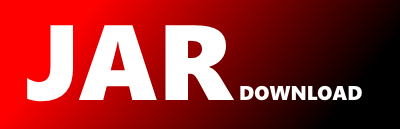
software.amazon.awssdk.services.costexplorer.model.Anomaly Maven / Gradle / Ivy
Show all versions of costexplorer Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.costexplorer.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An unusual cost pattern. This consists of the detailed metadata and the current status of the anomaly object.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Anomaly implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ANOMALY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AnomalyId").getter(getter(Anomaly::anomalyId)).setter(setter(Builder::anomalyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AnomalyId").build()).build();
private static final SdkField ANOMALY_START_DATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AnomalyStartDate").getter(getter(Anomaly::anomalyStartDate)).setter(setter(Builder::anomalyStartDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AnomalyStartDate").build()).build();
private static final SdkField ANOMALY_END_DATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AnomalyEndDate").getter(getter(Anomaly::anomalyEndDate)).setter(setter(Builder::anomalyEndDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AnomalyEndDate").build()).build();
private static final SdkField DIMENSION_VALUE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DimensionValue").getter(getter(Anomaly::dimensionValue)).setter(setter(Builder::dimensionValue))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DimensionValue").build()).build();
private static final SdkField> ROOT_CAUSES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("RootCauses")
.getter(getter(Anomaly::rootCauses))
.setter(setter(Builder::rootCauses))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RootCauses").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(RootCause::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ANOMALY_SCORE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("AnomalyScore").getter(getter(Anomaly::anomalyScore)).setter(setter(Builder::anomalyScore))
.constructor(AnomalyScore::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AnomalyScore").build()).build();
private static final SdkField IMPACT_FIELD = SdkField. builder(MarshallingType.SDK_POJO).memberName("Impact")
.getter(getter(Anomaly::impact)).setter(setter(Builder::impact)).constructor(Impact::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Impact").build()).build();
private static final SdkField MONITOR_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MonitorArn").getter(getter(Anomaly::monitorArn)).setter(setter(Builder::monitorArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MonitorArn").build()).build();
private static final SdkField FEEDBACK_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Feedback").getter(getter(Anomaly::feedbackAsString)).setter(setter(Builder::feedback))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Feedback").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ANOMALY_ID_FIELD,
ANOMALY_START_DATE_FIELD, ANOMALY_END_DATE_FIELD, DIMENSION_VALUE_FIELD, ROOT_CAUSES_FIELD, ANOMALY_SCORE_FIELD,
IMPACT_FIELD, MONITOR_ARN_FIELD, FEEDBACK_FIELD));
private static final long serialVersionUID = 1L;
private final String anomalyId;
private final String anomalyStartDate;
private final String anomalyEndDate;
private final String dimensionValue;
private final List rootCauses;
private final AnomalyScore anomalyScore;
private final Impact impact;
private final String monitorArn;
private final String feedback;
private Anomaly(BuilderImpl builder) {
this.anomalyId = builder.anomalyId;
this.anomalyStartDate = builder.anomalyStartDate;
this.anomalyEndDate = builder.anomalyEndDate;
this.dimensionValue = builder.dimensionValue;
this.rootCauses = builder.rootCauses;
this.anomalyScore = builder.anomalyScore;
this.impact = builder.impact;
this.monitorArn = builder.monitorArn;
this.feedback = builder.feedback;
}
/**
*
* The unique identifier for the anomaly.
*
*
* @return The unique identifier for the anomaly.
*/
public final String anomalyId() {
return anomalyId;
}
/**
*
* The first day the anomaly is detected.
*
*
* @return The first day the anomaly is detected.
*/
public final String anomalyStartDate() {
return anomalyStartDate;
}
/**
*
* The last day the anomaly is detected.
*
*
* @return The last day the anomaly is detected.
*/
public final String anomalyEndDate() {
return anomalyEndDate;
}
/**
*
* The dimension for the anomaly (for example, an Amazon Web Service in a service monitor).
*
*
* @return The dimension for the anomaly (for example, an Amazon Web Service in a service monitor).
