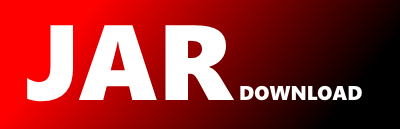
software.amazon.awssdk.services.databasemigration.model.PostgreSQLSettings Maven / Gradle / Ivy
Show all versions of databasemigration Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.databasemigration.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides information that defines a PostgreSQL endpoint.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class PostgreSQLSettings implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField AFTER_CONNECT_SCRIPT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AfterConnectScript").getter(getter(PostgreSQLSettings::afterConnectScript))
.setter(setter(Builder::afterConnectScript))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AfterConnectScript").build())
.build();
private static final SdkField CAPTURE_DDLS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("CaptureDdls").getter(getter(PostgreSQLSettings::captureDdls)).setter(setter(Builder::captureDdls))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CaptureDdls").build()).build();
private static final SdkField MAX_FILE_SIZE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxFileSize").getter(getter(PostgreSQLSettings::maxFileSize)).setter(setter(Builder::maxFileSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxFileSize").build()).build();
private static final SdkField DATABASE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DatabaseName").getter(getter(PostgreSQLSettings::databaseName)).setter(setter(Builder::databaseName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DatabaseName").build()).build();
private static final SdkField DDL_ARTIFACTS_SCHEMA_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DdlArtifactsSchema").getter(getter(PostgreSQLSettings::ddlArtifactsSchema))
.setter(setter(Builder::ddlArtifactsSchema))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DdlArtifactsSchema").build())
.build();
private static final SdkField EXECUTE_TIMEOUT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("ExecuteTimeout").getter(getter(PostgreSQLSettings::executeTimeout))
.setter(setter(Builder::executeTimeout))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExecuteTimeout").build()).build();
private static final SdkField FAIL_TASKS_ON_LOB_TRUNCATION_FIELD = SdkField
. builder(MarshallingType.BOOLEAN).memberName("FailTasksOnLobTruncation")
.getter(getter(PostgreSQLSettings::failTasksOnLobTruncation)).setter(setter(Builder::failTasksOnLobTruncation))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailTasksOnLobTruncation").build())
.build();
private static final SdkField HEARTBEAT_ENABLE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("HeartbeatEnable").getter(getter(PostgreSQLSettings::heartbeatEnable))
.setter(setter(Builder::heartbeatEnable))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HeartbeatEnable").build()).build();
private static final SdkField HEARTBEAT_SCHEMA_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HeartbeatSchema").getter(getter(PostgreSQLSettings::heartbeatSchema))
.setter(setter(Builder::heartbeatSchema))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HeartbeatSchema").build()).build();
private static final SdkField HEARTBEAT_FREQUENCY_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("HeartbeatFrequency").getter(getter(PostgreSQLSettings::heartbeatFrequency))
.setter(setter(Builder::heartbeatFrequency))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HeartbeatFrequency").build())
.build();
private static final SdkField PASSWORD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Password").getter(getter(PostgreSQLSettings::password)).setter(setter(Builder::password))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Password").build()).build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Port")
.getter(getter(PostgreSQLSettings::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Port").build()).build();
private static final SdkField SERVER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServerName").getter(getter(PostgreSQLSettings::serverName)).setter(setter(Builder::serverName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServerName").build()).build();
private static final SdkField USERNAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Username").getter(getter(PostgreSQLSettings::username)).setter(setter(Builder::username))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Username").build()).build();
private static final SdkField SLOT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SlotName").getter(getter(PostgreSQLSettings::slotName)).setter(setter(Builder::slotName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SlotName").build()).build();
private static final SdkField PLUGIN_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PluginName").getter(getter(PostgreSQLSettings::pluginNameAsString)).setter(setter(Builder::pluginName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PluginName").build()).build();
private static final SdkField SECRETS_MANAGER_ACCESS_ROLE_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SecretsManagerAccessRoleArn")
.getter(getter(PostgreSQLSettings::secretsManagerAccessRoleArn))
.setter(setter(Builder::secretsManagerAccessRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecretsManagerAccessRoleArn")
.build()).build();
private static final SdkField SECRETS_MANAGER_SECRET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SecretsManagerSecretId").getter(getter(PostgreSQLSettings::secretsManagerSecretId))
.setter(setter(Builder::secretsManagerSecretId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecretsManagerSecretId").build())
.build();
private static final SdkField TRIM_SPACE_IN_CHAR_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("TrimSpaceInChar").getter(getter(PostgreSQLSettings::trimSpaceInChar))
.setter(setter(Builder::trimSpaceInChar))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TrimSpaceInChar").build()).build();
private static final SdkField MAP_BOOLEAN_AS_BOOLEAN_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("MapBooleanAsBoolean").getter(getter(PostgreSQLSettings::mapBooleanAsBoolean))
.setter(setter(Builder::mapBooleanAsBoolean))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MapBooleanAsBoolean").build())
.build();
private static final SdkField MAP_JSONB_AS_CLOB_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("MapJsonbAsClob").getter(getter(PostgreSQLSettings::mapJsonbAsClob))
.setter(setter(Builder::mapJsonbAsClob))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MapJsonbAsClob").build()).build();
private static final SdkField MAP_LONG_VARCHAR_AS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MapLongVarcharAs").getter(getter(PostgreSQLSettings::mapLongVarcharAsAsString))
.setter(setter(Builder::mapLongVarcharAs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MapLongVarcharAs").build()).build();
private static final SdkField DATABASE_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DatabaseMode").getter(getter(PostgreSQLSettings::databaseModeAsString))
.setter(setter(Builder::databaseMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DatabaseMode").build()).build();
private static final SdkField BABELFISH_DATABASE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BabelfishDatabaseName").getter(getter(PostgreSQLSettings::babelfishDatabaseName))
.setter(setter(Builder::babelfishDatabaseName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BabelfishDatabaseName").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AFTER_CONNECT_SCRIPT_FIELD,
CAPTURE_DDLS_FIELD, MAX_FILE_SIZE_FIELD, DATABASE_NAME_FIELD, DDL_ARTIFACTS_SCHEMA_FIELD, EXECUTE_TIMEOUT_FIELD,
FAIL_TASKS_ON_LOB_TRUNCATION_FIELD, HEARTBEAT_ENABLE_FIELD, HEARTBEAT_SCHEMA_FIELD, HEARTBEAT_FREQUENCY_FIELD,
PASSWORD_FIELD, PORT_FIELD, SERVER_NAME_FIELD, USERNAME_FIELD, SLOT_NAME_FIELD, PLUGIN_NAME_FIELD,
SECRETS_MANAGER_ACCESS_ROLE_ARN_FIELD, SECRETS_MANAGER_SECRET_ID_FIELD, TRIM_SPACE_IN_CHAR_FIELD,
MAP_BOOLEAN_AS_BOOLEAN_FIELD, MAP_JSONB_AS_CLOB_FIELD, MAP_LONG_VARCHAR_AS_FIELD, DATABASE_MODE_FIELD,
BABELFISH_DATABASE_NAME_FIELD));
private static final long serialVersionUID = 1L;
private final String afterConnectScript;
private final Boolean captureDdls;
private final Integer maxFileSize;
private final String databaseName;
private final String ddlArtifactsSchema;
private final Integer executeTimeout;
private final Boolean failTasksOnLobTruncation;
private final Boolean heartbeatEnable;
private final String heartbeatSchema;
private final Integer heartbeatFrequency;
private final String password;
private final Integer port;
private final String serverName;
private final String username;
private final String slotName;
private final String pluginName;
private final String secretsManagerAccessRoleArn;
private final String secretsManagerSecretId;
private final Boolean trimSpaceInChar;
private final Boolean mapBooleanAsBoolean;
private final Boolean mapJsonbAsClob;
private final String mapLongVarcharAs;
private final String databaseMode;
private final String babelfishDatabaseName;
private PostgreSQLSettings(BuilderImpl builder) {
this.afterConnectScript = builder.afterConnectScript;
this.captureDdls = builder.captureDdls;
this.maxFileSize = builder.maxFileSize;
this.databaseName = builder.databaseName;
this.ddlArtifactsSchema = builder.ddlArtifactsSchema;
this.executeTimeout = builder.executeTimeout;
this.failTasksOnLobTruncation = builder.failTasksOnLobTruncation;
this.heartbeatEnable = builder.heartbeatEnable;
this.heartbeatSchema = builder.heartbeatSchema;
this.heartbeatFrequency = builder.heartbeatFrequency;
this.password = builder.password;
this.port = builder.port;
this.serverName = builder.serverName;
this.username = builder.username;
this.slotName = builder.slotName;
this.pluginName = builder.pluginName;
this.secretsManagerAccessRoleArn = builder.secretsManagerAccessRoleArn;
this.secretsManagerSecretId = builder.secretsManagerSecretId;
this.trimSpaceInChar = builder.trimSpaceInChar;
this.mapBooleanAsBoolean = builder.mapBooleanAsBoolean;
this.mapJsonbAsClob = builder.mapJsonbAsClob;
this.mapLongVarcharAs = builder.mapLongVarcharAs;
this.databaseMode = builder.databaseMode;
this.babelfishDatabaseName = builder.babelfishDatabaseName;
}
/**
*
* For use with change data capture (CDC) only, this attribute has DMS bypass foreign keys and user triggers to
* reduce the time it takes to bulk load data.
