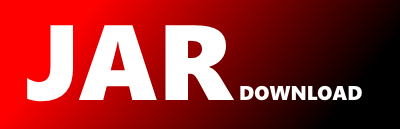
software.amazon.awssdk.services.costexplorer.CostExplorerAsyncClient Maven / Gradle / Ivy
Show all versions of costexplorer Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.costexplorer;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.costexplorer.model.GetCostAndUsageRequest;
import software.amazon.awssdk.services.costexplorer.model.GetCostAndUsageResponse;
import software.amazon.awssdk.services.costexplorer.model.GetCostForecastRequest;
import software.amazon.awssdk.services.costexplorer.model.GetCostForecastResponse;
import software.amazon.awssdk.services.costexplorer.model.GetDimensionValuesRequest;
import software.amazon.awssdk.services.costexplorer.model.GetDimensionValuesResponse;
import software.amazon.awssdk.services.costexplorer.model.GetReservationCoverageRequest;
import software.amazon.awssdk.services.costexplorer.model.GetReservationCoverageResponse;
import software.amazon.awssdk.services.costexplorer.model.GetReservationPurchaseRecommendationRequest;
import software.amazon.awssdk.services.costexplorer.model.GetReservationPurchaseRecommendationResponse;
import software.amazon.awssdk.services.costexplorer.model.GetReservationUtilizationRequest;
import software.amazon.awssdk.services.costexplorer.model.GetReservationUtilizationResponse;
import software.amazon.awssdk.services.costexplorer.model.GetTagsRequest;
import software.amazon.awssdk.services.costexplorer.model.GetTagsResponse;
/**
* Service client for accessing AWS Cost Explorer asynchronously. This can be created using the static
* {@link #builder()} method.
*
*
* The Cost Explorer API enables you to programmatically query your cost and usage data. You can query for aggregated
* data such as total monthly costs or total daily usage. You can also query for granular data, such as the number of
* daily write operations for Amazon DynamoDB database tables in your production environment.
*
*
* Service Endpoint
*
*
* The Cost Explorer API provides the following endpoint:
*
*
* -
*
* https://ce.us-east-1.amazonaws.com
*
*
*
*
* For information about costs associated with the Cost Explorer API, see AWS Cost Management Pricing.
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface CostExplorerAsyncClient extends SdkClient {
String SERVICE_NAME = "ce";
/**
* Create a {@link CostExplorerAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static CostExplorerAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link CostExplorerAsyncClient}.
*/
static CostExplorerAsyncClientBuilder builder() {
return new DefaultCostExplorerAsyncClientBuilder();
}
/**
*
* Retrieves cost and usage metrics for your account. You can specify which cost and usage-related metric, such as
* BlendedCosts
or UsageQuantity
, that you want the request to return. You can also filter
* and group your data by various dimensions, such as SERVICE
or AZ
, in a specific time
* range. For a complete list of valid dimensions, see the GetDimensionValues operation. Master accounts in an organization in AWS Organizations have access to all
* member accounts.
*
*
* @param getCostAndUsageRequest
* @return A Java Future containing the result of the GetCostAndUsage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - BillExpirationException The requested report expired. Update the date interval and try again.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - RequestChangedException Your request parameters changed between pages. Try again with the old
* parameters or without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetCostAndUsage
* @see AWS API
* Documentation
*/
default CompletableFuture getCostAndUsage(GetCostAndUsageRequest getCostAndUsageRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves cost and usage metrics for your account. You can specify which cost and usage-related metric, such as
* BlendedCosts
or UsageQuantity
, that you want the request to return. You can also filter
* and group your data by various dimensions, such as SERVICE
or AZ
, in a specific time
* range. For a complete list of valid dimensions, see the GetDimensionValues operation. Master accounts in an organization in AWS Organizations have access to all
* member accounts.
