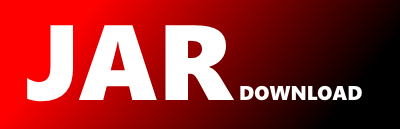
software.amazon.awssdk.services.connect.DefaultConnectClient Maven / Gradle / Ivy
Show all versions of connect Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.connect;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.connect.model.AccessDeniedException;
import software.amazon.awssdk.services.connect.model.AssociateApprovedOriginRequest;
import software.amazon.awssdk.services.connect.model.AssociateApprovedOriginResponse;
import software.amazon.awssdk.services.connect.model.AssociateBotRequest;
import software.amazon.awssdk.services.connect.model.AssociateBotResponse;
import software.amazon.awssdk.services.connect.model.AssociateDefaultVocabularyRequest;
import software.amazon.awssdk.services.connect.model.AssociateDefaultVocabularyResponse;
import software.amazon.awssdk.services.connect.model.AssociateInstanceStorageConfigRequest;
import software.amazon.awssdk.services.connect.model.AssociateInstanceStorageConfigResponse;
import software.amazon.awssdk.services.connect.model.AssociateLambdaFunctionRequest;
import software.amazon.awssdk.services.connect.model.AssociateLambdaFunctionResponse;
import software.amazon.awssdk.services.connect.model.AssociateLexBotRequest;
import software.amazon.awssdk.services.connect.model.AssociateLexBotResponse;
import software.amazon.awssdk.services.connect.model.AssociateQueueQuickConnectsRequest;
import software.amazon.awssdk.services.connect.model.AssociateQueueQuickConnectsResponse;
import software.amazon.awssdk.services.connect.model.AssociateRoutingProfileQueuesRequest;
import software.amazon.awssdk.services.connect.model.AssociateRoutingProfileQueuesResponse;
import software.amazon.awssdk.services.connect.model.AssociateSecurityKeyRequest;
import software.amazon.awssdk.services.connect.model.AssociateSecurityKeyResponse;
import software.amazon.awssdk.services.connect.model.ConnectException;
import software.amazon.awssdk.services.connect.model.ConnectRequest;
import software.amazon.awssdk.services.connect.model.ContactFlowNotPublishedException;
import software.amazon.awssdk.services.connect.model.ContactNotFoundException;
import software.amazon.awssdk.services.connect.model.CreateAgentStatusRequest;
import software.amazon.awssdk.services.connect.model.CreateAgentStatusResponse;
import software.amazon.awssdk.services.connect.model.CreateContactFlowModuleRequest;
import software.amazon.awssdk.services.connect.model.CreateContactFlowModuleResponse;
import software.amazon.awssdk.services.connect.model.CreateContactFlowRequest;
import software.amazon.awssdk.services.connect.model.CreateContactFlowResponse;
import software.amazon.awssdk.services.connect.model.CreateHoursOfOperationRequest;
import software.amazon.awssdk.services.connect.model.CreateHoursOfOperationResponse;
import software.amazon.awssdk.services.connect.model.CreateInstanceRequest;
import software.amazon.awssdk.services.connect.model.CreateInstanceResponse;
import software.amazon.awssdk.services.connect.model.CreateIntegrationAssociationRequest;
import software.amazon.awssdk.services.connect.model.CreateIntegrationAssociationResponse;
import software.amazon.awssdk.services.connect.model.CreateQueueRequest;
import software.amazon.awssdk.services.connect.model.CreateQueueResponse;
import software.amazon.awssdk.services.connect.model.CreateQuickConnectRequest;
import software.amazon.awssdk.services.connect.model.CreateQuickConnectResponse;
import software.amazon.awssdk.services.connect.model.CreateRoutingProfileRequest;
import software.amazon.awssdk.services.connect.model.CreateRoutingProfileResponse;
import software.amazon.awssdk.services.connect.model.CreateSecurityProfileRequest;
import software.amazon.awssdk.services.connect.model.CreateSecurityProfileResponse;
import software.amazon.awssdk.services.connect.model.CreateUseCaseRequest;
import software.amazon.awssdk.services.connect.model.CreateUseCaseResponse;
import software.amazon.awssdk.services.connect.model.CreateUserHierarchyGroupRequest;
import software.amazon.awssdk.services.connect.model.CreateUserHierarchyGroupResponse;
import software.amazon.awssdk.services.connect.model.CreateUserRequest;
import software.amazon.awssdk.services.connect.model.CreateUserResponse;
import software.amazon.awssdk.services.connect.model.CreateVocabularyRequest;
import software.amazon.awssdk.services.connect.model.CreateVocabularyResponse;
import software.amazon.awssdk.services.connect.model.DeleteContactFlowModuleRequest;
import software.amazon.awssdk.services.connect.model.DeleteContactFlowModuleResponse;
import software.amazon.awssdk.services.connect.model.DeleteContactFlowRequest;
import software.amazon.awssdk.services.connect.model.DeleteContactFlowResponse;
import software.amazon.awssdk.services.connect.model.DeleteHoursOfOperationRequest;
import software.amazon.awssdk.services.connect.model.DeleteHoursOfOperationResponse;
import software.amazon.awssdk.services.connect.model.DeleteInstanceRequest;
import software.amazon.awssdk.services.connect.model.DeleteInstanceResponse;
import software.amazon.awssdk.services.connect.model.DeleteIntegrationAssociationRequest;
import software.amazon.awssdk.services.connect.model.DeleteIntegrationAssociationResponse;
import software.amazon.awssdk.services.connect.model.DeleteQuickConnectRequest;
import software.amazon.awssdk.services.connect.model.DeleteQuickConnectResponse;
import software.amazon.awssdk.services.connect.model.DeleteSecurityProfileRequest;
import software.amazon.awssdk.services.connect.model.DeleteSecurityProfileResponse;
import software.amazon.awssdk.services.connect.model.DeleteUseCaseRequest;
import software.amazon.awssdk.services.connect.model.DeleteUseCaseResponse;
import software.amazon.awssdk.services.connect.model.DeleteUserHierarchyGroupRequest;
import software.amazon.awssdk.services.connect.model.DeleteUserHierarchyGroupResponse;
import software.amazon.awssdk.services.connect.model.DeleteUserRequest;
import software.amazon.awssdk.services.connect.model.DeleteUserResponse;
import software.amazon.awssdk.services.connect.model.DeleteVocabularyRequest;
import software.amazon.awssdk.services.connect.model.DeleteVocabularyResponse;
import software.amazon.awssdk.services.connect.model.DescribeAgentStatusRequest;
import software.amazon.awssdk.services.connect.model.DescribeAgentStatusResponse;
import software.amazon.awssdk.services.connect.model.DescribeContactFlowModuleRequest;
import software.amazon.awssdk.services.connect.model.DescribeContactFlowModuleResponse;
import software.amazon.awssdk.services.connect.model.DescribeContactFlowRequest;
import software.amazon.awssdk.services.connect.model.DescribeContactFlowResponse;
import software.amazon.awssdk.services.connect.model.DescribeContactRequest;
import software.amazon.awssdk.services.connect.model.DescribeContactResponse;
import software.amazon.awssdk.services.connect.model.DescribeHoursOfOperationRequest;
import software.amazon.awssdk.services.connect.model.DescribeHoursOfOperationResponse;
import software.amazon.awssdk.services.connect.model.DescribeInstanceAttributeRequest;
import software.amazon.awssdk.services.connect.model.DescribeInstanceAttributeResponse;
import software.amazon.awssdk.services.connect.model.DescribeInstanceRequest;
import software.amazon.awssdk.services.connect.model.DescribeInstanceResponse;
import software.amazon.awssdk.services.connect.model.DescribeInstanceStorageConfigRequest;
import software.amazon.awssdk.services.connect.model.DescribeInstanceStorageConfigResponse;
import software.amazon.awssdk.services.connect.model.DescribeQueueRequest;
import software.amazon.awssdk.services.connect.model.DescribeQueueResponse;
import software.amazon.awssdk.services.connect.model.DescribeQuickConnectRequest;
import software.amazon.awssdk.services.connect.model.DescribeQuickConnectResponse;
import software.amazon.awssdk.services.connect.model.DescribeRoutingProfileRequest;
import software.amazon.awssdk.services.connect.model.DescribeRoutingProfileResponse;
import software.amazon.awssdk.services.connect.model.DescribeSecurityProfileRequest;
import software.amazon.awssdk.services.connect.model.DescribeSecurityProfileResponse;
import software.amazon.awssdk.services.connect.model.DescribeUserHierarchyGroupRequest;
import software.amazon.awssdk.services.connect.model.DescribeUserHierarchyGroupResponse;
import software.amazon.awssdk.services.connect.model.DescribeUserHierarchyStructureRequest;
import software.amazon.awssdk.services.connect.model.DescribeUserHierarchyStructureResponse;
import software.amazon.awssdk.services.connect.model.DescribeUserRequest;
import software.amazon.awssdk.services.connect.model.DescribeUserResponse;
import software.amazon.awssdk.services.connect.model.DescribeVocabularyRequest;
import software.amazon.awssdk.services.connect.model.DescribeVocabularyResponse;
import software.amazon.awssdk.services.connect.model.DestinationNotAllowedException;
import software.amazon.awssdk.services.connect.model.DisassociateApprovedOriginRequest;
import software.amazon.awssdk.services.connect.model.DisassociateApprovedOriginResponse;
import software.amazon.awssdk.services.connect.model.DisassociateBotRequest;
import software.amazon.awssdk.services.connect.model.DisassociateBotResponse;
import software.amazon.awssdk.services.connect.model.DisassociateInstanceStorageConfigRequest;
import software.amazon.awssdk.services.connect.model.DisassociateInstanceStorageConfigResponse;
import software.amazon.awssdk.services.connect.model.DisassociateLambdaFunctionRequest;
import software.amazon.awssdk.services.connect.model.DisassociateLambdaFunctionResponse;
import software.amazon.awssdk.services.connect.model.DisassociateLexBotRequest;
import software.amazon.awssdk.services.connect.model.DisassociateLexBotResponse;
import software.amazon.awssdk.services.connect.model.DisassociateQueueQuickConnectsRequest;
import software.amazon.awssdk.services.connect.model.DisassociateQueueQuickConnectsResponse;
import software.amazon.awssdk.services.connect.model.DisassociateRoutingProfileQueuesRequest;
import software.amazon.awssdk.services.connect.model.DisassociateRoutingProfileQueuesResponse;
import software.amazon.awssdk.services.connect.model.DisassociateSecurityKeyRequest;
import software.amazon.awssdk.services.connect.model.DisassociateSecurityKeyResponse;
import software.amazon.awssdk.services.connect.model.DuplicateResourceException;
import software.amazon.awssdk.services.connect.model.GetContactAttributesRequest;
import software.amazon.awssdk.services.connect.model.GetContactAttributesResponse;
import software.amazon.awssdk.services.connect.model.GetCurrentMetricDataRequest;
import software.amazon.awssdk.services.connect.model.GetCurrentMetricDataResponse;
import software.amazon.awssdk.services.connect.model.GetFederationTokenRequest;
import software.amazon.awssdk.services.connect.model.GetFederationTokenResponse;
import software.amazon.awssdk.services.connect.model.GetMetricDataRequest;
import software.amazon.awssdk.services.connect.model.GetMetricDataResponse;
import software.amazon.awssdk.services.connect.model.IdempotencyException;
import software.amazon.awssdk.services.connect.model.InternalServiceException;
import software.amazon.awssdk.services.connect.model.InvalidContactFlowException;
import software.amazon.awssdk.services.connect.model.InvalidContactFlowModuleException;
import software.amazon.awssdk.services.connect.model.InvalidParameterException;
import software.amazon.awssdk.services.connect.model.InvalidRequestException;
import software.amazon.awssdk.services.connect.model.LimitExceededException;
import software.amazon.awssdk.services.connect.model.ListAgentStatusesRequest;
import software.amazon.awssdk.services.connect.model.ListAgentStatusesResponse;
import software.amazon.awssdk.services.connect.model.ListApprovedOriginsRequest;
import software.amazon.awssdk.services.connect.model.ListApprovedOriginsResponse;
import software.amazon.awssdk.services.connect.model.ListBotsRequest;
import software.amazon.awssdk.services.connect.model.ListBotsResponse;
import software.amazon.awssdk.services.connect.model.ListContactFlowModulesRequest;
import software.amazon.awssdk.services.connect.model.ListContactFlowModulesResponse;
import software.amazon.awssdk.services.connect.model.ListContactFlowsRequest;
import software.amazon.awssdk.services.connect.model.ListContactFlowsResponse;
import software.amazon.awssdk.services.connect.model.ListContactReferencesRequest;
import software.amazon.awssdk.services.connect.model.ListContactReferencesResponse;
import software.amazon.awssdk.services.connect.model.ListDefaultVocabulariesRequest;
import software.amazon.awssdk.services.connect.model.ListDefaultVocabulariesResponse;
import software.amazon.awssdk.services.connect.model.ListHoursOfOperationsRequest;
import software.amazon.awssdk.services.connect.model.ListHoursOfOperationsResponse;
import software.amazon.awssdk.services.connect.model.ListInstanceAttributesRequest;
import software.amazon.awssdk.services.connect.model.ListInstanceAttributesResponse;
import software.amazon.awssdk.services.connect.model.ListInstanceStorageConfigsRequest;
import software.amazon.awssdk.services.connect.model.ListInstanceStorageConfigsResponse;
import software.amazon.awssdk.services.connect.model.ListInstancesRequest;
import software.amazon.awssdk.services.connect.model.ListInstancesResponse;
import software.amazon.awssdk.services.connect.model.ListIntegrationAssociationsRequest;
import software.amazon.awssdk.services.connect.model.ListIntegrationAssociationsResponse;
import software.amazon.awssdk.services.connect.model.ListLambdaFunctionsRequest;
import software.amazon.awssdk.services.connect.model.ListLambdaFunctionsResponse;
import software.amazon.awssdk.services.connect.model.ListLexBotsRequest;
import software.amazon.awssdk.services.connect.model.ListLexBotsResponse;
import software.amazon.awssdk.services.connect.model.ListPhoneNumbersRequest;
import software.amazon.awssdk.services.connect.model.ListPhoneNumbersResponse;
import software.amazon.awssdk.services.connect.model.ListPromptsRequest;
import software.amazon.awssdk.services.connect.model.ListPromptsResponse;
import software.amazon.awssdk.services.connect.model.ListQueueQuickConnectsRequest;
import software.amazon.awssdk.services.connect.model.ListQueueQuickConnectsResponse;
import software.amazon.awssdk.services.connect.model.ListQueuesRequest;
import software.amazon.awssdk.services.connect.model.ListQueuesResponse;
import software.amazon.awssdk.services.connect.model.ListQuickConnectsRequest;
import software.amazon.awssdk.services.connect.model.ListQuickConnectsResponse;
import software.amazon.awssdk.services.connect.model.ListRoutingProfileQueuesRequest;
import software.amazon.awssdk.services.connect.model.ListRoutingProfileQueuesResponse;
import software.amazon.awssdk.services.connect.model.ListRoutingProfilesRequest;
import software.amazon.awssdk.services.connect.model.ListRoutingProfilesResponse;
import software.amazon.awssdk.services.connect.model.ListSecurityKeysRequest;
import software.amazon.awssdk.services.connect.model.ListSecurityKeysResponse;
import software.amazon.awssdk.services.connect.model.ListSecurityProfilePermissionsRequest;
import software.amazon.awssdk.services.connect.model.ListSecurityProfilePermissionsResponse;
import software.amazon.awssdk.services.connect.model.ListSecurityProfilesRequest;
import software.amazon.awssdk.services.connect.model.ListSecurityProfilesResponse;
import software.amazon.awssdk.services.connect.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.connect.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.connect.model.ListUseCasesRequest;
import software.amazon.awssdk.services.connect.model.ListUseCasesResponse;
import software.amazon.awssdk.services.connect.model.ListUserHierarchyGroupsRequest;
import software.amazon.awssdk.services.connect.model.ListUserHierarchyGroupsResponse;
import software.amazon.awssdk.services.connect.model.ListUsersRequest;
import software.amazon.awssdk.services.connect.model.ListUsersResponse;
import software.amazon.awssdk.services.connect.model.OutboundContactNotPermittedException;
import software.amazon.awssdk.services.connect.model.ResourceConflictException;
import software.amazon.awssdk.services.connect.model.ResourceInUseException;
import software.amazon.awssdk.services.connect.model.ResourceNotFoundException;
import software.amazon.awssdk.services.connect.model.ResumeContactRecordingRequest;
import software.amazon.awssdk.services.connect.model.ResumeContactRecordingResponse;
import software.amazon.awssdk.services.connect.model.SearchVocabulariesRequest;
import software.amazon.awssdk.services.connect.model.SearchVocabulariesResponse;
import software.amazon.awssdk.services.connect.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.connect.model.StartChatContactRequest;
import software.amazon.awssdk.services.connect.model.StartChatContactResponse;
import software.amazon.awssdk.services.connect.model.StartContactRecordingRequest;
import software.amazon.awssdk.services.connect.model.StartContactRecordingResponse;
import software.amazon.awssdk.services.connect.model.StartContactStreamingRequest;
import software.amazon.awssdk.services.connect.model.StartContactStreamingResponse;
import software.amazon.awssdk.services.connect.model.StartOutboundVoiceContactRequest;
import software.amazon.awssdk.services.connect.model.StartOutboundVoiceContactResponse;
import software.amazon.awssdk.services.connect.model.StartTaskContactRequest;
import software.amazon.awssdk.services.connect.model.StartTaskContactResponse;
import software.amazon.awssdk.services.connect.model.StopContactRecordingRequest;
import software.amazon.awssdk.services.connect.model.StopContactRecordingResponse;
import software.amazon.awssdk.services.connect.model.StopContactRequest;
import software.amazon.awssdk.services.connect.model.StopContactResponse;
import software.amazon.awssdk.services.connect.model.StopContactStreamingRequest;
import software.amazon.awssdk.services.connect.model.StopContactStreamingResponse;
import software.amazon.awssdk.services.connect.model.SuspendContactRecordingRequest;
import software.amazon.awssdk.services.connect.model.SuspendContactRecordingResponse;
import software.amazon.awssdk.services.connect.model.TagResourceRequest;
import software.amazon.awssdk.services.connect.model.TagResourceResponse;
import software.amazon.awssdk.services.connect.model.ThrottlingException;
import software.amazon.awssdk.services.connect.model.UntagResourceRequest;
import software.amazon.awssdk.services.connect.model.UntagResourceResponse;
import software.amazon.awssdk.services.connect.model.UpdateAgentStatusRequest;
import software.amazon.awssdk.services.connect.model.UpdateAgentStatusResponse;
import software.amazon.awssdk.services.connect.model.UpdateContactAttributesRequest;
import software.amazon.awssdk.services.connect.model.UpdateContactAttributesResponse;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowContentRequest;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowContentResponse;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowMetadataRequest;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowMetadataResponse;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowModuleContentRequest;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowModuleContentResponse;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowModuleMetadataRequest;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowModuleMetadataResponse;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowNameRequest;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowNameResponse;
import software.amazon.awssdk.services.connect.model.UpdateContactRequest;
import software.amazon.awssdk.services.connect.model.UpdateContactResponse;
import software.amazon.awssdk.services.connect.model.UpdateContactScheduleRequest;
import software.amazon.awssdk.services.connect.model.UpdateContactScheduleResponse;
import software.amazon.awssdk.services.connect.model.UpdateHoursOfOperationRequest;
import software.amazon.awssdk.services.connect.model.UpdateHoursOfOperationResponse;
import software.amazon.awssdk.services.connect.model.UpdateInstanceAttributeRequest;
import software.amazon.awssdk.services.connect.model.UpdateInstanceAttributeResponse;
import software.amazon.awssdk.services.connect.model.UpdateInstanceStorageConfigRequest;
import software.amazon.awssdk.services.connect.model.UpdateInstanceStorageConfigResponse;
import software.amazon.awssdk.services.connect.model.UpdateQueueHoursOfOperationRequest;
import software.amazon.awssdk.services.connect.model.UpdateQueueHoursOfOperationResponse;
import software.amazon.awssdk.services.connect.model.UpdateQueueMaxContactsRequest;
import software.amazon.awssdk.services.connect.model.UpdateQueueMaxContactsResponse;
import software.amazon.awssdk.services.connect.model.UpdateQueueNameRequest;
import software.amazon.awssdk.services.connect.model.UpdateQueueNameResponse;
import software.amazon.awssdk.services.connect.model.UpdateQueueOutboundCallerConfigRequest;
import software.amazon.awssdk.services.connect.model.UpdateQueueOutboundCallerConfigResponse;
import software.amazon.awssdk.services.connect.model.UpdateQueueStatusRequest;
import software.amazon.awssdk.services.connect.model.UpdateQueueStatusResponse;
import software.amazon.awssdk.services.connect.model.UpdateQuickConnectConfigRequest;
import software.amazon.awssdk.services.connect.model.UpdateQuickConnectConfigResponse;
import software.amazon.awssdk.services.connect.model.UpdateQuickConnectNameRequest;
import software.amazon.awssdk.services.connect.model.UpdateQuickConnectNameResponse;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileConcurrencyRequest;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileConcurrencyResponse;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileDefaultOutboundQueueRequest;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileDefaultOutboundQueueResponse;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileNameRequest;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileNameResponse;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileQueuesRequest;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileQueuesResponse;
import software.amazon.awssdk.services.connect.model.UpdateSecurityProfileRequest;
import software.amazon.awssdk.services.connect.model.UpdateSecurityProfileResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserHierarchyGroupNameRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserHierarchyGroupNameResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserHierarchyRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserHierarchyResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserHierarchyStructureRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserHierarchyStructureResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserIdentityInfoRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserIdentityInfoResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserPhoneConfigRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserPhoneConfigResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserRoutingProfileRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserRoutingProfileResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserSecurityProfilesRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserSecurityProfilesResponse;
import software.amazon.awssdk.services.connect.model.UserNotFoundException;
import software.amazon.awssdk.services.connect.paginators.GetCurrentMetricDataIterable;
import software.amazon.awssdk.services.connect.paginators.GetMetricDataIterable;
import software.amazon.awssdk.services.connect.paginators.ListAgentStatusesIterable;
import software.amazon.awssdk.services.connect.paginators.ListApprovedOriginsIterable;
