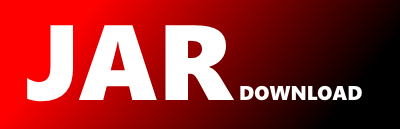
software.amazon.awssdk.services.costexplorer.model.RDSInstanceDetails Maven / Gradle / Ivy
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.costexplorer.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Details about the Amazon RDS instances that AWS recommends that you purchase.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RDSInstanceDetails implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField FAMILY_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RDSInstanceDetails::family)).setter(setter(Builder::family))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Family").build()).build();
private static final SdkField INSTANCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RDSInstanceDetails::instanceType)).setter(setter(Builder::instanceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceType").build()).build();
private static final SdkField REGION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RDSInstanceDetails::region)).setter(setter(Builder::region))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Region").build()).build();
private static final SdkField DATABASE_ENGINE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RDSInstanceDetails::databaseEngine)).setter(setter(Builder::databaseEngine))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DatabaseEngine").build()).build();
private static final SdkField DATABASE_EDITION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RDSInstanceDetails::databaseEdition)).setter(setter(Builder::databaseEdition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DatabaseEdition").build()).build();
private static final SdkField DEPLOYMENT_OPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RDSInstanceDetails::deploymentOption)).setter(setter(Builder::deploymentOption))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeploymentOption").build()).build();
private static final SdkField LICENSE_MODEL_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RDSInstanceDetails::licenseModel)).setter(setter(Builder::licenseModel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LicenseModel").build()).build();
private static final SdkField CURRENT_GENERATION_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(RDSInstanceDetails::currentGeneration)).setter(setter(Builder::currentGeneration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CurrentGeneration").build()).build();
private static final SdkField SIZE_FLEX_ELIGIBLE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(RDSInstanceDetails::sizeFlexEligible)).setter(setter(Builder::sizeFlexEligible))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SizeFlexEligible").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(FAMILY_FIELD,
INSTANCE_TYPE_FIELD, REGION_FIELD, DATABASE_ENGINE_FIELD, DATABASE_EDITION_FIELD, DEPLOYMENT_OPTION_FIELD,
LICENSE_MODEL_FIELD, CURRENT_GENERATION_FIELD, SIZE_FLEX_ELIGIBLE_FIELD));
private static final long serialVersionUID = 1L;
private final String family;
private final String instanceType;
private final String region;
private final String databaseEngine;
private final String databaseEdition;
private final String deploymentOption;
private final String licenseModel;
private final Boolean currentGeneration;
private final Boolean sizeFlexEligible;
private RDSInstanceDetails(BuilderImpl builder) {
this.family = builder.family;
this.instanceType = builder.instanceType;
this.region = builder.region;
this.databaseEngine = builder.databaseEngine;
this.databaseEdition = builder.databaseEdition;
this.deploymentOption = builder.deploymentOption;
this.licenseModel = builder.licenseModel;
this.currentGeneration = builder.currentGeneration;
this.sizeFlexEligible = builder.sizeFlexEligible;
}
/**
*
* The instance family of the recommended reservation.
*
*
* @return The instance family of the recommended reservation.
*/
public String family() {
return family;
}
/**
*
* The type of instance that AWS recommends.
*
*
* @return The type of instance that AWS recommends.
*/
public String instanceType() {
return instanceType;
}
/**
*
* The AWS Region of the recommended reservation.
*
*
* @return The AWS Region of the recommended reservation.
*/
public String region() {
return region;
}
/**
*
* The database engine that the recommended reservation supports.
*
*
* @return The database engine that the recommended reservation supports.
*/
public String databaseEngine() {
return databaseEngine;
}
/**
*
* The database edition that the recommended reservation supports.
*
*
* @return The database edition that the recommended reservation supports.
*/
public String databaseEdition() {
return databaseEdition;
}
/**
*
* Whether the recommendation is for a reservation in a single Availability Zone or a reservation with a backup in a
* second Availability Zone.
*
*
* @return Whether the recommendation is for a reservation in a single Availability Zone or a reservation with a
* backup in a second Availability Zone.
*/
public String deploymentOption() {
return deploymentOption;
}
/**
*
* The license model that the recommended reservation supports.
*
*
* @return The license model that the recommended reservation supports.
*/
public String licenseModel() {
return licenseModel;
}
/**
*
* Whether the recommendation is for a current-generation instance.
*
*
* @return Whether the recommendation is for a current-generation instance.
*/
public Boolean currentGeneration() {
return currentGeneration;
}
/**
*
* Whether the recommended reservation is size flexible.
*
*
* @return Whether the recommended reservation is size flexible.
*/
public Boolean sizeFlexEligible() {
return sizeFlexEligible;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(family());
hashCode = 31 * hashCode + Objects.hashCode(instanceType());
hashCode = 31 * hashCode + Objects.hashCode(region());
hashCode = 31 * hashCode + Objects.hashCode(databaseEngine());
hashCode = 31 * hashCode + Objects.hashCode(databaseEdition());
hashCode = 31 * hashCode + Objects.hashCode(deploymentOption());
hashCode = 31 * hashCode + Objects.hashCode(licenseModel());
hashCode = 31 * hashCode + Objects.hashCode(currentGeneration());
hashCode = 31 * hashCode + Objects.hashCode(sizeFlexEligible());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RDSInstanceDetails)) {
return false;
}
RDSInstanceDetails other = (RDSInstanceDetails) obj;
return Objects.equals(family(), other.family()) && Objects.equals(instanceType(), other.instanceType())
&& Objects.equals(region(), other.region()) && Objects.equals(databaseEngine(), other.databaseEngine())
&& Objects.equals(databaseEdition(), other.databaseEdition())
&& Objects.equals(deploymentOption(), other.deploymentOption())
&& Objects.equals(licenseModel(), other.licenseModel())
&& Objects.equals(currentGeneration(), other.currentGeneration())
&& Objects.equals(sizeFlexEligible(), other.sizeFlexEligible());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("RDSInstanceDetails").add("Family", family()).add("InstanceType", instanceType())
.add("Region", region()).add("DatabaseEngine", databaseEngine()).add("DatabaseEdition", databaseEdition())
.add("DeploymentOption", deploymentOption()).add("LicenseModel", licenseModel())
.add("CurrentGeneration", currentGeneration()).add("SizeFlexEligible", sizeFlexEligible()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Family":
return Optional.ofNullable(clazz.cast(family()));
case "InstanceType":
return Optional.ofNullable(clazz.cast(instanceType()));
case "Region":
return Optional.ofNullable(clazz.cast(region()));
case "DatabaseEngine":
return Optional.ofNullable(clazz.cast(databaseEngine()));
case "DatabaseEdition":
return Optional.ofNullable(clazz.cast(databaseEdition()));
case "DeploymentOption":
return Optional.ofNullable(clazz.cast(deploymentOption()));
case "LicenseModel":
return Optional.ofNullable(clazz.cast(licenseModel()));
case "CurrentGeneration":
return Optional.ofNullable(clazz.cast(currentGeneration()));
case "SizeFlexEligible":
return Optional.ofNullable(clazz.cast(sizeFlexEligible()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function