsRequest
* A {@link Consumer} that will call methods on {@link GetAutoScalingGroupRecommendationsRequest.Builder} to
* create a request.
* @return Result of the GetAutoScalingGroupRecommendations operation returned by the service.
* @throws OptInRequiredException
* The account is not opted in to AWS Compute Optimizer.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.GetAutoScalingGroupRecommendations
* @see AWS API Documentation
*/
default GetAutoScalingGroupRecommendationsResponse getAutoScalingGroupRecommendations(
Consumer getAutoScalingGroupRecommendationsRequest)
throws OptInRequiredException, InternalServerException, ServiceUnavailableException, AccessDeniedException,
InvalidParameterValueException, ResourceNotFoundException, MissingAuthenticationTokenException, ThrottlingException,
AwsServiceException, SdkClientException, ComputeOptimizerException {
return getAutoScalingGroupRecommendations(GetAutoScalingGroupRecommendationsRequest.builder()
.applyMutation(getAutoScalingGroupRecommendationsRequest).build());
}
/**
*
* Returns Amazon Elastic Block Store (Amazon EBS) volume recommendations.
*
*
* AWS Compute Optimizer generates recommendations for Amazon EBS volumes that meet a specific set of requirements.
* For more information, see the Supported resources and
* requirements in the AWS Compute Optimizer User Guide.
*
*
* @param getEbsVolumeRecommendationsRequest
* @return Result of the GetEBSVolumeRecommendations operation returned by the service.
* @throws OptInRequiredException
* The account is not opted in to AWS Compute Optimizer.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.GetEBSVolumeRecommendations
* @see AWS API Documentation
*/
default GetEbsVolumeRecommendationsResponse getEBSVolumeRecommendations(
GetEbsVolumeRecommendationsRequest getEbsVolumeRecommendationsRequest) throws OptInRequiredException,
InternalServerException, ServiceUnavailableException, AccessDeniedException, InvalidParameterValueException,
ResourceNotFoundException, MissingAuthenticationTokenException, ThrottlingException, AwsServiceException,
SdkClientException, ComputeOptimizerException {
throw new UnsupportedOperationException();
}
/**
*
* Returns Amazon Elastic Block Store (Amazon EBS) volume recommendations.
*
*
* AWS Compute Optimizer generates recommendations for Amazon EBS volumes that meet a specific set of requirements.
* For more information, see the Supported resources and
* requirements in the AWS Compute Optimizer User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetEbsVolumeRecommendationsRequest.Builder}
* avoiding the need to create one manually via {@link GetEbsVolumeRecommendationsRequest#builder()}
*
*
* @param getEbsVolumeRecommendationsRequest
* A {@link Consumer} that will call methods on {@link GetEBSVolumeRecommendationsRequest.Builder} to create
* a request.
* @return Result of the GetEBSVolumeRecommendations operation returned by the service.
* @throws OptInRequiredException
* The account is not opted in to AWS Compute Optimizer.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.GetEBSVolumeRecommendations
* @see AWS API Documentation
*/
default GetEbsVolumeRecommendationsResponse getEBSVolumeRecommendations(
Consumer getEbsVolumeRecommendationsRequest)
throws OptInRequiredException, InternalServerException, ServiceUnavailableException, AccessDeniedException,
InvalidParameterValueException, ResourceNotFoundException, MissingAuthenticationTokenException, ThrottlingException,
AwsServiceException, SdkClientException, ComputeOptimizerException {
return getEBSVolumeRecommendations(GetEbsVolumeRecommendationsRequest.builder()
.applyMutation(getEbsVolumeRecommendationsRequest).build());
}
/**
*
* Returns Amazon EC2 instance recommendations.
*
*
* AWS Compute Optimizer generates recommendations for Amazon Elastic Compute Cloud (Amazon EC2) instances that meet
* a specific set of requirements. For more information, see the Supported resources and
* requirements in the AWS Compute Optimizer User Guide.
*
*
* @param getEc2InstanceRecommendationsRequest
* @return Result of the GetEC2InstanceRecommendations operation returned by the service.
* @throws OptInRequiredException
* The account is not opted in to AWS Compute Optimizer.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.GetEC2InstanceRecommendations
* @see AWS API Documentation
*/
default GetEc2InstanceRecommendationsResponse getEC2InstanceRecommendations(
GetEc2InstanceRecommendationsRequest getEc2InstanceRecommendationsRequest) throws OptInRequiredException,
InternalServerException, ServiceUnavailableException, AccessDeniedException, InvalidParameterValueException,
ResourceNotFoundException, MissingAuthenticationTokenException, ThrottlingException, AwsServiceException,
SdkClientException, ComputeOptimizerException {
throw new UnsupportedOperationException();
}
/**
*
* Returns Amazon EC2 instance recommendations.
