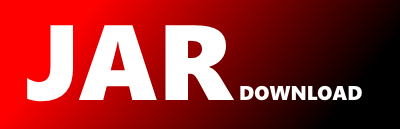
software.amazon.awssdk.services.costexplorer.CostExplorerAsyncClient Maven / Gradle / Ivy
Show all versions of costexplorer Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.costexplorer;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.services.costexplorer.model.CreateAnomalyMonitorRequest;
import software.amazon.awssdk.services.costexplorer.model.CreateAnomalyMonitorResponse;
import software.amazon.awssdk.services.costexplorer.model.CreateAnomalySubscriptionRequest;
import software.amazon.awssdk.services.costexplorer.model.CreateAnomalySubscriptionResponse;
import software.amazon.awssdk.services.costexplorer.model.CreateCostCategoryDefinitionRequest;
import software.amazon.awssdk.services.costexplorer.model.CreateCostCategoryDefinitionResponse;
import software.amazon.awssdk.services.costexplorer.model.DeleteAnomalyMonitorRequest;
import software.amazon.awssdk.services.costexplorer.model.DeleteAnomalyMonitorResponse;
import software.amazon.awssdk.services.costexplorer.model.DeleteAnomalySubscriptionRequest;
import software.amazon.awssdk.services.costexplorer.model.DeleteAnomalySubscriptionResponse;
import software.amazon.awssdk.services.costexplorer.model.DeleteCostCategoryDefinitionRequest;
import software.amazon.awssdk.services.costexplorer.model.DeleteCostCategoryDefinitionResponse;
import software.amazon.awssdk.services.costexplorer.model.DescribeCostCategoryDefinitionRequest;
import software.amazon.awssdk.services.costexplorer.model.DescribeCostCategoryDefinitionResponse;
import software.amazon.awssdk.services.costexplorer.model.GetAnomaliesRequest;
import software.amazon.awssdk.services.costexplorer.model.GetAnomaliesResponse;
import software.amazon.awssdk.services.costexplorer.model.GetAnomalyMonitorsRequest;
import software.amazon.awssdk.services.costexplorer.model.GetAnomalyMonitorsResponse;
import software.amazon.awssdk.services.costexplorer.model.GetAnomalySubscriptionsRequest;
import software.amazon.awssdk.services.costexplorer.model.GetAnomalySubscriptionsResponse;
import software.amazon.awssdk.services.costexplorer.model.GetCostAndUsageRequest;
import software.amazon.awssdk.services.costexplorer.model.GetCostAndUsageResponse;
import software.amazon.awssdk.services.costexplorer.model.GetCostAndUsageWithResourcesRequest;
import software.amazon.awssdk.services.costexplorer.model.GetCostAndUsageWithResourcesResponse;
import software.amazon.awssdk.services.costexplorer.model.GetCostCategoriesRequest;
import software.amazon.awssdk.services.costexplorer.model.GetCostCategoriesResponse;
import software.amazon.awssdk.services.costexplorer.model.GetCostForecastRequest;
import software.amazon.awssdk.services.costexplorer.model.GetCostForecastResponse;
import software.amazon.awssdk.services.costexplorer.model.GetDimensionValuesRequest;
import software.amazon.awssdk.services.costexplorer.model.GetDimensionValuesResponse;
import software.amazon.awssdk.services.costexplorer.model.GetReservationCoverageRequest;
import software.amazon.awssdk.services.costexplorer.model.GetReservationCoverageResponse;
import software.amazon.awssdk.services.costexplorer.model.GetReservationPurchaseRecommendationRequest;
import software.amazon.awssdk.services.costexplorer.model.GetReservationPurchaseRecommendationResponse;
import software.amazon.awssdk.services.costexplorer.model.GetReservationUtilizationRequest;
import software.amazon.awssdk.services.costexplorer.model.GetReservationUtilizationResponse;
import software.amazon.awssdk.services.costexplorer.model.GetRightsizingRecommendationRequest;
import software.amazon.awssdk.services.costexplorer.model.GetRightsizingRecommendationResponse;
import software.amazon.awssdk.services.costexplorer.model.GetSavingsPlanPurchaseRecommendationDetailsRequest;
import software.amazon.awssdk.services.costexplorer.model.GetSavingsPlanPurchaseRecommendationDetailsResponse;
import software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansCoverageRequest;
import software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansCoverageResponse;
import software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansPurchaseRecommendationRequest;
import software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansPurchaseRecommendationResponse;
import software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansUtilizationDetailsRequest;
import software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansUtilizationDetailsResponse;
import software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansUtilizationRequest;
import software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansUtilizationResponse;
import software.amazon.awssdk.services.costexplorer.model.GetTagsRequest;
import software.amazon.awssdk.services.costexplorer.model.GetTagsResponse;
import software.amazon.awssdk.services.costexplorer.model.GetUsageForecastRequest;
import software.amazon.awssdk.services.costexplorer.model.GetUsageForecastResponse;
import software.amazon.awssdk.services.costexplorer.model.ListCostAllocationTagsRequest;
import software.amazon.awssdk.services.costexplorer.model.ListCostAllocationTagsResponse;
import software.amazon.awssdk.services.costexplorer.model.ListCostCategoryDefinitionsRequest;
import software.amazon.awssdk.services.costexplorer.model.ListCostCategoryDefinitionsResponse;
import software.amazon.awssdk.services.costexplorer.model.ListSavingsPlansPurchaseRecommendationGenerationRequest;
import software.amazon.awssdk.services.costexplorer.model.ListSavingsPlansPurchaseRecommendationGenerationResponse;
import software.amazon.awssdk.services.costexplorer.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.costexplorer.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.costexplorer.model.ProvideAnomalyFeedbackRequest;
import software.amazon.awssdk.services.costexplorer.model.ProvideAnomalyFeedbackResponse;
import software.amazon.awssdk.services.costexplorer.model.StartSavingsPlansPurchaseRecommendationGenerationRequest;
import software.amazon.awssdk.services.costexplorer.model.StartSavingsPlansPurchaseRecommendationGenerationResponse;
import software.amazon.awssdk.services.costexplorer.model.TagResourceRequest;
import software.amazon.awssdk.services.costexplorer.model.TagResourceResponse;
import software.amazon.awssdk.services.costexplorer.model.UntagResourceRequest;
import software.amazon.awssdk.services.costexplorer.model.UntagResourceResponse;
import software.amazon.awssdk.services.costexplorer.model.UpdateAnomalyMonitorRequest;
import software.amazon.awssdk.services.costexplorer.model.UpdateAnomalyMonitorResponse;
import software.amazon.awssdk.services.costexplorer.model.UpdateAnomalySubscriptionRequest;
import software.amazon.awssdk.services.costexplorer.model.UpdateAnomalySubscriptionResponse;
import software.amazon.awssdk.services.costexplorer.model.UpdateCostAllocationTagsStatusRequest;
import software.amazon.awssdk.services.costexplorer.model.UpdateCostAllocationTagsStatusResponse;
import software.amazon.awssdk.services.costexplorer.model.UpdateCostCategoryDefinitionRequest;
import software.amazon.awssdk.services.costexplorer.model.UpdateCostCategoryDefinitionResponse;
import software.amazon.awssdk.services.costexplorer.paginators.GetSavingsPlansCoveragePublisher;
import software.amazon.awssdk.services.costexplorer.paginators.GetSavingsPlansUtilizationDetailsPublisher;
import software.amazon.awssdk.services.costexplorer.paginators.ListCostAllocationTagsPublisher;
import software.amazon.awssdk.services.costexplorer.paginators.ListCostCategoryDefinitionsPublisher;
/**
* Service client for accessing AWS Cost Explorer asynchronously. This can be created using the static
* {@link #builder()} method.
*
*
* You can use the Cost Explorer API to programmatically query your cost and usage data. You can query for aggregated
* data such as total monthly costs or total daily usage. You can also query for granular data. This might include the
* number of daily write operations for Amazon DynamoDB database tables in your production environment.
*
*
* Service Endpoint
*
*
* The Cost Explorer API provides the following endpoint:
*
*
* -
*
* https://ce.us-east-1.amazonaws.com
*
*
*
*
* For information about the costs that are associated with the Cost Explorer API, see Amazon Web Services Cost Management Pricing.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface CostExplorerAsyncClient extends AwsClient {
String SERVICE_NAME = "ce";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "ce";
/**
*
* Creates a new cost anomaly detection monitor with the requested type and monitor specification.
*
*
* @param createAnomalyMonitorRequest
* @return A Java Future containing the result of the CreateAnomalyMonitor operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.CreateAnomalyMonitor
* @see AWS API
* Documentation
*/
default CompletableFuture createAnomalyMonitor(
CreateAnomalyMonitorRequest createAnomalyMonitorRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new cost anomaly detection monitor with the requested type and monitor specification.
*
*
*
* This is a convenience which creates an instance of the {@link CreateAnomalyMonitorRequest.Builder} avoiding the
* need to create one manually via {@link CreateAnomalyMonitorRequest#builder()}
*
*
* @param createAnomalyMonitorRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.CreateAnomalyMonitorRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateAnomalyMonitor operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.CreateAnomalyMonitor
* @see AWS API
* Documentation
*/
default CompletableFuture createAnomalyMonitor(
Consumer createAnomalyMonitorRequest) {
return createAnomalyMonitor(CreateAnomalyMonitorRequest.builder().applyMutation(createAnomalyMonitorRequest).build());
}
/**
*
* Adds an alert subscription to a cost anomaly detection monitor. You can use each subscription to define
* subscribers with email or SNS notifications. Email subscribers can set an absolute or percentage threshold and a
* time frequency for receiving notifications.