*
*
*
* This is a convenience which creates an instance of the {@link GetCostAndUsageRequest.Builder} avoiding the need
* to create one manually via {@link GetCostAndUsageRequest#builder()}
*
*
* @param getCostAndUsageRequest
* A {@link Consumer} that will call methods on {@link GetCostAndUsageRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetCostAndUsage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - BillExpirationException The requested report expired. Update the date interval and try again.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - RequestChangedException Your request parameters changed between pages. Try again with the old
* parameters or without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetCostAndUsage
* @see AWS API
* Documentation
*/
default CompletableFuture getCostAndUsage(
Consumer getCostAndUsageRequest) {
return getCostAndUsage(GetCostAndUsageRequest.builder().applyMutation(getCostAndUsageRequest).build());
}
/**
*
* Retrieves a forecast for how much Amazon Web Services predicts that you will spend over the forecast time period
* that you select, based on your past costs.
*
*
* @param getCostForecastRequest
* @return A Java Future containing the result of the GetCostForecast operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetCostForecast
* @see AWS API
* Documentation
*/
default CompletableFuture getCostForecast(GetCostForecastRequest getCostForecastRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a forecast for how much Amazon Web Services predicts that you will spend over the forecast time period
* that you select, based on your past costs.
*
*
*
* This is a convenience which creates an instance of the {@link GetCostForecastRequest.Builder} avoiding the need
* to create one manually via {@link GetCostForecastRequest#builder()}
*
*
* @param getCostForecastRequest
* A {@link Consumer} that will call methods on {@link GetCostForecastRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetCostForecast operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetCostForecast
* @see AWS API
* Documentation
*/
default CompletableFuture getCostForecast(
Consumer getCostForecastRequest) {
return getCostForecast(GetCostForecastRequest.builder().applyMutation(getCostForecastRequest).build());
}
/**
*
* Retrieves all available filter values for a specified filter over a period of time. You can search the dimension
* values for an arbitrary string.
*
*
* @param getDimensionValuesRequest
* @return A Java Future containing the result of the GetDimensionValues operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - BillExpirationException The requested report expired. Update the date interval and try again.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - RequestChangedException Your request parameters changed between pages. Try again with the old
* parameters or without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetDimensionValues
* @see AWS API
* Documentation
*/
default CompletableFuture getDimensionValues(GetDimensionValuesRequest getDimensionValuesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves all available filter values for a specified filter over a period of time. You can search the dimension
* values for an arbitrary string.
*
*
*
* This is a convenience which creates an instance of the {@link GetDimensionValuesRequest.Builder} avoiding the
* need to create one manually via {@link GetDimensionValuesRequest#builder()}
*
*
* @param getDimensionValuesRequest
* A {@link Consumer} that will call methods on {@link GetDimensionValuesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetDimensionValues operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - BillExpirationException The requested report expired. Update the date interval and try again.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - RequestChangedException Your request parameters changed between pages. Try again with the old
* parameters or without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetDimensionValues
* @see AWS API
* Documentation
*/
default CompletableFuture getDimensionValues(
Consumer getDimensionValuesRequest) {
return getDimensionValues(GetDimensionValuesRequest.builder().applyMutation(getDimensionValuesRequest).build());
}
/**
*
* Retrieves the reservation coverage for your account. This enables you to see how much of your Amazon Elastic
* Compute Cloud, Amazon ElastiCache, Amazon Relational Database Service, or Amazon Redshift usage is covered by a
* reservation. An organization's master account can see the coverage of the associated member accounts. For any
* time period, you can filter data about reservation usage by the following dimensions:
*
*
* -
*
* AZ
*
*
* -
*
* CACHE_ENGINE
*
*
* -
*
* DATABASE_ENGINE
*
*
* -
*
* DEPLOYMENT_OPTION
*
*
* -
*
* INSTANCE_TYPE
*
*
* -
*
* LINKED_ACCOUNT
*
*
* -
*
* OPERATING_SYSTEM
*
*
* -
*
* PLATFORM
*
*
* -
*
* REGION
*
*
* -
*
* SERVICE
*
*
* -
*
* TAG
*
*
* -
*
* TENANCY
*
*
*
*
* To determine valid values for a dimension, use the GetDimensionValues
operation.
