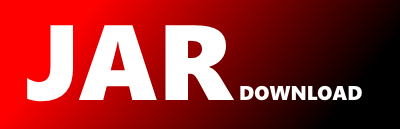
software.amazon.awssdk.services.costexplorer.DefaultCostExplorerClient Maven / Gradle / Ivy
Show all versions of costexplorer Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.costexplorer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.costexplorer.model.BillExpirationException;
import software.amazon.awssdk.services.costexplorer.model.CostExplorerException;
import software.amazon.awssdk.services.costexplorer.model.DataUnavailableException;
import software.amazon.awssdk.services.costexplorer.model.GetCostAndUsageRequest;
import software.amazon.awssdk.services.costexplorer.model.GetCostAndUsageResponse;
import software.amazon.awssdk.services.costexplorer.model.GetCostForecastRequest;
import software.amazon.awssdk.services.costexplorer.model.GetCostForecastResponse;
import software.amazon.awssdk.services.costexplorer.model.GetDimensionValuesRequest;
import software.amazon.awssdk.services.costexplorer.model.GetDimensionValuesResponse;
import software.amazon.awssdk.services.costexplorer.model.GetReservationCoverageRequest;
import software.amazon.awssdk.services.costexplorer.model.GetReservationCoverageResponse;
import software.amazon.awssdk.services.costexplorer.model.GetReservationPurchaseRecommendationRequest;
import software.amazon.awssdk.services.costexplorer.model.GetReservationPurchaseRecommendationResponse;
import software.amazon.awssdk.services.costexplorer.model.GetReservationUtilizationRequest;
import software.amazon.awssdk.services.costexplorer.model.GetReservationUtilizationResponse;
import software.amazon.awssdk.services.costexplorer.model.GetTagsRequest;
import software.amazon.awssdk.services.costexplorer.model.GetTagsResponse;
import software.amazon.awssdk.services.costexplorer.model.InvalidNextTokenException;
import software.amazon.awssdk.services.costexplorer.model.LimitExceededException;
import software.amazon.awssdk.services.costexplorer.model.RequestChangedException;
import software.amazon.awssdk.services.costexplorer.transform.GetCostAndUsageRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetCostForecastRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetDimensionValuesRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetReservationCoverageRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetReservationPurchaseRecommendationRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetReservationUtilizationRequestMarshaller;
import software.amazon.awssdk.services.costexplorer.transform.GetTagsRequestMarshaller;
/**
* Internal implementation of {@link CostExplorerClient}.
*
* @see CostExplorerClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultCostExplorerClient implements CostExplorerClient {
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultCostExplorerClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Retrieves cost and usage metrics for your account. You can specify which cost and usage-related metric, such as
* BlendedCosts
or UsageQuantity
, that you want the request to return. You can also filter
* and group your data by various dimensions, such as SERVICE
or AZ
, in a specific time
* range. For a complete list of valid dimensions, see the GetDimensionValues operation. Master accounts in an organization in AWS Organizations have access to all
* member accounts.
*
*
* @param getCostAndUsageRequest
* @return Result of the GetCostAndUsage operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws BillExpirationException
* The requested report expired. Update the date interval and try again.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws RequestChangedException
* Your request parameters changed between pages. Try again with the old parameters or without a pagination
* token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetCostAndUsage
* @see AWS API
* Documentation
*/
@Override
public GetCostAndUsageResponse getCostAndUsage(GetCostAndUsageRequest getCostAndUsageRequest) throws LimitExceededException,
BillExpirationException, DataUnavailableException, InvalidNextTokenException, RequestChangedException,
AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetCostAndUsageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetCostAndUsage").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getCostAndUsageRequest)
.withMarshaller(new GetCostAndUsageRequestMarshaller(protocolFactory)));
}
/**
*
* Retrieves a forecast for how much Amazon Web Services predicts that you will spend over the forecast time period
* that you select, based on your past costs.
*
*
* @param getCostForecastRequest
* @return Result of the GetCostForecast operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetCostForecast
* @see AWS API
* Documentation
*/
@Override
public GetCostForecastResponse getCostForecast(GetCostForecastRequest getCostForecastRequest) throws LimitExceededException,
DataUnavailableException, AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetCostForecastResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetCostForecast").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getCostForecastRequest)
.withMarshaller(new GetCostForecastRequestMarshaller(protocolFactory)));
}
/**
*
* Retrieves all available filter values for a specified filter over a period of time. You can search the dimension
* values for an arbitrary string.
*
*
* @param getDimensionValuesRequest
* @return Result of the GetDimensionValues operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws BillExpirationException
* The requested report expired. Update the date interval and try again.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws RequestChangedException
* Your request parameters changed between pages. Try again with the old parameters or without a pagination
* token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetDimensionValues
* @see AWS API
* Documentation
*/
@Override
public GetDimensionValuesResponse getDimensionValues(GetDimensionValuesRequest getDimensionValuesRequest)
throws LimitExceededException, BillExpirationException, DataUnavailableException, InvalidNextTokenException,
RequestChangedException, AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDimensionValuesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetDimensionValues").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getDimensionValuesRequest)
.withMarshaller(new GetDimensionValuesRequestMarshaller(protocolFactory)));
}
/**
*
* Retrieves the reservation coverage for your account. This enables you to see how much of your Amazon Elastic
* Compute Cloud, Amazon ElastiCache, Amazon Relational Database Service, or Amazon Redshift usage is covered by a
* reservation. An organization's master account can see the coverage of the associated member accounts. For any
* time period, you can filter data about reservation usage by the following dimensions:
*
*
* -
*
* AZ
*
*
* -
*
* CACHE_ENGINE
*
*
* -
*
* DATABASE_ENGINE
*
*
* -
*
* DEPLOYMENT_OPTION
*
*
* -
*
* INSTANCE_TYPE
*
*
* -
*
* LINKED_ACCOUNT
*
*
* -
*
* OPERATING_SYSTEM
*
*
* -
*
* PLATFORM
*
*
* -
*
* REGION
*
*
* -
*
* SERVICE
*
*
* -
*
* TAG
*
*
* -
*
* TENANCY
*
*
*
*
* To determine valid values for a dimension, use the GetDimensionValues
operation.
