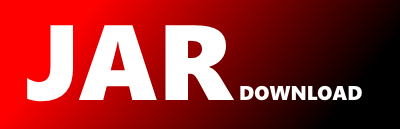
software.amazon.awssdk.services.connectparticipant.model.CreateParticipantConnectionRequest Maven / Gradle / Ivy
Show all versions of connectparticipant Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.connectparticipant.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateParticipantConnectionRequest extends ConnectParticipantRequest implements
ToCopyableBuilder {
private static final SdkField> TYPE_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Type")
.getter(getter(CreateParticipantConnectionRequest::typeAsStrings))
.setter(setter(Builder::typeWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Type").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PARTICIPANT_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ParticipantToken").getter(getter(CreateParticipantConnectionRequest::participantToken))
.setter(setter(Builder::participantToken))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("X-Amz-Bearer").build()).build();
private static final SdkField CONNECT_PARTICIPANT_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ConnectParticipant").getter(getter(CreateParticipantConnectionRequest::connectParticipant))
.setter(setter(Builder::connectParticipant))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConnectParticipant").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TYPE_FIELD,
PARTICIPANT_TOKEN_FIELD, CONNECT_PARTICIPANT_FIELD));
private final List type;
private final String participantToken;
private final Boolean connectParticipant;
private CreateParticipantConnectionRequest(BuilderImpl builder) {
super(builder);
this.type = builder.type;
this.participantToken = builder.participantToken;
this.connectParticipant = builder.connectParticipant;
}
/**
*
* Type of connection information required. If you need CONNECTION_CREDENTIALS
along with marking
* participant as connected, pass CONNECTION_CREDENTIALS
in Type
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasType} method.
*
*
* @return Type of connection information required. If you need CONNECTION_CREDENTIALS
along with
* marking participant as connected, pass CONNECTION_CREDENTIALS
in Type
.
*/
public final List type() {
return ConnectionTypeListCopier.copyStringToEnum(type);
}
/**
* For responses, this returns true if the service returned a value for the Type property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasType() {
return type != null && !(type instanceof SdkAutoConstructList);
}
/**
*
* Type of connection information required. If you need CONNECTION_CREDENTIALS
along with marking
* participant as connected, pass CONNECTION_CREDENTIALS
in Type
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasType} method.
*
*
* @return Type of connection information required. If you need CONNECTION_CREDENTIALS
along with
* marking participant as connected, pass CONNECTION_CREDENTIALS
in Type
.
*/
public final List typeAsStrings() {
return type;
}
/**
*
* This is a header parameter.
*
*
* The ParticipantToken as obtained from StartChatContact API
* response.
*
*
* @return This is a header parameter.
*
* The ParticipantToken as obtained from StartChatContact API response.
*/
public final String participantToken() {
return participantToken;
}
/**
*
* Amazon Connect Participant is used to mark the participant as connected for customer participant in message
* streaming, as well as for agent or manager participant in non-streaming chats.
*
*
* @return Amazon Connect Participant is used to mark the participant as connected for customer participant in
* message streaming, as well as for agent or manager participant in non-streaming chats.
*/
public final Boolean connectParticipant() {
return connectParticipant;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(hasType() ? typeAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(participantToken());
hashCode = 31 * hashCode + Objects.hashCode(connectParticipant());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateParticipantConnectionRequest)) {
return false;
}
CreateParticipantConnectionRequest other = (CreateParticipantConnectionRequest) obj;
return hasType() == other.hasType() && Objects.equals(typeAsStrings(), other.typeAsStrings())
&& Objects.equals(participantToken(), other.participantToken())
&& Objects.equals(connectParticipant(), other.connectParticipant());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateParticipantConnectionRequest").add("Type", hasType() ? typeAsStrings() : null)
.add("ParticipantToken", participantToken()).add("ConnectParticipant", connectParticipant()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Type":
return Optional.ofNullable(clazz.cast(typeAsStrings()));
case "ParticipantToken":
return Optional.ofNullable(clazz.cast(participantToken()));
case "ConnectParticipant":
return Optional.ofNullable(clazz.cast(connectParticipant()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function