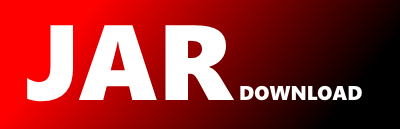
software.amazon.awssdk.services.connect.ConnectAsyncClient Maven / Gradle / Ivy
Show all versions of connect Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.connect;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.connect.model.AssociateApprovedOriginRequest;
import software.amazon.awssdk.services.connect.model.AssociateApprovedOriginResponse;
import software.amazon.awssdk.services.connect.model.AssociateBotRequest;
import software.amazon.awssdk.services.connect.model.AssociateBotResponse;
import software.amazon.awssdk.services.connect.model.AssociateDefaultVocabularyRequest;
import software.amazon.awssdk.services.connect.model.AssociateDefaultVocabularyResponse;
import software.amazon.awssdk.services.connect.model.AssociateInstanceStorageConfigRequest;
import software.amazon.awssdk.services.connect.model.AssociateInstanceStorageConfigResponse;
import software.amazon.awssdk.services.connect.model.AssociateLambdaFunctionRequest;
import software.amazon.awssdk.services.connect.model.AssociateLambdaFunctionResponse;
import software.amazon.awssdk.services.connect.model.AssociateLexBotRequest;
import software.amazon.awssdk.services.connect.model.AssociateLexBotResponse;
import software.amazon.awssdk.services.connect.model.AssociatePhoneNumberContactFlowRequest;
import software.amazon.awssdk.services.connect.model.AssociatePhoneNumberContactFlowResponse;
import software.amazon.awssdk.services.connect.model.AssociateQueueQuickConnectsRequest;
import software.amazon.awssdk.services.connect.model.AssociateQueueQuickConnectsResponse;
import software.amazon.awssdk.services.connect.model.AssociateRoutingProfileQueuesRequest;
import software.amazon.awssdk.services.connect.model.AssociateRoutingProfileQueuesResponse;
import software.amazon.awssdk.services.connect.model.AssociateSecurityKeyRequest;
import software.amazon.awssdk.services.connect.model.AssociateSecurityKeyResponse;
import software.amazon.awssdk.services.connect.model.ClaimPhoneNumberRequest;
import software.amazon.awssdk.services.connect.model.ClaimPhoneNumberResponse;
import software.amazon.awssdk.services.connect.model.CreateAgentStatusRequest;
import software.amazon.awssdk.services.connect.model.CreateAgentStatusResponse;
import software.amazon.awssdk.services.connect.model.CreateContactFlowModuleRequest;
import software.amazon.awssdk.services.connect.model.CreateContactFlowModuleResponse;
import software.amazon.awssdk.services.connect.model.CreateContactFlowRequest;
import software.amazon.awssdk.services.connect.model.CreateContactFlowResponse;
import software.amazon.awssdk.services.connect.model.CreateHoursOfOperationRequest;
import software.amazon.awssdk.services.connect.model.CreateHoursOfOperationResponse;
import software.amazon.awssdk.services.connect.model.CreateInstanceRequest;
import software.amazon.awssdk.services.connect.model.CreateInstanceResponse;
import software.amazon.awssdk.services.connect.model.CreateIntegrationAssociationRequest;
import software.amazon.awssdk.services.connect.model.CreateIntegrationAssociationResponse;
import software.amazon.awssdk.services.connect.model.CreateQueueRequest;
import software.amazon.awssdk.services.connect.model.CreateQueueResponse;
import software.amazon.awssdk.services.connect.model.CreateQuickConnectRequest;
import software.amazon.awssdk.services.connect.model.CreateQuickConnectResponse;
import software.amazon.awssdk.services.connect.model.CreateRoutingProfileRequest;
import software.amazon.awssdk.services.connect.model.CreateRoutingProfileResponse;
import software.amazon.awssdk.services.connect.model.CreateRuleRequest;
import software.amazon.awssdk.services.connect.model.CreateRuleResponse;
import software.amazon.awssdk.services.connect.model.CreateSecurityProfileRequest;
import software.amazon.awssdk.services.connect.model.CreateSecurityProfileResponse;
import software.amazon.awssdk.services.connect.model.CreateTaskTemplateRequest;
import software.amazon.awssdk.services.connect.model.CreateTaskTemplateResponse;
import software.amazon.awssdk.services.connect.model.CreateTrafficDistributionGroupRequest;
import software.amazon.awssdk.services.connect.model.CreateTrafficDistributionGroupResponse;
import software.amazon.awssdk.services.connect.model.CreateUseCaseRequest;
import software.amazon.awssdk.services.connect.model.CreateUseCaseResponse;
import software.amazon.awssdk.services.connect.model.CreateUserHierarchyGroupRequest;
import software.amazon.awssdk.services.connect.model.CreateUserHierarchyGroupResponse;
import software.amazon.awssdk.services.connect.model.CreateUserRequest;
import software.amazon.awssdk.services.connect.model.CreateUserResponse;
import software.amazon.awssdk.services.connect.model.CreateVocabularyRequest;
import software.amazon.awssdk.services.connect.model.CreateVocabularyResponse;
import software.amazon.awssdk.services.connect.model.DeleteContactFlowModuleRequest;
import software.amazon.awssdk.services.connect.model.DeleteContactFlowModuleResponse;
import software.amazon.awssdk.services.connect.model.DeleteContactFlowRequest;
import software.amazon.awssdk.services.connect.model.DeleteContactFlowResponse;
import software.amazon.awssdk.services.connect.model.DeleteHoursOfOperationRequest;
import software.amazon.awssdk.services.connect.model.DeleteHoursOfOperationResponse;
import software.amazon.awssdk.services.connect.model.DeleteInstanceRequest;
import software.amazon.awssdk.services.connect.model.DeleteInstanceResponse;
import software.amazon.awssdk.services.connect.model.DeleteIntegrationAssociationRequest;
import software.amazon.awssdk.services.connect.model.DeleteIntegrationAssociationResponse;
import software.amazon.awssdk.services.connect.model.DeleteQuickConnectRequest;
import software.amazon.awssdk.services.connect.model.DeleteQuickConnectResponse;
import software.amazon.awssdk.services.connect.model.DeleteRuleRequest;
import software.amazon.awssdk.services.connect.model.DeleteRuleResponse;
import software.amazon.awssdk.services.connect.model.DeleteSecurityProfileRequest;
import software.amazon.awssdk.services.connect.model.DeleteSecurityProfileResponse;
import software.amazon.awssdk.services.connect.model.DeleteTaskTemplateRequest;
import software.amazon.awssdk.services.connect.model.DeleteTaskTemplateResponse;
import software.amazon.awssdk.services.connect.model.DeleteTrafficDistributionGroupRequest;
import software.amazon.awssdk.services.connect.model.DeleteTrafficDistributionGroupResponse;
import software.amazon.awssdk.services.connect.model.DeleteUseCaseRequest;
import software.amazon.awssdk.services.connect.model.DeleteUseCaseResponse;
import software.amazon.awssdk.services.connect.model.DeleteUserHierarchyGroupRequest;
import software.amazon.awssdk.services.connect.model.DeleteUserHierarchyGroupResponse;
import software.amazon.awssdk.services.connect.model.DeleteUserRequest;
import software.amazon.awssdk.services.connect.model.DeleteUserResponse;
import software.amazon.awssdk.services.connect.model.DeleteVocabularyRequest;
import software.amazon.awssdk.services.connect.model.DeleteVocabularyResponse;
import software.amazon.awssdk.services.connect.model.DescribeAgentStatusRequest;
import software.amazon.awssdk.services.connect.model.DescribeAgentStatusResponse;
import software.amazon.awssdk.services.connect.model.DescribeContactFlowModuleRequest;
import software.amazon.awssdk.services.connect.model.DescribeContactFlowModuleResponse;
import software.amazon.awssdk.services.connect.model.DescribeContactFlowRequest;
import software.amazon.awssdk.services.connect.model.DescribeContactFlowResponse;
import software.amazon.awssdk.services.connect.model.DescribeContactRequest;
import software.amazon.awssdk.services.connect.model.DescribeContactResponse;
import software.amazon.awssdk.services.connect.model.DescribeHoursOfOperationRequest;
import software.amazon.awssdk.services.connect.model.DescribeHoursOfOperationResponse;
import software.amazon.awssdk.services.connect.model.DescribeInstanceAttributeRequest;
import software.amazon.awssdk.services.connect.model.DescribeInstanceAttributeResponse;
import software.amazon.awssdk.services.connect.model.DescribeInstanceRequest;
import software.amazon.awssdk.services.connect.model.DescribeInstanceResponse;
import software.amazon.awssdk.services.connect.model.DescribeInstanceStorageConfigRequest;
import software.amazon.awssdk.services.connect.model.DescribeInstanceStorageConfigResponse;
import software.amazon.awssdk.services.connect.model.DescribePhoneNumberRequest;
import software.amazon.awssdk.services.connect.model.DescribePhoneNumberResponse;
import software.amazon.awssdk.services.connect.model.DescribeQueueRequest;
import software.amazon.awssdk.services.connect.model.DescribeQueueResponse;
import software.amazon.awssdk.services.connect.model.DescribeQuickConnectRequest;
import software.amazon.awssdk.services.connect.model.DescribeQuickConnectResponse;
import software.amazon.awssdk.services.connect.model.DescribeRoutingProfileRequest;
import software.amazon.awssdk.services.connect.model.DescribeRoutingProfileResponse;
import software.amazon.awssdk.services.connect.model.DescribeRuleRequest;
import software.amazon.awssdk.services.connect.model.DescribeRuleResponse;
import software.amazon.awssdk.services.connect.model.DescribeSecurityProfileRequest;
import software.amazon.awssdk.services.connect.model.DescribeSecurityProfileResponse;
import software.amazon.awssdk.services.connect.model.DescribeTrafficDistributionGroupRequest;
import software.amazon.awssdk.services.connect.model.DescribeTrafficDistributionGroupResponse;
import software.amazon.awssdk.services.connect.model.DescribeUserHierarchyGroupRequest;
import software.amazon.awssdk.services.connect.model.DescribeUserHierarchyGroupResponse;
import software.amazon.awssdk.services.connect.model.DescribeUserHierarchyStructureRequest;
import software.amazon.awssdk.services.connect.model.DescribeUserHierarchyStructureResponse;
import software.amazon.awssdk.services.connect.model.DescribeUserRequest;
import software.amazon.awssdk.services.connect.model.DescribeUserResponse;
import software.amazon.awssdk.services.connect.model.DescribeVocabularyRequest;
import software.amazon.awssdk.services.connect.model.DescribeVocabularyResponse;
import software.amazon.awssdk.services.connect.model.DisassociateApprovedOriginRequest;
import software.amazon.awssdk.services.connect.model.DisassociateApprovedOriginResponse;
import software.amazon.awssdk.services.connect.model.DisassociateBotRequest;
import software.amazon.awssdk.services.connect.model.DisassociateBotResponse;
import software.amazon.awssdk.services.connect.model.DisassociateInstanceStorageConfigRequest;
import software.amazon.awssdk.services.connect.model.DisassociateInstanceStorageConfigResponse;
import software.amazon.awssdk.services.connect.model.DisassociateLambdaFunctionRequest;
import software.amazon.awssdk.services.connect.model.DisassociateLambdaFunctionResponse;
import software.amazon.awssdk.services.connect.model.DisassociateLexBotRequest;
import software.amazon.awssdk.services.connect.model.DisassociateLexBotResponse;
import software.amazon.awssdk.services.connect.model.DisassociatePhoneNumberContactFlowRequest;
import software.amazon.awssdk.services.connect.model.DisassociatePhoneNumberContactFlowResponse;
import software.amazon.awssdk.services.connect.model.DisassociateQueueQuickConnectsRequest;
import software.amazon.awssdk.services.connect.model.DisassociateQueueQuickConnectsResponse;
import software.amazon.awssdk.services.connect.model.DisassociateRoutingProfileQueuesRequest;
import software.amazon.awssdk.services.connect.model.DisassociateRoutingProfileQueuesResponse;
import software.amazon.awssdk.services.connect.model.DisassociateSecurityKeyRequest;
import software.amazon.awssdk.services.connect.model.DisassociateSecurityKeyResponse;
import software.amazon.awssdk.services.connect.model.DismissUserContactRequest;
import software.amazon.awssdk.services.connect.model.DismissUserContactResponse;
import software.amazon.awssdk.services.connect.model.GetContactAttributesRequest;
import software.amazon.awssdk.services.connect.model.GetContactAttributesResponse;
import software.amazon.awssdk.services.connect.model.GetCurrentMetricDataRequest;
import software.amazon.awssdk.services.connect.model.GetCurrentMetricDataResponse;
import software.amazon.awssdk.services.connect.model.GetCurrentUserDataRequest;
import software.amazon.awssdk.services.connect.model.GetCurrentUserDataResponse;
import software.amazon.awssdk.services.connect.model.GetFederationTokenRequest;
import software.amazon.awssdk.services.connect.model.GetFederationTokenResponse;
import software.amazon.awssdk.services.connect.model.GetMetricDataRequest;
import software.amazon.awssdk.services.connect.model.GetMetricDataResponse;
import software.amazon.awssdk.services.connect.model.GetTaskTemplateRequest;
import software.amazon.awssdk.services.connect.model.GetTaskTemplateResponse;
import software.amazon.awssdk.services.connect.model.GetTrafficDistributionRequest;
import software.amazon.awssdk.services.connect.model.GetTrafficDistributionResponse;
import software.amazon.awssdk.services.connect.model.ListAgentStatusesRequest;
import software.amazon.awssdk.services.connect.model.ListAgentStatusesResponse;
import software.amazon.awssdk.services.connect.model.ListApprovedOriginsRequest;
import software.amazon.awssdk.services.connect.model.ListApprovedOriginsResponse;
import software.amazon.awssdk.services.connect.model.ListBotsRequest;
import software.amazon.awssdk.services.connect.model.ListBotsResponse;
import software.amazon.awssdk.services.connect.model.ListContactFlowModulesRequest;
import software.amazon.awssdk.services.connect.model.ListContactFlowModulesResponse;
import software.amazon.awssdk.services.connect.model.ListContactFlowsRequest;
import software.amazon.awssdk.services.connect.model.ListContactFlowsResponse;
import software.amazon.awssdk.services.connect.model.ListContactReferencesRequest;
import software.amazon.awssdk.services.connect.model.ListContactReferencesResponse;
import software.amazon.awssdk.services.connect.model.ListDefaultVocabulariesRequest;
import software.amazon.awssdk.services.connect.model.ListDefaultVocabulariesResponse;
import software.amazon.awssdk.services.connect.model.ListHoursOfOperationsRequest;
import software.amazon.awssdk.services.connect.model.ListHoursOfOperationsResponse;
import software.amazon.awssdk.services.connect.model.ListInstanceAttributesRequest;
import software.amazon.awssdk.services.connect.model.ListInstanceAttributesResponse;
import software.amazon.awssdk.services.connect.model.ListInstanceStorageConfigsRequest;
import software.amazon.awssdk.services.connect.model.ListInstanceStorageConfigsResponse;
import software.amazon.awssdk.services.connect.model.ListInstancesRequest;
import software.amazon.awssdk.services.connect.model.ListInstancesResponse;
import software.amazon.awssdk.services.connect.model.ListIntegrationAssociationsRequest;
import software.amazon.awssdk.services.connect.model.ListIntegrationAssociationsResponse;
import software.amazon.awssdk.services.connect.model.ListLambdaFunctionsRequest;
import software.amazon.awssdk.services.connect.model.ListLambdaFunctionsResponse;
import software.amazon.awssdk.services.connect.model.ListLexBotsRequest;
import software.amazon.awssdk.services.connect.model.ListLexBotsResponse;
import software.amazon.awssdk.services.connect.model.ListPhoneNumbersRequest;
import software.amazon.awssdk.services.connect.model.ListPhoneNumbersResponse;
import software.amazon.awssdk.services.connect.model.ListPhoneNumbersV2Request;
import software.amazon.awssdk.services.connect.model.ListPhoneNumbersV2Response;
import software.amazon.awssdk.services.connect.model.ListPromptsRequest;
import software.amazon.awssdk.services.connect.model.ListPromptsResponse;
import software.amazon.awssdk.services.connect.model.ListQueueQuickConnectsRequest;
import software.amazon.awssdk.services.connect.model.ListQueueQuickConnectsResponse;
import software.amazon.awssdk.services.connect.model.ListQueuesRequest;
import software.amazon.awssdk.services.connect.model.ListQueuesResponse;
import software.amazon.awssdk.services.connect.model.ListQuickConnectsRequest;
import software.amazon.awssdk.services.connect.model.ListQuickConnectsResponse;
import software.amazon.awssdk.services.connect.model.ListRoutingProfileQueuesRequest;
import software.amazon.awssdk.services.connect.model.ListRoutingProfileQueuesResponse;
import software.amazon.awssdk.services.connect.model.ListRoutingProfilesRequest;
import software.amazon.awssdk.services.connect.model.ListRoutingProfilesResponse;
import software.amazon.awssdk.services.connect.model.ListRulesRequest;
import software.amazon.awssdk.services.connect.model.ListRulesResponse;
import software.amazon.awssdk.services.connect.model.ListSecurityKeysRequest;
import software.amazon.awssdk.services.connect.model.ListSecurityKeysResponse;
import software.amazon.awssdk.services.connect.model.ListSecurityProfilePermissionsRequest;
import software.amazon.awssdk.services.connect.model.ListSecurityProfilePermissionsResponse;
import software.amazon.awssdk.services.connect.model.ListSecurityProfilesRequest;
import software.amazon.awssdk.services.connect.model.ListSecurityProfilesResponse;
import software.amazon.awssdk.services.connect.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.connect.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.connect.model.ListTaskTemplatesRequest;
import software.amazon.awssdk.services.connect.model.ListTaskTemplatesResponse;
import software.amazon.awssdk.services.connect.model.ListTrafficDistributionGroupsRequest;
import software.amazon.awssdk.services.connect.model.ListTrafficDistributionGroupsResponse;
import software.amazon.awssdk.services.connect.model.ListUseCasesRequest;
import software.amazon.awssdk.services.connect.model.ListUseCasesResponse;
import software.amazon.awssdk.services.connect.model.ListUserHierarchyGroupsRequest;
import software.amazon.awssdk.services.connect.model.ListUserHierarchyGroupsResponse;
import software.amazon.awssdk.services.connect.model.ListUsersRequest;
import software.amazon.awssdk.services.connect.model.ListUsersResponse;
import software.amazon.awssdk.services.connect.model.MonitorContactRequest;
import software.amazon.awssdk.services.connect.model.MonitorContactResponse;
