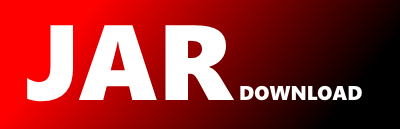
software.amazon.awssdk.services.databasemigration.model.DocDbSettings Maven / Gradle / Ivy
Show all versions of databasemigration Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.databasemigration.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides information that defines a DocumentDB endpoint.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DocDbSettings implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField USERNAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Username").getter(getter(DocDbSettings::username)).setter(setter(Builder::username))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Username").build()).build();
private static final SdkField PASSWORD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Password").getter(getter(DocDbSettings::password)).setter(setter(Builder::password))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Password").build()).build();
private static final SdkField SERVER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServerName").getter(getter(DocDbSettings::serverName)).setter(setter(Builder::serverName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServerName").build()).build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Port")
.getter(getter(DocDbSettings::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Port").build()).build();
private static final SdkField DATABASE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DatabaseName").getter(getter(DocDbSettings::databaseName)).setter(setter(Builder::databaseName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DatabaseName").build()).build();
private static final SdkField NESTING_LEVEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NestingLevel").getter(getter(DocDbSettings::nestingLevelAsString)).setter(setter(Builder::nestingLevel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NestingLevel").build()).build();
private static final SdkField EXTRACT_DOC_ID_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ExtractDocId").getter(getter(DocDbSettings::extractDocId)).setter(setter(Builder::extractDocId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExtractDocId").build()).build();
private static final SdkField DOCS_TO_INVESTIGATE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("DocsToInvestigate").getter(getter(DocDbSettings::docsToInvestigate))
.setter(setter(Builder::docsToInvestigate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DocsToInvestigate").build()).build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsKeyId").getter(getter(DocDbSettings::kmsKeyId)).setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KmsKeyId").build()).build();
private static final SdkField SECRETS_MANAGER_ACCESS_ROLE_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SecretsManagerAccessRoleArn")
.getter(getter(DocDbSettings::secretsManagerAccessRoleArn))
.setter(setter(Builder::secretsManagerAccessRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecretsManagerAccessRoleArn")
.build()).build();
private static final SdkField SECRETS_MANAGER_SECRET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SecretsManagerSecretId").getter(getter(DocDbSettings::secretsManagerSecretId))
.setter(setter(Builder::secretsManagerSecretId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecretsManagerSecretId").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(USERNAME_FIELD,
PASSWORD_FIELD, SERVER_NAME_FIELD, PORT_FIELD, DATABASE_NAME_FIELD, NESTING_LEVEL_FIELD, EXTRACT_DOC_ID_FIELD,
DOCS_TO_INVESTIGATE_FIELD, KMS_KEY_ID_FIELD, SECRETS_MANAGER_ACCESS_ROLE_ARN_FIELD, SECRETS_MANAGER_SECRET_ID_FIELD));
private static final long serialVersionUID = 1L;
private final String username;
private final String password;
private final String serverName;
private final Integer port;
private final String databaseName;
private final String nestingLevel;
private final Boolean extractDocId;
private final Integer docsToInvestigate;
private final String kmsKeyId;
private final String secretsManagerAccessRoleArn;
private final String secretsManagerSecretId;
private DocDbSettings(BuilderImpl builder) {
this.username = builder.username;
this.password = builder.password;
this.serverName = builder.serverName;
this.port = builder.port;
this.databaseName = builder.databaseName;
this.nestingLevel = builder.nestingLevel;
this.extractDocId = builder.extractDocId;
this.docsToInvestigate = builder.docsToInvestigate;
this.kmsKeyId = builder.kmsKeyId;
this.secretsManagerAccessRoleArn = builder.secretsManagerAccessRoleArn;
this.secretsManagerSecretId = builder.secretsManagerSecretId;
}
/**
*
* The user name you use to access the DocumentDB source endpoint.
*
*
* @return The user name you use to access the DocumentDB source endpoint.
*/
public final String username() {
return username;
}
/**
*
* The password for the user account you use to access the DocumentDB source endpoint.
*
*
* @return The password for the user account you use to access the DocumentDB source endpoint.
*/
public final String password() {
return password;
}
/**
*
* The name of the server on the DocumentDB source endpoint.
*
*
* @return The name of the server on the DocumentDB source endpoint.
*/
public final String serverName() {
return serverName;
}
/**
*
* The port value for the DocumentDB source endpoint.
*
*
* @return The port value for the DocumentDB source endpoint.
*/
public final Integer port() {
return port;
}
/**
*
* The database name on the DocumentDB source endpoint.
*
*
* @return The database name on the DocumentDB source endpoint.
*/
public final String databaseName() {
return databaseName;
}
/**
*
* Specifies either document or table mode.
*
*
* Default value is "none"
. Specify "none"
to use document mode. Specify
* "one"
to use table mode.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #nestingLevel} will
* return {@link NestingLevelValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #nestingLevelAsString}.
*
*
* @return Specifies either document or table mode.
*
* Default value is "none"
. Specify "none"
to use document mode. Specify
* "one"
to use table mode.
* @see NestingLevelValue
*/
public final NestingLevelValue nestingLevel() {
return NestingLevelValue.fromValue(nestingLevel);
}
/**
*
* Specifies either document or table mode.
*
*
* Default value is "none"
. Specify "none"
to use document mode. Specify
* "one"
to use table mode.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #nestingLevel} will
* return {@link NestingLevelValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #nestingLevelAsString}.
*
*
* @return Specifies either document or table mode.
*
* Default value is "none"
. Specify "none"
to use document mode. Specify
* "one"
to use table mode.
* @see NestingLevelValue
*/
public final String nestingLevelAsString() {
return nestingLevel;
}
/**
*
* Specifies the document ID. Use this setting when NestingLevel
is set to "none"
.
*
*
* Default value is "false"
.
*
*
* @return Specifies the document ID. Use this setting when NestingLevel
is set to "none"
.
*
*
* Default value is "false"
.
*/
public final Boolean extractDocId() {
return extractDocId;
}
/**
*
* Indicates the number of documents to preview to determine the document organization. Use this setting when
* NestingLevel
is set to "one"
.
*
*
* Must be a positive value greater than 0
. Default value is 1000
.
*
*
* @return Indicates the number of documents to preview to determine the document organization. Use this setting
* when NestingLevel
is set to "one"
.
*
* Must be a positive value greater than 0
. Default value is 1000
.
*/
public final Integer docsToInvestigate() {
return docsToInvestigate;
}
/**
*
* The KMS key identifier that is used to encrypt the content on the replication instance. If you don't specify a
* value for the KmsKeyId
parameter, then DMS uses your default encryption key. KMS creates the default
* encryption key for your Amazon Web Services account. Your Amazon Web Services account has a different default
* encryption key for each Amazon Web Services Region.
*
*
* @return The KMS key identifier that is used to encrypt the content on the replication instance. If you don't
* specify a value for the KmsKeyId
parameter, then DMS uses your default encryption key. KMS
* creates the default encryption key for your Amazon Web Services account. Your Amazon Web Services account
* has a different default encryption key for each Amazon Web Services Region.
*/
public final String kmsKeyId() {
return kmsKeyId;
}
/**
*
* The full Amazon Resource Name (ARN) of the IAM role that specifies DMS as the trusted entity and grants the
* required permissions to access the value in SecretsManagerSecret
. The role must allow the
* iam:PassRole
action. SecretsManagerSecret
has the value of the Amazon Web Services
* Secrets Manager secret that allows access to the DocumentDB endpoint.
*
*
*
* You can specify one of two sets of values for these permissions. You can specify the values for this setting and
* SecretsManagerSecretId
. Or you can specify clear-text values for UserName
,
* Password
, ServerName
, and Port
. You can't specify both. For more
* information on creating this SecretsManagerSecret
and the SecretsManagerAccessRoleArn
* and SecretsManagerSecretId
required to access it, see Using
* secrets to access Database Migration Service resources in the Database Migration Service User Guide.
*
*
*
* @return The full Amazon Resource Name (ARN) of the IAM role that specifies DMS as the trusted entity and grants
* the required permissions to access the value in SecretsManagerSecret
. The role must allow
* the iam:PassRole
action. SecretsManagerSecret
has the value of the Amazon Web
* Services Secrets Manager secret that allows access to the DocumentDB endpoint.
*
* You can specify one of two sets of values for these permissions. You can specify the values for this
* setting and SecretsManagerSecretId
. Or you can specify clear-text values for
* UserName
, Password
, ServerName
, and Port
. You can't
* specify both. For more information on creating this SecretsManagerSecret
and the
* SecretsManagerAccessRoleArn
and SecretsManagerSecretId
required to access it,
* see Using secrets to access Database Migration Service resources in the Database Migration Service
* User Guide.
*
*/
public final String secretsManagerAccessRoleArn() {
return secretsManagerAccessRoleArn;
}
/**
*
* The full ARN, partial ARN, or friendly name of the SecretsManagerSecret
that contains the DocumentDB
* endpoint connection details.
*
*
* @return The full ARN, partial ARN, or friendly name of the SecretsManagerSecret
that contains the
* DocumentDB endpoint connection details.
*/
public final String secretsManagerSecretId() {
return secretsManagerSecretId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(username());
hashCode = 31 * hashCode + Objects.hashCode(password());
hashCode = 31 * hashCode + Objects.hashCode(serverName());
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(databaseName());
hashCode = 31 * hashCode + Objects.hashCode(nestingLevelAsString());
hashCode = 31 * hashCode + Objects.hashCode(extractDocId());
hashCode = 31 * hashCode + Objects.hashCode(docsToInvestigate());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(secretsManagerAccessRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(secretsManagerSecretId());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DocDbSettings)) {
return false;
}
DocDbSettings other = (DocDbSettings) obj;
return Objects.equals(username(), other.username()) && Objects.equals(password(), other.password())
&& Objects.equals(serverName(), other.serverName()) && Objects.equals(port(), other.port())
&& Objects.equals(databaseName(), other.databaseName())
&& Objects.equals(nestingLevelAsString(), other.nestingLevelAsString())
&& Objects.equals(extractDocId(), other.extractDocId())
&& Objects.equals(docsToInvestigate(), other.docsToInvestigate()) && Objects.equals(kmsKeyId(), other.kmsKeyId())
&& Objects.equals(secretsManagerAccessRoleArn(), other.secretsManagerAccessRoleArn())
&& Objects.equals(secretsManagerSecretId(), other.secretsManagerSecretId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DocDbSettings").add("Username", username())
.add("Password", password() == null ? null : "*** Sensitive Data Redacted ***").add("ServerName", serverName())
.add("Port", port()).add("DatabaseName", databaseName()).add("NestingLevel", nestingLevelAsString())
.add("ExtractDocId", extractDocId()).add("DocsToInvestigate", docsToInvestigate()).add("KmsKeyId", kmsKeyId())
.add("SecretsManagerAccessRoleArn", secretsManagerAccessRoleArn())
.add("SecretsManagerSecretId", secretsManagerSecretId()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Username":
return Optional.ofNullable(clazz.cast(username()));
case "Password":
return Optional.ofNullable(clazz.cast(password()));
case "ServerName":
return Optional.ofNullable(clazz.cast(serverName()));
case "Port":
return Optional.ofNullable(clazz.cast(port()));
case "DatabaseName":
return Optional.ofNullable(clazz.cast(databaseName()));
case "NestingLevel":
return Optional.ofNullable(clazz.cast(nestingLevelAsString()));
case "ExtractDocId":
return Optional.ofNullable(clazz.cast(extractDocId()));
case "DocsToInvestigate":
return Optional.ofNullable(clazz.cast(docsToInvestigate()));
case "KmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "SecretsManagerAccessRoleArn":
return Optional.ofNullable(clazz.cast(secretsManagerAccessRoleArn()));
case "SecretsManagerSecretId":
return Optional.ofNullable(clazz.cast(secretsManagerSecretId()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function