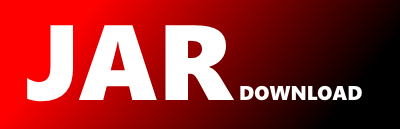
software.amazon.awssdk.services.costexplorer.DefaultCostExplorerClient Maven / Gradle / Ivy
Show all versions of costexplorer Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.costexplorer;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.costexplorer.model.BillExpirationException;
import software.amazon.awssdk.services.costexplorer.model.CostExplorerException;
import software.amazon.awssdk.services.costexplorer.model.CostExplorerRequest;
import software.amazon.awssdk.services.costexplorer.model.CreateAnomalyMonitorRequest;
import software.amazon.awssdk.services.costexplorer.model.CreateAnomalyMonitorResponse;
import software.amazon.awssdk.services.costexplorer.model.CreateAnomalySubscriptionRequest;
import software.amazon.awssdk.services.costexplorer.model.CreateAnomalySubscriptionResponse;
import software.amazon.awssdk.services.costexplorer.model.CreateCostCategoryDefinitionRequest;
import software.amazon.awssdk.services.costexplorer.model.CreateCostCategoryDefinitionResponse;
import software.amazon.awssdk.services.costexplorer.model.DataUnavailableException;
import software.amazon.awssdk.services.costexplorer.model.DeleteAnomalyMonitorRequest;
import software.amazon.awssdk.services.costexplorer.model.DeleteAnomalyMonitorResponse;
import software.amazon.awssdk.services.costexplorer.model.DeleteAnomalySubscriptionRequest;
import software.amazon.awssdk.services.costexplorer.model.DeleteAnomalySubscriptionResponse;
import software.amazon.awssdk.services.costexplorer.model.DeleteCostCategoryDefinitionRequest;
import software.amazon.awssdk.services.costexplorer.model.DeleteCostCategoryDefinitionResponse;
import software.amazon.awssdk.services.costexplorer.model.DescribeCostCategoryDefinitionRequest;
import software.amazon.awssdk.services.costexplorer.model.DescribeCostCategoryDefinitionResponse;
import software.amazon.awssdk.services.costexplorer.model.GetAnomaliesRequest;
import software.amazon.awssdk.services.costexplorer.model.GetAnomaliesResponse;
import software.amazon.awssdk.services.costexplorer.model.GetAnomalyMonitorsRequest;
import software.amazon.awssdk.services.costexplorer.model.GetAnomalyMonitorsResponse;
import software.amazon.awssdk.services.costexplorer.model.GetAnomalySubscriptionsRequest;
import software.amazon.awssdk.services.costexplorer.model.GetAnomalySubscriptionsResponse;
import software.amazon.awssdk.services.costexplorer.model.GetCostAndUsageRequest;
import software.amazon.awssdk.services.costexplorer.model.GetCostAndUsageResponse;
import software.amazon.awssdk.services.costexplorer.model.GetCostAndUsageWithResourcesRequest;
import software.amazon.awssdk.services.costexplorer.model.GetCostAndUsageWithResourcesResponse;
import software.amazon.awssdk.services.costexplorer.model.GetCostCategoriesRequest;
import software.amazon.awssdk.services.costexplorer.model.GetCostCategoriesResponse;
import software.amazon.awssdk.services.costexplorer.model.GetCostForecastRequest;
import software.amazon.awssdk.services.costexplorer.model.GetCostForecastResponse;
import software.amazon.awssdk.services.costexplorer.model.GetDimensionValuesRequest;
import software.amazon.awssdk.services.costexplorer.model.GetDimensionValuesResponse;
import software.amazon.awssdk.services.costexplorer.model.GetReservationCoverageRequest;
import software.amazon.awssdk.services.costexplorer.model.GetReservationCoverageResponse;
import software.amazon.awssdk.services.costexplorer.model.GetReservationPurchaseRecommendationRequest;
import software.amazon.awssdk.services.costexplorer.model.GetReservationPurchaseRecommendationResponse;
import software.amazon.awssdk.services.costexplorer.model.GetReservationUtilizationRequest;
import software.amazon.awssdk.services.costexplorer.model.GetReservationUtilizationResponse;
import software.amazon.awssdk.services.costexplorer.model.GetRightsizingRecommendationRequest;
import software.amazon.awssdk.services.costexplorer.model.GetRightsizingRecommendationResponse;
import software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansCoverageRequest;
import software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansCoverageResponse;
import software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansPurchaseRecommendationRequest;
import software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansPurchaseRecommendationResponse;
import software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansUtilizationDetailsRequest;
import software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansUtilizationDetailsResponse;
import software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansUtilizationRequest;
import software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansUtilizationResponse;
import software.amazon.awssdk.services.costexplorer.model.GetTagsRequest;
import software.amazon.awssdk.services.costexplorer.model.GetTagsResponse;
import software.amazon.awssdk.services.costexplorer.model.GetUsageForecastRequest;
import software.amazon.awssdk.services.costexplorer.model.GetUsageForecastResponse;
import software.amazon.awssdk.services.costexplorer.model.InvalidNextTokenException;
import software.amazon.awssdk.services.costexplorer.model.LimitExceededException;
import software.amazon.awssdk.services.costexplorer.model.ListCostCategoryDefinitionsRequest;
import software.amazon.awssdk.services.costexplorer.model.ListCostCategoryDefinitionsResponse;
import software.amazon.awssdk.services.costexplorer.model.ProvideAnomalyFeedbackRequest;
import software.amazon.awssdk.services.costexplorer.model.ProvideAnomalyFeedbackResponse;
import software.amazon.awssdk.services.costexplorer.model.RequestChangedException;
import software.amazon.awssdk.services.costexplorer.model.ResourceNotFoundException;
import software.amazon.awssdk.services.costexplorer.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.costexplorer.model.UnknownMonitorException;
import software.amazon.awssdk.services.costexplorer.model.UnknownSubscriptionException;
import software.amazon.awssdk.services.costexplorer.model.UnresolvableUsageUnitException;
import software.amazon.awssdk.services.costexplorer.model.UpdateAnomalyMonitorRequest;
import software.amazon.awssdk.services.costexplorer.model.UpdateAnomalyMonitorResponse;
import software.amazon.awssdk.services.costexplorer.model.UpdateAnomalySubscriptionRequest;
import software.amazon.awssdk.services.costexplorer.model.UpdateAnomalySubscriptionResponse;
import software.amazon.awssdk.services.costexplorer.model.UpdateCostCategoryDefinitionRequest;
import software.amazon.awssdk.services.costexplorer.model.UpdateCostCategoryDefinitionResponse;
import software.amazon.awssdk.services.costexplorer.paginators.GetSavingsPlansCoverageIterable;
import software.amazon.awssdk.services.costexplorer.paginators.GetSavingsPlansUtilizationDetailsIterable;
import software.amazon.awssdk.services.costexplorer.paginators.ListCostCategoryDefinitionsIterable;
import software.amazon.awssdk.services.costexplorer.transform.CreateAnomalyMonitorRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.CreateAnomalySubscriptionRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.CreateCostCategoryDefinitionRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.DeleteAnomalyMonitorRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.DeleteAnomalySubscriptionRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.DeleteCostCategoryDefinitionRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.DescribeCostCategoryDefinitionRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetAnomaliesRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetAnomalyMonitorsRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetAnomalySubscriptionsRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetCostAndUsageRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetCostAndUsageWithResourcesRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetCostCategoriesRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetCostForecastRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetDimensionValuesRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetReservationCoverageRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetReservationPurchaseRecommendationRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetReservationUtilizationRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetRightsizingRecommendationRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetSavingsPlansCoverageRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetSavingsPlansPurchaseRecommendationRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetSavingsPlansUtilizationDetailsRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetSavingsPlansUtilizationRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetTagsRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetUsageForecastRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.ListCostCategoryDefinitionsRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.ProvideAnomalyFeedbackRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.UpdateAnomalyMonitorRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.UpdateAnomalySubscriptionRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.UpdateCostCategoryDefinitionRequestMarshaller;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link CostExplorerClient}.
*
* @see CostExplorerClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultCostExplorerClient implements CostExplorerClient {
private static final Logger log = Logger.loggerFor(DefaultCostExplorerClient.class);
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultCostExplorerClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Creates a new cost anomaly detection monitor with the requested type and monitor specification.
*
*
* @param createAnomalyMonitorRequest
* @return Result of the CreateAnomalyMonitor operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.CreateAnomalyMonitor
* @see AWS API
* Documentation
*/
@Override
public CreateAnomalyMonitorResponse createAnomalyMonitor(CreateAnomalyMonitorRequest createAnomalyMonitorRequest)
throws LimitExceededException, AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateAnomalyMonitorResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createAnomalyMonitorRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateAnomalyMonitor");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateAnomalyMonitor").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createAnomalyMonitorRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateAnomalyMonitorRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Adds a subscription to a cost anomaly detection monitor. You can use each subscription to define subscribers with
* email or SNS notifications. Email subscribers can set a dollar threshold and a time frequency for receiving
* notifications.
*
*
* @param createAnomalySubscriptionRequest
* @return Result of the CreateAnomalySubscription operation returned by the service.
* @throws UnknownMonitorException
* The cost anomaly monitor does not exist for the account.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.CreateAnomalySubscription
* @see AWS
* API Documentation
*/
@Override
public CreateAnomalySubscriptionResponse createAnomalySubscription(
CreateAnomalySubscriptionRequest createAnomalySubscriptionRequest) throws UnknownMonitorException,
LimitExceededException, AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateAnomalySubscriptionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createAnomalySubscriptionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateAnomalySubscription");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateAnomalySubscription").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createAnomalySubscriptionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateAnomalySubscriptionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a new Cost Category with the requested name and rules.
*
*
* @param createCostCategoryDefinitionRequest
* @return Result of the CreateCostCategoryDefinition operation returned by the service.
* @throws ServiceQuotaExceededException
* You've reached the limit on the number of resources you can create, or exceeded the size of an individual
* resource.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.CreateCostCategoryDefinition
* @see AWS API Documentation
*/
@Override
public CreateCostCategoryDefinitionResponse createCostCategoryDefinition(
CreateCostCategoryDefinitionRequest createCostCategoryDefinitionRequest) throws ServiceQuotaExceededException,
LimitExceededException, AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateCostCategoryDefinitionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createCostCategoryDefinitionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateCostCategoryDefinition");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateCostCategoryDefinition").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createCostCategoryDefinitionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateCostCategoryDefinitionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a cost anomaly monitor.