import software.amazon.awssdk.services.connect.model.PutUserStatusRequest;
import software.amazon.awssdk.services.connect.model.PutUserStatusResponse;
import software.amazon.awssdk.services.connect.model.ReleasePhoneNumberRequest;
import software.amazon.awssdk.services.connect.model.ReleasePhoneNumberResponse;
import software.amazon.awssdk.services.connect.model.ReplicateInstanceRequest;
import software.amazon.awssdk.services.connect.model.ReplicateInstanceResponse;
import software.amazon.awssdk.services.connect.model.ResumeContactRecordingRequest;
import software.amazon.awssdk.services.connect.model.ResumeContactRecordingResponse;
import software.amazon.awssdk.services.connect.model.SearchAvailablePhoneNumbersRequest;
import software.amazon.awssdk.services.connect.model.SearchAvailablePhoneNumbersResponse;
import software.amazon.awssdk.services.connect.model.SearchQueuesRequest;
import software.amazon.awssdk.services.connect.model.SearchQueuesResponse;
import software.amazon.awssdk.services.connect.model.SearchRoutingProfilesRequest;
import software.amazon.awssdk.services.connect.model.SearchRoutingProfilesResponse;
import software.amazon.awssdk.services.connect.model.SearchSecurityProfilesRequest;
import software.amazon.awssdk.services.connect.model.SearchSecurityProfilesResponse;
import software.amazon.awssdk.services.connect.model.SearchUsersRequest;
import software.amazon.awssdk.services.connect.model.SearchUsersResponse;
import software.amazon.awssdk.services.connect.model.SearchVocabulariesRequest;
import software.amazon.awssdk.services.connect.model.SearchVocabulariesResponse;
import software.amazon.awssdk.services.connect.model.StartChatContactRequest;
import software.amazon.awssdk.services.connect.model.StartChatContactResponse;
import software.amazon.awssdk.services.connect.model.StartContactRecordingRequest;
import software.amazon.awssdk.services.connect.model.StartContactRecordingResponse;
import software.amazon.awssdk.services.connect.model.StartContactStreamingRequest;
import software.amazon.awssdk.services.connect.model.StartContactStreamingResponse;
import software.amazon.awssdk.services.connect.model.StartOutboundVoiceContactRequest;
import software.amazon.awssdk.services.connect.model.StartOutboundVoiceContactResponse;
import software.amazon.awssdk.services.connect.model.StartTaskContactRequest;
import software.amazon.awssdk.services.connect.model.StartTaskContactResponse;
import software.amazon.awssdk.services.connect.model.StopContactRecordingRequest;
import software.amazon.awssdk.services.connect.model.StopContactRecordingResponse;
import software.amazon.awssdk.services.connect.model.StopContactRequest;
import software.amazon.awssdk.services.connect.model.StopContactResponse;
import software.amazon.awssdk.services.connect.model.StopContactStreamingRequest;
import software.amazon.awssdk.services.connect.model.StopContactStreamingResponse;
import software.amazon.awssdk.services.connect.model.SuspendContactRecordingRequest;
import software.amazon.awssdk.services.connect.model.SuspendContactRecordingResponse;
import software.amazon.awssdk.services.connect.model.TagResourceRequest;
import software.amazon.awssdk.services.connect.model.TagResourceResponse;
import software.amazon.awssdk.services.connect.model.TransferContactRequest;
import software.amazon.awssdk.services.connect.model.TransferContactResponse;
import software.amazon.awssdk.services.connect.model.UntagResourceRequest;
import software.amazon.awssdk.services.connect.model.UntagResourceResponse;
import software.amazon.awssdk.services.connect.model.UpdateAgentStatusRequest;
import software.amazon.awssdk.services.connect.model.UpdateAgentStatusResponse;
import software.amazon.awssdk.services.connect.model.UpdateContactAttributesRequest;
import software.amazon.awssdk.services.connect.model.UpdateContactAttributesResponse;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowContentRequest;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowContentResponse;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowMetadataRequest;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowMetadataResponse;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowModuleContentRequest;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowModuleContentResponse;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowModuleMetadataRequest;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowModuleMetadataResponse;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowNameRequest;
import software.amazon.awssdk.services.connect.model.UpdateContactFlowNameResponse;
import software.amazon.awssdk.services.connect.model.UpdateContactRequest;
import software.amazon.awssdk.services.connect.model.UpdateContactResponse;
import software.amazon.awssdk.services.connect.model.UpdateContactScheduleRequest;
import software.amazon.awssdk.services.connect.model.UpdateContactScheduleResponse;
import software.amazon.awssdk.services.connect.model.UpdateHoursOfOperationRequest;
import software.amazon.awssdk.services.connect.model.UpdateHoursOfOperationResponse;
import software.amazon.awssdk.services.connect.model.UpdateInstanceAttributeRequest;
import software.amazon.awssdk.services.connect.model.UpdateInstanceAttributeResponse;
import software.amazon.awssdk.services.connect.model.UpdateInstanceStorageConfigRequest;
import software.amazon.awssdk.services.connect.model.UpdateInstanceStorageConfigResponse;
import software.amazon.awssdk.services.connect.model.UpdatePhoneNumberRequest;
import software.amazon.awssdk.services.connect.model.UpdatePhoneNumberResponse;
import software.amazon.awssdk.services.connect.model.UpdateQueueHoursOfOperationRequest;
import software.amazon.awssdk.services.connect.model.UpdateQueueHoursOfOperationResponse;
import software.amazon.awssdk.services.connect.model.UpdateQueueMaxContactsRequest;
import software.amazon.awssdk.services.connect.model.UpdateQueueMaxContactsResponse;
import software.amazon.awssdk.services.connect.model.UpdateQueueNameRequest;
import software.amazon.awssdk.services.connect.model.UpdateQueueNameResponse;
import software.amazon.awssdk.services.connect.model.UpdateQueueOutboundCallerConfigRequest;
import software.amazon.awssdk.services.connect.model.UpdateQueueOutboundCallerConfigResponse;
import software.amazon.awssdk.services.connect.model.UpdateQueueStatusRequest;
import software.amazon.awssdk.services.connect.model.UpdateQueueStatusResponse;
import software.amazon.awssdk.services.connect.model.UpdateQuickConnectConfigRequest;
import software.amazon.awssdk.services.connect.model.UpdateQuickConnectConfigResponse;
import software.amazon.awssdk.services.connect.model.UpdateQuickConnectNameRequest;
import software.amazon.awssdk.services.connect.model.UpdateQuickConnectNameResponse;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileConcurrencyRequest;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileConcurrencyResponse;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileDefaultOutboundQueueRequest;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileDefaultOutboundQueueResponse;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileNameRequest;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileNameResponse;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileQueuesRequest;
import software.amazon.awssdk.services.connect.model.UpdateRoutingProfileQueuesResponse;
import software.amazon.awssdk.services.connect.model.UpdateRuleRequest;
import software.amazon.awssdk.services.connect.model.UpdateRuleResponse;
import software.amazon.awssdk.services.connect.model.UpdateSecurityProfileRequest;
import software.amazon.awssdk.services.connect.model.UpdateSecurityProfileResponse;
import software.amazon.awssdk.services.connect.model.UpdateTaskTemplateRequest;
import software.amazon.awssdk.services.connect.model.UpdateTaskTemplateResponse;
import software.amazon.awssdk.services.connect.model.UpdateTrafficDistributionRequest;
import software.amazon.awssdk.services.connect.model.UpdateTrafficDistributionResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserHierarchyGroupNameRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserHierarchyGroupNameResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserHierarchyRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserHierarchyResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserHierarchyStructureRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserHierarchyStructureResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserIdentityInfoRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserIdentityInfoResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserPhoneConfigRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserPhoneConfigResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserRoutingProfileRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserRoutingProfileResponse;
import software.amazon.awssdk.services.connect.model.UpdateUserSecurityProfilesRequest;
import software.amazon.awssdk.services.connect.model.UpdateUserSecurityProfilesResponse;
import software.amazon.awssdk.services.connect.paginators.GetCurrentMetricDataPublisher;
import software.amazon.awssdk.services.connect.paginators.GetCurrentUserDataPublisher;
import software.amazon.awssdk.services.connect.paginators.GetMetricDataPublisher;
import software.amazon.awssdk.services.connect.paginators.ListAgentStatusesPublisher;
import software.amazon.awssdk.services.connect.paginators.ListApprovedOriginsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListBotsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListContactFlowModulesPublisher;
import software.amazon.awssdk.services.connect.paginators.ListContactFlowsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListContactReferencesPublisher;
import software.amazon.awssdk.services.connect.paginators.ListDefaultVocabulariesPublisher;
import software.amazon.awssdk.services.connect.paginators.ListHoursOfOperationsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListInstanceAttributesPublisher;
import software.amazon.awssdk.services.connect.paginators.ListInstanceStorageConfigsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListInstancesPublisher;
import software.amazon.awssdk.services.connect.paginators.ListIntegrationAssociationsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListLambdaFunctionsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListLexBotsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListPhoneNumbersPublisher;
import software.amazon.awssdk.services.connect.paginators.ListPhoneNumbersV2Publisher;
import software.amazon.awssdk.services.connect.paginators.ListPromptsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListQueueQuickConnectsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListQueuesPublisher;
import software.amazon.awssdk.services.connect.paginators.ListQuickConnectsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListRoutingProfileQueuesPublisher;
import software.amazon.awssdk.services.connect.paginators.ListRoutingProfilesPublisher;
import software.amazon.awssdk.services.connect.paginators.ListRulesPublisher;
import software.amazon.awssdk.services.connect.paginators.ListSecurityKeysPublisher;
import software.amazon.awssdk.services.connect.paginators.ListSecurityProfilePermissionsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListSecurityProfilesPublisher;
import software.amazon.awssdk.services.connect.paginators.ListTaskTemplatesPublisher;
import software.amazon.awssdk.services.connect.paginators.ListTrafficDistributionGroupsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListUseCasesPublisher;
import software.amazon.awssdk.services.connect.paginators.ListUserHierarchyGroupsPublisher;
import software.amazon.awssdk.services.connect.paginators.ListUsersPublisher;
import software.amazon.awssdk.services.connect.paginators.SearchAvailablePhoneNumbersPublisher;
import software.amazon.awssdk.services.connect.paginators.SearchQueuesPublisher;
import software.amazon.awssdk.services.connect.paginators.SearchRoutingProfilesPublisher;
import software.amazon.awssdk.services.connect.paginators.SearchSecurityProfilesPublisher;
import software.amazon.awssdk.services.connect.paginators.SearchUsersPublisher;
import software.amazon.awssdk.services.connect.paginators.SearchVocabulariesPublisher;
/**
* Service client for accessing Amazon Connect asynchronously. This can be created using the static {@link #builder()}
* method.
*
*
* Amazon Connect is a cloud-based contact center solution that you use to set up and manage a customer contact center
* and provide reliable customer engagement at any scale.
*
*
* Amazon Connect provides metrics and real-time reporting that enable you to optimize contact routing. You can also
* resolve customer issues more efficiently by getting customers in touch with the appropriate agents.
*
*
* There are limits to the number of Amazon Connect resources that you can create. There are also limits to the number
* of requests that you can make per second. For more information, see Amazon Connect
* Service Quotas in the Amazon Connect Administrator Guide.
*
*
* You can connect programmatically to an Amazon Web Services service by using an endpoint. For a list of Amazon Connect
* endpoints, see Amazon Connect
* Endpoints.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface ConnectAsyncClient extends SdkClient {
String SERVICE_NAME = "connect";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "connect";
/**
* Create a {@link ConnectAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static ConnectAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link ConnectAsyncClient}.
*/
static ConnectAsyncClientBuilder builder() {
return new DefaultConnectAsyncClientBuilder();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Associates an approved origin to an Amazon Connect instance.
*
*
* @param associateApprovedOriginRequest
* @return A Java Future containing the result of the AssociateApprovedOrigin operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceConflictException A resource already has that name.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateApprovedOrigin
* @see AWS API Documentation
*/
default CompletableFuture associateApprovedOrigin(
AssociateApprovedOriginRequest associateApprovedOriginRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Associates an approved origin to an Amazon Connect instance.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateApprovedOriginRequest.Builder} avoiding
* the need to create one manually via {@link AssociateApprovedOriginRequest#builder()}
*
*
* @param associateApprovedOriginRequest
* A {@link Consumer} that will call methods on {@link AssociateApprovedOriginRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the AssociateApprovedOrigin operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceConflictException A resource already has that name.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateApprovedOrigin
* @see AWS API Documentation
*/
default CompletableFuture associateApprovedOrigin(
Consumer associateApprovedOriginRequest) {
return associateApprovedOrigin(AssociateApprovedOriginRequest.builder().applyMutation(associateApprovedOriginRequest)
.build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Allows the specified Amazon Connect instance to access the specified Amazon Lex or Amazon Lex V2 bot.
*
*
* @param associateBotRequest
* @return A Java Future containing the result of the AssociateBot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceConflictException A resource already has that name.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateBot
* @see AWS API
* Documentation
*/
default CompletableFuture associateBot(AssociateBotRequest associateBotRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Allows the specified Amazon Connect instance to access the specified Amazon Lex or Amazon Lex V2 bot.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateBotRequest.Builder} avoiding the need to
* create one manually via {@link AssociateBotRequest#builder()}
*
*
* @param associateBotRequest
* A {@link Consumer} that will call methods on {@link AssociateBotRequest.Builder} to create a request.
* @return A Java Future containing the result of the AssociateBot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceConflictException A resource already has that name.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateBot
* @see AWS API
* Documentation
*/
default CompletableFuture associateBot(Consumer associateBotRequest) {
return associateBot(AssociateBotRequest.builder().applyMutation(associateBotRequest).build());
}
/**
*
* Associates an existing vocabulary as the default. Contact Lens for Amazon Connect uses the vocabulary in
* post-call and real-time analysis sessions for the given language.
*
*
* @param associateDefaultVocabularyRequest
* @return A Java Future containing the result of the AssociateDefaultVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateDefaultVocabulary
* @see AWS API Documentation
*/
default CompletableFuture associateDefaultVocabulary(
AssociateDefaultVocabularyRequest associateDefaultVocabularyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates an existing vocabulary as the default. Contact Lens for Amazon Connect uses the vocabulary in
* post-call and real-time analysis sessions for the given language.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateDefaultVocabularyRequest.Builder} avoiding
* the need to create one manually via {@link AssociateDefaultVocabularyRequest#builder()}
*
*
* @param associateDefaultVocabularyRequest
* A {@link Consumer} that will call methods on {@link AssociateDefaultVocabularyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the AssociateDefaultVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateDefaultVocabulary
* @see AWS API Documentation
*/
default CompletableFuture associateDefaultVocabulary(
Consumer associateDefaultVocabularyRequest) {
return associateDefaultVocabulary(AssociateDefaultVocabularyRequest.builder()
.applyMutation(associateDefaultVocabularyRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Associates a storage resource type for the first time. You can only associate one type of storage configuration
* in a single call. This means, for example, that you can't define an instance with multiple S3 buckets for storing
* chat transcripts.
*
*
* This API does not create a resource that doesn't exist. It only associates it to the instance. Ensure that the
* resource being specified in the storage configuration, like an S3 bucket, exists when being used for association.
*
*
* @param associateInstanceStorageConfigRequest
* @return A Java Future containing the result of the AssociateInstanceStorageConfig operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceConflictException A resource already has that name.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateInstanceStorageConfig
* @see AWS API Documentation
*/
default CompletableFuture associateInstanceStorageConfig(
AssociateInstanceStorageConfigRequest associateInstanceStorageConfigRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Associates a storage resource type for the first time. You can only associate one type of storage configuration
* in a single call. This means, for example, that you can't define an instance with multiple S3 buckets for storing
* chat transcripts.
*
*
* This API does not create a resource that doesn't exist. It only associates it to the instance. Ensure that the
* resource being specified in the storage configuration, like an S3 bucket, exists when being used for association.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateInstanceStorageConfigRequest.Builder}
* avoiding the need to create one manually via {@link AssociateInstanceStorageConfigRequest#builder()}
*
*
* @param associateInstanceStorageConfigRequest
* A {@link Consumer} that will call methods on {@link AssociateInstanceStorageConfigRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the AssociateInstanceStorageConfig operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceConflictException A resource already has that name.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateInstanceStorageConfig
* @see AWS API Documentation
*/
default CompletableFuture associateInstanceStorageConfig(
Consumer associateInstanceStorageConfigRequest) {
return associateInstanceStorageConfig(AssociateInstanceStorageConfigRequest.builder()
.applyMutation(associateInstanceStorageConfigRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Allows the specified Amazon Connect instance to access the specified Lambda function.
*
*
* @param associateLambdaFunctionRequest
* @return A Java Future containing the result of the AssociateLambdaFunction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceConflictException A resource already has that name.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateLambdaFunction
* @see AWS API Documentation
*/
default CompletableFuture associateLambdaFunction(
AssociateLambdaFunctionRequest associateLambdaFunctionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Allows the specified Amazon Connect instance to access the specified Lambda function.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateLambdaFunctionRequest.Builder} avoiding
* the need to create one manually via {@link AssociateLambdaFunctionRequest#builder()}
*
*
* @param associateLambdaFunctionRequest
* A {@link Consumer} that will call methods on {@link AssociateLambdaFunctionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the AssociateLambdaFunction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceConflictException A resource already has that name.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateLambdaFunction
* @see AWS API Documentation
*/
default CompletableFuture associateLambdaFunction(
Consumer associateLambdaFunctionRequest) {
return associateLambdaFunction(AssociateLambdaFunctionRequest.builder().applyMutation(associateLambdaFunctionRequest)
.build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Allows the specified Amazon Connect instance to access the specified Amazon Lex bot.
*
*
* @param associateLexBotRequest
* @return A Java Future containing the result of the AssociateLexBot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceConflictException A resource already has that name.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateLexBot
* @see AWS API
* Documentation
*/
default CompletableFuture associateLexBot(AssociateLexBotRequest associateLexBotRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Allows the specified Amazon Connect instance to access the specified Amazon Lex bot.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateLexBotRequest.Builder} avoiding the need
* to create one manually via {@link AssociateLexBotRequest#builder()}
*
*
* @param associateLexBotRequest
* A {@link Consumer} that will call methods on {@link AssociateLexBotRequest.Builder} to create a request.
* @return A Java Future containing the result of the AssociateLexBot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceConflictException A resource already has that name.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateLexBot
* @see AWS API
* Documentation
*/
default CompletableFuture associateLexBot(
Consumer associateLexBotRequest) {
return associateLexBot(AssociateLexBotRequest.builder().applyMutation(associateLexBotRequest).build());
}
/**
*
* Associates a flow with a phone number claimed to your Amazon Connect instance.
*
*
*
* If the number is claimed to a traffic distribution group, and you are calling this API using an instance in the
* Amazon Web Services Region where the traffic distribution group was created, you can use either a full phone
* number ARN or UUID value for the PhoneNumberId
URI request parameter. However, if the number is
* claimed to a traffic distribution group and you are calling this API using an instance in the alternate Amazon
* Web Services Region associated with the traffic distribution group, you must provide a full phone number ARN. If
* a UUID is provided in this scenario, you will receive a ResourceNotFoundException
.
*
*
*
* @param associatePhoneNumberContactFlowRequest
* @return A Java Future containing the result of the AssociatePhoneNumberContactFlow operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociatePhoneNumberContactFlow
* @see AWS API Documentation
*/
default CompletableFuture associatePhoneNumberContactFlow(
AssociatePhoneNumberContactFlowRequest associatePhoneNumberContactFlowRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates a flow with a phone number claimed to your Amazon Connect instance.
*
*
*
* If the number is claimed to a traffic distribution group, and you are calling this API using an instance in the
* Amazon Web Services Region where the traffic distribution group was created, you can use either a full phone
* number ARN or UUID value for the PhoneNumberId
URI request parameter. However, if the number is
* claimed to a traffic distribution group and you are calling this API using an instance in the alternate Amazon
* Web Services Region associated with the traffic distribution group, you must provide a full phone number ARN. If
* a UUID is provided in this scenario, you will receive a ResourceNotFoundException
.
*
*
*
* This is a convenience which creates an instance of the {@link AssociatePhoneNumberContactFlowRequest.Builder}
* avoiding the need to create one manually via {@link AssociatePhoneNumberContactFlowRequest#builder()}
*
*
* @param associatePhoneNumberContactFlowRequest
* A {@link Consumer} that will call methods on {@link AssociatePhoneNumberContactFlowRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the AssociatePhoneNumberContactFlow operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociatePhoneNumberContactFlow
* @see AWS API Documentation
*/
default CompletableFuture associatePhoneNumberContactFlow(
Consumer associatePhoneNumberContactFlowRequest) {
return associatePhoneNumberContactFlow(AssociatePhoneNumberContactFlowRequest.builder()
.applyMutation(associatePhoneNumberContactFlowRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Associates a set of quick connects with a queue.
*
*
* @param associateQueueQuickConnectsRequest
* @return A Java Future containing the result of the AssociateQueueQuickConnects operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateQueueQuickConnects
* @see AWS API Documentation
*/
default CompletableFuture associateQueueQuickConnects(
AssociateQueueQuickConnectsRequest associateQueueQuickConnectsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Associates a set of quick connects with a queue.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateQueueQuickConnectsRequest.Builder}
* avoiding the need to create one manually via {@link AssociateQueueQuickConnectsRequest#builder()}
*
*
* @param associateQueueQuickConnectsRequest
* A {@link Consumer} that will call methods on {@link AssociateQueueQuickConnectsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the AssociateQueueQuickConnects operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateQueueQuickConnects
* @see AWS API Documentation
*/
default CompletableFuture associateQueueQuickConnects(
Consumer associateQueueQuickConnectsRequest) {
return associateQueueQuickConnects(AssociateQueueQuickConnectsRequest.builder()
.applyMutation(associateQueueQuickConnectsRequest).build());
}
/**
*
* Associates a set of queues with a routing profile.
*
*
* @param associateRoutingProfileQueuesRequest
* @return A Java Future containing the result of the AssociateRoutingProfileQueues operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateRoutingProfileQueues
* @see AWS API Documentation
*/
default CompletableFuture associateRoutingProfileQueues(
AssociateRoutingProfileQueuesRequest associateRoutingProfileQueuesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates a set of queues with a routing profile.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateRoutingProfileQueuesRequest.Builder}
* avoiding the need to create one manually via {@link AssociateRoutingProfileQueuesRequest#builder()}
*
*
* @param associateRoutingProfileQueuesRequest
* A {@link Consumer} that will call methods on {@link AssociateRoutingProfileQueuesRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the AssociateRoutingProfileQueues operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateRoutingProfileQueues
* @see AWS API Documentation
*/
default CompletableFuture associateRoutingProfileQueues(
Consumer associateRoutingProfileQueuesRequest) {
return associateRoutingProfileQueues(AssociateRoutingProfileQueuesRequest.builder()
.applyMutation(associateRoutingProfileQueuesRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Associates a security key to the instance.
*
*
* @param associateSecurityKeyRequest
* @return A Java Future containing the result of the AssociateSecurityKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceConflictException A resource already has that name.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateSecurityKey
* @see AWS
* API Documentation
*/
default CompletableFuture associateSecurityKey(
AssociateSecurityKeyRequest associateSecurityKeyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Associates a security key to the instance.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateSecurityKeyRequest.Builder} avoiding the
* need to create one manually via {@link AssociateSecurityKeyRequest#builder()}
*
*
* @param associateSecurityKeyRequest
* A {@link Consumer} that will call methods on {@link AssociateSecurityKeyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the AssociateSecurityKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - ResourceConflictException A resource already has that name.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.AssociateSecurityKey
* @see AWS
* API Documentation
*/
default CompletableFuture associateSecurityKey(
Consumer associateSecurityKeyRequest) {
return associateSecurityKey(AssociateSecurityKeyRequest.builder().applyMutation(associateSecurityKeyRequest).build());
}
/**
*
* Claims an available phone number to your Amazon Connect instance or traffic distribution group. You can call this
* API only in the same Amazon Web Services Region where the Amazon Connect instance or traffic distribution group
* was created.
*
*
* For more information about how to use this operation, see Claim a phone number in your
* country and Claim phone numbers to traffic distribution groups in the Amazon Connect Administrator Guide.
*
*
*
* You can call the SearchAvailablePhoneNumbers API for available phone numbers that you can claim. Call the DescribePhoneNumber API to verify the status of a previous ClaimPhoneNumber
* operation.
*
*
*
* @param claimPhoneNumberRequest
* @return A Java Future containing the result of the ClaimPhoneNumber operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - IdempotencyException An entity with the same name already exists.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.ClaimPhoneNumber
* @see AWS API
* Documentation
*/
default CompletableFuture claimPhoneNumber(ClaimPhoneNumberRequest claimPhoneNumberRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Claims an available phone number to your Amazon Connect instance or traffic distribution group. You can call this
* API only in the same Amazon Web Services Region where the Amazon Connect instance or traffic distribution group
* was created.
*
*
* For more information about how to use this operation, see Claim a phone number in your
* country and Claim phone numbers to traffic distribution groups in the Amazon Connect Administrator Guide.
*
*
*
* You can call the SearchAvailablePhoneNumbers API for available phone numbers that you can claim. Call the DescribePhoneNumber API to verify the status of a previous ClaimPhoneNumber
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ClaimPhoneNumberRequest.Builder} avoiding the need
* to create one manually via {@link ClaimPhoneNumberRequest#builder()}
*
*
* @param claimPhoneNumberRequest
* A {@link Consumer} that will call methods on {@link ClaimPhoneNumberRequest.Builder} to create a request.
* @return A Java Future containing the result of the ClaimPhoneNumber operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - IdempotencyException An entity with the same name already exists.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.ClaimPhoneNumber
* @see AWS API
* Documentation
*/
default CompletableFuture claimPhoneNumber(
Consumer claimPhoneNumberRequest) {
return claimPhoneNumber(ClaimPhoneNumberRequest.builder().applyMutation(claimPhoneNumberRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Creates an agent status for the specified Amazon Connect instance.
*
*
* @param createAgentStatusRequest
* @return A Java Future containing the result of the CreateAgentStatus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateAgentStatus
* @see AWS API
* Documentation
*/
default CompletableFuture createAgentStatus(CreateAgentStatusRequest createAgentStatusRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Creates an agent status for the specified Amazon Connect instance.
*
*
*
* This is a convenience which creates an instance of the {@link CreateAgentStatusRequest.Builder} avoiding the need
* to create one manually via {@link CreateAgentStatusRequest#builder()}
*
*
* @param createAgentStatusRequest
* A {@link Consumer} that will call methods on {@link CreateAgentStatusRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateAgentStatus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateAgentStatus
* @see AWS API
* Documentation
*/
default CompletableFuture createAgentStatus(
Consumer createAgentStatusRequest) {
return createAgentStatus(CreateAgentStatusRequest.builder().applyMutation(createAgentStatusRequest).build());
}
/**
*
* Creates a flow for the specified Amazon Connect instance.
*
*
* You can also create and update flows using the Amazon Connect Flow
* language.
*
*
* @param createContactFlowRequest
* @return A Java Future containing the result of the CreateContactFlow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidContactFlowException The flow is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - DuplicateResourceException A resource with the specified name already exists.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateContactFlow
* @see AWS API
* Documentation
*/
default CompletableFuture createContactFlow(CreateContactFlowRequest createContactFlowRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a flow for the specified Amazon Connect instance.
*
*
* You can also create and update flows using the Amazon Connect Flow
* language.
*
*
*
* This is a convenience which creates an instance of the {@link CreateContactFlowRequest.Builder} avoiding the need
* to create one manually via {@link CreateContactFlowRequest#builder()}
*
*
* @param createContactFlowRequest
* A {@link Consumer} that will call methods on {@link CreateContactFlowRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateContactFlow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidContactFlowException The flow is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - DuplicateResourceException A resource with the specified name already exists.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateContactFlow
* @see AWS API
* Documentation
*/
default CompletableFuture createContactFlow(
Consumer createContactFlowRequest) {
return createContactFlow(CreateContactFlowRequest.builder().applyMutation(createContactFlowRequest).build());
}
/**
*
* Creates a flow module for the specified Amazon Connect instance.
*
*
* @param createContactFlowModuleRequest
* @return A Java Future containing the result of the CreateContactFlowModule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - InvalidRequestException The request is not valid.
* - InvalidContactFlowModuleException The problems with the module. Please fix before trying again.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - DuplicateResourceException A resource with the specified name already exists.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - IdempotencyException An entity with the same name already exists.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateContactFlowModule
* @see AWS API Documentation
*/
default CompletableFuture createContactFlowModule(
CreateContactFlowModuleRequest createContactFlowModuleRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a flow module for the specified Amazon Connect instance.
*
*
*
* This is a convenience which creates an instance of the {@link CreateContactFlowModuleRequest.Builder} avoiding
* the need to create one manually via {@link CreateContactFlowModuleRequest#builder()}
*
*
* @param createContactFlowModuleRequest
* A {@link Consumer} that will call methods on {@link CreateContactFlowModuleRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateContactFlowModule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - InvalidRequestException The request is not valid.
* - InvalidContactFlowModuleException The problems with the module. Please fix before trying again.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - DuplicateResourceException A resource with the specified name already exists.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - IdempotencyException An entity with the same name already exists.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateContactFlowModule
* @see AWS API Documentation
*/
default CompletableFuture createContactFlowModule(
Consumer createContactFlowModuleRequest) {
return createContactFlowModule(CreateContactFlowModuleRequest.builder().applyMutation(createContactFlowModuleRequest)
.build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Creates hours of operation.
*
*
* @param createHoursOfOperationRequest
* @return A Java Future containing the result of the CreateHoursOfOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DuplicateResourceException A resource with the specified name already exists.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateHoursOfOperation
* @see AWS API Documentation
*/
default CompletableFuture createHoursOfOperation(
CreateHoursOfOperationRequest createHoursOfOperationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Creates hours of operation.
*
*
*
* This is a convenience which creates an instance of the {@link CreateHoursOfOperationRequest.Builder} avoiding the
* need to create one manually via {@link CreateHoursOfOperationRequest#builder()}
*
*
* @param createHoursOfOperationRequest
* A {@link Consumer} that will call methods on {@link CreateHoursOfOperationRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateHoursOfOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DuplicateResourceException A resource with the specified name already exists.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateHoursOfOperation
* @see AWS API Documentation
*/
default CompletableFuture createHoursOfOperation(
Consumer createHoursOfOperationRequest) {
return createHoursOfOperation(CreateHoursOfOperationRequest.builder().applyMutation(createHoursOfOperationRequest)
.build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Initiates an Amazon Connect instance with all the supported channels enabled. It does not attach any storage,
* such as Amazon Simple Storage Service (Amazon S3) or Amazon Kinesis. It also does not allow for any
* configurations on features, such as Contact Lens for Amazon Connect.
*
*
* Amazon Connect enforces a limit on the total number of instances that you can create or delete in 30 days. If you
* exceed this limit, you will get an error message indicating there has been an excessive number of attempts at
* creating or deleting instances. You must wait 30 days before you can restart creating and deleting instances in
* your account.
*
*
* @param createInstanceRequest
* @return A Java Future containing the result of the CreateInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateInstance
* @see AWS API
* Documentation
*/
default CompletableFuture createInstance(CreateInstanceRequest createInstanceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Initiates an Amazon Connect instance with all the supported channels enabled. It does not attach any storage,
* such as Amazon Simple Storage Service (Amazon S3) or Amazon Kinesis. It also does not allow for any
* configurations on features, such as Contact Lens for Amazon Connect.
*
*
* Amazon Connect enforces a limit on the total number of instances that you can create or delete in 30 days. If you
* exceed this limit, you will get an error message indicating there has been an excessive number of attempts at
* creating or deleting instances. You must wait 30 days before you can restart creating and deleting instances in
* your account.
*
*
*
* This is a convenience which creates an instance of the {@link CreateInstanceRequest.Builder} avoiding the need to
* create one manually via {@link CreateInstanceRequest#builder()}
*
*
* @param createInstanceRequest
* A {@link Consumer} that will call methods on {@link CreateInstanceRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateInstance
* @see AWS API
* Documentation
*/
default CompletableFuture createInstance(Consumer createInstanceRequest) {
return createInstance(CreateInstanceRequest.builder().applyMutation(createInstanceRequest).build());
}
/**
*
* Creates an Amazon Web Services resource association with an Amazon Connect instance.
*
*
* @param createIntegrationAssociationRequest
* @return A Java Future containing the result of the CreateIntegrationAssociation operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateIntegrationAssociation
* @see AWS API Documentation
*/
default CompletableFuture createIntegrationAssociation(
CreateIntegrationAssociationRequest createIntegrationAssociationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an Amazon Web Services resource association with an Amazon Connect instance.
*
*
*
* This is a convenience which creates an instance of the {@link CreateIntegrationAssociationRequest.Builder}
* avoiding the need to create one manually via {@link CreateIntegrationAssociationRequest#builder()}
*
*
* @param createIntegrationAssociationRequest
* A {@link Consumer} that will call methods on {@link CreateIntegrationAssociationRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the CreateIntegrationAssociation operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateIntegrationAssociation
* @see AWS API Documentation
*/
default CompletableFuture createIntegrationAssociation(
Consumer createIntegrationAssociationRequest) {
return createIntegrationAssociation(CreateIntegrationAssociationRequest.builder()
.applyMutation(createIntegrationAssociationRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Creates a new queue for the specified Amazon Connect instance.
*
*
*
* If the number being used in the input is claimed to a traffic distribution group, and you are calling this API
* using an instance in the Amazon Web Services Region where the traffic distribution group was created, you can use
* either a full phone number ARN or UUID value for the OutboundCallerIdNumberId
value of the OutboundCallerConfig
* request body parameter. However, if the number is claimed to a traffic distribution group and you are calling
* this API using an instance in the alternate Amazon Web Services Region associated with the traffic distribution
* group, you must provide a full phone number ARN. If a UUID is provided in this scenario, you will receive a
* ResourceNotFoundException
.
*
*
*
* @param createQueueRequest
* @return A Java Future containing the result of the CreateQueue operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateQueue
* @see AWS API
* Documentation
*/
default CompletableFuture createQueue(CreateQueueRequest createQueueRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Creates a new queue for the specified Amazon Connect instance.
*
*
*
* If the number being used in the input is claimed to a traffic distribution group, and you are calling this API
* using an instance in the Amazon Web Services Region where the traffic distribution group was created, you can use
* either a full phone number ARN or UUID value for the OutboundCallerIdNumberId
value of the OutboundCallerConfig
* request body parameter. However, if the number is claimed to a traffic distribution group and you are calling
* this API using an instance in the alternate Amazon Web Services Region associated with the traffic distribution
* group, you must provide a full phone number ARN. If a UUID is provided in this scenario, you will receive a
* ResourceNotFoundException
.
*
*
*
* This is a convenience which creates an instance of the {@link CreateQueueRequest.Builder} avoiding the need to
* create one manually via {@link CreateQueueRequest#builder()}
*
*
* @param createQueueRequest
* A {@link Consumer} that will call methods on {@link CreateQueueRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateQueue operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateQueue
* @see AWS API
* Documentation
*/
default CompletableFuture createQueue(Consumer createQueueRequest) {
return createQueue(CreateQueueRequest.builder().applyMutation(createQueueRequest).build());
}
/**
*
* Creates a quick connect for the specified Amazon Connect instance.
*
*
* @param createQuickConnectRequest
* @return A Java Future containing the result of the CreateQuickConnect operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateQuickConnect
* @see AWS
* API Documentation
*/
default CompletableFuture createQuickConnect(CreateQuickConnectRequest createQuickConnectRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a quick connect for the specified Amazon Connect instance.
*
*
*
* This is a convenience which creates an instance of the {@link CreateQuickConnectRequest.Builder} avoiding the
* need to create one manually via {@link CreateQuickConnectRequest#builder()}
*
*
* @param createQuickConnectRequest
* A {@link Consumer} that will call methods on {@link CreateQuickConnectRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateQuickConnect operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateQuickConnect
* @see AWS
* API Documentation
*/
default CompletableFuture createQuickConnect(
Consumer createQuickConnectRequest) {
return createQuickConnect(CreateQuickConnectRequest.builder().applyMutation(createQuickConnectRequest).build());
}
/**
*
* Creates a new routing profile.
*
*
* @param createRoutingProfileRequest
* @return A Java Future containing the result of the CreateRoutingProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateRoutingProfile
* @see AWS
* API Documentation
*/
default CompletableFuture createRoutingProfile(
CreateRoutingProfileRequest createRoutingProfileRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new routing profile.
*
*
*
* This is a convenience which creates an instance of the {@link CreateRoutingProfileRequest.Builder} avoiding the
* need to create one manually via {@link CreateRoutingProfileRequest#builder()}
*
*
* @param createRoutingProfileRequest
* A {@link Consumer} that will call methods on {@link CreateRoutingProfileRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateRoutingProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateRoutingProfile
* @see AWS
* API Documentation
*/
default CompletableFuture createRoutingProfile(
Consumer createRoutingProfileRequest) {
return createRoutingProfile(CreateRoutingProfileRequest.builder().applyMutation(createRoutingProfileRequest).build());
}
/**
*
* Creates a rule for the specified Amazon Connect instance.
*
*
* Use the Rules
* Function language to code conditions for the rule.
*
*
* @param createRuleRequest
* @return A Java Future containing the result of the CreateRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - ResourceConflictException A resource already has that name.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateRule
* @see AWS API
* Documentation
*/
default CompletableFuture createRule(CreateRuleRequest createRuleRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a rule for the specified Amazon Connect instance.
*
*
* Use the Rules
* Function language to code conditions for the rule.
*
*
*
* This is a convenience which creates an instance of the {@link CreateRuleRequest.Builder} avoiding the need to
* create one manually via {@link CreateRuleRequest#builder()}
*
*
* @param createRuleRequest
* A {@link Consumer} that will call methods on {@link CreateRuleRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - ResourceConflictException A resource already has that name.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateRule
* @see AWS API
* Documentation
*/
default CompletableFuture createRule(Consumer createRuleRequest) {
return createRule(CreateRuleRequest.builder().applyMutation(createRuleRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Creates a security profile.
*
*
* @param createSecurityProfileRequest
* @return A Java Future containing the result of the CreateSecurityProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateSecurityProfile
* @see AWS
* API Documentation
*/
default CompletableFuture createSecurityProfile(
CreateSecurityProfileRequest createSecurityProfileRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Creates a security profile.
*
*
*
* This is a convenience which creates an instance of the {@link CreateSecurityProfileRequest.Builder} avoiding the
* need to create one manually via {@link CreateSecurityProfileRequest#builder()}
*
*
* @param createSecurityProfileRequest
* A {@link Consumer} that will call methods on {@link CreateSecurityProfileRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateSecurityProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateSecurityProfile
* @see AWS
* API Documentation
*/
default CompletableFuture createSecurityProfile(
Consumer createSecurityProfileRequest) {
return createSecurityProfile(CreateSecurityProfileRequest.builder().applyMutation(createSecurityProfileRequest).build());
}
/**
*
* Creates a new task template in the specified Amazon Connect instance.
*
*
* @param createTaskTemplateRequest
* @return A Java Future containing the result of the CreateTaskTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - PropertyValidationException The property is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateTaskTemplate
* @see AWS
* API Documentation
*/
default CompletableFuture createTaskTemplate(CreateTaskTemplateRequest createTaskTemplateRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new task template in the specified Amazon Connect instance.
*
*
*
* This is a convenience which creates an instance of the {@link CreateTaskTemplateRequest.Builder} avoiding the
* need to create one manually via {@link CreateTaskTemplateRequest#builder()}
*
*
* @param createTaskTemplateRequest
* A {@link Consumer} that will call methods on {@link CreateTaskTemplateRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateTaskTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - PropertyValidationException The property is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateTaskTemplate
* @see AWS
* API Documentation
*/
default CompletableFuture createTaskTemplate(
Consumer createTaskTemplateRequest) {
return createTaskTemplate(CreateTaskTemplateRequest.builder().applyMutation(createTaskTemplateRequest).build());
}
/**
*
* Creates a traffic distribution group given an Amazon Connect instance that has been replicated.
*
*
* For more information about creating traffic distribution groups, see Set up
* traffic distribution groups in the Amazon Connect Administrator Guide.
*
*
* @param createTrafficDistributionGroupRequest
* @return A Java Future containing the result of the CreateTrafficDistributionGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ResourceConflictException A resource already has that name.
* - ResourceNotReadyException The resource is not ready.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateTrafficDistributionGroup
* @see AWS API Documentation
*/
default CompletableFuture createTrafficDistributionGroup(
CreateTrafficDistributionGroupRequest createTrafficDistributionGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a traffic distribution group given an Amazon Connect instance that has been replicated.
*
*
* For more information about creating traffic distribution groups, see Set up
* traffic distribution groups in the Amazon Connect Administrator Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateTrafficDistributionGroupRequest.Builder}
* avoiding the need to create one manually via {@link CreateTrafficDistributionGroupRequest#builder()}
*
*
* @param createTrafficDistributionGroupRequest
* A {@link Consumer} that will call methods on {@link CreateTrafficDistributionGroupRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the CreateTrafficDistributionGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - ThrottlingException The throttling limit has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ResourceConflictException A resource already has that name.
* - ResourceNotReadyException The resource is not ready.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateTrafficDistributionGroup
* @see AWS API Documentation
*/
default CompletableFuture createTrafficDistributionGroup(
Consumer createTrafficDistributionGroupRequest) {
return createTrafficDistributionGroup(CreateTrafficDistributionGroupRequest.builder()
.applyMutation(createTrafficDistributionGroupRequest).build());
}
/**
*
* Creates a use case for an integration association.
*
*
* @param createUseCaseRequest
* @return A Java Future containing the result of the CreateUseCase operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateUseCase
* @see AWS API
* Documentation
*/
default CompletableFuture createUseCase(CreateUseCaseRequest createUseCaseRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a use case for an integration association.
*
*
*
* This is a convenience which creates an instance of the {@link CreateUseCaseRequest.Builder} avoiding the need to
* create one manually via {@link CreateUseCaseRequest#builder()}
*
*
* @param createUseCaseRequest
* A {@link Consumer} that will call methods on {@link CreateUseCaseRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateUseCase operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateUseCase
* @see AWS API
* Documentation
*/
default CompletableFuture createUseCase(Consumer createUseCaseRequest) {
return createUseCase(CreateUseCaseRequest.builder().applyMutation(createUseCaseRequest).build());
}
/**
*
* Creates a user account for the specified Amazon Connect instance.
*
*
* For information about how to create user accounts using the Amazon Connect console, see Add Users in the Amazon
* Connect Administrator Guide.
*
*
* @param createUserRequest
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateUser
* @see AWS API
* Documentation
*/
default CompletableFuture createUser(CreateUserRequest createUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a user account for the specified Amazon Connect instance.
*
*
* For information about how to create user accounts using the Amazon Connect console, see Add Users in the Amazon
* Connect Administrator Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateUserRequest.Builder} avoiding the need to
* create one manually via {@link CreateUserRequest#builder()}
*
*
* @param createUserRequest
* A {@link Consumer} that will call methods on {@link CreateUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - DuplicateResourceException A resource with the specified name already exists.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateUser
* @see AWS API
* Documentation
*/
default CompletableFuture createUser(Consumer createUserRequest) {
return createUser(CreateUserRequest.builder().applyMutation(createUserRequest).build());
}
/**
*
* Creates a new user hierarchy group.
*
*
* @param createUserHierarchyGroupRequest
* @return A Java Future containing the result of the CreateUserHierarchyGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - DuplicateResourceException A resource with the specified name already exists.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateUserHierarchyGroup
* @see AWS API Documentation
*/
default CompletableFuture createUserHierarchyGroup(
CreateUserHierarchyGroupRequest createUserHierarchyGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new user hierarchy group.
*
*
*
* This is a convenience which creates an instance of the {@link CreateUserHierarchyGroupRequest.Builder} avoiding
* the need to create one manually via {@link CreateUserHierarchyGroupRequest#builder()}
*
*
* @param createUserHierarchyGroupRequest
* A {@link Consumer} that will call methods on {@link CreateUserHierarchyGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateUserHierarchyGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - DuplicateResourceException A resource with the specified name already exists.
* - LimitExceededException The allowed limit for the resource has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateUserHierarchyGroup
* @see AWS API Documentation
*/
default CompletableFuture createUserHierarchyGroup(
Consumer createUserHierarchyGroupRequest) {
return createUserHierarchyGroup(CreateUserHierarchyGroupRequest.builder().applyMutation(createUserHierarchyGroupRequest)
.build());
}
/**
*
* Creates a custom vocabulary associated with your Amazon Connect instance. You can set a custom vocabulary to be
* your default vocabulary for a given language. Contact Lens for Amazon Connect uses the default vocabulary in
* post-call and real-time contact analysis sessions for that language.
*
*
* @param createVocabularyRequest
* @return A Java Future containing the result of the CreateVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - ResourceConflictException A resource already has that name.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateVocabulary
* @see AWS API
* Documentation
*/
default CompletableFuture createVocabulary(CreateVocabularyRequest createVocabularyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a custom vocabulary associated with your Amazon Connect instance. You can set a custom vocabulary to be
* your default vocabulary for a given language. Contact Lens for Amazon Connect uses the default vocabulary in
* post-call and real-time contact analysis sessions for that language.
*
*
*
* This is a convenience which creates an instance of the {@link CreateVocabularyRequest.Builder} avoiding the need
* to create one manually via {@link CreateVocabularyRequest#builder()}
*
*
* @param createVocabularyRequest
* A {@link Consumer} that will call methods on {@link CreateVocabularyRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - ResourceConflictException A resource already has that name.
* - ServiceQuotaExceededException The service quota has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.CreateVocabulary
* @see AWS API
* Documentation
*/
default CompletableFuture createVocabulary(
Consumer createVocabularyRequest) {
return createVocabulary(CreateVocabularyRequest.builder().applyMutation(createVocabularyRequest).build());
}
/**
*
* Deletes a flow for the specified Amazon Connect instance.
*
*
* @param deleteContactFlowRequest
* @return A Java Future containing the result of the DeleteContactFlow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteContactFlow
* @see AWS API
* Documentation
*/
default CompletableFuture deleteContactFlow(DeleteContactFlowRequest deleteContactFlowRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a flow for the specified Amazon Connect instance.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteContactFlowRequest.Builder} avoiding the need
* to create one manually via {@link DeleteContactFlowRequest#builder()}
*
*
* @param deleteContactFlowRequest
* A {@link Consumer} that will call methods on {@link DeleteContactFlowRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteContactFlow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteContactFlow
* @see AWS API
* Documentation
*/
default CompletableFuture deleteContactFlow(
Consumer deleteContactFlowRequest) {
return deleteContactFlow(DeleteContactFlowRequest.builder().applyMutation(deleteContactFlowRequest).build());
}
/**
*
* Deletes the specified flow module.
*
*
* @param deleteContactFlowModuleRequest
* @return A Java Future containing the result of the DeleteContactFlowModule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteContactFlowModule
* @see AWS API Documentation
*/
default CompletableFuture deleteContactFlowModule(
DeleteContactFlowModuleRequest deleteContactFlowModuleRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified flow module.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteContactFlowModuleRequest.Builder} avoiding
* the need to create one manually via {@link DeleteContactFlowModuleRequest#builder()}
*
*
* @param deleteContactFlowModuleRequest
* A {@link Consumer} that will call methods on {@link DeleteContactFlowModuleRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteContactFlowModule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteContactFlowModule
* @see AWS API Documentation
*/
default CompletableFuture deleteContactFlowModule(
Consumer deleteContactFlowModuleRequest) {
return deleteContactFlowModule(DeleteContactFlowModuleRequest.builder().applyMutation(deleteContactFlowModuleRequest)
.build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Deletes an hours of operation.
*
*
* @param deleteHoursOfOperationRequest
* @return A Java Future containing the result of the DeleteHoursOfOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteHoursOfOperation
* @see AWS API Documentation
*/
default CompletableFuture deleteHoursOfOperation(
DeleteHoursOfOperationRequest deleteHoursOfOperationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Deletes an hours of operation.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteHoursOfOperationRequest.Builder} avoiding the
* need to create one manually via {@link DeleteHoursOfOperationRequest#builder()}
*
*
* @param deleteHoursOfOperationRequest
* A {@link Consumer} that will call methods on {@link DeleteHoursOfOperationRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteHoursOfOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteHoursOfOperation
* @see AWS API Documentation
*/
default CompletableFuture deleteHoursOfOperation(
Consumer deleteHoursOfOperationRequest) {
return deleteHoursOfOperation(DeleteHoursOfOperationRequest.builder().applyMutation(deleteHoursOfOperationRequest)
.build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Deletes the Amazon Connect instance.
*
*
* Amazon Connect enforces a limit on the total number of instances that you can create or delete in 30 days. If you
* exceed this limit, you will get an error message indicating there has been an excessive number of attempts at
* creating or deleting instances. You must wait 30 days before you can restart creating and deleting instances in
* your account.
*
*
* @param deleteInstanceRequest
* @return A Java Future containing the result of the DeleteInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteInstance
* @see AWS API
* Documentation
*/
default CompletableFuture deleteInstance(DeleteInstanceRequest deleteInstanceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Deletes the Amazon Connect instance.
*
*
* Amazon Connect enforces a limit on the total number of instances that you can create or delete in 30 days. If you
* exceed this limit, you will get an error message indicating there has been an excessive number of attempts at
* creating or deleting instances. You must wait 30 days before you can restart creating and deleting instances in
* your account.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteInstanceRequest.Builder} avoiding the need to
* create one manually via {@link DeleteInstanceRequest#builder()}
*
*
* @param deleteInstanceRequest
* A {@link Consumer} that will call methods on {@link DeleteInstanceRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteInstance
* @see AWS API
* Documentation
*/
default CompletableFuture deleteInstance(Consumer deleteInstanceRequest) {
return deleteInstance(DeleteInstanceRequest.builder().applyMutation(deleteInstanceRequest).build());
}
/**
*
* Deletes an Amazon Web Services resource association from an Amazon Connect instance. The association must not
* have any use cases associated with it.
*
*
* @param deleteIntegrationAssociationRequest
* @return A Java Future containing the result of the DeleteIntegrationAssociation operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteIntegrationAssociation
* @see AWS API Documentation
*/
default CompletableFuture deleteIntegrationAssociation(
DeleteIntegrationAssociationRequest deleteIntegrationAssociationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an Amazon Web Services resource association from an Amazon Connect instance. The association must not
* have any use cases associated with it.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteIntegrationAssociationRequest.Builder}
* avoiding the need to create one manually via {@link DeleteIntegrationAssociationRequest#builder()}
*
*
* @param deleteIntegrationAssociationRequest
* A {@link Consumer} that will call methods on {@link DeleteIntegrationAssociationRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the DeleteIntegrationAssociation operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteIntegrationAssociation
* @see AWS API Documentation
*/
default CompletableFuture deleteIntegrationAssociation(
Consumer deleteIntegrationAssociationRequest) {
return deleteIntegrationAssociation(DeleteIntegrationAssociationRequest.builder()
.applyMutation(deleteIntegrationAssociationRequest).build());
}
/**
*
* Deletes a quick connect.
*
*
* @param deleteQuickConnectRequest
* @return A Java Future containing the result of the DeleteQuickConnect operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteQuickConnect
* @see AWS
* API Documentation
*/
default CompletableFuture deleteQuickConnect(DeleteQuickConnectRequest deleteQuickConnectRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a quick connect.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteQuickConnectRequest.Builder} avoiding the
* need to create one manually via {@link DeleteQuickConnectRequest#builder()}
*
*
* @param deleteQuickConnectRequest
* A {@link Consumer} that will call methods on {@link DeleteQuickConnectRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteQuickConnect operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteQuickConnect
* @see AWS
* API Documentation
*/
default CompletableFuture deleteQuickConnect(
Consumer deleteQuickConnectRequest) {
return deleteQuickConnect(DeleteQuickConnectRequest.builder().applyMutation(deleteQuickConnectRequest).build());
}
/**
*
* Deletes a rule for the specified Amazon Connect instance.
*
*
* @param deleteRuleRequest
* @return A Java Future containing the result of the DeleteRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteRule
* @see AWS API
* Documentation
*/
default CompletableFuture deleteRule(DeleteRuleRequest deleteRuleRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a rule for the specified Amazon Connect instance.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRuleRequest.Builder} avoiding the need to
* create one manually via {@link DeleteRuleRequest#builder()}
*
*
* @param deleteRuleRequest
* A {@link Consumer} that will call methods on {@link DeleteRuleRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteRule
* @see AWS API
* Documentation
*/
default CompletableFuture deleteRule(Consumer deleteRuleRequest) {
return deleteRule(DeleteRuleRequest.builder().applyMutation(deleteRuleRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Deletes a security profile.
*
*
* @param deleteSecurityProfileRequest
* @return A Java Future containing the result of the DeleteSecurityProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - ResourceInUseException That resource is already in use. Please try another.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteSecurityProfile
* @see AWS
* API Documentation
*/
default CompletableFuture deleteSecurityProfile(
DeleteSecurityProfileRequest deleteSecurityProfileRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Deletes a security profile.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSecurityProfileRequest.Builder} avoiding the
* need to create one manually via {@link DeleteSecurityProfileRequest#builder()}
*
*
* @param deleteSecurityProfileRequest
* A {@link Consumer} that will call methods on {@link DeleteSecurityProfileRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteSecurityProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - ResourceInUseException That resource is already in use. Please try another.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteSecurityProfile
* @see AWS
* API Documentation
*/
default CompletableFuture deleteSecurityProfile(
Consumer deleteSecurityProfileRequest) {
return deleteSecurityProfile(DeleteSecurityProfileRequest.builder().applyMutation(deleteSecurityProfileRequest).build());
}
/**
*
* Deletes the task template.
*
*
* @param deleteTaskTemplateRequest
* @return A Java Future containing the result of the DeleteTaskTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteTaskTemplate
* @see AWS
* API Documentation
*/
default CompletableFuture deleteTaskTemplate(DeleteTaskTemplateRequest deleteTaskTemplateRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the task template.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteTaskTemplateRequest.Builder} avoiding the
* need to create one manually via {@link DeleteTaskTemplateRequest#builder()}
*
*
* @param deleteTaskTemplateRequest
* A {@link Consumer} that will call methods on {@link DeleteTaskTemplateRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteTaskTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteTaskTemplate
* @see AWS
* API Documentation
*/
default CompletableFuture deleteTaskTemplate(
Consumer deleteTaskTemplateRequest) {
return deleteTaskTemplate(DeleteTaskTemplateRequest.builder().applyMutation(deleteTaskTemplateRequest).build());
}
/**
*
* Deletes a traffic distribution group. This API can be called only in the Region where the traffic distribution
* group is created.
*
*
* For more information about deleting traffic distribution groups, see Delete
* traffic distribution groups in the Amazon Connect Administrator Guide.
*
*
* @param deleteTrafficDistributionGroupRequest
* @return A Java Future containing the result of the DeleteTrafficDistributionGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - ResourceInUseException That resource is already in use. Please try another.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteTrafficDistributionGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteTrafficDistributionGroup(
DeleteTrafficDistributionGroupRequest deleteTrafficDistributionGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a traffic distribution group. This API can be called only in the Region where the traffic distribution
* group is created.
*
*
* For more information about deleting traffic distribution groups, see Delete
* traffic distribution groups in the Amazon Connect Administrator Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteTrafficDistributionGroupRequest.Builder}
* avoiding the need to create one manually via {@link DeleteTrafficDistributionGroupRequest#builder()}
*
*
* @param deleteTrafficDistributionGroupRequest
* A {@link Consumer} that will call methods on {@link DeleteTrafficDistributionGroupRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteTrafficDistributionGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - ResourceInUseException That resource is already in use. Please try another.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteTrafficDistributionGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteTrafficDistributionGroup(
Consumer deleteTrafficDistributionGroupRequest) {
return deleteTrafficDistributionGroup(DeleteTrafficDistributionGroupRequest.builder()
.applyMutation(deleteTrafficDistributionGroupRequest).build());
}
/**
*
* Deletes a use case from an integration association.
*
*
* @param deleteUseCaseRequest
* @return A Java Future containing the result of the DeleteUseCase operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteUseCase
* @see AWS API
* Documentation
*/
default CompletableFuture deleteUseCase(DeleteUseCaseRequest deleteUseCaseRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a use case from an integration association.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUseCaseRequest.Builder} avoiding the need to
* create one manually via {@link DeleteUseCaseRequest#builder()}
*
*
* @param deleteUseCaseRequest
* A {@link Consumer} that will call methods on {@link DeleteUseCaseRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteUseCase operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteUseCase
* @see AWS API
* Documentation
*/
default CompletableFuture deleteUseCase(Consumer deleteUseCaseRequest) {
return deleteUseCase(DeleteUseCaseRequest.builder().applyMutation(deleteUseCaseRequest).build());
}
/**
*
* Deletes a user account from the specified Amazon Connect instance.
*
*
* For information about what happens to a user's data when their account is deleted, see Delete Users from Your Amazon
* Connect Instance in the Amazon Connect Administrator Guide.
*
*
* @param deleteUserRequest
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteUser
* @see AWS API
* Documentation
*/
default CompletableFuture deleteUser(DeleteUserRequest deleteUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a user account from the specified Amazon Connect instance.
*
*
* For information about what happens to a user's data when their account is deleted, see Delete Users from Your Amazon
* Connect Instance in the Amazon Connect Administrator Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUserRequest.Builder} avoiding the need to
* create one manually via {@link DeleteUserRequest#builder()}
*
*
* @param deleteUserRequest
* A {@link Consumer} that will call methods on {@link DeleteUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteUser
* @see AWS API
* Documentation
*/
default CompletableFuture deleteUser(Consumer deleteUserRequest) {
return deleteUser(DeleteUserRequest.builder().applyMutation(deleteUserRequest).build());
}
/**
*
* Deletes an existing user hierarchy group. It must not be associated with any agents or have any active child
* groups.
*
*
* @param deleteUserHierarchyGroupRequest
* @return A Java Future containing the result of the DeleteUserHierarchyGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ResourceInUseException That resource is already in use. Please try another.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteUserHierarchyGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteUserHierarchyGroup(
DeleteUserHierarchyGroupRequest deleteUserHierarchyGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an existing user hierarchy group. It must not be associated with any agents or have any active child
* groups.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUserHierarchyGroupRequest.Builder} avoiding
* the need to create one manually via {@link DeleteUserHierarchyGroupRequest#builder()}
*
*
* @param deleteUserHierarchyGroupRequest
* A {@link Consumer} that will call methods on {@link DeleteUserHierarchyGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteUserHierarchyGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ResourceInUseException That resource is already in use. Please try another.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteUserHierarchyGroup
* @see AWS API Documentation
*/
default CompletableFuture deleteUserHierarchyGroup(
Consumer deleteUserHierarchyGroupRequest) {
return deleteUserHierarchyGroup(DeleteUserHierarchyGroupRequest.builder().applyMutation(deleteUserHierarchyGroupRequest)
.build());
}
/**
*
* Deletes the vocabulary that has the given identifier.
*
*
* @param deleteVocabularyRequest
* @return A Java Future containing the result of the DeleteVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - ResourceInUseException That resource is already in use. Please try another.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteVocabulary
* @see AWS API
* Documentation
*/
default CompletableFuture deleteVocabulary(DeleteVocabularyRequest deleteVocabularyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the vocabulary that has the given identifier.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteVocabularyRequest.Builder} avoiding the need
* to create one manually via {@link DeleteVocabularyRequest#builder()}
*
*
* @param deleteVocabularyRequest
* A {@link Consumer} that will call methods on {@link DeleteVocabularyRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - ResourceInUseException That resource is already in use. Please try another.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DeleteVocabulary
* @see AWS API
* Documentation
*/
default CompletableFuture deleteVocabulary(
Consumer deleteVocabularyRequest) {
return deleteVocabulary(DeleteVocabularyRequest.builder().applyMutation(deleteVocabularyRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Describes an agent status.
*
*
* @param describeAgentStatusRequest
* @return A Java Future containing the result of the DescribeAgentStatus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeAgentStatus
* @see AWS
* API Documentation
*/
default CompletableFuture describeAgentStatus(
DescribeAgentStatusRequest describeAgentStatusRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Describes an agent status.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAgentStatusRequest.Builder} avoiding the
* need to create one manually via {@link DescribeAgentStatusRequest#builder()}
*
*
* @param describeAgentStatusRequest
* A {@link Consumer} that will call methods on {@link DescribeAgentStatusRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeAgentStatus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeAgentStatus
* @see AWS
* API Documentation
*/
default CompletableFuture describeAgentStatus(
Consumer describeAgentStatusRequest) {
return describeAgentStatus(DescribeAgentStatusRequest.builder().applyMutation(describeAgentStatusRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Describes the specified contact.
*
*
*
* Contact information remains available in Amazon Connect for 24 months, and then it is deleted.
*
*
* Only data from November 12, 2021, and later is returned by this API.
*
*
*
* @param describeContactRequest
* @return A Java Future containing the result of the DescribeContact operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeContact
* @see AWS API
* Documentation
*/
default CompletableFuture describeContact(DescribeContactRequest describeContactRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Describes the specified contact.
*
*
*
* Contact information remains available in Amazon Connect for 24 months, and then it is deleted.
*
*
* Only data from November 12, 2021, and later is returned by this API.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeContactRequest.Builder} avoiding the need
* to create one manually via {@link DescribeContactRequest#builder()}
*
*
* @param describeContactRequest
* A {@link Consumer} that will call methods on {@link DescribeContactRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeContact operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeContact
* @see AWS API
* Documentation
*/
default CompletableFuture describeContact(
Consumer describeContactRequest) {
return describeContact(DescribeContactRequest.builder().applyMutation(describeContactRequest).build());
}
/**
*
* Describes the specified flow.
*
*
* You can also create and update flows using the Amazon Connect Flow
* language.
*
*
* @param describeContactFlowRequest
* @return A Java Future containing the result of the DescribeContactFlow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ContactFlowNotPublishedException The flow has not been published.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeContactFlow
* @see AWS
* API Documentation
*/
default CompletableFuture describeContactFlow(
DescribeContactFlowRequest describeContactFlowRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the specified flow.
*
*
* You can also create and update flows using the Amazon Connect Flow
* language.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeContactFlowRequest.Builder} avoiding the
* need to create one manually via {@link DescribeContactFlowRequest#builder()}
*
*
* @param describeContactFlowRequest
* A {@link Consumer} that will call methods on {@link DescribeContactFlowRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeContactFlow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ContactFlowNotPublishedException The flow has not been published.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeContactFlow
* @see AWS
* API Documentation
*/
default CompletableFuture describeContactFlow(
Consumer describeContactFlowRequest) {
return describeContactFlow(DescribeContactFlowRequest.builder().applyMutation(describeContactFlowRequest).build());
}
/**
*
* Describes the specified flow module.
*
*
* @param describeContactFlowModuleRequest
* @return A Java Future containing the result of the DescribeContactFlowModule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeContactFlowModule
* @see AWS API Documentation
*/
default CompletableFuture describeContactFlowModule(
DescribeContactFlowModuleRequest describeContactFlowModuleRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the specified flow module.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeContactFlowModuleRequest.Builder} avoiding
* the need to create one manually via {@link DescribeContactFlowModuleRequest#builder()}
*
*
* @param describeContactFlowModuleRequest
* A {@link Consumer} that will call methods on {@link DescribeContactFlowModuleRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeContactFlowModule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeContactFlowModule
* @see AWS API Documentation
*/
default CompletableFuture describeContactFlowModule(
Consumer describeContactFlowModuleRequest) {
return describeContactFlowModule(DescribeContactFlowModuleRequest.builder()
.applyMutation(describeContactFlowModuleRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Describes the hours of operation.
*
*
* @param describeHoursOfOperationRequest
* @return A Java Future containing the result of the DescribeHoursOfOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeHoursOfOperation
* @see AWS API Documentation
*/
default CompletableFuture describeHoursOfOperation(
DescribeHoursOfOperationRequest describeHoursOfOperationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Describes the hours of operation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeHoursOfOperationRequest.Builder} avoiding
* the need to create one manually via {@link DescribeHoursOfOperationRequest#builder()}
*
*
* @param describeHoursOfOperationRequest
* A {@link Consumer} that will call methods on {@link DescribeHoursOfOperationRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeHoursOfOperation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeHoursOfOperation
* @see AWS API Documentation
*/
default CompletableFuture describeHoursOfOperation(
Consumer describeHoursOfOperationRequest) {
return describeHoursOfOperation(DescribeHoursOfOperationRequest.builder().applyMutation(describeHoursOfOperationRequest)
.build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Returns the current state of the specified instance identifier. It tracks the instance while it is being created
* and returns an error status, if applicable.
*
*
* If an instance is not created successfully, the instance status reason field returns details relevant to the
* reason. The instance in a failed state is returned only for 24 hours after the CreateInstance API was invoked.
*
*
* @param describeInstanceRequest
* @return A Java Future containing the result of the DescribeInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeInstance
* @see AWS API
* Documentation
*/
default CompletableFuture describeInstance(DescribeInstanceRequest describeInstanceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Returns the current state of the specified instance identifier. It tracks the instance while it is being created
* and returns an error status, if applicable.
*
*
* If an instance is not created successfully, the instance status reason field returns details relevant to the
* reason. The instance in a failed state is returned only for 24 hours after the CreateInstance API was invoked.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeInstanceRequest.Builder} avoiding the need
* to create one manually via {@link DescribeInstanceRequest#builder()}
*
*
* @param describeInstanceRequest
* A {@link Consumer} that will call methods on {@link DescribeInstanceRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeInstance
* @see AWS API
* Documentation
*/
default CompletableFuture describeInstance(
Consumer describeInstanceRequest) {
return describeInstance(DescribeInstanceRequest.builder().applyMutation(describeInstanceRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Describes the specified instance attribute.
*
*
* @param describeInstanceAttributeRequest
* @return A Java Future containing the result of the DescribeInstanceAttribute operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeInstanceAttribute
* @see AWS API Documentation
*/
default CompletableFuture describeInstanceAttribute(
DescribeInstanceAttributeRequest describeInstanceAttributeRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Describes the specified instance attribute.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeInstanceAttributeRequest.Builder} avoiding
* the need to create one manually via {@link DescribeInstanceAttributeRequest#builder()}
*
*
* @param describeInstanceAttributeRequest
* A {@link Consumer} that will call methods on {@link DescribeInstanceAttributeRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeInstanceAttribute operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeInstanceAttribute
* @see AWS API Documentation
*/
default CompletableFuture describeInstanceAttribute(
Consumer describeInstanceAttributeRequest) {
return describeInstanceAttribute(DescribeInstanceAttributeRequest.builder()
.applyMutation(describeInstanceAttributeRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Retrieves the current storage configurations for the specified resource type, association ID, and instance ID.
*
*
* @param describeInstanceStorageConfigRequest
* @return A Java Future containing the result of the DescribeInstanceStorageConfig operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeInstanceStorageConfig
* @see AWS API Documentation
*/
default CompletableFuture describeInstanceStorageConfig(
DescribeInstanceStorageConfigRequest describeInstanceStorageConfigRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Retrieves the current storage configurations for the specified resource type, association ID, and instance ID.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeInstanceStorageConfigRequest.Builder}
* avoiding the need to create one manually via {@link DescribeInstanceStorageConfigRequest#builder()}
*
*
* @param describeInstanceStorageConfigRequest
* A {@link Consumer} that will call methods on {@link DescribeInstanceStorageConfigRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeInstanceStorageConfig operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeInstanceStorageConfig
* @see AWS API Documentation
*/
default CompletableFuture describeInstanceStorageConfig(
Consumer describeInstanceStorageConfigRequest) {
return describeInstanceStorageConfig(DescribeInstanceStorageConfigRequest.builder()
.applyMutation(describeInstanceStorageConfigRequest).build());
}
/**
*
* Gets details and status of a phone number that’s claimed to your Amazon Connect instance or traffic distribution
* group.
*
*
*
* If the number is claimed to a traffic distribution group, and you are calling in the Amazon Web Services Region
* where the traffic distribution group was created, you can use either a phone number ARN or UUID value for the
* PhoneNumberId
URI request parameter. However, if the number is claimed to a traffic distribution
* group and you are calling this API in the alternate Amazon Web Services Region associated with the traffic
* distribution group, you must provide a full phone number ARN. If a UUID is provided in this scenario, you will
* receive a ResourceNotFoundException
.
*
*
*
* @param describePhoneNumberRequest
* @return A Java Future containing the result of the DescribePhoneNumber operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribePhoneNumber
* @see AWS
* API Documentation
*/
default CompletableFuture describePhoneNumber(
DescribePhoneNumberRequest describePhoneNumberRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets details and status of a phone number that’s claimed to your Amazon Connect instance or traffic distribution
* group.
*
*
*
* If the number is claimed to a traffic distribution group, and you are calling in the Amazon Web Services Region
* where the traffic distribution group was created, you can use either a phone number ARN or UUID value for the
* PhoneNumberId
URI request parameter. However, if the number is claimed to a traffic distribution
* group and you are calling this API in the alternate Amazon Web Services Region associated with the traffic
* distribution group, you must provide a full phone number ARN. If a UUID is provided in this scenario, you will
* receive a ResourceNotFoundException
.
*
*
*
* This is a convenience which creates an instance of the {@link DescribePhoneNumberRequest.Builder} avoiding the
* need to create one manually via {@link DescribePhoneNumberRequest#builder()}
*
*
* @param describePhoneNumberRequest
* A {@link Consumer} that will call methods on {@link DescribePhoneNumberRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribePhoneNumber operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribePhoneNumber
* @see AWS
* API Documentation
*/
default CompletableFuture describePhoneNumber(
Consumer describePhoneNumberRequest) {
return describePhoneNumber(DescribePhoneNumberRequest.builder().applyMutation(describePhoneNumberRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Describes the specified queue.
*
*
* @param describeQueueRequest
* @return A Java Future containing the result of the DescribeQueue operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeQueue
* @see AWS API
* Documentation
*/
default CompletableFuture describeQueue(DescribeQueueRequest describeQueueRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Describes the specified queue.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeQueueRequest.Builder} avoiding the need to
* create one manually via {@link DescribeQueueRequest#builder()}
*
*
* @param describeQueueRequest
* A {@link Consumer} that will call methods on {@link DescribeQueueRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeQueue operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeQueue
* @see AWS API
* Documentation
*/
default CompletableFuture describeQueue(Consumer describeQueueRequest) {
return describeQueue(DescribeQueueRequest.builder().applyMutation(describeQueueRequest).build());
}
/**
*
* Describes the quick connect.
*
*
* @param describeQuickConnectRequest
* @return A Java Future containing the result of the DescribeQuickConnect operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeQuickConnect
* @see AWS
* API Documentation
*/
default CompletableFuture describeQuickConnect(
DescribeQuickConnectRequest describeQuickConnectRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the quick connect.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeQuickConnectRequest.Builder} avoiding the
* need to create one manually via {@link DescribeQuickConnectRequest#builder()}
*
*
* @param describeQuickConnectRequest
* A {@link Consumer} that will call methods on {@link DescribeQuickConnectRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeQuickConnect operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeQuickConnect
* @see AWS
* API Documentation
*/
default CompletableFuture describeQuickConnect(
Consumer describeQuickConnectRequest) {
return describeQuickConnect(DescribeQuickConnectRequest.builder().applyMutation(describeQuickConnectRequest).build());
}
/**
*
* Describes the specified routing profile.
*
*
* @param describeRoutingProfileRequest
* @return A Java Future containing the result of the DescribeRoutingProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeRoutingProfile
* @see AWS API Documentation
*/
default CompletableFuture describeRoutingProfile(
DescribeRoutingProfileRequest describeRoutingProfileRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the specified routing profile.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeRoutingProfileRequest.Builder} avoiding the
* need to create one manually via {@link DescribeRoutingProfileRequest#builder()}
*
*
* @param describeRoutingProfileRequest
* A {@link Consumer} that will call methods on {@link DescribeRoutingProfileRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeRoutingProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeRoutingProfile
* @see AWS API Documentation
*/
default CompletableFuture describeRoutingProfile(
Consumer describeRoutingProfileRequest) {
return describeRoutingProfile(DescribeRoutingProfileRequest.builder().applyMutation(describeRoutingProfileRequest)
.build());
}
/**
*
* Describes a rule for the specified Amazon Connect instance.
*
*
* @param describeRuleRequest
* @return A Java Future containing the result of the DescribeRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeRule
* @see AWS API
* Documentation
*/
default CompletableFuture describeRule(DescribeRuleRequest describeRuleRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes a rule for the specified Amazon Connect instance.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeRuleRequest.Builder} avoiding the need to
* create one manually via {@link DescribeRuleRequest#builder()}
*
*
* @param describeRuleRequest
* A {@link Consumer} that will call methods on {@link DescribeRuleRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeRule
* @see AWS API
* Documentation
*/
default CompletableFuture describeRule(Consumer describeRuleRequest) {
return describeRule(DescribeRuleRequest.builder().applyMutation(describeRuleRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Gets basic information about the security profle.
*
*
* @param describeSecurityProfileRequest
* @return A Java Future containing the result of the DescribeSecurityProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeSecurityProfile
* @see AWS API Documentation
*/
default CompletableFuture describeSecurityProfile(
DescribeSecurityProfileRequest describeSecurityProfileRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Gets basic information about the security profle.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeSecurityProfileRequest.Builder} avoiding
* the need to create one manually via {@link DescribeSecurityProfileRequest#builder()}
*
*
* @param describeSecurityProfileRequest
* A {@link Consumer} that will call methods on {@link DescribeSecurityProfileRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeSecurityProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeSecurityProfile
* @see AWS API Documentation
*/
default CompletableFuture describeSecurityProfile(
Consumer describeSecurityProfileRequest) {
return describeSecurityProfile(DescribeSecurityProfileRequest.builder().applyMutation(describeSecurityProfileRequest)
.build());
}
/**
*
* Gets details and status of a traffic distribution group.
*
*
* @param describeTrafficDistributionGroupRequest
* @return A Java Future containing the result of the DescribeTrafficDistributionGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeTrafficDistributionGroup
* @see AWS API Documentation
*/
default CompletableFuture describeTrafficDistributionGroup(
DescribeTrafficDistributionGroupRequest describeTrafficDistributionGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets details and status of a traffic distribution group.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTrafficDistributionGroupRequest.Builder}
* avoiding the need to create one manually via {@link DescribeTrafficDistributionGroupRequest#builder()}
*
*
* @param describeTrafficDistributionGroupRequest
* A {@link Consumer} that will call methods on {@link DescribeTrafficDistributionGroupRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeTrafficDistributionGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeTrafficDistributionGroup
* @see AWS API Documentation
*/
default CompletableFuture describeTrafficDistributionGroup(
Consumer describeTrafficDistributionGroupRequest) {
return describeTrafficDistributionGroup(DescribeTrafficDistributionGroupRequest.builder()
.applyMutation(describeTrafficDistributionGroupRequest).build());
}
/**
*
* Describes the specified user account. You can find the instance ID in the console (it’s the final part of the
* ARN). The console does not display the user IDs. Instead, list the users and note the IDs provided in the output.
*
*
* @param describeUserRequest
* @return A Java Future containing the result of the DescribeUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeUser
* @see AWS API
* Documentation
*/
default CompletableFuture describeUser(DescribeUserRequest describeUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the specified user account. You can find the instance ID in the console (it’s the final part of the
* ARN). The console does not display the user IDs. Instead, list the users and note the IDs provided in the output.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeUserRequest.Builder} avoiding the need to
* create one manually via {@link DescribeUserRequest#builder()}
*
*
* @param describeUserRequest
* A {@link Consumer} that will call methods on {@link DescribeUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeUser
* @see AWS API
* Documentation
*/
default CompletableFuture describeUser(Consumer describeUserRequest) {
return describeUser(DescribeUserRequest.builder().applyMutation(describeUserRequest).build());
}
/**
*
* Describes the specified hierarchy group.
*
*
* @param describeUserHierarchyGroupRequest
* @return A Java Future containing the result of the DescribeUserHierarchyGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeUserHierarchyGroup
* @see AWS API Documentation
*/
default CompletableFuture describeUserHierarchyGroup(
DescribeUserHierarchyGroupRequest describeUserHierarchyGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the specified hierarchy group.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeUserHierarchyGroupRequest.Builder} avoiding
* the need to create one manually via {@link DescribeUserHierarchyGroupRequest#builder()}
*
*
* @param describeUserHierarchyGroupRequest
* A {@link Consumer} that will call methods on {@link DescribeUserHierarchyGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeUserHierarchyGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeUserHierarchyGroup
* @see AWS API Documentation
*/
default CompletableFuture describeUserHierarchyGroup(
Consumer describeUserHierarchyGroupRequest) {
return describeUserHierarchyGroup(DescribeUserHierarchyGroupRequest.builder()
.applyMutation(describeUserHierarchyGroupRequest).build());
}
/**
*
* Describes the hierarchy structure of the specified Amazon Connect instance.
*
*
* @param describeUserHierarchyStructureRequest
* @return A Java Future containing the result of the DescribeUserHierarchyStructure operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeUserHierarchyStructure
* @see AWS API Documentation
*/
default CompletableFuture describeUserHierarchyStructure(
DescribeUserHierarchyStructureRequest describeUserHierarchyStructureRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the hierarchy structure of the specified Amazon Connect instance.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeUserHierarchyStructureRequest.Builder}
* avoiding the need to create one manually via {@link DescribeUserHierarchyStructureRequest#builder()}
*
*
* @param describeUserHierarchyStructureRequest
* A {@link Consumer} that will call methods on {@link DescribeUserHierarchyStructureRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeUserHierarchyStructure operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeUserHierarchyStructure
* @see AWS API Documentation
*/
default CompletableFuture describeUserHierarchyStructure(
Consumer describeUserHierarchyStructureRequest) {
return describeUserHierarchyStructure(DescribeUserHierarchyStructureRequest.builder()
.applyMutation(describeUserHierarchyStructureRequest).build());
}
/**
*
* Describes the specified vocabulary.
*
*
* @param describeVocabularyRequest
* @return A Java Future containing the result of the DescribeVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeVocabulary
* @see AWS
* API Documentation
*/
default CompletableFuture describeVocabulary(DescribeVocabularyRequest describeVocabularyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the specified vocabulary.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeVocabularyRequest.Builder} avoiding the
* need to create one manually via {@link DescribeVocabularyRequest#builder()}
*
*
* @param describeVocabularyRequest
* A {@link Consumer} that will call methods on {@link DescribeVocabularyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeVocabulary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DescribeVocabulary
* @see AWS
* API Documentation
*/
default CompletableFuture describeVocabulary(
Consumer describeVocabularyRequest) {
return describeVocabulary(DescribeVocabularyRequest.builder().applyMutation(describeVocabularyRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Revokes access to integrated applications from Amazon Connect.
*
*
* @param disassociateApprovedOriginRequest
* @return A Java Future containing the result of the DisassociateApprovedOrigin operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateApprovedOrigin
* @see AWS API Documentation
*/
default CompletableFuture disassociateApprovedOrigin(
DisassociateApprovedOriginRequest disassociateApprovedOriginRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Revokes access to integrated applications from Amazon Connect.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateApprovedOriginRequest.Builder} avoiding
* the need to create one manually via {@link DisassociateApprovedOriginRequest#builder()}
*
*
* @param disassociateApprovedOriginRequest
* A {@link Consumer} that will call methods on {@link DisassociateApprovedOriginRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DisassociateApprovedOrigin operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateApprovedOrigin
* @see AWS API Documentation
*/
default CompletableFuture disassociateApprovedOrigin(
Consumer disassociateApprovedOriginRequest) {
return disassociateApprovedOrigin(DisassociateApprovedOriginRequest.builder()
.applyMutation(disassociateApprovedOriginRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Revokes authorization from the specified instance to access the specified Amazon Lex or Amazon Lex V2 bot.
