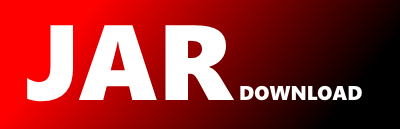
software.amazon.awssdk.services.costexplorer.model.ReservationAggregates Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cost-explorer Show documentation
Show all versions of cost-explorer Show documentation
The AWS Java SDK for AWS Cost Explorer module holds the client classes that are used for communicating
with AWS Cost Explorer Service
The newest version!
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.costexplorer.model;
import java.util.Objects;
import java.util.Optional;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.core.protocol.ProtocolMarshaller;
import software.amazon.awssdk.core.protocol.StructuredPojo;
import software.amazon.awssdk.services.costexplorer.transform.ReservationAggregatesMarshaller;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The aggregated numbers for your RI usage.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ReservationAggregates implements StructuredPojo,
ToCopyableBuilder {
private final String utilizationPercentage;
private final String purchasedHours;
private final String totalActualHours;
private final String unusedHours;
private ReservationAggregates(BuilderImpl builder) {
this.utilizationPercentage = builder.utilizationPercentage;
this.purchasedHours = builder.purchasedHours;
this.totalActualHours = builder.totalActualHours;
this.unusedHours = builder.unusedHours;
}
/**
*
* The percentage of RI time that you used.
*
*
* @return The percentage of RI time that you used.
*/
public String utilizationPercentage() {
return utilizationPercentage;
}
/**
*
* How many RI hours you purchased.
*
*
* @return How many RI hours you purchased.
*/
public String purchasedHours() {
return purchasedHours;
}
/**
*
* The total number of RI hours that you used.
*
*
* @return The total number of RI hours that you used.
*/
public String totalActualHours() {
return totalActualHours;
}
/**
*
* The number of RI hours that you didn't use.
*
*
* @return The number of RI hours that you didn't use.
*/
public String unusedHours() {
return unusedHours;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(utilizationPercentage());
hashCode = 31 * hashCode + Objects.hashCode(purchasedHours());
hashCode = 31 * hashCode + Objects.hashCode(totalActualHours());
hashCode = 31 * hashCode + Objects.hashCode(unusedHours());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ReservationAggregates)) {
return false;
}
ReservationAggregates other = (ReservationAggregates) obj;
return Objects.equals(utilizationPercentage(), other.utilizationPercentage())
&& Objects.equals(purchasedHours(), other.purchasedHours())
&& Objects.equals(totalActualHours(), other.totalActualHours())
&& Objects.equals(unusedHours(), other.unusedHours());
}
@Override
public String toString() {
return ToString.builder("ReservationAggregates").add("UtilizationPercentage", utilizationPercentage())
.add("PurchasedHours", purchasedHours()).add("TotalActualHours", totalActualHours())
.add("UnusedHours", unusedHours()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "UtilizationPercentage":
return Optional.ofNullable(clazz.cast(utilizationPercentage()));
case "PurchasedHours":
return Optional.ofNullable(clazz.cast(purchasedHours()));
case "TotalActualHours":
return Optional.ofNullable(clazz.cast(totalActualHours()));
case "UnusedHours":
return Optional.ofNullable(clazz.cast(unusedHours()));
default:
return Optional.empty();
}
}
@SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
ReservationAggregatesMarshaller.getInstance().marshall(this, protocolMarshaller);
}
public interface Builder extends CopyableBuilder {
/**
*
* The percentage of RI time that you used.
*
*
* @param utilizationPercentage
* The percentage of RI time that you used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder utilizationPercentage(String utilizationPercentage);
/**
*
* How many RI hours you purchased.
*
*
* @param purchasedHours
* How many RI hours you purchased.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder purchasedHours(String purchasedHours);
/**
*
* The total number of RI hours that you used.
*
*
* @param totalActualHours
* The total number of RI hours that you used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder totalActualHours(String totalActualHours);
/**
*
* The number of RI hours that you didn't use.
*
*
* @param unusedHours
* The number of RI hours that you didn't use.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder unusedHours(String unusedHours);
}
static final class BuilderImpl implements Builder {
private String utilizationPercentage;
private String purchasedHours;
private String totalActualHours;
private String unusedHours;
private BuilderImpl() {
}
private BuilderImpl(ReservationAggregates model) {
utilizationPercentage(model.utilizationPercentage);
purchasedHours(model.purchasedHours);
totalActualHours(model.totalActualHours);
unusedHours(model.unusedHours);
}
public final String getUtilizationPercentage() {
return utilizationPercentage;
}
@Override
public final Builder utilizationPercentage(String utilizationPercentage) {
this.utilizationPercentage = utilizationPercentage;
return this;
}
public final void setUtilizationPercentage(String utilizationPercentage) {
this.utilizationPercentage = utilizationPercentage;
}
public final String getPurchasedHours() {
return purchasedHours;
}
@Override
public final Builder purchasedHours(String purchasedHours) {
this.purchasedHours = purchasedHours;
return this;
}
public final void setPurchasedHours(String purchasedHours) {
this.purchasedHours = purchasedHours;
}
public final String getTotalActualHours() {
return totalActualHours;
}
@Override
public final Builder totalActualHours(String totalActualHours) {
this.totalActualHours = totalActualHours;
return this;
}
public final void setTotalActualHours(String totalActualHours) {
this.totalActualHours = totalActualHours;
}
public final String getUnusedHours() {
return unusedHours;
}
@Override
public final Builder unusedHours(String unusedHours) {
this.unusedHours = unusedHours;
return this;
}
public final void setUnusedHours(String unusedHours) {
this.unusedHours = unusedHours;
}
@Override
public ReservationAggregates build() {
return new ReservationAggregates(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy