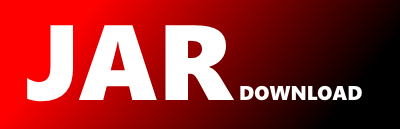
software.amazon.awssdk.services.databasemigration.model.ModifyDataProviderRequest Maven / Gradle / Ivy
Show all versions of databasemigration Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.databasemigration.model;
import java.beans.Transient;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class ModifyDataProviderRequest extends DatabaseMigrationRequest implements
ToCopyableBuilder {
private static final SdkField DATA_PROVIDER_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DataProviderIdentifier").getter(getter(ModifyDataProviderRequest::dataProviderIdentifier))
.setter(setter(Builder::dataProviderIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataProviderIdentifier").build())
.build();
private static final SdkField DATA_PROVIDER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DataProviderName").getter(getter(ModifyDataProviderRequest::dataProviderName))
.setter(setter(Builder::dataProviderName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataProviderName").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(ModifyDataProviderRequest::description))
.setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField ENGINE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Engine")
.getter(getter(ModifyDataProviderRequest::engine)).setter(setter(Builder::engine))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Engine").build()).build();
private static final SdkField EXACT_SETTINGS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ExactSettings").getter(getter(ModifyDataProviderRequest::exactSettings))
.setter(setter(Builder::exactSettings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExactSettings").build()).build();
private static final SdkField SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Settings")
.getter(getter(ModifyDataProviderRequest::settings)).setter(setter(Builder::settings))
.constructor(DataProviderSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Settings").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
DATA_PROVIDER_IDENTIFIER_FIELD, DATA_PROVIDER_NAME_FIELD, DESCRIPTION_FIELD, ENGINE_FIELD, EXACT_SETTINGS_FIELD,
SETTINGS_FIELD));
private final String dataProviderIdentifier;
private final String dataProviderName;
private final String description;
private final String engine;
private final Boolean exactSettings;
private final DataProviderSettings settings;
private ModifyDataProviderRequest(BuilderImpl builder) {
super(builder);
this.dataProviderIdentifier = builder.dataProviderIdentifier;
this.dataProviderName = builder.dataProviderName;
this.description = builder.description;
this.engine = builder.engine;
this.exactSettings = builder.exactSettings;
this.settings = builder.settings;
}
/**
*
* The identifier of the data provider. Identifiers must begin with a letter and must contain only ASCII letters,
* digits, and hyphens. They can't end with a hyphen, or contain two consecutive hyphens.
*
*
* @return The identifier of the data provider. Identifiers must begin with a letter and must contain only ASCII
* letters, digits, and hyphens. They can't end with a hyphen, or contain two consecutive hyphens.
*/
public final String dataProviderIdentifier() {
return dataProviderIdentifier;
}
/**
*
* The name of the data provider.
*
*
* @return The name of the data provider.
*/
public final String dataProviderName() {
return dataProviderName;
}
/**
*
* A user-friendly description of the data provider.
*
*
* @return A user-friendly description of the data provider.
*/
public final String description() {
return description;
}
/**
*
* The type of database engine for the data provider. Valid values include "aurora"
,
* "aurora-postgresql"
, "mysql"
, "oracle"
, "postgres"
,
* "sqlserver"
, redshift
, mariadb
, mongodb
, and
* docdb
. A value of "aurora"
represents Amazon Aurora MySQL-Compatible Edition.
*
*
* @return The type of database engine for the data provider. Valid values include "aurora"
,
* "aurora-postgresql"
, "mysql"
, "oracle"
, "postgres"
,
* "sqlserver"
, redshift
, mariadb
, mongodb
, and
* docdb
. A value of "aurora"
represents Amazon Aurora MySQL-Compatible Edition.
*/
public final String engine() {
return engine;
}
/**
*
* If this attribute is Y, the current call to ModifyDataProvider
replaces all existing data provider
* settings with the exact settings that you specify in this call. If this attribute is N, the current call to
* ModifyDataProvider
does two things:
*
*
* -
*
* It replaces any data provider settings that already exist with new values, for settings with the same names.
*
*
* -
*
* It creates new data provider settings that you specify in the call, for settings with different names.
*
*
*
*
* @return If this attribute is Y, the current call to ModifyDataProvider
replaces all existing data
* provider settings with the exact settings that you specify in this call. If this attribute is N, the
* current call to ModifyDataProvider
does two things:
*
* -
*
* It replaces any data provider settings that already exist with new values, for settings with the same
* names.
*
*
* -
*
* It creates new data provider settings that you specify in the call, for settings with different names.
*
*
*/
public final Boolean exactSettings() {
return exactSettings;
}
/**
*
* The settings in JSON format for a data provider.
*
*
* @return The settings in JSON format for a data provider.
*/
public final DataProviderSettings settings() {
return settings;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(dataProviderIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(dataProviderName());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(engine());
hashCode = 31 * hashCode + Objects.hashCode(exactSettings());
hashCode = 31 * hashCode + Objects.hashCode(settings());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ModifyDataProviderRequest)) {
return false;
}
ModifyDataProviderRequest other = (ModifyDataProviderRequest) obj;
return Objects.equals(dataProviderIdentifier(), other.dataProviderIdentifier())
&& Objects.equals(dataProviderName(), other.dataProviderName())
&& Objects.equals(description(), other.description()) && Objects.equals(engine(), other.engine())
&& Objects.equals(exactSettings(), other.exactSettings()) && Objects.equals(settings(), other.settings());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ModifyDataProviderRequest").add("DataProviderIdentifier", dataProviderIdentifier())
.add("DataProviderName", dataProviderName()).add("Description", description()).add("Engine", engine())
.add("ExactSettings", exactSettings()).add("Settings", settings()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DataProviderIdentifier":
return Optional.ofNullable(clazz.cast(dataProviderIdentifier()));
case "DataProviderName":
return Optional.ofNullable(clazz.cast(dataProviderName()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "Engine":
return Optional.ofNullable(clazz.cast(engine()));
case "ExactSettings":
return Optional.ofNullable(clazz.cast(exactSettings()));
case "Settings":
return Optional.ofNullable(clazz.cast(settings()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function