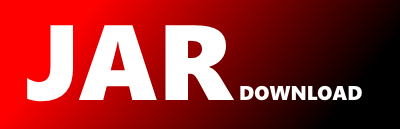
software.amazon.awssdk.services.computeoptimizer.model.EffectiveRecommendationPreferences Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.computeoptimizer.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the effective recommendation preferences for a resource.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class EffectiveRecommendationPreferences implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField> CPU_VENDOR_ARCHITECTURES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("cpuVendorArchitectures")
.getter(getter(EffectiveRecommendationPreferences::cpuVendorArchitecturesAsStrings))
.setter(setter(Builder::cpuVendorArchitecturesWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cpuVendorArchitectures").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ENHANCED_INFRASTRUCTURE_METRICS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("enhancedInfrastructureMetrics")
.getter(getter(EffectiveRecommendationPreferences::enhancedInfrastructureMetricsAsString))
.setter(setter(Builder::enhancedInfrastructureMetrics))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("enhancedInfrastructureMetrics")
.build()).build();
private static final SdkField INFERRED_WORKLOAD_TYPES_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("inferredWorkloadTypes")
.getter(getter(EffectiveRecommendationPreferences::inferredWorkloadTypesAsString))
.setter(setter(Builder::inferredWorkloadTypes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("inferredWorkloadTypes").build())
.build();
private static final SdkField EXTERNAL_METRICS_PREFERENCE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("externalMetricsPreference")
.getter(getter(EffectiveRecommendationPreferences::externalMetricsPreference))
.setter(setter(Builder::externalMetricsPreference)).constructor(ExternalMetricsPreference::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("externalMetricsPreference").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
CPU_VENDOR_ARCHITECTURES_FIELD, ENHANCED_INFRASTRUCTURE_METRICS_FIELD, INFERRED_WORKLOAD_TYPES_FIELD,
EXTERNAL_METRICS_PREFERENCE_FIELD));
private static final long serialVersionUID = 1L;
private final List cpuVendorArchitectures;
private final String enhancedInfrastructureMetrics;
private final String inferredWorkloadTypes;
private final ExternalMetricsPreference externalMetricsPreference;
private EffectiveRecommendationPreferences(BuilderImpl builder) {
this.cpuVendorArchitectures = builder.cpuVendorArchitectures;
this.enhancedInfrastructureMetrics = builder.enhancedInfrastructureMetrics;
this.inferredWorkloadTypes = builder.inferredWorkloadTypes;
this.externalMetricsPreference = builder.externalMetricsPreference;
}
/**
*
* Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute Optimizer
* returns recommendations that consist of Graviton2 instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization metrics
* for Graviton2 instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request, Compute
* Optimizer exports recommendations that consist of Graviton2 instance types only.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCpuVendorArchitectures} method.
*
*
* @return Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute
* Optimizer returns recommendations that consist of Graviton2 instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization
* metrics for Graviton2 instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request,
* Compute Optimizer exports recommendations that consist of Graviton2 instance types only.
*
*
*/
public final List cpuVendorArchitectures() {
return CpuVendorArchitecturesCopier.copyStringToEnum(cpuVendorArchitectures);
}
/**
* For responses, this returns true if the service returned a value for the CpuVendorArchitectures property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasCpuVendorArchitectures() {
return cpuVendorArchitectures != null && !(cpuVendorArchitectures instanceof SdkAutoConstructList);
}
/**
*
* Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute Optimizer
* returns recommendations that consist of Graviton2 instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization metrics
* for Graviton2 instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request, Compute
* Optimizer exports recommendations that consist of Graviton2 instance types only.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCpuVendorArchitectures} method.
*
*
* @return Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute
* Optimizer returns recommendations that consist of Graviton2 instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization
* metrics for Graviton2 instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request,
* Compute Optimizer exports recommendations that consist of Graviton2 instance types only.
*
*
*/
public final List cpuVendorArchitecturesAsStrings() {
return cpuVendorArchitectures;
}
/**
*
* Describes the activation status of the enhanced infrastructure metrics preference.
