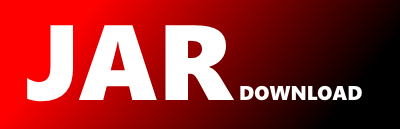
software.amazon.awssdk.services.connect.model.ReferenceSummary Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.connect.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.EnumSet;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructMap;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains summary information about a reference. ReferenceSummary
contains only one non null field
* between the URL and attachment based on the reference type.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ReferenceSummary implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField URL_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Url").getter(getter(ReferenceSummary::url)).setter(setter(Builder::url))
.constructor(UrlReference::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Url").build()).build();
private static final SdkField ATTACHMENT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Attachment")
.getter(getter(ReferenceSummary::attachment)).setter(setter(Builder::attachment))
.constructor(AttachmentReference::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Attachment").build()).build();
private static final SdkField STRING_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("String").getter(getter(ReferenceSummary::string)).setter(setter(Builder::string))
.constructor(StringReference::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("String").build()).build();
private static final SdkField NUMBER_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Number").getter(getter(ReferenceSummary::number)).setter(setter(Builder::number))
.constructor(NumberReference::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Number").build()).build();
private static final SdkField DATE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Date").getter(getter(ReferenceSummary::date)).setter(setter(Builder::date))
.constructor(DateReference::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Date").build()).build();
private static final SdkField EMAIL_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Email").getter(getter(ReferenceSummary::email)).setter(setter(Builder::email))
.constructor(EmailReference::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Email").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(URL_FIELD, ATTACHMENT_FIELD,
STRING_FIELD, NUMBER_FIELD, DATE_FIELD, EMAIL_FIELD));
private static final long serialVersionUID = 1L;
private final UrlReference url;
private final AttachmentReference attachment;
private final StringReference string;
private final NumberReference number;
private final DateReference date;
private final EmailReference email;
private final Type type;
private ReferenceSummary(BuilderImpl builder) {
this.url = builder.url;
this.attachment = builder.attachment;
this.string = builder.string;
this.number = builder.number;
this.date = builder.date;
this.email = builder.email;
this.type = builder.type;
}
/**
*
* Information about the reference when the referenceType
is URL
. Otherwise, null.
*
*
* @return Information about the reference when the referenceType
is URL
. Otherwise, null.
*/
public final UrlReference url() {
return url;
}
/**
*
* Information about the reference when the referenceType
is ATTACHMENT
. Otherwise, null.
*
*
* @return Information about the reference when the referenceType
is ATTACHMENT
.
* Otherwise, null.
*/
public final AttachmentReference attachment() {
return attachment;
}
/**
*
* Information about a reference when the referenceType
is STRING
. Otherwise, null.
*
*
* @return Information about a reference when the referenceType
is STRING
. Otherwise,
* null.
*/
public final StringReference string() {
return string;
}
/**
*
* Information about a reference when the referenceType
is NUMBER
. Otherwise, null.
*
*
* @return Information about a reference when the referenceType
is NUMBER
. Otherwise,
* null.
*/
public final NumberReference number() {
return number;
}
/**
*
* Information about a reference when the referenceType
is DATE
. Otherwise, null.
*
*
* @return Information about a reference when the referenceType
is DATE
. Otherwise, null.
*/
public final DateReference date() {
return date;
}
/**
*
* Information about a reference when the referenceType
is EMAIL
. Otherwise, null.
*
*
* @return Information about a reference when the referenceType
is EMAIL
. Otherwise, null.
*/
public final EmailReference email() {
return email;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(url());
hashCode = 31 * hashCode + Objects.hashCode(attachment());
hashCode = 31 * hashCode + Objects.hashCode(string());
hashCode = 31 * hashCode + Objects.hashCode(number());
hashCode = 31 * hashCode + Objects.hashCode(date());
hashCode = 31 * hashCode + Objects.hashCode(email());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ReferenceSummary)) {
return false;
}
ReferenceSummary other = (ReferenceSummary) obj;
return Objects.equals(url(), other.url()) && Objects.equals(attachment(), other.attachment())
&& Objects.equals(string(), other.string()) && Objects.equals(number(), other.number())
&& Objects.equals(date(), other.date()) && Objects.equals(email(), other.email());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ReferenceSummary").add("Url", url()).add("Attachment", attachment()).add("String", string())
.add("Number", number()).add("Date", date()).add("Email", email()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Url":
return Optional.ofNullable(clazz.cast(url()));
case "Attachment":
return Optional.ofNullable(clazz.cast(attachment()));
case "String":
return Optional.ofNullable(clazz.cast(string()));
case "Number":
return Optional.ofNullable(clazz.cast(number()));
case "Date":
return Optional.ofNullable(clazz.cast(date()));
case "Email":
return Optional.ofNullable(clazz.cast(email()));
default:
return Optional.empty();
}
}
/**
* Create an instance of this class with {@link #url()} initialized to the given value.
*
*
* Information about the reference when the referenceType
is URL
. Otherwise, null.
*
*
* @param url
* Information about the reference when the referenceType
is URL
. Otherwise, null.
