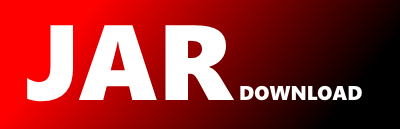
software.amazon.awssdk.services.connect.model.SearchCriteria Maven / Gradle / Ivy
Show all versions of connect Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.connect.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A structure of search criteria to be used to return contacts.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SearchCriteria implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField> AGENT_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AgentIds")
.getter(getter(SearchCriteria::agentIds))
.setter(setter(Builder::agentIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AgentIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField AGENT_HIERARCHY_GROUPS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AgentHierarchyGroups")
.getter(getter(SearchCriteria::agentHierarchyGroups)).setter(setter(Builder::agentHierarchyGroups))
.constructor(AgentHierarchyGroups::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AgentHierarchyGroups").build())
.build();
private static final SdkField> CHANNELS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Channels")
.getter(getter(SearchCriteria::channelsAsStrings))
.setter(setter(Builder::channelsWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Channels").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CONTACT_ANALYSIS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ContactAnalysis")
.getter(getter(SearchCriteria::contactAnalysis)).setter(setter(Builder::contactAnalysis))
.constructor(ContactAnalysis::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ContactAnalysis").build()).build();
private static final SdkField> INITIATION_METHODS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("InitiationMethods")
.getter(getter(SearchCriteria::initiationMethodsAsStrings))
.setter(setter(Builder::initiationMethodsWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InitiationMethods").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> QUEUE_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("QueueIds")
.getter(getter(SearchCriteria::queueIds))
.setter(setter(Builder::queueIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("QueueIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SEARCHABLE_CONTACT_ATTRIBUTES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("SearchableContactAttributes")
.getter(getter(SearchCriteria::searchableContactAttributes))
.setter(setter(Builder::searchableContactAttributes))
.constructor(SearchableContactAttributes::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SearchableContactAttributes")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AGENT_IDS_FIELD,
AGENT_HIERARCHY_GROUPS_FIELD, CHANNELS_FIELD, CONTACT_ANALYSIS_FIELD, INITIATION_METHODS_FIELD, QUEUE_IDS_FIELD,
SEARCHABLE_CONTACT_ATTRIBUTES_FIELD));
private static final long serialVersionUID = 1L;
private final List agentIds;
private final AgentHierarchyGroups agentHierarchyGroups;
private final List channels;
private final ContactAnalysis contactAnalysis;
private final List initiationMethods;
private final List queueIds;
private final SearchableContactAttributes searchableContactAttributes;
private SearchCriteria(BuilderImpl builder) {
this.agentIds = builder.agentIds;
this.agentHierarchyGroups = builder.agentHierarchyGroups;
this.channels = builder.channels;
this.contactAnalysis = builder.contactAnalysis;
this.initiationMethods = builder.initiationMethods;
this.queueIds = builder.queueIds;
this.searchableContactAttributes = builder.searchableContactAttributes;
}
/**
* For responses, this returns true if the service returned a value for the AgentIds property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasAgentIds() {
return agentIds != null && !(agentIds instanceof SdkAutoConstructList);
}
/**
*
* The identifiers of agents who handled the contacts.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAgentIds} method.
*
*
* @return The identifiers of agents who handled the contacts.
*/
public final List agentIds() {
return agentIds;
}
/**
*
* The agent hierarchy groups of the agent at the time of handling the contact.
*
*
* @return The agent hierarchy groups of the agent at the time of handling the contact.
*/
public final AgentHierarchyGroups agentHierarchyGroups() {
return agentHierarchyGroups;
}
/**
*
* The list of channels associated with contacts.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasChannels} method.
*
*
* @return The list of channels associated with contacts.
*/
public final List channels() {
return ChannelListCopier.copyStringToEnum(channels);
}
/**
* For responses, this returns true if the service returned a value for the Channels property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasChannels() {
return channels != null && !(channels instanceof SdkAutoConstructList);
}
/**
*
* The list of channels associated with contacts.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasChannels} method.
*
*
* @return The list of channels associated with contacts.
*/
public final List channelsAsStrings() {
return channels;
}
/**
*
* Search criteria based on analysis outputs from Amazon Connect Contact Lens.
*
*
* @return Search criteria based on analysis outputs from Amazon Connect Contact Lens.
*/
public final ContactAnalysis contactAnalysis() {
return contactAnalysis;
}
/**
*
* The list of initiation methods associated with contacts.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasInitiationMethods} method.
