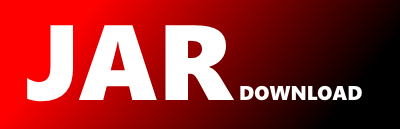
software.amazon.awssdk.services.costexplorer.model.ReservationPurchaseRecommendationDetail Maven / Gradle / Ivy
Show all versions of costexplorer Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.costexplorer.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Details about your recommended reservation purchase.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ReservationPurchaseRecommendationDetail implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ACCOUNT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AccountId").getter(getter(ReservationPurchaseRecommendationDetail::accountId))
.setter(setter(Builder::accountId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AccountId").build()).build();
private static final SdkField INSTANCE_DETAILS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("InstanceDetails")
.getter(getter(ReservationPurchaseRecommendationDetail::instanceDetails)).setter(setter(Builder::instanceDetails))
.constructor(InstanceDetails::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceDetails").build()).build();
private static final SdkField RECOMMENDED_NUMBER_OF_INSTANCES_TO_PURCHASE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("RecommendedNumberOfInstancesToPurchase")
.getter(getter(ReservationPurchaseRecommendationDetail::recommendedNumberOfInstancesToPurchase))
.setter(setter(Builder::recommendedNumberOfInstancesToPurchase))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("RecommendedNumberOfInstancesToPurchase").build()).build();
private static final SdkField RECOMMENDED_NORMALIZED_UNITS_TO_PURCHASE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("RecommendedNormalizedUnitsToPurchase")
.getter(getter(ReservationPurchaseRecommendationDetail::recommendedNormalizedUnitsToPurchase))
.setter(setter(Builder::recommendedNormalizedUnitsToPurchase))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("RecommendedNormalizedUnitsToPurchase").build()).build();
private static final SdkField MINIMUM_NUMBER_OF_INSTANCES_USED_PER_HOUR_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("MinimumNumberOfInstancesUsedPerHour")
.getter(getter(ReservationPurchaseRecommendationDetail::minimumNumberOfInstancesUsedPerHour))
.setter(setter(Builder::minimumNumberOfInstancesUsedPerHour))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("MinimumNumberOfInstancesUsedPerHour").build()).build();
private static final SdkField MINIMUM_NORMALIZED_UNITS_USED_PER_HOUR_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("MinimumNormalizedUnitsUsedPerHour")
.getter(getter(ReservationPurchaseRecommendationDetail::minimumNormalizedUnitsUsedPerHour))
.setter(setter(Builder::minimumNormalizedUnitsUsedPerHour))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MinimumNormalizedUnitsUsedPerHour")
.build()).build();
private static final SdkField MAXIMUM_NUMBER_OF_INSTANCES_USED_PER_HOUR_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("MaximumNumberOfInstancesUsedPerHour")
.getter(getter(ReservationPurchaseRecommendationDetail::maximumNumberOfInstancesUsedPerHour))
.setter(setter(Builder::maximumNumberOfInstancesUsedPerHour))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("MaximumNumberOfInstancesUsedPerHour").build()).build();
private static final SdkField MAXIMUM_NORMALIZED_UNITS_USED_PER_HOUR_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("MaximumNormalizedUnitsUsedPerHour")
.getter(getter(ReservationPurchaseRecommendationDetail::maximumNormalizedUnitsUsedPerHour))
.setter(setter(Builder::maximumNormalizedUnitsUsedPerHour))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaximumNormalizedUnitsUsedPerHour")
.build()).build();
private static final SdkField AVERAGE_NUMBER_OF_INSTANCES_USED_PER_HOUR_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("AverageNumberOfInstancesUsedPerHour")
.getter(getter(ReservationPurchaseRecommendationDetail::averageNumberOfInstancesUsedPerHour))
.setter(setter(Builder::averageNumberOfInstancesUsedPerHour))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("AverageNumberOfInstancesUsedPerHour").build()).build();