*
*
* Example: afterConnectScript=SET session_replication_role='replica'
*
*
* @return For use with change data capture (CDC) only, this attribute has DMS bypass foreign keys and user triggers
* to reduce the time it takes to bulk load data.
*
* Example: afterConnectScript=SET session_replication_role='replica'
*/
public final String afterConnectScript() {
return afterConnectScript;
}
/**
*
* To capture DDL events, DMS creates various artifacts in the PostgreSQL database when the task starts. You can
* later remove these artifacts.
*
*
* If this value is set to N
, you don't have to create tables or triggers on the source database.
*
*
* @return To capture DDL events, DMS creates various artifacts in the PostgreSQL database when the task starts. You
* can later remove these artifacts.
*
* If this value is set to N
, you don't have to create tables or triggers on the source
* database.
*/
public final Boolean captureDdls() {
return captureDdls;
}
/**
*
* Specifies the maximum size (in KB) of any .csv file used to transfer data to PostgreSQL.
*
*
* Example: maxFileSize=512
*
*
* @return Specifies the maximum size (in KB) of any .csv file used to transfer data to PostgreSQL.
*
* Example: maxFileSize=512
*/
public final Integer maxFileSize() {
return maxFileSize;
}
/**
*
* Database name for the endpoint.
*
*
* @return Database name for the endpoint.
*/
public final String databaseName() {
return databaseName;
}
/**
*
* The schema in which the operational DDL database artifacts are created.
*
*
* Example: ddlArtifactsSchema=xyzddlschema;
*
*
* @return The schema in which the operational DDL database artifacts are created.
*
* Example: ddlArtifactsSchema=xyzddlschema;
*/
public final String ddlArtifactsSchema() {
return ddlArtifactsSchema;
}
/**
*
* Sets the client statement timeout for the PostgreSQL instance, in seconds. The default value is 60 seconds.
*
*
* Example: executeTimeout=100;
*
*
* @return Sets the client statement timeout for the PostgreSQL instance, in seconds. The default value is 60
* seconds.
*
* Example: executeTimeout=100;
*/
public final Integer executeTimeout() {
return executeTimeout;
}
/**
*
* When set to true
, this value causes a task to fail if the actual size of a LOB column is greater
* than the specified LobMaxSize
.
*
*
* If task is set to Limited LOB mode and this option is set to true, the task fails instead of truncating the LOB
* data.
*
*
* @return When set to true
, this value causes a task to fail if the actual size of a LOB column is
* greater than the specified LobMaxSize
.
*
* If task is set to Limited LOB mode and this option is set to true, the task fails instead of truncating
* the LOB data.
*/
public final Boolean failTasksOnLobTruncation() {
return failTasksOnLobTruncation;
}
/**
*
* The write-ahead log (WAL) heartbeat feature mimics a dummy transaction. By doing this, it prevents idle logical
* replication slots from holding onto old WAL logs, which can result in storage full situations on the source. This
* heartbeat keeps restart_lsn
moving and prevents storage full scenarios.
