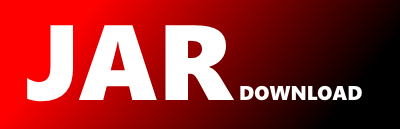
software.amazon.awssdk.services.connect.DefaultConnectAsyncClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.connect;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.connect.model.AssociateApprovedOriginRequest;
import software.amazon.awssdk.services.connect.model.AssociateApprovedOriginResponse;
import software.amazon.awssdk.services.connect.model.AssociateInstanceStorageConfigRequest;
import software.amazon.awssdk.services.connect.model.AssociateInstanceStorageConfigResponse;
import software.amazon.awssdk.services.connect.model.AssociateLambdaFunctionRequest;
import software.amazon.awssdk.services.connect.model.AssociateLambdaFunctionResponse;
import software.amazon.awssdk.services.connect.model.AssociateLexBotRequest;
import software.amazon.awssdk.services.connect.model.AssociateLexBotResponse;
import software.amazon.awssdk.services.connect.model.AssociateRoutingProfileQueuesRequest;
import software.amazon.awssdk.services.connect.model.AssociateRoutingProfileQueuesResponse;
import software.amazon.awssdk.services.connect.model.AssociateSecurityKeyRequest;
import software.amazon.awssdk.services.connect.model.AssociateSecurityKeyResponse;
import software.amazon.awssdk.services.connect.model.ConnectException;
import software.amazon.awssdk.services.connect.model.ConnectRequest;
import software.amazon.awssdk.services.connect.model.ContactFlowNotPublishedException;
import software.amazon.awssdk.services.connect.model.ContactNotFoundException;
import software.amazon.awssdk.services.connect.model.CreateContactFlowRequest;
import software.amazon.awssdk.services.connect.model.CreateContactFlowResponse;
import software.amazon.awssdk.services.connect.model.CreateInstanceRequest;
import software.amazon.awssdk.services.connect.model.CreateInstanceResponse;
import software.amazon.awssdk.services.connect.model.CreateIntegrationAssociationRequest;
import software.amazon.awssdk.services.connect.model.CreateIntegrationAssociationResponse;
import software.amazon.awssdk.services.connect.model.CreateQuickConnectRequest;
import software.amazon.awssdk.services.connect.model.CreateQuickConnectResponse;
import software.amazon.awssdk.services.connect.model.CreateRoutingProfileRequest;
import software.amazon.awssdk.services.connect.model.CreateRoutingProfileResponse;
import software.amazon.awssdk.services.connect.model.CreateUseCaseRequest;
import software.amazon.awssdk.services.connect.model.CreateUseCaseResponse;
import software.amazon.awssdk.services.connect.model.CreateUserHierarchyGroupRequest;
import software.amazon.awssdk.services.connect.model.CreateUserHierarchyGroupResponse;
import software.amazon.awssdk.services.connect.model.CreateUserRequest;
import software.amazon.awssdk.services.connect.model.CreateUserResponse;
import software.amazon.awssdk.services.connect.model.DeleteInstanceRequest;
import software.amazon.awssdk.services.connect.model.DeleteInstanceResponse;
import software.amazon.awssdk.services.connect.model.DeleteIntegrationAssociationRequest;
import software.amazon.awssdk.services.connect.model.DeleteIntegrationAssociationResponse;
import software.amazon.awssdk.services.connect.model.DeleteQuickConnectRequest;
import software.amazon.awssdk.services.connect.model.DeleteQuickConnectResponse;
import software.amazon.awssdk.services.connect.model.DeleteUseCaseRequest;
import software.amazon.awssdk.services.connect.model.DeleteUseCaseResponse;
import software.amazon.awssdk.services.connect.model.DeleteUserHierarchyGroupRequest;
import software.amazon.awssdk.services.connect.model.DeleteUserHierarchyGroupResponse;
import software.amazon.awssdk.services.connect.model.DeleteUserRequest;
import software.amazon.awssdk.services.connect.model.DeleteUserResponse;
import software.amazon.awssdk.services.connect.model.DescribeContactFlowRequest;
import software.amazon.awssdk.services.connect.model.DescribeContactFlowResponse;
import software.amazon.awssdk.services.connect.model.DescribeInstanceAttributeRequest;
import software.amazon.awssdk.services.connect.model.DescribeInstanceAttributeResponse;
import software.amazon.awssdk.services.connect.model.DescribeInstanceRequest;
import software.amazon.awssdk.services.connect.model.DescribeInstanceResponse;
import software.amazon.awssdk.services.connect.model.DescribeInstanceStorageConfigRequest;
import software.amazon.awssdk.services.connect.model.DescribeInstanceStorageConfigResponse;
import software.amazon.awssdk.services.connect.model.DescribeQuickConnectRequest;
import software.amazon.awssdk.services.connect.model.DescribeQuickConnectResponse;
import software.amazon.awssdk.services.connect.model.DescribeRoutingProfileRequest;
import software.amazon.awssdk.services.connect.model.DescribeRoutingProfileResponse;
import software.amazon.awssdk.services.connect.model.DescribeUserHierarchyGroupRequest;
import software.amazon.awssdk.services.connect.model.DescribeUserHierarchyGroupResponse;
import software.amazon.awssdk.services.connect.model.DescribeUserHierarchyStructureRequest;
import software.amazon.awssdk.services.connect.model.DescribeUserHierarchyStructureResponse;
import software.amazon.awssdk.services.connect.model.DescribeUserRequest;
import software.amazon.awssdk.services.connect.model.DescribeUserResponse;
import software.amazon.awssdk.services.connect.model.DestinationNotAllowedException;
import software.amazon.awssdk.services.connect.model.DisassociateApprovedOriginRequest;
import software.amazon.awssdk.services.connect.model.DisassociateApprovedOriginResponse;
import software.amazon.awssdk.services.connect.model.DisassociateInstanceStorageConfigRequest;
import software.amazon.awssdk.services.connect.model.DisassociateInstanceStorageConfigResponse;
import software.amazon.awssdk.services.connect.model.DisassociateLambdaFunctionRequest;
import software.amazon.awssdk.services.connect.model.DisassociateLambdaFunctionResponse;
import software.amazon.awssdk.services.connect.model.DisassociateLexBotRequest;
import software.amazon.awssdk.services.connect.model.DisassociateLexBotResponse;
import software.amazon.awssdk.services.connect.model.DisassociateRoutingProfileQueuesRequest;
import software.amazon.awssdk.services.connect.model.DisassociateRoutingProfileQueuesResponse;
import software.amazon.awssdk.services.connect.model.DisassociateSecurityKeyRequest;
import software.amazon.awssdk.services.connect.model.DisassociateSecurityKeyResponse;
import software.amazon.awssdk.services.connect.model.DuplicateResourceException;
import software.amazon.awssdk.services.connect.model.GetContactAttributesRequest;
import software.amazon.awssdk.services.connect.model.GetContactAttributesResponse;
import software.amazon.awssdk.services.connect.model.GetCurrentMetricDataRequest;
import software.amazon.awssdk.services.connect.model.GetCurrentMetricDataResponse;
import software.amazon.awssdk.services.connect.model.GetFederationTokenRequest;
import software.amazon.awssdk.services.connect.model.GetFederationTokenResponse;
import software.amazon.awssdk.services.connect.model.GetMetricDataRequest;
import software.amazon.awssdk.services.connect.model.GetMetricDataResponse;
import software.amazon.awssdk.services.connect.model.InternalServiceException;
import software.amazon.awssdk.services.connect.model.InvalidContactFlowException;
import software.amazon.awssdk.services.connect.model.InvalidParameterException;
import software.amazon.awssdk.services.connect.model.InvalidRequestException;
import software.amazon.awssdk.services.connect.model.LimitExceededException;
import software.amazon.awssdk.services.connect.model.ListApprovedOriginsRequest;
import software.amazon.awssdk.services.connect.model.ListApprovedOriginsResponse;
import software.amazon.awssdk.services.connect.model.ListContactFlowsRequest;
import software.amazon.awssdk.services.connect.model.ListContactFlowsResponse;
import software.amazon.awssdk.services.connect.model.ListHoursOfOperationsRequest;
import software.amazon.awssdk.services.connect.model.ListHoursOfOperationsResponse;
import software.amazon.awssdk.services.connect.model.ListInstanceAttributesRequest;
import software.amazon.awssdk.services.connect.model.ListInstanceAttributesResponse;
import software.amazon.awssdk.services.connect.model.ListInstanceStorageConfigsRequest;
import software.amazon.awssdk.services.connect.model.ListInstanceStorageConfigsResponse;
import software.amazon.awssdk.services.connect.model.ListInstancesRequest;
import software.amazon.awssdk.services.connect.model.ListInstancesResponse;
import software.amazon.awssdk.services.connect.model.ListIntegrationAssociationsRequest;
import software.amazon.awssdk.services.connect.model.ListIntegrationAssociationsResponse;
import software.amazon.awssdk.services.connect.model.ListLambdaFunctionsRequest;
import software.amazon.awssdk.services.connect.model.ListLambdaFunctionsResponse;
import software.amazon.awssdk.services.connect.model.ListLexBotsRequest;
import software.amazon.awssdk.services.connect.model.ListLexBotsResponse;
import software.amazon.awssdk.services.connect.model.ListPhoneNumbersRequest;
import software.amazon.awssdk.services.connect.model.ListPhoneNumbersResponse;
import software.amazon.awssdk.services.connect.model.ListPromptsRequest;
import software.amazon.awssdk.services.connect.model.ListPromptsResponse;
import software.amazon.awssdk.services.connect.model.ListQueuesRequest;
import software.amazon.awssdk.services.connect.model.ListQueuesResponse;
import software.amazon.awssdk.services.connect.model.ListQuickConnectsRequest;
import software.amazon.awssdk.services.connect.model.ListQuickConnectsResponse;
import software.amazon.awssdk.services.connect.model.ListRoutingProfileQueuesRequest;
import software.amazon.awssdk.services.connect.model.ListRoutingProfileQueuesResponse;
import software.amazon.awssdk.services.connect.model.ListRoutingProfilesRequest;
import software.amazon.awssdk.services.connect.model.ListRoutingProfilesResponse;
import software.amazon.awssdk.services.connect.model.ListSecurityKeysRequest;
import software.amazon.awssdk.services.connect.model.ListSecurityKeysResponse;
import software.amazon.awssdk.services.connect.model.ListSecurityProfilesRequest;
import software.amazon.awssdk.services.connect.model.ListSecurityProfilesResponse;
import software.amazon.awssdk.services.connect.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.connect.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.connect.model.ListUseCasesRequest;
import software.amazon.awssdk.services.connect.model.ListUseCasesResponse;
import software.amazon.awssdk.services.connect.model.ListUserHierarchyGroupsRequest;
import software.amazon.awssdk.services.connect.model.ListUserHierarchyGroupsResponse;
import software.amazon.awssdk.services.connect.model.ListUsersRequest;
import software.amazon.awssdk.services.connect.model.ListUsersResponse;
import software.amazon.awssdk.services.connect.model.OutboundContactNotPermittedException;
import software.amazon.awssdk.services.connect.model.ResourceConflictException;
import software.amazon.awssdk.services.connect.model.ResourceInUseException;
import software.amazon.awssdk.services.connect.model.ResourceNotFoundException;
import software.amazon.awssdk.services.connect.model.ResumeContactRecordingRequest;
import software.amazon.awssdk.services.connect.model.ResumeContactRecordingResponse;
import software.amazon.awssdk.services.connect.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.connect.model.StartChatContactRequest;
import software.amazon.awssdk.services.connect.model.StartChatContactResponse;
import software.amazon.awssdk.services.connect.model.StartContactRecordingRequest;
import software.amazon.awssdk.services.connect.model.StartContactRecordingResponse;
import software.amazon.awssdk.services.connect.model.StartOutboundVoiceContactRequest;
import software.amazon.awssdk.services.connect.model.StartOutboundVoiceContactResponse;
import software.amazon.awssdk.services.connect.model.StartTaskContactRequest;
import software.amazon.awssdk.services.connect.model.StartTaskContactResponse;
import software.amazon.awssdk.services.connect.model.StopContactRecordingRequest;
import software.amazon.awssdk.services.connect.model.StopContactRecordingResponse;
import software.amazon.awssdk.services.connect.model.StopContactRequest;
import software.amazon.awssdk.services.connect.model.StopContactResponse;
import software.amazon.awssdk.services.connect.model.SuspendContactRecordingRequest;
import software.amazon.awssdk.services.connect.model.SuspendContactRecordingResponse;
import software.amazon.awssdk.services.connect.model.TagResourceRequest;
import software.amazon.awssdk.services.connect.model.TagResourceResponse;
import software.amazon.awssdk.services.connect.model.ThrottlingException;
import software.amazon.awssdk.services.connect.model.UntagResourceRequest;
import software.amazon.awssdk.services.connect.model.UntagResourceResponse;
import software.amazon.awssdk.services.connect.model.UpdateContactAttributesRequest;
import software.amazon.awssdk.services.connect.model.UpdateContactAttributesResponse;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowContentRequest;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowContentResponse;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowNameRequest;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowNameResponse;
import software.amazon.awssdk.services.connect.model.UpdateInstanceAttributeRequest;
import software.amazon.awssdk.services.connect.model.UpdateInstanceAttributeResponse;
import software.amazon.awssdk.services.connect.model.UpdateInstanceStorageConfigRequest;
import software.amazon.awssdk.services.connect.model.UpdateInstanceStorageConfigResponse;
import software.amazon.awssdk.services.connect.model.UpdateQuickConnectConfigRequest;
import software.amazon.awssdk.services.connect.model.UpdateQuickConnectConfigResponse;
import software.amazon.awssdk.services.connect.model.UpdateQuickConnectNameRequest;
import software.amazon.awssdk.services.connect.model.UpdateQuickConnectNameResponse;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileConcurrencyRequest;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileConcurrencyResponse;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileDefaultOutboundQueueRequest;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileDefaultOutboundQueueResponse;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileNameRequest;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileNameResponse;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileQueuesRequest;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileQueuesResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserHierarchyGroupNameRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserHierarchyGroupNameResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserHierarchyRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserHierarchyResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserHierarchyStructureRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserHierarchyStructureResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserIdentityInfoRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserIdentityInfoResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserPhoneConfigRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserPhoneConfigResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserRoutingProfileRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserRoutingProfileResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserSecurityProfilesRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserSecurityProfilesResponse;
import software.amazon.awssdk.services.connect.model.UserNotFoundException;
import software.amazon.awssdk.services.connect.paginators.GetCurrentMetricDataPublisher;
import software.amazon.awssdk.services.connect.paginators.GetMetricDataPublisher;
import software.amazon.awssdk.services.connect.paginators.ListApprovedOriginsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListContactFlowsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListHoursOfOperationsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListInstanceAttributesPublisher;
import software.amazon.awssdk.services.connect.paginators.ListInstanceStorageConfigsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListInstancesPublisher;
import software.amazon.awssdk.services.connect.paginators.ListIntegrationAssociationsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListLambdaFunctionsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListLexBotsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListPhoneNumbersPublisher;
import software.amazon.awssdk.services.connect.paginators.ListPromptsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListQueuesPublisher;
import software.amazon.awssdk.services.connect.paginators.ListQuickConnectsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListRoutingProfileQueuesPublisher;
import software.amazon.awssdk.services.connect.paginators.ListRoutingProfilesPublisher;
import software.amazon.awssdk.services.connect.paginators.ListSecurityKeysPublisher;
import software.amazon.awssdk.services.connect.paginators.ListSecurityProfilesPublisher;
import software.amazon.awssdk.services.connect.paginators.ListUseCasesPublisher;
import software.amazon.awssdk.services.connect.paginators.ListUserHierarchyGroupsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListUsersPublisher;
