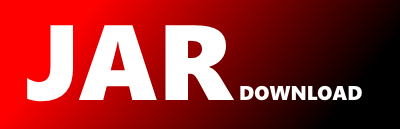
software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansPurchaseRecommendationRequest Maven / Gradle / Ivy
Show all versions of costexplorer Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.costexplorer.model;
import java.beans.Transient;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetSavingsPlansPurchaseRecommendationRequest extends CostExplorerRequest implements
ToCopyableBuilder {
private static final SdkField SAVINGS_PLANS_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SavingsPlansType")
.getter(getter(GetSavingsPlansPurchaseRecommendationRequest::savingsPlansTypeAsString))
.setter(setter(Builder::savingsPlansType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SavingsPlansType").build()).build();
private static final SdkField TERM_IN_YEARS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TermInYears").getter(getter(GetSavingsPlansPurchaseRecommendationRequest::termInYearsAsString))
.setter(setter(Builder::termInYears))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TermInYears").build()).build();
private static final SdkField PAYMENT_OPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PaymentOption").getter(getter(GetSavingsPlansPurchaseRecommendationRequest::paymentOptionAsString))
.setter(setter(Builder::paymentOption))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PaymentOption").build()).build();
private static final SdkField ACCOUNT_SCOPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AccountScope").getter(getter(GetSavingsPlansPurchaseRecommendationRequest::accountScopeAsString))
.setter(setter(Builder::accountScope))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AccountScope").build()).build();
private static final SdkField NEXT_PAGE_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NextPageToken").getter(getter(GetSavingsPlansPurchaseRecommendationRequest::nextPageToken))
.setter(setter(Builder::nextPageToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NextPageToken").build()).build();
private static final SdkField PAGE_SIZE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("PageSize").getter(getter(GetSavingsPlansPurchaseRecommendationRequest::pageSize))
.setter(setter(Builder::pageSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PageSize").build()).build();
private static final SdkField LOOKBACK_PERIOD_IN_DAYS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LookbackPeriodInDays")
.getter(getter(GetSavingsPlansPurchaseRecommendationRequest::lookbackPeriodInDaysAsString))
.setter(setter(Builder::lookbackPeriodInDays))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LookbackPeriodInDays").build())
.build();
private static final SdkField FILTER_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Filter").getter(getter(GetSavingsPlansPurchaseRecommendationRequest::filter))
.setter(setter(Builder::filter)).constructor(Expression::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Filter").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SAVINGS_PLANS_TYPE_FIELD,
TERM_IN_YEARS_FIELD, PAYMENT_OPTION_FIELD, ACCOUNT_SCOPE_FIELD, NEXT_PAGE_TOKEN_FIELD, PAGE_SIZE_FIELD,
LOOKBACK_PERIOD_IN_DAYS_FIELD, FILTER_FIELD));
private final String savingsPlansType;
private final String termInYears;
private final String paymentOption;
private final String accountScope;
private final String nextPageToken;
private final Integer pageSize;
private final String lookbackPeriodInDays;
private final Expression filter;
private GetSavingsPlansPurchaseRecommendationRequest(BuilderImpl builder) {
super(builder);
this.savingsPlansType = builder.savingsPlansType;
this.termInYears = builder.termInYears;
this.paymentOption = builder.paymentOption;
this.accountScope = builder.accountScope;
this.nextPageToken = builder.nextPageToken;
this.pageSize = builder.pageSize;
this.lookbackPeriodInDays = builder.lookbackPeriodInDays;
this.filter = builder.filter;
}
/**
*
* The Savings Plans recommendation type requested.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #savingsPlansType}
* will return {@link SupportedSavingsPlansType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #savingsPlansTypeAsString}.
*
*
* @return The Savings Plans recommendation type requested.
* @see SupportedSavingsPlansType
*/
public final SupportedSavingsPlansType savingsPlansType() {
return SupportedSavingsPlansType.fromValue(savingsPlansType);
}
/**
*
* The Savings Plans recommendation type requested.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #savingsPlansType}
* will return {@link SupportedSavingsPlansType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #savingsPlansTypeAsString}.
*
*
* @return The Savings Plans recommendation type requested.
* @see SupportedSavingsPlansType
*/
public final String savingsPlansTypeAsString() {
return savingsPlansType;
}
/**
*
* The savings plan recommendation term used to generate these recommendations.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #termInYears} will
* return {@link TermInYears#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #termInYearsAsString}.