*
*
* @return The write-ahead log (WAL) heartbeat feature mimics a dummy transaction. By doing this, it prevents idle
* logical replication slots from holding onto old WAL logs, which can result in storage full situations on
* the source. This heartbeat keeps restart_lsn
moving and prevents storage full scenarios.
*/
public final Boolean heartbeatEnable() {
return heartbeatEnable;
}
/**
*
* Sets the schema in which the heartbeat artifacts are created.
*
*
* @return Sets the schema in which the heartbeat artifacts are created.
*/
public final String heartbeatSchema() {
return heartbeatSchema;
}
/**
*
* Sets the WAL heartbeat frequency (in minutes).
*
*
* @return Sets the WAL heartbeat frequency (in minutes).
*/
public final Integer heartbeatFrequency() {
return heartbeatFrequency;
}
/**
*
* Endpoint connection password.
*
*
* @return Endpoint connection password.
*/
public final String password() {
return password;
}
/**
*
* Endpoint TCP port. The default is 5432.
*
*
* @return Endpoint TCP port. The default is 5432.
*/
public final Integer port() {
return port;
}
/**
*
* The host name of the endpoint database.
*
*
* For an Amazon RDS PostgreSQL instance, this is the output of DescribeDBInstances, in the
* Endpoint.Address
* field.
*
*
* For an Aurora PostgreSQL instance, this is the output of DescribeDBClusters, in the Endpoint
field.
*
*
* @return The host name of the endpoint database.
*
* For an Amazon RDS PostgreSQL instance, this is the output of DescribeDBInstances, in the
* Endpoint.Address
* field.
*
*
* For an Aurora PostgreSQL instance, this is the output of DescribeDBClusters, in the Endpoint
field.
*/
public final String serverName() {
return serverName;
}
/**
*
* Endpoint connection user name.
*
*
* @return Endpoint connection user name.
*/
public final String username() {
return username;
}
/**
*
* Sets the name of a previously created logical replication slot for a change data capture (CDC) load of the
* PostgreSQL source instance.
*
*
* When used with the CdcStartPosition
request parameter for the DMS API , this attribute also makes it
* possible to use native CDC start points. DMS verifies that the specified logical replication slot exists before
* starting the CDC load task. It also verifies that the task was created with a valid setting of
* CdcStartPosition
. If the specified slot doesn't exist or the task doesn't have a valid
* CdcStartPosition
setting, DMS raises an error.
*
*
* For more information about setting the CdcStartPosition
request parameter, see Determining a CDC native start point in the Database Migration Service User Guide. For more
* information about using CdcStartPosition
, see CreateReplicationTask, StartReplicationTask, and ModifyReplicationTask.
*
*
* @return Sets the name of a previously created logical replication slot for a change data capture (CDC) load of
* the PostgreSQL source instance.
*
* When used with the CdcStartPosition
request parameter for the DMS API , this attribute also
* makes it possible to use native CDC start points. DMS verifies that the specified logical replication
* slot exists before starting the CDC load task. It also verifies that the task was created with a valid
* setting of CdcStartPosition
. If the specified slot doesn't exist or the task doesn't have a
* valid CdcStartPosition
setting, DMS raises an error.
*
*
* For more information about setting the CdcStartPosition
request parameter, see Determining a CDC native start point in the Database Migration Service User Guide. For more
* information about using CdcStartPosition
, see CreateReplicationTask, StartReplicationTask, and ModifyReplicationTask.
*/
public final String slotName() {
return slotName;
}
/**
*
* Specifies the plugin to use to create a replication slot.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #pluginName} will
* return {@link PluginNameValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #pluginNameAsString}.
*
*
* @return Specifies the plugin to use to create a replication slot.
* @see PluginNameValue
*/
public final PluginNameValue pluginName() {
return PluginNameValue.fromValue(pluginName);
}
/**
*
* Specifies the plugin to use to create a replication slot.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #pluginName} will
* return {@link PluginNameValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #pluginNameAsString}.
*
*
* @return Specifies the plugin to use to create a replication slot.
* @see PluginNameValue
*/
public final String pluginNameAsString() {
return pluginName;
}
/**
*
* The full Amazon Resource Name (ARN) of the IAM role that specifies DMS as the trusted entity and grants the
* required permissions to access the value in SecretsManagerSecret
. The role must allow the
* iam:PassRole
action. SecretsManagerSecret
has the value of the Amazon Web Services
* Secrets Manager secret that allows access to the PostgreSQL endpoint.
*
*
*
* You can specify one of two sets of values for these permissions. You can specify the values for this setting and
* SecretsManagerSecretId
. Or you can specify clear-text values for UserName
,
* Password
, ServerName
, and Port
. You can't specify both. For more
* information on creating this SecretsManagerSecret
and the SecretsManagerAccessRoleArn
* and SecretsManagerSecretId
required to access it, see Using
* secrets to access Database Migration Service resources in the Database Migration Service User Guide.
*
*
*
* @return The full Amazon Resource Name (ARN) of the IAM role that specifies DMS as the trusted entity and grants
* the required permissions to access the value in SecretsManagerSecret
. The role must allow
* the iam:PassRole
action. SecretsManagerSecret
has the value of the Amazon Web
* Services Secrets Manager secret that allows access to the PostgreSQL endpoint.
*
* You can specify one of two sets of values for these permissions. You can specify the values for this
* setting and SecretsManagerSecretId
. Or you can specify clear-text values for
* UserName
, Password
, ServerName
, and Port
. You can't
* specify both. For more information on creating this SecretsManagerSecret
and the
* SecretsManagerAccessRoleArn
and SecretsManagerSecretId
required to access it,
* see Using secrets to access Database Migration Service resources in the Database Migration Service
* User Guide.
*
*/
public final String secretsManagerAccessRoleArn() {
return secretsManagerAccessRoleArn;
}
/**
*
* The full ARN, partial ARN, or friendly name of the SecretsManagerSecret
that contains the PostgreSQL
* endpoint connection details.
*
*
* @return The full ARN, partial ARN, or friendly name of the SecretsManagerSecret
that contains the
* PostgreSQL endpoint connection details.
*/
public final String secretsManagerSecretId() {
return secretsManagerSecretId;
}
/**
*
* Use the TrimSpaceInChar
source endpoint setting to trim data on CHAR and NCHAR data types during
* migration. The default value is true
.
*
*
* @return Use the TrimSpaceInChar
source endpoint setting to trim data on CHAR and NCHAR data types
* during migration. The default value is true
.
*/
public final Boolean trimSpaceInChar() {
return trimSpaceInChar;
}
/**
*
* When true, lets PostgreSQL migrate the boolean type as boolean. By default, PostgreSQL migrates booleans as
* varchar(5)
. You must set this setting on both the source and target endpoints for it to take effect.
*
*
* @return When true, lets PostgreSQL migrate the boolean type as boolean. By default, PostgreSQL migrates booleans
* as varchar(5)
. You must set this setting on both the source and target endpoints for it to
* take effect.
*/
public final Boolean mapBooleanAsBoolean() {
return mapBooleanAsBoolean;
}
/**
*
* When true, DMS migrates JSONB values as CLOB.
*
*
* @return When true, DMS migrates JSONB values as CLOB.
*/
public final Boolean mapJsonbAsClob() {
return mapJsonbAsClob;
}
/**
*
* When true, DMS migrates LONG values as VARCHAR.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mapLongVarcharAs}
* will return {@link LongVarcharMappingType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #mapLongVarcharAsAsString}.
*
*
* @return When true, DMS migrates LONG values as VARCHAR.
* @see LongVarcharMappingType
*/
public final LongVarcharMappingType mapLongVarcharAs() {
return LongVarcharMappingType.fromValue(mapLongVarcharAs);
}
/**
*
* When true, DMS migrates LONG values as VARCHAR.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mapLongVarcharAs}
* will return {@link LongVarcharMappingType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #mapLongVarcharAsAsString}.
*
*
* @return When true, DMS migrates LONG values as VARCHAR.
* @see LongVarcharMappingType
*/
public final String mapLongVarcharAsAsString() {
return mapLongVarcharAs;
}
/**
*
* Specifies the default behavior of the replication's handling of PostgreSQL- compatible endpoints that require
* some additional configuration, such as Babelfish endpoints.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #databaseMode} will
* return {@link DatabaseMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #databaseModeAsString}.