import software.amazon.awssdk.services.connect.paginators.ListBotsIterable;
import software.amazon.awssdk.services.connect.paginators.ListContactFlowModulesIterable;
import software.amazon.awssdk.services.connect.paginators.ListContactFlowsIterable;
import software.amazon.awssdk.services.connect.paginators.ListContactReferencesIterable;
import software.amazon.awssdk.services.connect.paginators.ListDefaultVocabulariesIterable;
import software.amazon.awssdk.services.connect.paginators.ListHoursOfOperationsIterable;
import software.amazon.awssdk.services.connect.paginators.ListInstanceAttributesIterable;
import software.amazon.awssdk.services.connect.paginators.ListInstanceStorageConfigsIterable;
import software.amazon.awssdk.services.connect.paginators.ListInstancesIterable;
import software.amazon.awssdk.services.connect.paginators.ListIntegrationAssociationsIterable;
import software.amazon.awssdk.services.connect.paginators.ListLambdaFunctionsIterable;
import software.amazon.awssdk.services.connect.paginators.ListLexBotsIterable;
import software.amazon.awssdk.services.connect.paginators.ListPhoneNumbersIterable;
import software.amazon.awssdk.services.connect.paginators.ListPromptsIterable;
import software.amazon.awssdk.services.connect.paginators.ListQueueQuickConnectsIterable;
import software.amazon.awssdk.services.connect.paginators.ListQueuesIterable;
import software.amazon.awssdk.services.connect.paginators.ListQuickConnectsIterable;
import software.amazon.awssdk.services.connect.paginators.ListRoutingProfileQueuesIterable;
import software.amazon.awssdk.services.connect.paginators.ListRoutingProfilesIterable;
import software.amazon.awssdk.services.connect.paginators.ListSecurityKeysIterable;
import software.amazon.awssdk.services.connect.paginators.ListSecurityProfilePermissionsIterable;
import software.amazon.awssdk.services.connect.paginators.ListSecurityProfilesIterable;
import software.amazon.awssdk.services.connect.paginators.ListUseCasesIterable;
import software.amazon.awssdk.services.connect.paginators.ListUserHierarchyGroupsIterable;
import software.amazon.awssdk.services.connect.paginators.ListUsersIterable;
import software.amazon.awssdk.services.connect.paginators.SearchVocabulariesIterable;
import software.amazon.awssdk.services.connect.transform.AssociateApprovedOriginRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.AssociateBotRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.AssociateDefaultVocabularyRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.AssociateInstanceStorageConfigRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.AssociateLambdaFunctionRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.AssociateLexBotRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.AssociateQueueQuickConnectsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.AssociateRoutingProfileQueuesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.AssociateSecurityKeyRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateAgentStatusRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateContactFlowModuleRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateContactFlowRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateHoursOfOperationRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateInstanceRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateIntegrationAssociationRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateQueueRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateQuickConnectRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateRoutingProfileRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateSecurityProfileRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateUseCaseRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateUserHierarchyGroupRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateUserRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.CreateVocabularyRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DeleteContactFlowModuleRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DeleteContactFlowRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DeleteHoursOfOperationRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DeleteInstanceRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DeleteIntegrationAssociationRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DeleteQuickConnectRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DeleteSecurityProfileRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DeleteUseCaseRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DeleteUserHierarchyGroupRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DeleteUserRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DeleteVocabularyRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeAgentStatusRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeContactFlowModuleRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeContactFlowRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeContactRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeHoursOfOperationRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeInstanceAttributeRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeInstanceRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeInstanceStorageConfigRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeQueueRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeQuickConnectRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeRoutingProfileRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeSecurityProfileRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeUserHierarchyGroupRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeUserHierarchyStructureRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeUserRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DescribeVocabularyRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DisassociateApprovedOriginRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DisassociateBotRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DisassociateInstanceStorageConfigRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DisassociateLambdaFunctionRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DisassociateLexBotRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DisassociateQueueQuickConnectsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DisassociateRoutingProfileQueuesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.DisassociateSecurityKeyRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.GetContactAttributesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.GetCurrentMetricDataRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.GetFederationTokenRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.GetMetricDataRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListAgentStatusesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListApprovedOriginsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListBotsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListContactFlowModulesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListContactFlowsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListContactReferencesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListDefaultVocabulariesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListHoursOfOperationsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListInstanceAttributesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListInstanceStorageConfigsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListInstancesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListIntegrationAssociationsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListLambdaFunctionsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListLexBotsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListPhoneNumbersRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListPromptsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListQueueQuickConnectsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListQueuesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListQuickConnectsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListRoutingProfileQueuesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListRoutingProfilesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListSecurityKeysRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListSecurityProfilePermissionsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListSecurityProfilesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListUseCasesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListUserHierarchyGroupsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ListUsersRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.ResumeContactRecordingRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.SearchVocabulariesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.StartChatContactRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.StartContactRecordingRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.StartContactStreamingRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.StartOutboundVoiceContactRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.StartTaskContactRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.StopContactRecordingRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.StopContactRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.StopContactStreamingRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.SuspendContactRecordingRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateAgentStatusRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateContactAttributesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateContactFlowContentRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateContactFlowMetadataRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateContactFlowModuleContentRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateContactFlowModuleMetadataRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateContactFlowNameRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateContactRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateContactScheduleRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateHoursOfOperationRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateInstanceAttributeRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateInstanceStorageConfigRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateQueueHoursOfOperationRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateQueueMaxContactsRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateQueueNameRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateQueueOutboundCallerConfigRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateQueueStatusRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateQuickConnectConfigRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateQuickConnectNameRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateRoutingProfileConcurrencyRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateRoutingProfileDefaultOutboundQueueRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateRoutingProfileNameRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateRoutingProfileQueuesRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateSecurityProfileRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateUserHierarchyGroupNameRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateUserHierarchyRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateUserHierarchyStructureRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateUserIdentityInfoRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateUserPhoneConfigRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateUserRoutingProfileRequestMarshaller;
import software.amazon.awssdk.services.connect.transform.UpdateUserSecurityProfilesRequestMarshaller;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link ConnectClient}.
