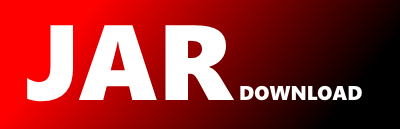
software.amazon.awssdk.services.costexplorer.model.EC2ResourceDetails Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.costexplorer.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Details on the Amazon EC2 Resource.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class EC2ResourceDetails implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField HOURLY_ON_DEMAND_RATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HourlyOnDemandRate").getter(getter(EC2ResourceDetails::hourlyOnDemandRate))
.setter(setter(Builder::hourlyOnDemandRate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HourlyOnDemandRate").build())
.build();
private static final SdkField INSTANCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InstanceType").getter(getter(EC2ResourceDetails::instanceType)).setter(setter(Builder::instanceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceType").build()).build();
private static final SdkField PLATFORM_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Platform").getter(getter(EC2ResourceDetails::platform)).setter(setter(Builder::platform))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Platform").build()).build();
private static final SdkField REGION_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Region")
.getter(getter(EC2ResourceDetails::region)).setter(setter(Builder::region))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Region").build()).build();
private static final SdkField SKU_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Sku")
.getter(getter(EC2ResourceDetails::sku)).setter(setter(Builder::sku))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Sku").build()).build();
private static final SdkField MEMORY_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Memory")
.getter(getter(EC2ResourceDetails::memory)).setter(setter(Builder::memory))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Memory").build()).build();
private static final SdkField NETWORK_PERFORMANCE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NetworkPerformance").getter(getter(EC2ResourceDetails::networkPerformance))
.setter(setter(Builder::networkPerformance))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NetworkPerformance").build())
.build();
private static final SdkField STORAGE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Storage")
.getter(getter(EC2ResourceDetails::storage)).setter(setter(Builder::storage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Storage").build()).build();
private static final SdkField VCPU_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Vcpu")
.getter(getter(EC2ResourceDetails::vcpu)).setter(setter(Builder::vcpu))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Vcpu").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(HOURLY_ON_DEMAND_RATE_FIELD,
INSTANCE_TYPE_FIELD, PLATFORM_FIELD, REGION_FIELD, SKU_FIELD, MEMORY_FIELD, NETWORK_PERFORMANCE_FIELD, STORAGE_FIELD,
VCPU_FIELD));
private static final long serialVersionUID = 1L;
private final String hourlyOnDemandRate;
private final String instanceType;
private final String platform;
private final String region;
private final String sku;
private final String memory;
private final String networkPerformance;
private final String storage;
private final String vcpu;
private EC2ResourceDetails(BuilderImpl builder) {
this.hourlyOnDemandRate = builder.hourlyOnDemandRate;
this.instanceType = builder.instanceType;
this.platform = builder.platform;
this.region = builder.region;
this.sku = builder.sku;
this.memory = builder.memory;
this.networkPerformance = builder.networkPerformance;
this.storage = builder.storage;
this.vcpu = builder.vcpu;
}
/**
*
* The hourly public On-Demand rate for the instance type.
*
*
* @return The hourly public On-Demand rate for the instance type.
*/
public final String hourlyOnDemandRate() {
return hourlyOnDemandRate;
}
/**
*
* The type of Amazon Web Services instance.
*
*
* @return The type of Amazon Web Services instance.
*/
public final String instanceType() {
return instanceType;
}
/**
*
* The platform of the Amazon Web Services instance. The platform is the specific combination of operating system,
* license model, and software on an instance.
*
*
* @return The platform of the Amazon Web Services instance. The platform is the specific combination of operating
* system, license model, and software on an instance.
*/
public final String platform() {
return platform;
}
/**
*
* The Amazon Web Services Region of the instance.
*
*
* @return The Amazon Web Services Region of the instance.
*/
public final String region() {
return region;
}
/**
*
* The SKU of the product.
*
*
* @return The SKU of the product.
*/
public final String sku() {
return sku;
}
/**
*
* The memory capacity of the Amazon Web Services instance.
*
*
* @return The memory capacity of the Amazon Web Services instance.
*/
public final String memory() {
return memory;
}
/**
*
* The network performance capacity of the Amazon Web Services instance.
*
*
* @return The network performance capacity of the Amazon Web Services instance.
*/
public final String networkPerformance() {
return networkPerformance;
}
/**
*
* The disk storage of the Amazon Web Services instance. This doesn't include EBS storage.
*
*
* @return The disk storage of the Amazon Web Services instance. This doesn't include EBS storage.
*/
public final String storage() {
return storage;
}
/**
*
* The number of VCPU cores in the Amazon Web Services instance type.
*
*
* @return The number of VCPU cores in the Amazon Web Services instance type.
*/
public final String vcpu() {
return vcpu;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hourlyOnDemandRate());
hashCode = 31 * hashCode + Objects.hashCode(instanceType());
hashCode = 31 * hashCode + Objects.hashCode(platform());
hashCode = 31 * hashCode + Objects.hashCode(region());
hashCode = 31 * hashCode + Objects.hashCode(sku());
hashCode = 31 * hashCode + Objects.hashCode(memory());
hashCode = 31 * hashCode + Objects.hashCode(networkPerformance());
hashCode = 31 * hashCode + Objects.hashCode(storage());
hashCode = 31 * hashCode + Objects.hashCode(vcpu());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof EC2ResourceDetails)) {
return false;
}
EC2ResourceDetails other = (EC2ResourceDetails) obj;
return Objects.equals(hourlyOnDemandRate(), other.hourlyOnDemandRate())
&& Objects.equals(instanceType(), other.instanceType()) && Objects.equals(platform(), other.platform())
&& Objects.equals(region(), other.region()) && Objects.equals(sku(), other.sku())
&& Objects.equals(memory(), other.memory()) && Objects.equals(networkPerformance(), other.networkPerformance())
&& Objects.equals(storage(), other.storage()) && Objects.equals(vcpu(), other.vcpu());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("EC2ResourceDetails").add("HourlyOnDemandRate", hourlyOnDemandRate())
.add("InstanceType", instanceType()).add("Platform", platform()).add("Region", region()).add("Sku", sku())
.add("Memory", memory()).add("NetworkPerformance", networkPerformance()).add("Storage", storage())
.add("Vcpu", vcpu()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "HourlyOnDemandRate":
return Optional.ofNullable(clazz.cast(hourlyOnDemandRate()));
case "InstanceType":
return Optional.ofNullable(clazz.cast(instanceType()));
case "Platform":
return Optional.ofNullable(clazz.cast(platform()));
case "Region":
return Optional.ofNullable(clazz.cast(region()));
case "Sku":
return Optional.ofNullable(clazz.cast(sku()));
case "Memory":
return Optional.ofNullable(clazz.cast(memory()));
case "NetworkPerformance":
return Optional.ofNullable(clazz.cast(networkPerformance()));
case "Storage":
return Optional.ofNullable(clazz.cast(storage()));
case "Vcpu":
return Optional.ofNullable(clazz.cast(vcpu()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function