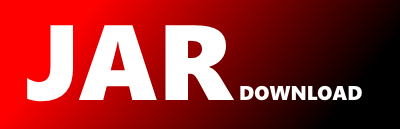
software.amazon.awssdk.services.databasemigration.model.ModifyReplicationTaskRequest Maven / Gradle / Ivy
Show all versions of databasemigration Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.databasemigration.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ModifyReplicationTaskRequest extends DatabaseMigrationRequest implements
ToCopyableBuilder {
private static final SdkField REPLICATION_TASK_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ModifyReplicationTaskRequest::replicationTaskArn)).setter(setter(Builder::replicationTaskArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationTaskArn").build())
.build();
private static final SdkField REPLICATION_TASK_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ModifyReplicationTaskRequest::replicationTaskIdentifier))
.setter(setter(Builder::replicationTaskIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationTaskIdentifier").build())
.build();
private static final SdkField MIGRATION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ModifyReplicationTaskRequest::migrationTypeAsString)).setter(setter(Builder::migrationType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MigrationType").build()).build();
private static final SdkField TABLE_MAPPINGS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ModifyReplicationTaskRequest::tableMappings)).setter(setter(Builder::tableMappings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TableMappings").build()).build();
private static final SdkField REPLICATION_TASK_SETTINGS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ModifyReplicationTaskRequest::replicationTaskSettings))
.setter(setter(Builder::replicationTaskSettings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationTaskSettings").build())
.build();
private static final SdkField CDC_START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(ModifyReplicationTaskRequest::cdcStartTime)).setter(setter(Builder::cdcStartTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CdcStartTime").build()).build();
private static final SdkField CDC_START_POSITION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ModifyReplicationTaskRequest::cdcStartPosition)).setter(setter(Builder::cdcStartPosition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CdcStartPosition").build()).build();
private static final SdkField CDC_STOP_POSITION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ModifyReplicationTaskRequest::cdcStopPosition)).setter(setter(Builder::cdcStopPosition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CdcStopPosition").build()).build();
private static final SdkField TASK_DATA_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ModifyReplicationTaskRequest::taskData)).setter(setter(Builder::taskData))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TaskData").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REPLICATION_TASK_ARN_FIELD,
REPLICATION_TASK_IDENTIFIER_FIELD, MIGRATION_TYPE_FIELD, TABLE_MAPPINGS_FIELD, REPLICATION_TASK_SETTINGS_FIELD,
CDC_START_TIME_FIELD, CDC_START_POSITION_FIELD, CDC_STOP_POSITION_FIELD, TASK_DATA_FIELD));
private final String replicationTaskArn;
private final String replicationTaskIdentifier;
private final String migrationType;
private final String tableMappings;
private final String replicationTaskSettings;
private final Instant cdcStartTime;
private final String cdcStartPosition;
private final String cdcStopPosition;
private final String taskData;
private ModifyReplicationTaskRequest(BuilderImpl builder) {
super(builder);
this.replicationTaskArn = builder.replicationTaskArn;
this.replicationTaskIdentifier = builder.replicationTaskIdentifier;
this.migrationType = builder.migrationType;
this.tableMappings = builder.tableMappings;
this.replicationTaskSettings = builder.replicationTaskSettings;
this.cdcStartTime = builder.cdcStartTime;
this.cdcStartPosition = builder.cdcStartPosition;
this.cdcStopPosition = builder.cdcStopPosition;
this.taskData = builder.taskData;
}
/**
*
* The Amazon Resource Name (ARN) of the replication task.
*
*
* @return The Amazon Resource Name (ARN) of the replication task.
*/
public String replicationTaskArn() {
return replicationTaskArn;
}
/**
*
* The replication task identifier.
*
*
* Constraints:
*
*
* -
*
* Must contain 1-255 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* @return The replication task identifier.
*
* Constraints:
*
*
* -
*
* Must contain 1-255 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*/
public String replicationTaskIdentifier() {
return replicationTaskIdentifier;
}
/**
*
* The migration type. Valid values: full-load
| cdc
| full-load-and-cdc
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #migrationType}
* will return {@link MigrationTypeValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #migrationTypeAsString}.