*
*
* @param disassociateBotRequest
* @return A Java Future containing the result of the DisassociateBot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateBot
* @see AWS API
* Documentation
*/
default CompletableFuture disassociateBot(DisassociateBotRequest disassociateBotRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Revokes authorization from the specified instance to access the specified Amazon Lex or Amazon Lex V2 bot.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateBotRequest.Builder} avoiding the need
* to create one manually via {@link DisassociateBotRequest#builder()}
*
*
* @param disassociateBotRequest
* A {@link Consumer} that will call methods on {@link DisassociateBotRequest.Builder} to create a request.
* @return A Java Future containing the result of the DisassociateBot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateBot
* @see AWS API
* Documentation
*/
default CompletableFuture disassociateBot(
Consumer disassociateBotRequest) {
return disassociateBot(DisassociateBotRequest.builder().applyMutation(disassociateBotRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Removes the storage type configurations for the specified resource type and association ID.
*
*
* @param disassociateInstanceStorageConfigRequest
* @return A Java Future containing the result of the DisassociateInstanceStorageConfig operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateInstanceStorageConfig
* @see AWS API Documentation
*/
default CompletableFuture disassociateInstanceStorageConfig(
DisassociateInstanceStorageConfigRequest disassociateInstanceStorageConfigRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Removes the storage type configurations for the specified resource type and association ID.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateInstanceStorageConfigRequest.Builder}
* avoiding the need to create one manually via {@link DisassociateInstanceStorageConfigRequest#builder()}
*
*
* @param disassociateInstanceStorageConfigRequest
* A {@link Consumer} that will call methods on {@link DisassociateInstanceStorageConfigRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DisassociateInstanceStorageConfig operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateInstanceStorageConfig
* @see AWS API Documentation
*/
default CompletableFuture disassociateInstanceStorageConfig(
Consumer disassociateInstanceStorageConfigRequest) {
return disassociateInstanceStorageConfig(DisassociateInstanceStorageConfigRequest.builder()
.applyMutation(disassociateInstanceStorageConfigRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Remove the Lambda function from the dropdown options available in the relevant flow blocks.
*
*
* @param disassociateLambdaFunctionRequest
* @return A Java Future containing the result of the DisassociateLambdaFunction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateLambdaFunction
* @see AWS API Documentation
*/
default CompletableFuture disassociateLambdaFunction(
DisassociateLambdaFunctionRequest disassociateLambdaFunctionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Remove the Lambda function from the dropdown options available in the relevant flow blocks.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateLambdaFunctionRequest.Builder} avoiding
* the need to create one manually via {@link DisassociateLambdaFunctionRequest#builder()}
*
*
* @param disassociateLambdaFunctionRequest
* A {@link Consumer} that will call methods on {@link DisassociateLambdaFunctionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DisassociateLambdaFunction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateLambdaFunction
* @see AWS API Documentation
*/
default CompletableFuture disassociateLambdaFunction(
Consumer disassociateLambdaFunctionRequest) {
return disassociateLambdaFunction(DisassociateLambdaFunctionRequest.builder()
.applyMutation(disassociateLambdaFunctionRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Revokes authorization from the specified instance to access the specified Amazon Lex bot.
*
*
* @param disassociateLexBotRequest
* @return A Java Future containing the result of the DisassociateLexBot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateLexBot
* @see AWS
* API Documentation
*/
default CompletableFuture disassociateLexBot(DisassociateLexBotRequest disassociateLexBotRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Revokes authorization from the specified instance to access the specified Amazon Lex bot.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateLexBotRequest.Builder} avoiding the
* need to create one manually via {@link DisassociateLexBotRequest#builder()}
*
*
* @param disassociateLexBotRequest
* A {@link Consumer} that will call methods on {@link DisassociateLexBotRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DisassociateLexBot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateLexBot
* @see AWS
* API Documentation
*/
default CompletableFuture disassociateLexBot(
Consumer disassociateLexBotRequest) {
return disassociateLexBot(DisassociateLexBotRequest.builder().applyMutation(disassociateLexBotRequest).build());
}
/**
*
* Removes the flow association from a phone number claimed to your Amazon Connect instance.
*
*
*
* If the number is claimed to a traffic distribution group, and you are calling this API using an instance in the
* Amazon Web Services Region where the traffic distribution group was created, you can use either a full phone
* number ARN or UUID value for the PhoneNumberId
URI request parameter. However, if the number is
* claimed to a traffic distribution group and you are calling this API using an instance in the alternate Amazon
* Web Services Region associated with the traffic distribution group, you must provide a full phone number ARN. If
* a UUID is provided in this scenario, you will receive a ResourceNotFoundException
.
*
*
*
* @param disassociatePhoneNumberContactFlowRequest
* @return A Java Future containing the result of the DisassociatePhoneNumberContactFlow operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociatePhoneNumberContactFlow
* @see AWS API Documentation
*/
default CompletableFuture disassociatePhoneNumberContactFlow(
DisassociatePhoneNumberContactFlowRequest disassociatePhoneNumberContactFlowRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes the flow association from a phone number claimed to your Amazon Connect instance.
*
*
*
* If the number is claimed to a traffic distribution group, and you are calling this API using an instance in the
* Amazon Web Services Region where the traffic distribution group was created, you can use either a full phone
* number ARN or UUID value for the PhoneNumberId
URI request parameter. However, if the number is
* claimed to a traffic distribution group and you are calling this API using an instance in the alternate Amazon
* Web Services Region associated with the traffic distribution group, you must provide a full phone number ARN. If
* a UUID is provided in this scenario, you will receive a ResourceNotFoundException
.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociatePhoneNumberContactFlowRequest.Builder}
* avoiding the need to create one manually via {@link DisassociatePhoneNumberContactFlowRequest#builder()}
*
*
* @param disassociatePhoneNumberContactFlowRequest
* A {@link Consumer} that will call methods on {@link DisassociatePhoneNumberContactFlowRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DisassociatePhoneNumberContactFlow operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociatePhoneNumberContactFlow
* @see AWS API Documentation
*/
default CompletableFuture disassociatePhoneNumberContactFlow(
Consumer disassociatePhoneNumberContactFlowRequest) {
return disassociatePhoneNumberContactFlow(DisassociatePhoneNumberContactFlowRequest.builder()
.applyMutation(disassociatePhoneNumberContactFlowRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Disassociates a set of quick connects from a queue.
*
*
* @param disassociateQueueQuickConnectsRequest
* @return A Java Future containing the result of the DisassociateQueueQuickConnects operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateQueueQuickConnects
* @see AWS API Documentation
*/
default CompletableFuture disassociateQueueQuickConnects(
DisassociateQueueQuickConnectsRequest disassociateQueueQuickConnectsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Disassociates a set of quick connects from a queue.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateQueueQuickConnectsRequest.Builder}
* avoiding the need to create one manually via {@link DisassociateQueueQuickConnectsRequest#builder()}
*
*
* @param disassociateQueueQuickConnectsRequest
* A {@link Consumer} that will call methods on {@link DisassociateQueueQuickConnectsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DisassociateQueueQuickConnects operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateQueueQuickConnects
* @see AWS API Documentation
*/
default CompletableFuture disassociateQueueQuickConnects(
Consumer disassociateQueueQuickConnectsRequest) {
return disassociateQueueQuickConnects(DisassociateQueueQuickConnectsRequest.builder()
.applyMutation(disassociateQueueQuickConnectsRequest).build());
}
/**
*
* Disassociates a set of queues from a routing profile.
*
*
* @param disassociateRoutingProfileQueuesRequest
* @return A Java Future containing the result of the DisassociateRoutingProfileQueues operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateRoutingProfileQueues
* @see AWS API Documentation
*/
default CompletableFuture disassociateRoutingProfileQueues(
DisassociateRoutingProfileQueuesRequest disassociateRoutingProfileQueuesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates a set of queues from a routing profile.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateRoutingProfileQueuesRequest.Builder}
* avoiding the need to create one manually via {@link DisassociateRoutingProfileQueuesRequest#builder()}
*
*
* @param disassociateRoutingProfileQueuesRequest
* A {@link Consumer} that will call methods on {@link DisassociateRoutingProfileQueuesRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DisassociateRoutingProfileQueues operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateRoutingProfileQueues
* @see AWS API Documentation
*/
default CompletableFuture disassociateRoutingProfileQueues(
Consumer disassociateRoutingProfileQueuesRequest) {
return disassociateRoutingProfileQueues(DisassociateRoutingProfileQueuesRequest.builder()
.applyMutation(disassociateRoutingProfileQueuesRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Deletes the specified security key.
*
*
* @param disassociateSecurityKeyRequest
* @return A Java Future containing the result of the DisassociateSecurityKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateSecurityKey
* @see AWS API Documentation
*/
default CompletableFuture disassociateSecurityKey(
DisassociateSecurityKeyRequest disassociateSecurityKeyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Deletes the specified security key.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateSecurityKeyRequest.Builder} avoiding
* the need to create one manually via {@link DisassociateSecurityKeyRequest#builder()}
*
*
* @param disassociateSecurityKeyRequest
* A {@link Consumer} that will call methods on {@link DisassociateSecurityKeyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DisassociateSecurityKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DisassociateSecurityKey
* @see AWS API Documentation
*/
default CompletableFuture disassociateSecurityKey(
Consumer disassociateSecurityKeyRequest) {
return disassociateSecurityKey(DisassociateSecurityKeyRequest.builder().applyMutation(disassociateSecurityKeyRequest)
.build());
}
/**
*
* Dismisses contacts from an agent’s CCP and returns the agent to an available state, which allows the agent to
* receive a new routed contact. Contacts can only be dismissed if they are in a MISSED
,
* ERROR
, ENDED
, or REJECTED
state in the Agent Event Stream.
*
*
* @param dismissUserContactRequest
* @return A Java Future containing the result of the DismissUserContact operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DismissUserContact
* @see AWS
* API Documentation
*/
default CompletableFuture dismissUserContact(DismissUserContactRequest dismissUserContactRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Dismisses contacts from an agent’s CCP and returns the agent to an available state, which allows the agent to
* receive a new routed contact. Contacts can only be dismissed if they are in a MISSED
,
* ERROR
, ENDED
, or REJECTED
state in the Agent Event Stream.
*
*
*
* This is a convenience which creates an instance of the {@link DismissUserContactRequest.Builder} avoiding the
* need to create one manually via {@link DismissUserContactRequest#builder()}
*
*
* @param dismissUserContactRequest
* A {@link Consumer} that will call methods on {@link DismissUserContactRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DismissUserContact operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.DismissUserContact
* @see AWS
* API Documentation
*/
default CompletableFuture dismissUserContact(
Consumer dismissUserContactRequest) {
return dismissUserContact(DismissUserContactRequest.builder().applyMutation(dismissUserContactRequest).build());
}
/**
*
* Retrieves the contact attributes for the specified contact.
*
*
* @param getContactAttributesRequest
* @return A Java Future containing the result of the GetContactAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetContactAttributes
* @see AWS
* API Documentation
*/
default CompletableFuture getContactAttributes(
GetContactAttributesRequest getContactAttributesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the contact attributes for the specified contact.
*
*
*
* This is a convenience which creates an instance of the {@link GetContactAttributesRequest.Builder} avoiding the
* need to create one manually via {@link GetContactAttributesRequest#builder()}
*
*
* @param getContactAttributesRequest
* A {@link Consumer} that will call methods on {@link GetContactAttributesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetContactAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetContactAttributes
* @see AWS
* API Documentation
*/
default CompletableFuture getContactAttributes(
Consumer getContactAttributesRequest) {
return getContactAttributes(GetContactAttributesRequest.builder().applyMutation(getContactAttributesRequest).build());
}
/**
*
* Gets the real-time metric data from the specified Amazon Connect instance.
*
*
* For a description of each metric, see Real-time Metrics
* Definitions in the Amazon Connect Administrator Guide.
*
*
* @param getCurrentMetricDataRequest
* @return A Java Future containing the result of the GetCurrentMetricData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetCurrentMetricData
* @see AWS
* API Documentation
*/
default CompletableFuture getCurrentMetricData(
GetCurrentMetricDataRequest getCurrentMetricDataRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the real-time metric data from the specified Amazon Connect instance.
*
*
* For a description of each metric, see Real-time Metrics
* Definitions in the Amazon Connect Administrator Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetCurrentMetricDataRequest.Builder} avoiding the
* need to create one manually via {@link GetCurrentMetricDataRequest#builder()}
*
*
* @param getCurrentMetricDataRequest
* A {@link Consumer} that will call methods on {@link GetCurrentMetricDataRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetCurrentMetricData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetCurrentMetricData
* @see AWS
* API Documentation
*/
default CompletableFuture getCurrentMetricData(
Consumer getCurrentMetricDataRequest) {
return getCurrentMetricData(GetCurrentMetricDataRequest.builder().applyMutation(getCurrentMetricDataRequest).build());
}
/**
*
* Gets the real-time metric data from the specified Amazon Connect instance.
*
*
* For a description of each metric, see Real-time Metrics
* Definitions in the Amazon Connect Administrator Guide.
*
*
*
* This is a variant of
* {@link #getCurrentMetricData(software.amazon.awssdk.services.connect.model.GetCurrentMetricDataRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.GetCurrentMetricDataPublisher publisher = client.getCurrentMetricDataPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.GetCurrentMetricDataPublisher publisher = client.getCurrentMetricDataPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.connect.model.GetCurrentMetricDataResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getCurrentMetricData(software.amazon.awssdk.services.connect.model.GetCurrentMetricDataRequest)}
* operation.
*
*
* @param getCurrentMetricDataRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetCurrentMetricData
* @see AWS
* API Documentation
*/
default GetCurrentMetricDataPublisher getCurrentMetricDataPaginator(GetCurrentMetricDataRequest getCurrentMetricDataRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the real-time metric data from the specified Amazon Connect instance.
*
*
* For a description of each metric, see Real-time Metrics
* Definitions in the Amazon Connect Administrator Guide.
*
*
*
* This is a variant of
* {@link #getCurrentMetricData(software.amazon.awssdk.services.connect.model.GetCurrentMetricDataRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.GetCurrentMetricDataPublisher publisher = client.getCurrentMetricDataPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.GetCurrentMetricDataPublisher publisher = client.getCurrentMetricDataPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.connect.model.GetCurrentMetricDataResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getCurrentMetricData(software.amazon.awssdk.services.connect.model.GetCurrentMetricDataRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetCurrentMetricDataRequest.Builder} avoiding the
* need to create one manually via {@link GetCurrentMetricDataRequest#builder()}
*
*
* @param getCurrentMetricDataRequest
* A {@link Consumer} that will call methods on {@link GetCurrentMetricDataRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetCurrentMetricData
* @see AWS
* API Documentation
*/
default GetCurrentMetricDataPublisher getCurrentMetricDataPaginator(
Consumer getCurrentMetricDataRequest) {
return getCurrentMetricDataPaginator(GetCurrentMetricDataRequest.builder().applyMutation(getCurrentMetricDataRequest)
.build());
}
/**
*
* Gets the real-time active user data from the specified Amazon Connect instance.
*
*
* @param getCurrentUserDataRequest
* @return A Java Future containing the result of the GetCurrentUserData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetCurrentUserData
* @see AWS
* API Documentation
*/
default CompletableFuture getCurrentUserData(GetCurrentUserDataRequest getCurrentUserDataRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the real-time active user data from the specified Amazon Connect instance.
*
*
*
* This is a convenience which creates an instance of the {@link GetCurrentUserDataRequest.Builder} avoiding the
* need to create one manually via {@link GetCurrentUserDataRequest#builder()}
*
*
* @param getCurrentUserDataRequest
* A {@link Consumer} that will call methods on {@link GetCurrentUserDataRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetCurrentUserData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetCurrentUserData
* @see AWS
* API Documentation
*/
default CompletableFuture getCurrentUserData(
Consumer getCurrentUserDataRequest) {
return getCurrentUserData(GetCurrentUserDataRequest.builder().applyMutation(getCurrentUserDataRequest).build());
}
/**
*
* Gets the real-time active user data from the specified Amazon Connect instance.
*
*
*
* This is a variant of
* {@link #getCurrentUserData(software.amazon.awssdk.services.connect.model.GetCurrentUserDataRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.GetCurrentUserDataPublisher publisher = client.getCurrentUserDataPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.GetCurrentUserDataPublisher publisher = client.getCurrentUserDataPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.connect.model.GetCurrentUserDataResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getCurrentUserData(software.amazon.awssdk.services.connect.model.GetCurrentUserDataRequest)}
* operation.
