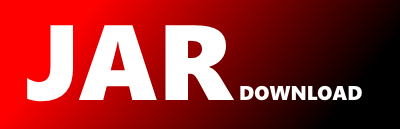
software.amazon.awssdk.services.costexplorer.model.SavingsPlansPurchaseRecommendationSummary Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.costexplorer.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Summary metrics for your Savings Plans Purchase Recommendations.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SavingsPlansPurchaseRecommendationSummary implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ESTIMATED_ROI_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SavingsPlansPurchaseRecommendationSummary::estimatedROI)).setter(setter(Builder::estimatedROI))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EstimatedROI").build()).build();
private static final SdkField CURRENCY_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SavingsPlansPurchaseRecommendationSummary::currencyCode)).setter(setter(Builder::currencyCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CurrencyCode").build()).build();
private static final SdkField ESTIMATED_TOTAL_COST_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SavingsPlansPurchaseRecommendationSummary::estimatedTotalCost))
.setter(setter(Builder::estimatedTotalCost))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EstimatedTotalCost").build())
.build();
private static final SdkField CURRENT_ON_DEMAND_SPEND_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SavingsPlansPurchaseRecommendationSummary::currentOnDemandSpend))
.setter(setter(Builder::currentOnDemandSpend))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CurrentOnDemandSpend").build())
.build();
private static final SdkField ESTIMATED_SAVINGS_AMOUNT_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SavingsPlansPurchaseRecommendationSummary::estimatedSavingsAmount))
.setter(setter(Builder::estimatedSavingsAmount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EstimatedSavingsAmount").build())
.build();
private static final SdkField TOTAL_RECOMMENDATION_COUNT_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SavingsPlansPurchaseRecommendationSummary::totalRecommendationCount))
.setter(setter(Builder::totalRecommendationCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TotalRecommendationCount").build())
.build();
private static final SdkField DAILY_COMMITMENT_TO_PURCHASE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(SavingsPlansPurchaseRecommendationSummary::dailyCommitmentToPurchase))
.setter(setter(Builder::dailyCommitmentToPurchase))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DailyCommitmentToPurchase").build())
.build();
private static final SdkField HOURLY_COMMITMENT_TO_PURCHASE_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(SavingsPlansPurchaseRecommendationSummary::hourlyCommitmentToPurchase))
.setter(setter(Builder::hourlyCommitmentToPurchase))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HourlyCommitmentToPurchase").build())
.build();
private static final SdkField ESTIMATED_SAVINGS_PERCENTAGE_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(SavingsPlansPurchaseRecommendationSummary::estimatedSavingsPercentage))
.setter(setter(Builder::estimatedSavingsPercentage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EstimatedSavingsPercentage").build())
.build();
private static final SdkField ESTIMATED_MONTHLY_SAVINGS_AMOUNT_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(SavingsPlansPurchaseRecommendationSummary::estimatedMonthlySavingsAmount))
.setter(setter(Builder::estimatedMonthlySavingsAmount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EstimatedMonthlySavingsAmount")
.build()).build();
private static final SdkField ESTIMATED_ON_DEMAND_COST_WITH_CURRENT_COMMITMENT_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(SavingsPlansPurchaseRecommendationSummary::estimatedOnDemandCostWithCurrentCommitment))
.setter(setter(Builder::estimatedOnDemandCostWithCurrentCommitment))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("EstimatedOnDemandCostWithCurrentCommitment").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ESTIMATED_ROI_FIELD,
CURRENCY_CODE_FIELD, ESTIMATED_TOTAL_COST_FIELD, CURRENT_ON_DEMAND_SPEND_FIELD, ESTIMATED_SAVINGS_AMOUNT_FIELD,
TOTAL_RECOMMENDATION_COUNT_FIELD, DAILY_COMMITMENT_TO_PURCHASE_FIELD, HOURLY_COMMITMENT_TO_PURCHASE_FIELD,
ESTIMATED_SAVINGS_PERCENTAGE_FIELD, ESTIMATED_MONTHLY_SAVINGS_AMOUNT_FIELD,
ESTIMATED_ON_DEMAND_COST_WITH_CURRENT_COMMITMENT_FIELD));
private static final long serialVersionUID = 1L;
private final String estimatedROI;
private final String currencyCode;
private final String estimatedTotalCost;
private final String currentOnDemandSpend;
private final String estimatedSavingsAmount;
private final String totalRecommendationCount;
private final String dailyCommitmentToPurchase;
private final String hourlyCommitmentToPurchase;
private final String estimatedSavingsPercentage;
private final String estimatedMonthlySavingsAmount;
private final String estimatedOnDemandCostWithCurrentCommitment;
private SavingsPlansPurchaseRecommendationSummary(BuilderImpl builder) {
this.estimatedROI = builder.estimatedROI;
this.currencyCode = builder.currencyCode;
this.estimatedTotalCost = builder.estimatedTotalCost;
this.currentOnDemandSpend = builder.currentOnDemandSpend;
this.estimatedSavingsAmount = builder.estimatedSavingsAmount;
this.totalRecommendationCount = builder.totalRecommendationCount;
this.dailyCommitmentToPurchase = builder.dailyCommitmentToPurchase;
this.hourlyCommitmentToPurchase = builder.hourlyCommitmentToPurchase;
this.estimatedSavingsPercentage = builder.estimatedSavingsPercentage;
this.estimatedMonthlySavingsAmount = builder.estimatedMonthlySavingsAmount;
this.estimatedOnDemandCostWithCurrentCommitment = builder.estimatedOnDemandCostWithCurrentCommitment;
}
/**
*
* The estimated return on investment based on the recommended Savings Plans and estimated savings.
