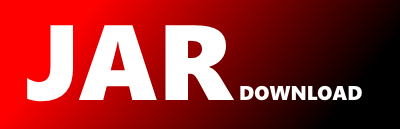
software.amazon.awssdk.services.databasemigration.model.CreateInstanceProfileRequest Maven / Gradle / Ivy
Show all versions of databasemigration Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.databasemigration.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateInstanceProfileRequest extends DatabaseMigrationRequest implements
ToCopyableBuilder {
private static final SdkField AVAILABILITY_ZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AvailabilityZone").getter(getter(CreateInstanceProfileRequest::availabilityZone))
.setter(setter(Builder::availabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailabilityZone").build()).build();
private static final SdkField KMS_KEY_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsKeyArn").getter(getter(CreateInstanceProfileRequest::kmsKeyArn)).setter(setter(Builder::kmsKeyArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KmsKeyArn").build()).build();
private static final SdkField PUBLICLY_ACCESSIBLE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("PubliclyAccessible").getter(getter(CreateInstanceProfileRequest::publiclyAccessible))
.setter(setter(Builder::publiclyAccessible))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PubliclyAccessible").build())
.build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateInstanceProfileRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField NETWORK_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NetworkType").getter(getter(CreateInstanceProfileRequest::networkType))
.setter(setter(Builder::networkType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NetworkType").build()).build();
private static final SdkField INSTANCE_PROFILE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InstanceProfileName").getter(getter(CreateInstanceProfileRequest::instanceProfileName))
.setter(setter(Builder::instanceProfileName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceProfileName").build())
.build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(CreateInstanceProfileRequest::description))
.setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField SUBNET_GROUP_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SubnetGroupIdentifier").getter(getter(CreateInstanceProfileRequest::subnetGroupIdentifier))
.setter(setter(Builder::subnetGroupIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SubnetGroupIdentifier").build())
.build();
private static final SdkField> VPC_SECURITY_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("VpcSecurityGroups")
.getter(getter(CreateInstanceProfileRequest::vpcSecurityGroups))
.setter(setter(Builder::vpcSecurityGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcSecurityGroups").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AVAILABILITY_ZONE_FIELD,
KMS_KEY_ARN_FIELD, PUBLICLY_ACCESSIBLE_FIELD, TAGS_FIELD, NETWORK_TYPE_FIELD, INSTANCE_PROFILE_NAME_FIELD,
DESCRIPTION_FIELD, SUBNET_GROUP_IDENTIFIER_FIELD, VPC_SECURITY_GROUPS_FIELD));
private final String availabilityZone;
private final String kmsKeyArn;
private final Boolean publiclyAccessible;
private final List tags;
private final String networkType;
private final String instanceProfileName;
private final String description;
private final String subnetGroupIdentifier;
private final List vpcSecurityGroups;
private CreateInstanceProfileRequest(BuilderImpl builder) {
super(builder);
this.availabilityZone = builder.availabilityZone;
this.kmsKeyArn = builder.kmsKeyArn;
this.publiclyAccessible = builder.publiclyAccessible;
this.tags = builder.tags;
this.networkType = builder.networkType;
this.instanceProfileName = builder.instanceProfileName;
this.description = builder.description;
this.subnetGroupIdentifier = builder.subnetGroupIdentifier;
this.vpcSecurityGroups = builder.vpcSecurityGroups;
}
/**
*
* The Availability Zone where the instance profile will be created. The default value is a random, system-chosen
* Availability Zone in the Amazon Web Services Region where your data provider is created, for examplem
* us-east-1d
.
*
*
* @return The Availability Zone where the instance profile will be created. The default value is a random,
* system-chosen Availability Zone in the Amazon Web Services Region where your data provider is created,
* for examplem us-east-1d
.
*/
public final String availabilityZone() {
return availabilityZone;
}
/**
*
* The Amazon Resource Name (ARN) of the KMS key that is used to encrypt the connection parameters for the instance
* profile.
*
*
* If you don't specify a value for the KmsKeyArn
parameter, then DMS uses your default encryption key.
*
*
* KMS creates the default encryption key for your Amazon Web Services account. Your Amazon Web Services account has
* a different default encryption key for each Amazon Web Services Region.
*
*
* @return The Amazon Resource Name (ARN) of the KMS key that is used to encrypt the connection parameters for the
* instance profile.
*
* If you don't specify a value for the KmsKeyArn
parameter, then DMS uses your default
* encryption key.
*
*
* KMS creates the default encryption key for your Amazon Web Services account. Your Amazon Web Services
* account has a different default encryption key for each Amazon Web Services Region.
