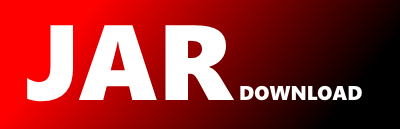
software.amazon.awssdk.services.databasemigration.model.ModifyReplicationInstanceRequest Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.databasemigration.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ModifyReplicationInstanceRequest extends DatabaseMigrationRequest implements
ToCopyableBuilder {
private static final SdkField REPLICATION_INSTANCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReplicationInstanceArn").getter(getter(ModifyReplicationInstanceRequest::replicationInstanceArn))
.setter(setter(Builder::replicationInstanceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationInstanceArn").build())
.build();
private static final SdkField ALLOCATED_STORAGE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("AllocatedStorage").getter(getter(ModifyReplicationInstanceRequest::allocatedStorage))
.setter(setter(Builder::allocatedStorage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllocatedStorage").build()).build();
private static final SdkField APPLY_IMMEDIATELY_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ApplyImmediately").getter(getter(ModifyReplicationInstanceRequest::applyImmediately))
.setter(setter(Builder::applyImmediately))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplyImmediately").build()).build();
private static final SdkField REPLICATION_INSTANCE_CLASS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReplicationInstanceClass").getter(getter(ModifyReplicationInstanceRequest::replicationInstanceClass))
.setter(setter(Builder::replicationInstanceClass))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationInstanceClass").build())
.build();
private static final SdkField> VPC_SECURITY_GROUP_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("VpcSecurityGroupIds")
.getter(getter(ModifyReplicationInstanceRequest::vpcSecurityGroupIds))
.setter(setter(Builder::vpcSecurityGroupIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcSecurityGroupIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PREFERRED_MAINTENANCE_WINDOW_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("PreferredMaintenanceWindow")
.getter(getter(ModifyReplicationInstanceRequest::preferredMaintenanceWindow))
.setter(setter(Builder::preferredMaintenanceWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredMaintenanceWindow").build())
.build();
private static final SdkField MULTI_AZ_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("MultiAZ").getter(getter(ModifyReplicationInstanceRequest::multiAZ)).setter(setter(Builder::multiAZ))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MultiAZ").build()).build();
private static final SdkField ENGINE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EngineVersion").getter(getter(ModifyReplicationInstanceRequest::engineVersion))
.setter(setter(Builder::engineVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngineVersion").build()).build();
private static final SdkField ALLOW_MAJOR_VERSION_UPGRADE_FIELD = SdkField
. builder(MarshallingType.BOOLEAN).memberName("AllowMajorVersionUpgrade")
.getter(getter(ModifyReplicationInstanceRequest::allowMajorVersionUpgrade))
.setter(setter(Builder::allowMajorVersionUpgrade))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllowMajorVersionUpgrade").build())
.build();
private static final SdkField AUTO_MINOR_VERSION_UPGRADE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("AutoMinorVersionUpgrade").getter(getter(ModifyReplicationInstanceRequest::autoMinorVersionUpgrade))
.setter(setter(Builder::autoMinorVersionUpgrade))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMinorVersionUpgrade").build())
.build();
private static final SdkField REPLICATION_INSTANCE_IDENTIFIER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ReplicationInstanceIdentifier")
.getter(getter(ModifyReplicationInstanceRequest::replicationInstanceIdentifier))
.setter(setter(Builder::replicationInstanceIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationInstanceIdentifier")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
REPLICATION_INSTANCE_ARN_FIELD, ALLOCATED_STORAGE_FIELD, APPLY_IMMEDIATELY_FIELD, REPLICATION_INSTANCE_CLASS_FIELD,
VPC_SECURITY_GROUP_IDS_FIELD, PREFERRED_MAINTENANCE_WINDOW_FIELD, MULTI_AZ_FIELD, ENGINE_VERSION_FIELD,
ALLOW_MAJOR_VERSION_UPGRADE_FIELD, AUTO_MINOR_VERSION_UPGRADE_FIELD, REPLICATION_INSTANCE_IDENTIFIER_FIELD));
private final String replicationInstanceArn;
private final Integer allocatedStorage;
private final Boolean applyImmediately;
private final String replicationInstanceClass;
private final List vpcSecurityGroupIds;
private final String preferredMaintenanceWindow;
private final Boolean multiAZ;
private final String engineVersion;
private final Boolean allowMajorVersionUpgrade;
private final Boolean autoMinorVersionUpgrade;
private final String replicationInstanceIdentifier;
private ModifyReplicationInstanceRequest(BuilderImpl builder) {
super(builder);
this.replicationInstanceArn = builder.replicationInstanceArn;
this.allocatedStorage = builder.allocatedStorage;
this.applyImmediately = builder.applyImmediately;
this.replicationInstanceClass = builder.replicationInstanceClass;
this.vpcSecurityGroupIds = builder.vpcSecurityGroupIds;
this.preferredMaintenanceWindow = builder.preferredMaintenanceWindow;
this.multiAZ = builder.multiAZ;
this.engineVersion = builder.engineVersion;
this.allowMajorVersionUpgrade = builder.allowMajorVersionUpgrade;
this.autoMinorVersionUpgrade = builder.autoMinorVersionUpgrade;
this.replicationInstanceIdentifier = builder.replicationInstanceIdentifier;
}
/**
*
* The Amazon Resource Name (ARN) of the replication instance.