*
*
* @return Specifies the default behavior of the replication's handling of PostgreSQL- compatible endpoints that
* require some additional configuration, such as Babelfish endpoints.
* @see DatabaseMode
*/
public final DatabaseMode databaseMode() {
return DatabaseMode.fromValue(databaseMode);
}
/**
*
* Specifies the default behavior of the replication's handling of PostgreSQL- compatible endpoints that require
* some additional configuration, such as Babelfish endpoints.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #databaseMode} will
* return {@link DatabaseMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #databaseModeAsString}.
*
*
* @return Specifies the default behavior of the replication's handling of PostgreSQL- compatible endpoints that
* require some additional configuration, such as Babelfish endpoints.
* @see DatabaseMode
*/
public final String databaseModeAsString() {
return databaseMode;
}
/**
*
* The Babelfish for Aurora PostgreSQL database name for the endpoint.
*
*
* @return The Babelfish for Aurora PostgreSQL database name for the endpoint.
*/
public final String babelfishDatabaseName() {
return babelfishDatabaseName;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(afterConnectScript());
hashCode = 31 * hashCode + Objects.hashCode(captureDdls());
hashCode = 31 * hashCode + Objects.hashCode(maxFileSize());
hashCode = 31 * hashCode + Objects.hashCode(databaseName());
hashCode = 31 * hashCode + Objects.hashCode(ddlArtifactsSchema());
hashCode = 31 * hashCode + Objects.hashCode(executeTimeout());
hashCode = 31 * hashCode + Objects.hashCode(failTasksOnLobTruncation());
hashCode = 31 * hashCode + Objects.hashCode(heartbeatEnable());
hashCode = 31 * hashCode + Objects.hashCode(heartbeatSchema());
hashCode = 31 * hashCode + Objects.hashCode(heartbeatFrequency());
hashCode = 31 * hashCode + Objects.hashCode(password());
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(serverName());
hashCode = 31 * hashCode + Objects.hashCode(username());
hashCode = 31 * hashCode + Objects.hashCode(slotName());
hashCode = 31 * hashCode + Objects.hashCode(pluginNameAsString());
hashCode = 31 * hashCode + Objects.hashCode(secretsManagerAccessRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(secretsManagerSecretId());
hashCode = 31 * hashCode + Objects.hashCode(trimSpaceInChar());
hashCode = 31 * hashCode + Objects.hashCode(mapBooleanAsBoolean());
hashCode = 31 * hashCode + Objects.hashCode(mapJsonbAsClob());
hashCode = 31 * hashCode + Objects.hashCode(mapLongVarcharAsAsString());
hashCode = 31 * hashCode + Objects.hashCode(databaseModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(babelfishDatabaseName());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PostgreSQLSettings)) {
return false;
}
PostgreSQLSettings other = (PostgreSQLSettings) obj;
return Objects.equals(afterConnectScript(), other.afterConnectScript())
&& Objects.equals(captureDdls(), other.captureDdls()) && Objects.equals(maxFileSize(), other.maxFileSize())
&& Objects.equals(databaseName(), other.databaseName())
&& Objects.equals(ddlArtifactsSchema(), other.ddlArtifactsSchema())
&& Objects.equals(executeTimeout(), other.executeTimeout())
&& Objects.equals(failTasksOnLobTruncation(), other.failTasksOnLobTruncation())
&& Objects.equals(heartbeatEnable(), other.heartbeatEnable())
&& Objects.equals(heartbeatSchema(), other.heartbeatSchema())
&& Objects.equals(heartbeatFrequency(), other.heartbeatFrequency())
&& Objects.equals(password(), other.password()) && Objects.equals(port(), other.port())
&& Objects.equals(serverName(), other.serverName()) && Objects.equals(username(), other.username())
&& Objects.equals(slotName(), other.slotName())
&& Objects.equals(pluginNameAsString(), other.pluginNameAsString())