*
* @see ConnectClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultConnectClient implements ConnectClient {
private static final Logger log = Logger.loggerFor(DefaultConnectClient.class);
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultConnectClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Associates an approved origin to an Amazon Connect instance.
*
*
* @param associateApprovedOriginRequest
* @return Result of the AssociateApprovedOrigin operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceConflictException
* A resource already has that name.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.AssociateApprovedOrigin
* @see AWS API Documentation
*/
@Override
public AssociateApprovedOriginResponse associateApprovedOrigin(AssociateApprovedOriginRequest associateApprovedOriginRequest)
throws ResourceNotFoundException, ResourceConflictException, InternalServiceException, InvalidRequestException,
InvalidParameterException, ServiceQuotaExceededException, ThrottlingException, AwsServiceException,
SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateApprovedOriginResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateApprovedOriginRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateApprovedOrigin");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateApprovedOrigin").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(associateApprovedOriginRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateApprovedOriginRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Allows the specified Amazon Connect instance to access the specified Amazon Lex or Amazon Lex V2 bot.
*
*
* @param associateBotRequest
* @return Result of the AssociateBot operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceConflictException
* A resource already has that name.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws LimitExceededException
* The allowed limit for the resource has been exceeded.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.AssociateBot
* @see AWS API
* Documentation
*/
@Override
public AssociateBotResponse associateBot(AssociateBotRequest associateBotRequest) throws ResourceNotFoundException,
ResourceConflictException, InternalServiceException, InvalidRequestException, LimitExceededException,
ServiceQuotaExceededException, ThrottlingException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
AssociateBotResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateBotRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateBot");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("AssociateBot").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(associateBotRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateBotRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Associates an existing vocabulary as the default. Contact Lens for Amazon Connect uses the vocabulary in
* post-call and real-time analysis sessions for the given language.
*
*
* @param associateDefaultVocabularyRequest
* @return Result of the AssociateDefaultVocabulary operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.AssociateDefaultVocabulary
* @see AWS API Documentation
*/
@Override
public AssociateDefaultVocabularyResponse associateDefaultVocabulary(
AssociateDefaultVocabularyRequest associateDefaultVocabularyRequest) throws InvalidRequestException,
ResourceNotFoundException, InternalServiceException, ThrottlingException, AccessDeniedException, AwsServiceException,
SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateDefaultVocabularyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateDefaultVocabularyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateDefaultVocabulary");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateDefaultVocabulary").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(associateDefaultVocabularyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateDefaultVocabularyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Associates a storage resource type for the first time. You can only associate one type of storage configuration
* in a single call. This means, for example, that you can't define an instance with multiple S3 buckets for storing
* chat transcripts.
*
*
* This API does not create a resource that doesn't exist. It only associates it to the instance. Ensure that the
* resource being specified in the storage configuration, like an S3 bucket, exists when being used for association.
*
*
* @param associateInstanceStorageConfigRequest
* @return Result of the AssociateInstanceStorageConfig operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceConflictException
* A resource already has that name.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.AssociateInstanceStorageConfig
* @see AWS API Documentation
*/
@Override
public AssociateInstanceStorageConfigResponse associateInstanceStorageConfig(
AssociateInstanceStorageConfigRequest associateInstanceStorageConfigRequest) throws ResourceNotFoundException,
ResourceConflictException, InternalServiceException, InvalidRequestException, InvalidParameterException,
ThrottlingException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateInstanceStorageConfigResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
associateInstanceStorageConfigRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateInstanceStorageConfig");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateInstanceStorageConfig").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(associateInstanceStorageConfigRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateInstanceStorageConfigRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Allows the specified Amazon Connect instance to access the specified Lambda function.
*
*
* @param associateLambdaFunctionRequest
* @return Result of the AssociateLambdaFunction operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceConflictException
* A resource already has that name.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.AssociateLambdaFunction
* @see AWS API Documentation
*/
@Override
public AssociateLambdaFunctionResponse associateLambdaFunction(AssociateLambdaFunctionRequest associateLambdaFunctionRequest)
throws ResourceNotFoundException, ResourceConflictException, InternalServiceException, InvalidRequestException,
InvalidParameterException, ServiceQuotaExceededException, ThrottlingException, AwsServiceException,
SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateLambdaFunctionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateLambdaFunctionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateLambdaFunction");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateLambdaFunction").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(associateLambdaFunctionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateLambdaFunctionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Allows the specified Amazon Connect instance to access the specified Amazon Lex bot.
*
*
* @param associateLexBotRequest
* @return Result of the AssociateLexBot operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceConflictException
* A resource already has that name.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.AssociateLexBot
* @see AWS API
* Documentation
*/
@Override
public AssociateLexBotResponse associateLexBot(AssociateLexBotRequest associateLexBotRequest)
throws ResourceNotFoundException, ResourceConflictException, InternalServiceException, InvalidRequestException,
InvalidParameterException, ServiceQuotaExceededException, ThrottlingException, AwsServiceException,
SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
AssociateLexBotResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateLexBotRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateLexBot");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("AssociateLexBot").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(associateLexBotRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateLexBotRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Associates a set of quick connects with a queue.
*
*
* @param associateQueueQuickConnectsRequest
* @return Result of the AssociateQueueQuickConnects operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws LimitExceededException
* The allowed limit for the resource has been exceeded.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.AssociateQueueQuickConnects
* @see AWS API Documentation
*/
@Override
public AssociateQueueQuickConnectsResponse associateQueueQuickConnects(
AssociateQueueQuickConnectsRequest associateQueueQuickConnectsRequest) throws InvalidRequestException,
InvalidParameterException, ResourceNotFoundException, LimitExceededException, ThrottlingException,
InternalServiceException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateQueueQuickConnectsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateQueueQuickConnectsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateQueueQuickConnects");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateQueueQuickConnects").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(associateQueueQuickConnectsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateQueueQuickConnectsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Associates a set of queues with a routing profile.
*
*
* @param associateRoutingProfileQueuesRequest
* @return Result of the AssociateRoutingProfileQueues operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.AssociateRoutingProfileQueues
* @see AWS API Documentation
*/
@Override
public AssociateRoutingProfileQueuesResponse associateRoutingProfileQueues(
AssociateRoutingProfileQueuesRequest associateRoutingProfileQueuesRequest) throws InvalidRequestException,
InvalidParameterException, ResourceNotFoundException, ThrottlingException, InternalServiceException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateRoutingProfileQueuesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
associateRoutingProfileQueuesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateRoutingProfileQueues");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateRoutingProfileQueues").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(associateRoutingProfileQueuesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateRoutingProfileQueuesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Associates a security key to the instance.
*
*
* @param associateSecurityKeyRequest
* @return Result of the AssociateSecurityKey operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceConflictException
* A resource already has that name.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.AssociateSecurityKey
* @see AWS
* API Documentation
*/
@Override
public AssociateSecurityKeyResponse associateSecurityKey(AssociateSecurityKeyRequest associateSecurityKeyRequest)
throws ResourceNotFoundException, ResourceConflictException, InternalServiceException, InvalidRequestException,
InvalidParameterException, ServiceQuotaExceededException, ThrottlingException, AwsServiceException,
SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateSecurityKeyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateSecurityKeyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateSecurityKey");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("AssociateSecurityKey").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(associateSecurityKeyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateSecurityKeyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Creates an agent status for the specified Amazon Connect instance.
*
*
* @param createAgentStatusRequest
* @return Result of the CreateAgentStatus operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws DuplicateResourceException
* A resource with the specified name already exists.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws LimitExceededException
* The allowed limit for the resource has been exceeded.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.CreateAgentStatus
* @see AWS API
* Documentation
*/
@Override
public CreateAgentStatusResponse createAgentStatus(CreateAgentStatusRequest createAgentStatusRequest)
throws InvalidRequestException, InvalidParameterException, DuplicateResourceException, ResourceNotFoundException,
LimitExceededException, ThrottlingException, InternalServiceException, AwsServiceException, SdkClientException,
ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateAgentStatusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createAgentStatusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateAgentStatus");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateAgentStatus").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createAgentStatusRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateAgentStatusRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a contact flow for the specified Amazon Connect instance.
*
*
* You can also create and update contact flows using the Amazon Connect Flow language.
*
*
* @param createContactFlowRequest
* @return Result of the CreateContactFlow operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidContactFlowException
* The contact flow is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws DuplicateResourceException
* A resource with the specified name already exists.
* @throws LimitExceededException
* The allowed limit for the resource has been exceeded.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.CreateContactFlow
* @see AWS API
* Documentation
*/
@Override
public CreateContactFlowResponse createContactFlow(CreateContactFlowRequest createContactFlowRequest)
throws InvalidRequestException, InvalidContactFlowException, InvalidParameterException, DuplicateResourceException,
LimitExceededException, ResourceNotFoundException, ThrottlingException, InternalServiceException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateContactFlowResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createContactFlowRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateContactFlow");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateContactFlow").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createContactFlowRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateContactFlowRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a contact flow module for the specified Amazon Connect instance.
*
*
* @param createContactFlowModuleRequest
* @return Result of the CreateContactFlowModule operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidContactFlowModuleException
* The problems with the module. Please fix before trying again.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws DuplicateResourceException
* A resource with the specified name already exists.