import software.amazon.awssdk.services.connect.transform.AssociateApprovedOriginRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.AssociateInstanceStorageConfigRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.AssociateLambdaFunctionRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.AssociateLexBotRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.AssociateRoutingProfileQueuesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.AssociateSecurityKeyRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateContactFlowRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateInstanceRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateIntegrationAssociationRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateQuickConnectRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateRoutingProfileRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateUseCaseRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateUserHierarchyGroupRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateUserRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DeleteInstanceRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DeleteIntegrationAssociationRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DeleteQuickConnectRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DeleteUseCaseRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DeleteUserHierarchyGroupRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DeleteUserRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeContactFlowRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeInstanceAttributeRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeInstanceRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeInstanceStorageConfigRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeQuickConnectRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeRoutingProfileRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeUserHierarchyGroupRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeUserHierarchyStructureRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeUserRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DisassociateApprovedOriginRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DisassociateInstanceStorageConfigRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DisassociateLambdaFunctionRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DisassociateLexBotRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DisassociateRoutingProfileQueuesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DisassociateSecurityKeyRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.GetContactAttributesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.GetCurrentMetricDataRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.GetFederationTokenRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.GetMetricDataRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListApprovedOriginsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListContactFlowsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListHoursOfOperationsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListInstanceAttributesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListInstanceStorageConfigsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListInstancesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListIntegrationAssociationsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListLambdaFunctionsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListLexBotsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListPhoneNumbersRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListPromptsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListQueuesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListQuickConnectsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListRoutingProfileQueuesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListRoutingProfilesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListSecurityKeysRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListSecurityProfilesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListUseCasesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListUserHierarchyGroupsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListUsersRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ResumeContactRecordingRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.StartChatContactRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.StartContactRecordingRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.StartOutboundVoiceContactRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.StartTaskContactRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.StopContactRecordingRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.StopContactRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.SuspendContactRecordingRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateContactAttributesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateContactFlowContentRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateContactFlowNameRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateInstanceAttributeRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateInstanceStorageConfigRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateQuickConnectConfigRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateQuickConnectNameRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateRoutingProfileConcurrencyRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateRoutingProfileDefaultOutboundQueueRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateRoutingProfileNameRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateRoutingProfileQueuesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateUserHierarchyGroupNameRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateUserHierarchyRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateUserHierarchyStructureRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateUserIdentityInfoRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateUserPhoneConfigRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateUserRoutingProfileRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateUserSecurityProfilesRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link ConnectAsyncClient}.
*
* @see ConnectAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultConnectAsyncClient implements ConnectAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultConnectAsyncClient.class);
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultConnectAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Associates an approved origin to an Amazon Connect instance.