private static final SdkField AVERAGE_NORMALIZED_UNITS_USED_PER_HOUR_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("AverageNormalizedUnitsUsedPerHour")
.getter(getter(ReservationPurchaseRecommendationDetail::averageNormalizedUnitsUsedPerHour))
.setter(setter(Builder::averageNormalizedUnitsUsedPerHour))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AverageNormalizedUnitsUsedPerHour")
.build()).build();
private static final SdkField AVERAGE_UTILIZATION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AverageUtilization").getter(getter(ReservationPurchaseRecommendationDetail::averageUtilization))
.setter(setter(Builder::averageUtilization))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AverageUtilization").build())
.build();
private static final SdkField ESTIMATED_BREAK_EVEN_IN_MONTHS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("EstimatedBreakEvenInMonths")
.getter(getter(ReservationPurchaseRecommendationDetail::estimatedBreakEvenInMonths))
.setter(setter(Builder::estimatedBreakEvenInMonths))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EstimatedBreakEvenInMonths").build())
.build();
private static final SdkField CURRENCY_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CurrencyCode").getter(getter(ReservationPurchaseRecommendationDetail::currencyCode))
.setter(setter(Builder::currencyCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CurrencyCode").build()).build();
private static final SdkField ESTIMATED_MONTHLY_SAVINGS_AMOUNT_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("EstimatedMonthlySavingsAmount")
.getter(getter(ReservationPurchaseRecommendationDetail::estimatedMonthlySavingsAmount))
.setter(setter(Builder::estimatedMonthlySavingsAmount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EstimatedMonthlySavingsAmount")
.build()).build();
private static final SdkField ESTIMATED_MONTHLY_SAVINGS_PERCENTAGE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("EstimatedMonthlySavingsPercentage")
.getter(getter(ReservationPurchaseRecommendationDetail::estimatedMonthlySavingsPercentage))
.setter(setter(Builder::estimatedMonthlySavingsPercentage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EstimatedMonthlySavingsPercentage")
.build()).build();
private static final SdkField ESTIMATED_MONTHLY_ON_DEMAND_COST_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("EstimatedMonthlyOnDemandCost")
.getter(getter(ReservationPurchaseRecommendationDetail::estimatedMonthlyOnDemandCost))
.setter(setter(Builder::estimatedMonthlyOnDemandCost))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EstimatedMonthlyOnDemandCost")
.build()).build();
private static final SdkField ESTIMATED_RESERVATION_COST_FOR_LOOKBACK_PERIOD_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("EstimatedReservationCostForLookbackPeriod")
.getter(getter(ReservationPurchaseRecommendationDetail::estimatedReservationCostForLookbackPeriod))
.setter(setter(Builder::estimatedReservationCostForLookbackPeriod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("EstimatedReservationCostForLookbackPeriod").build()).build();
private static final SdkField UPFRONT_COST_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UpfrontCost").getter(getter(ReservationPurchaseRecommendationDetail::upfrontCost))
.setter(setter(Builder::upfrontCost))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpfrontCost").build()).build();
private static final SdkField RECURRING_STANDARD_MONTHLY_COST_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("RecurringStandardMonthlyCost")
.getter(getter(ReservationPurchaseRecommendationDetail::recurringStandardMonthlyCost))
.setter(setter(Builder::recurringStandardMonthlyCost))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RecurringStandardMonthlyCost")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ACCOUNT_ID_FIELD,
INSTANCE_DETAILS_FIELD, RECOMMENDED_NUMBER_OF_INSTANCES_TO_PURCHASE_FIELD,
RECOMMENDED_NORMALIZED_UNITS_TO_PURCHASE_FIELD, MINIMUM_NUMBER_OF_INSTANCES_USED_PER_HOUR_FIELD,
MINIMUM_NORMALIZED_UNITS_USED_PER_HOUR_FIELD, MAXIMUM_NUMBER_OF_INSTANCES_USED_PER_HOUR_FIELD,
MAXIMUM_NORMALIZED_UNITS_USED_PER_HOUR_FIELD, AVERAGE_NUMBER_OF_INSTANCES_USED_PER_HOUR_FIELD,
