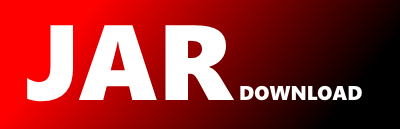
software.amazon.awssdk.services.databasemigration.model.RedshiftSettings Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.databasemigration.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides information that defines an Amazon Redshift endpoint.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RedshiftSettings implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ACCEPT_ANY_DATE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(RedshiftSettings::acceptAnyDate)).setter(setter(Builder::acceptAnyDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AcceptAnyDate").build()).build();
private static final SdkField AFTER_CONNECT_SCRIPT_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RedshiftSettings::afterConnectScript)).setter(setter(Builder::afterConnectScript))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AfterConnectScript").build())
.build();
private static final SdkField BUCKET_FOLDER_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RedshiftSettings::bucketFolder)).setter(setter(Builder::bucketFolder))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BucketFolder").build()).build();
private static final SdkField BUCKET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RedshiftSettings::bucketName)).setter(setter(Builder::bucketName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BucketName").build()).build();
private static final SdkField CONNECTION_TIMEOUT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(RedshiftSettings::connectionTimeout)).setter(setter(Builder::connectionTimeout))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConnectionTimeout").build()).build();
private static final SdkField DATABASE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RedshiftSettings::databaseName)).setter(setter(Builder::databaseName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DatabaseName").build()).build();
private static final SdkField DATE_FORMAT_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RedshiftSettings::dateFormat)).setter(setter(Builder::dateFormat))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DateFormat").build()).build();
private static final SdkField EMPTY_AS_NULL_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(RedshiftSettings::emptyAsNull)).setter(setter(Builder::emptyAsNull))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EmptyAsNull").build()).build();
private static final SdkField ENCRYPTION_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RedshiftSettings::encryptionModeAsString)).setter(setter(Builder::encryptionMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EncryptionMode").build()).build();
private static final SdkField FILE_TRANSFER_UPLOAD_STREAMS_FIELD = SdkField
. builder(MarshallingType.INTEGER).getter(getter(RedshiftSettings::fileTransferUploadStreams))
.setter(setter(Builder::fileTransferUploadStreams))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FileTransferUploadStreams").build())
.build();
private static final SdkField LOAD_TIMEOUT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(RedshiftSettings::loadTimeout)).setter(setter(Builder::loadTimeout))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LoadTimeout").build()).build();
private static final SdkField MAX_FILE_SIZE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(RedshiftSettings::maxFileSize)).setter(setter(Builder::maxFileSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxFileSize").build()).build();
private static final SdkField PASSWORD_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RedshiftSettings::password)).setter(setter(Builder::password))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Password").build()).build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(RedshiftSettings::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Port").build()).build();
private static final SdkField REMOVE_QUOTES_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(RedshiftSettings::removeQuotes)).setter(setter(Builder::removeQuotes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RemoveQuotes").build()).build();
private static final SdkField REPLACE_INVALID_CHARS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RedshiftSettings::replaceInvalidChars)).setter(setter(Builder::replaceInvalidChars))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplaceInvalidChars").build())
.build();
private static final SdkField REPLACE_CHARS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RedshiftSettings::replaceChars)).setter(setter(Builder::replaceChars))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplaceChars").build()).build();
private static final SdkField SERVER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RedshiftSettings::serverName)).setter(setter(Builder::serverName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServerName").build()).build();
private static final SdkField SERVICE_ACCESS_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RedshiftSettings::serviceAccessRoleArn)).setter(setter(Builder::serviceAccessRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceAccessRoleArn").build())
.build();