*
*
* @param associateApprovedOriginRequest
* @return A Java Future containing the result of the AssociateApprovedOrigin operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceConflictException A resource already has that name.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateApprovedOrigin
* @see AWS API Documentation
*/
@Override
public CompletableFuture associateApprovedOrigin(
AssociateApprovedOriginRequest associateApprovedOriginRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateApprovedOriginRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateApprovedOrigin");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateApprovedOriginResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateApprovedOrigin")
.withMarshaller(new AssociateApprovedOriginRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(associateApprovedOriginRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = associateApprovedOriginRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Associates a storage resource type for the first time. You can only associate one type of storage configuration
* in a single call. This means, for example, that you can't define an instance with multiple S3 buckets for storing
* chat transcripts.
*
*
* This API does not create a resource that doesn't exist. It only associates it to the instance. Ensure that the
* resource being specified in the storage configuration, like an Amazon S3 bucket, exists when being used for
* association.
*
*
* @param associateInstanceStorageConfigRequest
* @return A Java Future containing the result of the AssociateInstanceStorageConfig operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceConflictException A resource already has that name.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateInstanceStorageConfig
* @see AWS API Documentation
*/
@Override
public CompletableFuture associateInstanceStorageConfig(
AssociateInstanceStorageConfigRequest associateInstanceStorageConfigRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
associateInstanceStorageConfigRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateInstanceStorageConfig");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateInstanceStorageConfigResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateInstanceStorageConfig")
.withMarshaller(new AssociateInstanceStorageConfigRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(associateInstanceStorageConfigRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = associateInstanceStorageConfigRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Allows the specified Amazon Connect instance to access the specified Lambda function.
*
*
* @param associateLambdaFunctionRequest
* @return A Java Future containing the result of the AssociateLambdaFunction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceConflictException A resource already has that name.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateLambdaFunction
* @see AWS API Documentation
*/
@Override
public CompletableFuture associateLambdaFunction(
AssociateLambdaFunctionRequest associateLambdaFunctionRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateLambdaFunctionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateLambdaFunction");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateLambdaFunctionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateLambdaFunction")
.withMarshaller(new AssociateLambdaFunctionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(associateLambdaFunctionRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = associateLambdaFunctionRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Allows the specified Amazon Connect instance to access the specified Amazon Lex bot.
*
*
* @param associateLexBotRequest
* @return A Java Future containing the result of the AssociateLexBot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceConflictException A resource already has that name.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateLexBot
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture associateLexBot(AssociateLexBotRequest associateLexBotRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateLexBotRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateLexBot");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateLexBotResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateLexBot")
.withMarshaller(new AssociateLexBotRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(associateLexBotRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = associateLexBotRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Associates a set of queues with a routing profile.
*
*
* @param associateRoutingProfileQueuesRequest
* @return A Java Future containing the result of the AssociateRoutingProfileQueues operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateRoutingProfileQueues
* @see AWS API Documentation
*/
@Override
public CompletableFuture associateRoutingProfileQueues(
AssociateRoutingProfileQueuesRequest associateRoutingProfileQueuesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
associateRoutingProfileQueuesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateRoutingProfileQueues");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateRoutingProfileQueuesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateRoutingProfileQueues")
.withMarshaller(new AssociateRoutingProfileQueuesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(associateRoutingProfileQueuesRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = associateRoutingProfileQueuesRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Associates a security key to the instance.
*
*
* @param associateSecurityKeyRequest
* @return A Java Future containing the result of the AssociateSecurityKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceConflictException A resource already has that name.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateSecurityKey
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture associateSecurityKey(
AssociateSecurityKeyRequest associateSecurityKeyRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateSecurityKeyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateSecurityKey");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateSecurityKeyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateSecurityKey")
.withMarshaller(new AssociateSecurityKeyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(associateSecurityKeyRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = associateSecurityKeyRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a contact flow for the specified Amazon Connect instance.
*
*
* You can also create and update contact flows using the Amazon Connect Flow language.
*
*
* @param createContactFlowRequest
* @return A Java Future containing the result of the CreateContactFlow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidContactFlowException The contact flow is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - DuplicateResourceException A resource with the specified name already exists.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateContactFlow
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createContactFlow(CreateContactFlowRequest createContactFlowRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createContactFlowRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateContactFlow");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateContactFlowResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateContactFlow")
.withMarshaller(new CreateContactFlowRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createContactFlowRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createContactFlowRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Initiates an Amazon Connect instance with all the supported channels enabled. It does not attach any storage
* (such as Amazon S3, or Kinesis) or allow for any configurations on features such as Contact Lens for Amazon
* Connect.
*
*
* @param createInstanceRequest
* @return A Java Future containing the result of the CreateInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateInstance
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createInstance(CreateInstanceRequest createInstanceRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateInstance");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateInstance")
.withMarshaller(new CreateInstanceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createInstanceRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createInstanceRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Create an AppIntegration association with an Amazon Connect instance.