*
*
* @return The savings plan recommendation term used to generate these recommendations.
* @see TermInYears
*/
public final TermInYears termInYears() {
return TermInYears.fromValue(termInYears);
}
/**
*
* The savings plan recommendation term used to generate these recommendations.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #termInYears} will
* return {@link TermInYears#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #termInYearsAsString}.
*
*
* @return The savings plan recommendation term used to generate these recommendations.
* @see TermInYears
*/
public final String termInYearsAsString() {
return termInYears;
}
/**
*
* The payment option used to generate these recommendations.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #paymentOption}
* will return {@link PaymentOption#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #paymentOptionAsString}.
*
*
* @return The payment option used to generate these recommendations.
* @see PaymentOption
*/
public final PaymentOption paymentOption() {
return PaymentOption.fromValue(paymentOption);
}
/**
*
* The payment option used to generate these recommendations.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #paymentOption}
* will return {@link PaymentOption#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #paymentOptionAsString}.
*
*
* @return The payment option used to generate these recommendations.
* @see PaymentOption
*/
public final String paymentOptionAsString() {
return paymentOption;
}
/**
*
* The account scope that you want your recommendations for. Amazon Web Services calculates recommendations
* including the management account and member accounts if the value is set to PAYER
. If the value is
* LINKED
, recommendations are calculated for individual member accounts only.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #accountScope} will
* return {@link AccountScope#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #accountScopeAsString}.
*
*
* @return The account scope that you want your recommendations for. Amazon Web Services calculates recommendations
* including the management account and member accounts if the value is set to PAYER
. If the
* value is LINKED
, recommendations are calculated for individual member accounts only.
* @see AccountScope
*/
public final AccountScope accountScope() {
return AccountScope.fromValue(accountScope);
}
/**
*
* The account scope that you want your recommendations for. Amazon Web Services calculates recommendations
* including the management account and member accounts if the value is set to PAYER
. If the value is
* LINKED
, recommendations are calculated for individual member accounts only.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #accountScope} will
* return {@link AccountScope#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #accountScopeAsString}.
*
*
* @return The account scope that you want your recommendations for. Amazon Web Services calculates recommendations
* including the management account and member accounts if the value is set to PAYER
. If the
* value is LINKED
, recommendations are calculated for individual member accounts only.
* @see AccountScope
*/
public final String accountScopeAsString() {
return accountScope;
}
/**
*
* The token to retrieve the next set of results. Amazon Web Services provides the token when the response from a
* previous call has more results than the maximum page size.
*
*
* @return The token to retrieve the next set of results. Amazon Web Services provides the token when the response
* from a previous call has more results than the maximum page size.
*/
public final String nextPageToken() {
return nextPageToken;
}
/**
*
* The number of recommendations that you want returned in a single response object.
*
*
* @return The number of recommendations that you want returned in a single response object.
*/
public final Integer pageSize() {
return pageSize;
}
/**
*
* The lookback period used to generate the recommendation.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #lookbackPeriodInDays} will return {@link LookbackPeriodInDays#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #lookbackPeriodInDaysAsString}.
*
*
* @return The lookback period used to generate the recommendation.
* @see LookbackPeriodInDays
*/
public final LookbackPeriodInDays lookbackPeriodInDays() {
return LookbackPeriodInDays.fromValue(lookbackPeriodInDays);
}
/**
*
* The lookback period used to generate the recommendation.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #lookbackPeriodInDays} will return {@link LookbackPeriodInDays#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #lookbackPeriodInDaysAsString}.
*
*
* @return The lookback period used to generate the recommendation.
* @see LookbackPeriodInDays
*/
public final String lookbackPeriodInDaysAsString() {
return lookbackPeriodInDays;
}
/**
*
* You can filter your recommendations by Account ID with the LINKED_ACCOUNT
dimension. To filter your
* recommendations by Account ID, specify Key
as LINKED_ACCOUNT
and Value
as
* the comma-separated Acount ID(s) for which you want to see Savings Plans purchase recommendations.
*
*
* For GetSavingsPlansPurchaseRecommendation, the Filter
does not include CostCategories
* or Tags
. It only includes Dimensions
. With Dimensions
, Key
* must be LINKED_ACCOUNT
and Value
can be a single Account ID or multiple comma-separated
* Account IDs for which you want to see Savings Plans Purchase Recommendations. AND
and
* OR
operators are not supported.