*
*
* @param createAnomalySubscriptionRequest
* @return A Java Future containing the result of the CreateAnomalySubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnknownMonitorException The cost anomaly monitor does not exist for the account.
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.CreateAnomalySubscription
* @see AWS
* API Documentation
*/
default CompletableFuture createAnomalySubscription(
CreateAnomalySubscriptionRequest createAnomalySubscriptionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds an alert subscription to a cost anomaly detection monitor. You can use each subscription to define
* subscribers with email or SNS notifications. Email subscribers can set an absolute or percentage threshold and a
* time frequency for receiving notifications.
*
*
*
* This is a convenience which creates an instance of the {@link CreateAnomalySubscriptionRequest.Builder} avoiding
* the need to create one manually via {@link CreateAnomalySubscriptionRequest#builder()}
*
*
* @param createAnomalySubscriptionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.CreateAnomalySubscriptionRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the CreateAnomalySubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnknownMonitorException The cost anomaly monitor does not exist for the account.
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.CreateAnomalySubscription
* @see AWS
* API Documentation
*/
default CompletableFuture createAnomalySubscription(
Consumer createAnomalySubscriptionRequest) {
return createAnomalySubscription(CreateAnomalySubscriptionRequest.builder()
.applyMutation(createAnomalySubscriptionRequest).build());
}
/**
*
* Creates a new Cost Category with the requested name and rules.
*
*
* @param createCostCategoryDefinitionRequest
* @return A Java Future containing the result of the CreateCostCategoryDefinition operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceQuotaExceededException You've reached the limit on the number of resources you can create, or
* exceeded the size of an individual resource.
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.CreateCostCategoryDefinition
* @see AWS API Documentation
*/
default CompletableFuture createCostCategoryDefinition(
CreateCostCategoryDefinitionRequest createCostCategoryDefinitionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new Cost Category with the requested name and rules.
*
*
*
* This is a convenience which creates an instance of the {@link CreateCostCategoryDefinitionRequest.Builder}
* avoiding the need to create one manually via {@link CreateCostCategoryDefinitionRequest#builder()}
*
*
* @param createCostCategoryDefinitionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.CreateCostCategoryDefinitionRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the CreateCostCategoryDefinition operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceQuotaExceededException You've reached the limit on the number of resources you can create, or
* exceeded the size of an individual resource.
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.CreateCostCategoryDefinition
* @see AWS API Documentation
*/
default CompletableFuture createCostCategoryDefinition(
Consumer createCostCategoryDefinitionRequest) {
return createCostCategoryDefinition(CreateCostCategoryDefinitionRequest.builder()
.applyMutation(createCostCategoryDefinitionRequest).build());
}
/**
*
* Deletes a cost anomaly monitor.
*
*
* @param deleteAnomalyMonitorRequest
* @return A Java Future containing the result of the DeleteAnomalyMonitor operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - UnknownMonitorException The cost anomaly monitor does not exist for the account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.DeleteAnomalyMonitor
* @see AWS API
* Documentation
*/
default CompletableFuture deleteAnomalyMonitor(
DeleteAnomalyMonitorRequest deleteAnomalyMonitorRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a cost anomaly monitor.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAnomalyMonitorRequest.Builder} avoiding the
* need to create one manually via {@link DeleteAnomalyMonitorRequest#builder()}
*
*
* @param deleteAnomalyMonitorRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.DeleteAnomalyMonitorRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteAnomalyMonitor operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - UnknownMonitorException The cost anomaly monitor does not exist for the account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.DeleteAnomalyMonitor
* @see AWS API
* Documentation
*/
default CompletableFuture deleteAnomalyMonitor(
Consumer deleteAnomalyMonitorRequest) {
return deleteAnomalyMonitor(DeleteAnomalyMonitorRequest.builder().applyMutation(deleteAnomalyMonitorRequest).build());
}
/**
*
* Deletes a cost anomaly subscription.
*
*
* @param deleteAnomalySubscriptionRequest
* @return A Java Future containing the result of the DeleteAnomalySubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - UnknownSubscriptionException The cost anomaly subscription does not exist for the account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.DeleteAnomalySubscription
* @see AWS
* API Documentation
*/
default CompletableFuture deleteAnomalySubscription(
DeleteAnomalySubscriptionRequest deleteAnomalySubscriptionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a cost anomaly subscription.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAnomalySubscriptionRequest.Builder} avoiding
* the need to create one manually via {@link DeleteAnomalySubscriptionRequest#builder()}
*
*
* @param deleteAnomalySubscriptionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.DeleteAnomalySubscriptionRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteAnomalySubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - UnknownSubscriptionException The cost anomaly subscription does not exist for the account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.DeleteAnomalySubscription
* @see AWS
* API Documentation
*/
default CompletableFuture deleteAnomalySubscription(
Consumer deleteAnomalySubscriptionRequest) {
return deleteAnomalySubscription(DeleteAnomalySubscriptionRequest.builder()
.applyMutation(deleteAnomalySubscriptionRequest).build());
}
/**
*
* Deletes a Cost Category. Expenses from this month going forward will no longer be categorized with this Cost
* Category.
*
*
* @param deleteCostCategoryDefinitionRequest
* @return A Java Future containing the result of the DeleteCostCategoryDefinition operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified ARN in the request doesn't exist.
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.DeleteCostCategoryDefinition
* @see AWS API Documentation
*/
default CompletableFuture deleteCostCategoryDefinition(
DeleteCostCategoryDefinitionRequest deleteCostCategoryDefinitionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a Cost Category. Expenses from this month going forward will no longer be categorized with this Cost
* Category.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteCostCategoryDefinitionRequest.Builder}
* avoiding the need to create one manually via {@link DeleteCostCategoryDefinitionRequest#builder()}
*
*
* @param deleteCostCategoryDefinitionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.DeleteCostCategoryDefinitionRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteCostCategoryDefinition operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified ARN in the request doesn't exist.
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.DeleteCostCategoryDefinition
* @see AWS API Documentation
*/
default CompletableFuture deleteCostCategoryDefinition(
Consumer deleteCostCategoryDefinitionRequest) {
return deleteCostCategoryDefinition(DeleteCostCategoryDefinitionRequest.builder()
.applyMutation(deleteCostCategoryDefinitionRequest).build());
}
/**
*
* Returns the name, Amazon Resource Name (ARN), rules, definition, and effective dates of a Cost Category that's
* defined in the account.
*
*
* You have the option to use EffectiveOn
to return a Cost Category that's active on a specific date.
* If there's no EffectiveOn
specified, you see a Cost Category that's effective on the current date.
* If Cost Category is still effective, EffectiveEnd
is omitted in the response.
*
*
* @param describeCostCategoryDefinitionRequest
* @return A Java Future containing the result of the DescribeCostCategoryDefinition operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified ARN in the request doesn't exist.
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.DescribeCostCategoryDefinition
* @see AWS API Documentation
*/
default CompletableFuture describeCostCategoryDefinition(
DescribeCostCategoryDefinitionRequest describeCostCategoryDefinitionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the name, Amazon Resource Name (ARN), rules, definition, and effective dates of a Cost Category that's
* defined in the account.
*
*
* You have the option to use EffectiveOn
to return a Cost Category that's active on a specific date.
* If there's no EffectiveOn
specified, you see a Cost Category that's effective on the current date.
* If Cost Category is still effective, EffectiveEnd
is omitted in the response.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCostCategoryDefinitionRequest.Builder}
* avoiding the need to create one manually via {@link DescribeCostCategoryDefinitionRequest#builder()}
*
*
* @param describeCostCategoryDefinitionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.DescribeCostCategoryDefinitionRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DescribeCostCategoryDefinition operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified ARN in the request doesn't exist.
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.DescribeCostCategoryDefinition
* @see AWS API Documentation
*/
default CompletableFuture describeCostCategoryDefinition(
Consumer describeCostCategoryDefinitionRequest) {
return describeCostCategoryDefinition(DescribeCostCategoryDefinitionRequest.builder()
.applyMutation(describeCostCategoryDefinitionRequest).build());
}
/**
*
* Retrieves all of the cost anomalies detected on your account during the time period that's specified by the
* DateInterval
object. Anomalies are available for up to 90 days.
*
*
* @param getAnomaliesRequest
* @return A Java Future containing the result of the GetAnomalies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetAnomalies
* @see AWS API
* Documentation
*/
default CompletableFuture getAnomalies(GetAnomaliesRequest getAnomaliesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves all of the cost anomalies detected on your account during the time period that's specified by the
* DateInterval
object. Anomalies are available for up to 90 days.
*
*
*
* This is a convenience which creates an instance of the {@link GetAnomaliesRequest.Builder} avoiding the need to
* create one manually via {@link GetAnomaliesRequest#builder()}
*
*
* @param getAnomaliesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetAnomaliesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetAnomalies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetAnomalies
* @see AWS API
* Documentation
*/
default CompletableFuture getAnomalies(Consumer getAnomaliesRequest) {
return getAnomalies(GetAnomaliesRequest.builder().applyMutation(getAnomaliesRequest).build());
}
/**
*
* Retrieves the cost anomaly monitor definitions for your account. You can filter using a list of cost anomaly
* monitor Amazon Resource Names (ARNs).
*
*
* @param getAnomalyMonitorsRequest
* @return A Java Future containing the result of the GetAnomalyMonitors operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - UnknownMonitorException The cost anomaly monitor does not exist for the account.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetAnomalyMonitors
* @see AWS API
* Documentation
*/
default CompletableFuture getAnomalyMonitors(GetAnomalyMonitorsRequest getAnomalyMonitorsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the cost anomaly monitor definitions for your account. You can filter using a list of cost anomaly
* monitor Amazon Resource Names (ARNs).
*
*
*
* This is a convenience which creates an instance of the {@link GetAnomalyMonitorsRequest.Builder} avoiding the
* need to create one manually via {@link GetAnomalyMonitorsRequest#builder()}
*
*
* @param getAnomalyMonitorsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetAnomalyMonitorsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetAnomalyMonitors operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - UnknownMonitorException The cost anomaly monitor does not exist for the account.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetAnomalyMonitors
* @see AWS API
* Documentation
*/
default CompletableFuture getAnomalyMonitors(
Consumer getAnomalyMonitorsRequest) {
return getAnomalyMonitors(GetAnomalyMonitorsRequest.builder().applyMutation(getAnomalyMonitorsRequest).build());
}
/**
*
* Retrieves the cost anomaly subscription objects for your account. You can filter using a list of cost anomaly
* monitor Amazon Resource Names (ARNs).
*
*
* @param getAnomalySubscriptionsRequest
* @return A Java Future containing the result of the GetAnomalySubscriptions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - UnknownSubscriptionException The cost anomaly subscription does not exist for the account.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetAnomalySubscriptions
* @see AWS
* API Documentation
*/
default CompletableFuture getAnomalySubscriptions(
GetAnomalySubscriptionsRequest getAnomalySubscriptionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the cost anomaly subscription objects for your account. You can filter using a list of cost anomaly
* monitor Amazon Resource Names (ARNs).
*
*
*
* This is a convenience which creates an instance of the {@link GetAnomalySubscriptionsRequest.Builder} avoiding
* the need to create one manually via {@link GetAnomalySubscriptionsRequest#builder()}
*
*
* @param getAnomalySubscriptionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetAnomalySubscriptionsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetAnomalySubscriptions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - UnknownSubscriptionException The cost anomaly subscription does not exist for the account.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetAnomalySubscriptions
* @see AWS
* API Documentation
*/
default CompletableFuture getAnomalySubscriptions(
Consumer getAnomalySubscriptionsRequest) {
return getAnomalySubscriptions(GetAnomalySubscriptionsRequest.builder().applyMutation(getAnomalySubscriptionsRequest)
.build());
}
/**
*
* Retrieves cost and usage metrics for your account. You can specify which cost and usage-related metric that you
* want the request to return. For example, you can specify BlendedCosts
or UsageQuantity
.
* You can also filter and group your data by various dimensions, such as SERVICE
or AZ
,
* in a specific time range. For a complete list of valid dimensions, see the GetDimensionValues operation. Management account in an organization in Organizations have access to all
* member accounts.
*
*
* For information about filter limitations, see Quotas and
* restrictions in the Billing and Cost Management User Guide.
*
*
* @param getCostAndUsageRequest
* @return A Java Future containing the result of the GetCostAndUsage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - BillExpirationException The requested report expired. Update the date interval and try again.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - RequestChangedException Your request parameters changed between pages. Try again with the old
* parameters or without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetCostAndUsage
* @see AWS API
* Documentation
*/
default CompletableFuture getCostAndUsage(GetCostAndUsageRequest getCostAndUsageRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves cost and usage metrics for your account. You can specify which cost and usage-related metric that you
* want the request to return. For example, you can specify BlendedCosts
or UsageQuantity
.
* You can also filter and group your data by various dimensions, such as SERVICE
or AZ
,
* in a specific time range. For a complete list of valid dimensions, see the GetDimensionValues operation. Management account in an organization in Organizations have access to all
* member accounts.
*
*
* For information about filter limitations, see Quotas and
* restrictions in the Billing and Cost Management User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetCostAndUsageRequest.Builder} avoiding the need
* to create one manually via {@link GetCostAndUsageRequest#builder()}
*
*
* @param getCostAndUsageRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetCostAndUsageRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetCostAndUsage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - BillExpirationException The requested report expired. Update the date interval and try again.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - RequestChangedException Your request parameters changed between pages. Try again with the old
* parameters or without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetCostAndUsage
* @see AWS API
* Documentation
*/
default CompletableFuture getCostAndUsage(
Consumer getCostAndUsageRequest) {
return getCostAndUsage(GetCostAndUsageRequest.builder().applyMutation(getCostAndUsageRequest).build());
}
/**
*
* Retrieves cost and usage metrics with resources for your account. You can specify which cost and usage-related
* metric, such as BlendedCosts
or UsageQuantity
, that you want the request to return. You
* can also filter and group your data by various dimensions, such as SERVICE
or AZ
, in a
* specific time range. For a complete list of valid dimensions, see the GetDimensionValues operation. Management account in an organization in Organizations have access to all
* member accounts. This API is currently available for the Amazon Elastic Compute Cloud – Compute service only.
*
*
*
* This is an opt-in only feature. You can enable this feature from the Cost Explorer Settings page. For information
* about how to access the Settings page, see Controlling Access for Cost
* Explorer in the Billing and Cost Management User Guide.
*
*
*
* @param getCostAndUsageWithResourcesRequest
* @return A Java Future containing the result of the GetCostAndUsageWithResources operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DataUnavailableException The requested data is unavailable.
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - BillExpirationException The requested report expired. Update the date interval and try again.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - RequestChangedException Your request parameters changed between pages. Try again with the old
* parameters or without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetCostAndUsageWithResources
* @see AWS API Documentation
*/
default CompletableFuture getCostAndUsageWithResources(
GetCostAndUsageWithResourcesRequest getCostAndUsageWithResourcesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves cost and usage metrics with resources for your account. You can specify which cost and usage-related
* metric, such as BlendedCosts
or UsageQuantity
, that you want the request to return. You
* can also filter and group your data by various dimensions, such as SERVICE
or AZ
, in a
* specific time range. For a complete list of valid dimensions, see the GetDimensionValues operation. Management account in an organization in Organizations have access to all
* member accounts. This API is currently available for the Amazon Elastic Compute Cloud – Compute service only.
*
*
*
* This is an opt-in only feature. You can enable this feature from the Cost Explorer Settings page. For information
* about how to access the Settings page, see Controlling Access for Cost
* Explorer in the Billing and Cost Management User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetCostAndUsageWithResourcesRequest.Builder}
* avoiding the need to create one manually via {@link GetCostAndUsageWithResourcesRequest#builder()}
*
*
* @param getCostAndUsageWithResourcesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetCostAndUsageWithResourcesRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetCostAndUsageWithResources operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - DataUnavailableException The requested data is unavailable.
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - BillExpirationException The requested report expired. Update the date interval and try again.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - RequestChangedException Your request parameters changed between pages. Try again with the old
* parameters or without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetCostAndUsageWithResources
* @see AWS API Documentation
*/
default CompletableFuture getCostAndUsageWithResources(
Consumer getCostAndUsageWithResourcesRequest) {
return getCostAndUsageWithResources(GetCostAndUsageWithResourcesRequest.builder()
.applyMutation(getCostAndUsageWithResourcesRequest).build());
}
/**
*
* Retrieves an array of Cost Category names and values incurred cost.
*
*
*
* If some Cost Category names and values are not associated with any cost, they will not be returned by this API.
*
*
*
* @param getCostCategoriesRequest
* @return A Java Future containing the result of the GetCostCategories operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - BillExpirationException The requested report expired. Update the date interval and try again.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - RequestChangedException Your request parameters changed between pages. Try again with the old
* parameters or without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetCostCategories
* @see AWS API
* Documentation
*/
default CompletableFuture getCostCategories(GetCostCategoriesRequest getCostCategoriesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves an array of Cost Category names and values incurred cost.
*
*
*
* If some Cost Category names and values are not associated with any cost, they will not be returned by this API.
*
*
*
* This is a convenience which creates an instance of the {@link GetCostCategoriesRequest.Builder} avoiding the need
* to create one manually via {@link GetCostCategoriesRequest#builder()}
*
*
* @param getCostCategoriesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetCostCategoriesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetCostCategories operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - BillExpirationException The requested report expired. Update the date interval and try again.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - RequestChangedException Your request parameters changed between pages. Try again with the old
* parameters or without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetCostCategories
* @see AWS API
* Documentation
*/
default CompletableFuture getCostCategories(
Consumer getCostCategoriesRequest) {
return getCostCategories(GetCostCategoriesRequest.builder().applyMutation(getCostCategoriesRequest).build());
}
/**
*
* Retrieves a forecast for how much Amazon Web Services predicts that you will spend over the forecast time period
* that you select, based on your past costs.
*
*
* @param getCostForecastRequest
* @return A Java Future containing the result of the GetCostForecast operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetCostForecast
* @see AWS API
* Documentation
*/
default CompletableFuture getCostForecast(GetCostForecastRequest getCostForecastRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a forecast for how much Amazon Web Services predicts that you will spend over the forecast time period
* that you select, based on your past costs.
*
*
*
* This is a convenience which creates an instance of the {@link GetCostForecastRequest.Builder} avoiding the need
* to create one manually via {@link GetCostForecastRequest#builder()}
*
*
* @param getCostForecastRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetCostForecastRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetCostForecast operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetCostForecast
* @see AWS API
* Documentation
*/
default CompletableFuture getCostForecast(
Consumer getCostForecastRequest) {
return getCostForecast(GetCostForecastRequest.builder().applyMutation(getCostForecastRequest).build());
}
/**
*
* Retrieves all available filter values for a specified filter over a period of time. You can search the dimension
* values for an arbitrary string.
*
*
* @param getDimensionValuesRequest
* @return A Java Future containing the result of the GetDimensionValues operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - BillExpirationException The requested report expired. Update the date interval and try again.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - RequestChangedException Your request parameters changed between pages. Try again with the old
* parameters or without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetDimensionValues
* @see AWS API
* Documentation
*/
default CompletableFuture getDimensionValues(GetDimensionValuesRequest getDimensionValuesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves all available filter values for a specified filter over a period of time. You can search the dimension
* values for an arbitrary string.
*
*
*
* This is a convenience which creates an instance of the {@link GetDimensionValuesRequest.Builder} avoiding the
* need to create one manually via {@link GetDimensionValuesRequest#builder()}
*
*
* @param getDimensionValuesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetDimensionValuesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetDimensionValues operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - BillExpirationException The requested report expired. Update the date interval and try again.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - RequestChangedException Your request parameters changed between pages. Try again with the old
* parameters or without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetDimensionValues
* @see AWS API
* Documentation
*/
default CompletableFuture getDimensionValues(
Consumer getDimensionValuesRequest) {
return getDimensionValues(GetDimensionValuesRequest.builder().applyMutation(getDimensionValuesRequest).build());
}
/**
*
* Retrieves the reservation coverage for your account, which you can use to see how much of your Amazon Elastic
* Compute Cloud, Amazon ElastiCache, Amazon Relational Database Service, or Amazon Redshift usage is covered by a
* reservation. An organization's management account can see the coverage of the associated member accounts. This
* supports dimensions, Cost Categories, and nested expressions. For any time period, you can filter data about
* reservation usage by the following dimensions:
*
*
* -
*
* AZ
*
*
* -
*
* CACHE_ENGINE
*
*
* -
*
* DATABASE_ENGINE
*
*
* -
*
* DEPLOYMENT_OPTION
*
*
* -
*
* INSTANCE_TYPE
*
*
* -
*
* LINKED_ACCOUNT
*
*
* -
*
* OPERATING_SYSTEM
*
*
* -
*
* PLATFORM
*
*
* -
*
* REGION
*
*
* -
*
* SERVICE
*
*
* -
*
* TAG
*
*
* -
*
* TENANCY
*
*
*
*
* To determine valid values for a dimension, use the GetDimensionValues
operation.
*
*
* @param getReservationCoverageRequest
* You can use the following request parameters to query for how much of your instance usage a reservation
* covered.
* @return A Java Future containing the result of the GetReservationCoverage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetReservationCoverage
* @see AWS API
* Documentation
*/
default CompletableFuture getReservationCoverage(
GetReservationCoverageRequest getReservationCoverageRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the reservation coverage for your account, which you can use to see how much of your Amazon Elastic
* Compute Cloud, Amazon ElastiCache, Amazon Relational Database Service, or Amazon Redshift usage is covered by a
* reservation. An organization's management account can see the coverage of the associated member accounts. This
* supports dimensions, Cost Categories, and nested expressions. For any time period, you can filter data about
* reservation usage by the following dimensions:
*
*
* -
*
* AZ
*
*
* -
*
* CACHE_ENGINE
*
*
* -
*
* DATABASE_ENGINE
*
*
* -
*
* DEPLOYMENT_OPTION
*
*
* -
*
* INSTANCE_TYPE
*
*
* -
*
* LINKED_ACCOUNT
*
*
* -
*
* OPERATING_SYSTEM
*
*
* -
*
* PLATFORM
*
*
* -
*
* REGION
*
*
* -
*
* SERVICE
*
*
* -
*
* TAG
*
*
* -
*
* TENANCY
*
*
*
*
* To determine valid values for a dimension, use the GetDimensionValues
operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetReservationCoverageRequest.Builder} avoiding the
* need to create one manually via {@link GetReservationCoverageRequest#builder()}
*
*
* @param getReservationCoverageRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetReservationCoverageRequest.Builder} to create
* a request. You can use the following request parameters to query for how much of your instance usage a
* reservation covered.
* @return A Java Future containing the result of the GetReservationCoverage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetReservationCoverage
* @see AWS API
* Documentation
*/
default CompletableFuture getReservationCoverage(
Consumer getReservationCoverageRequest) {
return getReservationCoverage(GetReservationCoverageRequest.builder().applyMutation(getReservationCoverageRequest)
.build());
}
/**
*
* Gets recommendations for reservation purchases. These recommendations might help you to reduce your costs.
* Reservations provide a discounted hourly rate (up to 75%) compared to On-Demand pricing.
*
*
* Amazon Web Services generates your recommendations by identifying your On-Demand usage during a specific time
* period and collecting your usage into categories that are eligible for a reservation. After Amazon Web Services
* has these categories, it simulates every combination of reservations in each category of usage to identify the
* best number of each type of Reserved Instance (RI) to purchase to maximize your estimated savings.
*
*
* For example, Amazon Web Services automatically aggregates your Amazon EC2 Linux, shared tenancy, and c4 family
* usage in the US West (Oregon) Region and recommends that you buy size-flexible regional reservations to apply to
* the c4 family usage. Amazon Web Services recommends the smallest size instance in an instance family. This makes
* it easier to purchase a size-flexible Reserved Instance (RI). Amazon Web Services also shows the equal number of
* normalized units. This way, you can purchase any instance size that you want. For this example, your RI
* recommendation is for c4.large
because that is the smallest size instance in the c4 instance family.
*
*
* @param getReservationPurchaseRecommendationRequest
* @return A Java Future containing the result of the GetReservationPurchaseRecommendation operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetReservationPurchaseRecommendation
* @see AWS API Documentation
*/
default CompletableFuture getReservationPurchaseRecommendation(
GetReservationPurchaseRecommendationRequest getReservationPurchaseRecommendationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets recommendations for reservation purchases. These recommendations might help you to reduce your costs.
* Reservations provide a discounted hourly rate (up to 75%) compared to On-Demand pricing.
*
*
* Amazon Web Services generates your recommendations by identifying your On-Demand usage during a specific time
* period and collecting your usage into categories that are eligible for a reservation. After Amazon Web Services
* has these categories, it simulates every combination of reservations in each category of usage to identify the
* best number of each type of Reserved Instance (RI) to purchase to maximize your estimated savings.
*
*
* For example, Amazon Web Services automatically aggregates your Amazon EC2 Linux, shared tenancy, and c4 family
* usage in the US West (Oregon) Region and recommends that you buy size-flexible regional reservations to apply to
* the c4 family usage. Amazon Web Services recommends the smallest size instance in an instance family. This makes
* it easier to purchase a size-flexible Reserved Instance (RI). Amazon Web Services also shows the equal number of
* normalized units. This way, you can purchase any instance size that you want. For this example, your RI
* recommendation is for c4.large
because that is the smallest size instance in the c4 instance family.
*
*
*
* This is a convenience which creates an instance of the
* {@link GetReservationPurchaseRecommendationRequest.Builder} avoiding the need to create one manually via
* {@link GetReservationPurchaseRecommendationRequest#builder()}
*
*
* @param getReservationPurchaseRecommendationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetReservationPurchaseRecommendationRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the GetReservationPurchaseRecommendation operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetReservationPurchaseRecommendation
* @see AWS API Documentation
*/
default CompletableFuture getReservationPurchaseRecommendation(
Consumer getReservationPurchaseRecommendationRequest) {
return getReservationPurchaseRecommendation(GetReservationPurchaseRecommendationRequest.builder()
.applyMutation(getReservationPurchaseRecommendationRequest).build());
}
/**
*
* Retrieves the reservation utilization for your account. Management account in an organization have access to
* member accounts. You can filter data by dimensions in a time period. You can use GetDimensionValues
* to determine the possible dimension values. Currently, you can group only by SUBSCRIPTION_ID
.
*
*
* @param getReservationUtilizationRequest
* @return A Java Future containing the result of the GetReservationUtilization operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetReservationUtilization
* @see AWS
* API Documentation
*/
default CompletableFuture getReservationUtilization(
GetReservationUtilizationRequest getReservationUtilizationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the reservation utilization for your account. Management account in an organization have access to
* member accounts. You can filter data by dimensions in a time period. You can use GetDimensionValues
* to determine the possible dimension values. Currently, you can group only by SUBSCRIPTION_ID
.
*
*
*
* This is a convenience which creates an instance of the {@link GetReservationUtilizationRequest.Builder} avoiding
* the need to create one manually via {@link GetReservationUtilizationRequest#builder()}
*
*
* @param getReservationUtilizationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetReservationUtilizationRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetReservationUtilization operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetReservationUtilization
* @see AWS
* API Documentation
*/
default CompletableFuture getReservationUtilization(
Consumer getReservationUtilizationRequest) {
return getReservationUtilization(GetReservationUtilizationRequest.builder()
.applyMutation(getReservationUtilizationRequest).build());
}
/**
*
* Creates recommendations that help you save cost by identifying idle and underutilized Amazon EC2 instances.
*
*
* Recommendations are generated to either downsize or terminate instances, along with providing savings detail and
* metrics. For more information about calculation and function, see Optimizing Your Cost with
* Rightsizing Recommendations in the Billing and Cost Management User Guide.