*
*
* A status of Active
confirms that the preference is applied in the latest recommendation refresh, and
* a status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* For more information, see Enhanced
* infrastructure metrics in the Compute Optimizer User Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #enhancedInfrastructureMetrics} will return {@link EnhancedInfrastructureMetrics#UNKNOWN_TO_SDK_VERSION}.
* The raw value returned by the service is available from {@link #enhancedInfrastructureMetricsAsString}.
*
*
* @return Describes the activation status of the enhanced infrastructure metrics preference.
*
* A status of Active
confirms that the preference is applied in the latest recommendation
* refresh, and a status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* For more information, see Enhanced infrastructure metrics in the Compute Optimizer User Guide.
* @see EnhancedInfrastructureMetrics
*/
public final EnhancedInfrastructureMetrics enhancedInfrastructureMetrics() {
return EnhancedInfrastructureMetrics.fromValue(enhancedInfrastructureMetrics);
}
/**
*
* Describes the activation status of the enhanced infrastructure metrics preference.
*
*
* A status of Active
confirms that the preference is applied in the latest recommendation refresh, and
* a status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* For more information, see Enhanced
* infrastructure metrics in the Compute Optimizer User Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #enhancedInfrastructureMetrics} will return {@link EnhancedInfrastructureMetrics#UNKNOWN_TO_SDK_VERSION}.
* The raw value returned by the service is available from {@link #enhancedInfrastructureMetricsAsString}.
*
*
* @return Describes the activation status of the enhanced infrastructure metrics preference.
*
* A status of Active
confirms that the preference is applied in the latest recommendation
* refresh, and a status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* For more information, see Enhanced infrastructure metrics in the Compute Optimizer User Guide.
* @see EnhancedInfrastructureMetrics
*/
public final String enhancedInfrastructureMetricsAsString() {
return enhancedInfrastructureMetrics;
}
/**
*
* Describes the activation status of the inferred workload types preference.
*
*
* A status of Active
confirms that the preference is applied in the latest recommendation refresh. A
* status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #inferredWorkloadTypes} will return {@link InferredWorkloadTypesPreference#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #inferredWorkloadTypesAsString}.
*
*
* @return Describes the activation status of the inferred workload types preference.
*
* A status of Active
confirms that the preference is applied in the latest recommendation
* refresh. A status of Inactive
confirms that it's not yet applied to recommendations.
* @see InferredWorkloadTypesPreference
*/
public final InferredWorkloadTypesPreference inferredWorkloadTypes() {
return InferredWorkloadTypesPreference.fromValue(inferredWorkloadTypes);
}
/**
*
* Describes the activation status of the inferred workload types preference.
*
*
* A status of Active
confirms that the preference is applied in the latest recommendation refresh. A
* status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #inferredWorkloadTypes} will return {@link InferredWorkloadTypesPreference#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #inferredWorkloadTypesAsString}.
*
*
* @return Describes the activation status of the inferred workload types preference.
*
* A status of Active
confirms that the preference is applied in the latest recommendation
* refresh. A status of Inactive
confirms that it's not yet applied to recommendations.
* @see InferredWorkloadTypesPreference
*/
public final String inferredWorkloadTypesAsString() {
return inferredWorkloadTypes;
}
/**
*
* An object that describes the external metrics recommendation preference.
*
*
* If the preference is applied in the latest recommendation refresh, an object with a valid source
* value appears in the response. If the preference isn't applied to the recommendations already, then this object
* doesn't appear in the response.
*
*
* @return An object that describes the external metrics recommendation preference.
*
* If the preference is applied in the latest recommendation refresh, an object with a valid
* source
value appears in the response. If the preference isn't applied to the recommendations
* already, then this object doesn't appear in the response.