private static final SdkField SERVER_SIDE_ENCRYPTION_KMS_KEY_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(RedshiftSettings::serverSideEncryptionKmsKeyId))
.setter(setter(Builder::serverSideEncryptionKmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServerSideEncryptionKmsKeyId")
.build()).build();
private static final SdkField TIME_FORMAT_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RedshiftSettings::timeFormat)).setter(setter(Builder::timeFormat))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TimeFormat").build()).build();
private static final SdkField TRIM_BLANKS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(RedshiftSettings::trimBlanks)).setter(setter(Builder::trimBlanks))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TrimBlanks").build()).build();
private static final SdkField TRUNCATE_COLUMNS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(RedshiftSettings::truncateColumns)).setter(setter(Builder::truncateColumns))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TruncateColumns").build()).build();
private static final SdkField USERNAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RedshiftSettings::username)).setter(setter(Builder::username))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Username").build()).build();
private static final SdkField WRITE_BUFFER_SIZE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(RedshiftSettings::writeBufferSize)).setter(setter(Builder::writeBufferSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WriteBufferSize").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ACCEPT_ANY_DATE_FIELD,
AFTER_CONNECT_SCRIPT_FIELD, BUCKET_FOLDER_FIELD, BUCKET_NAME_FIELD, CONNECTION_TIMEOUT_FIELD, DATABASE_NAME_FIELD,
DATE_FORMAT_FIELD, EMPTY_AS_NULL_FIELD, ENCRYPTION_MODE_FIELD, FILE_TRANSFER_UPLOAD_STREAMS_FIELD,
LOAD_TIMEOUT_FIELD, MAX_FILE_SIZE_FIELD, PASSWORD_FIELD, PORT_FIELD, REMOVE_QUOTES_FIELD,
REPLACE_INVALID_CHARS_FIELD, REPLACE_CHARS_FIELD, SERVER_NAME_FIELD, SERVICE_ACCESS_ROLE_ARN_FIELD,
SERVER_SIDE_ENCRYPTION_KMS_KEY_ID_FIELD, TIME_FORMAT_FIELD, TRIM_BLANKS_FIELD, TRUNCATE_COLUMNS_FIELD,
USERNAME_FIELD, WRITE_BUFFER_SIZE_FIELD));
private static final long serialVersionUID = 1L;
private final Boolean acceptAnyDate;
private final String afterConnectScript;
private final String bucketFolder;
private final String bucketName;
private final Integer connectionTimeout;
private final String databaseName;
private final String dateFormat;
private final Boolean emptyAsNull;
private final String encryptionMode;
private final Integer fileTransferUploadStreams;
private final Integer loadTimeout;
private final Integer maxFileSize;
private final String password;
private final Integer port;
private final Boolean removeQuotes;
private final String replaceInvalidChars;
private final String replaceChars;
private final String serverName;
private final String serviceAccessRoleArn;
private final String serverSideEncryptionKmsKeyId;
private final String timeFormat;
private final Boolean trimBlanks;
private final Boolean truncateColumns;
private final String username;
private final Integer writeBufferSize;
private RedshiftSettings(BuilderImpl builder) {
this.acceptAnyDate = builder.acceptAnyDate;
this.afterConnectScript = builder.afterConnectScript;
this.bucketFolder = builder.bucketFolder;
this.bucketName = builder.bucketName;
this.connectionTimeout = builder.connectionTimeout;
this.databaseName = builder.databaseName;
this.dateFormat = builder.dateFormat;
this.emptyAsNull = builder.emptyAsNull;
this.encryptionMode = builder.encryptionMode;
this.fileTransferUploadStreams = builder.fileTransferUploadStreams;
this.loadTimeout = builder.loadTimeout;
this.maxFileSize = builder.maxFileSize;
this.password = builder.password;
this.port = builder.port;
this.removeQuotes = builder.removeQuotes;
this.replaceInvalidChars = builder.replaceInvalidChars;
this.replaceChars = builder.replaceChars;
this.serverName = builder.serverName;
this.serviceAccessRoleArn = builder.serviceAccessRoleArn;
this.serverSideEncryptionKmsKeyId = builder.serverSideEncryptionKmsKeyId;
this.timeFormat = builder.timeFormat;
this.trimBlanks = builder.trimBlanks;
this.truncateColumns = builder.truncateColumns;
this.username = builder.username;
this.writeBufferSize = builder.writeBufferSize;
}
/**
*
* A value that indicates to allow any date format, including invalid formats such as 00/00/00 00:00:00, to be
* loaded without generating an error. You can choose true
or false
(the default).
*
*
* This parameter applies only to TIMESTAMP and DATE columns. Always use ACCEPTANYDATE with the DATEFORMAT
* parameter. If the date format for the data doesn't match the DATEFORMAT specification, Amazon Redshift inserts a
* NULL value into that field.
*
*
* @return A value that indicates to allow any date format, including invalid formats such as 00/00/00 00:00:00, to
* be loaded without generating an error. You can choose true
or false
(the
* default).
*
* This parameter applies only to TIMESTAMP and DATE columns. Always use ACCEPTANYDATE with the DATEFORMAT
* parameter. If the date format for the data doesn't match the DATEFORMAT specification, Amazon Redshift
* inserts a NULL value into that field.