*
*
* @param getRightsizingRecommendationRequest
* @return A Java Future containing the result of the GetRightsizingRecommendation operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetRightsizingRecommendation
* @see AWS API Documentation
*/
default CompletableFuture getRightsizingRecommendation(
GetRightsizingRecommendationRequest getRightsizingRecommendationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates recommendations that help you save cost by identifying idle and underutilized Amazon EC2 instances.
*
*
* Recommendations are generated to either downsize or terminate instances, along with providing savings detail and
* metrics. For more information about calculation and function, see Optimizing Your Cost with
* Rightsizing Recommendations in the Billing and Cost Management User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetRightsizingRecommendationRequest.Builder}
* avoiding the need to create one manually via {@link GetRightsizingRecommendationRequest#builder()}
*
*
* @param getRightsizingRecommendationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetRightsizingRecommendationRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetRightsizingRecommendation operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetRightsizingRecommendation
* @see AWS API Documentation
*/
default CompletableFuture getRightsizingRecommendation(
Consumer getRightsizingRecommendationRequest) {
return getRightsizingRecommendation(GetRightsizingRecommendationRequest.builder()
.applyMutation(getRightsizingRecommendationRequest).build());
}
/**
*
* Retrieves the details for a Savings Plan recommendation. These details include the hourly data-points that
* construct the cost, coverage, and utilization charts.
*
*
* @param getSavingsPlanPurchaseRecommendationDetailsRequest
* @return A Java Future containing the result of the GetSavingsPlanPurchaseRecommendationDetails operation returned
* by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetSavingsPlanPurchaseRecommendationDetails
* @see AWS API Documentation
*/
default CompletableFuture getSavingsPlanPurchaseRecommendationDetails(
GetSavingsPlanPurchaseRecommendationDetailsRequest getSavingsPlanPurchaseRecommendationDetailsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the details for a Savings Plan recommendation. These details include the hourly data-points that
* construct the cost, coverage, and utilization charts.
*
*
*
* This is a convenience which creates an instance of the
* {@link GetSavingsPlanPurchaseRecommendationDetailsRequest.Builder} avoiding the need to create one manually via
* {@link GetSavingsPlanPurchaseRecommendationDetailsRequest#builder()}
*
*
* @param getSavingsPlanPurchaseRecommendationDetailsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetSavingsPlanPurchaseRecommendationDetailsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the GetSavingsPlanPurchaseRecommendationDetails operation returned
* by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetSavingsPlanPurchaseRecommendationDetails
* @see AWS API Documentation
*/
default CompletableFuture getSavingsPlanPurchaseRecommendationDetails(
Consumer getSavingsPlanPurchaseRecommendationDetailsRequest) {
return getSavingsPlanPurchaseRecommendationDetails(GetSavingsPlanPurchaseRecommendationDetailsRequest.builder()
.applyMutation(getSavingsPlanPurchaseRecommendationDetailsRequest).build());
}
/**
*
* Retrieves the Savings Plans covered for your account. This enables you to see how much of your cost is covered by
* a Savings Plan. An organization’s management account can see the coverage of the associated member accounts. This
* supports dimensions, Cost Categories, and nested expressions. For any time period, you can filter data for
* Savings Plans usage with the following dimensions:
*
*
* -
*
* LINKED_ACCOUNT
*
*
* -
*
* REGION
*
*
* -
*
* SERVICE
*
*
* -
*
* INSTANCE_FAMILY
*
*
*
*
* To determine valid values for a dimension, use the GetDimensionValues
operation.
*
*
* @param getSavingsPlansCoverageRequest
* @return A Java Future containing the result of the GetSavingsPlansCoverage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetSavingsPlansCoverage
* @see AWS
* API Documentation
*/
default CompletableFuture getSavingsPlansCoverage(
GetSavingsPlansCoverageRequest getSavingsPlansCoverageRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the Savings Plans covered for your account. This enables you to see how much of your cost is covered by
* a Savings Plan. An organization’s management account can see the coverage of the associated member accounts. This
* supports dimensions, Cost Categories, and nested expressions. For any time period, you can filter data for
* Savings Plans usage with the following dimensions:
*
*
* -
*
* LINKED_ACCOUNT
*
*
* -
*
* REGION
*
*
* -
*
* SERVICE
*
*
* -
*
* INSTANCE_FAMILY
*
*
*
*
* To determine valid values for a dimension, use the GetDimensionValues
operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetSavingsPlansCoverageRequest.Builder} avoiding
* the need to create one manually via {@link GetSavingsPlansCoverageRequest#builder()}
*
*
* @param getSavingsPlansCoverageRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansCoverageRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetSavingsPlansCoverage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetSavingsPlansCoverage
* @see AWS
* API Documentation
*/
default CompletableFuture getSavingsPlansCoverage(
Consumer getSavingsPlansCoverageRequest) {
return getSavingsPlansCoverage(GetSavingsPlansCoverageRequest.builder().applyMutation(getSavingsPlansCoverageRequest)
.build());
}
/**
*
* This is a variant of
* {@link #getSavingsPlansCoverage(software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansCoverageRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.GetSavingsPlansCoveragePublisher publisher = client.getSavingsPlansCoveragePaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.GetSavingsPlansCoveragePublisher publisher = client.getSavingsPlansCoveragePaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansCoverageResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getSavingsPlansCoverage(software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansCoverageRequest)}
* operation.
*
*
* @param getSavingsPlansCoverageRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetSavingsPlansCoverage
* @see AWS
* API Documentation
*/
default GetSavingsPlansCoveragePublisher getSavingsPlansCoveragePaginator(
GetSavingsPlansCoverageRequest getSavingsPlansCoverageRequest) {
return new GetSavingsPlansCoveragePublisher(this, getSavingsPlansCoverageRequest);
}
/**
*
* This is a variant of
* {@link #getSavingsPlansCoverage(software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansCoverageRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.GetSavingsPlansCoveragePublisher publisher = client.getSavingsPlansCoveragePaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.GetSavingsPlansCoveragePublisher publisher = client.getSavingsPlansCoveragePaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansCoverageResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getSavingsPlansCoverage(software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansCoverageRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetSavingsPlansCoverageRequest.Builder} avoiding
* the need to create one manually via {@link GetSavingsPlansCoverageRequest#builder()}
*
*
* @param getSavingsPlansCoverageRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansCoverageRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetSavingsPlansCoverage
* @see AWS
* API Documentation
*/
default GetSavingsPlansCoveragePublisher getSavingsPlansCoveragePaginator(
Consumer getSavingsPlansCoverageRequest) {
return getSavingsPlansCoveragePaginator(GetSavingsPlansCoverageRequest.builder()
.applyMutation(getSavingsPlansCoverageRequest).build());
}
/**
*
* Retrieves the Savings Plans recommendations for your account. First use
* StartSavingsPlansPurchaseRecommendationGeneration
to generate a new set of recommendations, and then
* use GetSavingsPlansPurchaseRecommendation
to retrieve them.
*
*
* @param getSavingsPlansPurchaseRecommendationRequest
* @return A Java Future containing the result of the GetSavingsPlansPurchaseRecommendation operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetSavingsPlansPurchaseRecommendation
* @see AWS API Documentation
*/
default CompletableFuture getSavingsPlansPurchaseRecommendation(
GetSavingsPlansPurchaseRecommendationRequest getSavingsPlansPurchaseRecommendationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the Savings Plans recommendations for your account. First use
* StartSavingsPlansPurchaseRecommendationGeneration
to generate a new set of recommendations, and then
* use GetSavingsPlansPurchaseRecommendation
to retrieve them.
*
*
*
* This is a convenience which creates an instance of the
* {@link GetSavingsPlansPurchaseRecommendationRequest.Builder} avoiding the need to create one manually via
* {@link GetSavingsPlansPurchaseRecommendationRequest#builder()}
*
*
* @param getSavingsPlansPurchaseRecommendationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansPurchaseRecommendationRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the GetSavingsPlansPurchaseRecommendation operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetSavingsPlansPurchaseRecommendation
* @see AWS API Documentation
*/
default CompletableFuture getSavingsPlansPurchaseRecommendation(
Consumer getSavingsPlansPurchaseRecommendationRequest) {
return getSavingsPlansPurchaseRecommendation(GetSavingsPlansPurchaseRecommendationRequest.builder()
.applyMutation(getSavingsPlansPurchaseRecommendationRequest).build());
}
/**
*
* Retrieves the Savings Plans utilization for your account across date ranges with daily or monthly granularity.
* Management account in an organization have access to member accounts. You can use GetDimensionValues
* in SAVINGS_PLANS
to determine the possible dimension values.
*
*
*
* You can't group by any dimension values for GetSavingsPlansUtilization
.
*
*
*
* @param getSavingsPlansUtilizationRequest
* @return A Java Future containing the result of the GetSavingsPlansUtilization operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetSavingsPlansUtilization
* @see AWS
* API Documentation
*/
default CompletableFuture getSavingsPlansUtilization(
GetSavingsPlansUtilizationRequest getSavingsPlansUtilizationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the Savings Plans utilization for your account across date ranges with daily or monthly granularity.
* Management account in an organization have access to member accounts. You can use GetDimensionValues
* in SAVINGS_PLANS
to determine the possible dimension values.
*
*
*
* You can't group by any dimension values for GetSavingsPlansUtilization
.