*
*
* AWS Compute Optimizer generates recommendations for Amazon Elastic Compute Cloud (Amazon EC2) instances that meet
* a specific set of requirements. For more information, see the Supported resources and
* requirements in the AWS Compute Optimizer User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetEc2InstanceRecommendationsRequest.Builder}
* avoiding the need to create one manually via {@link GetEc2InstanceRecommendationsRequest#builder()}
*
*
* @param getEc2InstanceRecommendationsRequest
* A {@link Consumer} that will call methods on {@link GetEC2InstanceRecommendationsRequest.Builder} to
* create a request.
* @return Result of the GetEC2InstanceRecommendations operation returned by the service.
* @throws OptInRequiredException
* The account is not opted in to AWS Compute Optimizer.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.GetEC2InstanceRecommendations
* @see AWS API Documentation
*/
default GetEc2InstanceRecommendationsResponse getEC2InstanceRecommendations(
Consumer getEc2InstanceRecommendationsRequest)
throws OptInRequiredException, InternalServerException, ServiceUnavailableException, AccessDeniedException,
InvalidParameterValueException, ResourceNotFoundException, MissingAuthenticationTokenException, ThrottlingException,
AwsServiceException, SdkClientException, ComputeOptimizerException {
return getEC2InstanceRecommendations(GetEc2InstanceRecommendationsRequest.builder()
.applyMutation(getEc2InstanceRecommendationsRequest).build());
}
/**
*
* Returns the projected utilization metrics of Amazon EC2 instance recommendations.
*
*
*
* The Cpu
and Memory
metrics are the only projected utilization metrics returned when you
* run this action. Additionally, the Memory
metric is returned only for resources that have the
* unified CloudWatch agent installed on them. For more information, see Enabling Memory Utilization
* with the CloudWatch Agent.
*
*
*
* @param getEc2RecommendationProjectedMetricsRequest
* @return Result of the GetEC2RecommendationProjectedMetrics operation returned by the service.
* @throws OptInRequiredException
* The account is not opted in to AWS Compute Optimizer.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.GetEC2RecommendationProjectedMetrics
* @see AWS API Documentation
*/
default GetEc2RecommendationProjectedMetricsResponse getEC2RecommendationProjectedMetrics(
GetEc2RecommendationProjectedMetricsRequest getEc2RecommendationProjectedMetricsRequest)
throws OptInRequiredException, InternalServerException, ServiceUnavailableException, AccessDeniedException,
InvalidParameterValueException, ResourceNotFoundException, MissingAuthenticationTokenException, ThrottlingException,
AwsServiceException, SdkClientException, ComputeOptimizerException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the projected utilization metrics of Amazon EC2 instance recommendations.
*
*
*
* The Cpu
and Memory
metrics are the only projected utilization metrics returned when you
* run this action. Additionally, the Memory
metric is returned only for resources that have the
* unified CloudWatch agent installed on them. For more information, see Enabling Memory Utilization
* with the CloudWatch Agent.
*
*
*
* This is a convenience which creates an instance of the
* {@link GetEc2RecommendationProjectedMetricsRequest.Builder} avoiding the need to create one manually via
* {@link GetEc2RecommendationProjectedMetricsRequest#builder()}
*
*
* @param getEc2RecommendationProjectedMetricsRequest
* A {@link Consumer} that will call methods on {@link GetEC2RecommendationProjectedMetricsRequest.Builder}
* to create a request.
* @return Result of the GetEC2RecommendationProjectedMetrics operation returned by the service.
* @throws OptInRequiredException
* The account is not opted in to AWS Compute Optimizer.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.GetEC2RecommendationProjectedMetrics
* @see AWS API Documentation
*/
default GetEc2RecommendationProjectedMetricsResponse getEC2RecommendationProjectedMetrics(
Consumer getEc2RecommendationProjectedMetricsRequest)
throws OptInRequiredException, InternalServerException, ServiceUnavailableException, AccessDeniedException,
InvalidParameterValueException, ResourceNotFoundException, MissingAuthenticationTokenException, ThrottlingException,
AwsServiceException, SdkClientException, ComputeOptimizerException {
return getEC2RecommendationProjectedMetrics(GetEc2RecommendationProjectedMetricsRequest.builder()
.applyMutation(getEc2RecommendationProjectedMetricsRequest).build());
}
/**
*
* Returns the enrollment (opt in) status of an account to the AWS Compute Optimizer service.
*
*
* If the account is the management account of an organization, this action also confirms the enrollment status of
* member accounts within the organization.
*
*
* @param getEnrollmentStatusRequest
* @return Result of the GetEnrollmentStatus operation returned by the service.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.GetEnrollmentStatus
* @see AWS API Documentation
*/
default GetEnrollmentStatusResponse getEnrollmentStatus(GetEnrollmentStatusRequest getEnrollmentStatusRequest)
throws InternalServerException, ServiceUnavailableException, AccessDeniedException, InvalidParameterValueException,
MissingAuthenticationTokenException, ThrottlingException, AwsServiceException, SdkClientException,
ComputeOptimizerException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the enrollment (opt in) status of an account to the AWS Compute Optimizer service.
*
*
* If the account is the management account of an organization, this action also confirms the enrollment status of
* member accounts within the organization.
*
*
*
* This is a convenience which creates an instance of the {@link GetEnrollmentStatusRequest.Builder} avoiding the
* need to create one manually via {@link GetEnrollmentStatusRequest#builder()}
*
*
* @param getEnrollmentStatusRequest
* A {@link Consumer} that will call methods on {@link GetEnrollmentStatusRequest.Builder} to create a
* request.
* @return Result of the GetEnrollmentStatus operation returned by the service.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.GetEnrollmentStatus
* @see AWS API Documentation
*/
default GetEnrollmentStatusResponse getEnrollmentStatus(
Consumer getEnrollmentStatusRequest) throws InternalServerException,
ServiceUnavailableException, AccessDeniedException, InvalidParameterValueException,
MissingAuthenticationTokenException, ThrottlingException, AwsServiceException, SdkClientException,
ComputeOptimizerException {
return getEnrollmentStatus(GetEnrollmentStatusRequest.builder().applyMutation(getEnrollmentStatusRequest).build());
}
/**
*
* Returns AWS Lambda function recommendations.
*
*
* AWS Compute Optimizer generates recommendations for functions that meet a specific set of requirements. For more
* information, see the Supported resources and
* requirements in the AWS Compute Optimizer User Guide.
*
*
* @param getLambdaFunctionRecommendationsRequest
* @return Result of the GetLambdaFunctionRecommendations operation returned by the service.
* @throws OptInRequiredException
* The account is not opted in to AWS Compute Optimizer.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws LimitExceededException
* The request exceeds a limit of the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.GetLambdaFunctionRecommendations
* @see AWS API Documentation
*/
default GetLambdaFunctionRecommendationsResponse getLambdaFunctionRecommendations(
GetLambdaFunctionRecommendationsRequest getLambdaFunctionRecommendationsRequest) throws OptInRequiredException,
InternalServerException, ServiceUnavailableException, AccessDeniedException, InvalidParameterValueException,
MissingAuthenticationTokenException, ThrottlingException, LimitExceededException, AwsServiceException,
SdkClientException, ComputeOptimizerException {
throw new UnsupportedOperationException();
}
/**
*
* Returns AWS Lambda function recommendations.
*
*
* AWS Compute Optimizer generates recommendations for functions that meet a specific set of requirements. For more
* information, see the Supported resources and
* requirements in the AWS Compute Optimizer User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetLambdaFunctionRecommendationsRequest.Builder}
* avoiding the need to create one manually via {@link GetLambdaFunctionRecommendationsRequest#builder()}
*
*
* @param getLambdaFunctionRecommendationsRequest
* A {@link Consumer} that will call methods on {@link GetLambdaFunctionRecommendationsRequest.Builder} to
* create a request.
* @return Result of the GetLambdaFunctionRecommendations operation returned by the service.
* @throws OptInRequiredException
* The account is not opted in to AWS Compute Optimizer.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws LimitExceededException
* The request exceeds a limit of the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.GetLambdaFunctionRecommendations
* @see AWS API Documentation
*/
default GetLambdaFunctionRecommendationsResponse getLambdaFunctionRecommendations(
Consumer getLambdaFunctionRecommendationsRequest)
throws OptInRequiredException, InternalServerException, ServiceUnavailableException, AccessDeniedException,
InvalidParameterValueException, MissingAuthenticationTokenException, ThrottlingException, LimitExceededException,
AwsServiceException, SdkClientException, ComputeOptimizerException {
return getLambdaFunctionRecommendations(GetLambdaFunctionRecommendationsRequest.builder()
.applyMutation(getLambdaFunctionRecommendationsRequest).build());
}
/**
*
* Returns the optimization findings for an account.