*
*
* @param createIntegrationAssociationRequest
* @return A Java Future containing the result of the CreateIntegrationAssociation operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateIntegrationAssociation
* @see AWS API Documentation
*/
@Override
public CompletableFuture createIntegrationAssociation(
CreateIntegrationAssociationRequest createIntegrationAssociationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createIntegrationAssociationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateIntegrationAssociation");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateIntegrationAssociationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateIntegrationAssociation")
.withMarshaller(new CreateIntegrationAssociationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createIntegrationAssociationRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createIntegrationAssociationRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Creates a quick connect for the specified Amazon Connect instance.
*
*
* @param createQuickConnectRequest
* @return A Java Future containing the result of the CreateQuickConnect operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateQuickConnect
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createQuickConnect(CreateQuickConnectRequest createQuickConnectRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createQuickConnectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateQuickConnect");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateQuickConnectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateQuickConnect")
.withMarshaller(new CreateQuickConnectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createQuickConnectRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createQuickConnectRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new routing profile.
*
*
* @param createRoutingProfileRequest
* @return A Java Future containing the result of the CreateRoutingProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateRoutingProfile
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createRoutingProfile(
CreateRoutingProfileRequest createRoutingProfileRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createRoutingProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateRoutingProfile");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateRoutingProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateRoutingProfile")
.withMarshaller(new CreateRoutingProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createRoutingProfileRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createRoutingProfileRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Creates a use case for an AppIntegration association.
*
*
* @param createUseCaseRequest
* @return A Java Future containing the result of the CreateUseCase operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateUseCase
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createUseCase(CreateUseCaseRequest createUseCaseRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createUseCaseRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateUseCase");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateUseCaseResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateUseCase")
.withMarshaller(new CreateUseCaseRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createUseCaseRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createUseCaseRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a user account for the specified Amazon Connect instance.
*
*
* For information about how to create user accounts using the Amazon Connect console, see Add Users in the Amazon
* Connect Administrator Guide.
*
*
* @param createUserRequest
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateUser
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createUser(CreateUserRequest createUserRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateUser");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateUserResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("CreateUser")
.withMarshaller(new CreateUserRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createUserRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createUserRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new user hierarchy group.
*
*
* @param createUserHierarchyGroupRequest
* @return A Java Future containing the result of the CreateUserHierarchyGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - DuplicateResourceException A resource with the specified name already exists.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateUserHierarchyGroup
* @see AWS API Documentation
*/
@Override
public CompletableFuture createUserHierarchyGroup(
CreateUserHierarchyGroupRequest createUserHierarchyGroupRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createUserHierarchyGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateUserHierarchyGroup");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateUserHierarchyGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateUserHierarchyGroup")
.withMarshaller(new CreateUserHierarchyGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createUserHierarchyGroupRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createUserHierarchyGroupRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Deletes the Amazon Connect instance.
*
*
* @param deleteInstanceRequest
* @return A Java Future containing the result of the DeleteInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteInstance
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteInstance(DeleteInstanceRequest deleteInstanceRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteInstance");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteInstance")
.withMarshaller(new DeleteInstanceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteInstanceRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteInstanceRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Deletes an AppIntegration association from an Amazon Connect instance. The association must not have any use
* cases associated with it.
*
*
* @param deleteIntegrationAssociationRequest
* @return A Java Future containing the result of the DeleteIntegrationAssociation operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteIntegrationAssociation
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteIntegrationAssociation(
DeleteIntegrationAssociationRequest deleteIntegrationAssociationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteIntegrationAssociationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteIntegrationAssociation");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteIntegrationAssociationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteIntegrationAssociation")
.withMarshaller(new DeleteIntegrationAssociationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteIntegrationAssociationRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteIntegrationAssociationRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Deletes a quick connect.
*
*
* @param deleteQuickConnectRequest
* @return A Java Future containing the result of the DeleteQuickConnect operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteQuickConnect
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteQuickConnect(DeleteQuickConnectRequest deleteQuickConnectRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteQuickConnectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteQuickConnect");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteQuickConnectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteQuickConnect")
.withMarshaller(new DeleteQuickConnectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteQuickConnectRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteQuickConnectRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Deletes a use case from an AppIntegration association.
*
*
* @param deleteUseCaseRequest
* @return A Java Future containing the result of the DeleteUseCase operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteUseCase
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteUseCase(DeleteUseCaseRequest deleteUseCaseRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteUseCaseRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteUseCase");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteUseCaseResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteUseCase")
.withMarshaller(new DeleteUseCaseRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteUseCaseRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteUseCaseRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a user account from the specified Amazon Connect instance.
*
*
* For information about what happens to a user's data when their account is deleted, see Delete Users from Your Amazon
* Connect Instance in the Amazon Connect Administrator Guide.