*
*
*
* This is a convenience which creates an instance of the {@link GetSavingsPlansUtilizationRequest.Builder} avoiding
* the need to create one manually via {@link GetSavingsPlansUtilizationRequest#builder()}
*
*
* @param getSavingsPlansUtilizationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansUtilizationRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetSavingsPlansUtilization operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetSavingsPlansUtilization
* @see AWS
* API Documentation
*/
default CompletableFuture getSavingsPlansUtilization(
Consumer getSavingsPlansUtilizationRequest) {
return getSavingsPlansUtilization(GetSavingsPlansUtilizationRequest.builder()
.applyMutation(getSavingsPlansUtilizationRequest).build());
}
/**
*
* Retrieves attribute data along with aggregate utilization and savings data for a given time period. This doesn't
* support granular or grouped data (daily/monthly) in response. You can't retrieve data by dates in a single
* response similar to GetSavingsPlanUtilization
, but you have the option to make multiple calls to
* GetSavingsPlanUtilizationDetails
by providing individual dates. You can use
* GetDimensionValues
in SAVINGS_PLANS
to determine the possible dimension values.
*
*
*
* GetSavingsPlanUtilizationDetails
internally groups data by SavingsPlansArn
.
*
*
*
* @param getSavingsPlansUtilizationDetailsRequest
* @return A Java Future containing the result of the GetSavingsPlansUtilizationDetails operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetSavingsPlansUtilizationDetails
* @see AWS API Documentation
*/
default CompletableFuture getSavingsPlansUtilizationDetails(
GetSavingsPlansUtilizationDetailsRequest getSavingsPlansUtilizationDetailsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves attribute data along with aggregate utilization and savings data for a given time period. This doesn't
* support granular or grouped data (daily/monthly) in response. You can't retrieve data by dates in a single
* response similar to GetSavingsPlanUtilization
, but you have the option to make multiple calls to
* GetSavingsPlanUtilizationDetails
by providing individual dates. You can use
* GetDimensionValues
in SAVINGS_PLANS
to determine the possible dimension values.
*
*
*
* GetSavingsPlanUtilizationDetails
internally groups data by SavingsPlansArn
.
*
*
*
* This is a convenience which creates an instance of the {@link GetSavingsPlansUtilizationDetailsRequest.Builder}
* avoiding the need to create one manually via {@link GetSavingsPlansUtilizationDetailsRequest#builder()}
*
*
* @param getSavingsPlansUtilizationDetailsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansUtilizationDetailsRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the GetSavingsPlansUtilizationDetails operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetSavingsPlansUtilizationDetails
* @see AWS API Documentation
*/
default CompletableFuture getSavingsPlansUtilizationDetails(
Consumer getSavingsPlansUtilizationDetailsRequest) {
return getSavingsPlansUtilizationDetails(GetSavingsPlansUtilizationDetailsRequest.builder()
.applyMutation(getSavingsPlansUtilizationDetailsRequest).build());
}
/**
*
* This is a variant of
* {@link #getSavingsPlansUtilizationDetails(software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansUtilizationDetailsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.GetSavingsPlansUtilizationDetailsPublisher publisher = client.getSavingsPlansUtilizationDetailsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.GetSavingsPlansUtilizationDetailsPublisher publisher = client.getSavingsPlansUtilizationDetailsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansUtilizationDetailsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getSavingsPlansUtilizationDetails(software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansUtilizationDetailsRequest)}
* operation.
*
*
* @param getSavingsPlansUtilizationDetailsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetSavingsPlansUtilizationDetails
* @see AWS API Documentation
*/
default GetSavingsPlansUtilizationDetailsPublisher getSavingsPlansUtilizationDetailsPaginator(
GetSavingsPlansUtilizationDetailsRequest getSavingsPlansUtilizationDetailsRequest) {
return new GetSavingsPlansUtilizationDetailsPublisher(this, getSavingsPlansUtilizationDetailsRequest);
}
/**
*
* This is a variant of
* {@link #getSavingsPlansUtilizationDetails(software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansUtilizationDetailsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.GetSavingsPlansUtilizationDetailsPublisher publisher = client.getSavingsPlansUtilizationDetailsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.GetSavingsPlansUtilizationDetailsPublisher publisher = client.getSavingsPlansUtilizationDetailsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansUtilizationDetailsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getSavingsPlansUtilizationDetails(software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansUtilizationDetailsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetSavingsPlansUtilizationDetailsRequest.Builder}
* avoiding the need to create one manually via {@link GetSavingsPlansUtilizationDetailsRequest#builder()}
*
*
* @param getSavingsPlansUtilizationDetailsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetSavingsPlansUtilizationDetailsRequest.Builder}
* to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetSavingsPlansUtilizationDetails
* @see AWS API Documentation
*/
default GetSavingsPlansUtilizationDetailsPublisher getSavingsPlansUtilizationDetailsPaginator(
Consumer getSavingsPlansUtilizationDetailsRequest) {
return getSavingsPlansUtilizationDetailsPaginator(GetSavingsPlansUtilizationDetailsRequest.builder()
.applyMutation(getSavingsPlansUtilizationDetailsRequest).build());
}
/**
*
* Queries for available tag keys and tag values for a specified period. You can search the tag values for an
* arbitrary string.
*
*
* @param getTagsRequest
* @return A Java Future containing the result of the GetTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - BillExpirationException The requested report expired. Update the date interval and try again.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - RequestChangedException Your request parameters changed between pages. Try again with the old
* parameters or without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetTags
* @see AWS API
* Documentation
*/
default CompletableFuture getTags(GetTagsRequest getTagsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Queries for available tag keys and tag values for a specified period. You can search the tag values for an
* arbitrary string.
*
*
*
* This is a convenience which creates an instance of the {@link GetTagsRequest.Builder} avoiding the need to create
* one manually via {@link GetTagsRequest#builder()}
*
*
* @param getTagsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetTagsRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - BillExpirationException The requested report expired. Update the date interval and try again.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - RequestChangedException Your request parameters changed between pages. Try again with the old
* parameters or without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetTags
* @see AWS API
* Documentation
*/
default CompletableFuture getTags(Consumer getTagsRequest) {
return getTags(GetTagsRequest.builder().applyMutation(getTagsRequest).build());
}
/**
*
* Retrieves a forecast for how much Amazon Web Services predicts that you will use over the forecast time period
* that you select, based on your past usage.
*
*
* @param getUsageForecastRequest
* @return A Java Future containing the result of the GetUsageForecast operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - UnresolvableUsageUnitException Cost Explorer was unable to identify the usage unit. Provide
*
UsageType/UsageTypeGroup
filter selections that contain matching units, for example:
* hours
.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetUsageForecast
* @see AWS API
* Documentation
*/
default CompletableFuture getUsageForecast(GetUsageForecastRequest getUsageForecastRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a forecast for how much Amazon Web Services predicts that you will use over the forecast time period
* that you select, based on your past usage.
*
*
*
* This is a convenience which creates an instance of the {@link GetUsageForecastRequest.Builder} avoiding the need
* to create one manually via {@link GetUsageForecastRequest#builder()}
*
*
* @param getUsageForecastRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.GetUsageForecastRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetUsageForecast operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - UnresolvableUsageUnitException Cost Explorer was unable to identify the usage unit. Provide
*
UsageType/UsageTypeGroup
filter selections that contain matching units, for example:
* hours
.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetUsageForecast
* @see AWS API
* Documentation
*/
default CompletableFuture getUsageForecast(
Consumer getUsageForecastRequest) {
return getUsageForecast(GetUsageForecastRequest.builder().applyMutation(getUsageForecastRequest).build());
}
/**
*
* Get a list of cost allocation tags. All inputs in the API are optional and serve as filters. By default, all cost
* allocation tags are returned.
*
*
* @param listCostAllocationTagsRequest
* @return A Java Future containing the result of the ListCostAllocationTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.ListCostAllocationTags
* @see AWS API
* Documentation
*/
default CompletableFuture listCostAllocationTags(
ListCostAllocationTagsRequest listCostAllocationTagsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Get a list of cost allocation tags. All inputs in the API are optional and serve as filters. By default, all cost
* allocation tags are returned.
*
*
*
* This is a convenience which creates an instance of the {@link ListCostAllocationTagsRequest.Builder} avoiding the
* need to create one manually via {@link ListCostAllocationTagsRequest#builder()}
*
*
* @param listCostAllocationTagsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.ListCostAllocationTagsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the ListCostAllocationTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.ListCostAllocationTags
* @see AWS API
* Documentation
*/
default CompletableFuture listCostAllocationTags(
Consumer listCostAllocationTagsRequest) {
return listCostAllocationTags(ListCostAllocationTagsRequest.builder().applyMutation(listCostAllocationTagsRequest)
.build());
}
/**
*
* This is a variant of
* {@link #listCostAllocationTags(software.amazon.awssdk.services.costexplorer.model.ListCostAllocationTagsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.ListCostAllocationTagsPublisher publisher = client.listCostAllocationTagsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.ListCostAllocationTagsPublisher publisher = client.listCostAllocationTagsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.costexplorer.model.ListCostAllocationTagsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listCostAllocationTags(software.amazon.awssdk.services.costexplorer.model.ListCostAllocationTagsRequest)}
* operation.
*
*
* @param listCostAllocationTagsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.ListCostAllocationTags
* @see AWS API
* Documentation
*/
default ListCostAllocationTagsPublisher listCostAllocationTagsPaginator(
ListCostAllocationTagsRequest listCostAllocationTagsRequest) {
return new ListCostAllocationTagsPublisher(this, listCostAllocationTagsRequest);
}
/**
*
* This is a variant of
* {@link #listCostAllocationTags(software.amazon.awssdk.services.costexplorer.model.ListCostAllocationTagsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.ListCostAllocationTagsPublisher publisher = client.listCostAllocationTagsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.ListCostAllocationTagsPublisher publisher = client.listCostAllocationTagsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.costexplorer.model.ListCostAllocationTagsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listCostAllocationTags(software.amazon.awssdk.services.costexplorer.model.ListCostAllocationTagsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListCostAllocationTagsRequest.Builder} avoiding the
* need to create one manually via {@link ListCostAllocationTagsRequest#builder()}
*
*
* @param listCostAllocationTagsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.ListCostAllocationTagsRequest.Builder} to create
* a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.ListCostAllocationTags
* @see AWS API
* Documentation
*/
default ListCostAllocationTagsPublisher listCostAllocationTagsPaginator(
Consumer listCostAllocationTagsRequest) {
return listCostAllocationTagsPaginator(ListCostAllocationTagsRequest.builder()
.applyMutation(listCostAllocationTagsRequest).build());
}
/**
*
* Returns the name, Amazon Resource Name (ARN), NumberOfRules
and effective dates of all Cost
* Categories defined in the account. You have the option to use EffectiveOn
to return a list of Cost
* Categories that were active on a specific date. If there is no EffectiveOn
specified, you’ll see
* Cost Categories that are effective on the current date. If Cost Category is still effective,
* EffectiveEnd
is omitted in the response. ListCostCategoryDefinitions
supports
* pagination. The request can have a MaxResults
range up to 100.
*
*
* @param listCostCategoryDefinitionsRequest
* @return A Java Future containing the result of the ListCostCategoryDefinitions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.ListCostCategoryDefinitions
* @see AWS API Documentation
*/
default CompletableFuture listCostCategoryDefinitions(
ListCostCategoryDefinitionsRequest listCostCategoryDefinitionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the name, Amazon Resource Name (ARN), NumberOfRules
and effective dates of all Cost
* Categories defined in the account. You have the option to use EffectiveOn
to return a list of Cost
* Categories that were active on a specific date. If there is no EffectiveOn
specified, you’ll see
* Cost Categories that are effective on the current date. If Cost Category is still effective,
* EffectiveEnd
is omitted in the response. ListCostCategoryDefinitions
supports
* pagination. The request can have a MaxResults
range up to 100.
*
*
*
* This is a convenience which creates an instance of the {@link ListCostCategoryDefinitionsRequest.Builder}
* avoiding the need to create one manually via {@link ListCostCategoryDefinitionsRequest#builder()}
*
*
* @param listCostCategoryDefinitionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.ListCostCategoryDefinitionsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ListCostCategoryDefinitions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.ListCostCategoryDefinitions
* @see AWS API Documentation
*/
default CompletableFuture listCostCategoryDefinitions(
Consumer listCostCategoryDefinitionsRequest) {
return listCostCategoryDefinitions(ListCostCategoryDefinitionsRequest.builder()
.applyMutation(listCostCategoryDefinitionsRequest).build());
}
/**
*
* This is a variant of
* {@link #listCostCategoryDefinitions(software.amazon.awssdk.services.costexplorer.model.ListCostCategoryDefinitionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.ListCostCategoryDefinitionsPublisher publisher = client.listCostCategoryDefinitionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.ListCostCategoryDefinitionsPublisher publisher = client.listCostCategoryDefinitionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.costexplorer.model.ListCostCategoryDefinitionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listCostCategoryDefinitions(software.amazon.awssdk.services.costexplorer.model.ListCostCategoryDefinitionsRequest)}
* operation.
*
*
* @param listCostCategoryDefinitionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.ListCostCategoryDefinitions
* @see AWS API Documentation
*/
default ListCostCategoryDefinitionsPublisher listCostCategoryDefinitionsPaginator(
ListCostCategoryDefinitionsRequest listCostCategoryDefinitionsRequest) {
return new ListCostCategoryDefinitionsPublisher(this, listCostCategoryDefinitionsRequest);
}
/**
*
* This is a variant of
* {@link #listCostCategoryDefinitions(software.amazon.awssdk.services.costexplorer.model.ListCostCategoryDefinitionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.ListCostCategoryDefinitionsPublisher publisher = client.listCostCategoryDefinitionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.costexplorer.paginators.ListCostCategoryDefinitionsPublisher publisher = client.listCostCategoryDefinitionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.costexplorer.model.ListCostCategoryDefinitionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listCostCategoryDefinitions(software.amazon.awssdk.services.costexplorer.model.ListCostCategoryDefinitionsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListCostCategoryDefinitionsRequest.Builder}
* avoiding the need to create one manually via {@link ListCostCategoryDefinitionsRequest#builder()}
*
*
* @param listCostCategoryDefinitionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.ListCostCategoryDefinitionsRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.ListCostCategoryDefinitions
* @see AWS API Documentation
*/
default ListCostCategoryDefinitionsPublisher listCostCategoryDefinitionsPaginator(
Consumer listCostCategoryDefinitionsRequest) {
return listCostCategoryDefinitionsPaginator(ListCostCategoryDefinitionsRequest.builder()
.applyMutation(listCostCategoryDefinitionsRequest).build());
}
/**
*
* Retrieves a list of your historical recommendation generations within the past 30 days.
*
*
* @param listSavingsPlansPurchaseRecommendationGenerationRequest
* @return A Java Future containing the result of the ListSavingsPlansPurchaseRecommendationGeneration operation
* returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - DataUnavailableException The requested data is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.ListSavingsPlansPurchaseRecommendationGeneration
* @see AWS API Documentation
*/
default CompletableFuture listSavingsPlansPurchaseRecommendationGeneration(
ListSavingsPlansPurchaseRecommendationGenerationRequest listSavingsPlansPurchaseRecommendationGenerationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of your historical recommendation generations within the past 30 days.
*
*
*
* This is a convenience which creates an instance of the
* {@link ListSavingsPlansPurchaseRecommendationGenerationRequest.Builder} avoiding the need to create one manually
* via {@link ListSavingsPlansPurchaseRecommendationGenerationRequest#builder()}
*
*
* @param listSavingsPlansPurchaseRecommendationGenerationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.ListSavingsPlansPurchaseRecommendationGenerationRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the ListSavingsPlansPurchaseRecommendationGeneration operation
* returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - DataUnavailableException The requested data is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.ListSavingsPlansPurchaseRecommendationGeneration
* @see AWS API Documentation
*/
default CompletableFuture listSavingsPlansPurchaseRecommendationGeneration(
Consumer listSavingsPlansPurchaseRecommendationGenerationRequest) {
return listSavingsPlansPurchaseRecommendationGeneration(ListSavingsPlansPurchaseRecommendationGenerationRequest.builder()
.applyMutation(listSavingsPlansPurchaseRecommendationGenerationRequest).build());
}
/**
*
* Returns a list of resource tags associated with the resource specified by the Amazon Resource Name (ARN).
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified ARN in the request doesn't exist.
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of resource tags associated with the resource specified by the Amazon Resource Name (ARN).
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified ARN in the request doesn't exist.
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Modifies the feedback property of a given cost anomaly.
*
*
* @param provideAnomalyFeedbackRequest
* @return A Java Future containing the result of the ProvideAnomalyFeedback operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.ProvideAnomalyFeedback
* @see AWS API
* Documentation
*/
default CompletableFuture provideAnomalyFeedback(
ProvideAnomalyFeedbackRequest provideAnomalyFeedbackRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies the feedback property of a given cost anomaly.
*
*
*
* This is a convenience which creates an instance of the {@link ProvideAnomalyFeedbackRequest.Builder} avoiding the
* need to create one manually via {@link ProvideAnomalyFeedbackRequest#builder()}
*
*
* @param provideAnomalyFeedbackRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.ProvideAnomalyFeedbackRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the ProvideAnomalyFeedback operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.ProvideAnomalyFeedback
* @see AWS API
* Documentation
*/
default CompletableFuture provideAnomalyFeedback(
Consumer provideAnomalyFeedbackRequest) {
return provideAnomalyFeedback(ProvideAnomalyFeedbackRequest.builder().applyMutation(provideAnomalyFeedbackRequest)
.build());
}
/**
*
* Requests a Savings Plans recommendation generation. This enables you to calculate a fresh set of Savings Plans
* recommendations that takes your latest usage data and current Savings Plans inventory into account. You can
* refresh Savings Plans recommendations up to three times daily for a consolidated billing family.
*
*
*
* StartSavingsPlansPurchaseRecommendationGeneration
has no request syntax because no input parameters
* are needed to support this operation.
*
*
*
* @param startSavingsPlansPurchaseRecommendationGenerationRequest
* @return A Java Future containing the result of the StartSavingsPlansPurchaseRecommendationGeneration operation
* returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - ServiceQuotaExceededException You've reached the limit on the number of resources you can create, or
* exceeded the size of an individual resource.
* - GenerationExistsException A request to generate a recommendation is already in progress.
* - DataUnavailableException The requested data is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.StartSavingsPlansPurchaseRecommendationGeneration
* @see AWS API Documentation
*/
default CompletableFuture startSavingsPlansPurchaseRecommendationGeneration(
StartSavingsPlansPurchaseRecommendationGenerationRequest startSavingsPlansPurchaseRecommendationGenerationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Requests a Savings Plans recommendation generation. This enables you to calculate a fresh set of Savings Plans
* recommendations that takes your latest usage data and current Savings Plans inventory into account. You can
* refresh Savings Plans recommendations up to three times daily for a consolidated billing family.