*
*
* @param deleteAnomalyMonitorRequest
* @return Result of the DeleteAnomalyMonitor operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws UnknownMonitorException
* The cost anomaly monitor does not exist for the account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.DeleteAnomalyMonitor
* @see AWS API
* Documentation
*/
@Override
public DeleteAnomalyMonitorResponse deleteAnomalyMonitor(DeleteAnomalyMonitorRequest deleteAnomalyMonitorRequest)
throws LimitExceededException, UnknownMonitorException, AwsServiceException, SdkClientException,
CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteAnomalyMonitorResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAnomalyMonitorRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAnomalyMonitor");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteAnomalyMonitor").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteAnomalyMonitorRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteAnomalyMonitorRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a cost anomaly subscription.
*
*
* @param deleteAnomalySubscriptionRequest
* @return Result of the DeleteAnomalySubscription operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws UnknownSubscriptionException
* The cost anomaly subscription does not exist for the account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.DeleteAnomalySubscription
* @see AWS
* API Documentation
*/
@Override
public DeleteAnomalySubscriptionResponse deleteAnomalySubscription(
DeleteAnomalySubscriptionRequest deleteAnomalySubscriptionRequest) throws LimitExceededException,
UnknownSubscriptionException, AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteAnomalySubscriptionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAnomalySubscriptionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAnomalySubscription");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteAnomalySubscription").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteAnomalySubscriptionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteAnomalySubscriptionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a Cost Category. Expenses from this month going forward will no longer be categorized with this Cost
* Category.
*
*
* @param deleteCostCategoryDefinitionRequest
* @return Result of the DeleteCostCategoryDefinition operation returned by the service.
* @throws ResourceNotFoundException
* The specified ARN in the request doesn't exist.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.DeleteCostCategoryDefinition
* @see AWS API Documentation
*/
@Override
public DeleteCostCategoryDefinitionResponse deleteCostCategoryDefinition(
DeleteCostCategoryDefinitionRequest deleteCostCategoryDefinitionRequest) throws ResourceNotFoundException,
LimitExceededException, AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteCostCategoryDefinitionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteCostCategoryDefinitionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteCostCategoryDefinition");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteCostCategoryDefinition").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteCostCategoryDefinitionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteCostCategoryDefinitionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the name, ARN, rules, definition, and effective dates of a Cost Category that's defined in the account.
*
*
* You have the option to use EffectiveOn
to return a Cost Category that is active on a specific date.
* If there is no EffectiveOn
specified, you’ll see a Cost Category that is effective on the current
* date. If Cost Category is still effective, EffectiveEnd
is omitted in the response.
*
*
* @param describeCostCategoryDefinitionRequest
* @return Result of the DescribeCostCategoryDefinition operation returned by the service.
* @throws ResourceNotFoundException
* The specified ARN in the request doesn't exist.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.DescribeCostCategoryDefinition
* @see AWS API Documentation
*/
@Override
public DescribeCostCategoryDefinitionResponse describeCostCategoryDefinition(
DescribeCostCategoryDefinitionRequest describeCostCategoryDefinitionRequest) throws ResourceNotFoundException,
LimitExceededException, AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeCostCategoryDefinitionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeCostCategoryDefinitionRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeCostCategoryDefinition");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeCostCategoryDefinition").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeCostCategoryDefinitionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeCostCategoryDefinitionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves all of the cost anomalies detected on your account, during the time period specified by the
* DateInterval
object.
*
*
* @param getAnomaliesRequest
* @return Result of the GetAnomalies operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetAnomalies
* @see AWS API
* Documentation
*/
@Override
public GetAnomaliesResponse getAnomalies(GetAnomaliesRequest getAnomaliesRequest) throws LimitExceededException,
InvalidNextTokenException, AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetAnomaliesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAnomaliesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAnomalies");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetAnomalies").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getAnomaliesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetAnomaliesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the cost anomaly monitor definitions for your account. You can filter using a list of cost anomaly
* monitor Amazon Resource Names (ARNs).
*
*
* @param getAnomalyMonitorsRequest
* @return Result of the GetAnomalyMonitors operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws UnknownMonitorException
* The cost anomaly monitor does not exist for the account.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetAnomalyMonitors
* @see AWS API
* Documentation
*/
@Override
public GetAnomalyMonitorsResponse getAnomalyMonitors(GetAnomalyMonitorsRequest getAnomalyMonitorsRequest)
throws LimitExceededException, UnknownMonitorException, InvalidNextTokenException, AwsServiceException,
SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetAnomalyMonitorsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAnomalyMonitorsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAnomalyMonitors");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetAnomalyMonitors").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getAnomalyMonitorsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetAnomalyMonitorsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the cost anomaly subscription objects for your account. You can filter using a list of cost anomaly
* monitor Amazon Resource Names (ARNs).
*
*
* @param getAnomalySubscriptionsRequest
* @return Result of the GetAnomalySubscriptions operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws UnknownSubscriptionException
* The cost anomaly subscription does not exist for the account.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetAnomalySubscriptions
* @see AWS
* API Documentation
*/
@Override
public GetAnomalySubscriptionsResponse getAnomalySubscriptions(GetAnomalySubscriptionsRequest getAnomalySubscriptionsRequest)
throws LimitExceededException, UnknownSubscriptionException, InvalidNextTokenException, AwsServiceException,
SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetAnomalySubscriptionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAnomalySubscriptionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAnomalySubscriptions");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAnomalySubscriptions").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getAnomalySubscriptionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetAnomalySubscriptionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves cost and usage metrics for your account. You can specify which cost and usage-related metric, such as
* BlendedCosts
or UsageQuantity
, that you want the request to return. You can also filter
* and group your data by various dimensions, such as SERVICE
or AZ
, in a specific time
* range. For a complete list of valid dimensions, see the GetDimensionValues operation. Management account in an organization in AWS Organizations have access to all
* member accounts.
*
*
* For information about filter limitations, see Quotas and
* restrictions in the Billing and Cost Management User Guide.
*
*
* @param getCostAndUsageRequest
* @return Result of the GetCostAndUsage operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws BillExpirationException
* The requested report expired. Update the date interval and try again.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws RequestChangedException
* Your request parameters changed between pages. Try again with the old parameters or without a pagination
* token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetCostAndUsage
* @see AWS API
* Documentation
*/
@Override
public GetCostAndUsageResponse getCostAndUsage(GetCostAndUsageRequest getCostAndUsageRequest) throws LimitExceededException,
BillExpirationException, DataUnavailableException, InvalidNextTokenException, RequestChangedException,
AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetCostAndUsageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getCostAndUsageRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetCostAndUsage");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetCostAndUsage").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getCostAndUsageRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetCostAndUsageRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves cost and usage metrics with resources for your account. You can specify which cost and usage-related
* metric, such as BlendedCosts
or UsageQuantity
, that you want the request to return. You
* can also filter and group your data by various dimensions, such as SERVICE
or AZ
, in a
* specific time range. For a complete list of valid dimensions, see the GetDimensionValues operation. Management account in an organization in AWS Organizations have access to all
* member accounts. This API is currently available for the Amazon Elastic Compute Cloud – Compute service only.
*
*
*
* This is an opt-in only feature. You can enable this feature from the Cost Explorer Settings page. For information
* on how to access the Settings page, see Controlling Access for Cost
* Explorer in the AWS Billing and Cost Management User Guide.
*
*
*
* @param getCostAndUsageWithResourcesRequest
* @return Result of the GetCostAndUsageWithResources operation returned by the service.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws BillExpirationException
* The requested report expired. Update the date interval and try again.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws RequestChangedException
* Your request parameters changed between pages. Try again with the old parameters or without a pagination
* token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetCostAndUsageWithResources
* @see AWS API Documentation
*/
@Override
public GetCostAndUsageWithResourcesResponse getCostAndUsageWithResources(
GetCostAndUsageWithResourcesRequest getCostAndUsageWithResourcesRequest) throws DataUnavailableException,
LimitExceededException, BillExpirationException, InvalidNextTokenException, RequestChangedException,
AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetCostAndUsageWithResourcesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getCostAndUsageWithResourcesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetCostAndUsageWithResources");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetCostAndUsageWithResources").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getCostAndUsageWithResourcesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetCostAndUsageWithResourcesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves an array of Cost Category names and values incurred cost.
*
*
*
* If some Cost Category names and values are not associated with any cost, they will not be returned by this API.
*
*
*
* @param getCostCategoriesRequest
* @return Result of the GetCostCategories operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws BillExpirationException
* The requested report expired. Update the date interval and try again.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws RequestChangedException
* Your request parameters changed between pages. Try again with the old parameters or without a pagination
* token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetCostCategories
* @see AWS API
* Documentation
*/
@Override
public GetCostCategoriesResponse getCostCategories(GetCostCategoriesRequest getCostCategoriesRequest)
throws LimitExceededException, BillExpirationException, DataUnavailableException, InvalidNextTokenException,
RequestChangedException, AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetCostCategoriesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getCostCategoriesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetCostCategories");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetCostCategories").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getCostCategoriesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetCostCategoriesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a forecast for how much Amazon Web Services predicts that you will spend over the forecast time period
* that you select, based on your past costs.
*
*
* @param getCostForecastRequest
* @return Result of the GetCostForecast operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetCostForecast
* @see AWS API
* Documentation
*/
@Override
public GetCostForecastResponse getCostForecast(GetCostForecastRequest getCostForecastRequest) throws LimitExceededException,
DataUnavailableException, AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetCostForecastResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getCostForecastRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetCostForecast");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetCostForecast").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getCostForecastRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetCostForecastRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves all available filter values for a specified filter over a period of time. You can search the dimension
* values for an arbitrary string.
*
*
* @param getDimensionValuesRequest
* @return Result of the GetDimensionValues operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws BillExpirationException
* The requested report expired. Update the date interval and try again.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws RequestChangedException
* Your request parameters changed between pages. Try again with the old parameters or without a pagination
* token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetDimensionValues
* @see AWS API
* Documentation
*/
@Override
public GetDimensionValuesResponse getDimensionValues(GetDimensionValuesRequest getDimensionValuesRequest)
throws LimitExceededException, BillExpirationException, DataUnavailableException, InvalidNextTokenException,
RequestChangedException, AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDimensionValuesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDimensionValuesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDimensionValues");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetDimensionValues").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getDimensionValuesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetDimensionValuesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the reservation coverage for your account. This enables you to see how much of your Amazon Elastic
* Compute Cloud, Amazon ElastiCache, Amazon Relational Database Service, or Amazon Redshift usage is covered by a
* reservation. An organization's management account can see the coverage of the associated member accounts. This
* supports dimensions, Cost Categories, and nested expressions. For any time period, you can filter data about
* reservation usage by the following dimensions:
*
*
* -
*
* AZ
*
*
* -
*
* CACHE_ENGINE
*
*
* -
*
* DATABASE_ENGINE
*
*
* -
*
* DEPLOYMENT_OPTION
*
*
* -
*
* INSTANCE_TYPE
*
*
* -
*
* LINKED_ACCOUNT
*
*
* -
*
* OPERATING_SYSTEM
*
*
* -
*
* PLATFORM
*
*
* -
*
* REGION
*
*
* -
*
* SERVICE
*
*
* -
*
* TAG
*
*
* -
*
* TENANCY
*
*
*
*
* To determine valid values for a dimension, use the GetDimensionValues
operation.
*
*
* @param getReservationCoverageRequest
* You can use the following request parameters to query for how much of your instance usage a reservation
* covered.
* @return Result of the GetReservationCoverage operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetReservationCoverage
* @see AWS API
* Documentation
*/
@Override
public GetReservationCoverageResponse getReservationCoverage(GetReservationCoverageRequest getReservationCoverageRequest)
throws LimitExceededException, DataUnavailableException, InvalidNextTokenException, AwsServiceException,
SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetReservationCoverageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getReservationCoverageRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetReservationCoverage");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetReservationCoverage").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getReservationCoverageRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetReservationCoverageRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets recommendations for which reservations to purchase. These recommendations could help you reduce your costs.
* Reservations provide a discounted hourly rate (up to 75%) compared to On-Demand pricing.
*
*
* AWS generates your recommendations by identifying your On-Demand usage during a specific time period and
* collecting your usage into categories that are eligible for a reservation. After AWS has these categories, it
* simulates every combination of reservations in each category of usage to identify the best number of each type of
* RI to purchase to maximize your estimated savings.
*
*
* For example, AWS automatically aggregates your Amazon EC2 Linux, shared tenancy, and c4 family usage in the US
* West (Oregon) Region and recommends that you buy size-flexible regional reservations to apply to the c4 family
* usage. AWS recommends the smallest size instance in an instance family. This makes it easier to purchase a
* size-flexible RI. AWS also shows the equal number of normalized units so that you can purchase any instance size
* that you want. For this example, your RI recommendation would be for c4.large
because that is the
* smallest size instance in the c4 instance family.
*
*
* @param getReservationPurchaseRecommendationRequest
* @return Result of the GetReservationPurchaseRecommendation operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetReservationPurchaseRecommendation
* @see AWS API Documentation
*/
@Override
public GetReservationPurchaseRecommendationResponse getReservationPurchaseRecommendation(
GetReservationPurchaseRecommendationRequest getReservationPurchaseRecommendationRequest)
throws LimitExceededException, DataUnavailableException, InvalidNextTokenException, AwsServiceException,
SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, GetReservationPurchaseRecommendationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getReservationPurchaseRecommendationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetReservationPurchaseRecommendation");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetReservationPurchaseRecommendation").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler)
.withInput(getReservationPurchaseRecommendationRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetReservationPurchaseRecommendationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the reservation utilization for your account. Management account in an organization have access to
* member accounts. You can filter data by dimensions in a time period. You can use GetDimensionValues
* to determine the possible dimension values. Currently, you can group only by SUBSCRIPTION_ID
.
*
*
* @param getReservationUtilizationRequest
* @return Result of the GetReservationUtilization operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetReservationUtilization
* @see AWS
* API Documentation
*/
@Override
public GetReservationUtilizationResponse getReservationUtilization(
GetReservationUtilizationRequest getReservationUtilizationRequest) throws LimitExceededException,
DataUnavailableException, InvalidNextTokenException, AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetReservationUtilizationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getReservationUtilizationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetReservationUtilization");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetReservationUtilization").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getReservationUtilizationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetReservationUtilizationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates recommendations that help you save cost by identifying idle and underutilized Amazon EC2 instances.
*
*
* Recommendations are generated to either downsize or terminate instances, along with providing savings detail and
* metrics. For details on calculation and function, see Optimizing Your Cost with
* Rightsizing Recommendations in the AWS Billing and Cost Management User Guide.
*
*
* @param getRightsizingRecommendationRequest
* @return Result of the GetRightsizingRecommendation operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetRightsizingRecommendation
* @see AWS API Documentation
*/
@Override
public GetRightsizingRecommendationResponse getRightsizingRecommendation(
GetRightsizingRecommendationRequest getRightsizingRecommendationRequest) throws LimitExceededException,
InvalidNextTokenException, AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetRightsizingRecommendationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getRightsizingRecommendationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetRightsizingRecommendation");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetRightsizingRecommendation").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getRightsizingRecommendationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetRightsizingRecommendationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the Savings Plans covered for your account. This enables you to see how much of your cost is covered by
* a Savings Plan. An organization’s management account can see the coverage of the associated member accounts. This
* supports dimensions, Cost Categories, and nested expressions. For any time period, you can filter data for
* Savings Plans usage with the following dimensions:
*
*
* -
*
* LINKED_ACCOUNT
*
*
* -
*
* REGION
*
*
* -
*
* SERVICE
*
*
* -
*
* INSTANCE_FAMILY
*
*
*
*
* To determine valid values for a dimension, use the GetDimensionValues
operation.