*
*
* @param deleteUserRequest
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteUser
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteUser(DeleteUserRequest deleteUserRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteUser");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteUserResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("DeleteUser")
.withMarshaller(new DeleteUserRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteUserRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteUserRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an existing user hierarchy group. It must not be associated with any agents or have any active child
* groups.
*
*
* @param deleteUserHierarchyGroupRequest
* @return A Java Future containing the result of the DeleteUserHierarchyGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ResourceInUseException That resource is already in use. Please try another.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteUserHierarchyGroup
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteUserHierarchyGroup(
DeleteUserHierarchyGroupRequest deleteUserHierarchyGroupRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteUserHierarchyGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteUserHierarchyGroup");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteUserHierarchyGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteUserHierarchyGroup")
.withMarshaller(new DeleteUserHierarchyGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteUserHierarchyGroupRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteUserHierarchyGroupRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the specified contact flow.
*
*
* You can also create and update contact flows using the Amazon Connect Flow language.
*
*
* @param describeContactFlowRequest
* @return A Java Future containing the result of the DescribeContactFlow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ContactFlowNotPublishedException The contact flow has not been published.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeContactFlow
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeContactFlow(
DescribeContactFlowRequest describeContactFlowRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeContactFlowRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeContactFlow");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeContactFlowResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeContactFlow")
.withMarshaller(new DescribeContactFlowRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeContactFlowRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeContactFlowRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Returns the current state of the specified instance identifier. It tracks the instance while it is being created
* and returns an error status if applicable.
*
*
* If an instance is not created successfully, the instance status reason field returns details relevant to the
* reason. The instance in a failed state is returned only for 24 hours after the CreateInstance API was invoked.
*
*
* @param describeInstanceRequest
* @return A Java Future containing the result of the DescribeInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeInstance
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeInstance(DescribeInstanceRequest describeInstanceRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeInstance");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeInstance")
.withMarshaller(new DescribeInstanceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeInstanceRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeInstanceRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Describes the specified instance attribute.
*
*
* @param describeInstanceAttributeRequest
* @return A Java Future containing the result of the DescribeInstanceAttribute operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeInstanceAttribute
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeInstanceAttribute(
DescribeInstanceAttributeRequest describeInstanceAttributeRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeInstanceAttributeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeInstanceAttribute");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeInstanceAttributeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeInstanceAttribute")
.withMarshaller(new DescribeInstanceAttributeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeInstanceAttributeRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeInstanceAttributeRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Retrieves the current storage configurations for the specified resource type, association ID, and instance ID.
*
*
* @param describeInstanceStorageConfigRequest
* @return A Java Future containing the result of the DescribeInstanceStorageConfig operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeInstanceStorageConfig
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeInstanceStorageConfig(
DescribeInstanceStorageConfigRequest describeInstanceStorageConfigRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeInstanceStorageConfigRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeInstanceStorageConfig");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeInstanceStorageConfigResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeInstanceStorageConfig")
.withMarshaller(new DescribeInstanceStorageConfigRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeInstanceStorageConfigRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeInstanceStorageConfigRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Describes the quick connect.
*
*
* @param describeQuickConnectRequest
* @return A Java Future containing the result of the DescribeQuickConnect operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeQuickConnect
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeQuickConnect(
DescribeQuickConnectRequest describeQuickConnectRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeQuickConnectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeQuickConnect");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeQuickConnectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeQuickConnect")
.withMarshaller(new DescribeQuickConnectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeQuickConnectRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeQuickConnectRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the specified routing profile.
*
*
* @param describeRoutingProfileRequest
* @return A Java Future containing the result of the DescribeRoutingProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeRoutingProfile
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeRoutingProfile(
DescribeRoutingProfileRequest describeRoutingProfileRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeRoutingProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeRoutingProfile");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeRoutingProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeRoutingProfile")
.withMarshaller(new DescribeRoutingProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeRoutingProfileRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeRoutingProfileRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the specified user account. You can find the instance ID in the console (it’s the final part of the
* ARN). The console does not display the user IDs. Instead, list the users and note the IDs provided in the output.
*
*
* @param describeUserRequest
* @return A Java Future containing the result of the DescribeUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeUser
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeUser(DescribeUserRequest describeUserRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeUser");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeUserResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeUser").withMarshaller(new DescribeUserRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeUserRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeUserRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the specified hierarchy group.
*
*
* @param describeUserHierarchyGroupRequest
* @return A Java Future containing the result of the DescribeUserHierarchyGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeUserHierarchyGroup
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeUserHierarchyGroup(
DescribeUserHierarchyGroupRequest describeUserHierarchyGroupRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeUserHierarchyGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeUserHierarchyGroup");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeUserHierarchyGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeUserHierarchyGroup")
.withMarshaller(new DescribeUserHierarchyGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeUserHierarchyGroupRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeUserHierarchyGroupRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the hierarchy structure of the specified Amazon Connect instance.