*
*
* @return The list of initiation methods associated with contacts.
*/
public final List initiationMethods() {
return InitiationMethodListCopier.copyStringToEnum(initiationMethods);
}
/**
* For responses, this returns true if the service returned a value for the InitiationMethods property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasInitiationMethods() {
return initiationMethods != null && !(initiationMethods instanceof SdkAutoConstructList);
}
/**
*
* The list of initiation methods associated with contacts.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasInitiationMethods} method.
*
*
* @return The list of initiation methods associated with contacts.
*/
public final List initiationMethodsAsStrings() {
return initiationMethods;
}
/**
* For responses, this returns true if the service returned a value for the QueueIds property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasQueueIds() {
return queueIds != null && !(queueIds instanceof SdkAutoConstructList);
}
/**
*
* The list of queue IDs associated with contacts.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasQueueIds} method.
*
*
* @return The list of queue IDs associated with contacts.
*/
public final List queueIds() {
return queueIds;
}
/**
*
* The search criteria based on user-defined contact attributes that have been configured for contact search. For
* more information, see Search by custom
* contact attributes in the Amazon Connect Administrator Guide.
*
*
*
* To use SearchableContactAttributes
in a search request, the GetContactAttributes
action
* is required to perform an API request. For more information, see https://docs.aws.amazon.com/service-authorization/latest/reference/list_amazonconnect.html#amazonconnect-actions
* -as-permissionsActions defined by Amazon Connect.
*
*
*
* @return The search criteria based on user-defined contact attributes that have been configured for contact
* search. For more information, see Search by
* custom contact attributes in the Amazon Connect Administrator Guide.
*
* To use SearchableContactAttributes
in a search request, the
* GetContactAttributes
action is required to perform an API request. For more information, see
* https://docs.aws.amazon.com/service-authorization/latest/reference/list_amazonconnect.html#amazonconnect
* -actions-as-permissionsActions defined by Amazon Connect.
*
*/
public final SearchableContactAttributes searchableContactAttributes() {
return searchableContactAttributes;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasAgentIds() ? agentIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(agentHierarchyGroups());
hashCode = 31 * hashCode + Objects.hashCode(hasChannels() ? channelsAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(contactAnalysis());
hashCode = 31 * hashCode + Objects.hashCode(hasInitiationMethods() ? initiationMethodsAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasQueueIds() ? queueIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(searchableContactAttributes());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SearchCriteria)) {
return false;
}
SearchCriteria other = (SearchCriteria) obj;
return hasAgentIds() == other.hasAgentIds() && Objects.equals(agentIds(), other.agentIds())
&& Objects.equals(agentHierarchyGroups(), other.agentHierarchyGroups()) && hasChannels() == other.hasChannels()
&& Objects.equals(channelsAsStrings(), other.channelsAsStrings())
&& Objects.equals(contactAnalysis(), other.contactAnalysis())
&& hasInitiationMethods() == other.hasInitiationMethods()
&& Objects.equals(initiationMethodsAsStrings(), other.initiationMethodsAsStrings())
&& hasQueueIds() == other.hasQueueIds() && Objects.equals(queueIds(), other.queueIds())
&& Objects.equals(searchableContactAttributes(), other.searchableContactAttributes());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SearchCriteria").add("AgentIds", hasAgentIds() ? agentIds() : null)
.add("AgentHierarchyGroups", agentHierarchyGroups()).add("Channels", hasChannels() ? channelsAsStrings() : null)
.add("ContactAnalysis", contactAnalysis())
.add("InitiationMethods", hasInitiationMethods() ? initiationMethodsAsStrings() : null)
.add("QueueIds", hasQueueIds() ? queueIds() : null)
.add("SearchableContactAttributes", searchableContactAttributes()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AgentIds":
return Optional.ofNullable(clazz.cast(agentIds()));
case "AgentHierarchyGroups":
return Optional.ofNullable(clazz.cast(agentHierarchyGroups()));
case "Channels":
return Optional.ofNullable(clazz.cast(channelsAsStrings()));
case "ContactAnalysis":
return Optional.ofNullable(clazz.cast(contactAnalysis()));
case "InitiationMethods":
return Optional.ofNullable(clazz.cast(initiationMethodsAsStrings()));
case "QueueIds":
return Optional.ofNullable(clazz.cast(queueIds()));
case "SearchableContactAttributes":
return Optional.ofNullable(clazz.cast(searchableContactAttributes()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function