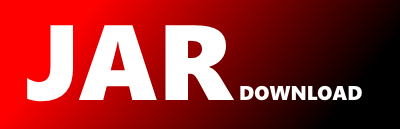
software.amazon.awssdk.services.costexplorer.DefaultCostExplorerClient Maven / Gradle / Ivy
Show all versions of cost-explorer Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.costexplorer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.protocol.json.AwsJsonProtocol;
import software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolMetadata;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.internal.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.protocol.json.JsonClientMetadata;
import software.amazon.awssdk.core.protocol.json.JsonErrorResponseMetadata;
import software.amazon.awssdk.core.protocol.json.JsonErrorShapeMetadata;
import software.amazon.awssdk.core.protocol.json.JsonOperationMetadata;
import software.amazon.awssdk.services.costexplorer.model.CostExplorerException;
import software.amazon.awssdk.services.costexplorer.model.GetCostAndUsageRequest;
import software.amazon.awssdk.services.costexplorer.model.GetCostAndUsageResponse;
import software.amazon.awssdk.services.costexplorer.model.GetDimensionValuesRequest;
import software.amazon.awssdk.services.costexplorer.model.GetDimensionValuesResponse;
import software.amazon.awssdk.services.costexplorer.model.GetReservationUtilizationRequest;
import software.amazon.awssdk.services.costexplorer.model.GetReservationUtilizationResponse;
import software.amazon.awssdk.services.costexplorer.model.GetTagsRequest;
import software.amazon.awssdk.services.costexplorer.model.GetTagsResponse;
import software.amazon.awssdk.services.costexplorer.model.LimitExceededException;
import software.amazon.awssdk.services.costexplorer.transform.GetCostAndUsageRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetCostAndUsageResponseUnmarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetDimensionValuesRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetDimensionValuesResponseUnmarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetReservationUtilizationRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetReservationUtilizationResponseUnmarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetTagsRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetTagsResponseUnmarshaller;
/**
* Internal implementation of {@link CostExplorerClient}.
*
* @see CostExplorerClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultCostExplorerClient implements CostExplorerClient {
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultCostExplorerClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.protocolFactory = init();
this.clientConfiguration = clientConfiguration;
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Retrieve cost and usage metrics for your account. You can specify which cost and usage-related metric, such as
* BlendedCosts
or UsageQuantity
, that you want the request to return. You can also filter
* and group your data by various dimensions, such as AWS Service
or AvailabilityZone
, in
* a specific time range. See the GetDimensionValues
action for a complete list of the valid
* dimensions. Master accounts in an organization have access to all member accounts.
*
*
* @param getCostAndUsageRequest
* @return Result of the GetCostAndUsage operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetCostAndUsage
* @see AWS API
* Documentation
*/
@Override
public GetCostAndUsageResponse getCostAndUsage(GetCostAndUsageRequest getCostAndUsageRequest) throws LimitExceededException,
AwsServiceException, SdkClientException, CostExplorerException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetCostAndUsageResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getCostAndUsageRequest).withMarshaller(new GetCostAndUsageRequestMarshaller(protocolFactory)));
}
/**
*
* You can use GetDimensionValues
to retrieve all available filter values for a specific filter over a
* period of time. You can search the dimension values for an arbitrary string.
*
*
* @param getDimensionValuesRequest
* @return Result of the GetDimensionValues operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetDimensionValues
* @see AWS API
* Documentation
*/
@Override
public GetDimensionValuesResponse getDimensionValues(GetDimensionValuesRequest getDimensionValuesRequest)
throws LimitExceededException, AwsServiceException, SdkClientException, CostExplorerException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetDimensionValuesResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getDimensionValuesRequest).withMarshaller(new GetDimensionValuesRequestMarshaller(protocolFactory)));
}
/**
*
* You can retrieve the Reservation utilization for your account. Master accounts in an organization have access to
* their associated member accounts. You can filter data by dimensions in a time period. You can use
* GetDimensionValues
to determine the possible dimension values. Currently, you can group only by
* SUBSCRIPTION_ID
.
*
*
* @param getReservationUtilizationRequest
* @return Result of the GetReservationUtilization operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetReservationUtilization
* @see AWS
* API Documentation
*/
@Override
public GetReservationUtilizationResponse getReservationUtilization(
GetReservationUtilizationRequest getReservationUtilizationRequest) throws LimitExceededException,
AwsServiceException, SdkClientException, CostExplorerException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetReservationUtilizationResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getReservationUtilizationRequest)
.withMarshaller(new GetReservationUtilizationRequestMarshaller(protocolFactory)));
}
/**
*
* You can query for available tag keys and tag values for a specified period. You can search the tag values for an
* arbitrary string.
*
*
* @param getTagsRequest
* @return Result of the GetTags operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetTags
* @see AWS API
* Documentation
*/
@Override
public GetTagsResponse getTags(GetTagsRequest getTagsRequest) throws LimitExceededException, AwsServiceException,
SdkClientException, CostExplorerException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetTagsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler).withInput(getTagsRequest)
.withMarshaller(new GetTagsRequestMarshaller(protocolFactory)));
}
private HttpResponseHandler createErrorResponseHandler() {
return protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
}
private software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolFactory init() {
return new AwsJsonProtocolFactory(new JsonClientMetadata()
.withSupportsCbor(false)
.withSupportsIon(false)
.withBaseServiceExceptionClass(software.amazon.awssdk.services.costexplorer.model.CostExplorerException.class)
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("LimitExceededException").withModeledClass(
LimitExceededException.class)), AwsJsonProtocolMetadata.builder().protocolVersion("1.1")
.protocol(AwsJsonProtocol.AWS_JSON).build());
}
@Override
public void close() {
clientHandler.close();
}
}