*
*
* @param getSavingsPlansCoverageRequest
* @return Result of the GetSavingsPlansCoverage operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetSavingsPlansCoverage
* @see AWS
* API Documentation
*/
@Override
public GetSavingsPlansCoverageResponse getSavingsPlansCoverage(GetSavingsPlansCoverageRequest getSavingsPlansCoverageRequest)
throws LimitExceededException, DataUnavailableException, InvalidNextTokenException, AwsServiceException,
SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetSavingsPlansCoverageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getSavingsPlansCoverageRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetSavingsPlansCoverage");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetSavingsPlansCoverage").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getSavingsPlansCoverageRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetSavingsPlansCoverageRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the Savings Plans covered for your account. This enables you to see how much of your cost is covered by
* a Savings Plan. An organization’s management account can see the coverage of the associated member accounts. This
* supports dimensions, Cost Categories, and nested expressions. For any time period, you can filter data for
* Savings Plans usage with the following dimensions:
*
*
* -
*
* LINKED_ACCOUNT
*
*
* -
*
* REGION
*
*
* -
*
* SERVICE
*
*
* -
*
* INSTANCE_FAMILY
*
*
*
*
* To determine valid values for a dimension, use the GetDimensionValues
operation.
*
*
*
* This is a variant of
* {@link #getSavingsPlansCoverage(software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansCoverageRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.GetSavingsPlansCoverageIterable responses = client.getSavingsPlansCoveragePaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.costexplorer.paginators.GetSavingsPlansCoverageIterable responses = client
* .getSavingsPlansCoveragePaginator(request);
* for (software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansCoverageResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.GetSavingsPlansCoverageIterable responses = client.getSavingsPlansCoveragePaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getSavingsPlansCoverage(software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansCoverageRequest)}
* operation.
*
*
* @param getSavingsPlansCoverageRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetSavingsPlansCoverage
* @see AWS
* API Documentation
*/
@Override
public GetSavingsPlansCoverageIterable getSavingsPlansCoveragePaginator(
GetSavingsPlansCoverageRequest getSavingsPlansCoverageRequest) throws LimitExceededException,
DataUnavailableException, InvalidNextTokenException, AwsServiceException, SdkClientException, CostExplorerException {
return new GetSavingsPlansCoverageIterable(this, applyPaginatorUserAgent(getSavingsPlansCoverageRequest));
}
/**
*
* Retrieves your request parameters, Savings Plan Recommendations Summary and Details.
*
*
* @param getSavingsPlansPurchaseRecommendationRequest
* @return Result of the GetSavingsPlansPurchaseRecommendation operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetSavingsPlansPurchaseRecommendation
* @see AWS API Documentation
*/
@Override
public GetSavingsPlansPurchaseRecommendationResponse getSavingsPlansPurchaseRecommendation(
GetSavingsPlansPurchaseRecommendationRequest getSavingsPlansPurchaseRecommendationRequest)
throws LimitExceededException, InvalidNextTokenException, AwsServiceException, SdkClientException,
CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, GetSavingsPlansPurchaseRecommendationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getSavingsPlansPurchaseRecommendationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetSavingsPlansPurchaseRecommendation");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetSavingsPlansPurchaseRecommendation").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler)
.withInput(getSavingsPlansPurchaseRecommendationRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetSavingsPlansPurchaseRecommendationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the Savings Plans utilization for your account across date ranges with daily or monthly granularity.
* Management account in an organization have access to member accounts. You can use GetDimensionValues
* in SAVINGS_PLANS
to determine the possible dimension values.
*
*
*
* You cannot group by any dimension values for GetSavingsPlansUtilization
.
*
*
*
* @param getSavingsPlansUtilizationRequest
* @return Result of the GetSavingsPlansUtilization operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetSavingsPlansUtilization
* @see AWS
* API Documentation
*/
@Override
public GetSavingsPlansUtilizationResponse getSavingsPlansUtilization(
GetSavingsPlansUtilizationRequest getSavingsPlansUtilizationRequest) throws LimitExceededException,
DataUnavailableException, AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetSavingsPlansUtilizationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getSavingsPlansUtilizationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetSavingsPlansUtilization");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetSavingsPlansUtilization").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getSavingsPlansUtilizationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetSavingsPlansUtilizationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves attribute data along with aggregate utilization and savings data for a given time period. This doesn't
* support granular or grouped data (daily/monthly) in response. You can't retrieve data by dates in a single
* response similar to GetSavingsPlanUtilization
, but you have the option to make multiple calls to
* GetSavingsPlanUtilizationDetails
by providing individual dates. You can use
* GetDimensionValues
in SAVINGS_PLANS
to determine the possible dimension values.
*
*
*
* GetSavingsPlanUtilizationDetails
internally groups data by SavingsPlansArn
.
*
*
*
* @param getSavingsPlansUtilizationDetailsRequest
* @return Result of the GetSavingsPlansUtilizationDetails operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetSavingsPlansUtilizationDetails
* @see AWS API Documentation
*/
@Override
public GetSavingsPlansUtilizationDetailsResponse getSavingsPlansUtilizationDetails(
GetSavingsPlansUtilizationDetailsRequest getSavingsPlansUtilizationDetailsRequest) throws LimitExceededException,
DataUnavailableException, InvalidNextTokenException, AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetSavingsPlansUtilizationDetailsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getSavingsPlansUtilizationDetailsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetSavingsPlansUtilizationDetails");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetSavingsPlansUtilizationDetails").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getSavingsPlansUtilizationDetailsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetSavingsPlansUtilizationDetailsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves attribute data along with aggregate utilization and savings data for a given time period. This doesn't
* support granular or grouped data (daily/monthly) in response. You can't retrieve data by dates in a single
* response similar to GetSavingsPlanUtilization
, but you have the option to make multiple calls to
* GetSavingsPlanUtilizationDetails
by providing individual dates. You can use
* GetDimensionValues
in SAVINGS_PLANS
to determine the possible dimension values.