*
*
* @param describeUserHierarchyStructureRequest
* @return A Java Future containing the result of the DescribeUserHierarchyStructure operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeUserHierarchyStructure
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeUserHierarchyStructure(
DescribeUserHierarchyStructureRequest describeUserHierarchyStructureRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeUserHierarchyStructureRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeUserHierarchyStructure");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeUserHierarchyStructureResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeUserHierarchyStructure")
.withMarshaller(new DescribeUserHierarchyStructureRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeUserHierarchyStructureRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeUserHierarchyStructureRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Revokes access to integrated applications from Amazon Connect.
*
*
* @param disassociateApprovedOriginRequest
* @return A Java Future containing the result of the DisassociateApprovedOrigin operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateApprovedOrigin
* @see AWS API Documentation
*/
@Override
public CompletableFuture disassociateApprovedOrigin(
DisassociateApprovedOriginRequest disassociateApprovedOriginRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateApprovedOriginRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateApprovedOrigin");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateApprovedOriginResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateApprovedOrigin")
.withMarshaller(new DisassociateApprovedOriginRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(disassociateApprovedOriginRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = disassociateApprovedOriginRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Removes the storage type configurations for the specified resource type and association ID.
*
*
* @param disassociateInstanceStorageConfigRequest
* @return A Java Future containing the result of the DisassociateInstanceStorageConfig operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateInstanceStorageConfig
* @see AWS API Documentation
*/
@Override
public CompletableFuture disassociateInstanceStorageConfig(
DisassociateInstanceStorageConfigRequest disassociateInstanceStorageConfigRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
disassociateInstanceStorageConfigRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateInstanceStorageConfig");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, DisassociateInstanceStorageConfigResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateInstanceStorageConfig")
.withMarshaller(new DisassociateInstanceStorageConfigRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(disassociateInstanceStorageConfigRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = disassociateInstanceStorageConfigRequest
.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Remove the Lambda function from the drop-down options available in the relevant contact flow blocks.
*
*
* @param disassociateLambdaFunctionRequest
* @return A Java Future containing the result of the DisassociateLambdaFunction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateLambdaFunction
* @see AWS API Documentation
*/
@Override
public CompletableFuture disassociateLambdaFunction(
DisassociateLambdaFunctionRequest disassociateLambdaFunctionRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateLambdaFunctionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateLambdaFunction");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateLambdaFunctionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateLambdaFunction")
.withMarshaller(new DisassociateLambdaFunctionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(disassociateLambdaFunctionRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = disassociateLambdaFunctionRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Revokes authorization from the specified instance to access the specified Amazon Lex bot.
*
*
* @param disassociateLexBotRequest
* @return A Java Future containing the result of the DisassociateLexBot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateLexBot
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture disassociateLexBot(DisassociateLexBotRequest disassociateLexBotRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateLexBotRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateLexBot");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateLexBotResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateLexBot")
.withMarshaller(new DisassociateLexBotRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(disassociateLexBotRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = disassociateLexBotRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disassociates a set of queues from a routing profile.
*
*
* @param disassociateRoutingProfileQueuesRequest
* @return A Java Future containing the result of the DisassociateRoutingProfileQueues operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateRoutingProfileQueues
* @see AWS API Documentation
*/
@Override
public CompletableFuture disassociateRoutingProfileQueues(
DisassociateRoutingProfileQueuesRequest disassociateRoutingProfileQueuesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
disassociateRoutingProfileQueuesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateRoutingProfileQueues");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, DisassociateRoutingProfileQueuesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateRoutingProfileQueues")
.withMarshaller(new DisassociateRoutingProfileQueuesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(disassociateRoutingProfileQueuesRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = disassociateRoutingProfileQueuesRequest
.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Deletes the specified security key.
*
*
* @param disassociateSecurityKeyRequest
* @return A Java Future containing the result of the DisassociateSecurityKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateSecurityKey
* @see AWS API Documentation
*/
@Override
public CompletableFuture disassociateSecurityKey(
DisassociateSecurityKeyRequest disassociateSecurityKeyRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateSecurityKeyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateSecurityKey");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateSecurityKeyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateSecurityKey")
.withMarshaller(new DisassociateSecurityKeyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(disassociateSecurityKeyRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = disassociateSecurityKeyRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves the contact attributes for the specified contact.
*
*
* @param getContactAttributesRequest
* @return A Java Future containing the result of the GetContactAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed due to an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetContactAttributes
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture getContactAttributes(
GetContactAttributesRequest getContactAttributesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getContactAttributesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetContactAttributes");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetContactAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams