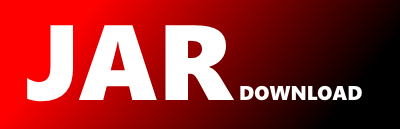
software.amazon.awssdk.services.costexplorer.model.TargetInstance Maven / Gradle / Ivy
Show all versions of costexplorer Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.costexplorer.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Details on recommended instance.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class TargetInstance implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ESTIMATED_MONTHLY_COST_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EstimatedMonthlyCost").getter(getter(TargetInstance::estimatedMonthlyCost))
.setter(setter(Builder::estimatedMonthlyCost))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EstimatedMonthlyCost").build())
.build();
private static final SdkField ESTIMATED_MONTHLY_SAVINGS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EstimatedMonthlySavings").getter(getter(TargetInstance::estimatedMonthlySavings))
.setter(setter(Builder::estimatedMonthlySavings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EstimatedMonthlySavings").build())
.build();
private static final SdkField CURRENCY_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CurrencyCode").getter(getter(TargetInstance::currencyCode)).setter(setter(Builder::currencyCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CurrencyCode").build()).build();
private static final SdkField DEFAULT_TARGET_INSTANCE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("DefaultTargetInstance").getter(getter(TargetInstance::defaultTargetInstance))
.setter(setter(Builder::defaultTargetInstance))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DefaultTargetInstance").build())
.build();
private static final SdkField RESOURCE_DETAILS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ResourceDetails")
.getter(getter(TargetInstance::resourceDetails)).setter(setter(Builder::resourceDetails))
.constructor(ResourceDetails::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceDetails").build()).build();
private static final SdkField EXPECTED_RESOURCE_UTILIZATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("ExpectedResourceUtilization")
.getter(getter(TargetInstance::expectedResourceUtilization))
.setter(setter(Builder::expectedResourceUtilization))
.constructor(ResourceUtilization::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExpectedResourceUtilization")
.build()).build();
private static final SdkField> PLATFORM_DIFFERENCES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("PlatformDifferences")
.getter(getter(TargetInstance::platformDifferencesAsStrings))
.setter(setter(Builder::platformDifferencesWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PlatformDifferences").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ESTIMATED_MONTHLY_COST_FIELD,
ESTIMATED_MONTHLY_SAVINGS_FIELD, CURRENCY_CODE_FIELD, DEFAULT_TARGET_INSTANCE_FIELD, RESOURCE_DETAILS_FIELD,
EXPECTED_RESOURCE_UTILIZATION_FIELD, PLATFORM_DIFFERENCES_FIELD));
private static final long serialVersionUID = 1L;
private final String estimatedMonthlyCost;
private final String estimatedMonthlySavings;
private final String currencyCode;
private final Boolean defaultTargetInstance;
private final ResourceDetails resourceDetails;
private final ResourceUtilization expectedResourceUtilization;
private final List platformDifferences;
private TargetInstance(BuilderImpl builder) {
this.estimatedMonthlyCost = builder.estimatedMonthlyCost;
this.estimatedMonthlySavings = builder.estimatedMonthlySavings;
this.currencyCode = builder.currencyCode;
this.defaultTargetInstance = builder.defaultTargetInstance;
this.resourceDetails = builder.resourceDetails;
this.expectedResourceUtilization = builder.expectedResourceUtilization;
this.platformDifferences = builder.platformDifferences;
}
/**
*
* Expected cost to operate this instance type on a monthly basis.
*
*
* @return Expected cost to operate this instance type on a monthly basis.
*/
public final String estimatedMonthlyCost() {
return estimatedMonthlyCost;
}
/**
*
* Estimated savings resulting from modification, on a monthly basis.
*
*
* @return Estimated savings resulting from modification, on a monthly basis.
*/
public final String estimatedMonthlySavings() {
return estimatedMonthlySavings;
}
/**
*
* The currency code that AWS used to calculate the costs for this instance.
*
*
* @return The currency code that AWS used to calculate the costs for this instance.
*/
public final String currencyCode() {
return currencyCode;
}
/**
*
* Indicates whether this recommendation is the defaulted AWS recommendation.
