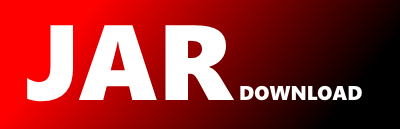
software.amazon.awssdk.services.connect.model.CreatePersistentContactAssociationRequest Maven / Gradle / Ivy
Show all versions of connect Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.connect.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreatePersistentContactAssociationRequest extends ConnectRequest implements
ToCopyableBuilder {
private static final SdkField INSTANCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InstanceId").getter(getter(CreatePersistentContactAssociationRequest::instanceId))
.setter(setter(Builder::instanceId))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("InstanceId").build()).build();
private static final SdkField INITIAL_CONTACT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InitialContactId").getter(getter(CreatePersistentContactAssociationRequest::initialContactId))
.setter(setter(Builder::initialContactId))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("InitialContactId").build()).build();
private static final SdkField REHYDRATION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RehydrationType").getter(getter(CreatePersistentContactAssociationRequest::rehydrationTypeAsString))
.setter(setter(Builder::rehydrationType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RehydrationType").build()).build();
private static final SdkField SOURCE_CONTACT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceContactId").getter(getter(CreatePersistentContactAssociationRequest::sourceContactId))
.setter(setter(Builder::sourceContactId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceContactId").build()).build();
private static final SdkField CLIENT_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClientToken").getter(getter(CreatePersistentContactAssociationRequest::clientToken))
.setter(setter(Builder::clientToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClientToken").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(INSTANCE_ID_FIELD,
INITIAL_CONTACT_ID_FIELD, REHYDRATION_TYPE_FIELD, SOURCE_CONTACT_ID_FIELD, CLIENT_TOKEN_FIELD));
private final String instanceId;
private final String initialContactId;
private final String rehydrationType;
private final String sourceContactId;
private final String clientToken;
private CreatePersistentContactAssociationRequest(BuilderImpl builder) {
super(builder);
this.instanceId = builder.instanceId;
this.initialContactId = builder.initialContactId;
this.rehydrationType = builder.rehydrationType;
this.sourceContactId = builder.sourceContactId;
this.clientToken = builder.clientToken;
}
/**
*
* The identifier of the Amazon Connect instance. You can find the instance ID in
* the Amazon Resource Name (ARN) of the instance.
*
*
* @return The identifier of the Amazon Connect instance. You can find the instance
* ID in the Amazon Resource Name (ARN) of the instance.
*/
public final String instanceId() {
return instanceId;
}
/**
*
* This is the contactId of the current contact that the CreatePersistentContactAssociation
API is
* being called from.
*
*
* @return This is the contactId of the current contact that the CreatePersistentContactAssociation
API
* is being called from.
*/
public final String initialContactId() {
return initialContactId;
}
/**
*
* The contactId chosen for rehydration depends on the type chosen.
*
*
* -
*
* ENTIRE_PAST_SESSION
: Rehydrates a chat from the most recently terminated past chat contact of the
* specified past ended chat session. To use this type, provide the initialContactId
of the past ended
* chat session in the sourceContactId
field. In this type, Amazon Connect determines what the most
* recent chat contact on the past ended chat session and uses it to start a persistent chat.
*
*
* -
*
* FROM_SEGMENT
: Rehydrates a chat from the specified past chat contact provided in the
* sourceContactId
field.
*
*
*
*
* The actual contactId used for rehydration is provided in the response of this API.
*
*
* To illustrate how to use rehydration type, consider the following example: A customer starts a chat session.
* Agent a1 accepts the chat and a conversation starts between the customer and Agent a1. This first contact creates
* a contact ID C1. Agent a1 then transfers the chat to Agent a2. This creates another contact ID C2.
* At this point Agent a2 ends the chat. The customer is forwarded to the disconnect flow for a post chat survey
* that creates another contact ID C3. After the chat survey, the chat session ends. Later, the customer
* returns and wants to resume their past chat session. At this point, the customer can have following use cases:
*
*
* -
*
* Use Case 1: The customer wants to continue the past chat session but they want to hide the post chat
* survey. For this they will use the following configuration:
*
*
* -
*
* Configuration
*
*
* -
*
* SourceContactId = "C2"
*
*
* -
*
* RehydrationType = "FROM_SEGMENT"
*
*
*
*
* -
*
* Expected behavior
*
*
* -
*
* This starts a persistent chat session from the specified past ended contact (C2). Transcripts of past chat
* sessions C2 and C1 are accessible in the current persistent chat session. Note that chat segment C3 is dropped
* from the persistent chat session.
*
*
*
*
*
*
* -
*
* Use Case 2: The customer wants to continue the past chat session and see the transcript of the entire past
* engagement, including the post chat survey. For this they will use the following configuration:
*
*
* -
*
* Configuration
*
*
* -
*
* SourceContactId = "C1"
*
*
* -
*
* RehydrationType = "ENTIRE_PAST_SESSION"
*
*
*
*
* -
*
* Expected behavior
*
*
* -
*
* This starts a persistent chat session from the most recently ended chat contact (C3). Transcripts of past chat
* sessions C3, C2 and C1 are accessible in the current persistent chat session.
*
*
*
*
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #rehydrationType}
* will return {@link RehydrationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #rehydrationTypeAsString}.
*
*
* @return The contactId chosen for rehydration depends on the type chosen.
*
* -
*
* ENTIRE_PAST_SESSION
: Rehydrates a chat from the most recently terminated past chat contact
* of the specified past ended chat session. To use this type, provide the initialContactId
of
* the past ended chat session in the sourceContactId
field. In this type, Amazon Connect
* determines what the most recent chat contact on the past ended chat session and uses it to start a
* persistent chat.
*
*
* -
*
* FROM_SEGMENT
: Rehydrates a chat from the specified past chat contact provided in the
* sourceContactId
field.
*
*
*
*
* The actual contactId used for rehydration is provided in the response of this API.
*
*
* To illustrate how to use rehydration type, consider the following example: A customer starts a chat
* session. Agent a1 accepts the chat and a conversation starts between the customer and Agent a1. This
* first contact creates a contact ID C1. Agent a1 then transfers the chat to Agent a2. This creates
* another contact ID C2. At this point Agent a2 ends the chat. The customer is forwarded to the
* disconnect flow for a post chat survey that creates another contact ID C3. After the chat survey,
* the chat session ends. Later, the customer returns and wants to resume their past chat session. At this
* point, the customer can have following use cases:
*
*
* -
*
* Use Case 1: The customer wants to continue the past chat session but they want to hide the post
* chat survey. For this they will use the following configuration:
*
*
* -
*
* Configuration
*
*
* -
*
* SourceContactId = "C2"
*
*
* -
*
* RehydrationType = "FROM_SEGMENT"
*
*
*
*
* -
*
* Expected behavior
*
*
* -
*
* This starts a persistent chat session from the specified past ended contact (C2). Transcripts of past
* chat sessions C2 and C1 are accessible in the current persistent chat session. Note that chat segment C3
* is dropped from the persistent chat session.
*
*
*
*
*
*
* -
*
* Use Case 2: The customer wants to continue the past chat session and see the transcript of the
* entire past engagement, including the post chat survey. For this they will use the following
* configuration:
*
*
* -
*
* Configuration
*
*
* -
*
* SourceContactId = "C1"
*
*
* -
*
* RehydrationType = "ENTIRE_PAST_SESSION"
*
*
*
*
* -
*
* Expected behavior
*
*
* -
*
* This starts a persistent chat session from the most recently ended chat contact (C3). Transcripts of past
* chat sessions C3, C2 and C1 are accessible in the current persistent chat session.
*
*
*
*
*
*
* @see RehydrationType
*/
public final RehydrationType rehydrationType() {
return RehydrationType.fromValue(rehydrationType);
}
/**
*
* The contactId chosen for rehydration depends on the type chosen.
*
*
* -
*
* ENTIRE_PAST_SESSION
: Rehydrates a chat from the most recently terminated past chat contact of the
* specified past ended chat session. To use this type, provide the initialContactId
of the past ended
* chat session in the sourceContactId
field. In this type, Amazon Connect determines what the most
* recent chat contact on the past ended chat session and uses it to start a persistent chat.
*
*
* -
*
* FROM_SEGMENT
: Rehydrates a chat from the specified past chat contact provided in the
* sourceContactId
field.
*
*
*
*
* The actual contactId used for rehydration is provided in the response of this API.
*
*
* To illustrate how to use rehydration type, consider the following example: A customer starts a chat session.
* Agent a1 accepts the chat and a conversation starts between the customer and Agent a1. This first contact creates
* a contact ID C1. Agent a1 then transfers the chat to Agent a2. This creates another contact ID C2.
* At this point Agent a2 ends the chat. The customer is forwarded to the disconnect flow for a post chat survey
* that creates another contact ID C3. After the chat survey, the chat session ends. Later, the customer
* returns and wants to resume their past chat session. At this point, the customer can have following use cases:
*
*
* -
*
* Use Case 1: The customer wants to continue the past chat session but they want to hide the post chat
* survey. For this they will use the following configuration:
*
*
* -
*
* Configuration
*
*
* -
*
* SourceContactId = "C2"
*
*
* -
*
* RehydrationType = "FROM_SEGMENT"
*
*
*
*
* -
*
* Expected behavior
*
*
* -
*
* This starts a persistent chat session from the specified past ended contact (C2). Transcripts of past chat
* sessions C2 and C1 are accessible in the current persistent chat session. Note that chat segment C3 is dropped
* from the persistent chat session.