*
*
* @return You can filter your recommendations by Account ID with the LINKED_ACCOUNT
dimension. To
* filter your recommendations by Account ID, specify Key
as LINKED_ACCOUNT
and
* Value
as the comma-separated Acount ID(s) for which you want to see Savings Plans purchase
* recommendations.
*
* For GetSavingsPlansPurchaseRecommendation, the Filter
does not include
* CostCategories
or Tags
. It only includes Dimensions
. With
* Dimensions
, Key
must be LINKED_ACCOUNT
and Value
can
* be a single Account ID or multiple comma-separated Account IDs for which you want to see Savings Plans
* Purchase Recommendations. AND
and OR
operators are not supported.
*/
public final Expression filter() {
return filter;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(savingsPlansTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(termInYearsAsString());
hashCode = 31 * hashCode + Objects.hashCode(paymentOptionAsString());
hashCode = 31 * hashCode + Objects.hashCode(accountScopeAsString());
hashCode = 31 * hashCode + Objects.hashCode(nextPageToken());
hashCode = 31 * hashCode + Objects.hashCode(pageSize());
hashCode = 31 * hashCode + Objects.hashCode(lookbackPeriodInDaysAsString());
hashCode = 31 * hashCode + Objects.hashCode(filter());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetSavingsPlansPurchaseRecommendationRequest)) {
return false;
}
GetSavingsPlansPurchaseRecommendationRequest other = (GetSavingsPlansPurchaseRecommendationRequest) obj;
return Objects.equals(savingsPlansTypeAsString(), other.savingsPlansTypeAsString())
&& Objects.equals(termInYearsAsString(), other.termInYearsAsString())
&& Objects.equals(paymentOptionAsString(), other.paymentOptionAsString())
&& Objects.equals(accountScopeAsString(), other.accountScopeAsString())
&& Objects.equals(nextPageToken(), other.nextPageToken()) && Objects.equals(pageSize(), other.pageSize())
&& Objects.equals(lookbackPeriodInDaysAsString(), other.lookbackPeriodInDaysAsString())
&& Objects.equals(filter(), other.filter());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetSavingsPlansPurchaseRecommendationRequest")
.add("SavingsPlansType", savingsPlansTypeAsString()).add("TermInYears", termInYearsAsString())
.add("PaymentOption", paymentOptionAsString()).add("AccountScope", accountScopeAsString())
.add("NextPageToken", nextPageToken()).add("PageSize", pageSize())
.add("LookbackPeriodInDays", lookbackPeriodInDaysAsString()).add("Filter", filter()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "SavingsPlansType":
return Optional.ofNullable(clazz.cast(savingsPlansTypeAsString()));
case "TermInYears":
return Optional.ofNullable(clazz.cast(termInYearsAsString()));
case "PaymentOption":
return Optional.ofNullable(clazz.cast(paymentOptionAsString()));
case "AccountScope":
return Optional.ofNullable(clazz.cast(accountScopeAsString()));
case "NextPageToken":
return Optional.ofNullable(clazz.cast(nextPageToken()));
case "PageSize":
return Optional.ofNullable(clazz.cast(pageSize()));
case "LookbackPeriodInDays":
return Optional.ofNullable(clazz.cast(lookbackPeriodInDaysAsString()));
case "Filter":
return Optional.ofNullable(clazz.cast(filter()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* For GetSavingsPlansPurchaseRecommendation, the Filter
does not include
* CostCategories
or Tags
. It only includes Dimensions
. With
* Dimensions
, Key
must be LINKED_ACCOUNT
and Value
* can be a single Account ID or multiple comma-separated Account IDs for which you want to see Savings
* Plans Purchase Recommendations. AND
and OR
operators are not supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder filter(Expression filter);
/**
*
* You can filter your recommendations by Account ID with the LINKED_ACCOUNT
dimension. To filter
* your recommendations by Account ID, specify Key
as LINKED_ACCOUNT
and
* Value
as the comma-separated Acount ID(s) for which you want to see Savings Plans purchase
* recommendations.
*
*
* For GetSavingsPlansPurchaseRecommendation, the Filter
does not include
* CostCategories
or Tags
. It only includes Dimensions
. With
* Dimensions
, Key
must be LINKED_ACCOUNT
and Value
can be a
* single Account ID or multiple comma-separated Account IDs for which you want to see Savings Plans Purchase
* Recommendations. AND
and OR
operators are not supported.
*
* This is a convenience that creates an instance of the {@link Expression.Builder} avoiding the need to create
* one manually via {@link Expression#builder()}.