AVERAGE_NORMALIZED_UNITS_USED_PER_HOUR_FIELD, AVERAGE_UTILIZATION_FIELD, ESTIMATED_BREAK_EVEN_IN_MONTHS_FIELD,
CURRENCY_CODE_FIELD, ESTIMATED_MONTHLY_SAVINGS_AMOUNT_FIELD, ESTIMATED_MONTHLY_SAVINGS_PERCENTAGE_FIELD,
ESTIMATED_MONTHLY_ON_DEMAND_COST_FIELD, ESTIMATED_RESERVATION_COST_FOR_LOOKBACK_PERIOD_FIELD, UPFRONT_COST_FIELD,
RECURRING_STANDARD_MONTHLY_COST_FIELD));
private static final long serialVersionUID = 1L;
private final String accountId;
private final InstanceDetails instanceDetails;
private final String recommendedNumberOfInstancesToPurchase;
private final String recommendedNormalizedUnitsToPurchase;
private final String minimumNumberOfInstancesUsedPerHour;
private final String minimumNormalizedUnitsUsedPerHour;
private final String maximumNumberOfInstancesUsedPerHour;
private final String maximumNormalizedUnitsUsedPerHour;
private final String averageNumberOfInstancesUsedPerHour;
private final String averageNormalizedUnitsUsedPerHour;
private final String averageUtilization;
private final String estimatedBreakEvenInMonths;
private final String currencyCode;
private final String estimatedMonthlySavingsAmount;
private final String estimatedMonthlySavingsPercentage;
private final String estimatedMonthlyOnDemandCost;
private final String estimatedReservationCostForLookbackPeriod;
private final String upfrontCost;
private final String recurringStandardMonthlyCost;
private ReservationPurchaseRecommendationDetail(BuilderImpl builder) {
this.accountId = builder.accountId;
this.instanceDetails = builder.instanceDetails;
this.recommendedNumberOfInstancesToPurchase = builder.recommendedNumberOfInstancesToPurchase;
this.recommendedNormalizedUnitsToPurchase = builder.recommendedNormalizedUnitsToPurchase;
this.minimumNumberOfInstancesUsedPerHour = builder.minimumNumberOfInstancesUsedPerHour;
this.minimumNormalizedUnitsUsedPerHour = builder.minimumNormalizedUnitsUsedPerHour;
this.maximumNumberOfInstancesUsedPerHour = builder.maximumNumberOfInstancesUsedPerHour;
this.maximumNormalizedUnitsUsedPerHour = builder.maximumNormalizedUnitsUsedPerHour;
this.averageNumberOfInstancesUsedPerHour = builder.averageNumberOfInstancesUsedPerHour;
this.averageNormalizedUnitsUsedPerHour = builder.averageNormalizedUnitsUsedPerHour;
this.averageUtilization = builder.averageUtilization;
this.estimatedBreakEvenInMonths = builder.estimatedBreakEvenInMonths;
this.currencyCode = builder.currencyCode;
this.estimatedMonthlySavingsAmount = builder.estimatedMonthlySavingsAmount;
this.estimatedMonthlySavingsPercentage = builder.estimatedMonthlySavingsPercentage;
this.estimatedMonthlyOnDemandCost = builder.estimatedMonthlyOnDemandCost;
this.estimatedReservationCostForLookbackPeriod = builder.estimatedReservationCostForLookbackPeriod;
this.upfrontCost = builder.upfrontCost;
this.recurringStandardMonthlyCost = builder.recurringStandardMonthlyCost;
}
/**
*
* The account that this RI recommendation is for.
*
*
* @return The account that this RI recommendation is for.
*/
public String accountId() {
return accountId;
}
/**
*
* Details about the instances that AWS recommends that you purchase.
*
*
* @return Details about the instances that AWS recommends that you purchase.
*/
public InstanceDetails instanceDetails() {
return instanceDetails;
}
/**
*
* The number of instances that AWS recommends that you purchase.
*
*
* @return The number of instances that AWS recommends that you purchase.
*/
public String recommendedNumberOfInstancesToPurchase() {
return recommendedNumberOfInstancesToPurchase;
}
/**
*
* The number of normalized units that AWS recommends that you purchase.
*
*
* @return The number of normalized units that AWS recommends that you purchase.
*/
public String recommendedNormalizedUnitsToPurchase() {
return recommendedNormalizedUnitsToPurchase;
}
/**
*
* The minimum number of instances that you used in an hour during the historical period. AWS uses this to calculate
* your recommended reservation purchases.
*
*
* @return The minimum number of instances that you used in an hour during the historical period. AWS uses this to
* calculate your recommended reservation purchases.
*/
public String minimumNumberOfInstancesUsedPerHour() {
return minimumNumberOfInstancesUsedPerHour;
}
/**
*
* The minimum number of normalized units that you used in an hour during the historical period. AWS uses this to
* calculate your recommended reservation purchases.
*
*
* @return The minimum number of normalized units that you used in an hour during the historical period. AWS uses
* this to calculate your recommended reservation purchases.
*/
public String minimumNormalizedUnitsUsedPerHour() {
return minimumNormalizedUnitsUsedPerHour;
}
/**
*
* The maximum number of instances that you used in an hour during the historical period. AWS uses this to calculate
* your recommended reservation purchases.
*
*
* @return The maximum number of instances that you used in an hour during the historical period. AWS uses this to
* calculate your recommended reservation purchases.
*/
public String maximumNumberOfInstancesUsedPerHour() {
return maximumNumberOfInstancesUsedPerHour;
}
/**
*
* The maximum number of normalized units that you used in an hour during the historical period. AWS uses this to
* calculate your recommended reservation purchases.
*
*
* @return The maximum number of normalized units that you used in an hour during the historical period. AWS uses
* this to calculate your recommended reservation purchases.
*/
public String maximumNormalizedUnitsUsedPerHour() {
return maximumNormalizedUnitsUsedPerHour;
}
/**
*
* The average number of instances that you used in an hour during the historical period. AWS uses this to calculate
* your recommended reservation purchases.
*
*
* @return The average number of instances that you used in an hour during the historical period. AWS uses this to
* calculate your recommended reservation purchases.
*/
public String averageNumberOfInstancesUsedPerHour() {
return averageNumberOfInstancesUsedPerHour;
}
/**
*
* The average number of normalized units that you used in an hour during the historical period. AWS uses this to
* calculate your recommended reservation purchases.
*
*
* @return The average number of normalized units that you used in an hour during the historical period. AWS uses
* this to calculate your recommended reservation purchases.
*/
public String averageNormalizedUnitsUsedPerHour() {
return averageNormalizedUnitsUsedPerHour;
}
/**
*
* The average utilization of your instances. AWS uses this to calculate your recommended reservation purchases.
*
*
* @return The average utilization of your instances. AWS uses this to calculate your recommended reservation
* purchases.
*/
public String averageUtilization() {
return averageUtilization;
}
/**
*
* How long AWS estimates that it takes for this instance to start saving you money, in months.
*
*
* @return How long AWS estimates that it takes for this instance to start saving you money, in months.
*/
public String estimatedBreakEvenInMonths() {
return estimatedBreakEvenInMonths;
}
/**
*
* The currency code that AWS used to calculate the costs for this instance.
*
*
* @return The currency code that AWS used to calculate the costs for this instance.
*/
public String currencyCode() {
return currencyCode;
}
/**
*
* How much AWS estimates that this specific recommendation could save you in a month.
*
*
* @return How much AWS estimates that this specific recommendation could save you in a month.
*/
public String estimatedMonthlySavingsAmount() {
return estimatedMonthlySavingsAmount;
}
/**
*
* How much AWS estimates that this specific recommendation could save you in a month, as a percentage of your
* overall costs.
*
*
* @return How much AWS estimates that this specific recommendation could save you in a month, as a percentage of
* your overall costs.
*/
public String estimatedMonthlySavingsPercentage() {
return estimatedMonthlySavingsPercentage;
}
/**
*
* How much AWS estimates that you spend on On-Demand Instances in a month.
*
*
* @return How much AWS estimates that you spend on On-Demand Instances in a month.
*/
public String estimatedMonthlyOnDemandCost() {
return estimatedMonthlyOnDemandCost;
}
/**
*
* How much AWS estimates that you would have spent for all usage during the specified historical period if you had
* a reservation.
*
*
* @return How much AWS estimates that you would have spent for all usage during the specified historical period if
* you had a reservation.
*/
public String estimatedReservationCostForLookbackPeriod() {
return estimatedReservationCostForLookbackPeriod;
}
/**
*
* How much purchasing this instance costs you upfront.
*
*
* @return How much purchasing this instance costs you upfront.
*/
public String upfrontCost() {
return upfrontCost;
}
/**
*
* How much purchasing this instance costs you on a monthly basis.
*
*
* @return How much purchasing this instance costs you on a monthly basis.
*/
public String recurringStandardMonthlyCost() {
return recurringStandardMonthlyCost;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(accountId());
hashCode = 31 * hashCode + Objects.hashCode(instanceDetails());
hashCode = 31 * hashCode + Objects.hashCode(recommendedNumberOfInstancesToPurchase());
hashCode = 31 * hashCode + Objects.hashCode(recommendedNormalizedUnitsToPurchase());
hashCode = 31 * hashCode + Objects.hashCode(minimumNumberOfInstancesUsedPerHour());
hashCode = 31 * hashCode + Objects.hashCode(minimumNormalizedUnitsUsedPerHour());
hashCode = 31 * hashCode + Objects.hashCode(maximumNumberOfInstancesUsedPerHour());
hashCode = 31 * hashCode + Objects.hashCode(maximumNormalizedUnitsUsedPerHour());
hashCode = 31 * hashCode + Objects.hashCode(averageNumberOfInstancesUsedPerHour());
hashCode = 31 * hashCode + Objects.hashCode(averageNormalizedUnitsUsedPerHour());
hashCode = 31 * hashCode + Objects.hashCode(averageUtilization());
hashCode = 31 * hashCode + Objects.hashCode(estimatedBreakEvenInMonths());
hashCode = 31 * hashCode + Objects.hashCode(currencyCode());
hashCode = 31 * hashCode + Objects.hashCode(estimatedMonthlySavingsAmount());
hashCode = 31 * hashCode + Objects.hashCode(estimatedMonthlySavingsPercentage());
hashCode = 31 * hashCode + Objects.hashCode(estimatedMonthlyOnDemandCost());
hashCode = 31 * hashCode + Objects.hashCode(estimatedReservationCostForLookbackPeriod());
hashCode = 31 * hashCode + Objects.hashCode(upfrontCost());
hashCode = 31 * hashCode + Objects.hashCode(recurringStandardMonthlyCost());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ReservationPurchaseRecommendationDetail)) {
return false;
}
ReservationPurchaseRecommendationDetail other = (ReservationPurchaseRecommendationDetail) obj;
return Objects.equals(accountId(), other.accountId()) && Objects.equals(instanceDetails(), other.instanceDetails())
&& Objects.equals(recommendedNumberOfInstancesToPurchase(), other.recommendedNumberOfInstancesToPurchase())
&& Objects.equals(recommendedNormalizedUnitsToPurchase(), other.recommendedNormalizedUnitsToPurchase())
&& Objects.equals(minimumNumberOfInstancesUsedPerHour(), other.minimumNumberOfInstancesUsedPerHour())
&& Objects.equals(minimumNormalizedUnitsUsedPerHour(), other.minimumNormalizedUnitsUsedPerHour())
&& Objects.equals(maximumNumberOfInstancesUsedPerHour(), other.maximumNumberOfInstancesUsedPerHour())
&& Objects.equals(maximumNormalizedUnitsUsedPerHour(), other.maximumNormalizedUnitsUsedPerHour())
&& Objects.equals(averageNumberOfInstancesUsedPerHour(), other.averageNumberOfInstancesUsedPerHour())
&& Objects.equals(averageNormalizedUnitsUsedPerHour(), other.averageNormalizedUnitsUsedPerHour())
&& Objects.equals(averageUtilization(), other.averageUtilization())
&& Objects.equals(estimatedBreakEvenInMonths(), other.estimatedBreakEvenInMonths())
&& Objects.equals(currencyCode(), other.currencyCode())
&& Objects.equals(estimatedMonthlySavingsAmount(), other.estimatedMonthlySavingsAmount())
&& Objects.equals(estimatedMonthlySavingsPercentage(), other.estimatedMonthlySavingsPercentage())
&& Objects.equals(estimatedMonthlyOnDemandCost(), other.estimatedMonthlyOnDemandCost())
&& Objects.equals(estimatedReservationCostForLookbackPeriod(), other.estimatedReservationCostForLookbackPeriod())
&& Objects.equals(upfrontCost(), other.upfrontCost())
&& Objects.equals(recurringStandardMonthlyCost(), other.recurringStandardMonthlyCost());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("ReservationPurchaseRecommendationDetail").add("AccountId", accountId())
