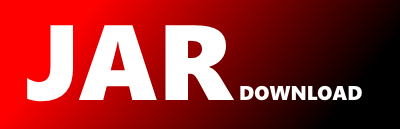
software.amazon.awssdk.services.costoptimizationhub.model.Recommendation Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.costoptimizationhub.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a recommendation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Recommendation implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ACCOUNT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("accountId").getter(getter(Recommendation::accountId)).setter(setter(Builder::accountId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("accountId").build()).build();
private static final SdkField ACTION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("actionType").getter(getter(Recommendation::actionType)).setter(setter(Builder::actionType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("actionType").build()).build();
private static final SdkField CURRENCY_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("currencyCode").getter(getter(Recommendation::currencyCode)).setter(setter(Builder::currencyCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("currencyCode").build()).build();
private static final SdkField CURRENT_RESOURCE_SUMMARY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("currentResourceSummary").getter(getter(Recommendation::currentResourceSummary))
.setter(setter(Builder::currentResourceSummary))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("currentResourceSummary").build())
.build();
private static final SdkField CURRENT_RESOURCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("currentResourceType").getter(getter(Recommendation::currentResourceType))
.setter(setter(Builder::currentResourceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("currentResourceType").build())
.build();
private static final SdkField ESTIMATED_MONTHLY_COST_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("estimatedMonthlyCost").getter(getter(Recommendation::estimatedMonthlyCost))
.setter(setter(Builder::estimatedMonthlyCost))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("estimatedMonthlyCost").build())
.build();
private static final SdkField ESTIMATED_MONTHLY_SAVINGS_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("estimatedMonthlySavings").getter(getter(Recommendation::estimatedMonthlySavings))
.setter(setter(Builder::estimatedMonthlySavings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("estimatedMonthlySavings").build())
.build();
private static final SdkField ESTIMATED_SAVINGS_PERCENTAGE_FIELD = SdkField
. builder(MarshallingType.DOUBLE)
.memberName("estimatedSavingsPercentage")
.getter(getter(Recommendation::estimatedSavingsPercentage))
.setter(setter(Builder::estimatedSavingsPercentage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("estimatedSavingsPercentage").build())
.build();
private static final SdkField IMPLEMENTATION_EFFORT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("implementationEffort").getter(getter(Recommendation::implementationEffort))
.setter(setter(Builder::implementationEffort))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("implementationEffort").build())
.build();
private static final SdkField LAST_REFRESH_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("lastRefreshTimestamp").getter(getter(Recommendation::lastRefreshTimestamp))
.setter(setter(Builder::lastRefreshTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastRefreshTimestamp").build())
.build();
private static final SdkField RECOMMENDATION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("recommendationId").getter(getter(Recommendation::recommendationId))
.setter(setter(Builder::recommendationId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("recommendationId").build()).build();
private static final SdkField RECOMMENDATION_LOOKBACK_PERIOD_IN_DAYS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("recommendationLookbackPeriodInDays")
.getter(getter(Recommendation::recommendationLookbackPeriodInDays))
.setter(setter(Builder::recommendationLookbackPeriodInDays))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("recommendationLookbackPeriodInDays")
.build()).build();
private static final SdkField RECOMMENDED_RESOURCE_SUMMARY_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("recommendedResourceSummary")
.getter(getter(Recommendation::recommendedResourceSummary))
.setter(setter(Builder::recommendedResourceSummary))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("recommendedResourceSummary").build())
.build();
private static final SdkField RECOMMENDED_RESOURCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("recommendedResourceType").getter(getter(Recommendation::recommendedResourceType))
.setter(setter(Builder::recommendedResourceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("recommendedResourceType").build())
.build();
private static final SdkField REGION_FIELD = SdkField. builder(MarshallingType.STRING).memberName("region")
.getter(getter(Recommendation::region)).setter(setter(Builder::region))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("region").build()).build();
private static final SdkField RESOURCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("resourceArn").getter(getter(Recommendation::resourceArn)).setter(setter(Builder::resourceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resourceArn").build()).build();
private static final SdkField RESOURCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("resourceId").getter(getter(Recommendation::resourceId)).setter(setter(Builder::resourceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resourceId").build()).build();
private static final SdkField RESTART_NEEDED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("restartNeeded").getter(getter(Recommendation::restartNeeded)).setter(setter(Builder::restartNeeded))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("restartNeeded").build()).build();
private static final SdkField ROLLBACK_POSSIBLE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("rollbackPossible").getter(getter(Recommendation::rollbackPossible))
.setter(setter(Builder::rollbackPossible))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("rollbackPossible").build()).build();
private static final SdkField SOURCE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("source")
.getter(getter(Recommendation::sourceAsString)).setter(setter(Builder::source))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("source").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("tags")
.getter(getter(Recommendation::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ACCOUNT_ID_FIELD,
ACTION_TYPE_FIELD, CURRENCY_CODE_FIELD, CURRENT_RESOURCE_SUMMARY_FIELD, CURRENT_RESOURCE_TYPE_FIELD,
ESTIMATED_MONTHLY_COST_FIELD, ESTIMATED_MONTHLY_SAVINGS_FIELD, ESTIMATED_SAVINGS_PERCENTAGE_FIELD,
IMPLEMENTATION_EFFORT_FIELD, LAST_REFRESH_TIMESTAMP_FIELD, RECOMMENDATION_ID_FIELD,
RECOMMENDATION_LOOKBACK_PERIOD_IN_DAYS_FIELD, RECOMMENDED_RESOURCE_SUMMARY_FIELD, RECOMMENDED_RESOURCE_TYPE_FIELD,
REGION_FIELD, RESOURCE_ARN_FIELD, RESOURCE_ID_FIELD, RESTART_NEEDED_FIELD, ROLLBACK_POSSIBLE_FIELD, SOURCE_FIELD,
TAGS_FIELD));
private static final long serialVersionUID = 1L;
private final String accountId;
private final String actionType;
private final String currencyCode;
private final String currentResourceSummary;
private final String currentResourceType;
private final Double estimatedMonthlyCost;
private final Double estimatedMonthlySavings;
private final Double estimatedSavingsPercentage;
private final String implementationEffort;
private final Instant lastRefreshTimestamp;
private final String recommendationId;
private final Integer recommendationLookbackPeriodInDays;
private final String recommendedResourceSummary;
private final String recommendedResourceType;
private final String region;
private final String resourceArn;
private final String resourceId;
private final Boolean restartNeeded;
private final Boolean rollbackPossible;
private final String source;
private final List tags;
private Recommendation(BuilderImpl builder) {
this.accountId = builder.accountId;
this.actionType = builder.actionType;
this.currencyCode = builder.currencyCode;
this.currentResourceSummary = builder.currentResourceSummary;
this.currentResourceType = builder.currentResourceType;
this.estimatedMonthlyCost = builder.estimatedMonthlyCost;
this.estimatedMonthlySavings = builder.estimatedMonthlySavings;
this.estimatedSavingsPercentage = builder.estimatedSavingsPercentage;
this.implementationEffort = builder.implementationEffort;
this.lastRefreshTimestamp = builder.lastRefreshTimestamp;
this.recommendationId = builder.recommendationId;
this.recommendationLookbackPeriodInDays = builder.recommendationLookbackPeriodInDays;
this.recommendedResourceSummary = builder.recommendedResourceSummary;
this.recommendedResourceType = builder.recommendedResourceType;
this.region = builder.region;
this.resourceArn = builder.resourceArn;
this.resourceId = builder.resourceId;
this.restartNeeded = builder.restartNeeded;
this.rollbackPossible = builder.rollbackPossible;
this.source = builder.source;
this.tags = builder.tags;
}
/**
*
* The account that the recommendation is for.
*
*
* @return The account that the recommendation is for.
*/
public final String accountId() {
return accountId;
}
/**
*
* The type of tasks that can be carried out by this action.
*
*
* @return The type of tasks that can be carried out by this action.
*/
public final String actionType() {
return actionType;
}
/**
*
* The currency code used for the recommendation.
*
*
* @return The currency code used for the recommendation.
*/
public final String currencyCode() {
return currencyCode;
}
/**
*
* Describes the current resource.
*
*
* @return Describes the current resource.
*/
public final String currentResourceSummary() {
return currentResourceSummary;
}
/**
*
* The current resource type.
*
*
* @return The current resource type.
*/
public final String currentResourceType() {
return currentResourceType;
}
/**
*
* The estimated monthly cost for the recommendation.
*
*
* @return The estimated monthly cost for the recommendation.
*/
public final Double estimatedMonthlyCost() {
return estimatedMonthlyCost;
}
/**
*
* The estimated monthly savings amount for the recommendation.
*
*
* @return The estimated monthly savings amount for the recommendation.
*/
public final Double estimatedMonthlySavings() {
return estimatedMonthlySavings;
}
/**
*
* The estimated savings percentage relative to the total cost over the cost calculation lookback period.