*
* When the {@link Consumer} completes, {@link Expression.Builder#build()} is called immediately and its result
* is passed to {@link #filter(Expression)}.
*
* @param filter
* a consumer that will call methods on {@link Expression.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #filter(Expression)
*/
default Builder filter(Consumer filter) {
return filter(Expression.builder().applyMutation(filter).build());
}
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends CostExplorerRequest.BuilderImpl implements Builder {
private String savingsPlansType;
private String termInYears;
private String paymentOption;
private String accountScope;
private String nextPageToken;
private Integer pageSize;
private String lookbackPeriodInDays;
private Expression filter;
private BuilderImpl() {
}
private BuilderImpl(GetSavingsPlansPurchaseRecommendationRequest model) {
super(model);
savingsPlansType(model.savingsPlansType);
termInYears(model.termInYears);
paymentOption(model.paymentOption);
accountScope(model.accountScope);
nextPageToken(model.nextPageToken);
pageSize(model.pageSize);
lookbackPeriodInDays(model.lookbackPeriodInDays);
filter(model.filter);
}
public final String getSavingsPlansType() {
return savingsPlansType;
}
public final void setSavingsPlansType(String savingsPlansType) {
this.savingsPlansType = savingsPlansType;
}
@Override
@Transient
public final Builder savingsPlansType(String savingsPlansType) {
this.savingsPlansType = savingsPlansType;
return this;
}
@Override
@Transient
public final Builder savingsPlansType(SupportedSavingsPlansType savingsPlansType) {
this.savingsPlansType(savingsPlansType == null ? null : savingsPlansType.toString());
return this;
}
public final String getTermInYears() {
return termInYears;
}
public final void setTermInYears(String termInYears) {
this.termInYears = termInYears;
}
@Override
@Transient
public final Builder termInYears(String termInYears) {
this.termInYears = termInYears;
return this;
}
@Override
@Transient
public final Builder termInYears(TermInYears termInYears) {
this.termInYears(termInYears == null ? null : termInYears.toString());
return this;
}
public final String getPaymentOption() {
return paymentOption;
}
public final void setPaymentOption(String paymentOption) {
this.paymentOption = paymentOption;
}
@Override
@Transient
public final Builder paymentOption(String paymentOption) {
this.paymentOption = paymentOption;
return this;
}
@Override
@Transient
public final Builder paymentOption(PaymentOption paymentOption) {
this.paymentOption(paymentOption == null ? null : paymentOption.toString());
return this;
}
public final String getAccountScope() {
return accountScope;
}
public final void setAccountScope(String accountScope) {
this.accountScope = accountScope;
}
@Override
@Transient
public final Builder accountScope(String accountScope) {
this.accountScope = accountScope;
return this;
}
@Override
@Transient
public final Builder accountScope(AccountScope accountScope) {
this.accountScope(accountScope == null ? null : accountScope.toString());
return this;
}
public final String getNextPageToken() {
return nextPageToken;
}
public final void setNextPageToken(String nextPageToken) {
this.nextPageToken = nextPageToken;
}
@Override
@Transient
public final Builder nextPageToken(String nextPageToken) {
this.nextPageToken = nextPageToken;
return this;
}
public final Integer getPageSize() {
return pageSize;
}
public final void setPageSize(Integer pageSize) {
this.pageSize = pageSize;
}
@Override
@Transient
public final Builder pageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
public final String getLookbackPeriodInDays() {
return lookbackPeriodInDays;
}
public final void setLookbackPeriodInDays(String lookbackPeriodInDays) {
this.lookbackPeriodInDays = lookbackPeriodInDays;
}
@Override
@Transient
public final Builder lookbackPeriodInDays(String lookbackPeriodInDays) {
this.lookbackPeriodInDays = lookbackPeriodInDays;
return this;
}
@Override
@Transient
public final Builder lookbackPeriodInDays(LookbackPeriodInDays lookbackPeriodInDays) {
this.lookbackPeriodInDays(lookbackPeriodInDays == null ? null : lookbackPeriodInDays.toString());
return this;
}
public final Expression.Builder getFilter() {
return filter != null ? filter.toBuilder() : null;
}
public final void setFilter(Expression.BuilderImpl filter) {
this.filter = filter != null ? filter.build() : null;
}
@Override
@Transient
public final Builder filter(Expression filter) {
this.filter = filter;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public GetSavingsPlansPurchaseRecommendationRequest build() {
return new GetSavingsPlansPurchaseRecommendationRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}