*
*
*
* GetSavingsPlanUtilizationDetails
internally groups data by SavingsPlansArn
.
*
*
*
* This is a variant of
* {@link #getSavingsPlansUtilizationDetails(software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansUtilizationDetailsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.GetSavingsPlansUtilizationDetailsIterable responses = client.getSavingsPlansUtilizationDetailsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.costexplorer.paginators.GetSavingsPlansUtilizationDetailsIterable responses = client
* .getSavingsPlansUtilizationDetailsPaginator(request);
* for (software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansUtilizationDetailsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.GetSavingsPlansUtilizationDetailsIterable responses = client.getSavingsPlansUtilizationDetailsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getSavingsPlansUtilizationDetails(software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansUtilizationDetailsRequest)}
* operation.
*
*
* @param getSavingsPlansUtilizationDetailsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetSavingsPlansUtilizationDetails
* @see AWS API Documentation
*/
@Override
public GetSavingsPlansUtilizationDetailsIterable getSavingsPlansUtilizationDetailsPaginator(
GetSavingsPlansUtilizationDetailsRequest getSavingsPlansUtilizationDetailsRequest) throws LimitExceededException,
DataUnavailableException, InvalidNextTokenException, AwsServiceException, SdkClientException, CostExplorerException {
return new GetSavingsPlansUtilizationDetailsIterable(this,
applyPaginatorUserAgent(getSavingsPlansUtilizationDetailsRequest));
}
/**
*
* Queries for available tag keys and tag values for a specified period. You can search the tag values for an
* arbitrary string.
*
*
* @param getTagsRequest
* @return Result of the GetTags operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws BillExpirationException
* The requested report expired. Update the date interval and try again.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws RequestChangedException
* Your request parameters changed between pages. Try again with the old parameters or without a pagination
* token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetTags
* @see AWS API
* Documentation
*/
@Override
public GetTagsResponse getTags(GetTagsRequest getTagsRequest) throws LimitExceededException, BillExpirationException,
DataUnavailableException, InvalidNextTokenException, RequestChangedException, AwsServiceException,
SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetTagsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getTagsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetTags");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetTags").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getTagsRequest)
.withMetricCollector(apiCallMetricCollector).withMarshaller(new GetTagsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a forecast for how much Amazon Web Services predicts that you will use over the forecast time period
* that you select, based on your past usage.
*
*
* @param getUsageForecastRequest
* @return Result of the GetUsageForecast operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws UnresolvableUsageUnitException
* Cost Explorer was unable to identify the usage unit. Provide UsageType/UsageTypeGroup
filter
* selections that contain matching units, for example: hours
.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetUsageForecast
* @see AWS API
* Documentation
*/
@Override
public GetUsageForecastResponse getUsageForecast(GetUsageForecastRequest getUsageForecastRequest)
throws LimitExceededException, DataUnavailableException, UnresolvableUsageUnitException, AwsServiceException,
SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetUsageForecastResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getUsageForecastRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetUsageForecast");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetUsageForecast").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getUsageForecastRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetUsageForecastRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the name, ARN, NumberOfRules
and effective dates of all Cost Categories defined in the
* account. You have the option to use EffectiveOn
to return a list of Cost Categories that were active
* on a specific date. If there is no EffectiveOn
specified, you’ll see Cost Categories that are
* effective on the current date. If Cost Category is still effective, EffectiveEnd
is omitted in the
* response. ListCostCategoryDefinitions
supports pagination. The request can have a
* MaxResults
range up to 100.
*
*
* @param listCostCategoryDefinitionsRequest
* @return Result of the ListCostCategoryDefinitions operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.ListCostCategoryDefinitions
* @see AWS API Documentation
*/
@Override
public ListCostCategoryDefinitionsResponse listCostCategoryDefinitions(
ListCostCategoryDefinitionsRequest listCostCategoryDefinitionsRequest) throws LimitExceededException,
AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListCostCategoryDefinitionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listCostCategoryDefinitionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListCostCategoryDefinitions");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListCostCategoryDefinitions").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listCostCategoryDefinitionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListCostCategoryDefinitionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the name, ARN, NumberOfRules
and effective dates of all Cost Categories defined in the
* account. You have the option to use EffectiveOn
to return a list of Cost Categories that were active
* on a specific date. If there is no EffectiveOn
specified, you’ll see Cost Categories that are
* effective on the current date. If Cost Category is still effective, EffectiveEnd
is omitted in the
* response. ListCostCategoryDefinitions
supports pagination. The request can have a
* MaxResults
range up to 100.
*
*
*
* This is a variant of
* {@link #listCostCategoryDefinitions(software.amazon.awssdk.services.costexplorer.model.ListCostCategoryDefinitionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.ListCostCategoryDefinitionsIterable responses = client.listCostCategoryDefinitionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.costexplorer.paginators.ListCostCategoryDefinitionsIterable responses = client
* .listCostCategoryDefinitionsPaginator(request);
* for (software.amazon.awssdk.services.costexplorer.model.ListCostCategoryDefinitionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.ListCostCategoryDefinitionsIterable responses = client.listCostCategoryDefinitionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listCostCategoryDefinitions(software.amazon.awssdk.services.costexplorer.model.ListCostCategoryDefinitionsRequest)}
* operation.
