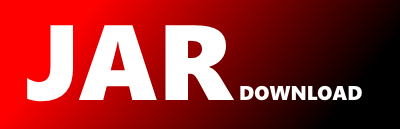
software.amazon.awssdk.services.databasemigration.model.RdsRequirements Maven / Gradle / Ivy
Show all versions of databasemigration Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.databasemigration.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides information that describes the requirements to the target engine on Amazon RDS.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RdsRequirements implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ENGINE_EDITION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EngineEdition").getter(getter(RdsRequirements::engineEdition)).setter(setter(Builder::engineEdition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngineEdition").build()).build();
private static final SdkField INSTANCE_VCPU_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("InstanceVcpu").getter(getter(RdsRequirements::instanceVcpu)).setter(setter(Builder::instanceVcpu))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceVcpu").build()).build();
private static final SdkField INSTANCE_MEMORY_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("InstanceMemory").getter(getter(RdsRequirements::instanceMemory)).setter(setter(Builder::instanceMemory))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceMemory").build()).build();
private static final SdkField STORAGE_SIZE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("StorageSize").getter(getter(RdsRequirements::storageSize)).setter(setter(Builder::storageSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StorageSize").build()).build();
private static final SdkField STORAGE_IOPS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("StorageIops").getter(getter(RdsRequirements::storageIops)).setter(setter(Builder::storageIops))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StorageIops").build()).build();
private static final SdkField DEPLOYMENT_OPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DeploymentOption").getter(getter(RdsRequirements::deploymentOption))
.setter(setter(Builder::deploymentOption))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeploymentOption").build()).build();
private static final SdkField ENGINE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EngineVersion").getter(getter(RdsRequirements::engineVersion)).setter(setter(Builder::engineVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngineVersion").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ENGINE_EDITION_FIELD,
INSTANCE_VCPU_FIELD, INSTANCE_MEMORY_FIELD, STORAGE_SIZE_FIELD, STORAGE_IOPS_FIELD, DEPLOYMENT_OPTION_FIELD,
ENGINE_VERSION_FIELD));
private static final long serialVersionUID = 1L;
private final String engineEdition;
private final Double instanceVcpu;
private final Double instanceMemory;
private final Integer storageSize;
private final Integer storageIops;
private final String deploymentOption;
private final String engineVersion;
private RdsRequirements(BuilderImpl builder) {
this.engineEdition = builder.engineEdition;
this.instanceVcpu = builder.instanceVcpu;
this.instanceMemory = builder.instanceMemory;
this.storageSize = builder.storageSize;
this.storageIops = builder.storageIops;
this.deploymentOption = builder.deploymentOption;
this.engineVersion = builder.engineVersion;
}
/**
*
* The required target Amazon RDS engine edition.
*
*
* @return The required target Amazon RDS engine edition.
*/
public final String engineEdition() {
return engineEdition;
}
/**
*
* The required number of virtual CPUs (vCPU) on the Amazon RDS DB instance.
*
*
* @return The required number of virtual CPUs (vCPU) on the Amazon RDS DB instance.
*/
public final Double instanceVcpu() {
return instanceVcpu;
}
/**
*
* The required memory on the Amazon RDS DB instance.
*
*
* @return The required memory on the Amazon RDS DB instance.
*/
public final Double instanceMemory() {
return instanceMemory;
}
/**
*
* The required Amazon RDS DB instance storage size.
*
*
* @return The required Amazon RDS DB instance storage size.
*/
public final Integer storageSize() {
return storageSize;
}
/**
*
* The required number of I/O operations completed each second (IOPS) on your Amazon RDS DB instance.
*
*
* @return The required number of I/O operations completed each second (IOPS) on your Amazon RDS DB instance.
*/
public final Integer storageIops() {
return storageIops;
}
/**
*
* The required deployment option for the Amazon RDS DB instance. Valid values include "MULTI_AZ"
for
* Multi-AZ deployments and "SINGLE_AZ"
for Single-AZ deployments.
*
*
* @return The required deployment option for the Amazon RDS DB instance. Valid values include
* "MULTI_AZ"
for Multi-AZ deployments and "SINGLE_AZ"
for Single-AZ deployments.
*/
public final String deploymentOption() {
return deploymentOption;
}
/**
*
* The required target Amazon RDS engine version.
*
*
* @return The required target Amazon RDS engine version.
*/
public final String engineVersion() {
return engineVersion;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(engineEdition());
hashCode = 31 * hashCode + Objects.hashCode(instanceVcpu());
hashCode = 31 * hashCode + Objects.hashCode(instanceMemory());
hashCode = 31 * hashCode + Objects.hashCode(storageSize());
hashCode = 31 * hashCode + Objects.hashCode(storageIops());
hashCode = 31 * hashCode + Objects.hashCode(deploymentOption());
hashCode = 31 * hashCode + Objects.hashCode(engineVersion());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RdsRequirements)) {
return false;
}
RdsRequirements other = (RdsRequirements) obj;
return Objects.equals(engineEdition(), other.engineEdition()) && Objects.equals(instanceVcpu(), other.instanceVcpu())
&& Objects.equals(instanceMemory(), other.instanceMemory()) && Objects.equals(storageSize(), other.storageSize())
&& Objects.equals(storageIops(), other.storageIops())
&& Objects.equals(deploymentOption(), other.deploymentOption())
&& Objects.equals(engineVersion(), other.engineVersion());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("RdsRequirements").add("EngineEdition", engineEdition()).add("InstanceVcpu", instanceVcpu())
.add("InstanceMemory", instanceMemory()).add("StorageSize", storageSize()).add("StorageIops", storageIops())
.add("DeploymentOption", deploymentOption()).add("EngineVersion", engineVersion()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EngineEdition":
return Optional.ofNullable(clazz.cast(engineEdition()));
case "InstanceVcpu":
return Optional.ofNullable(clazz.cast(instanceVcpu()));
case "InstanceMemory":
return Optional.ofNullable(clazz.cast(instanceMemory()));
case "StorageSize":
return Optional.ofNullable(clazz.cast(storageSize()));
case "StorageIops":
return Optional.ofNullable(clazz.cast(storageIops()));
case "DeploymentOption":
return Optional.ofNullable(clazz.cast(deploymentOption()));
case "EngineVersion":
return Optional.ofNullable(clazz.cast(engineVersion()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function