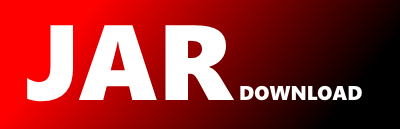
software.amazon.awssdk.services.costexplorer.CostExplorerClient Maven / Gradle / Ivy
Show all versions of cost-explorer Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.costexplorer;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.costexplorer.model.CostExplorerException;
import software.amazon.awssdk.services.costexplorer.model.GetCostAndUsageRequest;
import software.amazon.awssdk.services.costexplorer.model.GetCostAndUsageResponse;
import software.amazon.awssdk.services.costexplorer.model.GetDimensionValuesRequest;
import software.amazon.awssdk.services.costexplorer.model.GetDimensionValuesResponse;
import software.amazon.awssdk.services.costexplorer.model.GetReservationUtilizationRequest;
import software.amazon.awssdk.services.costexplorer.model.GetReservationUtilizationResponse;
import software.amazon.awssdk.services.costexplorer.model.GetTagsRequest;
import software.amazon.awssdk.services.costexplorer.model.GetTagsResponse;
import software.amazon.awssdk.services.costexplorer.model.LimitExceededException;
/**
* Service client for accessing AWS Cost Explorer. This can be created using the static {@link #builder()} method.
*
*
* The Cost Explorer API allows you to programmatically query your cost and usage data. You can query for aggregated
* data such as total monthly costs or total daily usage. You can also query for granular data, such as the number of
* daily write operations for DynamoDB database tables in your production environment.
*
*
* Service Endpoint
*
*
* The Cost Explorer API provides the following endpoint:
*
*
* -
*
* https://ce.us-east-1.amazonaws.com
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface CostExplorerClient extends SdkClient {
String SERVICE_NAME = "ce";
/**
* Create a {@link CostExplorerClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static CostExplorerClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link CostExplorerClient}.
*/
static CostExplorerClientBuilder builder() {
return new DefaultCostExplorerClientBuilder();
}
/**
*
* Retrieve cost and usage metrics for your account. You can specify which cost and usage-related metric, such as
* BlendedCosts
or UsageQuantity
, that you want the request to return. You can also filter
* and group your data by various dimensions, such as AWS Service
or AvailabilityZone
, in
* a specific time range. See the GetDimensionValues
action for a complete list of the valid
* dimensions. Master accounts in an organization have access to all member accounts.
*
*
* @param getCostAndUsageRequest
* @return Result of the GetCostAndUsage operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetCostAndUsage
* @see AWS API
* Documentation
*/
default GetCostAndUsageResponse getCostAndUsage(GetCostAndUsageRequest getCostAndUsageRequest) throws LimitExceededException,
AwsServiceException, SdkClientException, CostExplorerException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieve cost and usage metrics for your account. You can specify which cost and usage-related metric, such as
* BlendedCosts
or UsageQuantity
, that you want the request to return. You can also filter
* and group your data by various dimensions, such as AWS Service
or AvailabilityZone
, in
* a specific time range. See the GetDimensionValues
action for a complete list of the valid
* dimensions. Master accounts in an organization have access to all member accounts.
*
*
*
* This is a convenience which creates an instance of the {@link GetCostAndUsageRequest.Builder} avoiding the need
* to create one manually via {@link GetCostAndUsageRequest#builder()}
*
*
* @param getCostAndUsageRequest
* A {@link Consumer} that will call methods on {@link GetCostAndUsageRequest.Builder} to create a request.
* @return Result of the GetCostAndUsage operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetCostAndUsage
* @see AWS API
* Documentation
*/
default GetCostAndUsageResponse getCostAndUsage(Consumer getCostAndUsageRequest)
throws LimitExceededException, AwsServiceException, SdkClientException, CostExplorerException {
return getCostAndUsage(GetCostAndUsageRequest.builder().applyMutation(getCostAndUsageRequest).build());
}
/**
*
* You can use GetDimensionValues
to retrieve all available filter values for a specific filter over a
* period of time. You can search the dimension values for an arbitrary string.