*/
public final ExternalMetricsPreference externalMetricsPreference() {
return externalMetricsPreference;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasCpuVendorArchitectures() ? cpuVendorArchitecturesAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(enhancedInfrastructureMetricsAsString());
hashCode = 31 * hashCode + Objects.hashCode(inferredWorkloadTypesAsString());
hashCode = 31 * hashCode + Objects.hashCode(externalMetricsPreference());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof EffectiveRecommendationPreferences)) {
return false;
}
EffectiveRecommendationPreferences other = (EffectiveRecommendationPreferences) obj;
return hasCpuVendorArchitectures() == other.hasCpuVendorArchitectures()
&& Objects.equals(cpuVendorArchitecturesAsStrings(), other.cpuVendorArchitecturesAsStrings())
&& Objects.equals(enhancedInfrastructureMetricsAsString(), other.enhancedInfrastructureMetricsAsString())
&& Objects.equals(inferredWorkloadTypesAsString(), other.inferredWorkloadTypesAsString())
&& Objects.equals(externalMetricsPreference(), other.externalMetricsPreference());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("EffectiveRecommendationPreferences")
.add("CpuVendorArchitectures", hasCpuVendorArchitectures() ? cpuVendorArchitecturesAsStrings() : null)
.add("EnhancedInfrastructureMetrics", enhancedInfrastructureMetricsAsString())
.add("InferredWorkloadTypes", inferredWorkloadTypesAsString())
.add("ExternalMetricsPreference", externalMetricsPreference()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "cpuVendorArchitectures":
return Optional.ofNullable(clazz.cast(cpuVendorArchitecturesAsStrings()));
case "enhancedInfrastructureMetrics":
return Optional.ofNullable(clazz.cast(enhancedInfrastructureMetricsAsString()));
case "inferredWorkloadTypes":
return Optional.ofNullable(clazz.cast(inferredWorkloadTypesAsString()));
case "externalMetricsPreference":
return Optional.ofNullable(clazz.cast(externalMetricsPreference()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute
* Optimizer returns recommendations that consist of Graviton2 instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization
* metrics for Graviton2 instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request,
* Compute Optimizer exports recommendations that consist of Graviton2 instance types only.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cpuVendorArchitecturesWithStrings(Collection cpuVendorArchitectures);
/**
*
* Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute
* Optimizer returns recommendations that consist of Graviton2 instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization
* metrics for Graviton2 instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request, Compute
* Optimizer exports recommendations that consist of Graviton2 instance types only.
*
*
*
*
* @param cpuVendorArchitectures
* Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute
* Optimizer returns recommendations that consist of Graviton2 instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization
* metrics for Graviton2 instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request,
* Compute Optimizer exports recommendations that consist of Graviton2 instance types only.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cpuVendorArchitecturesWithStrings(String... cpuVendorArchitectures);
/**
*
* Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute
* Optimizer returns recommendations that consist of Graviton2 instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization
* metrics for Graviton2 instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request, Compute
* Optimizer exports recommendations that consist of Graviton2 instance types only.
*
*
*
*
* @param cpuVendorArchitectures
* Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute
* Optimizer returns recommendations that consist of Graviton2 instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization
* metrics for Graviton2 instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request,
* Compute Optimizer exports recommendations that consist of Graviton2 instance types only.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cpuVendorArchitectures(Collection cpuVendorArchitectures);
/**
*
* Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute
* Optimizer returns recommendations that consist of Graviton2 instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization
* metrics for Graviton2 instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request, Compute
* Optimizer exports recommendations that consist of Graviton2 instance types only.
*
*
*
*
* @param cpuVendorArchitectures
* Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute
* Optimizer returns recommendations that consist of Graviton2 instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization
* metrics for Graviton2 instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request,
* Compute Optimizer exports recommendations that consist of Graviton2 instance types only.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cpuVendorArchitectures(CpuVendorArchitecture... cpuVendorArchitectures);
/**
*
* Describes the activation status of the enhanced infrastructure metrics preference.
*
*
* A status of Active
confirms that the preference is applied in the latest recommendation refresh,
* and a status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* For more information, see Enhanced
* infrastructure metrics in the Compute Optimizer User Guide.
*
*
* @param enhancedInfrastructureMetrics
* Describes the activation status of the enhanced infrastructure metrics preference.