*/
public Boolean acceptAnyDate() {
return acceptAnyDate;
}
/**
*
* Code to run after connecting. This parameter should contain the code itself, not the name of a file containing
* the code.
*
*
* @return Code to run after connecting. This parameter should contain the code itself, not the name of a file
* containing the code.
*/
public String afterConnectScript() {
return afterConnectScript;
}
/**
*
* The location where the comma-separated value (.csv) files are stored before being uploaded to the S3 bucket.
*
*
* @return The location where the comma-separated value (.csv) files are stored before being uploaded to the S3
* bucket.
*/
public String bucketFolder() {
return bucketFolder;
}
/**
*
* The name of the S3 bucket you want to use
*
*
* @return The name of the S3 bucket you want to use
*/
public String bucketName() {
return bucketName;
}
/**
*
* A value that sets the amount of time to wait (in milliseconds) before timing out, beginning from when you
* initially establish a connection.
*
*
* @return A value that sets the amount of time to wait (in milliseconds) before timing out, beginning from when you
* initially establish a connection.
*/
public Integer connectionTimeout() {
return connectionTimeout;
}
/**
*
* The name of the Amazon Redshift data warehouse (service) that you are working with.
*
*
* @return The name of the Amazon Redshift data warehouse (service) that you are working with.
*/
public String databaseName() {
return databaseName;
}
/**
*
* The date format that you are using. Valid values are auto
(case-sensitive), your date format string
* enclosed in quotes, or NULL. If this parameter is left unset (NULL), it defaults to a format of 'YYYY-MM-DD'.
* Using auto
recognizes most strings, even some that aren't supported when you use a date format
* string.
*
*
* If your date and time values use formats different from each other, set this to auto
.
*
*
* @return The date format that you are using. Valid values are auto
(case-sensitive), your date format
* string enclosed in quotes, or NULL. If this parameter is left unset (NULL), it defaults to a format of
* 'YYYY-MM-DD'. Using auto
recognizes most strings, even some that aren't supported when you
* use a date format string.
*
* If your date and time values use formats different from each other, set this to auto
.
*/
public String dateFormat() {
return dateFormat;
}
/**
*
* A value that specifies whether AWS DMS should migrate empty CHAR and VARCHAR fields as NULL. A value of
* true
sets empty CHAR and VARCHAR fields to null. The default is false
.
*
*
* @return A value that specifies whether AWS DMS should migrate empty CHAR and VARCHAR fields as NULL. A value of
* true
sets empty CHAR and VARCHAR fields to null. The default is false
.
*/
public Boolean emptyAsNull() {
return emptyAsNull;
}
/**
*
* The type of server-side encryption that you want to use for your data. This encryption type is part of the
* endpoint settings or the extra connections attributes for Amazon S3. You can choose either SSE_S3
* (the default) or SSE_KMS
. To use SSE_S3
, create an AWS Identity and Access Management
* (IAM) role with a policy that allows "arn:aws:s3:::*"
to use the following actions:
* "s3:PutObject", "s3:ListBucket"
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #encryptionMode}
* will return {@link EncryptionModeValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #encryptionModeAsString}.
*
*
* @return The type of server-side encryption that you want to use for your data. This encryption type is part of
* the endpoint settings or the extra connections attributes for Amazon S3. You can choose either
* SSE_S3
(the default) or SSE_KMS
. To use SSE_S3
, create an AWS
* Identity and Access Management (IAM) role with a policy that allows "arn:aws:s3:::*"
to use
* the following actions: "s3:PutObject", "s3:ListBucket"
* @see EncryptionModeValue
*/
public EncryptionModeValue encryptionMode() {
return EncryptionModeValue.fromValue(encryptionMode);
}
/**
*
* The type of server-side encryption that you want to use for your data. This encryption type is part of the
* endpoint settings or the extra connections attributes for Amazon S3. You can choose either SSE_S3
* (the default) or SSE_KMS
. To use SSE_S3
, create an AWS Identity and Access Management
* (IAM) role with a policy that allows "arn:aws:s3:::*"
to use the following actions:
* "s3:PutObject", "s3:ListBucket"
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #encryptionMode}
* will return {@link EncryptionModeValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #encryptionModeAsString}.
