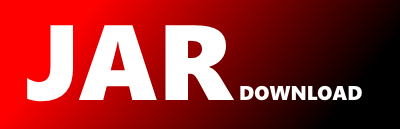
software.amazon.awssdk.services.databasemigration.model.DescribeApplicableIndividualAssessmentsRequest Maven / Gradle / Ivy
Show all versions of databasemigration Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.databasemigration.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeApplicableIndividualAssessmentsRequest extends DatabaseMigrationRequest implements
ToCopyableBuilder {
private static final SdkField REPLICATION_TASK_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReplicationTaskArn").getter(getter(DescribeApplicableIndividualAssessmentsRequest::replicationTaskArn))
.setter(setter(Builder::replicationTaskArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationTaskArn").build())
.build();
private static final SdkField REPLICATION_INSTANCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReplicationInstanceArn")
.getter(getter(DescribeApplicableIndividualAssessmentsRequest::replicationInstanceArn))
.setter(setter(Builder::replicationInstanceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationInstanceArn").build())
.build();
private static final SdkField SOURCE_ENGINE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceEngineName").getter(getter(DescribeApplicableIndividualAssessmentsRequest::sourceEngineName))
.setter(setter(Builder::sourceEngineName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceEngineName").build()).build();
private static final SdkField TARGET_ENGINE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TargetEngineName").getter(getter(DescribeApplicableIndividualAssessmentsRequest::targetEngineName))
.setter(setter(Builder::targetEngineName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetEngineName").build()).build();
private static final SdkField MIGRATION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MigrationType").getter(getter(DescribeApplicableIndividualAssessmentsRequest::migrationTypeAsString))
.setter(setter(Builder::migrationType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MigrationType").build()).build();
private static final SdkField MAX_RECORDS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxRecords").getter(getter(DescribeApplicableIndividualAssessmentsRequest::maxRecords))
.setter(setter(Builder::maxRecords))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxRecords").build()).build();
private static final SdkField MARKER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Marker")
.getter(getter(DescribeApplicableIndividualAssessmentsRequest::marker)).setter(setter(Builder::marker))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Marker").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REPLICATION_TASK_ARN_FIELD,
REPLICATION_INSTANCE_ARN_FIELD, SOURCE_ENGINE_NAME_FIELD, TARGET_ENGINE_NAME_FIELD, MIGRATION_TYPE_FIELD,
MAX_RECORDS_FIELD, MARKER_FIELD));
private final String replicationTaskArn;
private final String replicationInstanceArn;
private final String sourceEngineName;
private final String targetEngineName;
private final String migrationType;
private final Integer maxRecords;
private final String marker;
private DescribeApplicableIndividualAssessmentsRequest(BuilderImpl builder) {
super(builder);
this.replicationTaskArn = builder.replicationTaskArn;
this.replicationInstanceArn = builder.replicationInstanceArn;
this.sourceEngineName = builder.sourceEngineName;
this.targetEngineName = builder.targetEngineName;
this.migrationType = builder.migrationType;
this.maxRecords = builder.maxRecords;
this.marker = builder.marker;
}
/**
*
* Amazon Resource Name (ARN) of a migration task on which you want to base the default list of individual
* assessments.
*
*
* @return Amazon Resource Name (ARN) of a migration task on which you want to base the default list of individual
* assessments.
*/
public final String replicationTaskArn() {
return replicationTaskArn;
}
/**
*
* ARN of a replication instance on which you want to base the default list of individual assessments.
*
*
* @return ARN of a replication instance on which you want to base the default list of individual assessments.
*/
public final String replicationInstanceArn() {
return replicationInstanceArn;
}
/**
*
* Name of a database engine that the specified replication instance supports as a source.
*
*
* @return Name of a database engine that the specified replication instance supports as a source.
*/
public final String sourceEngineName() {
return sourceEngineName;
}
/**
*
* Name of a database engine that the specified replication instance supports as a target.
*
*
* @return Name of a database engine that the specified replication instance supports as a target.
*/
public final String targetEngineName() {
return targetEngineName;
}
/**
*
* Name of the migration type that each provided individual assessment must support.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #migrationType}
* will return {@link MigrationTypeValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #migrationTypeAsString}.
*
*
* @return Name of the migration type that each provided individual assessment must support.
* @see MigrationTypeValue
*/
public final MigrationTypeValue migrationType() {
return MigrationTypeValue.fromValue(migrationType);
}
/**
*
* Name of the migration type that each provided individual assessment must support.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #migrationType}
* will return {@link MigrationTypeValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #migrationTypeAsString}.
*
*
* @return Name of the migration type that each provided individual assessment must support.
* @see MigrationTypeValue
*/
public final String migrationTypeAsString() {
return migrationType;
}
/**
*
* Maximum number of records to include in the response. If more records exist than the specified
* MaxRecords
value, a pagination token called a marker is included in the response so that the
* remaining results can be retrieved.
*
*
* @return Maximum number of records to include in the response. If more records exist than the specified
* MaxRecords
value, a pagination token called a marker is included in the response so that the
* remaining results can be retrieved.
*/
public final Integer maxRecords() {
return maxRecords;
}
/**
*
* Optional pagination token provided by a previous request. If this parameter is specified, the response includes
* only records beyond the marker, up to the value specified by MaxRecords
.
*
*
* @return Optional pagination token provided by a previous request. If this parameter is specified, the response
* includes only records beyond the marker, up to the value specified by MaxRecords
.
*/
public final String marker() {
return marker;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(replicationTaskArn());
hashCode = 31 * hashCode + Objects.hashCode(replicationInstanceArn());
hashCode = 31 * hashCode + Objects.hashCode(sourceEngineName());
hashCode = 31 * hashCode + Objects.hashCode(targetEngineName());
hashCode = 31 * hashCode + Objects.hashCode(migrationTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(maxRecords());
hashCode = 31 * hashCode + Objects.hashCode(marker());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeApplicableIndividualAssessmentsRequest)) {
return false;
}
DescribeApplicableIndividualAssessmentsRequest other = (DescribeApplicableIndividualAssessmentsRequest) obj;
return Objects.equals(replicationTaskArn(), other.replicationTaskArn())
&& Objects.equals(replicationInstanceArn(), other.replicationInstanceArn())
&& Objects.equals(sourceEngineName(), other.sourceEngineName())
&& Objects.equals(targetEngineName(), other.targetEngineName())
&& Objects.equals(migrationTypeAsString(), other.migrationTypeAsString())
&& Objects.equals(maxRecords(), other.maxRecords()) && Objects.equals(marker(), other.marker());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DescribeApplicableIndividualAssessmentsRequest").add("ReplicationTaskArn", replicationTaskArn())
.add("ReplicationInstanceArn", replicationInstanceArn()).add("SourceEngineName", sourceEngineName())
.add("TargetEngineName", targetEngineName()).add("MigrationType", migrationTypeAsString())
.add("MaxRecords", maxRecords()).add("Marker", marker()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ReplicationTaskArn":
return Optional.ofNullable(clazz.cast(replicationTaskArn()));
case "ReplicationInstanceArn":
return Optional.ofNullable(clazz.cast(replicationInstanceArn()));
case "SourceEngineName":
return Optional.ofNullable(clazz.cast(sourceEngineName()));
case "TargetEngineName":
return Optional.ofNullable(clazz.cast(targetEngineName()));
case "MigrationType":
return Optional.ofNullable(clazz.cast(migrationTypeAsString()));
case "MaxRecords":
return Optional.ofNullable(clazz.cast(maxRecords()));
case "Marker":
return Optional.ofNullable(clazz.cast(marker()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function