*/
public final String kmsKeyArn() {
return kmsKeyArn;
}
/**
*
* Specifies the accessibility options for the instance profile. A value of true
represents an instance
* profile with a public IP address. A value of false
represents an instance profile with a private IP
* address. The default value is true
.
*
*
* @return Specifies the accessibility options for the instance profile. A value of true
represents an
* instance profile with a public IP address. A value of false
represents an instance profile
* with a private IP address. The default value is true
.
*/
public final Boolean publiclyAccessible() {
return publiclyAccessible;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* One or more tags to be assigned to the instance profile.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return One or more tags to be assigned to the instance profile.
*/
public final List tags() {
return tags;
}
/**
*
* Specifies the network type for the instance profile. A value of IPV4
represents an instance profile
* with IPv4 network type and only supports IPv4 addressing. A value of IPV6
represents an instance
* profile with IPv6 network type and only supports IPv6 addressing. A value of DUAL
represents an
* instance profile with dual network type that supports IPv4 and IPv6 addressing.
*
*
* @return Specifies the network type for the instance profile. A value of IPV4
represents an instance
* profile with IPv4 network type and only supports IPv4 addressing. A value of IPV6
represents
* an instance profile with IPv6 network type and only supports IPv6 addressing. A value of
* DUAL
represents an instance profile with dual network type that supports IPv4 and IPv6
* addressing.
*/
public final String networkType() {
return networkType;
}
/**
*
* A user-friendly name for the instance profile.
*
*
* @return A user-friendly name for the instance profile.
*/
public final String instanceProfileName() {
return instanceProfileName;
}
/**
*
* A user-friendly description of the instance profile.
*
*
* @return A user-friendly description of the instance profile.
*/
public final String description() {
return description;
}
/**
*
* A subnet group to associate with the instance profile.
*
*
* @return A subnet group to associate with the instance profile.
*/
public final String subnetGroupIdentifier() {
return subnetGroupIdentifier;
}
/**
* For responses, this returns true if the service returned a value for the VpcSecurityGroups property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasVpcSecurityGroups() {
return vpcSecurityGroups != null && !(vpcSecurityGroups instanceof SdkAutoConstructList);
}
/**
*
* Specifies the VPC security group names to be used with the instance profile. The VPC security group must work
* with the VPC containing the instance profile.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasVpcSecurityGroups} method.
*
*
* @return Specifies the VPC security group names to be used with the instance profile. The VPC security group must
* work with the VPC containing the instance profile.
*/
public final List vpcSecurityGroups() {
return vpcSecurityGroups;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(availabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyArn());
hashCode = 31 * hashCode + Objects.hashCode(publiclyAccessible());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(networkType());
hashCode = 31 * hashCode + Objects.hashCode(instanceProfileName());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(subnetGroupIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(hasVpcSecurityGroups() ? vpcSecurityGroups() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateInstanceProfileRequest)) {
return false;
}
CreateInstanceProfileRequest other = (CreateInstanceProfileRequest) obj;
return Objects.equals(availabilityZone(), other.availabilityZone()) && Objects.equals(kmsKeyArn(), other.kmsKeyArn())
&& Objects.equals(publiclyAccessible(), other.publiclyAccessible()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && Objects.equals(networkType(), other.networkType())
&& Objects.equals(instanceProfileName(), other.instanceProfileName())
&& Objects.equals(description(), other.description())
&& Objects.equals(subnetGroupIdentifier(), other.subnetGroupIdentifier())
&& hasVpcSecurityGroups() == other.hasVpcSecurityGroups()
&& Objects.equals(vpcSecurityGroups(), other.vpcSecurityGroups());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateInstanceProfileRequest").add("AvailabilityZone", availabilityZone())
.add("KmsKeyArn", kmsKeyArn()).add("PubliclyAccessible", publiclyAccessible())
.add("Tags", hasTags() ? tags() : null).add("NetworkType", networkType())
.add("InstanceProfileName", instanceProfileName()).add("Description", description())
.add("SubnetGroupIdentifier", subnetGroupIdentifier())
.add("VpcSecurityGroups", hasVpcSecurityGroups() ? vpcSecurityGroups() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AvailabilityZone":
return Optional.ofNullable(clazz.cast(availabilityZone()));
case "KmsKeyArn":
return Optional.ofNullable(clazz.cast(kmsKeyArn()));
case "PubliclyAccessible":
return Optional.ofNullable(clazz.cast(publiclyAccessible()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "NetworkType":
return Optional.ofNullable(clazz.cast(networkType()));
case "InstanceProfileName":
return Optional.ofNullable(clazz.cast(instanceProfileName()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "SubnetGroupIdentifier":
return Optional.ofNullable(clazz.cast(subnetGroupIdentifier()));
case "VpcSecurityGroups":
return Optional.ofNullable(clazz.cast(vpcSecurityGroups()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function