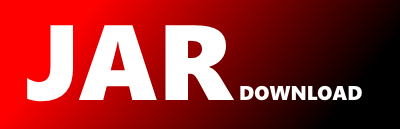
software.amazon.awssdk.services.connect.model.Contact Maven / Gradle / Ivy
Show all versions of connect Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.connect.model;
import java.beans.Transient;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains information about a contact.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Contact implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Arn")
.getter(getter(Contact::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Arn").build()).build();
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(Contact::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField INITIAL_CONTACT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InitialContactId").getter(getter(Contact::initialContactId)).setter(setter(Builder::initialContactId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InitialContactId").build()).build();
private static final SdkField PREVIOUS_CONTACT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PreviousContactId").getter(getter(Contact::previousContactId))
.setter(setter(Builder::previousContactId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreviousContactId").build()).build();
private static final SdkField INITIATION_METHOD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InitiationMethod").getter(getter(Contact::initiationMethodAsString))
.setter(setter(Builder::initiationMethod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InitiationMethod").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(Contact::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(Contact::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField CHANNEL_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Channel")
.getter(getter(Contact::channelAsString)).setter(setter(Builder::channel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Channel").build()).build();
private static final SdkField QUEUE_INFO_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("QueueInfo").getter(getter(Contact::queueInfo)).setter(setter(Builder::queueInfo))
.constructor(QueueInfo::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("QueueInfo").build()).build();
private static final SdkField AGENT_INFO_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("AgentInfo").getter(getter(Contact::agentInfo)).setter(setter(Builder::agentInfo))
.constructor(AgentInfo::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AgentInfo").build()).build();
private static final SdkField INITIATION_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("InitiationTimestamp").getter(getter(Contact::initiationTimestamp))
.setter(setter(Builder::initiationTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InitiationTimestamp").build())
.build();
private static final SdkField DISCONNECT_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("DisconnectTimestamp").getter(getter(Contact::disconnectTimestamp))
.setter(setter(Builder::disconnectTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DisconnectTimestamp").build())
.build();
private static final SdkField LAST_UPDATE_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastUpdateTimestamp").getter(getter(Contact::lastUpdateTimestamp))
.setter(setter(Builder::lastUpdateTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastUpdateTimestamp").build())
.build();
private static final SdkField SCHEDULED_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("ScheduledTimestamp").getter(getter(Contact::scheduledTimestamp))
.setter(setter(Builder::scheduledTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ScheduledTimestamp").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ARN_FIELD, ID_FIELD,
INITIAL_CONTACT_ID_FIELD, PREVIOUS_CONTACT_ID_FIELD, INITIATION_METHOD_FIELD, NAME_FIELD, DESCRIPTION_FIELD,
CHANNEL_FIELD, QUEUE_INFO_FIELD, AGENT_INFO_FIELD, INITIATION_TIMESTAMP_FIELD, DISCONNECT_TIMESTAMP_FIELD,
LAST_UPDATE_TIMESTAMP_FIELD, SCHEDULED_TIMESTAMP_FIELD));
private static final long serialVersionUID = 1L;
private final String arn;
private final String id;
private final String initialContactId;
private final String previousContactId;
private final String initiationMethod;
private final String name;
private final String description;
private final String channel;
private final QueueInfo queueInfo;
private final AgentInfo agentInfo;
private final Instant initiationTimestamp;
private final Instant disconnectTimestamp;
private final Instant lastUpdateTimestamp;
private final Instant scheduledTimestamp;
private Contact(BuilderImpl builder) {
this.arn = builder.arn;
this.id = builder.id;
this.initialContactId = builder.initialContactId;
this.previousContactId = builder.previousContactId;
this.initiationMethod = builder.initiationMethod;
this.name = builder.name;
this.description = builder.description;
this.channel = builder.channel;
this.queueInfo = builder.queueInfo;
this.agentInfo = builder.agentInfo;
this.initiationTimestamp = builder.initiationTimestamp;
this.disconnectTimestamp = builder.disconnectTimestamp;
this.lastUpdateTimestamp = builder.lastUpdateTimestamp;
this.scheduledTimestamp = builder.scheduledTimestamp;
}
/**
*
* The Amazon Resource Name (ARN) for the contact.
*
*
* @return The Amazon Resource Name (ARN) for the contact.
*/
public final String arn() {
return arn;
}
/**
*
* The identifier for the contact.
*
*
* @return The identifier for the contact.
*/
public final String id() {
return id;
}
/**
*
* If this contact is related to other contacts, this is the ID of the initial contact.
*
*
* @return If this contact is related to other contacts, this is the ID of the initial contact.
*/
public final String initialContactId() {
return initialContactId;
}
/**
*
* If this contact is not the first contact, this is the ID of the previous contact.
*
*
* @return If this contact is not the first contact, this is the ID of the previous contact.
*/
public final String previousContactId() {
return previousContactId;
}
/**
*
* Indicates how the contact was initiated.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #initiationMethod}
* will return {@link ContactInitiationMethod#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #initiationMethodAsString}.
*
*
* @return Indicates how the contact was initiated.
* @see ContactInitiationMethod
*/
public final ContactInitiationMethod initiationMethod() {
return ContactInitiationMethod.fromValue(initiationMethod);
}
/**
*
* Indicates how the contact was initiated.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #initiationMethod}
* will return {@link ContactInitiationMethod#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #initiationMethodAsString}.
*
*
* @return Indicates how the contact was initiated.
* @see ContactInitiationMethod
*/
public final String initiationMethodAsString() {
return initiationMethod;
}
/**
*
* The name of the contact.
*
*
* @return The name of the contact.