*
*
* @param getReservationCoverageRequest
* You can use the following request parameters to query for how much of your instance usage a reservation
* covered.
* @return Result of the GetReservationCoverage operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetReservationCoverage
* @see AWS API
* Documentation
*/
@Override
public GetReservationCoverageResponse getReservationCoverage(GetReservationCoverageRequest getReservationCoverageRequest)
throws LimitExceededException, DataUnavailableException, InvalidNextTokenException, AwsServiceException,
SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetReservationCoverageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetReservationCoverage").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getReservationCoverageRequest)
.withMarshaller(new GetReservationCoverageRequestMarshaller(protocolFactory)));
}
/**
*
* Gets recommendations for which reservations to purchase. These recommendations could help you reduce your costs.
* Reservations provide a discounted hourly rate (up to 75%) compared to On-Demand pricing.
*
*
* AWS generates your recommendations by identifying your On-Demand usage during a specific time period and
* collecting your usage into categories that are eligible for a reservation. After AWS has these categories, it
* simulates every combination of reservations in each category of usage to identify the best number of each type of
* RI to purchase to maximize your estimated savings.
*
*
* For example, AWS automatically aggregates your Amazon EC2 Linux, shared tenancy, and c4 family usage in the US
* West (Oregon) Region and recommends that you buy size-flexible regional reservations to apply to the c4 family
* usage. AWS recommends the smallest size instance in an instance family. This makes it easier to purchase a
* size-flexible RI. AWS also shows the equal number of normalized units so that you can purchase any instance size
* that you want. For this example, your RI recommendation would be for c4.large
because that is the
* smallest size instance in the c4 instance family.
*
*
* @param getReservationPurchaseRecommendationRequest
* @return Result of the GetReservationPurchaseRecommendation operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetReservationPurchaseRecommendation
* @see AWS API Documentation
*/
@Override
public GetReservationPurchaseRecommendationResponse getReservationPurchaseRecommendation(
GetReservationPurchaseRecommendationRequest getReservationPurchaseRecommendationRequest)
throws LimitExceededException, DataUnavailableException, InvalidNextTokenException, AwsServiceException,
SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, GetReservationPurchaseRecommendationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetReservationPurchaseRecommendation").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getReservationPurchaseRecommendationRequest)
.withMarshaller(new GetReservationPurchaseRecommendationRequestMarshaller(protocolFactory)));
}
/**
*
* Retrieves the reservation utilization for your account. Master accounts in an organization have access to member
* accounts. You can filter data by dimensions in a time period. You can use GetDimensionValues
to
* determine the possible dimension values. Currently, you can group only by SUBSCRIPTION_ID
.
*
*
* @param getReservationUtilizationRequest
* @return Result of the GetReservationUtilization operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetReservationUtilization
* @see AWS
* API Documentation
*/
@Override
public GetReservationUtilizationResponse getReservationUtilization(
GetReservationUtilizationRequest getReservationUtilizationRequest) throws LimitExceededException,
DataUnavailableException, InvalidNextTokenException, AwsServiceException, SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetReservationUtilizationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetReservationUtilization").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getReservationUtilizationRequest)
.withMarshaller(new GetReservationUtilizationRequestMarshaller(protocolFactory)));
}
/**
*
* Queries for available tag keys and tag values for a specified period. You can search the tag values for an
* arbitrary string.
*
*
* @param getTagsRequest
* @return Result of the GetTags operation returned by the service.
* @throws LimitExceededException
* You made too many calls in a short period of time. Try again later.
* @throws BillExpirationException
* The requested report expired. Update the date interval and try again.
* @throws DataUnavailableException
* The requested data is unavailable.
* @throws InvalidNextTokenException
* The pagination token is invalid. Try again without a pagination token.
* @throws RequestChangedException
* Your request parameters changed between pages. Try again with the old parameters or without a pagination
* token.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws CostExplorerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample CostExplorerClient.GetTags
* @see AWS API
* Documentation
*/
@Override
public GetTagsResponse getTags(GetTagsRequest getTagsRequest) throws LimitExceededException, BillExpirationException,
DataUnavailableException, InvalidNextTokenException, RequestChangedException, AwsServiceException,
SdkClientException, CostExplorerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetTagsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
return clientHandler.execute(new ClientExecutionParams().withOperationName("GetTags")
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler).withInput(getTagsRequest)
.withMarshaller(new GetTagsRequestMarshaller(protocolFactory)));
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(CostExplorerException::builder)
.protocol(AwsJsonProtocol.AWS_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("RequestChangedException")
.exceptionBuilderSupplier(RequestChangedException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DataUnavailableException")
.exceptionBuilderSupplier(DataUnavailableException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("BillExpirationException")
.exceptionBuilderSupplier(BillExpirationException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidNextTokenException")
.exceptionBuilderSupplier(InvalidNextTokenException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LimitExceededException")
.exceptionBuilderSupplier(LimitExceededException::builder).build());
}
@Override
public void close() {
clientHandler.close();
}
}