* @throws LimitExceededException
* The allowed limit for the resource has been exceeded.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws IdempotencyException
* An entity with the same name already exists.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.CreateContactFlowModule
* @see AWS API Documentation
*/
@Override
public CreateContactFlowModuleResponse createContactFlowModule(CreateContactFlowModuleRequest createContactFlowModuleRequest)
throws AccessDeniedException, InvalidRequestException, InvalidContactFlowModuleException, InvalidParameterException,
DuplicateResourceException, LimitExceededException, ResourceNotFoundException, ThrottlingException,
IdempotencyException, InternalServiceException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateContactFlowModuleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createContactFlowModuleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateContactFlowModule");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateContactFlowModule").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createContactFlowModuleRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateContactFlowModuleRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Creates hours of operation.
*
*
* @param createHoursOfOperationRequest
* @return Result of the CreateHoursOfOperation operation returned by the service.
* @throws DuplicateResourceException
* A resource with the specified name already exists.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws LimitExceededException
* The allowed limit for the resource has been exceeded.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.CreateHoursOfOperation
* @see AWS API Documentation
*/
@Override
public CreateHoursOfOperationResponse createHoursOfOperation(CreateHoursOfOperationRequest createHoursOfOperationRequest)
throws DuplicateResourceException, InvalidRequestException, InvalidParameterException, ResourceNotFoundException,
LimitExceededException, ThrottlingException, InternalServiceException, AwsServiceException, SdkClientException,
ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateHoursOfOperationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createHoursOfOperationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateHoursOfOperation");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateHoursOfOperation").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createHoursOfOperationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateHoursOfOperationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Initiates an Amazon Connect instance with all the supported channels enabled. It does not attach any storage,
* such as Amazon Simple Storage Service (Amazon S3) or Amazon Kinesis. It also does not allow for any
* configurations on features, such as Contact Lens for Amazon Connect.
*
*
* Amazon Connect enforces a limit on the total number of instances that you can create or delete in 30 days. If you
* exceed this limit, you will get an error message indicating there has been an excessive number of attempts at
* creating or deleting instances. You must wait 30 days before you can restart creating and deleting instances in
* your account.
*
*
* @param createInstanceRequest
* @return Result of the CreateInstance operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.CreateInstance
* @see AWS API
* Documentation
*/
@Override
public CreateInstanceResponse createInstance(CreateInstanceRequest createInstanceRequest) throws InvalidRequestException,
ServiceQuotaExceededException, ThrottlingException, ResourceNotFoundException, InternalServiceException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateInstance");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateInstance").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createInstanceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateInstanceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates an Amazon Web Services resource association with an Amazon Connect instance.
*
*
* @param createIntegrationAssociationRequest
* @return Result of the CreateIntegrationAssociation operation returned by the service.
* @throws DuplicateResourceException
* A resource with the specified name already exists.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.CreateIntegrationAssociation
* @see AWS API Documentation
*/
@Override
public CreateIntegrationAssociationResponse createIntegrationAssociation(
CreateIntegrationAssociationRequest createIntegrationAssociationRequest) throws DuplicateResourceException,
ResourceNotFoundException, InternalServiceException, InvalidRequestException, ThrottlingException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateIntegrationAssociationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createIntegrationAssociationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateIntegrationAssociation");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateIntegrationAssociation").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createIntegrationAssociationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateIntegrationAssociationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Creates a new queue for the specified Amazon Connect instance.
*
*
* @param createQueueRequest
* @return Result of the CreateQueue operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws DuplicateResourceException
* A resource with the specified name already exists.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws LimitExceededException
* The allowed limit for the resource has been exceeded.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.CreateQueue
* @see AWS API
* Documentation
*/
@Override
public CreateQueueResponse createQueue(CreateQueueRequest createQueueRequest) throws InvalidRequestException,
InvalidParameterException, DuplicateResourceException, ResourceNotFoundException, LimitExceededException,
ThrottlingException, InternalServiceException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateQueueResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createQueueRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateQueue");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateQueue").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createQueueRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateQueueRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a quick connect for the specified Amazon Connect instance.
*
*
* @param createQuickConnectRequest
* @return Result of the CreateQuickConnect operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws DuplicateResourceException
* A resource with the specified name already exists.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws LimitExceededException
* The allowed limit for the resource has been exceeded.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.CreateQuickConnect
* @see AWS
* API Documentation
*/
@Override
public CreateQuickConnectResponse createQuickConnect(CreateQuickConnectRequest createQuickConnectRequest)
throws InvalidRequestException, InvalidParameterException, DuplicateResourceException, ResourceNotFoundException,
LimitExceededException, ThrottlingException, InternalServiceException, AwsServiceException, SdkClientException,
ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateQuickConnectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createQuickConnectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateQuickConnect");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateQuickConnect").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createQuickConnectRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateQuickConnectRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a new routing profile.
*
*
* @param createRoutingProfileRequest
* @return Result of the CreateRoutingProfile operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws DuplicateResourceException
* A resource with the specified name already exists.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws LimitExceededException
* The allowed limit for the resource has been exceeded.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.CreateRoutingProfile
* @see AWS
* API Documentation
*/
@Override
public CreateRoutingProfileResponse createRoutingProfile(CreateRoutingProfileRequest createRoutingProfileRequest)
throws InvalidRequestException, InvalidParameterException, DuplicateResourceException, ResourceNotFoundException,
LimitExceededException, ThrottlingException, InternalServiceException, AwsServiceException, SdkClientException,
ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateRoutingProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createRoutingProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateRoutingProfile");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateRoutingProfile").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createRoutingProfileRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateRoutingProfileRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Creates a security profile.
*
*
* @param createSecurityProfileRequest
* @return Result of the CreateSecurityProfile operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws LimitExceededException
* The allowed limit for the resource has been exceeded.
* @throws DuplicateResourceException
* A resource with the specified name already exists.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.CreateSecurityProfile
* @see AWS
* API Documentation
*/
@Override
public CreateSecurityProfileResponse createSecurityProfile(CreateSecurityProfileRequest createSecurityProfileRequest)
throws InvalidRequestException, InvalidParameterException, LimitExceededException, DuplicateResourceException,
ResourceNotFoundException, ThrottlingException, InternalServiceException, AwsServiceException, SdkClientException,
ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateSecurityProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createSecurityProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateSecurityProfile");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateSecurityProfile").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createSecurityProfileRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateSecurityProfileRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a use case for an integration association.
*
*
* @param createUseCaseRequest
* @return Result of the CreateUseCase operation returned by the service.
* @throws DuplicateResourceException
* A resource with the specified name already exists.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.CreateUseCase
* @see AWS API
* Documentation
*/
@Override
public CreateUseCaseResponse createUseCase(CreateUseCaseRequest createUseCaseRequest) throws DuplicateResourceException,
ResourceNotFoundException, InternalServiceException, InvalidRequestException, ThrottlingException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateUseCaseResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createUseCaseRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateUseCase");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateUseCase").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createUseCaseRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateUseCaseRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a user account for the specified Amazon Connect instance.
*
*
* For information about how to create user accounts using the Amazon Connect console, see Add Users in the Amazon
* Connect Administrator Guide.
*
*
* @param createUserRequest
* @return Result of the CreateUser operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws LimitExceededException
* The allowed limit for the resource has been exceeded.
* @throws DuplicateResourceException
* A resource with the specified name already exists.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.CreateUser
* @see AWS API
* Documentation
*/
@Override
public CreateUserResponse createUser(CreateUserRequest createUserRequest) throws InvalidRequestException,
InvalidParameterException, LimitExceededException, DuplicateResourceException, ResourceNotFoundException,
ThrottlingException, InternalServiceException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateUserResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateUser");
return clientHandler
.execute(new ClientExecutionParams().withOperationName("CreateUser")
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(createUserRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateUserRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a new user hierarchy group.
*
*
* @param createUserHierarchyGroupRequest
* @return Result of the CreateUserHierarchyGroup operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws DuplicateResourceException
* A resource with the specified name already exists.
* @throws LimitExceededException
* The allowed limit for the resource has been exceeded.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.CreateUserHierarchyGroup
* @see AWS API Documentation
*/
@Override
public CreateUserHierarchyGroupResponse createUserHierarchyGroup(
CreateUserHierarchyGroupRequest createUserHierarchyGroupRequest) throws InvalidRequestException,
InvalidParameterException, DuplicateResourceException, LimitExceededException, ResourceNotFoundException,
ThrottlingException, InternalServiceException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateUserHierarchyGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createUserHierarchyGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateUserHierarchyGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateUserHierarchyGroup").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createUserHierarchyGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateUserHierarchyGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a custom vocabulary associated with your Amazon Connect instance. You can set a custom vocabulary to be
* your default vocabulary for a given language. Contact Lens for Amazon Connect uses the default vocabulary in
* post-call and real-time contact analysis sessions for that language.
*
*
* @param createVocabularyRequest
* @return Result of the CreateVocabulary operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws ResourceConflictException
* A resource already has that name.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.CreateVocabulary
* @see AWS API
* Documentation
*/
@Override
public CreateVocabularyResponse createVocabulary(CreateVocabularyRequest createVocabularyRequest)
throws InvalidRequestException, ResourceNotFoundException, InternalServiceException, ThrottlingException,
AccessDeniedException, ResourceConflictException, ServiceQuotaExceededException, AwsServiceException,
SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateVocabularyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createVocabularyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateVocabulary");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateVocabulary").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createVocabularyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateVocabularyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a contact flow for the specified Amazon Connect instance.
