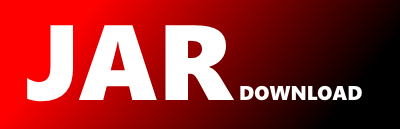
software.amazon.awssdk.services.databasemigration.model.TimestreamSettings Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.databasemigration.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides information that defines an Amazon Timestream endpoint.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class TimestreamSettings implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField DATABASE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DatabaseName").getter(getter(TimestreamSettings::databaseName)).setter(setter(Builder::databaseName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DatabaseName").build()).build();
private static final SdkField MEMORY_DURATION_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MemoryDuration").getter(getter(TimestreamSettings::memoryDuration))
.setter(setter(Builder::memoryDuration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MemoryDuration").build()).build();
private static final SdkField MAGNETIC_DURATION_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MagneticDuration").getter(getter(TimestreamSettings::magneticDuration))
.setter(setter(Builder::magneticDuration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MagneticDuration").build()).build();
private static final SdkField CDC_INSERTS_AND_UPDATES_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("CdcInsertsAndUpdates").getter(getter(TimestreamSettings::cdcInsertsAndUpdates))
.setter(setter(Builder::cdcInsertsAndUpdates))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CdcInsertsAndUpdates").build())
.build();
private static final SdkField ENABLE_MAGNETIC_STORE_WRITES_FIELD = SdkField
. builder(MarshallingType.BOOLEAN).memberName("EnableMagneticStoreWrites")
.getter(getter(TimestreamSettings::enableMagneticStoreWrites)).setter(setter(Builder::enableMagneticStoreWrites))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnableMagneticStoreWrites").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DATABASE_NAME_FIELD,
MEMORY_DURATION_FIELD, MAGNETIC_DURATION_FIELD, CDC_INSERTS_AND_UPDATES_FIELD, ENABLE_MAGNETIC_STORE_WRITES_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("DatabaseName", DATABASE_NAME_FIELD);
put("MemoryDuration", MEMORY_DURATION_FIELD);
put("MagneticDuration", MAGNETIC_DURATION_FIELD);
put("CdcInsertsAndUpdates", CDC_INSERTS_AND_UPDATES_FIELD);
put("EnableMagneticStoreWrites", ENABLE_MAGNETIC_STORE_WRITES_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String databaseName;
private final Integer memoryDuration;
private final Integer magneticDuration;
private final Boolean cdcInsertsAndUpdates;
private final Boolean enableMagneticStoreWrites;
private TimestreamSettings(BuilderImpl builder) {
this.databaseName = builder.databaseName;
this.memoryDuration = builder.memoryDuration;
this.magneticDuration = builder.magneticDuration;
this.cdcInsertsAndUpdates = builder.cdcInsertsAndUpdates;
this.enableMagneticStoreWrites = builder.enableMagneticStoreWrites;
}
/**
*
* Database name for the endpoint.
*
*
* @return Database name for the endpoint.
*/
public final String databaseName() {
return databaseName;
}
/**
*
* Set this attribute to specify the length of time to store all of the tables in memory that are migrated into
* Amazon Timestream from the source database. Time is measured in units of hours. When Timestream data comes in, it
* first resides in memory for the specified duration, which allows quick access to it.
*
*
* @return Set this attribute to specify the length of time to store all of the tables in memory that are migrated
* into Amazon Timestream from the source database. Time is measured in units of hours. When Timestream data
* comes in, it first resides in memory for the specified duration, which allows quick access to it.
*/
public final Integer memoryDuration() {
return memoryDuration;
}
/**
*
* Set this attribute to specify the default magnetic duration applied to the Amazon Timestream tables in days. This
* is the number of days that records remain in magnetic store before being discarded. For more information, see Storage in the Amazon Timestream Developer Guide.
*
*
* @return Set this attribute to specify the default magnetic duration applied to the Amazon Timestream tables in
* days. This is the number of days that records remain in magnetic store before being discarded. For more
* information, see Storage in the Amazon Timestream Developer
* Guide.
*/
public final Integer magneticDuration() {
return magneticDuration;
}
/**
*
* Set this attribute to true
to specify that DMS only applies inserts and updates, and not deletes.
* Amazon Timestream does not allow deleting records, so if this value is false
, DMS nulls out the
* corresponding record in the Timestream database rather than deleting it.
*
*
* @return Set this attribute to true
to specify that DMS only applies inserts and updates, and not
* deletes. Amazon Timestream does not allow deleting records, so if this value is false
, DMS
* nulls out the corresponding record in the Timestream database rather than deleting it.
*/
public final Boolean cdcInsertsAndUpdates() {
return cdcInsertsAndUpdates;
}
/**
*
* Set this attribute to true
to enable memory store writes. When this value is false
, DMS
* does not write records that are older in days than the value specified in MagneticDuration
, because
* Amazon Timestream does not allow memory writes by default. For more information, see Storage in the Amazon Timestream Developer Guide.
*
*
* @return Set this attribute to true
to enable memory store writes. When this value is
* false
, DMS does not write records that are older in days than the value specified in
* MagneticDuration
, because Amazon Timestream does not allow memory writes by default. For
* more information, see Storage in the Amazon Timestream Developer
* Guide.
*/
public final Boolean enableMagneticStoreWrites() {
return enableMagneticStoreWrites;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(databaseName());
hashCode = 31 * hashCode + Objects.hashCode(memoryDuration());
hashCode = 31 * hashCode + Objects.hashCode(magneticDuration());
hashCode = 31 * hashCode + Objects.hashCode(cdcInsertsAndUpdates());
hashCode = 31 * hashCode + Objects.hashCode(enableMagneticStoreWrites());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof TimestreamSettings)) {
return false;
}
TimestreamSettings other = (TimestreamSettings) obj;
return Objects.equals(databaseName(), other.databaseName()) && Objects.equals(memoryDuration(), other.memoryDuration())
&& Objects.equals(magneticDuration(), other.magneticDuration())
&& Objects.equals(cdcInsertsAndUpdates(), other.cdcInsertsAndUpdates())
&& Objects.equals(enableMagneticStoreWrites(), other.enableMagneticStoreWrites());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("TimestreamSettings").add("DatabaseName", databaseName()).add("MemoryDuration", memoryDuration())
.add("MagneticDuration", magneticDuration()).add("CdcInsertsAndUpdates", cdcInsertsAndUpdates())
.add("EnableMagneticStoreWrites", enableMagneticStoreWrites()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DatabaseName":
return Optional.ofNullable(clazz.cast(databaseName()));
case "MemoryDuration":
return Optional.ofNullable(clazz.cast(memoryDuration()));
case "MagneticDuration":
return Optional.ofNullable(clazz.cast(magneticDuration()));
case "CdcInsertsAndUpdates":
return Optional.ofNullable(clazz.cast(cdcInsertsAndUpdates()));
case "EnableMagneticStoreWrites":
return Optional.ofNullable(clazz.cast(enableMagneticStoreWrites()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function