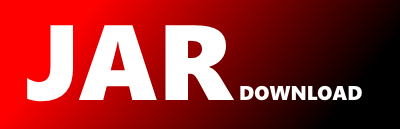
software.amazon.awssdk.services.databasemigration.model.MicrosoftSQLServerSettings Maven / Gradle / Ivy
Show all versions of databasemigration Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.databasemigration.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides information that defines a Microsoft SQL Server endpoint.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class MicrosoftSQLServerSettings implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Port")
.getter(getter(MicrosoftSQLServerSettings::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Port").build()).build();
private static final SdkField BCP_PACKET_SIZE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("BcpPacketSize").getter(getter(MicrosoftSQLServerSettings::bcpPacketSize))
.setter(setter(Builder::bcpPacketSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BcpPacketSize").build()).build();
private static final SdkField DATABASE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DatabaseName").getter(getter(MicrosoftSQLServerSettings::databaseName))
.setter(setter(Builder::databaseName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DatabaseName").build()).build();
private static final SdkField CONTROL_TABLES_FILE_GROUP_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ControlTablesFileGroup").getter(getter(MicrosoftSQLServerSettings::controlTablesFileGroup))
.setter(setter(Builder::controlTablesFileGroup))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ControlTablesFileGroup").build())
.build();
private static final SdkField PASSWORD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Password").getter(getter(MicrosoftSQLServerSettings::password)).setter(setter(Builder::password))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Password").build()).build();
private static final SdkField QUERY_SINGLE_ALWAYS_ON_NODE_FIELD = SdkField
. builder(MarshallingType.BOOLEAN).memberName("QuerySingleAlwaysOnNode")
.getter(getter(MicrosoftSQLServerSettings::querySingleAlwaysOnNode)).setter(setter(Builder::querySingleAlwaysOnNode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("QuerySingleAlwaysOnNode").build())
.build();
private static final SdkField READ_BACKUP_ONLY_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ReadBackupOnly").getter(getter(MicrosoftSQLServerSettings::readBackupOnly))
.setter(setter(Builder::readBackupOnly))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReadBackupOnly").build()).build();
private static final SdkField SAFEGUARD_POLICY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SafeguardPolicy").getter(getter(MicrosoftSQLServerSettings::safeguardPolicyAsString))
.setter(setter(Builder::safeguardPolicy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SafeguardPolicy").build()).build();
private static final SdkField SERVER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServerName").getter(getter(MicrosoftSQLServerSettings::serverName)).setter(setter(Builder::serverName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServerName").build()).build();
private static final SdkField USERNAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Username").getter(getter(MicrosoftSQLServerSettings::username)).setter(setter(Builder::username))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Username").build()).build();
private static final SdkField USE_BCP_FULL_LOAD_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("UseBcpFullLoad").getter(getter(MicrosoftSQLServerSettings::useBcpFullLoad))
.setter(setter(Builder::useBcpFullLoad))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UseBcpFullLoad").build()).build();
private static final SdkField USE_THIRD_PARTY_BACKUP_DEVICE_FIELD = SdkField
. builder(MarshallingType.BOOLEAN).memberName("UseThirdPartyBackupDevice")
.getter(getter(MicrosoftSQLServerSettings::useThirdPartyBackupDevice))
.setter(setter(Builder::useThirdPartyBackupDevice))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UseThirdPartyBackupDevice").build())
.build();
private static final SdkField SECRETS_MANAGER_ACCESS_ROLE_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SecretsManagerAccessRoleArn")
.getter(getter(MicrosoftSQLServerSettings::secretsManagerAccessRoleArn))
.setter(setter(Builder::secretsManagerAccessRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecretsManagerAccessRoleArn")
.build()).build();
private static final SdkField SECRETS_MANAGER_SECRET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SecretsManagerSecretId").getter(getter(MicrosoftSQLServerSettings::secretsManagerSecretId))
.setter(setter(Builder::secretsManagerSecretId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecretsManagerSecretId").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(PORT_FIELD,
BCP_PACKET_SIZE_FIELD, DATABASE_NAME_FIELD, CONTROL_TABLES_FILE_GROUP_FIELD, PASSWORD_FIELD,
QUERY_SINGLE_ALWAYS_ON_NODE_FIELD, READ_BACKUP_ONLY_FIELD, SAFEGUARD_POLICY_FIELD, SERVER_NAME_FIELD, USERNAME_FIELD,
USE_BCP_FULL_LOAD_FIELD, USE_THIRD_PARTY_BACKUP_DEVICE_FIELD, SECRETS_MANAGER_ACCESS_ROLE_ARN_FIELD,
SECRETS_MANAGER_SECRET_ID_FIELD));
private static final long serialVersionUID = 1L;
private final Integer port;
private final Integer bcpPacketSize;
private final String databaseName;
private final String controlTablesFileGroup;
private final String password;
private final Boolean querySingleAlwaysOnNode;
private final Boolean readBackupOnly;
private final String safeguardPolicy;
private final String serverName;
private final String username;
private final Boolean useBcpFullLoad;
private final Boolean useThirdPartyBackupDevice;
private final String secretsManagerAccessRoleArn;
private final String secretsManagerSecretId;
private MicrosoftSQLServerSettings(BuilderImpl builder) {
this.port = builder.port;
this.bcpPacketSize = builder.bcpPacketSize;
this.databaseName = builder.databaseName;
this.controlTablesFileGroup = builder.controlTablesFileGroup;
this.password = builder.password;
this.querySingleAlwaysOnNode = builder.querySingleAlwaysOnNode;
this.readBackupOnly = builder.readBackupOnly;
this.safeguardPolicy = builder.safeguardPolicy;
this.serverName = builder.serverName;
this.username = builder.username;
this.useBcpFullLoad = builder.useBcpFullLoad;
this.useThirdPartyBackupDevice = builder.useThirdPartyBackupDevice;
this.secretsManagerAccessRoleArn = builder.secretsManagerAccessRoleArn;
this.secretsManagerSecretId = builder.secretsManagerSecretId;
}
/**
*
* Endpoint TCP port.
*
*
* @return Endpoint TCP port.
*/
public final Integer port() {
return port;
}
/**
*
* The maximum size of the packets (in bytes) used to transfer data using BCP.
*
*
* @return The maximum size of the packets (in bytes) used to transfer data using BCP.
*/
public final Integer bcpPacketSize() {
return bcpPacketSize;
}
/**
*
* Database name for the endpoint.
*
*
* @return Database name for the endpoint.
*/
public final String databaseName() {
return databaseName;
}
/**
*
* Specifies a file group for the DMS internal tables. When the replication task starts, all the internal DMS
* control tables (awsdms_ apply_exception, awsdms_apply, awsdms_changes) are created for the specified file group.
*
*
* @return Specifies a file group for the DMS internal tables. When the replication task starts, all the internal
* DMS control tables (awsdms_ apply_exception, awsdms_apply, awsdms_changes) are created for the specified
* file group.
*/
public final String controlTablesFileGroup() {
return controlTablesFileGroup;
}
/**
*
* Endpoint connection password.
*
*
* @return Endpoint connection password.
*/
public final String password() {
return password;
}
/**
*
* Cleans and recreates table metadata information on the replication instance when a mismatch occurs. An example is
* a situation where running an alter DDL statement on a table might result in different information about the table
* cached in the replication instance.
*
*
* @return Cleans and recreates table metadata information on the replication instance when a mismatch occurs. An
* example is a situation where running an alter DDL statement on a table might result in different
* information about the table cached in the replication instance.
*/
public final Boolean querySingleAlwaysOnNode() {
return querySingleAlwaysOnNode;
}
/**
*
* When this attribute is set to Y
, DMS only reads changes from transaction log backups and doesn't
* read from the active transaction log file during ongoing replication. Setting this parameter to Y
* enables you to control active transaction log file growth during full load and ongoing replication tasks.
* However, it can add some source latency to ongoing replication.
*
*
* @return When this attribute is set to Y
, DMS only reads changes from transaction log backups and
* doesn't read from the active transaction log file during ongoing replication. Setting this parameter to
* Y
enables you to control active transaction log file growth during full load and ongoing
* replication tasks. However, it can add some source latency to ongoing replication.
*/
public final Boolean readBackupOnly() {
return readBackupOnly;
}
/**
*
* Use this attribute to minimize the need to access the backup log and enable DMS to prevent truncation using one
* of the following two methods.
*
*
* Start transactions in the database: This is the default method. When this method is used, DMS prevents
* TLOG truncation by mimicking a transaction in the database. As long as such a transaction is open, changes that
* appear after the transaction started aren't truncated. If you need Microsoft Replication to be enabled in your
* database, then you must choose this method.
*
*
* Exclusively use sp_repldone within a single task: When this method is used, DMS reads the changes and then
* uses sp_repldone to mark the TLOG transactions as ready for truncation. Although this method doesn't involve any
* transactional activities, it can only be used when Microsoft Replication isn't running. Also, when using this
* method, only one DMS task can access the database at any given time. Therefore, if you need to run parallel DMS
* tasks against the same database, use the default method.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #safeguardPolicy}
* will return {@link SafeguardPolicy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #safeguardPolicyAsString}.
*
*
* @return Use this attribute to minimize the need to access the backup log and enable DMS to prevent truncation
* using one of the following two methods.
*
* Start transactions in the database: This is the default method. When this method is used, DMS
* prevents TLOG truncation by mimicking a transaction in the database. As long as such a transaction is
* open, changes that appear after the transaction started aren't truncated. If you need Microsoft
* Replication to be enabled in your database, then you must choose this method.
*
*
* Exclusively use sp_repldone within a single task: When this method is used, DMS reads the changes
* and then uses sp_repldone to mark the TLOG transactions as ready for truncation. Although this method
* doesn't involve any transactional activities, it can only be used when Microsoft Replication isn't
* running. Also, when using this method, only one DMS task can access the database at any given time.
* Therefore, if you need to run parallel DMS tasks against the same database, use the default method.
* @see SafeguardPolicy
*/
public final SafeguardPolicy safeguardPolicy() {
return SafeguardPolicy.fromValue(safeguardPolicy);
}
/**
*
* Use this attribute to minimize the need to access the backup log and enable DMS to prevent truncation using one
* of the following two methods.
*
*
* Start transactions in the database: This is the default method. When this method is used, DMS prevents
* TLOG truncation by mimicking a transaction in the database. As long as such a transaction is open, changes that
* appear after the transaction started aren't truncated. If you need Microsoft Replication to be enabled in your
* database, then you must choose this method.
*
*
* Exclusively use sp_repldone within a single task: When this method is used, DMS reads the changes and then
* uses sp_repldone to mark the TLOG transactions as ready for truncation. Although this method doesn't involve any
* transactional activities, it can only be used when Microsoft Replication isn't running. Also, when using this
* method, only one DMS task can access the database at any given time. Therefore, if you need to run parallel DMS
* tasks against the same database, use the default method.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #safeguardPolicy}
* will return {@link SafeguardPolicy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #safeguardPolicyAsString}.
*
*
* @return Use this attribute to minimize the need to access the backup log and enable DMS to prevent truncation
* using one of the following two methods.
*
* Start transactions in the database: This is the default method. When this method is used, DMS
* prevents TLOG truncation by mimicking a transaction in the database. As long as such a transaction is
* open, changes that appear after the transaction started aren't truncated. If you need Microsoft
* Replication to be enabled in your database, then you must choose this method.
*
*
* Exclusively use sp_repldone within a single task: When this method is used, DMS reads the changes
* and then uses sp_repldone to mark the TLOG transactions as ready for truncation. Although this method
* doesn't involve any transactional activities, it can only be used when Microsoft Replication isn't
* running. Also, when using this method, only one DMS task can access the database at any given time.
* Therefore, if you need to run parallel DMS tasks against the same database, use the default method.
* @see SafeguardPolicy
*/
public final String safeguardPolicyAsString() {
return safeguardPolicy;
}
/**
*
* Fully qualified domain name of the endpoint.
*
*
* @return Fully qualified domain name of the endpoint.
*/
public final String serverName() {
return serverName;
}
/**
*
* Endpoint connection user name.
*
*
* @return Endpoint connection user name.
*/
public final String username() {
return username;
}
/**
*
* Use this to attribute to transfer data for full-load operations using BCP. When the target table contains an
* identity column that does not exist in the source table, you must disable the use BCP for loading table option.
*
*
* @return Use this to attribute to transfer data for full-load operations using BCP. When the target table contains
* an identity column that does not exist in the source table, you must disable the use BCP for loading
* table option.
*/
public final Boolean useBcpFullLoad() {
return useBcpFullLoad;
}
/**
*
* When this attribute is set to Y
, DMS processes third-party transaction log backups if they are
* created in native format.
*
*
* @return When this attribute is set to Y
, DMS processes third-party transaction log backups if they
* are created in native format.
*/
public final Boolean useThirdPartyBackupDevice() {
return useThirdPartyBackupDevice;
}
/**
*
* The full Amazon Resource Name (ARN) of the IAM role that specifies DMS as the trusted entity and grants the
* required permissions to access the value in SecretsManagerSecret
. The role must allow the
* iam:PassRole
action. SecretsManagerSecret
has the value of the Amazon Web Services
* Secrets Manager secret that allows access to the SQL Server endpoint.
*
*
*
* You can specify one of two sets of values for these permissions. You can specify the values for this setting and
* SecretsManagerSecretId
. Or you can specify clear-text values for UserName
,
* Password
, ServerName
, and Port
. You can't specify both. For more
* information on creating this SecretsManagerSecret
and the SecretsManagerAccessRoleArn
* and SecretsManagerSecretId
required to access it, see Using
* secrets to access Database Migration Service resources in the Database Migration Service User Guide.
*
*
*
* @return The full Amazon Resource Name (ARN) of the IAM role that specifies DMS as the trusted entity and grants
* the required permissions to access the value in SecretsManagerSecret
. The role must allow
* the iam:PassRole
action. SecretsManagerSecret
has the value of the Amazon Web
* Services Secrets Manager secret that allows access to the SQL Server endpoint.
*
* You can specify one of two sets of values for these permissions. You can specify the values for this
* setting and SecretsManagerSecretId
. Or you can specify clear-text values for
* UserName
, Password
, ServerName
, and Port
. You can't
* specify both. For more information on creating this SecretsManagerSecret
and the
* SecretsManagerAccessRoleArn
and SecretsManagerSecretId
required to access it,
* see Using secrets to access Database Migration Service resources in the Database Migration Service
* User Guide.
*
*/
public final String secretsManagerAccessRoleArn() {
return secretsManagerAccessRoleArn;
}
/**
*
* The full ARN, partial ARN, or friendly name of the SecretsManagerSecret
that contains the SQL Server
* endpoint connection details.
*
*
* @return The full ARN, partial ARN, or friendly name of the SecretsManagerSecret
that contains the
* SQL Server endpoint connection details.
*/
public final String secretsManagerSecretId() {
return secretsManagerSecretId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(bcpPacketSize());
hashCode = 31 * hashCode + Objects.hashCode(databaseName());
hashCode = 31 * hashCode + Objects.hashCode(controlTablesFileGroup());
hashCode = 31 * hashCode + Objects.hashCode(password());
hashCode = 31 * hashCode + Objects.hashCode(querySingleAlwaysOnNode());
hashCode = 31 * hashCode + Objects.hashCode(readBackupOnly());
hashCode = 31 * hashCode + Objects.hashCode(safeguardPolicyAsString());
hashCode = 31 * hashCode + Objects.hashCode(serverName());
hashCode = 31 * hashCode + Objects.hashCode(username());
hashCode = 31 * hashCode + Objects.hashCode(useBcpFullLoad());
hashCode = 31 * hashCode + Objects.hashCode(useThirdPartyBackupDevice());
hashCode = 31 * hashCode + Objects.hashCode(secretsManagerAccessRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(secretsManagerSecretId());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof MicrosoftSQLServerSettings)) {
return false;
}
MicrosoftSQLServerSettings other = (MicrosoftSQLServerSettings) obj;
return Objects.equals(port(), other.port()) && Objects.equals(bcpPacketSize(), other.bcpPacketSize())
&& Objects.equals(databaseName(), other.databaseName())
&& Objects.equals(controlTablesFileGroup(), other.controlTablesFileGroup())
&& Objects.equals(password(), other.password())
&& Objects.equals(querySingleAlwaysOnNode(), other.querySingleAlwaysOnNode())
&& Objects.equals(readBackupOnly(), other.readBackupOnly())
&& Objects.equals(safeguardPolicyAsString(), other.safeguardPolicyAsString())
&& Objects.equals(serverName(), other.serverName()) && Objects.equals(username(), other.username())
&& Objects.equals(useBcpFullLoad(), other.useBcpFullLoad())
&& Objects.equals(useThirdPartyBackupDevice(), other.useThirdPartyBackupDevice())
&& Objects.equals(secretsManagerAccessRoleArn(), other.secretsManagerAccessRoleArn())
&& Objects.equals(secretsManagerSecretId(), other.secretsManagerSecretId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("MicrosoftSQLServerSettings").add("Port", port()).add("BcpPacketSize", bcpPacketSize())
.add("DatabaseName", databaseName()).add("ControlTablesFileGroup", controlTablesFileGroup())
.add("Password", password() == null ? null : "*** Sensitive Data Redacted ***")
.add("QuerySingleAlwaysOnNode", querySingleAlwaysOnNode()).add("ReadBackupOnly", readBackupOnly())
.add("SafeguardPolicy", safeguardPolicyAsString()).add("ServerName", serverName()).add("Username", username())
.add("UseBcpFullLoad", useBcpFullLoad()).add("UseThirdPartyBackupDevice", useThirdPartyBackupDevice())
.add("SecretsManagerAccessRoleArn", secretsManagerAccessRoleArn())
.add("SecretsManagerSecretId", secretsManagerSecretId()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Port":
return Optional.ofNullable(clazz.cast(port()));
case "BcpPacketSize":
return Optional.ofNullable(clazz.cast(bcpPacketSize()));
case "DatabaseName":
return Optional.ofNullable(clazz.cast(databaseName()));
case "ControlTablesFileGroup":
return Optional.ofNullable(clazz.cast(controlTablesFileGroup()));
case "Password":
return Optional.ofNullable(clazz.cast(password()));
case "QuerySingleAlwaysOnNode":
return Optional.ofNullable(clazz.cast(querySingleAlwaysOnNode()));
case "ReadBackupOnly":
return Optional.ofNullable(clazz.cast(readBackupOnly()));
case "SafeguardPolicy":
return Optional.ofNullable(clazz.cast(safeguardPolicyAsString()));
case "ServerName":
return Optional.ofNullable(clazz.cast(serverName()));
case "Username":
return Optional.ofNullable(clazz.cast(username()));
case "UseBcpFullLoad":
return Optional.ofNullable(clazz.cast(useBcpFullLoad()));
case "UseThirdPartyBackupDevice":
return Optional.ofNullable(clazz.cast(useThirdPartyBackupDevice()));
case "SecretsManagerAccessRoleArn":
return Optional.ofNullable(clazz.cast(secretsManagerAccessRoleArn()));
case "SecretsManagerSecretId":
return Optional.ofNullable(clazz.cast(secretsManagerSecretId()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function