*
*
* @param deleteContactFlowRequest
* @return Result of the DeleteContactFlow operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DeleteContactFlow
* @see AWS API
* Documentation
*/
@Override
public DeleteContactFlowResponse deleteContactFlow(DeleteContactFlowRequest deleteContactFlowRequest)
throws AccessDeniedException, InvalidRequestException, InvalidParameterException, ResourceNotFoundException,
InternalServiceException, ThrottlingException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteContactFlowResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteContactFlowRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteContactFlow");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteContactFlow").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteContactFlowRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteContactFlowRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the specified contact flow module.
*
*
* @param deleteContactFlowModuleRequest
* @return Result of the DeleteContactFlowModule operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DeleteContactFlowModule
* @see AWS API Documentation
*/
@Override
public DeleteContactFlowModuleResponse deleteContactFlowModule(DeleteContactFlowModuleRequest deleteContactFlowModuleRequest)
throws AccessDeniedException, InvalidRequestException, InvalidParameterException, ResourceNotFoundException,
ThrottlingException, InternalServiceException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteContactFlowModuleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteContactFlowModuleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteContactFlowModule");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteContactFlowModule").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteContactFlowModuleRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteContactFlowModuleRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Deletes an hours of operation.
*
*
* @param deleteHoursOfOperationRequest
* @return Result of the DeleteHoursOfOperation operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DeleteHoursOfOperation
* @see AWS API Documentation
*/
@Override
public DeleteHoursOfOperationResponse deleteHoursOfOperation(DeleteHoursOfOperationRequest deleteHoursOfOperationRequest)
throws InvalidRequestException, InvalidParameterException, ResourceNotFoundException, ThrottlingException,
InternalServiceException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteHoursOfOperationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteHoursOfOperationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteHoursOfOperation");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteHoursOfOperation").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteHoursOfOperationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteHoursOfOperationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Deletes the Amazon Connect instance.
*
*
* Amazon Connect enforces a limit on the total number of instances that you can create or delete in 30 days. If you
* exceed this limit, you will get an error message indicating there has been an excessive number of attempts at
* creating or deleting instances. You must wait 30 days before you can restart creating and deleting instances in
* your account.
*
*
* @param deleteInstanceRequest
* @return Result of the DeleteInstance operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DeleteInstance
* @see AWS API
* Documentation
*/
@Override
public DeleteInstanceResponse deleteInstance(DeleteInstanceRequest deleteInstanceRequest) throws ResourceNotFoundException,
InternalServiceException, InvalidRequestException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteInstance");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteInstance").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteInstanceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteInstanceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes an Amazon Web Services resource association from an Amazon Connect instance. The association must not
* have any use cases associated with it.
*
*
* @param deleteIntegrationAssociationRequest
* @return Result of the DeleteIntegrationAssociation operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DeleteIntegrationAssociation
* @see AWS API Documentation
*/
@Override
public DeleteIntegrationAssociationResponse deleteIntegrationAssociation(
DeleteIntegrationAssociationRequest deleteIntegrationAssociationRequest) throws ResourceNotFoundException,
InternalServiceException, InvalidRequestException, ThrottlingException, AwsServiceException, SdkClientException,
ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteIntegrationAssociationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteIntegrationAssociationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteIntegrationAssociation");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteIntegrationAssociation").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteIntegrationAssociationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteIntegrationAssociationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a quick connect.
*
*
* @param deleteQuickConnectRequest
* @return Result of the DeleteQuickConnect operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DeleteQuickConnect
* @see AWS
* API Documentation
*/
@Override
public DeleteQuickConnectResponse deleteQuickConnect(DeleteQuickConnectRequest deleteQuickConnectRequest)
throws InvalidRequestException, InvalidParameterException, ResourceNotFoundException, ThrottlingException,
InternalServiceException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteQuickConnectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteQuickConnectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteQuickConnect");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteQuickConnect").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteQuickConnectRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteQuickConnectRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Deletes a security profile.
*
*
* @param deleteSecurityProfileRequest
* @return Result of the DeleteSecurityProfile operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws ResourceInUseException
* That resource is already in use. Please try another.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DeleteSecurityProfile
* @see AWS
* API Documentation
*/
@Override
public DeleteSecurityProfileResponse deleteSecurityProfile(DeleteSecurityProfileRequest deleteSecurityProfileRequest)
throws InvalidRequestException, InvalidParameterException, ResourceNotFoundException, ThrottlingException,
InternalServiceException, AccessDeniedException, ResourceInUseException, AwsServiceException, SdkClientException,
ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteSecurityProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteSecurityProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteSecurityProfile");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteSecurityProfile").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteSecurityProfileRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteSecurityProfileRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a use case from an integration association.
*
*
* @param deleteUseCaseRequest
* @return Result of the DeleteUseCase operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DeleteUseCase
* @see AWS API
* Documentation
*/
@Override
public DeleteUseCaseResponse deleteUseCase(DeleteUseCaseRequest deleteUseCaseRequest) throws ResourceNotFoundException,
InternalServiceException, InvalidRequestException, ThrottlingException, AwsServiceException, SdkClientException,
ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteUseCaseResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteUseCaseRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteUseCase");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteUseCase").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteUseCaseRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteUseCaseRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a user account from the specified Amazon Connect instance.
*
*
* For information about what happens to a user's data when their account is deleted, see Delete Users from Your Amazon
* Connect Instance in the Amazon Connect Administrator Guide.
*
*
* @param deleteUserRequest
* @return Result of the DeleteUser operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DeleteUser
* @see AWS API
* Documentation
*/
@Override
public DeleteUserResponse deleteUser(DeleteUserRequest deleteUserRequest) throws InvalidRequestException,
InvalidParameterException, ResourceNotFoundException, ThrottlingException, InternalServiceException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteUserResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteUser");
return clientHandler
.execute(new ClientExecutionParams().withOperationName("DeleteUser")
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteUserRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteUserRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes an existing user hierarchy group. It must not be associated with any agents or have any active child
* groups.
*
*
* @param deleteUserHierarchyGroupRequest
* @return Result of the DeleteUserHierarchyGroup operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceInUseException
* That resource is already in use. Please try another.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DeleteUserHierarchyGroup
* @see AWS API Documentation
*/
@Override
public DeleteUserHierarchyGroupResponse deleteUserHierarchyGroup(
DeleteUserHierarchyGroupRequest deleteUserHierarchyGroupRequest) throws InvalidRequestException,
InvalidParameterException, ResourceNotFoundException, ResourceInUseException, ThrottlingException,
InternalServiceException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteUserHierarchyGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteUserHierarchyGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteUserHierarchyGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteUserHierarchyGroup").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteUserHierarchyGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteUserHierarchyGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the vocabulary that has the given identifier.
*
*
* @param deleteVocabularyRequest
* @return Result of the DeleteVocabulary operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws ResourceInUseException
* That resource is already in use. Please try another.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DeleteVocabulary
* @see AWS API
* Documentation
*/
@Override
public DeleteVocabularyResponse deleteVocabulary(DeleteVocabularyRequest deleteVocabularyRequest)
throws InvalidRequestException, ResourceNotFoundException, InternalServiceException, ThrottlingException,
AccessDeniedException, ResourceInUseException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteVocabularyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteVocabularyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteVocabulary");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteVocabulary").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteVocabularyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteVocabularyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Describes an agent status.
*
*
* @param describeAgentStatusRequest
* @return Result of the DescribeAgentStatus operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DescribeAgentStatus
* @see AWS
* API Documentation
*/
@Override
public DescribeAgentStatusResponse describeAgentStatus(DescribeAgentStatusRequest describeAgentStatusRequest)
throws InvalidRequestException, InvalidParameterException, ResourceNotFoundException, ThrottlingException,
InternalServiceException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeAgentStatusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeAgentStatusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeAgentStatus");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeAgentStatus").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeAgentStatusRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeAgentStatusRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Describes the specified contact.
*
*
*
* Contact information remains available in Amazon Connect for 24 months, and then it is deleted.
*
*
*
* @param describeContactRequest
* @return Result of the DescribeContact operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DescribeContact
* @see AWS API
* Documentation
*/
@Override
public DescribeContactResponse describeContact(DescribeContactRequest describeContactRequest) throws InvalidRequestException,
InvalidParameterException, ResourceNotFoundException, InternalServiceException, ThrottlingException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeContactResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeContactRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeContact");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeContact").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeContactRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeContactRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the specified contact flow.
*
*
* You can also create and update contact flows using the Amazon Connect Flow language.
*
*
* @param describeContactFlowRequest
* @return Result of the DescribeContactFlow operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ContactFlowNotPublishedException
* The contact flow has not been published.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DescribeContactFlow
* @see AWS
* API Documentation
*/
@Override
public DescribeContactFlowResponse describeContactFlow(DescribeContactFlowRequest describeContactFlowRequest)
throws InvalidRequestException, InvalidParameterException, ResourceNotFoundException,
ContactFlowNotPublishedException, ThrottlingException, InternalServiceException, AwsServiceException,
SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeContactFlowResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeContactFlowRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeContactFlow");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeContactFlow").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeContactFlowRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeContactFlowRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the specified contact flow module.
*
*
* @param describeContactFlowModuleRequest
* @return Result of the DescribeContactFlowModule operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DescribeContactFlowModule
* @see AWS API Documentation
*/
@Override
public DescribeContactFlowModuleResponse describeContactFlowModule(
DescribeContactFlowModuleRequest describeContactFlowModuleRequest) throws AccessDeniedException,
InvalidRequestException, InvalidParameterException, ResourceNotFoundException, ThrottlingException,
InternalServiceException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeContactFlowModuleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeContactFlowModuleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeContactFlowModule");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeContactFlowModule").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeContactFlowModuleRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeContactFlowModuleRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Describes the hours of operation.
