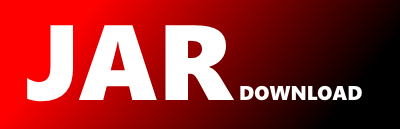
software.amazon.awssdk.services.databasemigration.model.ReplicationConfig Maven / Gradle / Ivy
Show all versions of databasemigration Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.databasemigration.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* This object provides configuration information about a serverless replication.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ReplicationConfig implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField REPLICATION_CONFIG_IDENTIFIER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ReplicationConfigIdentifier")
.getter(getter(ReplicationConfig::replicationConfigIdentifier))
.setter(setter(Builder::replicationConfigIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationConfigIdentifier")
.build()).build();
private static final SdkField REPLICATION_CONFIG_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReplicationConfigArn").getter(getter(ReplicationConfig::replicationConfigArn))
.setter(setter(Builder::replicationConfigArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationConfigArn").build())
.build();
private static final SdkField SOURCE_ENDPOINT_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceEndpointArn").getter(getter(ReplicationConfig::sourceEndpointArn))
.setter(setter(Builder::sourceEndpointArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceEndpointArn").build()).build();
private static final SdkField TARGET_ENDPOINT_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TargetEndpointArn").getter(getter(ReplicationConfig::targetEndpointArn))
.setter(setter(Builder::targetEndpointArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetEndpointArn").build()).build();
private static final SdkField REPLICATION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReplicationType").getter(getter(ReplicationConfig::replicationTypeAsString))
.setter(setter(Builder::replicationType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationType").build()).build();
private static final SdkField COMPUTE_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ComputeConfig")
.getter(getter(ReplicationConfig::computeConfig)).setter(setter(Builder::computeConfig))
.constructor(ComputeConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ComputeConfig").build()).build();
private static final SdkField REPLICATION_SETTINGS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReplicationSettings").getter(getter(ReplicationConfig::replicationSettings))
.setter(setter(Builder::replicationSettings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationSettings").build())
.build();
private static final SdkField SUPPLEMENTAL_SETTINGS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SupplementalSettings").getter(getter(ReplicationConfig::supplementalSettings))
.setter(setter(Builder::supplementalSettings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SupplementalSettings").build())
.build();
private static final SdkField TABLE_MAPPINGS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TableMappings").getter(getter(ReplicationConfig::tableMappings)).setter(setter(Builder::tableMappings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TableMappings").build()).build();
private static final SdkField REPLICATION_CONFIG_CREATE_TIME_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("ReplicationConfigCreateTime")
.getter(getter(ReplicationConfig::replicationConfigCreateTime))
.setter(setter(Builder::replicationConfigCreateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationConfigCreateTime")
.build()).build();
private static final SdkField REPLICATION_CONFIG_UPDATE_TIME_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("ReplicationConfigUpdateTime")
.getter(getter(ReplicationConfig::replicationConfigUpdateTime))
.setter(setter(Builder::replicationConfigUpdateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationConfigUpdateTime")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
REPLICATION_CONFIG_IDENTIFIER_FIELD, REPLICATION_CONFIG_ARN_FIELD, SOURCE_ENDPOINT_ARN_FIELD,
TARGET_ENDPOINT_ARN_FIELD, REPLICATION_TYPE_FIELD, COMPUTE_CONFIG_FIELD, REPLICATION_SETTINGS_FIELD,
SUPPLEMENTAL_SETTINGS_FIELD, TABLE_MAPPINGS_FIELD, REPLICATION_CONFIG_CREATE_TIME_FIELD,
REPLICATION_CONFIG_UPDATE_TIME_FIELD));
private static final long serialVersionUID = 1L;
private final String replicationConfigIdentifier;
private final String replicationConfigArn;
private final String sourceEndpointArn;
private final String targetEndpointArn;
private final String replicationType;
private final ComputeConfig computeConfig;
private final String replicationSettings;
private final String supplementalSettings;
private final String tableMappings;
private final Instant replicationConfigCreateTime;
private final Instant replicationConfigUpdateTime;
private ReplicationConfig(BuilderImpl builder) {
this.replicationConfigIdentifier = builder.replicationConfigIdentifier;
this.replicationConfigArn = builder.replicationConfigArn;
this.sourceEndpointArn = builder.sourceEndpointArn;
this.targetEndpointArn = builder.targetEndpointArn;
this.replicationType = builder.replicationType;
this.computeConfig = builder.computeConfig;
this.replicationSettings = builder.replicationSettings;
this.supplementalSettings = builder.supplementalSettings;
this.tableMappings = builder.tableMappings;
this.replicationConfigCreateTime = builder.replicationConfigCreateTime;
this.replicationConfigUpdateTime = builder.replicationConfigUpdateTime;
}
/**
*
* The identifier for the ReplicationConfig
associated with the replication.
*
*
* @return The identifier for the ReplicationConfig
associated with the replication.
*/
public final String replicationConfigIdentifier() {
return replicationConfigIdentifier;
}
/**
*
* The Amazon Resource Name (ARN) of this DMS Serverless replication configuration.
*
*
* @return The Amazon Resource Name (ARN) of this DMS Serverless replication configuration.
*/
public final String replicationConfigArn() {
return replicationConfigArn;
}
/**
*
* The Amazon Resource Name (ARN) of the source endpoint for this DMS serverless replication configuration.
*
*
* @return The Amazon Resource Name (ARN) of the source endpoint for this DMS serverless replication configuration.