*
*
* @return The estimated return on investment based on the recommended Savings Plans and estimated savings.
*/
public String estimatedROI() {
return estimatedROI;
}
/**
*
* The currency code Amazon Web Services used to generate the recommendations and present potential savings.
*
*
* @return The currency code Amazon Web Services used to generate the recommendations and present potential savings.
*/
public String currencyCode() {
return currencyCode;
}
/**
*
* The estimated total cost of the usage after purchasing the recommended Savings Plans. This is a sum of the cost
* of Savings Plans during this term, and the remaining On-Demand usage.
*
*
* @return The estimated total cost of the usage after purchasing the recommended Savings Plans. This is a sum of
* the cost of Savings Plans during this term, and the remaining On-Demand usage.
*/
public String estimatedTotalCost() {
return estimatedTotalCost;
}
/**
*
* The current total on demand spend of the applicable usage types over the lookback period.
*
*
* @return The current total on demand spend of the applicable usage types over the lookback period.
*/
public String currentOnDemandSpend() {
return currentOnDemandSpend;
}
/**
*
* The estimated total savings over the lookback period, based on the purchase of the recommended Savings Plans.
*
*
* @return The estimated total savings over the lookback period, based on the purchase of the recommended Savings
* Plans.
*/
public String estimatedSavingsAmount() {
return estimatedSavingsAmount;
}
/**
*
* The aggregate number of Savings Plans recommendations that exist for your account.
*
*
* @return The aggregate number of Savings Plans recommendations that exist for your account.
*/
public String totalRecommendationCount() {
return totalRecommendationCount;
}
/**
*
* The recommended Savings Plans cost on a daily (24 hourly) basis.
*
*
* @return The recommended Savings Plans cost on a daily (24 hourly) basis.
*/
public String dailyCommitmentToPurchase() {
return dailyCommitmentToPurchase;
}
/**
*
* The recommended hourly commitment based on the recommendation parameters.
*
*
* @return The recommended hourly commitment based on the recommendation parameters.
*/
public String hourlyCommitmentToPurchase() {
return hourlyCommitmentToPurchase;
}
/**
*
* The estimated savings relative to the total cost of On-Demand usage, over the lookback period. This is calculated
* as estimatedSavingsAmount
/ CurrentOnDemandSpend
*100.
*
*
* @return The estimated savings relative to the total cost of On-Demand usage, over the lookback period. This is
* calculated as estimatedSavingsAmount
/ CurrentOnDemandSpend
*100.
*/
public String estimatedSavingsPercentage() {
return estimatedSavingsPercentage;
}
/**
*
* The estimated monthly savings amount, based on the recommended Savings Plans purchase.
*
*
* @return The estimated monthly savings amount, based on the recommended Savings Plans purchase.
*/
public String estimatedMonthlySavingsAmount() {
return estimatedMonthlySavingsAmount;
}
/**
*
* The estimated On-Demand costs you would expect with no additional commitment, based on your usage of the selected
* time period and the Savings Plans you own.
*
*
* @return The estimated On-Demand costs you would expect with no additional commitment, based on your usage of the
* selected time period and the Savings Plans you own.
*/
public String estimatedOnDemandCostWithCurrentCommitment() {
return estimatedOnDemandCostWithCurrentCommitment;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(estimatedROI());
hashCode = 31 * hashCode + Objects.hashCode(currencyCode());
hashCode = 31 * hashCode + Objects.hashCode(estimatedTotalCost());
hashCode = 31 * hashCode + Objects.hashCode(currentOnDemandSpend());
hashCode = 31 * hashCode + Objects.hashCode(estimatedSavingsAmount());
hashCode = 31 * hashCode + Objects.hashCode(totalRecommendationCount());
hashCode = 31 * hashCode + Objects.hashCode(dailyCommitmentToPurchase());
hashCode = 31 * hashCode + Objects.hashCode(hourlyCommitmentToPurchase());
hashCode = 31 * hashCode + Objects.hashCode(estimatedSavingsPercentage());
hashCode = 31 * hashCode + Objects.hashCode(estimatedMonthlySavingsAmount());
hashCode = 31 * hashCode + Objects.hashCode(estimatedOnDemandCostWithCurrentCommitment());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SavingsPlansPurchaseRecommendationSummary)) {
return false;
}
SavingsPlansPurchaseRecommendationSummary other = (SavingsPlansPurchaseRecommendationSummary) obj;
return Objects.equals(estimatedROI(), other.estimatedROI())
&& Objects.equals(currencyCode(), other.currencyCode())
&& Objects.equals(estimatedTotalCost(), other.estimatedTotalCost())
&& Objects.equals(currentOnDemandSpend(), other.currentOnDemandSpend())
&& Objects.equals(estimatedSavingsAmount(), other.estimatedSavingsAmount())
&& Objects.equals(totalRecommendationCount(), other.totalRecommendationCount())
&& Objects.equals(dailyCommitmentToPurchase(), other.dailyCommitmentToPurchase())
&& Objects.equals(hourlyCommitmentToPurchase(), other.hourlyCommitmentToPurchase())
&& Objects.equals(estimatedSavingsPercentage(), other.estimatedSavingsPercentage())
&& Objects.equals(estimatedMonthlySavingsAmount(), other.estimatedMonthlySavingsAmount())
&& Objects.equals(estimatedOnDemandCostWithCurrentCommitment(),
other.estimatedOnDemandCostWithCurrentCommitment());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("SavingsPlansPurchaseRecommendationSummary").add("EstimatedROI", estimatedROI())
.add("CurrencyCode", currencyCode()).add("EstimatedTotalCost", estimatedTotalCost())
.add("CurrentOnDemandSpend", currentOnDemandSpend()).add("EstimatedSavingsAmount", estimatedSavingsAmount())
.add("TotalRecommendationCount", totalRecommendationCount())
.add("DailyCommitmentToPurchase", dailyCommitmentToPurchase())
.add("HourlyCommitmentToPurchase", hourlyCommitmentToPurchase())
.add("EstimatedSavingsPercentage", estimatedSavingsPercentage())
.add("EstimatedMonthlySavingsAmount", estimatedMonthlySavingsAmount())
.add("EstimatedOnDemandCostWithCurrentCommitment", estimatedOnDemandCostWithCurrentCommitment()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EstimatedROI":
return Optional.ofNullable(clazz.cast(estimatedROI()));
case "CurrencyCode":
return Optional.ofNullable(clazz.cast(currencyCode()));
case "EstimatedTotalCost":
return Optional.ofNullable(clazz.cast(estimatedTotalCost()));
case "CurrentOnDemandSpend":
return Optional.ofNullable(clazz.cast(currentOnDemandSpend()));
case "EstimatedSavingsAmount":
return Optional.ofNullable(clazz.cast(estimatedSavingsAmount()));
case "TotalRecommendationCount":
return Optional.ofNullable(clazz.cast(totalRecommendationCount()));
case "DailyCommitmentToPurchase":
return Optional.ofNullable(clazz.cast(dailyCommitmentToPurchase()));
case "HourlyCommitmentToPurchase":
return Optional.ofNullable(clazz.cast(hourlyCommitmentToPurchase()));
case "EstimatedSavingsPercentage":
return Optional.ofNullable(clazz.cast(estimatedSavingsPercentage()));
case "EstimatedMonthlySavingsAmount":
return Optional.ofNullable(clazz.cast(estimatedMonthlySavingsAmount()));
case "EstimatedOnDemandCostWithCurrentCommitment":
return Optional.ofNullable(clazz.cast(estimatedOnDemandCostWithCurrentCommitment()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function