*
*
* @param describeHoursOfOperationRequest
* @return Result of the DescribeHoursOfOperation operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DescribeHoursOfOperation
* @see AWS API Documentation
*/
@Override
public DescribeHoursOfOperationResponse describeHoursOfOperation(
DescribeHoursOfOperationRequest describeHoursOfOperationRequest) throws InvalidRequestException,
InvalidParameterException, ResourceNotFoundException, ThrottlingException, InternalServiceException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeHoursOfOperationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeHoursOfOperationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeHoursOfOperation");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeHoursOfOperation").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeHoursOfOperationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeHoursOfOperationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Returns the current state of the specified instance identifier. It tracks the instance while it is being created
* and returns an error status, if applicable.
*
*
* If an instance is not created successfully, the instance status reason field returns details relevant to the
* reason. The instance in a failed state is returned only for 24 hours after the CreateInstance API was invoked.
*
*
* @param describeInstanceRequest
* @return Result of the DescribeInstance operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DescribeInstance
* @see AWS API
* Documentation
*/
@Override
public DescribeInstanceResponse describeInstance(DescribeInstanceRequest describeInstanceRequest)
throws InvalidRequestException, ResourceNotFoundException, InternalServiceException, AwsServiceException,
SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeInstance");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeInstance").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeInstanceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeInstanceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Describes the specified instance attribute.
*
*
* @param describeInstanceAttributeRequest
* @return Result of the DescribeInstanceAttribute operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DescribeInstanceAttribute
* @see AWS API Documentation
*/
@Override
public DescribeInstanceAttributeResponse describeInstanceAttribute(
DescribeInstanceAttributeRequest describeInstanceAttributeRequest) throws ResourceNotFoundException,
InternalServiceException, InvalidRequestException, InvalidParameterException, ThrottlingException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeInstanceAttributeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeInstanceAttributeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeInstanceAttribute");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeInstanceAttribute").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeInstanceAttributeRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeInstanceAttributeRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Retrieves the current storage configurations for the specified resource type, association ID, and instance ID.
*
*
* @param describeInstanceStorageConfigRequest
* @return Result of the DescribeInstanceStorageConfig operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DescribeInstanceStorageConfig
* @see AWS API Documentation
*/
@Override
public DescribeInstanceStorageConfigResponse describeInstanceStorageConfig(
DescribeInstanceStorageConfigRequest describeInstanceStorageConfigRequest) throws ResourceNotFoundException,
InternalServiceException, InvalidRequestException, InvalidParameterException, ThrottlingException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeInstanceStorageConfigResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeInstanceStorageConfigRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeInstanceStorageConfig");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeInstanceStorageConfig").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeInstanceStorageConfigRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeInstanceStorageConfigRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Describes the specified queue.
*
*
* @param describeQueueRequest
* @return Result of the DescribeQueue operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DescribeQueue
* @see AWS API
* Documentation
*/
@Override
public DescribeQueueResponse describeQueue(DescribeQueueRequest describeQueueRequest) throws InvalidRequestException,
InvalidParameterException, ResourceNotFoundException, ThrottlingException, InternalServiceException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeQueueResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeQueueRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeQueue");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeQueue").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeQueueRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeQueueRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the quick connect.
*
*
* @param describeQuickConnectRequest
* @return Result of the DescribeQuickConnect operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DescribeQuickConnect
* @see AWS
* API Documentation
*/
@Override
public DescribeQuickConnectResponse describeQuickConnect(DescribeQuickConnectRequest describeQuickConnectRequest)
throws InvalidRequestException, InvalidParameterException, ResourceNotFoundException, ThrottlingException,
InternalServiceException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeQuickConnectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeQuickConnectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeQuickConnect");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeQuickConnect").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeQuickConnectRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeQuickConnectRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the specified routing profile.
*
*
* @param describeRoutingProfileRequest
* @return Result of the DescribeRoutingProfile operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DescribeRoutingProfile
* @see AWS API Documentation
*/
@Override
public DescribeRoutingProfileResponse describeRoutingProfile(DescribeRoutingProfileRequest describeRoutingProfileRequest)
throws InvalidRequestException, InvalidParameterException, ResourceNotFoundException, ThrottlingException,
InternalServiceException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeRoutingProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeRoutingProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeRoutingProfile");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeRoutingProfile").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeRoutingProfileRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeRoutingProfileRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Gets basic information about the security profle.
*
*
* @param describeSecurityProfileRequest
* @return Result of the DescribeSecurityProfile operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DescribeSecurityProfile
* @see AWS API Documentation
*/
@Override
public DescribeSecurityProfileResponse describeSecurityProfile(DescribeSecurityProfileRequest describeSecurityProfileRequest)
throws InvalidRequestException, InvalidParameterException, ResourceNotFoundException, ThrottlingException,
InternalServiceException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeSecurityProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeSecurityProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeSecurityProfile");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeSecurityProfile").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeSecurityProfileRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeSecurityProfileRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the specified user account. You can find the instance ID in the console (it’s the final part of the
* ARN). The console does not display the user IDs. Instead, list the users and note the IDs provided in the output.
*
*
* @param describeUserRequest
* @return Result of the DescribeUser operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DescribeUser
* @see AWS API
* Documentation
*/
@Override
public DescribeUserResponse describeUser(DescribeUserRequest describeUserRequest) throws InvalidRequestException,
InvalidParameterException, ResourceNotFoundException, ThrottlingException, InternalServiceException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeUserResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeUser");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeUser").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeUserRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeUserRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the specified hierarchy group.
*
*
* @param describeUserHierarchyGroupRequest
* @return Result of the DescribeUserHierarchyGroup operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DescribeUserHierarchyGroup
* @see AWS API Documentation
*/
@Override
public DescribeUserHierarchyGroupResponse describeUserHierarchyGroup(
DescribeUserHierarchyGroupRequest describeUserHierarchyGroupRequest) throws InvalidRequestException,
InvalidParameterException, ResourceNotFoundException, ThrottlingException, InternalServiceException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeUserHierarchyGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeUserHierarchyGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeUserHierarchyGroup");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeUserHierarchyGroup").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeUserHierarchyGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeUserHierarchyGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the hierarchy structure of the specified Amazon Connect instance.
*
*
* @param describeUserHierarchyStructureRequest
* @return Result of the DescribeUserHierarchyStructure operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DescribeUserHierarchyStructure
* @see AWS API Documentation
*/
@Override
public DescribeUserHierarchyStructureResponse describeUserHierarchyStructure(
DescribeUserHierarchyStructureRequest describeUserHierarchyStructureRequest) throws InvalidRequestException,
InvalidParameterException, ResourceNotFoundException, ThrottlingException, InternalServiceException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeUserHierarchyStructureResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeUserHierarchyStructureRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeUserHierarchyStructure");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeUserHierarchyStructure").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeUserHierarchyStructureRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeUserHierarchyStructureRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the specified vocabulary.
*
*
* @param describeVocabularyRequest
* @return Result of the DescribeVocabulary operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DescribeVocabulary
* @see AWS
* API Documentation
*/
@Override
public DescribeVocabularyResponse describeVocabulary(DescribeVocabularyRequest describeVocabularyRequest)
throws InvalidRequestException, ResourceNotFoundException, InternalServiceException, ThrottlingException,
AccessDeniedException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeVocabularyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeVocabularyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeVocabulary");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeVocabulary").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeVocabularyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeVocabularyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Revokes access to integrated applications from Amazon Connect.
*
*
* @param disassociateApprovedOriginRequest
* @return Result of the DisassociateApprovedOrigin operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DisassociateApprovedOrigin
* @see AWS API Documentation
*/
@Override
public DisassociateApprovedOriginResponse disassociateApprovedOrigin(
DisassociateApprovedOriginRequest disassociateApprovedOriginRequest) throws ResourceNotFoundException,
InternalServiceException, InvalidRequestException, InvalidParameterException, ThrottlingException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateApprovedOriginResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateApprovedOriginRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateApprovedOrigin");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateApprovedOrigin").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(disassociateApprovedOriginRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateApprovedOriginRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Revokes authorization from the specified instance to access the specified Amazon Lex or Amazon Lex V2 bot.
*
*
* @param disassociateBotRequest
* @return Result of the DisassociateBot operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DisassociateBot
* @see AWS API
* Documentation
*/
@Override
public DisassociateBotResponse disassociateBot(DisassociateBotRequest disassociateBotRequest)
throws ResourceNotFoundException, InternalServiceException, InvalidRequestException, ThrottlingException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DisassociateBotResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateBotRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateBot");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DisassociateBot").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(disassociateBotRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateBotRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Removes the storage type configurations for the specified resource type and association ID.
*
*
* @param disassociateInstanceStorageConfigRequest
* @return Result of the DisassociateInstanceStorageConfig operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DisassociateInstanceStorageConfig
* @see AWS API Documentation
*/
@Override
public DisassociateInstanceStorageConfigResponse disassociateInstanceStorageConfig(
DisassociateInstanceStorageConfigRequest disassociateInstanceStorageConfigRequest) throws ResourceNotFoundException,
InternalServiceException, InvalidRequestException, InvalidParameterException, ThrottlingException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateInstanceStorageConfigResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
disassociateInstanceStorageConfigRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateInstanceStorageConfig");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateInstanceStorageConfig").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(disassociateInstanceStorageConfigRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateInstanceStorageConfigRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Remove the Lambda function from the dropdown options available in the relevant contact flow blocks.
*
*
* @param disassociateLambdaFunctionRequest
* @return Result of the DisassociateLambdaFunction operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DisassociateLambdaFunction
* @see AWS API Documentation
*/
@Override
public DisassociateLambdaFunctionResponse disassociateLambdaFunction(
DisassociateLambdaFunctionRequest disassociateLambdaFunctionRequest) throws ResourceNotFoundException,
InternalServiceException, InvalidRequestException, InvalidParameterException, ThrottlingException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateLambdaFunctionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateLambdaFunctionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateLambdaFunction");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateLambdaFunction").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(disassociateLambdaFunctionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateLambdaFunctionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Revokes authorization from the specified instance to access the specified Amazon Lex bot.
*
*
* @param disassociateLexBotRequest
* @return Result of the DisassociateLexBot operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DisassociateLexBot
* @see AWS
* API Documentation
*/
@Override
public DisassociateLexBotResponse disassociateLexBot(DisassociateLexBotRequest disassociateLexBotRequest)
throws ResourceNotFoundException, InternalServiceException, InvalidRequestException, InvalidParameterException,
ThrottlingException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateLexBotResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateLexBotRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateLexBot");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DisassociateLexBot").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(disassociateLexBotRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateLexBotRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Disassociates a set of quick connects from a queue.
*
*
* @param disassociateQueueQuickConnectsRequest
* @return Result of the DisassociateQueueQuickConnects operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DisassociateQueueQuickConnects
* @see AWS API Documentation
*/
@Override
public DisassociateQueueQuickConnectsResponse disassociateQueueQuickConnects(
DisassociateQueueQuickConnectsRequest disassociateQueueQuickConnectsRequest) throws InvalidRequestException,
InvalidParameterException, ResourceNotFoundException, ThrottlingException, InternalServiceException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateQueueQuickConnectsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
disassociateQueueQuickConnectsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateQueueQuickConnects");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateQueueQuickConnects").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(disassociateQueueQuickConnectsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateQueueQuickConnectsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Disassociates a set of queues from a routing profile.
*
*
* @param disassociateRoutingProfileQueuesRequest
* @return Result of the DisassociateRoutingProfileQueues operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DisassociateRoutingProfileQueues
* @see AWS API Documentation
*/
@Override
public DisassociateRoutingProfileQueuesResponse disassociateRoutingProfileQueues(
DisassociateRoutingProfileQueuesRequest disassociateRoutingProfileQueuesRequest) throws InvalidRequestException,
InvalidParameterException, ResourceNotFoundException, ThrottlingException, InternalServiceException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateRoutingProfileQueuesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
disassociateRoutingProfileQueuesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateRoutingProfileQueues");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateRoutingProfileQueues").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(disassociateRoutingProfileQueuesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateRoutingProfileQueuesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Deletes the specified security key.
*
*
* @param disassociateSecurityKeyRequest
* @return Result of the DisassociateSecurityKey operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.DisassociateSecurityKey
* @see AWS API Documentation
*/
@Override
public DisassociateSecurityKeyResponse disassociateSecurityKey(DisassociateSecurityKeyRequest disassociateSecurityKeyRequest)
throws ResourceNotFoundException, InternalServiceException, InvalidRequestException, InvalidParameterException,
ThrottlingException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateSecurityKeyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateSecurityKeyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateSecurityKey");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateSecurityKey").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(disassociateSecurityKeyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateSecurityKeyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the contact attributes for the specified contact.
*
*
* @param getContactAttributesRequest
* @return Result of the GetContactAttributes operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.GetContactAttributes
* @see AWS
* API Documentation
*/
@Override
public GetContactAttributesResponse getContactAttributes(GetContactAttributesRequest getContactAttributesRequest)
throws InvalidRequestException, ResourceNotFoundException, InternalServiceException, AwsServiceException,
SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetContactAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getContactAttributesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetContactAttributes");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetContactAttributes").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getContactAttributesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetContactAttributesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets the real-time metric data from the specified Amazon Connect instance.
*
*
* For a description of each metric, see Real-time Metrics
* Definitions in the Amazon Connect Administrator Guide.
*
*
* @param getCurrentMetricDataRequest
* @return Result of the GetCurrentMetricData operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.GetCurrentMetricData
* @see AWS
* API Documentation
*/
@Override
public GetCurrentMetricDataResponse getCurrentMetricData(GetCurrentMetricDataRequest getCurrentMetricDataRequest)
throws InvalidRequestException, InvalidParameterException, InternalServiceException, ThrottlingException,
ResourceNotFoundException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetCurrentMetricDataResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getCurrentMetricDataRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetCurrentMetricData");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetCurrentMetricData").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getCurrentMetricDataRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetCurrentMetricDataRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets the real-time metric data from the specified Amazon Connect instance.
*
*
* For a description of each metric, see Real-time Metrics
* Definitions in the Amazon Connect Administrator Guide.
*
*
*
* This is a variant of
* {@link #getCurrentMetricData(software.amazon.awssdk.services.connect.model.GetCurrentMetricDataRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.GetCurrentMetricDataIterable responses = client.getCurrentMetricDataPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.connect.paginators.GetCurrentMetricDataIterable responses = client
* .getCurrentMetricDataPaginator(request);
* for (software.amazon.awssdk.services.connect.model.GetCurrentMetricDataResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.GetCurrentMetricDataIterable responses = client.getCurrentMetricDataPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getCurrentMetricData(software.amazon.awssdk.services.connect.model.GetCurrentMetricDataRequest)}
* operation.
*
*
* @param getCurrentMetricDataRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.GetCurrentMetricData
* @see AWS
* API Documentation
*/
@Override
public GetCurrentMetricDataIterable getCurrentMetricDataPaginator(GetCurrentMetricDataRequest getCurrentMetricDataRequest)
throws InvalidRequestException, InvalidParameterException, InternalServiceException, ThrottlingException,
ResourceNotFoundException, AwsServiceException, SdkClientException, ConnectException {
return new GetCurrentMetricDataIterable(this, applyPaginatorUserAgent(getCurrentMetricDataRequest));
}
/**
*
* Retrieves a token for federation.
*
*
*
* This API doesn't support root users. If you try to invoke GetFederationToken with root credentials, an error
* message similar to the following one appears:
*
*
* Provided identity: Principal: .... User: .... cannot be used for federation with Amazon Connect
*
*
*
* @param getFederationTokenRequest
* @return Result of the GetFederationToken operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws UserNotFoundException
* No user with the specified credentials was found in the Amazon Connect instance.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws DuplicateResourceException
* A resource with the specified name already exists.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.GetFederationToken
* @see AWS
* API Documentation
*/
@Override
public GetFederationTokenResponse getFederationToken(GetFederationTokenRequest getFederationTokenRequest)
throws InvalidRequestException, InvalidParameterException, ResourceNotFoundException, UserNotFoundException,
InternalServiceException, DuplicateResourceException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetFederationTokenResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getFederationTokenRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetFederationToken");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetFederationToken").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getFederationTokenRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetFederationTokenRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets historical metric data from the specified Amazon Connect instance.
*
*
* For a description of each historical metric, see Historical
* Metrics Definitions in the Amazon Connect Administrator Guide.
*
*
* @param getMetricDataRequest
* @return Result of the GetMetricData operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.GetMetricData
* @see AWS API
* Documentation
*/
@Override
public GetMetricDataResponse getMetricData(GetMetricDataRequest getMetricDataRequest) throws InvalidRequestException,
InvalidParameterException, InternalServiceException, ThrottlingException, ResourceNotFoundException,
AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetMetricDataResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getMetricDataRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Connect");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetMetricData");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetMetricData").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getMetricDataRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetMetricDataRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets historical metric data from the specified Amazon Connect instance.
*
*
* For a description of each historical metric, see Historical
* Metrics Definitions in the Amazon Connect Administrator Guide.
*
*
*
* This is a variant of {@link #getMetricData(software.amazon.awssdk.services.connect.model.GetMetricDataRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.GetMetricDataIterable responses = client.getMetricDataPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.connect.paginators.GetMetricDataIterable responses = client.getMetricDataPaginator(request);
* for (software.amazon.awssdk.services.connect.model.GetMetricDataResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.GetMetricDataIterable responses = client.getMetricDataPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getMetricData(software.amazon.awssdk.services.connect.model.GetMetricDataRequest)} operation.
*
*
* @param getMetricDataRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.GetMetricData
* @see AWS API
* Documentation
*/
@Override
public GetMetricDataIterable getMetricDataPaginator(GetMetricDataRequest getMetricDataRequest)
throws InvalidRequestException, InvalidParameterException, InternalServiceException, ThrottlingException,
ResourceNotFoundException, AwsServiceException, SdkClientException, ConnectException {
return new GetMetricDataIterable(this, applyPaginatorUserAgent(getMetricDataRequest));
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Lists agent statuses.
*
*
* @param listAgentStatusesRequest
* @return Result of the ListAgentStatuses operation returned by the service.
* @throws InvalidRequestException
* The request is not valid.
* @throws InvalidParameterException
* One or more of the specified parameters are not valid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @throws InternalServiceException
* Request processing failed because of an error or failure with the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ConnectException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ConnectClient.ListAgentStatuses
* @see AWS API
* Documentation
*/
@Override
public ListAgentStatusesResponse listAgentStatuses(ListAgentStatusesRequest listAgentStatusesRequest)
throws InvalidRequestException, InvalidParameterException, ResourceNotFoundException, ThrottlingException,
InternalServiceException, AwsServiceException, SdkClientException, ConnectException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListAgentStatusesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List