*
*
* @return The estimated savings percentage relative to the total cost over the cost calculation lookback period.
*/
public final Double estimatedSavingsPercentage() {
return estimatedSavingsPercentage;
}
/**
*
* The effort required to implement the recommendation.
*
*
* @return The effort required to implement the recommendation.
*/
public final String implementationEffort() {
return implementationEffort;
}
/**
*
* The time when the recommendation was last generated.
*
*
* @return The time when the recommendation was last generated.
*/
public final Instant lastRefreshTimestamp() {
return lastRefreshTimestamp;
}
/**
*
* The ID for the recommendation.
*
*
* @return The ID for the recommendation.
*/
public final String recommendationId() {
return recommendationId;
}
/**
*
* The lookback period that's used to generate the recommendation.
*
*
* @return The lookback period that's used to generate the recommendation.
*/
public final Integer recommendationLookbackPeriodInDays() {
return recommendationLookbackPeriodInDays;
}
/**
*
* Describes the recommended resource.
*
*
* @return Describes the recommended resource.
*/
public final String recommendedResourceSummary() {
return recommendedResourceSummary;
}
/**
*
* The recommended resource type.
*
*
* @return The recommended resource type.
*/
public final String recommendedResourceType() {
return recommendedResourceType;
}
/**
*
* The Amazon Web Services Region of the resource.
*
*
* @return The Amazon Web Services Region of the resource.
*/
public final String region() {
return region;
}
/**
*
* The Amazon Resource Name (ARN) for the recommendation.
*
*
* @return The Amazon Resource Name (ARN) for the recommendation.
*/
public final String resourceArn() {
return resourceArn;
}
/**
*
* The resource ID for the recommendation.
*
*
* @return The resource ID for the recommendation.
*/
public final String resourceId() {
return resourceId;
}
/**
*
* Whether or not implementing the recommendation requires a restart.
*
*
* @return Whether or not implementing the recommendation requires a restart.
*/
public final Boolean restartNeeded() {
return restartNeeded;
}
/**
*
* Whether or not implementing the recommendation can be rolled back.
*
*
* @return Whether or not implementing the recommendation can be rolled back.
*/
public final Boolean rollbackPossible() {
return rollbackPossible;
}
/**
*
* The source of the recommendation.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #source} will
* return {@link Source#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #sourceAsString}.
*
*
* @return The source of the recommendation.
* @see Source
*/
public final Source source() {
return Source.fromValue(source);
}
/**
*
* The source of the recommendation.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #source} will
* return {@link Source#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #sourceAsString}.
*
*
* @return The source of the recommendation.
* @see Source
*/
public final String sourceAsString() {
return source;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* A list of tags assigned to the recommendation.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return A list of tags assigned to the recommendation.
*/
public final List tags() {
return tags;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(accountId());
hashCode = 31 * hashCode + Objects.hashCode(actionType());
hashCode = 31 * hashCode + Objects.hashCode(currencyCode());
hashCode = 31 * hashCode + Objects.hashCode(currentResourceSummary());
hashCode = 31 * hashCode + Objects.hashCode(currentResourceType());
hashCode = 31 * hashCode + Objects.hashCode(estimatedMonthlyCost());
hashCode = 31 * hashCode + Objects.hashCode(estimatedMonthlySavings());
hashCode = 31 * hashCode + Objects.hashCode(estimatedSavingsPercentage());
hashCode = 31 * hashCode + Objects.hashCode(implementationEffort());
hashCode = 31 * hashCode + Objects.hashCode(lastRefreshTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(recommendationId());
hashCode = 31 * hashCode + Objects.hashCode(recommendationLookbackPeriodInDays());
hashCode = 31 * hashCode + Objects.hashCode(recommendedResourceSummary());
hashCode = 31 * hashCode + Objects.hashCode(recommendedResourceType());
hashCode = 31 * hashCode + Objects.hashCode(region());
hashCode = 31 * hashCode + Objects.hashCode(resourceArn());
hashCode = 31 * hashCode + Objects.hashCode(resourceId());
hashCode = 31 * hashCode + Objects.hashCode(restartNeeded());
hashCode = 31 * hashCode + Objects.hashCode(rollbackPossible());
hashCode = 31 * hashCode + Objects.hashCode(sourceAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Recommendation)) {
return false;
}
Recommendation other = (Recommendation) obj;
return Objects.equals(accountId(), other.accountId()) && Objects.equals(actionType(), other.actionType())
&& Objects.equals(currencyCode(), other.currencyCode())
&& Objects.equals(currentResourceSummary(), other.currentResourceSummary())
&& Objects.equals(currentResourceType(), other.currentResourceType())
&& Objects.equals(estimatedMonthlyCost(), other.estimatedMonthlyCost())
&& Objects.equals(estimatedMonthlySavings(), other.estimatedMonthlySavings())
&& Objects.equals(estimatedSavingsPercentage(), other.estimatedSavingsPercentage())
&& Objects.equals(implementationEffort(), other.implementationEffort())
&& Objects.equals(lastRefreshTimestamp(), other.lastRefreshTimestamp())
&& Objects.equals(recommendationId(), other.recommendationId())
&& Objects.equals(recommendationLookbackPeriodInDays(), other.recommendationLookbackPeriodInDays())
&& Objects.equals(recommendedResourceSummary(), other.recommendedResourceSummary())
&& Objects.equals(recommendedResourceType(), other.recommendedResourceType())
&& Objects.equals(region(), other.region()) && Objects.equals(resourceArn(), other.resourceArn())
&& Objects.equals(resourceId(), other.resourceId()) && Objects.equals(restartNeeded(), other.restartNeeded())
&& Objects.equals(rollbackPossible(), other.rollbackPossible())
&& Objects.equals(sourceAsString(), other.sourceAsString()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Recommendation").add("AccountId", accountId()).add("ActionType", actionType())
.add("CurrencyCode", currencyCode()).add("CurrentResourceSummary", currentResourceSummary())
.add("CurrentResourceType", currentResourceType()).add("EstimatedMonthlyCost", estimatedMonthlyCost())
.add("EstimatedMonthlySavings", estimatedMonthlySavings())
.add("EstimatedSavingsPercentage", estimatedSavingsPercentage())
.add("ImplementationEffort", implementationEffort()).add("LastRefreshTimestamp", lastRefreshTimestamp())
.add("RecommendationId", recommendationId())
.add("RecommendationLookbackPeriodInDays", recommendationLookbackPeriodInDays())
.add("RecommendedResourceSummary", recommendedResourceSummary())
.add("RecommendedResourceType", recommendedResourceType()).add("Region", region())
.add("ResourceArn", resourceArn()).add("ResourceId", resourceId()).add("RestartNeeded", restartNeeded())
.add("RollbackPossible", rollbackPossible()).add("Source", sourceAsString())
.add("Tags", hasTags() ? tags() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "accountId":
return Optional.ofNullable(clazz.cast(accountId()));
case "actionType":
return Optional.ofNullable(clazz.cast(actionType()));
case "currencyCode":
return Optional.ofNullable(clazz.cast(currencyCode()));
case "currentResourceSummary":
return Optional.ofNullable(clazz.cast(currentResourceSummary()));
case "currentResourceType":
return Optional.ofNullable(clazz.cast(currentResourceType()));
case "estimatedMonthlyCost":
return Optional.ofNullable(clazz.cast(estimatedMonthlyCost()));
case "estimatedMonthlySavings":
return Optional.ofNullable(clazz.cast(estimatedMonthlySavings()));
case "estimatedSavingsPercentage":
return Optional.ofNullable(clazz.cast(estimatedSavingsPercentage()));
case "implementationEffort":
return Optional.ofNullable(clazz.cast(implementationEffort()));
case "lastRefreshTimestamp":
return Optional.ofNullable(clazz.cast(lastRefreshTimestamp()));
case "recommendationId":
return Optional.ofNullable(clazz.cast(recommendationId()));
case "recommendationLookbackPeriodInDays":
return Optional.ofNullable(clazz.cast(recommendationLookbackPeriodInDays()));
case "recommendedResourceSummary":
return Optional.ofNullable(clazz.cast(recommendedResourceSummary()));
case "recommendedResourceType":
return Optional.ofNullable(clazz.cast(recommendedResourceType()));
case "region":
return Optional.ofNullable(clazz.cast(region()));
case "resourceArn":
return Optional.ofNullable(clazz.cast(resourceArn()));
case "resourceId":
return Optional.ofNullable(clazz.cast(resourceId()));
case "restartNeeded":
return Optional.ofNullable(clazz.cast(restartNeeded()));
case "rollbackPossible":
return Optional.ofNullable(clazz.cast(rollbackPossible()));
case "source":
return Optional.ofNullable(clazz.cast(sourceAsString()));
case "tags":
return Optional.ofNullable(clazz.cast(tags()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function