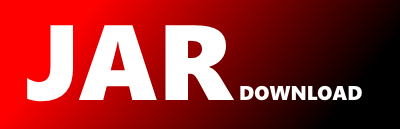
software.amazon.awssdk.services.costexplorer.model.ResultByTime Maven / Gradle / Ivy
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.costexplorer.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.core.protocol.ProtocolMarshaller;
import software.amazon.awssdk.core.protocol.StructuredPojo;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructMap;
import software.amazon.awssdk.services.costexplorer.transform.ResultByTimeMarshaller;
import software.amazon.awssdk.utils.CollectionUtils;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The result that is associated with a time period.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ResultByTime implements StructuredPojo, ToCopyableBuilder {
private final DateInterval timePeriod;
private final Map total;
private final List groups;
private final Boolean estimated;
private ResultByTime(BuilderImpl builder) {
this.timePeriod = builder.timePeriod;
this.total = builder.total;
this.groups = builder.groups;
this.estimated = builder.estimated;
}
/**
*
* The time period covered by a result.
*
*
* @return The time period covered by a result.
*/
public DateInterval timePeriod() {
return timePeriod;
}
/**
*
* The total amount of cost or usage accrued during the time period.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The total amount of cost or usage accrued during the time period.
*/
public Map total() {
return total;
}
/**
*
* The groups that are included in this time period.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The groups that are included in this time period.
*/
public List groups() {
return groups;
}
/**
*
* Whether or not this result is estimated.
*
*
* @return Whether or not this result is estimated.
*/
public Boolean estimated() {
return estimated;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(timePeriod());
hashCode = 31 * hashCode + Objects.hashCode(total());
hashCode = 31 * hashCode + Objects.hashCode(groups());
hashCode = 31 * hashCode + Objects.hashCode(estimated());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ResultByTime)) {
return false;
}
ResultByTime other = (ResultByTime) obj;
return Objects.equals(timePeriod(), other.timePeriod()) && Objects.equals(total(), other.total())
&& Objects.equals(groups(), other.groups()) && Objects.equals(estimated(), other.estimated());
}
@Override
public String toString() {
return ToString.builder("ResultByTime").add("TimePeriod", timePeriod()).add("Total", total()).add("Groups", groups())
.add("Estimated", estimated()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "TimePeriod":
return Optional.ofNullable(clazz.cast(timePeriod()));
case "Total":
return Optional.ofNullable(clazz.cast(total()));
case "Groups":
return Optional.ofNullable(clazz.cast(groups()));
case "Estimated":
return Optional.ofNullable(clazz.cast(estimated()));
default:
return Optional.empty();
}
}
@SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
ResultByTimeMarshaller.getInstance().marshall(this, protocolMarshaller);
}
public interface Builder extends CopyableBuilder {
/**
*
* The time period covered by a result.
*
*
* @param timePeriod
* The time period covered by a result.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder timePeriod(DateInterval timePeriod);
/**
*
* The time period covered by a result.
*
* This is a convenience that creates an instance of the {@link DateInterval.Builder} avoiding the need to
* create one manually via {@link DateInterval#builder()}.
*
* When the {@link Consumer} completes, {@link DateInterval.Builder#build()} is called immediately and its
* result is passed to {@link #timePeriod(DateInterval)}.
*
* @param timePeriod
* a consumer that will call methods on {@link DateInterval.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #timePeriod(DateInterval)
*/
default Builder timePeriod(Consumer timePeriod) {
return timePeriod(DateInterval.builder().applyMutation(timePeriod).build());
}
/**
*
* The total amount of cost or usage accrued during the time period.
*
*
* @param total
* The total amount of cost or usage accrued during the time period.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder total(Map total);
/**
*
* The groups that are included in this time period.
*
*
* @param groups
* The groups that are included in this time period.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder groups(Collection groups);
/**
*
* The groups that are included in this time period.
*
*
* @param groups
* The groups that are included in this time period.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder groups(Group... groups);
/**
*
* The groups that are included in this time period.
*
* This is a convenience that creates an instance of the {@link List.Builder} avoiding the need to create
* one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and its result
* is passed to {@link #groups(List)}.
*
* @param groups
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #groups(List)
*/
Builder groups(Consumer... groups);
/**
*
* Whether or not this result is estimated.
*
*
* @param estimated
* Whether or not this result is estimated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder estimated(Boolean estimated);
}
static final class BuilderImpl implements Builder {
private DateInterval timePeriod;
private Map total = DefaultSdkAutoConstructMap.getInstance();
private List groups = DefaultSdkAutoConstructList.getInstance();
private Boolean estimated;
private BuilderImpl() {
}
private BuilderImpl(ResultByTime model) {
timePeriod(model.timePeriod);
total(model.total);
groups(model.groups);
estimated(model.estimated);
}
public final DateInterval.Builder getTimePeriod() {
return timePeriod != null ? timePeriod.toBuilder() : null;
}
@Override
public final Builder timePeriod(DateInterval timePeriod) {
this.timePeriod = timePeriod;
return this;
}
public final void setTimePeriod(DateInterval.BuilderImpl timePeriod) {
this.timePeriod = timePeriod != null ? timePeriod.build() : null;
}
public final Map getTotal() {
return total != null ? CollectionUtils.mapValues(total, MetricValue::toBuilder) : null;
}
@Override
public final Builder total(Map total) {
this.total = MetricsCopier.copy(total);
return this;
}
public final void setTotal(Map total) {
this.total = MetricsCopier.copyFromBuilder(total);
}
public final Collection getGroups() {
return groups != null ? groups.stream().map(Group::toBuilder).collect(Collectors.toList()) : null;
}
@Override
public final Builder groups(Collection groups) {
this.groups = GroupsCopier.copy(groups);
return this;
}
@Override
@SafeVarargs
public final Builder groups(Group... groups) {
groups(Arrays.asList(groups));
return this;
}
@Override
@SafeVarargs
public final Builder groups(Consumer... groups) {
groups(Stream.of(groups).map(c -> Group.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final void setGroups(Collection groups) {
this.groups = GroupsCopier.copyFromBuilder(groups);
}
public final Boolean getEstimated() {
return estimated;
}
@Override
public final Builder estimated(Boolean estimated) {
this.estimated = estimated;
return this;
}
public final void setEstimated(Boolean estimated) {
this.estimated = estimated;
}
@Override
public ResultByTime build() {
return new ResultByTime(this);
}
}
}