*
*
* @return The type of server-side encryption that you want to use for your data. This encryption type is part of
* the endpoint settings or the extra connections attributes for Amazon S3. You can choose either
* SSE_S3
(the default) or SSE_KMS
. To use SSE_S3
, create an AWS
* Identity and Access Management (IAM) role with a policy that allows "arn:aws:s3:::*"
to use
* the following actions: "s3:PutObject", "s3:ListBucket"
* @see EncryptionModeValue
*/
public String encryptionModeAsString() {
return encryptionMode;
}
/**
*
* The number of threads used to upload a single file. This parameter accepts a value from 1 through 64. It defaults
* to 10.
*
*
* @return The number of threads used to upload a single file. This parameter accepts a value from 1 through 64. It
* defaults to 10.
*/
public Integer fileTransferUploadStreams() {
return fileTransferUploadStreams;
}
/**
*
* The amount of time to wait (in milliseconds) before timing out, beginning from when you begin loading.
*
*
* @return The amount of time to wait (in milliseconds) before timing out, beginning from when you begin loading.
*/
public Integer loadTimeout() {
return loadTimeout;
}
/**
*
* The maximum size (in KB) of any .csv file used to transfer data to Amazon Redshift. This accepts a value from 1
* through 1,048,576. It defaults to 32,768 KB (32 MB).
*
*
* @return The maximum size (in KB) of any .csv file used to transfer data to Amazon Redshift. This accepts a value
* from 1 through 1,048,576. It defaults to 32,768 KB (32 MB).
*/
public Integer maxFileSize() {
return maxFileSize;
}
/**
*
* The password for the user named in the username
property.
*
*
* @return The password for the user named in the username
property.
*/
public String password() {
return password;
}
/**
*
* The port number for Amazon Redshift. The default value is 5439.
*
*
* @return The port number for Amazon Redshift. The default value is 5439.
*/
public Integer port() {
return port;
}
/**
*
* A value that specifies to remove surrounding quotation marks from strings in the incoming data. All characters
* within the quotation marks, including delimiters, are retained. Choose true
to remove quotation
* marks. The default is false
.
*
*
* @return A value that specifies to remove surrounding quotation marks from strings in the incoming data. All
* characters within the quotation marks, including delimiters, are retained. Choose true
to
* remove quotation marks. The default is false
.
*/
public Boolean removeQuotes() {
return removeQuotes;
}
/**
*
* A list of characters that you want to replace. Use with ReplaceChars
.
*
*
* @return A list of characters that you want to replace. Use with ReplaceChars
.
*/
public String replaceInvalidChars() {
return replaceInvalidChars;
}
/**
*
* A value that specifies to replaces the invalid characters specified in ReplaceInvalidChars
,
* substituting the specified characters instead. The default is "?"
.
*
*
* @return A value that specifies to replaces the invalid characters specified in ReplaceInvalidChars
,
* substituting the specified characters instead. The default is "?"
.
*/
public String replaceChars() {
return replaceChars;
}
/**
*
* The name of the Amazon Redshift cluster you are using.
*
*
* @return The name of the Amazon Redshift cluster you are using.
*/
public String serverName() {
return serverName;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role that has access to the Amazon Redshift service.
*
*
* @return The Amazon Resource Name (ARN) of the IAM role that has access to the Amazon Redshift service.
*/
public String serviceAccessRoleArn() {
return serviceAccessRoleArn;
}
/**
*
* The AWS KMS key ID. If you are using SSE_KMS
for the EncryptionMode
, provide this key
* ID. The key that you use needs an attached policy that enables IAM user permissions and allows use of the key.
*
*
* @return The AWS KMS key ID. If you are using SSE_KMS
for the EncryptionMode
, provide
* this key ID. The key that you use needs an attached policy that enables IAM user permissions and allows
* use of the key.
*/
public String serverSideEncryptionKmsKeyId() {
return serverSideEncryptionKmsKeyId;
}
/**
*
* The time format that you want to use. Valid values are auto
(case-sensitive),
* 'timeformat_string'
, 'epochsecs'
, or 'epochmillisecs'
. It defaults to 10.
* Using auto
recognizes most strings, even some that aren't supported when you use a time format
* string.
*
*
* If your date and time values use formats different from each other, set this parameter to auto
.
*
*
* @return The time format that you want to use. Valid values are auto
(case-sensitive),
* 'timeformat_string'
, 'epochsecs'
, or 'epochmillisecs'
. It defaults
* to 10. Using auto
recognizes most strings, even some that aren't supported when you use a
* time format string.