*/
public final String dimensionValue() {
return dimensionValue;
}
/**
* For responses, this returns true if the service returned a value for the RootCauses property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasRootCauses() {
return rootCauses != null && !(rootCauses instanceof SdkAutoConstructList);
}
/**
*
* The list of identified root causes for the anomaly.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasRootCauses} method.
*
*
* @return The list of identified root causes for the anomaly.
*/
public final List rootCauses() {
return rootCauses;
}
/**
*
* The latest and maximum score for the anomaly.
*
*
* @return The latest and maximum score for the anomaly.
*/
public final AnomalyScore anomalyScore() {
return anomalyScore;
}
/**
*
* The dollar impact for the anomaly.
*
*
* @return The dollar impact for the anomaly.
*/
public final Impact impact() {
return impact;
}
/**
*
* The Amazon Resource Name (ARN) for the cost monitor that generated this anomaly.
*
*
* @return The Amazon Resource Name (ARN) for the cost monitor that generated this anomaly.
*/
public final String monitorArn() {
return monitorArn;
}
/**
*
* The feedback value.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #feedback} will
* return {@link AnomalyFeedbackType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #feedbackAsString}.
*
*
* @return The feedback value.
* @see AnomalyFeedbackType
*/
public final AnomalyFeedbackType feedback() {
return AnomalyFeedbackType.fromValue(feedback);
}
/**
*
* The feedback value.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #feedback} will
* return {@link AnomalyFeedbackType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #feedbackAsString}.
*
*
* @return The feedback value.
* @see AnomalyFeedbackType
*/
public final String feedbackAsString() {
return feedback;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(anomalyId());
hashCode = 31 * hashCode + Objects.hashCode(anomalyStartDate());
hashCode = 31 * hashCode + Objects.hashCode(anomalyEndDate());
hashCode = 31 * hashCode + Objects.hashCode(dimensionValue());
hashCode = 31 * hashCode + Objects.hashCode(hasRootCauses() ? rootCauses() : null);
hashCode = 31 * hashCode + Objects.hashCode(anomalyScore());
hashCode = 31 * hashCode + Objects.hashCode(impact());
hashCode = 31 * hashCode + Objects.hashCode(monitorArn());
hashCode = 31 * hashCode + Objects.hashCode(feedbackAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Anomaly)) {
return false;
}
Anomaly other = (Anomaly) obj;
return Objects.equals(anomalyId(), other.anomalyId()) && Objects.equals(anomalyStartDate(), other.anomalyStartDate())
&& Objects.equals(anomalyEndDate(), other.anomalyEndDate())
&& Objects.equals(dimensionValue(), other.dimensionValue()) && hasRootCauses() == other.hasRootCauses()
&& Objects.equals(rootCauses(), other.rootCauses()) && Objects.equals(anomalyScore(), other.anomalyScore())
&& Objects.equals(impact(), other.impact()) && Objects.equals(monitorArn(), other.monitorArn())
&& Objects.equals(feedbackAsString(), other.feedbackAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Anomaly").add("AnomalyId", anomalyId()).add("AnomalyStartDate", anomalyStartDate())
.add("AnomalyEndDate", anomalyEndDate()).add("DimensionValue", dimensionValue())
.add("RootCauses", hasRootCauses() ? rootCauses() : null).add("AnomalyScore", anomalyScore())
.add("Impact", impact()).add("MonitorArn", monitorArn()).add("Feedback", feedbackAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AnomalyId":
return Optional.ofNullable(clazz.cast(anomalyId()));
case "AnomalyStartDate":
return Optional.ofNullable(clazz.cast(anomalyStartDate()));
case "AnomalyEndDate":
return Optional.ofNullable(clazz.cast(anomalyEndDate()));
case "DimensionValue":
return Optional.ofNullable(clazz.cast(dimensionValue()));
case "RootCauses":
return Optional.ofNullable(clazz.cast(rootCauses()));
case "AnomalyScore":
return Optional.ofNullable(clazz.cast(anomalyScore()));
case "Impact":
return Optional.ofNullable(clazz.cast(impact()));
case "MonitorArn":
return Optional.ofNullable(clazz.cast(monitorArn()));
case "Feedback":
return Optional.ofNullable(clazz.cast(feedbackAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function