*
*
* @return The Amazon Resource Name (ARN) of the replication instance.
*/
public String replicationInstanceArn() {
return replicationInstanceArn;
}
/**
*
* The amount of storage (in gigabytes) to be allocated for the replication instance.
*
*
* @return The amount of storage (in gigabytes) to be allocated for the replication instance.
*/
public Integer allocatedStorage() {
return allocatedStorage;
}
/**
*
* Indicates whether the changes should be applied immediately or during the next maintenance window.
*
*
* @return Indicates whether the changes should be applied immediately or during the next maintenance window.
*/
public Boolean applyImmediately() {
return applyImmediately;
}
/**
*
* The compute and memory capacity of the replication instance as defined for the specified replication instance
* class. For example to specify the instance class dms.c4.large, set this parameter to "dms.c4.large"
.
*
*
* For more information on the settings and capacities for the available replication instance classes, see Selecting the right AWS DMS replication instance for your migration.
*
*
* @return The compute and memory capacity of the replication instance as defined for the specified replication
* instance class. For example to specify the instance class dms.c4.large, set this parameter to
* "dms.c4.large"
.
*
* For more information on the settings and capacities for the available replication instance classes, see
* Selecting the right AWS DMS replication instance for your migration.
*/
public String replicationInstanceClass() {
return replicationInstanceClass;
}
/**
* Returns true if the VpcSecurityGroupIds property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public boolean hasVpcSecurityGroupIds() {
return vpcSecurityGroupIds != null && !(vpcSecurityGroupIds instanceof SdkAutoConstructList);
}
/**
*
* Specifies the VPC security group to be used with the replication instance. The VPC security group must work with
* the VPC containing the replication instance.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasVpcSecurityGroupIds()} to see if a value was sent in this field.
*
*
* @return Specifies the VPC security group to be used with the replication instance. The VPC security group must
* work with the VPC containing the replication instance.
*/
public List vpcSecurityGroupIds() {
return vpcSecurityGroupIds;
}
/**
*
* The weekly time range (in UTC) during which system maintenance can occur, which might result in an outage.
* Changing this parameter does not result in an outage, except in the following situation, and the change is
* asynchronously applied as soon as possible. If moving this window to the current time, there must be at least 30
* minutes between the current time and end of the window to ensure pending changes are applied.
*
*
* Default: Uses existing setting
*
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* Valid Days: Mon | Tue | Wed | Thu | Fri | Sat | Sun
*
*
* Constraints: Must be at least 30 minutes
*
*
* @return The weekly time range (in UTC) during which system maintenance can occur, which might result in an
* outage. Changing this parameter does not result in an outage, except in the following situation, and the
* change is asynchronously applied as soon as possible. If moving this window to the current time, there
* must be at least 30 minutes between the current time and end of the window to ensure pending changes are
* applied.
*
* Default: Uses existing setting
*
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* Valid Days: Mon | Tue | Wed | Thu | Fri | Sat | Sun
*
*
* Constraints: Must be at least 30 minutes
*/
public String preferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
/**
*
* Specifies whether the replication instance is a Multi-AZ deployment. You can't set the
* AvailabilityZone
parameter if the Multi-AZ parameter is set to true
.
*
*
* @return Specifies whether the replication instance is a Multi-AZ deployment. You can't set the
* AvailabilityZone
parameter if the Multi-AZ parameter is set to true
.
*/
public Boolean multiAZ() {
return multiAZ;
}
/**
*
* The engine version number of the replication instance.
*
*
* When modifying a major engine version of an instance, also set AllowMajorVersionUpgrade
to
* true
.
*
*
* @return The engine version number of the replication instance.
*
* When modifying a major engine version of an instance, also set AllowMajorVersionUpgrade
to
* true
.
*/
public String engineVersion() {
return engineVersion;
}
/**
*
* Indicates that major version upgrades are allowed. Changing this parameter does not result in an outage, and the
* change is asynchronously applied as soon as possible.
*
*
* This parameter must be set to true
when specifying a value for the EngineVersion
* parameter that is a different major version than the replication instance's current version.
*
*
* @return Indicates that major version upgrades are allowed. Changing this parameter does not result in an outage,
* and the change is asynchronously applied as soon as possible.
*
* This parameter must be set to true
when specifying a value for the
* EngineVersion
parameter that is a different major version than the replication instance's
* current version.
*/
public Boolean allowMajorVersionUpgrade() {
return allowMajorVersionUpgrade;
}
/**
*
* A value that indicates that minor version upgrades are applied automatically to the replication instance during
* the maintenance window. Changing this parameter doesn't result in an outage, except in the case dsecribed
* following. The change is asynchronously applied as soon as possible.
*
*
* An outage does result if these factors apply:
*
*
* -
*
* This parameter is set to true
during the maintenance window.