*
*
*
*
*
*
* -
*
* Use Case 2: The customer wants to continue the past chat session and see the transcript of the entire past
* engagement, including the post chat survey. For this they will use the following configuration:
*
*
* -
*
* Configuration
*
*
* -
*
* SourceContactId = "C1"
*
*
* -
*
* RehydrationType = "ENTIRE_PAST_SESSION"
*
*
*
*
* -
*
* Expected behavior
*
*
* -
*
* This starts a persistent chat session from the most recently ended chat contact (C3). Transcripts of past chat
* sessions C3, C2 and C1 are accessible in the current persistent chat session.
*
*
*
*
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #rehydrationType}
* will return {@link RehydrationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #rehydrationTypeAsString}.
*
*
* @return The contactId chosen for rehydration depends on the type chosen.
*
* -
*
* ENTIRE_PAST_SESSION
: Rehydrates a chat from the most recently terminated past chat contact
* of the specified past ended chat session. To use this type, provide the initialContactId
of
* the past ended chat session in the sourceContactId
field. In this type, Amazon Connect
* determines what the most recent chat contact on the past ended chat session and uses it to start a
* persistent chat.
*
*
* -
*
* FROM_SEGMENT
: Rehydrates a chat from the specified past chat contact provided in the
* sourceContactId
field.
*
*
*
*
* The actual contactId used for rehydration is provided in the response of this API.
*
*
* To illustrate how to use rehydration type, consider the following example: A customer starts a chat
* session. Agent a1 accepts the chat and a conversation starts between the customer and Agent a1. This
* first contact creates a contact ID C1. Agent a1 then transfers the chat to Agent a2. This creates
* another contact ID C2. At this point Agent a2 ends the chat. The customer is forwarded to the
* disconnect flow for a post chat survey that creates another contact ID C3. After the chat survey,
* the chat session ends. Later, the customer returns and wants to resume their past chat session. At this
* point, the customer can have following use cases:
*
*
* -
*
* Use Case 1: The customer wants to continue the past chat session but they want to hide the post
* chat survey. For this they will use the following configuration:
*
*
* -
*
* Configuration
*
*
* -
*
* SourceContactId = "C2"
*
*
* -
*
* RehydrationType = "FROM_SEGMENT"
*
*
*
*
* -
*
* Expected behavior
*
*
* -
*
* This starts a persistent chat session from the specified past ended contact (C2). Transcripts of past
* chat sessions C2 and C1 are accessible in the current persistent chat session. Note that chat segment C3
* is dropped from the persistent chat session.
*
*
*
*
*
*
* -
*
* Use Case 2: The customer wants to continue the past chat session and see the transcript of the
* entire past engagement, including the post chat survey. For this they will use the following
* configuration:
*
*
* -
*
* Configuration
*
*
* -
*
* SourceContactId = "C1"
*
*
* -
*
* RehydrationType = "ENTIRE_PAST_SESSION"
*
*
*
*
* -
*
* Expected behavior
*
*
* -
*
* This starts a persistent chat session from the most recently ended chat contact (C3). Transcripts of past
* chat sessions C3, C2 and C1 are accessible in the current persistent chat session.
*
*
*
*
*
*
* @see RehydrationType
*/
public final String rehydrationTypeAsString() {
return rehydrationType;
}
/**
*
* The contactId from which a persistent chat session must be started.
*
*
* @return The contactId from which a persistent chat session must be started.
*/
public final String sourceContactId() {
return sourceContactId;
}
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. If not provided,
* the Amazon Web Services SDK populates this field. For more information about idempotency, see Making retries safe with
* idempotent APIs.
*
*
* @return A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. If not
* provided, the Amazon Web Services SDK populates this field. For more information about idempotency, see
* Making
* retries safe with idempotent APIs.
*/
public final String clientToken() {
return clientToken;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(instanceId());
hashCode = 31 * hashCode + Objects.hashCode(initialContactId());
hashCode = 31 * hashCode + Objects.hashCode(rehydrationTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(sourceContactId());
hashCode = 31 * hashCode + Objects.hashCode(clientToken());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreatePersistentContactAssociationRequest)) {
return false;
}
CreatePersistentContactAssociationRequest other = (CreatePersistentContactAssociationRequest) obj;
return Objects.equals(instanceId(), other.instanceId()) && Objects.equals(initialContactId(), other.initialContactId())
&& Objects.equals(rehydrationTypeAsString(), other.rehydrationTypeAsString())
&& Objects.equals(sourceContactId(), other.sourceContactId())
&& Objects.equals(clientToken(), other.clientToken());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreatePersistentContactAssociationRequest").add("InstanceId", instanceId())
.add("InitialContactId", initialContactId()).add("RehydrationType", rehydrationTypeAsString())
.add("SourceContactId", sourceContactId()).add("ClientToken", clientToken()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "InstanceId":
return Optional.ofNullable(clazz.cast(instanceId()));
case "InitialContactId":
return Optional.ofNullable(clazz.cast(initialContactId()));
case "RehydrationType":
return Optional.ofNullable(clazz.cast(rehydrationTypeAsString()));
case "SourceContactId":
return Optional.ofNullable(clazz.cast(sourceContactId()));
case "ClientToken":
return Optional.ofNullable(clazz.cast(clientToken()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function