*/
public final String name() {
return name;
}
/**
*
* The description of the contact.
*
*
* @return The description of the contact.
*/
public final String description() {
return description;
}
/**
*
* How the contact reached your contact center.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #channel} will
* return {@link Channel#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #channelAsString}.
*
*
* @return How the contact reached your contact center.
* @see Channel
*/
public final Channel channel() {
return Channel.fromValue(channel);
}
/**
*
* How the contact reached your contact center.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #channel} will
* return {@link Channel#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #channelAsString}.
*
*
* @return How the contact reached your contact center.
* @see Channel
*/
public final String channelAsString() {
return channel;
}
/**
*
* If this contact was queued, this contains information about the queue.
*
*
* @return If this contact was queued, this contains information about the queue.
*/
public final QueueInfo queueInfo() {
return queueInfo;
}
/**
*
* Information about the agent who accepted the contact.
*
*
* @return Information about the agent who accepted the contact.
*/
public final AgentInfo agentInfo() {
return agentInfo;
}
/**
*
* The date and time this contact was initiated, in UTC time. For INBOUND
, this is when the contact
* arrived. For OUTBOUND
, this is when the agent began dialing. For CALLBACK
, this is when
* the callback contact was created. For TRANSFER
and QUEUE_TRANSFER
, this is when the
* transfer was initiated. For API
, this is when the request arrived.
*
*
* @return The date and time this contact was initiated, in UTC time. For INBOUND
, this is when the
* contact arrived. For OUTBOUND
, this is when the agent began dialing. For
* CALLBACK
, this is when the callback contact was created. For TRANSFER
and
* QUEUE_TRANSFER
, this is when the transfer was initiated. For API
, this is when
* the request arrived.
*/
public final Instant initiationTimestamp() {
return initiationTimestamp;
}
/**
*
* The timestamp when the customer endpoint disconnected from Amazon Connect.
*
*
* @return The timestamp when the customer endpoint disconnected from Amazon Connect.
*/
public final Instant disconnectTimestamp() {
return disconnectTimestamp;
}
/**
*
* The timestamp when contact was last updated.
*
*
* @return The timestamp when contact was last updated.
*/
public final Instant lastUpdateTimestamp() {
return lastUpdateTimestamp;
}
/**
*
* The timestamp, in Unix epoch time format, at which to start running the inbound flow.
*
*
* @return The timestamp, in Unix epoch time format, at which to start running the inbound flow.
*/
public final Instant scheduledTimestamp() {
return scheduledTimestamp;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(initialContactId());
hashCode = 31 * hashCode + Objects.hashCode(previousContactId());
hashCode = 31 * hashCode + Objects.hashCode(initiationMethodAsString());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(channelAsString());
hashCode = 31 * hashCode + Objects.hashCode(queueInfo());
hashCode = 31 * hashCode + Objects.hashCode(agentInfo());
hashCode = 31 * hashCode + Objects.hashCode(initiationTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(disconnectTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(lastUpdateTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(scheduledTimestamp());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Contact)) {
return false;
}
Contact other = (Contact) obj;
return Objects.equals(arn(), other.arn()) && Objects.equals(id(), other.id())
&& Objects.equals(initialContactId(), other.initialContactId())
&& Objects.equals(previousContactId(), other.previousContactId())
&& Objects.equals(initiationMethodAsString(), other.initiationMethodAsString())
&& Objects.equals(name(), other.name()) && Objects.equals(description(), other.description())
&& Objects.equals(channelAsString(), other.channelAsString()) && Objects.equals(queueInfo(), other.queueInfo())
&& Objects.equals(agentInfo(), other.agentInfo())
&& Objects.equals(initiationTimestamp(), other.initiationTimestamp())
&& Objects.equals(disconnectTimestamp(), other.disconnectTimestamp())
&& Objects.equals(lastUpdateTimestamp(), other.lastUpdateTimestamp())
&& Objects.equals(scheduledTimestamp(), other.scheduledTimestamp());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Contact").add("Arn", arn()).add("Id", id()).add("InitialContactId", initialContactId())
.add("PreviousContactId", previousContactId()).add("InitiationMethod", initiationMethodAsString())
.add("Name", name()).add("Description", description()).add("Channel", channelAsString())
.add("QueueInfo", queueInfo()).add("AgentInfo", agentInfo()).add("InitiationTimestamp", initiationTimestamp())
.add("DisconnectTimestamp", disconnectTimestamp()).add("LastUpdateTimestamp", lastUpdateTimestamp())
.add("ScheduledTimestamp", scheduledTimestamp()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Arn":
return Optional.ofNullable(clazz.cast(arn()));
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "InitialContactId":
return Optional.ofNullable(clazz.cast(initialContactId()));
case "PreviousContactId":
return Optional.ofNullable(clazz.cast(previousContactId()));
case "InitiationMethod":
return Optional.ofNullable(clazz.cast(initiationMethodAsString()));
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "Channel":
return Optional.ofNullable(clazz.cast(channelAsString()));
case "QueueInfo":
return Optional.ofNullable(clazz.cast(queueInfo()));
case "AgentInfo":
return Optional.ofNullable(clazz.cast(agentInfo()));
case "InitiationTimestamp":
return Optional.ofNullable(clazz.cast(initiationTimestamp()));
case "DisconnectTimestamp":
return Optional.ofNullable(clazz.cast(disconnectTimestamp()));
case "LastUpdateTimestamp":
return Optional.ofNullable(clazz.cast(lastUpdateTimestamp()));
case "ScheduledTimestamp":
return Optional.ofNullable(clazz.cast(scheduledTimestamp()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function