*
*
* -
*
* A newer minor version is available.
*
*
* -
*
* AWS DMS has enabled automatic patching for the given engine version.
*
*
*
*
* @return A value that indicates that minor version upgrades are applied automatically to the replication instance
* during the maintenance window. Changing this parameter doesn't result in an outage, except in the case
* dsecribed following. The change is asynchronously applied as soon as possible.
*
* An outage does result if these factors apply:
*
*
* -
*
* This parameter is set to true
during the maintenance window.
*
*
* -
*
* A newer minor version is available.
*
*
* -
*
* AWS DMS has enabled automatic patching for the given engine version.
*
*
*/
public Boolean autoMinorVersionUpgrade() {
return autoMinorVersionUpgrade;
}
/**
*
* The replication instance identifier. This parameter is stored as a lowercase string.
*
*
* @return The replication instance identifier. This parameter is stored as a lowercase string.
*/
public String replicationInstanceIdentifier() {
return replicationInstanceIdentifier;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(replicationInstanceArn());
hashCode = 31 * hashCode + Objects.hashCode(allocatedStorage());
hashCode = 31 * hashCode + Objects.hashCode(applyImmediately());
hashCode = 31 * hashCode + Objects.hashCode(replicationInstanceClass());
hashCode = 31 * hashCode + Objects.hashCode(hasVpcSecurityGroupIds() ? vpcSecurityGroupIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(preferredMaintenanceWindow());
hashCode = 31 * hashCode + Objects.hashCode(multiAZ());
hashCode = 31 * hashCode + Objects.hashCode(engineVersion());
hashCode = 31 * hashCode + Objects.hashCode(allowMajorVersionUpgrade());
hashCode = 31 * hashCode + Objects.hashCode(autoMinorVersionUpgrade());
hashCode = 31 * hashCode + Objects.hashCode(replicationInstanceIdentifier());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ModifyReplicationInstanceRequest)) {
return false;
}
ModifyReplicationInstanceRequest other = (ModifyReplicationInstanceRequest) obj;
return Objects.equals(replicationInstanceArn(), other.replicationInstanceArn())
&& Objects.equals(allocatedStorage(), other.allocatedStorage())
&& Objects.equals(applyImmediately(), other.applyImmediately())
&& Objects.equals(replicationInstanceClass(), other.replicationInstanceClass())
&& hasVpcSecurityGroupIds() == other.hasVpcSecurityGroupIds()
&& Objects.equals(vpcSecurityGroupIds(), other.vpcSecurityGroupIds())
&& Objects.equals(preferredMaintenanceWindow(), other.preferredMaintenanceWindow())
&& Objects.equals(multiAZ(), other.multiAZ()) && Objects.equals(engineVersion(), other.engineVersion())
&& Objects.equals(allowMajorVersionUpgrade(), other.allowMajorVersionUpgrade())
&& Objects.equals(autoMinorVersionUpgrade(), other.autoMinorVersionUpgrade())
&& Objects.equals(replicationInstanceIdentifier(), other.replicationInstanceIdentifier());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("ModifyReplicationInstanceRequest").add("ReplicationInstanceArn", replicationInstanceArn())
.add("AllocatedStorage", allocatedStorage()).add("ApplyImmediately", applyImmediately())
.add("ReplicationInstanceClass", replicationInstanceClass())
.add("VpcSecurityGroupIds", hasVpcSecurityGroupIds() ? vpcSecurityGroupIds() : null)
.add("PreferredMaintenanceWindow", preferredMaintenanceWindow()).add("MultiAZ", multiAZ())
.add("EngineVersion", engineVersion()).add("AllowMajorVersionUpgrade", allowMajorVersionUpgrade())
.add("AutoMinorVersionUpgrade", autoMinorVersionUpgrade())
.add("ReplicationInstanceIdentifier", replicationInstanceIdentifier()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ReplicationInstanceArn":
return Optional.ofNullable(clazz.cast(replicationInstanceArn()));
case "AllocatedStorage":
return Optional.ofNullable(clazz.cast(allocatedStorage()));
case "ApplyImmediately":
return Optional.ofNullable(clazz.cast(applyImmediately()));
case "ReplicationInstanceClass":
return Optional.ofNullable(clazz.cast(replicationInstanceClass()));
case "VpcSecurityGroupIds":
return Optional.ofNullable(clazz.cast(vpcSecurityGroupIds()));
case "PreferredMaintenanceWindow":
return Optional.ofNullable(clazz.cast(preferredMaintenanceWindow()));
case "MultiAZ":
return Optional.ofNullable(clazz.cast(multiAZ()));
case "EngineVersion":
return Optional.ofNullable(clazz.cast(engineVersion()));
case "AllowMajorVersionUpgrade":
return Optional.ofNullable(clazz.cast(allowMajorVersionUpgrade()));
case "AutoMinorVersionUpgrade":
return Optional.ofNullable(clazz.cast(autoMinorVersionUpgrade()));
case "ReplicationInstanceIdentifier":
return Optional.ofNullable(clazz.cast(replicationInstanceIdentifier()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function