*
*
*
* StartSavingsPlansPurchaseRecommendationGeneration
has no request syntax because no input parameters
* are needed to support this operation.
*
*
*
* This is a convenience which creates an instance of the
* {@link StartSavingsPlansPurchaseRecommendationGenerationRequest.Builder} avoiding the need to create one manually
* via {@link StartSavingsPlansPurchaseRecommendationGenerationRequest#builder()}
*
*
* @param startSavingsPlansPurchaseRecommendationGenerationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.StartSavingsPlansPurchaseRecommendationGenerationRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the StartSavingsPlansPurchaseRecommendationGeneration operation
* returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - ServiceQuotaExceededException You've reached the limit on the number of resources you can create, or
* exceeded the size of an individual resource.
* - GenerationExistsException A request to generate a recommendation is already in progress.
* - DataUnavailableException The requested data is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.StartSavingsPlansPurchaseRecommendationGeneration
* @see AWS API Documentation
*/
default CompletableFuture startSavingsPlansPurchaseRecommendationGeneration(
Consumer startSavingsPlansPurchaseRecommendationGenerationRequest) {
return startSavingsPlansPurchaseRecommendationGeneration(StartSavingsPlansPurchaseRecommendationGenerationRequest
.builder().applyMutation(startSavingsPlansPurchaseRecommendationGenerationRequest).build());
}
/**
*
* An API operation for adding one or more tags (key-value pairs) to a resource.
*
*
* You can use the TagResource
operation with a resource that already has tags. If you specify a new
* tag key for the resource, this tag is appended to the list of tags associated with the resource. If you specify a
* tag key that is already associated with the resource, the new tag value you specify replaces the previous value
* for that tag.
*
*
* Although the maximum number of array members is 200, user-tag maximum is 50. The remaining are reserved for
* Amazon Web Services use.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified ARN in the request doesn't exist.
* - TooManyTagsException Can occur if you specify a number of tags for a resource greater than the
* maximum 50 user tags per resource.
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* An API operation for adding one or more tags (key-value pairs) to a resource.
*
*
* You can use the TagResource
operation with a resource that already has tags. If you specify a new
* tag key for the resource, this tag is appended to the list of tags associated with the resource. If you specify a
* tag key that is already associated with the resource, the new tag value you specify replaces the previous value
* for that tag.
*
*
* Although the maximum number of array members is 200, user-tag maximum is 50. The remaining are reserved for
* Amazon Web Services use.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified ARN in the request doesn't exist.
* - TooManyTagsException Can occur if you specify a number of tags for a resource greater than the
* maximum 50 user tags per resource.
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes one or more tags from a resource. Specify only tag keys in your request. Don't specify the value.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified ARN in the request doesn't exist.
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes one or more tags from a resource. Specify only tag keys in your request. Don't specify the value.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.UntagResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified ARN in the request doesn't exist.
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates an existing cost anomaly monitor. The changes made are applied going forward, and doesn't change
* anomalies detected in the past.
*
*
* @param updateAnomalyMonitorRequest
* @return A Java Future containing the result of the UpdateAnomalyMonitor operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - UnknownMonitorException The cost anomaly monitor does not exist for the account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.UpdateAnomalyMonitor
* @see AWS API
* Documentation
*/
default CompletableFuture updateAnomalyMonitor(
UpdateAnomalyMonitorRequest updateAnomalyMonitorRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates an existing cost anomaly monitor. The changes made are applied going forward, and doesn't change
* anomalies detected in the past.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateAnomalyMonitorRequest.Builder} avoiding the
* need to create one manually via {@link UpdateAnomalyMonitorRequest#builder()}
*
*
* @param updateAnomalyMonitorRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.UpdateAnomalyMonitorRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateAnomalyMonitor operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - UnknownMonitorException The cost anomaly monitor does not exist for the account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.UpdateAnomalyMonitor
* @see AWS API
* Documentation
*/
default CompletableFuture updateAnomalyMonitor(
Consumer updateAnomalyMonitorRequest) {
return updateAnomalyMonitor(UpdateAnomalyMonitorRequest.builder().applyMutation(updateAnomalyMonitorRequest).build());
}
/**
*
* Updates an existing cost anomaly subscription. Specify the fields that you want to update. Omitted fields are
* unchanged.
*
*
*
* The JSON below describes the generic construct for each type. See Request Parameters for possible values as they apply to AnomalySubscription
.
*
*
*
* @param updateAnomalySubscriptionRequest
* @return A Java Future containing the result of the UpdateAnomalySubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - UnknownMonitorException The cost anomaly monitor does not exist for the account.
* - UnknownSubscriptionException The cost anomaly subscription does not exist for the account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.UpdateAnomalySubscription
* @see AWS
* API Documentation
*/
default CompletableFuture updateAnomalySubscription(
UpdateAnomalySubscriptionRequest updateAnomalySubscriptionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates an existing cost anomaly subscription. Specify the fields that you want to update. Omitted fields are
* unchanged.
*
*
*
* The JSON below describes the generic construct for each type. See Request Parameters for possible values as they apply to AnomalySubscription
.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateAnomalySubscriptionRequest.Builder} avoiding
* the need to create one manually via {@link UpdateAnomalySubscriptionRequest#builder()}
*
*
* @param updateAnomalySubscriptionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.UpdateAnomalySubscriptionRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the UpdateAnomalySubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - UnknownMonitorException The cost anomaly monitor does not exist for the account.
* - UnknownSubscriptionException The cost anomaly subscription does not exist for the account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.UpdateAnomalySubscription
* @see AWS
* API Documentation
*/
default CompletableFuture updateAnomalySubscription(
Consumer updateAnomalySubscriptionRequest) {
return updateAnomalySubscription(UpdateAnomalySubscriptionRequest.builder()
.applyMutation(updateAnomalySubscriptionRequest).build());
}
/**
*
* Updates status for cost allocation tags in bulk, with maximum batch size of 20. If the tag status that's updated
* is the same as the existing tag status, the request doesn't fail. Instead, it doesn't have any effect on the tag
* status (for example, activating the active tag).
*
*
* @param updateCostAllocationTagsStatusRequest
* @return A Java Future containing the result of the UpdateCostAllocationTagsStatus operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.UpdateCostAllocationTagsStatus
* @see AWS API Documentation
*/
default CompletableFuture updateCostAllocationTagsStatus(
UpdateCostAllocationTagsStatusRequest updateCostAllocationTagsStatusRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates status for cost allocation tags in bulk, with maximum batch size of 20. If the tag status that's updated
* is the same as the existing tag status, the request doesn't fail. Instead, it doesn't have any effect on the tag
* status (for example, activating the active tag).
*
*
*
* This is a convenience which creates an instance of the {@link UpdateCostAllocationTagsStatusRequest.Builder}
* avoiding the need to create one manually via {@link UpdateCostAllocationTagsStatusRequest#builder()}
*
*
* @param updateCostAllocationTagsStatusRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.UpdateCostAllocationTagsStatusRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the UpdateCostAllocationTagsStatus operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.UpdateCostAllocationTagsStatus
* @see AWS API Documentation
*/
default CompletableFuture updateCostAllocationTagsStatus(
Consumer updateCostAllocationTagsStatusRequest) {
return updateCostAllocationTagsStatus(UpdateCostAllocationTagsStatusRequest.builder()
.applyMutation(updateCostAllocationTagsStatusRequest).build());
}
/**
*
* Updates an existing Cost Category. Changes made to the Cost Category rules will be used to categorize the current
* month’s expenses and future expenses. This won’t change categorization for the previous months.
*
*
* @param updateCostCategoryDefinitionRequest
* @return A Java Future containing the result of the UpdateCostCategoryDefinition operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified ARN in the request doesn't exist.
* - ServiceQuotaExceededException You've reached the limit on the number of resources you can create, or
* exceeded the size of an individual resource.
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.UpdateCostCategoryDefinition
* @see AWS API Documentation
*/
default CompletableFuture updateCostCategoryDefinition(
UpdateCostCategoryDefinitionRequest updateCostCategoryDefinitionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates an existing Cost Category. Changes made to the Cost Category rules will be used to categorize the current
* month’s expenses and future expenses. This won’t change categorization for the previous months.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateCostCategoryDefinitionRequest.Builder}
* avoiding the need to create one manually via {@link UpdateCostCategoryDefinitionRequest#builder()}
*
*
* @param updateCostCategoryDefinitionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.costexplorer.model.UpdateCostCategoryDefinitionRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the UpdateCostCategoryDefinition operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified ARN in the request doesn't exist.
* - ServiceQuotaExceededException You've reached the limit on the number of resources you can create, or
* exceeded the size of an individual resource.
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.UpdateCostCategoryDefinition
* @see AWS API Documentation
*/
default CompletableFuture updateCostCategoryDefinition(
Consumer updateCostCategoryDefinitionRequest) {
return updateCostCategoryDefinition(UpdateCostCategoryDefinitionRequest.builder()
.applyMutation(updateCostCategoryDefinitionRequest).build());
}
@Override
default CostExplorerServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link CostExplorerAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static CostExplorerAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link CostExplorerAsyncClient}.
*/
static CostExplorerAsyncClientBuilder builder() {
return new DefaultCostExplorerAsyncClientBuilder();
}
}