*
*
* For example, it returns the number of Amazon EC2 instances in an account that are under-provisioned,
* over-provisioned, or optimized. It also returns the number of Auto Scaling groups in an account that are not
* optimized, or optimized.
*
*
* @param getRecommendationSummariesRequest
* @return Result of the GetRecommendationSummaries operation returned by the service.
* @throws OptInRequiredException
* The account is not opted in to AWS Compute Optimizer.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.GetRecommendationSummaries
* @see AWS API Documentation
*/
default GetRecommendationSummariesResponse getRecommendationSummaries(
GetRecommendationSummariesRequest getRecommendationSummariesRequest) throws OptInRequiredException,
InternalServerException, ServiceUnavailableException, AccessDeniedException, InvalidParameterValueException,
MissingAuthenticationTokenException, ThrottlingException, AwsServiceException, SdkClientException,
ComputeOptimizerException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the optimization findings for an account.
*
*
* For example, it returns the number of Amazon EC2 instances in an account that are under-provisioned,
* over-provisioned, or optimized. It also returns the number of Auto Scaling groups in an account that are not
* optimized, or optimized.
*
*
*
* This is a convenience which creates an instance of the {@link GetRecommendationSummariesRequest.Builder} avoiding
* the need to create one manually via {@link GetRecommendationSummariesRequest#builder()}
*
*
* @param getRecommendationSummariesRequest
* A {@link Consumer} that will call methods on {@link GetRecommendationSummariesRequest.Builder} to create a
* request.
* @return Result of the GetRecommendationSummaries operation returned by the service.
* @throws OptInRequiredException
* The account is not opted in to AWS Compute Optimizer.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.GetRecommendationSummaries
* @see AWS API Documentation
*/
default GetRecommendationSummariesResponse getRecommendationSummaries(
Consumer getRecommendationSummariesRequest) throws OptInRequiredException,
InternalServerException, ServiceUnavailableException, AccessDeniedException, InvalidParameterValueException,
MissingAuthenticationTokenException, ThrottlingException, AwsServiceException, SdkClientException,
ComputeOptimizerException {
return getRecommendationSummaries(GetRecommendationSummariesRequest.builder()
.applyMutation(getRecommendationSummariesRequest).build());
}
/**
*
* Updates the enrollment (opt in) status of an account to the AWS Compute Optimizer service.
*
*
* If the account is a management account of an organization, this action can also be used to enroll member accounts
* within the organization.
*
*
* @param updateEnrollmentStatusRequest
* @return Result of the UpdateEnrollmentStatus operation returned by the service.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.UpdateEnrollmentStatus
* @see AWS API Documentation
*/
default UpdateEnrollmentStatusResponse updateEnrollmentStatus(UpdateEnrollmentStatusRequest updateEnrollmentStatusRequest)
throws InternalServerException, ServiceUnavailableException, AccessDeniedException, InvalidParameterValueException,
MissingAuthenticationTokenException, ThrottlingException, AwsServiceException, SdkClientException,
ComputeOptimizerException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the enrollment (opt in) status of an account to the AWS Compute Optimizer service.
*
*
* If the account is a management account of an organization, this action can also be used to enroll member accounts
* within the organization.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateEnrollmentStatusRequest.Builder} avoiding the
* need to create one manually via {@link UpdateEnrollmentStatusRequest#builder()}
*
*
* @param updateEnrollmentStatusRequest
* A {@link Consumer} that will call methods on {@link UpdateEnrollmentStatusRequest.Builder} to create a
* request.
* @return Result of the UpdateEnrollmentStatus operation returned by the service.
* @throws InternalServerException
* An internal error has occurred. Try your call again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the server.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws InvalidParameterValueException
* An invalid or out-of-range value was supplied for the input parameter.
* @throws MissingAuthenticationTokenException
* The request must contain either a valid (registered) AWS access key ID or X.509 certificate.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ComputeOptimizerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ComputeOptimizerClient.UpdateEnrollmentStatus
* @see AWS API Documentation
*/
default UpdateEnrollmentStatusResponse updateEnrollmentStatus(
Consumer updateEnrollmentStatusRequest) throws InternalServerException,
ServiceUnavailableException, AccessDeniedException, InvalidParameterValueException,
MissingAuthenticationTokenException, ThrottlingException, AwsServiceException, SdkClientException,
ComputeOptimizerException {
return updateEnrollmentStatus(UpdateEnrollmentStatusRequest.builder().applyMutation(updateEnrollmentStatusRequest)
.build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of("compute-optimizer");
}
}