*
* If your date and time values use formats different from each other, set this parameter to
* auto
.
*/
public String timeFormat() {
return timeFormat;
}
/**
*
* A value that specifies to remove the trailing white space characters from a VARCHAR string. This parameter
* applies only to columns with a VARCHAR data type. Choose true
to remove unneeded white space. The
* default is false
.
*
*
* @return A value that specifies to remove the trailing white space characters from a VARCHAR string. This
* parameter applies only to columns with a VARCHAR data type. Choose true
to remove unneeded
* white space. The default is false
.
*/
public Boolean trimBlanks() {
return trimBlanks;
}
/**
*
* A value that specifies to truncate data in columns to the appropriate number of characters, so that the data fits
* in the column. This parameter applies only to columns with a VARCHAR or CHAR data type, and rows with a size of 4
* MB or less. Choose true
to truncate data. The default is false
.
*
*
* @return A value that specifies to truncate data in columns to the appropriate number of characters, so that the
* data fits in the column. This parameter applies only to columns with a VARCHAR or CHAR data type, and
* rows with a size of 4 MB or less. Choose true
to truncate data. The default is
* false
.
*/
public Boolean truncateColumns() {
return truncateColumns;
}
/**
*
* An Amazon Redshift user name for a registered user.
*
*
* @return An Amazon Redshift user name for a registered user.
*/
public String username() {
return username;
}
/**
*
* The size of the write buffer to use in rows. Valid values range from 1 through 2,048. The default is 1,024. Use
* this setting to tune performance.
*
*
* @return The size of the write buffer to use in rows. Valid values range from 1 through 2,048. The default is
* 1,024. Use this setting to tune performance.
*/
public Integer writeBufferSize() {
return writeBufferSize;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(acceptAnyDate());
hashCode = 31 * hashCode + Objects.hashCode(afterConnectScript());
hashCode = 31 * hashCode + Objects.hashCode(bucketFolder());
hashCode = 31 * hashCode + Objects.hashCode(bucketName());
hashCode = 31 * hashCode + Objects.hashCode(connectionTimeout());
hashCode = 31 * hashCode + Objects.hashCode(databaseName());
hashCode = 31 * hashCode + Objects.hashCode(dateFormat());
hashCode = 31 * hashCode + Objects.hashCode(emptyAsNull());
hashCode = 31 * hashCode + Objects.hashCode(encryptionModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(fileTransferUploadStreams());
hashCode = 31 * hashCode + Objects.hashCode(loadTimeout());
hashCode = 31 * hashCode + Objects.hashCode(maxFileSize());
hashCode = 31 * hashCode + Objects.hashCode(password());
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(removeQuotes());
hashCode = 31 * hashCode + Objects.hashCode(replaceInvalidChars());
hashCode = 31 * hashCode + Objects.hashCode(replaceChars());
hashCode = 31 * hashCode + Objects.hashCode(serverName());
hashCode = 31 * hashCode + Objects.hashCode(serviceAccessRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(serverSideEncryptionKmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(timeFormat());
hashCode = 31 * hashCode + Objects.hashCode(trimBlanks());
hashCode = 31 * hashCode + Objects.hashCode(truncateColumns());
hashCode = 31 * hashCode + Objects.hashCode(username());
hashCode = 31 * hashCode + Objects.hashCode(writeBufferSize());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RedshiftSettings)) {
return false;
}
RedshiftSettings other = (RedshiftSettings) obj;
return Objects.equals(acceptAnyDate(), other.acceptAnyDate())
&& Objects.equals(afterConnectScript(), other.afterConnectScript())
&& Objects.equals(bucketFolder(), other.bucketFolder()) && Objects.equals(bucketName(), other.bucketName())
&& Objects.equals(connectionTimeout(), other.connectionTimeout())
&& Objects.equals(databaseName(), other.databaseName()) && Objects.equals(dateFormat(), other.dateFormat())
&& Objects.equals(emptyAsNull(), other.emptyAsNull())
&& Objects.equals(encryptionModeAsString(), other.encryptionModeAsString())