*
*
* @param getCurrentUserDataRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetCurrentUserData
* @see AWS
* API Documentation
*/
default GetCurrentUserDataPublisher getCurrentUserDataPaginator(GetCurrentUserDataRequest getCurrentUserDataRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the real-time active user data from the specified Amazon Connect instance.
*
*
*
* This is a variant of
* {@link #getCurrentUserData(software.amazon.awssdk.services.connect.model.GetCurrentUserDataRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.GetCurrentUserDataPublisher publisher = client.getCurrentUserDataPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.GetCurrentUserDataPublisher publisher = client.getCurrentUserDataPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.connect.model.GetCurrentUserDataResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getCurrentUserData(software.amazon.awssdk.services.connect.model.GetCurrentUserDataRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetCurrentUserDataRequest.Builder} avoiding the
* need to create one manually via {@link GetCurrentUserDataRequest#builder()}
*
*
* @param getCurrentUserDataRequest
* A {@link Consumer} that will call methods on {@link GetCurrentUserDataRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetCurrentUserData
* @see AWS
* API Documentation
*/
default GetCurrentUserDataPublisher getCurrentUserDataPaginator(
Consumer getCurrentUserDataRequest) {
return getCurrentUserDataPaginator(GetCurrentUserDataRequest.builder().applyMutation(getCurrentUserDataRequest).build());
}
/**
*
* Retrieves a token for federation.
*
*
*
* This API doesn't support root users. If you try to invoke GetFederationToken with root credentials, an error
* message similar to the following one appears:
*
*
* Provided identity: Principal: .... User: .... cannot be used for federation with Amazon Connect
*
*
*
* @param getFederationTokenRequest
* @return A Java Future containing the result of the GetFederationToken operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - UserNotFoundException No user with the specified credentials was found in the Amazon Connect
* instance.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - DuplicateResourceException A resource with the specified name already exists.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetFederationToken
* @see AWS
* API Documentation
*/
default CompletableFuture getFederationToken(GetFederationTokenRequest getFederationTokenRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a token for federation.
*
*
*
* This API doesn't support root users. If you try to invoke GetFederationToken with root credentials, an error
* message similar to the following one appears:
*
*
* Provided identity: Principal: .... User: .... cannot be used for federation with Amazon Connect
*
*
*
* This is a convenience which creates an instance of the {@link GetFederationTokenRequest.Builder} avoiding the
* need to create one manually via {@link GetFederationTokenRequest#builder()}
*
*
* @param getFederationTokenRequest
* A {@link Consumer} that will call methods on {@link GetFederationTokenRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetFederationToken operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - UserNotFoundException No user with the specified credentials was found in the Amazon Connect
* instance.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - DuplicateResourceException A resource with the specified name already exists.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetFederationToken
* @see AWS
* API Documentation
*/
default CompletableFuture getFederationToken(
Consumer getFederationTokenRequest) {
return getFederationToken(GetFederationTokenRequest.builder().applyMutation(getFederationTokenRequest).build());
}
/**
*
* Gets historical metric data from the specified Amazon Connect instance.
*
*
* For a description of each historical metric, see Historical
* Metrics Definitions in the Amazon Connect Administrator Guide.
*
*
* @param getMetricDataRequest
* @return A Java Future containing the result of the GetMetricData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetMetricData
* @see AWS API
* Documentation
*/
default CompletableFuture getMetricData(GetMetricDataRequest getMetricDataRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets historical metric data from the specified Amazon Connect instance.
*
*
* For a description of each historical metric, see Historical
* Metrics Definitions in the Amazon Connect Administrator Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetMetricDataRequest.Builder} avoiding the need to
* create one manually via {@link GetMetricDataRequest#builder()}
*
*
* @param getMetricDataRequest
* A {@link Consumer} that will call methods on {@link GetMetricDataRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetMetricData operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetMetricData
* @see AWS API
* Documentation
*/
default CompletableFuture getMetricData(Consumer getMetricDataRequest) {
return getMetricData(GetMetricDataRequest.builder().applyMutation(getMetricDataRequest).build());
}
/**
*
* Gets historical metric data from the specified Amazon Connect instance.
*
*
* For a description of each historical metric, see Historical
* Metrics Definitions in the Amazon Connect Administrator Guide.
*
*
*
* This is a variant of {@link #getMetricData(software.amazon.awssdk.services.connect.model.GetMetricDataRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.GetMetricDataPublisher publisher = client.getMetricDataPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.GetMetricDataPublisher publisher = client.getMetricDataPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.connect.model.GetMetricDataResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getMetricData(software.amazon.awssdk.services.connect.model.GetMetricDataRequest)} operation.
*
*
* @param getMetricDataRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetMetricData
* @see AWS API
* Documentation
*/
default GetMetricDataPublisher getMetricDataPaginator(GetMetricDataRequest getMetricDataRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets historical metric data from the specified Amazon Connect instance.
*
*
* For a description of each historical metric, see Historical
* Metrics Definitions in the Amazon Connect Administrator Guide.
*
*
*
* This is a variant of {@link #getMetricData(software.amazon.awssdk.services.connect.model.GetMetricDataRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.GetMetricDataPublisher publisher = client.getMetricDataPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.GetMetricDataPublisher publisher = client.getMetricDataPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.connect.model.GetMetricDataResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getMetricData(software.amazon.awssdk.services.connect.model.GetMetricDataRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link GetMetricDataRequest.Builder} avoiding the need to
* create one manually via {@link GetMetricDataRequest#builder()}
*
*
* @param getMetricDataRequest
* A {@link Consumer} that will call methods on {@link GetMetricDataRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - ResourceNotFoundException The specified resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetMetricData
* @see AWS API
* Documentation
*/
default GetMetricDataPublisher getMetricDataPaginator(Consumer getMetricDataRequest) {
return getMetricDataPaginator(GetMetricDataRequest.builder().applyMutation(getMetricDataRequest).build());
}
/**
*
* Gets details about a specific task template in the specified Amazon Connect instance.
*
*
* @param getTaskTemplateRequest
* @return A Java Future containing the result of the GetTaskTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetTaskTemplate
* @see AWS API
* Documentation
*/
default CompletableFuture getTaskTemplate(GetTaskTemplateRequest getTaskTemplateRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets details about a specific task template in the specified Amazon Connect instance.
*
*
*
* This is a convenience which creates an instance of the {@link GetTaskTemplateRequest.Builder} avoiding the need
* to create one manually via {@link GetTaskTemplateRequest#builder()}
*
*
* @param getTaskTemplateRequest
* A {@link Consumer} that will call methods on {@link GetTaskTemplateRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetTaskTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetTaskTemplate
* @see AWS API
* Documentation
*/
default CompletableFuture getTaskTemplate(
Consumer getTaskTemplateRequest) {
return getTaskTemplate(GetTaskTemplateRequest.builder().applyMutation(getTaskTemplateRequest).build());
}
/**
*
* Retrieves the current traffic distribution for a given traffic distribution group.
*
*
* @param getTrafficDistributionRequest
* @return A Java Future containing the result of the GetTrafficDistribution operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetTrafficDistribution
* @see AWS API Documentation
*/
default CompletableFuture getTrafficDistribution(
GetTrafficDistributionRequest getTrafficDistributionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the current traffic distribution for a given traffic distribution group.
*
*
*
* This is a convenience which creates an instance of the {@link GetTrafficDistributionRequest.Builder} avoiding the
* need to create one manually via {@link GetTrafficDistributionRequest#builder()}
*
*
* @param getTrafficDistributionRequest
* A {@link Consumer} that will call methods on {@link GetTrafficDistributionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetTrafficDistribution operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - AccessDeniedException You do not have sufficient permissions to perform this action.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.GetTrafficDistribution
* @see AWS API Documentation
*/
default CompletableFuture getTrafficDistribution(
Consumer getTrafficDistributionRequest) {
return getTrafficDistribution(GetTrafficDistributionRequest.builder().applyMutation(getTrafficDistributionRequest)
.build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Lists agent statuses.
*
*
* @param listAgentStatusesRequest
* @return A Java Future containing the result of the ListAgentStatuses operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.ListAgentStatuses
* @see AWS API
* Documentation
*/
default CompletableFuture listAgentStatuses(ListAgentStatusesRequest listAgentStatusesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Lists agent statuses.
*
*
*
* This is a convenience which creates an instance of the {@link ListAgentStatusesRequest.Builder} avoiding the need
* to create one manually via {@link ListAgentStatusesRequest#builder()}
*
*
* @param listAgentStatusesRequest
* A {@link Consumer} that will call methods on {@link ListAgentStatusRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListAgentStatuses operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.ListAgentStatuses
* @see AWS API
* Documentation
*/
default CompletableFuture listAgentStatuses(
Consumer listAgentStatusesRequest) {
return listAgentStatuses(ListAgentStatusesRequest.builder().applyMutation(listAgentStatusesRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Lists agent statuses.
*
*
*
* This is a variant of
* {@link #listAgentStatuses(software.amazon.awssdk.services.connect.model.ListAgentStatusesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.ListAgentStatusesPublisher publisher = client.listAgentStatusesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.ListAgentStatusesPublisher publisher = client.listAgentStatusesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.connect.model.ListAgentStatusesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAgentStatuses(software.amazon.awssdk.services.connect.model.ListAgentStatusesRequest)} operation.
*
*
* @param listAgentStatusesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.ListAgentStatuses
* @see AWS API
* Documentation
*/
default ListAgentStatusesPublisher listAgentStatusesPaginator(ListAgentStatusesRequest listAgentStatusesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Lists agent statuses.
*
*
*
* This is a variant of
* {@link #listAgentStatuses(software.amazon.awssdk.services.connect.model.ListAgentStatusesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.ListAgentStatusesPublisher publisher = client.listAgentStatusesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.ListAgentStatusesPublisher publisher = client.listAgentStatusesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.connect.model.ListAgentStatusesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAgentStatuses(software.amazon.awssdk.services.connect.model.ListAgentStatusesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListAgentStatusesRequest.Builder} avoiding the need
* to create one manually via {@link ListAgentStatusesRequest#builder()}
*
*
* @param listAgentStatusesRequest
* A {@link Consumer} that will call methods on {@link ListAgentStatusRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ResourceNotFoundException The specified resource was not found.
* - ThrottlingException The throttling limit has been exceeded.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.ListAgentStatuses
* @see AWS API
* Documentation
*/
default ListAgentStatusesPublisher listAgentStatusesPaginator(
Consumer listAgentStatusesRequest) {
return listAgentStatusesPaginator(ListAgentStatusesRequest.builder().applyMutation(listAgentStatusesRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Returns a paginated list of all approved origins associated with the instance.
*
*
* @param listApprovedOriginsRequest
* @return A Java Future containing the result of the ListApprovedOrigins operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.ListApprovedOrigins
* @see AWS
* API Documentation
*/
default CompletableFuture listApprovedOrigins(
ListApprovedOriginsRequest listApprovedOriginsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Returns a paginated list of all approved origins associated with the instance.
*
*
*
* This is a convenience which creates an instance of the {@link ListApprovedOriginsRequest.Builder} avoiding the
* need to create one manually via {@link ListApprovedOriginsRequest#builder()}
*
*
* @param listApprovedOriginsRequest
* A {@link Consumer} that will call methods on {@link ListApprovedOriginsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListApprovedOrigins operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.ListApprovedOrigins
* @see AWS
* API Documentation
*/
default CompletableFuture listApprovedOrigins(
Consumer listApprovedOriginsRequest) {
return listApprovedOrigins(ListApprovedOriginsRequest.builder().applyMutation(listApprovedOriginsRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Returns a paginated list of all approved origins associated with the instance.
*
*
*
* This is a variant of
* {@link #listApprovedOrigins(software.amazon.awssdk.services.connect.model.ListApprovedOriginsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.ListApprovedOriginsPublisher publisher = client.listApprovedOriginsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.ListApprovedOriginsPublisher publisher = client.listApprovedOriginsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.connect.model.ListApprovedOriginsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listApprovedOrigins(software.amazon.awssdk.services.connect.model.ListApprovedOriginsRequest)}
* operation.
*
*
* @param listApprovedOriginsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.ListApprovedOrigins
* @see AWS
* API Documentation
*/
default ListApprovedOriginsPublisher listApprovedOriginsPaginator(ListApprovedOriginsRequest listApprovedOriginsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* Returns a paginated list of all approved origins associated with the instance.
*
*
*
* This is a variant of
* {@link #listApprovedOrigins(software.amazon.awssdk.services.connect.model.ListApprovedOriginsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.ListApprovedOriginsPublisher publisher = client.listApprovedOriginsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.ListApprovedOriginsPublisher publisher = client.listApprovedOriginsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.connect.model.ListApprovedOriginsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listApprovedOrigins(software.amazon.awssdk.services.connect.model.ListApprovedOriginsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListApprovedOriginsRequest.Builder} avoiding the
* need to create one manually via {@link ListApprovedOriginsRequest#builder()}
*
*
* @param listApprovedOriginsRequest
* A {@link Consumer} that will call methods on {@link ListApprovedOriginsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - InvalidParameterException One or more of the specified parameters are not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.ListApprovedOrigins
* @see AWS
* API Documentation
*/
default ListApprovedOriginsPublisher listApprovedOriginsPaginator(
Consumer listApprovedOriginsRequest) {
return listApprovedOriginsPaginator(ListApprovedOriginsRequest.builder().applyMutation(listApprovedOriginsRequest)
.build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* For the specified version of Amazon Lex, returns a paginated list of all the Amazon Lex bots currently associated
* with the instance. Use this API to returns both Amazon Lex V1 and V2 bots.
*
*
* @param listBotsRequest
* @return A Java Future containing the result of the ListBots operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.ListBots
* @see AWS API
* Documentation
*/
default CompletableFuture listBots(ListBotsRequest listBotsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* For the specified version of Amazon Lex, returns a paginated list of all the Amazon Lex bots currently associated
* with the instance. Use this API to returns both Amazon Lex V1 and V2 bots.
*
*
*
* This is a convenience which creates an instance of the {@link ListBotsRequest.Builder} avoiding the need to
* create one manually via {@link ListBotsRequest#builder()}
*
*
* @param listBotsRequest
* A {@link Consumer} that will call methods on {@link ListBotsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListBots operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.ListBots
* @see AWS API
* Documentation
*/
default CompletableFuture listBots(Consumer listBotsRequest) {
return listBots(ListBotsRequest.builder().applyMutation(listBotsRequest).build());
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* For the specified version of Amazon Lex, returns a paginated list of all the Amazon Lex bots currently associated
* with the instance. Use this API to returns both Amazon Lex V1 and V2 bots.
*
*
*
* This is a variant of {@link #listBots(software.amazon.awssdk.services.connect.model.ListBotsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.ListBotsPublisher publisher = client.listBotsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.ListBotsPublisher publisher = client.listBotsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.connect.model.ListBotsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBots(software.amazon.awssdk.services.connect.model.ListBotsRequest)} operation.
*
*
* @param listBotsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.ListBots
* @see AWS API
* Documentation
*/
default ListBotsPublisher listBotsPaginator(ListBotsRequest listBotsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* This API is in preview release for Amazon Connect and is subject to change.
*
*
* For the specified version of Amazon Lex, returns a paginated list of all the Amazon Lex bots currently associated
* with the instance. Use this API to returns both Amazon Lex V1 and V2 bots.
*
*
*
* This is a variant of {@link #listBots(software.amazon.awssdk.services.connect.model.ListBotsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.ListBotsPublisher publisher = client.listBotsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.connect.paginators.ListBotsPublisher publisher = client.listBotsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.connect.model.ListBotsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBots(software.amazon.awssdk.services.connect.model.ListBotsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListBotsRequest.Builder} avoiding the need to
* create one manually via {@link ListBotsRequest#builder()}
*
*
* @param listBotsRequest
* A {@link Consumer} that will call methods on {@link ListBotsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalServiceException Request processing failed because of an error or failure with the service.
* - InvalidRequestException The request is not valid.
* - ThrottlingException The throttling limit has been exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ConnectException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ConnectAsyncClient.ListBots
* @see AWS API
* Documentation
*/
default ListBotsPublisher listBotsPaginator(Consumer listBotsRequest) {
return listBotsPaginator(ListBotsRequest.builder().applyMutation(listBotsRequest).build());
}
/**
*
* Provides information about the flow modules for the specified Amazon Connect instance.
*
*
* @param listContactFlowModulesRequest
* @return A Java Future containing the result of the ListContactFlowModules operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
*