&& Objects.equals(secretsManagerAccessRoleArn(), other.secretsManagerAccessRoleArn())
&& Objects.equals(secretsManagerSecretId(), other.secretsManagerSecretId())
&& Objects.equals(trimSpaceInChar(), other.trimSpaceInChar())
&& Objects.equals(mapBooleanAsBoolean(), other.mapBooleanAsBoolean())
&& Objects.equals(mapJsonbAsClob(), other.mapJsonbAsClob())
&& Objects.equals(mapLongVarcharAsAsString(), other.mapLongVarcharAsAsString())
&& Objects.equals(databaseModeAsString(), other.databaseModeAsString())
&& Objects.equals(babelfishDatabaseName(), other.babelfishDatabaseName());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PostgreSQLSettings").add("AfterConnectScript", afterConnectScript())
.add("CaptureDdls", captureDdls()).add("MaxFileSize", maxFileSize()).add("DatabaseName", databaseName())
.add("DdlArtifactsSchema", ddlArtifactsSchema()).add("ExecuteTimeout", executeTimeout())
.add("FailTasksOnLobTruncation", failTasksOnLobTruncation()).add("HeartbeatEnable", heartbeatEnable())
.add("HeartbeatSchema", heartbeatSchema()).add("HeartbeatFrequency", heartbeatFrequency())
.add("Password", password() == null ? null : "*** Sensitive Data Redacted ***").add("Port", port())
.add("ServerName", serverName()).add("Username", username()).add("SlotName", slotName())
.add("PluginName", pluginNameAsString()).add("SecretsManagerAccessRoleArn", secretsManagerAccessRoleArn())
.add("SecretsManagerSecretId", secretsManagerSecretId()).add("TrimSpaceInChar", trimSpaceInChar())
.add("MapBooleanAsBoolean", mapBooleanAsBoolean()).add("MapJsonbAsClob", mapJsonbAsClob())
.add("MapLongVarcharAs", mapLongVarcharAsAsString()).add("DatabaseMode", databaseModeAsString())
.add("BabelfishDatabaseName", babelfishDatabaseName()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AfterConnectScript":
return Optional.ofNullable(clazz.cast(afterConnectScript()));
case "CaptureDdls":
return Optional.ofNullable(clazz.cast(captureDdls()));
case "MaxFileSize":
return Optional.ofNullable(clazz.cast(maxFileSize()));
case "DatabaseName":
return Optional.ofNullable(clazz.cast(databaseName()));
case "DdlArtifactsSchema":
return Optional.ofNullable(clazz.cast(ddlArtifactsSchema()));
case "ExecuteTimeout":
return Optional.ofNullable(clazz.cast(executeTimeout()));
case "FailTasksOnLobTruncation":
return Optional.ofNullable(clazz.cast(failTasksOnLobTruncation()));
case "HeartbeatEnable":
return Optional.ofNullable(clazz.cast(heartbeatEnable()));
case "HeartbeatSchema":
return Optional.ofNullable(clazz.cast(heartbeatSchema()));
case "HeartbeatFrequency":
return Optional.ofNullable(clazz.cast(heartbeatFrequency()));
case "Password":
return Optional.ofNullable(clazz.cast(password()));
case "Port":
return Optional.ofNullable(clazz.cast(port()));
case "ServerName":
return Optional.ofNullable(clazz.cast(serverName()));
case "Username":
return Optional.ofNullable(clazz.cast(username()));
case "SlotName":
return Optional.ofNullable(clazz.cast(slotName()));
case "PluginName":
return Optional.ofNullable(clazz.cast(pluginNameAsString()));
case "SecretsManagerAccessRoleArn":
return Optional.ofNullable(clazz.cast(secretsManagerAccessRoleArn()));
case "SecretsManagerSecretId":
return Optional.ofNullable(clazz.cast(secretsManagerSecretId()));
case "TrimSpaceInChar":
return Optional.ofNullable(clazz.cast(trimSpaceInChar()));
case "MapBooleanAsBoolean":
return Optional.ofNullable(clazz.cast(mapBooleanAsBoolean()));
case "MapJsonbAsClob":
return Optional.ofNullable(clazz.cast(mapJsonbAsClob()));
case "MapLongVarcharAs":
return Optional.ofNullable(clazz.cast(mapLongVarcharAsAsString()));
case "DatabaseMode":
return Optional.ofNullable(clazz.cast(databaseModeAsString()));
case "BabelfishDatabaseName":
return Optional.ofNullable(clazz.cast(babelfishDatabaseName()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function