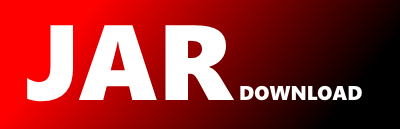
software.amazon.awssdk.services.connect.model.RealTimeContactAnalysisSegmentAttachments Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.connect.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Segment containing list of attachments.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RealTimeContactAnalysisSegmentAttachments implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(RealTimeContactAnalysisSegmentAttachments::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField PARTICIPANT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ParticipantId").getter(getter(RealTimeContactAnalysisSegmentAttachments::participantId))
.setter(setter(Builder::participantId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ParticipantId").build()).build();
private static final SdkField PARTICIPANT_ROLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ParticipantRole").getter(getter(RealTimeContactAnalysisSegmentAttachments::participantRoleAsString))
.setter(setter(Builder::participantRole))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ParticipantRole").build()).build();
private static final SdkField DISPLAY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DisplayName").getter(getter(RealTimeContactAnalysisSegmentAttachments::displayName))
.setter(setter(Builder::displayName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DisplayName").build()).build();
private static final SdkField> ATTACHMENTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Attachments")
.getter(getter(RealTimeContactAnalysisSegmentAttachments::attachments))
.setter(setter(Builder::attachments))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Attachments").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(RealTimeContactAnalysisAttachment::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField TIME_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Time")
.getter(getter(RealTimeContactAnalysisSegmentAttachments::time)).setter(setter(Builder::time))
.constructor(RealTimeContactAnalysisTimeData::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Time").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD,
PARTICIPANT_ID_FIELD, PARTICIPANT_ROLE_FIELD, DISPLAY_NAME_FIELD, ATTACHMENTS_FIELD, TIME_FIELD));
private static final long serialVersionUID = 1L;
private final String id;
private final String participantId;
private final String participantRole;
private final String displayName;
private final List attachments;
private final RealTimeContactAnalysisTimeData time;
private RealTimeContactAnalysisSegmentAttachments(BuilderImpl builder) {
this.id = builder.id;
this.participantId = builder.participantId;
this.participantRole = builder.participantRole;
this.displayName = builder.displayName;
this.attachments = builder.attachments;
this.time = builder.time;
}
/**
*
* The identifier of the segment.
*
*
* @return The identifier of the segment.
*/
public final String id() {
return id;
}
/**
*
* The identifier of the participant.
*
*
* @return The identifier of the participant.
*/
public final String participantId() {
return participantId;
}
/**
*
* The role of the participant. For example, is it a customer, agent, or system.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #participantRole}
* will return {@link ParticipantRole#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #participantRoleAsString}.
*
*
* @return The role of the participant. For example, is it a customer, agent, or system.
* @see ParticipantRole
*/
public final ParticipantRole participantRole() {
return ParticipantRole.fromValue(participantRole);
}
/**
*
* The role of the participant. For example, is it a customer, agent, or system.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #participantRole}
* will return {@link ParticipantRole#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #participantRoleAsString}.
*
*
* @return The role of the participant. For example, is it a customer, agent, or system.
* @see ParticipantRole
*/
public final String participantRoleAsString() {
return participantRole;
}
/**
*
* The display name of the participant. Can be redacted.
*
*
* @return The display name of the participant. Can be redacted.
*/
public final String displayName() {
return displayName;
}
/**
* For responses, this returns true if the service returned a value for the Attachments property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasAttachments() {
return attachments != null && !(attachments instanceof SdkAutoConstructList);
}
/**
*
* List of objects describing an individual attachment.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAttachments} method.
*
*
* @return List of objects describing an individual attachment.
*/
public final List attachments() {
return attachments;
}
/**
*
* Field describing the time of the event. It can have different representations of time.
*
*
* @return Field describing the time of the event. It can have different representations of time.
*/
public final RealTimeContactAnalysisTimeData time() {
return time;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(participantId());
hashCode = 31 * hashCode + Objects.hashCode(participantRoleAsString());
hashCode = 31 * hashCode + Objects.hashCode(displayName());
hashCode = 31 * hashCode + Objects.hashCode(hasAttachments() ? attachments() : null);
hashCode = 31 * hashCode + Objects.hashCode(time());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RealTimeContactAnalysisSegmentAttachments)) {
return false;
}
RealTimeContactAnalysisSegmentAttachments other = (RealTimeContactAnalysisSegmentAttachments) obj;
return Objects.equals(id(), other.id()) && Objects.equals(participantId(), other.participantId())
&& Objects.equals(participantRoleAsString(), other.participantRoleAsString())
&& Objects.equals(displayName(), other.displayName()) && hasAttachments() == other.hasAttachments()
&& Objects.equals(attachments(), other.attachments()) && Objects.equals(time(), other.time());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("RealTimeContactAnalysisSegmentAttachments").add("Id", id())
.add("ParticipantId", participantId()).add("ParticipantRole", participantRoleAsString())
.add("DisplayName", displayName()).add("Attachments", hasAttachments() ? attachments() : null)
.add("Time", time()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "ParticipantId":
return Optional.ofNullable(clazz.cast(participantId()));
case "ParticipantRole":
return Optional.ofNullable(clazz.cast(participantRoleAsString()));
case "DisplayName":
return Optional.ofNullable(clazz.cast(displayName()));
case "Attachments":
return Optional.ofNullable(clazz.cast(attachments()));
case "Time":
return Optional.ofNullable(clazz.cast(time()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function