*
*
* @return The migration type. Valid values: full-load
| cdc
|
* full-load-and-cdc
* @see MigrationTypeValue
*/
public MigrationTypeValue migrationType() {
return MigrationTypeValue.fromValue(migrationType);
}
/**
*
* The migration type. Valid values: full-load
| cdc
| full-load-and-cdc
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #migrationType}
* will return {@link MigrationTypeValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #migrationTypeAsString}.
*
*
* @return The migration type. Valid values: full-load
| cdc
|
* full-load-and-cdc
* @see MigrationTypeValue
*/
public String migrationTypeAsString() {
return migrationType;
}
/**
*
* When using the AWS CLI or boto3, provide the path of the JSON file that contains the table mappings. Precede the
* path with file://
. When working with the DMS API, provide the JSON as the parameter value, for
* example: --table-mappings file://mappingfile.json
*
*
* @return When using the AWS CLI or boto3, provide the path of the JSON file that contains the table mappings.
* Precede the path with file://
. When working with the DMS API, provide the JSON as the
* parameter value, for example: --table-mappings file://mappingfile.json
*/
public String tableMappings() {
return tableMappings;
}
/**
*
* JSON file that contains settings for the task, such as task metadata settings.
*
*
* @return JSON file that contains settings for the task, such as task metadata settings.
*/
public String replicationTaskSettings() {
return replicationTaskSettings;
}
/**
*
* Indicates the start time for a change data capture (CDC) operation. Use either CdcStartTime or CdcStartPosition
* to specify when you want a CDC operation to start. Specifying both values results in an error.
*
*
* Timestamp Example: --cdc-start-time “2018-03-08T12:12:12”
*
*
* @return Indicates the start time for a change data capture (CDC) operation. Use either CdcStartTime or
* CdcStartPosition to specify when you want a CDC operation to start. Specifying both values results in an
* error.
*
* Timestamp Example: --cdc-start-time “2018-03-08T12:12:12”
*/
public Instant cdcStartTime() {
return cdcStartTime;
}
/**
*
* Indicates when you want a change data capture (CDC) operation to start. Use either CdcStartPosition or
* CdcStartTime to specify when you want a CDC operation to start. Specifying both values results in an error.
*
*
* The value can be in date, checkpoint, or LSN/SCN format.
*
*
* Date Example: --cdc-start-position “2018-03-08T12:12:12”
*
*
* Checkpoint Example: --cdc-start-position
* "checkpoint:V1#27#mysql-bin-changelog.157832:1975:-1:2002:677883278264080:mysql-bin-changelog.157832:1876#0#0#*#0#93"
*
*
* LSN Example: --cdc-start-position “mysql-bin-changelog.000024:373”
*
*
*
* When you use this task setting with a source PostgreSQL database, a logical replication slot should already be
* created and associated with the source endpoint. You can verify this by setting the slotName
extra
* connection attribute to the name of this logical replication slot. For more information, see Extra Connection Attributes When Using PostgreSQL as a Source for AWS DMS.
*
*
*
* @return Indicates when you want a change data capture (CDC) operation to start. Use either CdcStartPosition or
* CdcStartTime to specify when you want a CDC operation to start. Specifying both values results in an
* error.
*
* The value can be in date, checkpoint, or LSN/SCN format.
*
*
* Date Example: --cdc-start-position “2018-03-08T12:12:12”
*
*
* Checkpoint Example: --cdc-start-position
* "checkpoint:V1#27#mysql-bin-changelog.157832:1975:-1:2002:677883278264080:mysql-bin-changelog.157832:1876#0#0#*#0#93"
*
*
* LSN Example: --cdc-start-position “mysql-bin-changelog.000024:373”
*
*
*
* When you use this task setting with a source PostgreSQL database, a logical replication slot should
* already be created and associated with the source endpoint. You can verify this by setting the
* slotName
extra connection attribute to the name of this logical replication slot. For more
* information, see Extra Connection Attributes When Using PostgreSQL as a Source for AWS DMS.
