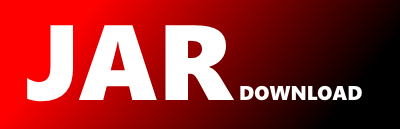
software.amazon.awssdk.services.databasemigration.model.FleetAdvisorSchemaObjectResponse Maven / Gradle / Ivy
Show all versions of databasemigration Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.databasemigration.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a schema object in a Fleet Advisor collector inventory.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class FleetAdvisorSchemaObjectResponse implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField SCHEMA_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SchemaId").getter(getter(FleetAdvisorSchemaObjectResponse::schemaId)).setter(setter(Builder::schemaId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SchemaId").build()).build();
private static final SdkField OBJECT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ObjectType").getter(getter(FleetAdvisorSchemaObjectResponse::objectType))
.setter(setter(Builder::objectType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ObjectType").build()).build();
private static final SdkField NUMBER_OF_OBJECTS_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("NumberOfObjects").getter(getter(FleetAdvisorSchemaObjectResponse::numberOfObjects))
.setter(setter(Builder::numberOfObjects))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumberOfObjects").build()).build();
private static final SdkField CODE_LINE_COUNT_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("CodeLineCount").getter(getter(FleetAdvisorSchemaObjectResponse::codeLineCount))
.setter(setter(Builder::codeLineCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CodeLineCount").build()).build();
private static final SdkField CODE_SIZE_FIELD = SdkField. builder(MarshallingType.LONG).memberName("CodeSize")
.getter(getter(FleetAdvisorSchemaObjectResponse::codeSize)).setter(setter(Builder::codeSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CodeSize").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SCHEMA_ID_FIELD,
OBJECT_TYPE_FIELD, NUMBER_OF_OBJECTS_FIELD, CODE_LINE_COUNT_FIELD, CODE_SIZE_FIELD));
private static final long serialVersionUID = 1L;
private final String schemaId;
private final String objectType;
private final Long numberOfObjects;
private final Long codeLineCount;
private final Long codeSize;
private FleetAdvisorSchemaObjectResponse(BuilderImpl builder) {
this.schemaId = builder.schemaId;
this.objectType = builder.objectType;
this.numberOfObjects = builder.numberOfObjects;
this.codeLineCount = builder.codeLineCount;
this.codeSize = builder.codeSize;
}
/**
*
* The ID of a schema object in a Fleet Advisor collector inventory.
*
*
* @return The ID of a schema object in a Fleet Advisor collector inventory.
*/
public final String schemaId() {
return schemaId;
}
/**
*
* The type of the schema object, as reported by the database engine. Examples include the following:
*
*
* -
*
* function
*
*
* -
*
* trigger
*
*
* -
*
* SYSTEM_TABLE
*
*
* -
*
* QUEUE
*
*
*
*
* @return The type of the schema object, as reported by the database engine. Examples include the following:
*
* -
*
* function
*
*
* -
*
* trigger
*
*
* -
*
* SYSTEM_TABLE
*
*
* -
*
* QUEUE
*
*
*/
public final String objectType() {
return objectType;
}
/**
*
* The number of objects in a schema object in a Fleet Advisor collector inventory.
*
*
* @return The number of objects in a schema object in a Fleet Advisor collector inventory.
*/
public final Long numberOfObjects() {
return numberOfObjects;
}
/**
*
* The number of lines of code in a schema object in a Fleet Advisor collector inventory.
*
*
* @return The number of lines of code in a schema object in a Fleet Advisor collector inventory.
*/
public final Long codeLineCount() {
return codeLineCount;
}
/**
*
* The size level of the code in a schema object in a Fleet Advisor collector inventory.
*
*
* @return The size level of the code in a schema object in a Fleet Advisor collector inventory.
*/
public final Long codeSize() {
return codeSize;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(schemaId());
hashCode = 31 * hashCode + Objects.hashCode(objectType());
hashCode = 31 * hashCode + Objects.hashCode(numberOfObjects());
hashCode = 31 * hashCode + Objects.hashCode(codeLineCount());
hashCode = 31 * hashCode + Objects.hashCode(codeSize());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof FleetAdvisorSchemaObjectResponse)) {
return false;
}
FleetAdvisorSchemaObjectResponse other = (FleetAdvisorSchemaObjectResponse) obj;
return Objects.equals(schemaId(), other.schemaId()) && Objects.equals(objectType(), other.objectType())
&& Objects.equals(numberOfObjects(), other.numberOfObjects())
&& Objects.equals(codeLineCount(), other.codeLineCount()) && Objects.equals(codeSize(), other.codeSize());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("FleetAdvisorSchemaObjectResponse").add("SchemaId", schemaId()).add("ObjectType", objectType())
.add("NumberOfObjects", numberOfObjects()).add("CodeLineCount", codeLineCount()).add("CodeSize", codeSize())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "SchemaId":
return Optional.ofNullable(clazz.cast(schemaId()));
case "ObjectType":
return Optional.ofNullable(clazz.cast(objectType()));
case "NumberOfObjects":
return Optional.ofNullable(clazz.cast(numberOfObjects()));
case "CodeLineCount":
return Optional.ofNullable(clazz.cast(codeLineCount()));
case "CodeSize":
return Optional.ofNullable(clazz.cast(codeSize()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function