*
*
* @param getDimensionValuesRequest
* @return Result of the GetDimensionValues operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetDimensionValues
* @see AWS API
* Documentation
*/
default GetDimensionValuesResponse getDimensionValues(GetDimensionValuesRequest getDimensionValuesRequest)
throws LimitExceededException, AwsServiceException, SdkClientException, CostExplorerException {
throw new UnsupportedOperationException();
}
/**
*
* You can use GetDimensionValues
to retrieve all available filter values for a specific filter over a
* period of time. You can search the dimension values for an arbitrary string.
*
*
*
* This is a convenience which creates an instance of the {@link GetDimensionValuesRequest.Builder} avoiding the
* need to create one manually via {@link GetDimensionValuesRequest#builder()}
*
*
* @param getDimensionValuesRequest
* A {@link Consumer} that will call methods on {@link GetDimensionValuesRequest.Builder} to create a
* request.
* @return Result of the GetDimensionValues operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetDimensionValues
* @see AWS API
* Documentation
*/
default GetDimensionValuesResponse getDimensionValues(Consumer getDimensionValuesRequest)
throws LimitExceededException, AwsServiceException, SdkClientException, CostExplorerException {
return getDimensionValues(GetDimensionValuesRequest.builder().applyMutation(getDimensionValuesRequest).build());
}
/**
*
* You can retrieve the Reservation utilization for your account. Master accounts in an organization have access to
* their associated member accounts. You can filter data by dimensions in a time period. You can use
* GetDimensionValues
to determine the possible dimension values. Currently, you can group only by
* SUBSCRIPTION_ID
.
*
*
* @param getReservationUtilizationRequest
* @return Result of the GetReservationUtilization operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetReservationUtilization
* @see AWS
* API Documentation
*/
default GetReservationUtilizationResponse getReservationUtilization(
GetReservationUtilizationRequest getReservationUtilizationRequest) throws LimitExceededException,
AwsServiceException, SdkClientException, CostExplorerException {
throw new UnsupportedOperationException();
}
/**
*
* You can retrieve the Reservation utilization for your account. Master accounts in an organization have access to
* their associated member accounts. You can filter data by dimensions in a time period. You can use
* GetDimensionValues
to determine the possible dimension values. Currently, you can group only by
* SUBSCRIPTION_ID
.
*
*
*
* This is a convenience which creates an instance of the {@link GetReservationUtilizationRequest.Builder} avoiding
* the need to create one manually via {@link GetReservationUtilizationRequest#builder()}
*
*
* @param getReservationUtilizationRequest
* A {@link Consumer} that will call methods on {@link GetReservationUtilizationRequest.Builder} to create a
* request.
* @return Result of the GetReservationUtilization operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetReservationUtilization
* @see AWS
* API Documentation
*/
default GetReservationUtilizationResponse getReservationUtilization(
Consumer getReservationUtilizationRequest) throws LimitExceededException,
AwsServiceException, SdkClientException, CostExplorerException {
return getReservationUtilization(GetReservationUtilizationRequest.builder()
.applyMutation(getReservationUtilizationRequest).build());
}
/**
*
* You can query for available tag keys and tag values for a specified period. You can search the tag values for an
* arbitrary string.
*
*
* @param getTagsRequest
* @return Result of the GetTags operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetTags
* @see AWS API
* Documentation
*/
default GetTagsResponse getTags(GetTagsRequest getTagsRequest) throws LimitExceededException, AwsServiceException,
SdkClientException, CostExplorerException {
throw new UnsupportedOperationException();
}
/**
*
* You can query for available tag keys and tag values for a specified period. You can search the tag values for an
* arbitrary string.
*
*
*
* This is a convenience which creates an instance of the {@link GetTagsRequest.Builder} avoiding the need to create
* one manually via {@link GetTagsRequest#builder()}
*
*
* @param getTagsRequest
* A {@link Consumer} that will call methods on {@link GetTagsRequest.Builder} to create a request.
* @return Result of the GetTags operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetTags
* @see AWS API
* Documentation
*/
default GetTagsResponse getTags(Consumer getTagsRequest) throws LimitExceededException,
AwsServiceException, SdkClientException, CostExplorerException {
return getTags(GetTagsRequest.builder().applyMutation(getTagsRequest).build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of("ce");
}
}