&& Objects.equals(fileTransferUploadStreams(), other.fileTransferUploadStreams())
&& Objects.equals(loadTimeout(), other.loadTimeout()) && Objects.equals(maxFileSize(), other.maxFileSize())
&& Objects.equals(password(), other.password()) && Objects.equals(port(), other.port())
&& Objects.equals(removeQuotes(), other.removeQuotes())
&& Objects.equals(replaceInvalidChars(), other.replaceInvalidChars())
&& Objects.equals(replaceChars(), other.replaceChars()) && Objects.equals(serverName(), other.serverName())
&& Objects.equals(serviceAccessRoleArn(), other.serviceAccessRoleArn())
&& Objects.equals(serverSideEncryptionKmsKeyId(), other.serverSideEncryptionKmsKeyId())
&& Objects.equals(timeFormat(), other.timeFormat()) && Objects.equals(trimBlanks(), other.trimBlanks())
&& Objects.equals(truncateColumns(), other.truncateColumns()) && Objects.equals(username(), other.username())
&& Objects.equals(writeBufferSize(), other.writeBufferSize());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("RedshiftSettings").add("AcceptAnyDate", acceptAnyDate())
.add("AfterConnectScript", afterConnectScript()).add("BucketFolder", bucketFolder())
.add("BucketName", bucketName()).add("ConnectionTimeout", connectionTimeout())
.add("DatabaseName", databaseName()).add("DateFormat", dateFormat()).add("EmptyAsNull", emptyAsNull())
.add("EncryptionMode", encryptionModeAsString()).add("FileTransferUploadStreams", fileTransferUploadStreams())
.add("LoadTimeout", loadTimeout()).add("MaxFileSize", maxFileSize())
.add("Password", password() == null ? null : "*** Sensitive Data Redacted ***").add("Port", port())
.add("RemoveQuotes", removeQuotes()).add("ReplaceInvalidChars", replaceInvalidChars())
.add("ReplaceChars", replaceChars()).add("ServerName", serverName())
.add("ServiceAccessRoleArn", serviceAccessRoleArn())
.add("ServerSideEncryptionKmsKeyId", serverSideEncryptionKmsKeyId()).add("TimeFormat", timeFormat())
.add("TrimBlanks", trimBlanks()).add("TruncateColumns", truncateColumns()).add("Username", username())
.add("WriteBufferSize", writeBufferSize()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AcceptAnyDate":
return Optional.ofNullable(clazz.cast(acceptAnyDate()));
case "AfterConnectScript":
return Optional.ofNullable(clazz.cast(afterConnectScript()));
case "BucketFolder":
return Optional.ofNullable(clazz.cast(bucketFolder()));
case "BucketName":
return Optional.ofNullable(clazz.cast(bucketName()));
case "ConnectionTimeout":
return Optional.ofNullable(clazz.cast(connectionTimeout()));
case "DatabaseName":
return Optional.ofNullable(clazz.cast(databaseName()));
case "DateFormat":
return Optional.ofNullable(clazz.cast(dateFormat()));
case "EmptyAsNull":
return Optional.ofNullable(clazz.cast(emptyAsNull()));
case "EncryptionMode":
return Optional.ofNullable(clazz.cast(encryptionModeAsString()));
case "FileTransferUploadStreams":
return Optional.ofNullable(clazz.cast(fileTransferUploadStreams()));
case "LoadTimeout":
return Optional.ofNullable(clazz.cast(loadTimeout()));
case "MaxFileSize":
return Optional.ofNullable(clazz.cast(maxFileSize()));
case "Password":
return Optional.ofNullable(clazz.cast(password()));
case "Port":
return Optional.ofNullable(clazz.cast(port()));
case "RemoveQuotes":
return Optional.ofNullable(clazz.cast(removeQuotes()));
case "ReplaceInvalidChars":
return Optional.ofNullable(clazz.cast(replaceInvalidChars()));
case "ReplaceChars":
return Optional.ofNullable(clazz.cast(replaceChars()));
case "ServerName":
return Optional.ofNullable(clazz.cast(serverName()));
case "ServiceAccessRoleArn":
return Optional.ofNullable(clazz.cast(serviceAccessRoleArn()));
case "ServerSideEncryptionKmsKeyId":
return Optional.ofNullable(clazz.cast(serverSideEncryptionKmsKeyId()));
case "TimeFormat":
return Optional.ofNullable(clazz.cast(timeFormat()));
case "TrimBlanks":
return Optional.ofNullable(clazz.cast(trimBlanks()));
case "TruncateColumns":
return Optional.ofNullable(clazz.cast(truncateColumns()));
case "Username":
return Optional.ofNullable(clazz.cast(username()));
case "WriteBufferSize":
return Optional.ofNullable(clazz.cast(writeBufferSize()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function