.add("InstanceDetails", instanceDetails())
.add("RecommendedNumberOfInstancesToPurchase", recommendedNumberOfInstancesToPurchase())
.add("RecommendedNormalizedUnitsToPurchase", recommendedNormalizedUnitsToPurchase())
.add("MinimumNumberOfInstancesUsedPerHour", minimumNumberOfInstancesUsedPerHour())
.add("MinimumNormalizedUnitsUsedPerHour", minimumNormalizedUnitsUsedPerHour())
.add("MaximumNumberOfInstancesUsedPerHour", maximumNumberOfInstancesUsedPerHour())
.add("MaximumNormalizedUnitsUsedPerHour", maximumNormalizedUnitsUsedPerHour())
.add("AverageNumberOfInstancesUsedPerHour", averageNumberOfInstancesUsedPerHour())
.add("AverageNormalizedUnitsUsedPerHour", averageNormalizedUnitsUsedPerHour())
.add("AverageUtilization", averageUtilization()).add("EstimatedBreakEvenInMonths", estimatedBreakEvenInMonths())
.add("CurrencyCode", currencyCode()).add("EstimatedMonthlySavingsAmount", estimatedMonthlySavingsAmount())
.add("EstimatedMonthlySavingsPercentage", estimatedMonthlySavingsPercentage())
.add("EstimatedMonthlyOnDemandCost", estimatedMonthlyOnDemandCost())
.add("EstimatedReservationCostForLookbackPeriod", estimatedReservationCostForLookbackPeriod())
.add("UpfrontCost", upfrontCost()).add("RecurringStandardMonthlyCost", recurringStandardMonthlyCost()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AccountId":
return Optional.ofNullable(clazz.cast(accountId()));
case "InstanceDetails":
return Optional.ofNullable(clazz.cast(instanceDetails()));
case "RecommendedNumberOfInstancesToPurchase":
return Optional.ofNullable(clazz.cast(recommendedNumberOfInstancesToPurchase()));
case "RecommendedNormalizedUnitsToPurchase":
return Optional.ofNullable(clazz.cast(recommendedNormalizedUnitsToPurchase()));
case "MinimumNumberOfInstancesUsedPerHour":
return Optional.ofNullable(clazz.cast(minimumNumberOfInstancesUsedPerHour()));
case "MinimumNormalizedUnitsUsedPerHour":
return Optional.ofNullable(clazz.cast(minimumNormalizedUnitsUsedPerHour()));
case "MaximumNumberOfInstancesUsedPerHour":
return Optional.ofNullable(clazz.cast(maximumNumberOfInstancesUsedPerHour()));
case "MaximumNormalizedUnitsUsedPerHour":
return Optional.ofNullable(clazz.cast(maximumNormalizedUnitsUsedPerHour()));
case "AverageNumberOfInstancesUsedPerHour":
return Optional.ofNullable(clazz.cast(averageNumberOfInstancesUsedPerHour()));
case "AverageNormalizedUnitsUsedPerHour":
return Optional.ofNullable(clazz.cast(averageNormalizedUnitsUsedPerHour()));
case "AverageUtilization":
return Optional.ofNullable(clazz.cast(averageUtilization()));
case "EstimatedBreakEvenInMonths":
return Optional.ofNullable(clazz.cast(estimatedBreakEvenInMonths()));
case "CurrencyCode":
return Optional.ofNullable(clazz.cast(currencyCode()));
case "EstimatedMonthlySavingsAmount":
return Optional.ofNullable(clazz.cast(estimatedMonthlySavingsAmount()));
case "EstimatedMonthlySavingsPercentage":
return Optional.ofNullable(clazz.cast(estimatedMonthlySavingsPercentage()));
case "EstimatedMonthlyOnDemandCost":
return Optional.ofNullable(clazz.cast(estimatedMonthlyOnDemandCost()));
case "EstimatedReservationCostForLookbackPeriod":
return Optional.ofNullable(clazz.cast(estimatedReservationCostForLookbackPeriod()));
case "UpfrontCost":
return Optional.ofNullable(clazz.cast(upfrontCost()));
case "RecurringStandardMonthlyCost":
return Optional.ofNullable(clazz.cast(recurringStandardMonthlyCost()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function