*/
public static ReferenceSummary fromUrl(UrlReference url) {
return builder().url(url).build();
}
/**
* Create an instance of this class with {@link #url()} initialized to the given value.
*
*
* Information about the reference when the referenceType
is URL
. Otherwise, null.
*
*
* @param url
* Information about the reference when the referenceType
is URL
. Otherwise, null.
*/
public static ReferenceSummary fromUrl(Consumer url) {
UrlReference.Builder builder = UrlReference.builder();
url.accept(builder);
return fromUrl(builder.build());
}
/**
* Create an instance of this class with {@link #attachment()} initialized to the given value.
*
*
* Information about the reference when the referenceType
is ATTACHMENT
. Otherwise, null.
*
*
* @param attachment
* Information about the reference when the referenceType
is ATTACHMENT
. Otherwise,
* null.
*/
public static ReferenceSummary fromAttachment(AttachmentReference attachment) {
return builder().attachment(attachment).build();
}
/**
* Create an instance of this class with {@link #attachment()} initialized to the given value.
*
*
* Information about the reference when the referenceType
is ATTACHMENT
. Otherwise, null.
*
*
* @param attachment
* Information about the reference when the referenceType
is ATTACHMENT
. Otherwise,
* null.
*/
public static ReferenceSummary fromAttachment(Consumer attachment) {
AttachmentReference.Builder builder = AttachmentReference.builder();
attachment.accept(builder);
return fromAttachment(builder.build());
}
/**
* Create an instance of this class with {@link #string()} initialized to the given value.
*
*
* Information about a reference when the referenceType
is STRING
. Otherwise, null.
*
*
* @param string
* Information about a reference when the referenceType
is STRING
. Otherwise, null.
*/
public static ReferenceSummary fromString(StringReference string) {
return builder().string(string).build();
}
/**
* Create an instance of this class with {@link #string()} initialized to the given value.
*
*
* Information about a reference when the referenceType
is STRING
. Otherwise, null.
*
*
* @param string
* Information about a reference when the referenceType
is STRING
. Otherwise, null.
*/
public static ReferenceSummary fromString(Consumer string) {
StringReference.Builder builder = StringReference.builder();
string.accept(builder);
return fromString(builder.build());
}
/**
* Create an instance of this class with {@link #number()} initialized to the given value.
*
*
* Information about a reference when the referenceType
is NUMBER
. Otherwise, null.
*
*
* @param number
* Information about a reference when the referenceType
is NUMBER
. Otherwise, null.
*/
public static ReferenceSummary fromNumber(NumberReference number) {
return builder().number(number).build();
}
/**
* Create an instance of this class with {@link #number()} initialized to the given value.
*
*
* Information about a reference when the referenceType
is NUMBER
. Otherwise, null.
*
*
* @param number
* Information about a reference when the referenceType
is NUMBER
. Otherwise, null.
*/
public static ReferenceSummary fromNumber(Consumer number) {
NumberReference.Builder builder = NumberReference.builder();
number.accept(builder);
return fromNumber(builder.build());
}
/**
* Create an instance of this class with {@link #date()} initialized to the given value.
*
*
* Information about a reference when the referenceType
is DATE
. Otherwise, null.
*
*
* @param date
* Information about a reference when the referenceType
is DATE
. Otherwise, null.
*/
public static ReferenceSummary fromDate(DateReference date) {
return builder().date(date).build();
}
/**
* Create an instance of this class with {@link #date()} initialized to the given value.
*
*
* Information about a reference when the referenceType
is DATE
. Otherwise, null.
*
*
* @param date
* Information about a reference when the referenceType
is DATE
. Otherwise, null.
*/
public static ReferenceSummary fromDate(Consumer date) {
DateReference.Builder builder = DateReference.builder();
date.accept(builder);
return fromDate(builder.build());
}
/**
* Create an instance of this class with {@link #email()} initialized to the given value.
*
*
* Information about a reference when the referenceType
is EMAIL
. Otherwise, null.
*
*
* @param email
* Information about a reference when the referenceType
is EMAIL
. Otherwise, null.
*/
public static ReferenceSummary fromEmail(EmailReference email) {
return builder().email(email).build();
}
/**
* Create an instance of this class with {@link #email()} initialized to the given value.
*
*
* Information about a reference when the referenceType
is EMAIL
. Otherwise, null.
*
*
* @param email
* Information about a reference when the referenceType
is EMAIL
. Otherwise, null.
*/
public static ReferenceSummary fromEmail(Consumer email) {
EmailReference.Builder builder = EmailReference.builder();
email.accept(builder);
return fromEmail(builder.build());
}
/**
* Retrieve an enum value representing which member of this object is populated.
*
* When this class is returned in a service response, this will be {@link Type#UNKNOWN_TO_SDK_VERSION} if the
* service returned a member that is only known to a newer SDK version.
*
* When this class is created directly in your code, this will be {@link Type#UNKNOWN_TO_SDK_VERSION} if zero
* members are set, and {@code null} if more than one member is set.
*/
public Type type() {
return type;
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function