*
*
* @param getReservationCoverageRequest
* You can use the following request parameters to query for how much of your instance usage a reservation
* covered.
* @return A Java Future containing the result of the GetReservationCoverage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetReservationCoverage
* @see AWS API
* Documentation
*/
default CompletableFuture getReservationCoverage(
GetReservationCoverageRequest getReservationCoverageRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the reservation coverage for your account. This enables you to see how much of your Amazon Elastic
* Compute Cloud, Amazon ElastiCache, Amazon Relational Database Service, or Amazon Redshift usage is covered by a
* reservation. An organization's master account can see the coverage of the associated member accounts. For any
* time period, you can filter data about reservation usage by the following dimensions:
*
*
* -
*
* AZ
*
*
* -
*
* CACHE_ENGINE
*
*
* -
*
* DATABASE_ENGINE
*
*
* -
*
* DEPLOYMENT_OPTION
*
*
* -
*
* INSTANCE_TYPE
*
*
* -
*
* LINKED_ACCOUNT
*
*
* -
*
* OPERATING_SYSTEM
*
*
* -
*
* PLATFORM
*
*
* -
*
* REGION
*
*
* -
*
* SERVICE
*
*
* -
*
* TAG
*
*
* -
*
* TENANCY
*
*
*
*
* To determine valid values for a dimension, use the GetDimensionValues
operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetReservationCoverageRequest.Builder} avoiding the
* need to create one manually via {@link GetReservationCoverageRequest#builder()}
*
*
* @param getReservationCoverageRequest
* A {@link Consumer} that will call methods on {@link GetReservationCoverageRequest.Builder} to create a
* request. You can use the following request parameters to query for how much of your instance usage a
* reservation covered.
* @return A Java Future containing the result of the GetReservationCoverage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetReservationCoverage
* @see AWS API
* Documentation
*/
default CompletableFuture getReservationCoverage(
Consumer getReservationCoverageRequest) {
return getReservationCoverage(GetReservationCoverageRequest.builder().applyMutation(getReservationCoverageRequest)
.build());
}
/**
*
* Gets recommendations for which reservations to purchase. These recommendations could help you reduce your costs.
* Reservations provide a discounted hourly rate (up to 75%) compared to On-Demand pricing.
*
*
* AWS generates your recommendations by identifying your On-Demand usage during a specific time period and
* collecting your usage into categories that are eligible for a reservation. After AWS has these categories, it
* simulates every combination of reservations in each category of usage to identify the best number of each type of
* RI to purchase to maximize your estimated savings.
*
*
* For example, AWS automatically aggregates your Amazon EC2 Linux, shared tenancy, and c4 family usage in the US
* West (Oregon) Region and recommends that you buy size-flexible regional reservations to apply to the c4 family
* usage. AWS recommends the smallest size instance in an instance family. This makes it easier to purchase a
* size-flexible RI. AWS also shows the equal number of normalized units so that you can purchase any instance size
* that you want. For this example, your RI recommendation would be for c4.large
because that is the
* smallest size instance in the c4 instance family.
*
*
* @param getReservationPurchaseRecommendationRequest
* @return A Java Future containing the result of the GetReservationPurchaseRecommendation operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetReservationPurchaseRecommendation
* @see AWS API Documentation
*/
default CompletableFuture getReservationPurchaseRecommendation(
GetReservationPurchaseRecommendationRequest getReservationPurchaseRecommendationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets recommendations for which reservations to purchase. These recommendations could help you reduce your costs.
* Reservations provide a discounted hourly rate (up to 75%) compared to On-Demand pricing.
*
*
* AWS generates your recommendations by identifying your On-Demand usage during a specific time period and
* collecting your usage into categories that are eligible for a reservation. After AWS has these categories, it
* simulates every combination of reservations in each category of usage to identify the best number of each type of
* RI to purchase to maximize your estimated savings.
*
*
* For example, AWS automatically aggregates your Amazon EC2 Linux, shared tenancy, and c4 family usage in the US
* West (Oregon) Region and recommends that you buy size-flexible regional reservations to apply to the c4 family
* usage. AWS recommends the smallest size instance in an instance family. This makes it easier to purchase a
* size-flexible RI. AWS also shows the equal number of normalized units so that you can purchase any instance size
* that you want. For this example, your RI recommendation would be for c4.large
because that is the
* smallest size instance in the c4 instance family.
*
*
*
* This is a convenience which creates an instance of the
* {@link GetReservationPurchaseRecommendationRequest.Builder} avoiding the need to create one manually via
* {@link GetReservationPurchaseRecommendationRequest#builder()}
*
*
* @param getReservationPurchaseRecommendationRequest
* A {@link Consumer} that will call methods on {@link GetReservationPurchaseRecommendationRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the GetReservationPurchaseRecommendation operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetReservationPurchaseRecommendation
* @see AWS API Documentation
*/
default CompletableFuture getReservationPurchaseRecommendation(
Consumer getReservationPurchaseRecommendationRequest) {
return getReservationPurchaseRecommendation(GetReservationPurchaseRecommendationRequest.builder()
.applyMutation(getReservationPurchaseRecommendationRequest).build());
}
/**
*
* Retrieves the reservation utilization for your account. Master accounts in an organization have access to member
* accounts. You can filter data by dimensions in a time period. You can use GetDimensionValues
to
* determine the possible dimension values. Currently, you can group only by SUBSCRIPTION_ID
.
*
*
* @param getReservationUtilizationRequest
* @return A Java Future containing the result of the GetReservationUtilization operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetReservationUtilization
* @see AWS
* API Documentation
*/
default CompletableFuture getReservationUtilization(
GetReservationUtilizationRequest getReservationUtilizationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the reservation utilization for your account. Master accounts in an organization have access to member
* accounts. You can filter data by dimensions in a time period. You can use GetDimensionValues
to
* determine the possible dimension values. Currently, you can group only by SUBSCRIPTION_ID
.
*
*
*
* This is a convenience which creates an instance of the {@link GetReservationUtilizationRequest.Builder} avoiding
* the need to create one manually via {@link GetReservationUtilizationRequest#builder()}
*
*
* @param getReservationUtilizationRequest
* A {@link Consumer} that will call methods on {@link GetReservationUtilizationRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetReservationUtilization operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetReservationUtilization
* @see AWS
* API Documentation
*/
default CompletableFuture getReservationUtilization(
Consumer getReservationUtilizationRequest) {
return getReservationUtilization(GetReservationUtilizationRequest.builder()
.applyMutation(getReservationUtilizationRequest).build());
}
/**
*
* Queries for available tag keys and tag values for a specified period. You can search the tag values for an
* arbitrary string.
*
*
* @param getTagsRequest
* @return A Java Future containing the result of the GetTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - BillExpirationException The requested report expired. Update the date interval and try again.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - RequestChangedException Your request parameters changed between pages. Try again with the old
* parameters or without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetTags
* @see AWS API
* Documentation
*/
default CompletableFuture getTags(GetTagsRequest getTagsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Queries for available tag keys and tag values for a specified period. You can search the tag values for an
* arbitrary string.
*
*
*
* This is a convenience which creates an instance of the {@link GetTagsRequest.Builder} avoiding the need to create
* one manually via {@link GetTagsRequest#builder()}
*
*
* @param getTagsRequest
* A {@link Consumer} that will call methods on {@link GetTagsRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitExceededException You made too many calls in a short period of time. Try again later.
* - BillExpirationException The requested report expired. Update the date interval and try again.
* - DataUnavailableException The requested data is unavailable.
* - InvalidNextTokenException The pagination token is invalid. Try again without a pagination token.
* - RequestChangedException Your request parameters changed between pages. Try again with the old
* parameters or without a pagination token.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - CostExplorerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample CostExplorerAsyncClient.GetTags
* @see AWS API
* Documentation
*/
default CompletableFuture getTags(Consumer getTagsRequest) {
return getTags(GetTagsRequest.builder().applyMutation(getTagsRequest).build());
}
}