*
*
* @param listCostCategoryDefinitionsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.ListCostCategoryDefinitions
* @see AWS API Documentation
*/
@Override
public ListCostCategoryDefinitionsIterable listCostCategoryDefinitionsPaginator(
ListCostCategoryDefinitionsRequest listCostCategoryDefinitionsRequest) throws LimitExceededException,
AwsServiceException, SdkClientException, CostExplorerException {
return new ListCostCategoryDefinitionsIterable(this, applyPaginatorUserAgent(listCostCategoryDefinitionsRequest));
}
/**
*
* Modifies the feedback property of a given cost anomaly.
*
*
* @param provideAnomalyFeedbackRequest
* @return Result of the ProvideAnomalyFeedback operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.ProvideAnomalyFeedback
* @see AWS API
* Documentation
*/
@Override
public ProvideAnomalyFeedbackResponse provideAnomalyFeedback(ProvideAnomalyFeedbackRequest provideAnomalyFeedbackRequest)
throws LimitExceededException, AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ProvideAnomalyFeedbackResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, provideAnomalyFeedbackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ProvideAnomalyFeedback");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ProvideAnomalyFeedback").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(provideAnomalyFeedbackRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ProvideAnomalyFeedbackRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates an existing cost anomaly monitor. The changes made are applied going forward, and does not change
* anomalies detected in the past.
*
*
* @param updateAnomalyMonitorRequest
* @return Result of the UpdateAnomalyMonitor operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws UnknownMonitorException
* The cost anomaly monitor does not exist for the account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.UpdateAnomalyMonitor
* @see AWS API
* Documentation
*/
@Override
public UpdateAnomalyMonitorResponse updateAnomalyMonitor(UpdateAnomalyMonitorRequest updateAnomalyMonitorRequest)
throws LimitExceededException, UnknownMonitorException, AwsServiceException, SdkClientException,
CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateAnomalyMonitorResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateAnomalyMonitorRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateAnomalyMonitor");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateAnomalyMonitor").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateAnomalyMonitorRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateAnomalyMonitorRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates an existing cost anomaly monitor subscription.
*
*
* @param updateAnomalySubscriptionRequest
* @return Result of the UpdateAnomalySubscription operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws UnknownMonitorException
* The cost anomaly monitor does not exist for the account.
* @throws UnknownSubscriptionException
* The cost anomaly subscription does not exist for the account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.UpdateAnomalySubscription
* @see AWS
* API Documentation
*/
@Override
public UpdateAnomalySubscriptionResponse updateAnomalySubscription(
UpdateAnomalySubscriptionRequest updateAnomalySubscriptionRequest) throws LimitExceededException,
UnknownMonitorException, UnknownSubscriptionException, AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateAnomalySubscriptionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateAnomalySubscriptionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateAnomalySubscription");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateAnomalySubscription").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateAnomalySubscriptionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateAnomalySubscriptionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates an existing Cost Category. Changes made to the Cost Category rules will be used to categorize the current
* month’s expenses and future expenses. This won’t change categorization for the previous months.
*
*
* @param updateCostCategoryDefinitionRequest
* @return Result of the UpdateCostCategoryDefinition operation returned by the service.
* @throws ResourceNotFoundException
* The specified ARN in the request doesn't exist.
* @throws ServiceQuotaExceededException
* You've reached the limit on the number of resources you can create, or exceeded the size of an individual
* resource.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.UpdateCostCategoryDefinition
* @see AWS API Documentation
*/
@Override
public UpdateCostCategoryDefinitionResponse updateCostCategoryDefinition(
UpdateCostCategoryDefinitionRequest updateCostCategoryDefinitionRequest) throws ResourceNotFoundException,
ServiceQuotaExceededException, LimitExceededException, AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateCostCategoryDefinitionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateCostCategoryDefinitionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Cost Explorer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateCostCategoryDefinition");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateCostCategoryDefinition").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateCostCategoryDefinitionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateCostCategoryDefinitionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(CostExplorerException::builder)
.protocol(AwsJsonProtocol.AWS_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("UnresolvableUsageUnitException")
.exceptionBuilderSupplier(UnresolvableUsageUnitException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("UnknownMonitorException")
.exceptionBuilderSupplier(UnknownMonitorException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("UnknownSubscriptionException")
.exceptionBuilderSupplier(UnknownSubscriptionException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("RequestChangedException")
.exceptionBuilderSupplier(RequestChangedException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DataUnavailableException")
.exceptionBuilderSupplier(DataUnavailableException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServiceQuotaExceededException")
.exceptionBuilderSupplier(ServiceQuotaExceededException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidNextTokenException")
.exceptionBuilderSupplier(InvalidNextTokenException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LimitExceededException")
.exceptionBuilderSupplier(LimitExceededException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("BillExpirationException")
.exceptionBuilderSupplier(BillExpirationException::builder).build());
}
@Override
public void close() {
clientHandler.close();
}
private T applyPaginatorUserAgent(T request) {
Consumer userAgentApplier = b -> b.addApiName(ApiName.builder()
.version(VersionInfo.SDK_VERSION).name("PAGINATED").build());
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(userAgentApplier).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(userAgentApplier).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
}