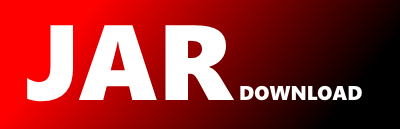
software.amazon.awssdk.services.costexplorer.model.Coverage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of costexplorer Show documentation
Show all versions of costexplorer Show documentation
The AWS Java SDK for AWS Cost Explorer module holds the client classes that are used for communicating
with AWS Cost Explorer Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.costexplorer.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The amount of instance usage that a reservation covered.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Coverage implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField COVERAGE_HOURS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("CoverageHours")
.getter(getter(Coverage::coverageHours)).setter(setter(Builder::coverageHours)).constructor(CoverageHours::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CoverageHours").build()).build();
private static final SdkField COVERAGE_NORMALIZED_UNITS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("CoverageNormalizedUnits")
.getter(getter(Coverage::coverageNormalizedUnits)).setter(setter(Builder::coverageNormalizedUnits))
.constructor(CoverageNormalizedUnits::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CoverageNormalizedUnits").build())
.build();
private static final SdkField COVERAGE_COST_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("CoverageCost").getter(getter(Coverage::coverageCost)).setter(setter(Builder::coverageCost))
.constructor(CoverageCost::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CoverageCost").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(COVERAGE_HOURS_FIELD,
COVERAGE_NORMALIZED_UNITS_FIELD, COVERAGE_COST_FIELD));
private static final long serialVersionUID = 1L;
private final CoverageHours coverageHours;
private final CoverageNormalizedUnits coverageNormalizedUnits;
private final CoverageCost coverageCost;
private Coverage(BuilderImpl builder) {
this.coverageHours = builder.coverageHours;
this.coverageNormalizedUnits = builder.coverageNormalizedUnits;
this.coverageCost = builder.coverageCost;
}
/**
*
* The amount of instance usage that the reservation covered, in hours.
*
*
* @return The amount of instance usage that the reservation covered, in hours.
*/
public CoverageHours coverageHours() {
return coverageHours;
}
/**
*
* The amount of instance usage that the reservation covered, in normalized units.
*
*
* @return The amount of instance usage that the reservation covered, in normalized units.
*/
public CoverageNormalizedUnits coverageNormalizedUnits() {
return coverageNormalizedUnits;
}
/**
*
* The amount of cost that the reservation covered.
*
*
* @return The amount of cost that the reservation covered.
*/
public CoverageCost coverageCost() {
return coverageCost;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(coverageHours());
hashCode = 31 * hashCode + Objects.hashCode(coverageNormalizedUnits());
hashCode = 31 * hashCode + Objects.hashCode(coverageCost());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Coverage)) {
return false;
}
Coverage other = (Coverage) obj;
return Objects.equals(coverageHours(), other.coverageHours())
&& Objects.equals(coverageNormalizedUnits(), other.coverageNormalizedUnits())
&& Objects.equals(coverageCost(), other.coverageCost());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("Coverage").add("CoverageHours", coverageHours())
.add("CoverageNormalizedUnits", coverageNormalizedUnits()).add("CoverageCost", coverageCost()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CoverageHours":
return Optional.ofNullable(clazz.cast(coverageHours()));
case "CoverageNormalizedUnits":
return Optional.ofNullable(clazz.cast(coverageNormalizedUnits()));
case "CoverageCost":
return Optional.ofNullable(clazz.cast(coverageCost()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy