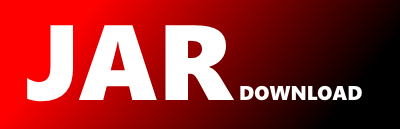
software.amazon.awssdk.services.costexplorer.model.ReservationAggregates Maven / Gradle / Ivy
Show all versions of costexplorer Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.costexplorer.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The aggregated numbers for your reservation usage.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ReservationAggregates implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField UTILIZATION_PERCENTAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UtilizationPercentage").getter(getter(ReservationAggregates::utilizationPercentage))
.setter(setter(Builder::utilizationPercentage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UtilizationPercentage").build())
.build();
private static final SdkField UTILIZATION_PERCENTAGE_IN_UNITS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("UtilizationPercentageInUnits")
.getter(getter(ReservationAggregates::utilizationPercentageInUnits))
.setter(setter(Builder::utilizationPercentageInUnits))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UtilizationPercentageInUnits")
.build()).build();
private static final SdkField PURCHASED_HOURS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PurchasedHours").getter(getter(ReservationAggregates::purchasedHours))
.setter(setter(Builder::purchasedHours))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PurchasedHours").build()).build();
private static final SdkField PURCHASED_UNITS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PurchasedUnits").getter(getter(ReservationAggregates::purchasedUnits))
.setter(setter(Builder::purchasedUnits))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PurchasedUnits").build()).build();
private static final SdkField TOTAL_ACTUAL_HOURS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TotalActualHours").getter(getter(ReservationAggregates::totalActualHours))
.setter(setter(Builder::totalActualHours))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TotalActualHours").build()).build();
private static final SdkField TOTAL_ACTUAL_UNITS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TotalActualUnits").getter(getter(ReservationAggregates::totalActualUnits))
.setter(setter(Builder::totalActualUnits))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TotalActualUnits").build()).build();
private static final SdkField UNUSED_HOURS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UnusedHours").getter(getter(ReservationAggregates::unusedHours)).setter(setter(Builder::unusedHours))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UnusedHours").build()).build();
private static final SdkField UNUSED_UNITS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UnusedUnits").getter(getter(ReservationAggregates::unusedUnits)).setter(setter(Builder::unusedUnits))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UnusedUnits").build()).build();
private static final SdkField ON_DEMAND_COST_OF_RI_HOURS_USED_FIELD = SdkField
. builder(MarshallingType.STRING).memberName("OnDemandCostOfRIHoursUsed")
.getter(getter(ReservationAggregates::onDemandCostOfRIHoursUsed)).setter(setter(Builder::onDemandCostOfRIHoursUsed))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OnDemandCostOfRIHoursUsed").build())
.build();
private static final SdkField NET_RI_SAVINGS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NetRISavings").getter(getter(ReservationAggregates::netRISavings)).setter(setter(Builder::netRISavings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NetRISavings").build()).build();
private static final SdkField TOTAL_POTENTIAL_RI_SAVINGS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TotalPotentialRISavings").getter(getter(ReservationAggregates::totalPotentialRISavings))
.setter(setter(Builder::totalPotentialRISavings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TotalPotentialRISavings").build())
.build();
private static final SdkField AMORTIZED_UPFRONT_FEE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AmortizedUpfrontFee").getter(getter(ReservationAggregates::amortizedUpfrontFee))
.setter(setter(Builder::amortizedUpfrontFee))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AmortizedUpfrontFee").build())
.build();
private static final SdkField AMORTIZED_RECURRING_FEE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AmortizedRecurringFee").getter(getter(ReservationAggregates::amortizedRecurringFee))
.setter(setter(Builder::amortizedRecurringFee))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AmortizedRecurringFee").build())
.build();
private static final SdkField TOTAL_AMORTIZED_FEE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TotalAmortizedFee").getter(getter(ReservationAggregates::totalAmortizedFee))
.setter(setter(Builder::totalAmortizedFee))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TotalAmortizedFee").build()).build();
private static final SdkField RI_COST_FOR_UNUSED_HOURS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RICostForUnusedHours").getter(getter(ReservationAggregates::riCostForUnusedHours))
.setter(setter(Builder::riCostForUnusedHours))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RICostForUnusedHours").build())
.build();
private static final SdkField REALIZED_SAVINGS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RealizedSavings").getter(getter(ReservationAggregates::realizedSavings))
.setter(setter(Builder::realizedSavings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RealizedSavings").build()).build();
private static final SdkField UNREALIZED_SAVINGS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UnrealizedSavings").getter(getter(ReservationAggregates::unrealizedSavings))
.setter(setter(Builder::unrealizedSavings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UnrealizedSavings").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(UTILIZATION_PERCENTAGE_FIELD,
UTILIZATION_PERCENTAGE_IN_UNITS_FIELD, PURCHASED_HOURS_FIELD, PURCHASED_UNITS_FIELD, TOTAL_ACTUAL_HOURS_FIELD,
TOTAL_ACTUAL_UNITS_FIELD, UNUSED_HOURS_FIELD, UNUSED_UNITS_FIELD, ON_DEMAND_COST_OF_RI_HOURS_USED_FIELD,
NET_RI_SAVINGS_FIELD, TOTAL_POTENTIAL_RI_SAVINGS_FIELD, AMORTIZED_UPFRONT_FEE_FIELD, AMORTIZED_RECURRING_FEE_FIELD,
TOTAL_AMORTIZED_FEE_FIELD, RI_COST_FOR_UNUSED_HOURS_FIELD, REALIZED_SAVINGS_FIELD, UNREALIZED_SAVINGS_FIELD));
private static final long serialVersionUID = 1L;
private final String utilizationPercentage;
private final String utilizationPercentageInUnits;
private final String purchasedHours;
private final String purchasedUnits;
private final String totalActualHours;
private final String totalActualUnits;
private final String unusedHours;
private final String unusedUnits;
private final String onDemandCostOfRIHoursUsed;
private final String netRISavings;
private final String totalPotentialRISavings;
private final String amortizedUpfrontFee;
private final String amortizedRecurringFee;
private final String totalAmortizedFee;
private final String riCostForUnusedHours;
private final String realizedSavings;
private final String unrealizedSavings;
private ReservationAggregates(BuilderImpl builder) {
this.utilizationPercentage = builder.utilizationPercentage;
this.utilizationPercentageInUnits = builder.utilizationPercentageInUnits;
this.purchasedHours = builder.purchasedHours;
this.purchasedUnits = builder.purchasedUnits;
this.totalActualHours = builder.totalActualHours;
this.totalActualUnits = builder.totalActualUnits;
this.unusedHours = builder.unusedHours;
this.unusedUnits = builder.unusedUnits;
this.onDemandCostOfRIHoursUsed = builder.onDemandCostOfRIHoursUsed;
this.netRISavings = builder.netRISavings;
this.totalPotentialRISavings = builder.totalPotentialRISavings;
this.amortizedUpfrontFee = builder.amortizedUpfrontFee;
this.amortizedRecurringFee = builder.amortizedRecurringFee;
this.totalAmortizedFee = builder.totalAmortizedFee;
this.riCostForUnusedHours = builder.riCostForUnusedHours;
this.realizedSavings = builder.realizedSavings;
this.unrealizedSavings = builder.unrealizedSavings;
}
/**
*
* The percentage of reservation time that you used.
*
*
* @return The percentage of reservation time that you used.
*/
public final String utilizationPercentage() {
return utilizationPercentage;
}
/**
*
* The percentage of Amazon EC2 reservation time that you used. It's converted to normalized units. Normalized units
* are available only for Amazon EC2 usage after November 11, 2017.
*
*
* @return The percentage of Amazon EC2 reservation time that you used. It's converted to normalized units.
* Normalized units are available only for Amazon EC2 usage after November 11, 2017.
*/
public final String utilizationPercentageInUnits() {
return utilizationPercentageInUnits;
}
/**
*
* How many reservation hours that you purchased.
*
*
* @return How many reservation hours that you purchased.
*/
public final String purchasedHours() {
return purchasedHours;
}
/**
*
* The number of Amazon EC2 reservation hours that you purchased. It's converted to normalized units. Normalized
* units are available only for Amazon EC2 usage after November 11, 2017.
*
*
* @return The number of Amazon EC2 reservation hours that you purchased. It's converted to normalized units.
* Normalized units are available only for Amazon EC2 usage after November 11, 2017.
*/
public final String purchasedUnits() {
return purchasedUnits;
}
/**
*
* The total number of reservation hours that you used.
*
*
* @return The total number of reservation hours that you used.
*/
public final String totalActualHours() {
return totalActualHours;
}
/**
*
* The total number of Amazon EC2 reservation hours that you used. It's converted to normalized units. Normalized
* units are available only for Amazon EC2 usage after November 11, 2017.
*
*
* @return The total number of Amazon EC2 reservation hours that you used. It's converted to normalized units.
* Normalized units are available only for Amazon EC2 usage after November 11, 2017.
*/
public final String totalActualUnits() {
return totalActualUnits;
}
/**
*
* The number of reservation hours that you didn't use.
*
*
* @return The number of reservation hours that you didn't use.
*/
public final String unusedHours() {
return unusedHours;
}
/**
*
* The number of Amazon EC2 reservation hours that you didn't use. It's converted to normalized units. Normalized
* units are available only for Amazon EC2 usage after November 11, 2017.
*
*
* @return The number of Amazon EC2 reservation hours that you didn't use. It's converted to normalized units.
* Normalized units are available only for Amazon EC2 usage after November 11, 2017.
*/
public final String unusedUnits() {
return unusedUnits;
}
/**
*
* How much your reservation costs if charged On-Demand rates.
*
*
* @return How much your reservation costs if charged On-Demand rates.
*/
public final String onDemandCostOfRIHoursUsed() {
return onDemandCostOfRIHoursUsed;
}
/**
*
* How much you saved due to purchasing and utilizing reservation. Amazon Web Services calculates this by
* subtracting TotalAmortizedFee
from OnDemandCostOfRIHoursUsed
.
*
*
* @return How much you saved due to purchasing and utilizing reservation. Amazon Web Services calculates this by
* subtracting TotalAmortizedFee
from OnDemandCostOfRIHoursUsed
.
*/
public final String netRISavings() {
return netRISavings;
}
/**
*
* How much you might save if you use your entire reservation.
*
*
* @return How much you might save if you use your entire reservation.
*/
public final String totalPotentialRISavings() {
return totalPotentialRISavings;
}
/**
*
* The upfront cost of your reservation. It's amortized over the reservation period.
*
*
* @return The upfront cost of your reservation. It's amortized over the reservation period.
*/
public final String amortizedUpfrontFee() {
return amortizedUpfrontFee;
}
/**
*
* The monthly cost of your reservation. It's amortized over the reservation period.
*
*
* @return The monthly cost of your reservation. It's amortized over the reservation period.
*/
public final String amortizedRecurringFee() {
return amortizedRecurringFee;
}
/**
*
* The total cost of your reservation. It's amortized over the reservation period.
*
*
* @return The total cost of your reservation. It's amortized over the reservation period.
*/
public final String totalAmortizedFee() {
return totalAmortizedFee;
}
/**
*
* The cost of unused hours for your reservation.
*
*
* @return The cost of unused hours for your reservation.
*/
public final String riCostForUnusedHours() {
return riCostForUnusedHours;
}
/**
*
* The realized savings because of purchasing and using a reservation.
*
*
* @return The realized savings because of purchasing and using a reservation.
*/
public final String realizedSavings() {
return realizedSavings;
}
/**
*
* The unrealized savings because of purchasing and using a reservation.
*
*
* @return The unrealized savings because of purchasing and using a reservation.
*/
public final String unrealizedSavings() {
return unrealizedSavings;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(utilizationPercentage());
hashCode = 31 * hashCode + Objects.hashCode(utilizationPercentageInUnits());
hashCode = 31 * hashCode + Objects.hashCode(purchasedHours());
hashCode = 31 * hashCode + Objects.hashCode(purchasedUnits());
hashCode = 31 * hashCode + Objects.hashCode(totalActualHours());
hashCode = 31 * hashCode + Objects.hashCode(totalActualUnits());
hashCode = 31 * hashCode + Objects.hashCode(unusedHours());
hashCode = 31 * hashCode + Objects.hashCode(unusedUnits());
hashCode = 31 * hashCode + Objects.hashCode(onDemandCostOfRIHoursUsed());
hashCode = 31 * hashCode + Objects.hashCode(netRISavings());
hashCode = 31 * hashCode + Objects.hashCode(totalPotentialRISavings());
hashCode = 31 * hashCode + Objects.hashCode(amortizedUpfrontFee());
hashCode = 31 * hashCode + Objects.hashCode(amortizedRecurringFee());
hashCode = 31 * hashCode + Objects.hashCode(totalAmortizedFee());
hashCode = 31 * hashCode + Objects.hashCode(riCostForUnusedHours());
hashCode = 31 * hashCode + Objects.hashCode(realizedSavings());
hashCode = 31 * hashCode + Objects.hashCode(unrealizedSavings());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ReservationAggregates)) {
return false;
}
ReservationAggregates other = (ReservationAggregates) obj;
return Objects.equals(utilizationPercentage(), other.utilizationPercentage())
&& Objects.equals(utilizationPercentageInUnits(), other.utilizationPercentageInUnits())
&& Objects.equals(purchasedHours(), other.purchasedHours())
&& Objects.equals(purchasedUnits(), other.purchasedUnits())
&& Objects.equals(totalActualHours(), other.totalActualHours())
&& Objects.equals(totalActualUnits(), other.totalActualUnits())
&& Objects.equals(unusedHours(), other.unusedHours()) && Objects.equals(unusedUnits(), other.unusedUnits())
&& Objects.equals(onDemandCostOfRIHoursUsed(), other.onDemandCostOfRIHoursUsed())
&& Objects.equals(netRISavings(), other.netRISavings())
&& Objects.equals(totalPotentialRISavings(), other.totalPotentialRISavings())
&& Objects.equals(amortizedUpfrontFee(), other.amortizedUpfrontFee())
&& Objects.equals(amortizedRecurringFee(), other.amortizedRecurringFee())
&& Objects.equals(totalAmortizedFee(), other.totalAmortizedFee())
&& Objects.equals(riCostForUnusedHours(), other.riCostForUnusedHours())
&& Objects.equals(realizedSavings(), other.realizedSavings())
&& Objects.equals(unrealizedSavings(), other.unrealizedSavings());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ReservationAggregates").add("UtilizationPercentage", utilizationPercentage())
.add("UtilizationPercentageInUnits", utilizationPercentageInUnits()).add("PurchasedHours", purchasedHours())
.add("PurchasedUnits", purchasedUnits()).add("TotalActualHours", totalActualHours())
.add("TotalActualUnits", totalActualUnits()).add("UnusedHours", unusedHours()).add("UnusedUnits", unusedUnits())
.add("OnDemandCostOfRIHoursUsed", onDemandCostOfRIHoursUsed()).add("NetRISavings", netRISavings())
.add("TotalPotentialRISavings", totalPotentialRISavings()).add("AmortizedUpfrontFee", amortizedUpfrontFee())
.add("AmortizedRecurringFee", amortizedRecurringFee()).add("TotalAmortizedFee", totalAmortizedFee())
.add("RICostForUnusedHours", riCostForUnusedHours()).add("RealizedSavings", realizedSavings())
.add("UnrealizedSavings", unrealizedSavings()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "UtilizationPercentage":
return Optional.ofNullable(clazz.cast(utilizationPercentage()));
case "UtilizationPercentageInUnits":
return Optional.ofNullable(clazz.cast(utilizationPercentageInUnits()));
case "PurchasedHours":
return Optional.ofNullable(clazz.cast(purchasedHours()));
case "PurchasedUnits":
return Optional.ofNullable(clazz.cast(purchasedUnits()));
case "TotalActualHours":
return Optional.ofNullable(clazz.cast(totalActualHours()));
case "TotalActualUnits":
return Optional.ofNullable(clazz.cast(totalActualUnits()));
case "UnusedHours":
return Optional.ofNullable(clazz.cast(unusedHours()));
case "UnusedUnits":
return Optional.ofNullable(clazz.cast(unusedUnits()));
case "OnDemandCostOfRIHoursUsed":
return Optional.ofNullable(clazz.cast(onDemandCostOfRIHoursUsed()));
case "NetRISavings":
return Optional.ofNullable(clazz.cast(netRISavings()));
case "TotalPotentialRISavings":
return Optional.ofNullable(clazz.cast(totalPotentialRISavings()));
case "AmortizedUpfrontFee":
return Optional.ofNullable(clazz.cast(amortizedUpfrontFee()));
case "AmortizedRecurringFee":
return Optional.ofNullable(clazz.cast(amortizedRecurringFee()));
case "TotalAmortizedFee":
return Optional.ofNullable(clazz.cast(totalAmortizedFee()));
case "RICostForUnusedHours":
return Optional.ofNullable(clazz.cast(riCostForUnusedHours()));
case "RealizedSavings":
return Optional.ofNullable(clazz.cast(realizedSavings()));
case "UnrealizedSavings":
return Optional.ofNullable(clazz.cast(unrealizedSavings()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function