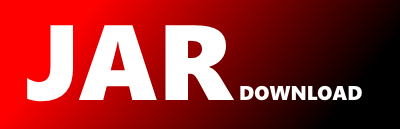
software.amazon.awssdk.services.databasemigration.model.RedisSettings Maven / Gradle / Ivy
Show all versions of databasemigration Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.databasemigration.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides information that defines a Redis target endpoint.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RedisSettings implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField SERVER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServerName").getter(getter(RedisSettings::serverName)).setter(setter(Builder::serverName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServerName").build()).build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Port")
.getter(getter(RedisSettings::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Port").build()).build();
private static final SdkField SSL_SECURITY_PROTOCOL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SslSecurityProtocol").getter(getter(RedisSettings::sslSecurityProtocolAsString))
.setter(setter(Builder::sslSecurityProtocol))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SslSecurityProtocol").build())
.build();
private static final SdkField AUTH_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AuthType").getter(getter(RedisSettings::authTypeAsString)).setter(setter(Builder::authType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthType").build()).build();
private static final SdkField AUTH_USER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AuthUserName").getter(getter(RedisSettings::authUserName)).setter(setter(Builder::authUserName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthUserName").build()).build();
private static final SdkField AUTH_PASSWORD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AuthPassword").getter(getter(RedisSettings::authPassword)).setter(setter(Builder::authPassword))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthPassword").build()).build();
private static final SdkField SSL_CA_CERTIFICATE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SslCaCertificateArn").getter(getter(RedisSettings::sslCaCertificateArn))
.setter(setter(Builder::sslCaCertificateArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SslCaCertificateArn").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays
.asList(SERVER_NAME_FIELD, PORT_FIELD, SSL_SECURITY_PROTOCOL_FIELD, AUTH_TYPE_FIELD, AUTH_USER_NAME_FIELD,
AUTH_PASSWORD_FIELD, SSL_CA_CERTIFICATE_ARN_FIELD));
private static final long serialVersionUID = 1L;
private final String serverName;
private final Integer port;
private final String sslSecurityProtocol;
private final String authType;
private final String authUserName;
private final String authPassword;
private final String sslCaCertificateArn;
private RedisSettings(BuilderImpl builder) {
this.serverName = builder.serverName;
this.port = builder.port;
this.sslSecurityProtocol = builder.sslSecurityProtocol;
this.authType = builder.authType;
this.authUserName = builder.authUserName;
this.authPassword = builder.authPassword;
this.sslCaCertificateArn = builder.sslCaCertificateArn;
}
/**
*
* Fully qualified domain name of the endpoint.
*
*
* @return Fully qualified domain name of the endpoint.
*/
public final String serverName() {
return serverName;
}
/**
*
* Transmission Control Protocol (TCP) port for the endpoint.
*
*
* @return Transmission Control Protocol (TCP) port for the endpoint.
*/
public final Integer port() {
return port;
}
/**
*
* The connection to a Redis target endpoint using Transport Layer Security (TLS). Valid values include
* plaintext
and ssl-encryption
. The default is ssl-encryption
. The
* ssl-encryption
option makes an encrypted connection. Optionally, you can identify an Amazon Resource
* Name (ARN) for an SSL certificate authority (CA) using the SslCaCertificateArn
setting. If an ARN
* isn't given for a CA, DMS uses the Amazon root CA.
*
*
* The plaintext
option doesn't provide Transport Layer Security (TLS) encryption for traffic between
* endpoint and database.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #sslSecurityProtocol} will return {@link SslSecurityProtocolValue#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #sslSecurityProtocolAsString}.
*
*
* @return The connection to a Redis target endpoint using Transport Layer Security (TLS). Valid values include
* plaintext
and ssl-encryption
. The default is ssl-encryption
. The
* ssl-encryption
option makes an encrypted connection. Optionally, you can identify an Amazon
* Resource Name (ARN) for an SSL certificate authority (CA) using the SslCaCertificateArn
* setting. If an ARN isn't given for a CA, DMS uses the Amazon root CA.
*
* The plaintext
option doesn't provide Transport Layer Security (TLS) encryption for traffic
* between endpoint and database.
* @see SslSecurityProtocolValue
*/
public final SslSecurityProtocolValue sslSecurityProtocol() {
return SslSecurityProtocolValue.fromValue(sslSecurityProtocol);
}
/**
*
* The connection to a Redis target endpoint using Transport Layer Security (TLS). Valid values include
* plaintext
and ssl-encryption
. The default is ssl-encryption
. The
* ssl-encryption
option makes an encrypted connection. Optionally, you can identify an Amazon Resource
* Name (ARN) for an SSL certificate authority (CA) using the SslCaCertificateArn
setting. If an ARN
* isn't given for a CA, DMS uses the Amazon root CA.
*
*
* The plaintext
option doesn't provide Transport Layer Security (TLS) encryption for traffic between
* endpoint and database.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #sslSecurityProtocol} will return {@link SslSecurityProtocolValue#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #sslSecurityProtocolAsString}.
*
*
* @return The connection to a Redis target endpoint using Transport Layer Security (TLS). Valid values include
* plaintext
and ssl-encryption
. The default is ssl-encryption
. The
* ssl-encryption
option makes an encrypted connection. Optionally, you can identify an Amazon
* Resource Name (ARN) for an SSL certificate authority (CA) using the SslCaCertificateArn
* setting. If an ARN isn't given for a CA, DMS uses the Amazon root CA.
*
* The plaintext
option doesn't provide Transport Layer Security (TLS) encryption for traffic
* between endpoint and database.
* @see SslSecurityProtocolValue
*/
public final String sslSecurityProtocolAsString() {
return sslSecurityProtocol;
}
/**
*
* The type of authentication to perform when connecting to a Redis target. Options include none
,
* auth-token
, and auth-role
. The auth-token
option requires an
* AuthPassword
value to be provided. The auth-role
option requires
* AuthUserName
and AuthPassword
values to be provided.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #authType} will
* return {@link RedisAuthTypeValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #authTypeAsString}.
*
*
* @return The type of authentication to perform when connecting to a Redis target. Options include
* none
, auth-token
, and auth-role
. The auth-token
* option requires an AuthPassword
value to be provided. The auth-role
option
* requires AuthUserName
and AuthPassword
values to be provided.
* @see RedisAuthTypeValue
*/
public final RedisAuthTypeValue authType() {
return RedisAuthTypeValue.fromValue(authType);
}
/**
*
* The type of authentication to perform when connecting to a Redis target. Options include none
,
* auth-token
, and auth-role
. The auth-token
option requires an
* AuthPassword
value to be provided. The auth-role
option requires
* AuthUserName
and AuthPassword
values to be provided.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #authType} will
* return {@link RedisAuthTypeValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #authTypeAsString}.
*
*
* @return The type of authentication to perform when connecting to a Redis target. Options include
* none
, auth-token
, and auth-role
. The auth-token
* option requires an AuthPassword
value to be provided. The auth-role
option
* requires AuthUserName
and AuthPassword
values to be provided.
* @see RedisAuthTypeValue
*/
public final String authTypeAsString() {
return authType;
}
/**
*
* The user name provided with the auth-role
option of the AuthType
setting for a Redis
* target endpoint.
*
*
* @return The user name provided with the auth-role
option of the AuthType
setting for a
* Redis target endpoint.
*/
public final String authUserName() {
return authUserName;
}
/**
*
* The password provided with the auth-role
and auth-token
options of the
* AuthType
setting for a Redis target endpoint.
*
*
* @return The password provided with the auth-role
and auth-token
options of the
* AuthType
setting for a Redis target endpoint.
*/
public final String authPassword() {
return authPassword;
}
/**
*
* The Amazon Resource Name (ARN) for the certificate authority (CA) that DMS uses to connect to your Redis target
* endpoint.
*
*
* @return The Amazon Resource Name (ARN) for the certificate authority (CA) that DMS uses to connect to your Redis
* target endpoint.
*/
public final String sslCaCertificateArn() {
return sslCaCertificateArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(serverName());
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(sslSecurityProtocolAsString());
hashCode = 31 * hashCode + Objects.hashCode(authTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(authUserName());
hashCode = 31 * hashCode + Objects.hashCode(authPassword());
hashCode = 31 * hashCode + Objects.hashCode(sslCaCertificateArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RedisSettings)) {
return false;
}
RedisSettings other = (RedisSettings) obj;
return Objects.equals(serverName(), other.serverName()) && Objects.equals(port(), other.port())
&& Objects.equals(sslSecurityProtocolAsString(), other.sslSecurityProtocolAsString())
&& Objects.equals(authTypeAsString(), other.authTypeAsString())
&& Objects.equals(authUserName(), other.authUserName()) && Objects.equals(authPassword(), other.authPassword())
&& Objects.equals(sslCaCertificateArn(), other.sslCaCertificateArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("RedisSettings").add("ServerName", serverName()).add("Port", port())
.add("SslSecurityProtocol", sslSecurityProtocolAsString()).add("AuthType", authTypeAsString())
.add("AuthUserName", authUserName())
.add("AuthPassword", authPassword() == null ? null : "*** Sensitive Data Redacted ***")
.add("SslCaCertificateArn", sslCaCertificateArn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ServerName":
return Optional.ofNullable(clazz.cast(serverName()));
case "Port":
return Optional.ofNullable(clazz.cast(port()));
case "SslSecurityProtocol":
return Optional.ofNullable(clazz.cast(sslSecurityProtocolAsString()));
case "AuthType":
return Optional.ofNullable(clazz.cast(authTypeAsString()));
case "AuthUserName":
return Optional.ofNullable(clazz.cast(authUserName()));
case "AuthPassword":
return Optional.ofNullable(clazz.cast(authPassword()));
case "SslCaCertificateArn":
return Optional.ofNullable(clazz.cast(sslCaCertificateArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function