*
*/
public String cdcStartPosition() {
return cdcStartPosition;
}
/**
*
* Indicates when you want a change data capture (CDC) operation to stop. The value can be either server time or
* commit time.
*
*
* Server time example: --cdc-stop-position “server_time:2018-02-09T12:12:12”
*
*
* Commit time example: --cdc-stop-position “commit_time: 2018-02-09T12:12:12 “
*
*
* @return Indicates when you want a change data capture (CDC) operation to stop. The value can be either server
* time or commit time.
*
* Server time example: --cdc-stop-position “server_time:2018-02-09T12:12:12”
*
*
* Commit time example: --cdc-stop-position “commit_time: 2018-02-09T12:12:12 “
*/
public String cdcStopPosition() {
return cdcStopPosition;
}
/**
*
* Supplemental information that the task requires to migrate the data for certain source and target endpoints. For
* more information, see Specifying Supplemental Data for
* Task Settings in the AWS Database Migration Service User Guide.
*
*
* @return Supplemental information that the task requires to migrate the data for certain source and target
* endpoints. For more information, see Specifying Supplemental
* Data for Task Settings in the AWS Database Migration Service User Guide.
*/
public String taskData() {
return taskData;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(replicationTaskArn());
hashCode = 31 * hashCode + Objects.hashCode(replicationTaskIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(migrationTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(tableMappings());
hashCode = 31 * hashCode + Objects.hashCode(replicationTaskSettings());
hashCode = 31 * hashCode + Objects.hashCode(cdcStartTime());
hashCode = 31 * hashCode + Objects.hashCode(cdcStartPosition());
hashCode = 31 * hashCode + Objects.hashCode(cdcStopPosition());
hashCode = 31 * hashCode + Objects.hashCode(taskData());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ModifyReplicationTaskRequest)) {
return false;
}
ModifyReplicationTaskRequest other = (ModifyReplicationTaskRequest) obj;
return Objects.equals(replicationTaskArn(), other.replicationTaskArn())
&& Objects.equals(replicationTaskIdentifier(), other.replicationTaskIdentifier())
&& Objects.equals(migrationTypeAsString(), other.migrationTypeAsString())
&& Objects.equals(tableMappings(), other.tableMappings())
&& Objects.equals(replicationTaskSettings(), other.replicationTaskSettings())
&& Objects.equals(cdcStartTime(), other.cdcStartTime())
&& Objects.equals(cdcStartPosition(), other.cdcStartPosition())
&& Objects.equals(cdcStopPosition(), other.cdcStopPosition()) && Objects.equals(taskData(), other.taskData());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("ModifyReplicationTaskRequest").add("ReplicationTaskArn", replicationTaskArn())
.add("ReplicationTaskIdentifier", replicationTaskIdentifier()).add("MigrationType", migrationTypeAsString())
.add("TableMappings", tableMappings()).add("ReplicationTaskSettings", replicationTaskSettings())
.add("CdcStartTime", cdcStartTime()).add("CdcStartPosition", cdcStartPosition())
.add("CdcStopPosition", cdcStopPosition()).add("TaskData", taskData()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ReplicationTaskArn":
return Optional.ofNullable(clazz.cast(replicationTaskArn()));
case "ReplicationTaskIdentifier":
return Optional.ofNullable(clazz.cast(replicationTaskIdentifier()));
case "MigrationType":
return Optional.ofNullable(clazz.cast(migrationTypeAsString()));
case "TableMappings":
return Optional.ofNullable(clazz.cast(tableMappings()));
case "ReplicationTaskSettings":
return Optional.ofNullable(clazz.cast(replicationTaskSettings()));
case "CdcStartTime":
return Optional.ofNullable(clazz.cast(cdcStartTime()));
case "CdcStartPosition":
return Optional.ofNullable(clazz.cast(cdcStartPosition()));
case "CdcStopPosition":
return Optional.ofNullable(clazz.cast(cdcStopPosition()));
case "TaskData":
return Optional.ofNullable(clazz.cast(taskData()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function