*
*
* @return Indicates whether this recommendation is the defaulted AWS recommendation.
*/
public final Boolean defaultTargetInstance() {
return defaultTargetInstance;
}
/**
*
* Details on the target instance type.
*
*
* @return Details on the target instance type.
*/
public final ResourceDetails resourceDetails() {
return resourceDetails;
}
/**
*
* Expected utilization metrics for target instance type.
*
*
* @return Expected utilization metrics for target instance type.
*/
public final ResourceUtilization expectedResourceUtilization() {
return expectedResourceUtilization;
}
/**
*
* Explains the actions you might need to take in order to successfully migrate your workloads from the current
* instance type to the recommended instance type.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasPlatformDifferences()} to see if a value was sent in this field.
*
*
* @return Explains the actions you might need to take in order to successfully migrate your workloads from the
* current instance type to the recommended instance type.
*/
public final List platformDifferences() {
return PlatformDifferencesCopier.copyStringToEnum(platformDifferences);
}
/**
* Returns true if the PlatformDifferences property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasPlatformDifferences() {
return platformDifferences != null && !(platformDifferences instanceof SdkAutoConstructList);
}
/**
*
* Explains the actions you might need to take in order to successfully migrate your workloads from the current
* instance type to the recommended instance type.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasPlatformDifferences()} to see if a value was sent in this field.
*
*
* @return Explains the actions you might need to take in order to successfully migrate your workloads from the
* current instance type to the recommended instance type.
*/
public final List platformDifferencesAsStrings() {
return platformDifferences;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(estimatedMonthlyCost());
hashCode = 31 * hashCode + Objects.hashCode(estimatedMonthlySavings());
hashCode = 31 * hashCode + Objects.hashCode(currencyCode());
hashCode = 31 * hashCode + Objects.hashCode(defaultTargetInstance());
hashCode = 31 * hashCode + Objects.hashCode(resourceDetails());
hashCode = 31 * hashCode + Objects.hashCode(expectedResourceUtilization());
hashCode = 31 * hashCode + Objects.hashCode(hasPlatformDifferences() ? platformDifferencesAsStrings() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof TargetInstance)) {
return false;
}
TargetInstance other = (TargetInstance) obj;
return Objects.equals(estimatedMonthlyCost(), other.estimatedMonthlyCost())
&& Objects.equals(estimatedMonthlySavings(), other.estimatedMonthlySavings())
&& Objects.equals(currencyCode(), other.currencyCode())
&& Objects.equals(defaultTargetInstance(), other.defaultTargetInstance())
&& Objects.equals(resourceDetails(), other.resourceDetails())
&& Objects.equals(expectedResourceUtilization(), other.expectedResourceUtilization())
&& hasPlatformDifferences() == other.hasPlatformDifferences()
&& Objects.equals(platformDifferencesAsStrings(), other.platformDifferencesAsStrings());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("TargetInstance").add("EstimatedMonthlyCost", estimatedMonthlyCost())
.add("EstimatedMonthlySavings", estimatedMonthlySavings()).add("CurrencyCode", currencyCode())
.add("DefaultTargetInstance", defaultTargetInstance()).add("ResourceDetails", resourceDetails())
.add("ExpectedResourceUtilization", expectedResourceUtilization())
.add("PlatformDifferences", hasPlatformDifferences() ? platformDifferencesAsStrings() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EstimatedMonthlyCost":
return Optional.ofNullable(clazz.cast(estimatedMonthlyCost()));
case "EstimatedMonthlySavings":
return Optional.ofNullable(clazz.cast(estimatedMonthlySavings()));
case "CurrencyCode":
return Optional.ofNullable(clazz.cast(currencyCode()));
case "DefaultTargetInstance":
return Optional.ofNullable(clazz.cast(defaultTargetInstance()));
case "ResourceDetails":
return Optional.ofNullable(clazz.cast(resourceDetails()));
case "ExpectedResourceUtilization":
return Optional.ofNullable(clazz.cast(expectedResourceUtilization()));
case "PlatformDifferences":
return Optional.ofNullable(clazz.cast(platformDifferencesAsStrings()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function