*
* A status of Active
confirms that the preference is applied in the latest recommendation
* refresh, and a status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* For more information, see Enhanced infrastructure metrics in the Compute Optimizer User Guide.
* @see EnhancedInfrastructureMetrics
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnhancedInfrastructureMetrics
*/
Builder enhancedInfrastructureMetrics(String enhancedInfrastructureMetrics);
/**
*
* Describes the activation status of the enhanced infrastructure metrics preference.
*
*
* A status of Active
confirms that the preference is applied in the latest recommendation refresh,
* and a status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* For more information, see Enhanced
* infrastructure metrics in the Compute Optimizer User Guide.
*
*
* @param enhancedInfrastructureMetrics
* Describes the activation status of the enhanced infrastructure metrics preference.
*
* A status of Active
confirms that the preference is applied in the latest recommendation
* refresh, and a status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* For more information, see Enhanced infrastructure metrics in the Compute Optimizer User Guide.
* @see EnhancedInfrastructureMetrics
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnhancedInfrastructureMetrics
*/
Builder enhancedInfrastructureMetrics(EnhancedInfrastructureMetrics enhancedInfrastructureMetrics);
/**
*
* Describes the activation status of the inferred workload types preference.
*
*
* A status of Active
confirms that the preference is applied in the latest recommendation refresh.
* A status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* @param inferredWorkloadTypes
* Describes the activation status of the inferred workload types preference.
*
* A status of Active
confirms that the preference is applied in the latest recommendation
* refresh. A status of Inactive
confirms that it's not yet applied to recommendations.
* @see InferredWorkloadTypesPreference
* @return Returns a reference to this object so that method calls can be chained together.
* @see InferredWorkloadTypesPreference
*/
Builder inferredWorkloadTypes(String inferredWorkloadTypes);
/**
*
* Describes the activation status of the inferred workload types preference.
*
*
* A status of Active
confirms that the preference is applied in the latest recommendation refresh.
* A status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* @param inferredWorkloadTypes
* Describes the activation status of the inferred workload types preference.
*
* A status of Active
confirms that the preference is applied in the latest recommendation
* refresh. A status of Inactive
confirms that it's not yet applied to recommendations.
* @see InferredWorkloadTypesPreference
* @return Returns a reference to this object so that method calls can be chained together.
* @see InferredWorkloadTypesPreference
*/
Builder inferredWorkloadTypes(InferredWorkloadTypesPreference inferredWorkloadTypes);
/**
*
* An object that describes the external metrics recommendation preference.
*
*
* If the preference is applied in the latest recommendation refresh, an object with a valid source
* value appears in the response. If the preference isn't applied to the recommendations already, then this
* object doesn't appear in the response.
*
*
* @param externalMetricsPreference
* An object that describes the external metrics recommendation preference.
*
* If the preference is applied in the latest recommendation refresh, an object with a valid
* source
value appears in the response. If the preference isn't applied to the
* recommendations already, then this object doesn't appear in the response.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder externalMetricsPreference(ExternalMetricsPreference externalMetricsPreference);
/**
*
* An object that describes the external metrics recommendation preference.
*
*
* If the preference is applied in the latest recommendation refresh, an object with a valid source
* value appears in the response. If the preference isn't applied to the recommendations already, then this
* object doesn't appear in the response.
*
* This is a convenience method that creates an instance of the {@link ExternalMetricsPreference.Builder}
* avoiding the need to create one manually via {@link ExternalMetricsPreference#builder()}.
*
*
* When the {@link Consumer} completes, {@link ExternalMetricsPreference.Builder#build()} is called immediately
* and its result is passed to {@link #externalMetricsPreference(ExternalMetricsPreference)}.
*
* @param externalMetricsPreference
* a consumer that will call methods on {@link ExternalMetricsPreference.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #externalMetricsPreference(ExternalMetricsPreference)
*/
default Builder externalMetricsPreference(Consumer externalMetricsPreference) {
return externalMetricsPreference(ExternalMetricsPreference.builder().applyMutation(externalMetricsPreference).build());
}
}
static final class BuilderImpl implements Builder {
private List cpuVendorArchitectures = DefaultSdkAutoConstructList.getInstance();
private String enhancedInfrastructureMetrics;
private String inferredWorkloadTypes;
private ExternalMetricsPreference externalMetricsPreference;
private BuilderImpl() {
}
private BuilderImpl(EffectiveRecommendationPreferences model) {
cpuVendorArchitecturesWithStrings(model.cpuVendorArchitectures);
enhancedInfrastructureMetrics(model.enhancedInfrastructureMetrics);
inferredWorkloadTypes(model.inferredWorkloadTypes);
externalMetricsPreference(model.externalMetricsPreference);
}
public final Collection getCpuVendorArchitectures() {
if (cpuVendorArchitectures instanceof SdkAutoConstructList) {
return null;
}
return cpuVendorArchitectures;
}
public final void setCpuVendorArchitectures(Collection cpuVendorArchitectures) {
this.cpuVendorArchitectures = CpuVendorArchitecturesCopier.copy(cpuVendorArchitectures);
}
@Override
public final Builder cpuVendorArchitecturesWithStrings(Collection cpuVendorArchitectures) {
this.cpuVendorArchitectures = CpuVendorArchitecturesCopier.copy(cpuVendorArchitectures);
return this;
}
@Override
@SafeVarargs
public final Builder cpuVendorArchitecturesWithStrings(String... cpuVendorArchitectures) {
cpuVendorArchitecturesWithStrings(Arrays.asList(cpuVendorArchitectures));
return this;
}
@Override
public final Builder cpuVendorArchitectures(Collection cpuVendorArchitectures) {
this.cpuVendorArchitectures = CpuVendorArchitecturesCopier.copyEnumToString(cpuVendorArchitectures);
return this;
}
@Override
@SafeVarargs
public final Builder cpuVendorArchitectures(CpuVendorArchitecture... cpuVendorArchitectures) {
cpuVendorArchitectures(Arrays.asList(cpuVendorArchitectures));
return this;
}
public final String getEnhancedInfrastructureMetrics() {
return enhancedInfrastructureMetrics;
}
public final void setEnhancedInfrastructureMetrics(String enhancedInfrastructureMetrics) {
this.enhancedInfrastructureMetrics = enhancedInfrastructureMetrics;
}
@Override
public final Builder enhancedInfrastructureMetrics(String enhancedInfrastructureMetrics) {
this.enhancedInfrastructureMetrics = enhancedInfrastructureMetrics;
return this;
}
@Override
public final Builder enhancedInfrastructureMetrics(EnhancedInfrastructureMetrics enhancedInfrastructureMetrics) {
this.enhancedInfrastructureMetrics(enhancedInfrastructureMetrics == null ? null : enhancedInfrastructureMetrics
.toString());
return this;
}
public final String getInferredWorkloadTypes() {
return inferredWorkloadTypes;
}
public final void setInferredWorkloadTypes(String inferredWorkloadTypes) {
this.inferredWorkloadTypes = inferredWorkloadTypes;
}
@Override
public final Builder inferredWorkloadTypes(String inferredWorkloadTypes) {
this.inferredWorkloadTypes = inferredWorkloadTypes;
return this;
}
@Override
public final Builder inferredWorkloadTypes(InferredWorkloadTypesPreference inferredWorkloadTypes) {
this.inferredWorkloadTypes(inferredWorkloadTypes == null ? null : inferredWorkloadTypes.toString());
return this;
}
public final ExternalMetricsPreference.Builder getExternalMetricsPreference() {
return externalMetricsPreference != null ? externalMetricsPreference.toBuilder() : null;
}
public final void setExternalMetricsPreference(ExternalMetricsPreference.BuilderImpl externalMetricsPreference) {
this.externalMetricsPreference = externalMetricsPreference != null ? externalMetricsPreference.build() : null;
}
@Override
public final Builder externalMetricsPreference(ExternalMetricsPreference externalMetricsPreference) {
this.externalMetricsPreference = externalMetricsPreference;
return this;
}
@Override
public EffectiveRecommendationPreferences build() {
return new EffectiveRecommendationPreferences(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}