*/
public final String sourceEndpointArn() {
return sourceEndpointArn;
}
/**
*
* The Amazon Resource Name (ARN) of the target endpoint for this DMS serverless replication configuration.
*
*
* @return The Amazon Resource Name (ARN) of the target endpoint for this DMS serverless replication configuration.
*/
public final String targetEndpointArn() {
return targetEndpointArn;
}
/**
*
* The type of the replication.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #replicationType}
* will return {@link MigrationTypeValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #replicationTypeAsString}.
*
*
* @return The type of the replication.
* @see MigrationTypeValue
*/
public final MigrationTypeValue replicationType() {
return MigrationTypeValue.fromValue(replicationType);
}
/**
*
* The type of the replication.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #replicationType}
* will return {@link MigrationTypeValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #replicationTypeAsString}.
*
*
* @return The type of the replication.
* @see MigrationTypeValue
*/
public final String replicationTypeAsString() {
return replicationType;
}
/**
*
* Configuration parameters for provisioning an DMS serverless replication.
*
*
* @return Configuration parameters for provisioning an DMS serverless replication.
*/
public final ComputeConfig computeConfig() {
return computeConfig;
}
/**
*
* Configuration parameters for an DMS serverless replication.
*
*
* @return Configuration parameters for an DMS serverless replication.
*/
public final String replicationSettings() {
return replicationSettings;
}
/**
*
* Additional parameters for an DMS serverless replication.
*
*
* @return Additional parameters for an DMS serverless replication.
*/
public final String supplementalSettings() {
return supplementalSettings;
}
/**
*
* Table mappings specified in the replication.
*
*
* @return Table mappings specified in the replication.
*/
public final String tableMappings() {
return tableMappings;
}
/**
*
* The time the serverless replication config was created.
*
*
* @return The time the serverless replication config was created.
*/
public final Instant replicationConfigCreateTime() {
return replicationConfigCreateTime;
}
/**
*
* The time the serverless replication config was updated.
*
*
* @return The time the serverless replication config was updated.
*/
public final Instant replicationConfigUpdateTime() {
return replicationConfigUpdateTime;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(replicationConfigIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(replicationConfigArn());
hashCode = 31 * hashCode + Objects.hashCode(sourceEndpointArn());
hashCode = 31 * hashCode + Objects.hashCode(targetEndpointArn());
hashCode = 31 * hashCode + Objects.hashCode(replicationTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(computeConfig());
hashCode = 31 * hashCode + Objects.hashCode(replicationSettings());
hashCode = 31 * hashCode + Objects.hashCode(supplementalSettings());
hashCode = 31 * hashCode + Objects.hashCode(tableMappings());
hashCode = 31 * hashCode + Objects.hashCode(replicationConfigCreateTime());
hashCode = 31 * hashCode + Objects.hashCode(replicationConfigUpdateTime());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ReplicationConfig)) {
return false;
}
ReplicationConfig other = (ReplicationConfig) obj;
return Objects.equals(replicationConfigIdentifier(), other.replicationConfigIdentifier())
&& Objects.equals(replicationConfigArn(), other.replicationConfigArn())
&& Objects.equals(sourceEndpointArn(), other.sourceEndpointArn())
&& Objects.equals(targetEndpointArn(), other.targetEndpointArn())
&& Objects.equals(replicationTypeAsString(), other.replicationTypeAsString())
&& Objects.equals(computeConfig(), other.computeConfig())
&& Objects.equals(replicationSettings(), other.replicationSettings())
&& Objects.equals(supplementalSettings(), other.supplementalSettings())
&& Objects.equals(tableMappings(), other.tableMappings())
&& Objects.equals(replicationConfigCreateTime(), other.replicationConfigCreateTime())
&& Objects.equals(replicationConfigUpdateTime(), other.replicationConfigUpdateTime());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ReplicationConfig").add("ReplicationConfigIdentifier", replicationConfigIdentifier())
.add("ReplicationConfigArn", replicationConfigArn()).add("SourceEndpointArn", sourceEndpointArn())
.add("TargetEndpointArn", targetEndpointArn()).add("ReplicationType", replicationTypeAsString())
.add("ComputeConfig", computeConfig()).add("ReplicationSettings", replicationSettings())
.add("SupplementalSettings", supplementalSettings()).add("TableMappings", tableMappings())
.add("ReplicationConfigCreateTime", replicationConfigCreateTime())
.add("ReplicationConfigUpdateTime", replicationConfigUpdateTime()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ReplicationConfigIdentifier":
return Optional.ofNullable(clazz.cast(replicationConfigIdentifier()));
case "ReplicationConfigArn":
return Optional.ofNullable(clazz.cast(replicationConfigArn()));
case "SourceEndpointArn":
return Optional.ofNullable(clazz.cast(sourceEndpointArn()));
case "TargetEndpointArn":
return Optional.ofNullable(clazz.cast(targetEndpointArn()));
case "ReplicationType":
return Optional.ofNullable(clazz.cast(replicationTypeAsString()));
case "ComputeConfig":
return Optional.ofNullable(clazz.cast(computeConfig()));
case "ReplicationSettings":
return Optional.ofNullable(clazz.cast(replicationSettings()));
case "SupplementalSettings":
return Optional.ofNullable(clazz.cast(supplementalSettings()));
case "TableMappings":
return Optional.ofNullable(clazz.cast(tableMappings()));
case "ReplicationConfigCreateTime":
return Optional.ofNullable(clazz.cast(replicationConfigCreateTime()));
case "ReplicationConfigUpdateTime":
return Optional.ofNullable(clazz.cast(replicationConfigUpdateTime()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function